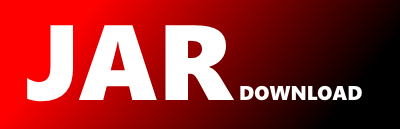
software.amazon.awssdk.services.migrationhub.DefaultMigrationHubClient Maven / Gradle / Ivy
Show all versions of migrationhub Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.migrationhub;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.migrationhub.internal.MigrationHubServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.migrationhub.model.AccessDeniedException;
import software.amazon.awssdk.services.migrationhub.model.AssociateCreatedArtifactRequest;
import software.amazon.awssdk.services.migrationhub.model.AssociateCreatedArtifactResponse;
import software.amazon.awssdk.services.migrationhub.model.AssociateDiscoveredResourceRequest;
import software.amazon.awssdk.services.migrationhub.model.AssociateDiscoveredResourceResponse;
import software.amazon.awssdk.services.migrationhub.model.AssociateSourceResourceRequest;
import software.amazon.awssdk.services.migrationhub.model.AssociateSourceResourceResponse;
import software.amazon.awssdk.services.migrationhub.model.CreateProgressUpdateStreamRequest;
import software.amazon.awssdk.services.migrationhub.model.CreateProgressUpdateStreamResponse;
import software.amazon.awssdk.services.migrationhub.model.DeleteProgressUpdateStreamRequest;
import software.amazon.awssdk.services.migrationhub.model.DeleteProgressUpdateStreamResponse;
import software.amazon.awssdk.services.migrationhub.model.DescribeApplicationStateRequest;
import software.amazon.awssdk.services.migrationhub.model.DescribeApplicationStateResponse;
import software.amazon.awssdk.services.migrationhub.model.DescribeMigrationTaskRequest;
import software.amazon.awssdk.services.migrationhub.model.DescribeMigrationTaskResponse;
import software.amazon.awssdk.services.migrationhub.model.DisassociateCreatedArtifactRequest;
import software.amazon.awssdk.services.migrationhub.model.DisassociateCreatedArtifactResponse;
import software.amazon.awssdk.services.migrationhub.model.DisassociateDiscoveredResourceRequest;
import software.amazon.awssdk.services.migrationhub.model.DisassociateDiscoveredResourceResponse;
import software.amazon.awssdk.services.migrationhub.model.DisassociateSourceResourceRequest;
import software.amazon.awssdk.services.migrationhub.model.DisassociateSourceResourceResponse;
import software.amazon.awssdk.services.migrationhub.model.DryRunOperationException;
import software.amazon.awssdk.services.migrationhub.model.HomeRegionNotSetException;
import software.amazon.awssdk.services.migrationhub.model.ImportMigrationTaskRequest;
import software.amazon.awssdk.services.migrationhub.model.ImportMigrationTaskResponse;
import software.amazon.awssdk.services.migrationhub.model.InternalServerErrorException;
import software.amazon.awssdk.services.migrationhub.model.InvalidInputException;
import software.amazon.awssdk.services.migrationhub.model.ListApplicationStatesRequest;
import software.amazon.awssdk.services.migrationhub.model.ListApplicationStatesResponse;
import software.amazon.awssdk.services.migrationhub.model.ListCreatedArtifactsRequest;
import software.amazon.awssdk.services.migrationhub.model.ListCreatedArtifactsResponse;
import software.amazon.awssdk.services.migrationhub.model.ListDiscoveredResourcesRequest;
import software.amazon.awssdk.services.migrationhub.model.ListDiscoveredResourcesResponse;
import software.amazon.awssdk.services.migrationhub.model.ListMigrationTaskUpdatesRequest;
import software.amazon.awssdk.services.migrationhub.model.ListMigrationTaskUpdatesResponse;
import software.amazon.awssdk.services.migrationhub.model.ListMigrationTasksRequest;
import software.amazon.awssdk.services.migrationhub.model.ListMigrationTasksResponse;
import software.amazon.awssdk.services.migrationhub.model.ListProgressUpdateStreamsRequest;
import software.amazon.awssdk.services.migrationhub.model.ListProgressUpdateStreamsResponse;
import software.amazon.awssdk.services.migrationhub.model.ListSourceResourcesRequest;
import software.amazon.awssdk.services.migrationhub.model.ListSourceResourcesResponse;
import software.amazon.awssdk.services.migrationhub.model.MigrationHubException;
import software.amazon.awssdk.services.migrationhub.model.NotifyApplicationStateRequest;
import software.amazon.awssdk.services.migrationhub.model.NotifyApplicationStateResponse;
import software.amazon.awssdk.services.migrationhub.model.NotifyMigrationTaskStateRequest;
import software.amazon.awssdk.services.migrationhub.model.NotifyMigrationTaskStateResponse;
import software.amazon.awssdk.services.migrationhub.model.PolicyErrorException;
import software.amazon.awssdk.services.migrationhub.model.PutResourceAttributesRequest;
import software.amazon.awssdk.services.migrationhub.model.PutResourceAttributesResponse;
import software.amazon.awssdk.services.migrationhub.model.ResourceNotFoundException;
import software.amazon.awssdk.services.migrationhub.model.ServiceUnavailableException;
import software.amazon.awssdk.services.migrationhub.model.ThrottlingException;
import software.amazon.awssdk.services.migrationhub.model.UnauthorizedOperationException;
import software.amazon.awssdk.services.migrationhub.transform.AssociateCreatedArtifactRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.AssociateDiscoveredResourceRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.AssociateSourceResourceRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.CreateProgressUpdateStreamRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DeleteProgressUpdateStreamRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DescribeApplicationStateRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DescribeMigrationTaskRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DisassociateCreatedArtifactRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DisassociateDiscoveredResourceRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.DisassociateSourceResourceRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ImportMigrationTaskRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListApplicationStatesRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListCreatedArtifactsRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListDiscoveredResourcesRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListMigrationTaskUpdatesRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListMigrationTasksRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListProgressUpdateStreamsRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.ListSourceResourcesRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.NotifyApplicationStateRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.NotifyMigrationTaskStateRequestMarshaller;
import software.amazon.awssdk.services.migrationhub.transform.PutResourceAttributesRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link MigrationHubClient}.
*
* @see MigrationHubClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMigrationHubClient implements MigrationHubClient {
private static final Logger log = Logger.loggerFor(DefaultMigrationHubClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMigrationHubClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Associates a created artifact of an AWS cloud resource, the target receiving the migration, with the migration
* task performed by a migration tool. This API has the following traits:
*
*
* -
*
* Migration tools can call the AssociateCreatedArtifact
operation to indicate which AWS artifact is
* associated with a migration task.
*
*
* -
*
* The created artifact name must be provided in ARN (Amazon Resource Name) format which will contain information
* about type and region; for example: arn:aws:ec2:us-east-1:488216288981:image/ami-6d0ba87b
.
*
*
* -
*
* Examples of the AWS resource behind the created artifact are, AMI's, EC2 instance, or DMS endpoint, etc.
*
*
*
*
* @param associateCreatedArtifactRequest
* @return Result of the AssociateCreatedArtifact operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.AssociateCreatedArtifact
* @see AWS API Documentation
*/
@Override
public AssociateCreatedArtifactResponse associateCreatedArtifact(
AssociateCreatedArtifactRequest associateCreatedArtifactRequest) throws AccessDeniedException, ThrottlingException,
InternalServerErrorException, ServiceUnavailableException, DryRunOperationException, UnauthorizedOperationException,
InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException,
MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateCreatedArtifactResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateCreatedArtifactRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateCreatedArtifactRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateCreatedArtifact");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateCreatedArtifact").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateCreatedArtifactRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateCreatedArtifactRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates a discovered resource ID from Application Discovery Service with a migration task.
*
*
* @param associateDiscoveredResourceRequest
* @return Result of the AssociateDiscoveredResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws PolicyErrorException
* Exception raised when there are problems accessing Application Discovery Service (Application Discovery
* Service); most likely due to a misconfigured policy or the migrationhub-discovery
role is
* missing or not configured correctly.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.AssociateDiscoveredResource
* @see AWS API Documentation
*/
@Override
public AssociateDiscoveredResourceResponse associateDiscoveredResource(
AssociateDiscoveredResourceRequest associateDiscoveredResourceRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, PolicyErrorException, ResourceNotFoundException,
HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateDiscoveredResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateDiscoveredResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateDiscoveredResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateDiscoveredResource");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateDiscoveredResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateDiscoveredResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateDiscoveredResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates a source resource with a migration task. For example, the source resource can be a source server, an
* application, or a migration wave.
*
*
* @param associateSourceResourceRequest
* @return Result of the AssociateSourceResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.AssociateSourceResource
* @see AWS API Documentation
*/
@Override
public AssociateSourceResourceResponse associateSourceResource(AssociateSourceResourceRequest associateSourceResourceRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
DryRunOperationException, UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateSourceResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateSourceResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateSourceResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateSourceResource");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateSourceResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(associateSourceResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateSourceResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a progress update stream which is an AWS resource used for access control as well as a namespace for
* migration task names that is implicitly linked to your AWS account. It must uniquely identify the migration tool
* as it is used for all updates made by the tool; however, it does not need to be unique for each AWS account
* because it is scoped to the AWS account.
*
*
* @param createProgressUpdateStreamRequest
* @return Result of the CreateProgressUpdateStream operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.CreateProgressUpdateStream
* @see AWS API Documentation
*/
@Override
public CreateProgressUpdateStreamResponse createProgressUpdateStream(
CreateProgressUpdateStreamRequest createProgressUpdateStreamRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, HomeRegionNotSetException, AwsServiceException,
SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateProgressUpdateStreamResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createProgressUpdateStreamRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createProgressUpdateStreamRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateProgressUpdateStream");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateProgressUpdateStream").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(createProgressUpdateStreamRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateProgressUpdateStreamRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a progress update stream, including all of its tasks, which was previously created as an AWS resource
* used for access control. This API has the following traits:
*
*
* -
*
* The only parameter needed for DeleteProgressUpdateStream
is the stream name (same as a
* CreateProgressUpdateStream
call).
*
*
* -
*
* The call will return, and a background process will asynchronously delete the stream and all of its resources
* (tasks, associated resources, resource attributes, created artifacts).
*
*
* -
*
* If the stream takes time to be deleted, it might still show up on a ListProgressUpdateStreams
call.
*
*
* -
*
* CreateProgressUpdateStream
, ImportMigrationTask
, NotifyMigrationTaskState
,
* and all Associate[*] APIs related to the tasks belonging to the stream will throw "InvalidInputException" if the
* stream of the same name is in the process of being deleted.
*
*
* -
*
* Once the stream and all of its resources are deleted, CreateProgressUpdateStream
for a stream of the
* same name will succeed, and that stream will be an entirely new logical resource (without any resources
* associated with the old stream).
*
*
*
*
* @param deleteProgressUpdateStreamRequest
* @return Result of the DeleteProgressUpdateStream operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DeleteProgressUpdateStream
* @see AWS API Documentation
*/
@Override
public DeleteProgressUpdateStreamResponse deleteProgressUpdateStream(
DeleteProgressUpdateStreamRequest deleteProgressUpdateStreamRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteProgressUpdateStreamResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteProgressUpdateStreamRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteProgressUpdateStreamRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteProgressUpdateStream");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteProgressUpdateStream").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(deleteProgressUpdateStreamRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteProgressUpdateStreamRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets the migration status of an application.
*
*
* @param describeApplicationStateRequest
* @return Result of the DescribeApplicationState operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws PolicyErrorException
* Exception raised when there are problems accessing Application Discovery Service (Application Discovery
* Service); most likely due to a misconfigured policy or the migrationhub-discovery
role is
* missing or not configured correctly.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DescribeApplicationState
* @see AWS API Documentation
*/
@Override
public DescribeApplicationStateResponse describeApplicationState(
DescribeApplicationStateRequest describeApplicationStateRequest) throws AccessDeniedException, ThrottlingException,
InternalServerErrorException, ServiceUnavailableException, InvalidInputException, PolicyErrorException,
ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeApplicationStateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeApplicationStateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeApplicationStateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApplicationState");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeApplicationState").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeApplicationStateRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeApplicationStateRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of all attributes associated with a specific migration task.
*
*
* @param describeMigrationTaskRequest
* @return Result of the DescribeMigrationTask operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DescribeMigrationTask
* @see AWS API Documentation
*/
@Override
public DescribeMigrationTaskResponse describeMigrationTask(DescribeMigrationTaskRequest describeMigrationTaskRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException,
MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeMigrationTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeMigrationTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeMigrationTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeMigrationTask");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeMigrationTask").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(describeMigrationTaskRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeMigrationTaskRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates a created artifact of an AWS resource with a migration task performed by a migration tool that was
* previously associated. This API has the following traits:
*
*
* -
*
* A migration user can call the DisassociateCreatedArtifacts
operation to disassociate a created AWS
* Artifact from a migration task.
*
*
* -
*
* The created artifact name must be provided in ARN (Amazon Resource Name) format which will contain information
* about type and region; for example: arn:aws:ec2:us-east-1:488216288981:image/ami-6d0ba87b
.
*
*
* -
*
* Examples of the AWS resource behind the created artifact are, AMI's, EC2 instance, or RDS instance, etc.
*
*
*
*
* @param disassociateCreatedArtifactRequest
* @return Result of the DisassociateCreatedArtifact operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DisassociateCreatedArtifact
* @see AWS API Documentation
*/
@Override
public DisassociateCreatedArtifactResponse disassociateCreatedArtifact(
DisassociateCreatedArtifactRequest disassociateCreatedArtifactRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateCreatedArtifactResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateCreatedArtifactRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateCreatedArtifactRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateCreatedArtifact");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateCreatedArtifact").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateCreatedArtifactRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateCreatedArtifactRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociate an Application Discovery Service discovered resource from a migration task.
*
*
* @param disassociateDiscoveredResourceRequest
* @return Result of the DisassociateDiscoveredResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DisassociateDiscoveredResource
* @see AWS API Documentation
*/
@Override
public DisassociateDiscoveredResourceResponse disassociateDiscoveredResource(
DisassociateDiscoveredResourceRequest disassociateDiscoveredResourceRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateDiscoveredResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateDiscoveredResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateDiscoveredResourceRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateDiscoveredResource");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateDiscoveredResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateDiscoveredResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateDiscoveredResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the association between a source resource and a migration task.
*
*
* @param disassociateSourceResourceRequest
* @return Result of the DisassociateSourceResource operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.DisassociateSourceResource
* @see AWS API Documentation
*/
@Override
public DisassociateSourceResourceResponse disassociateSourceResource(
DisassociateSourceResourceRequest disassociateSourceResourceRequest) throws AccessDeniedException,
ThrottlingException, InternalServerErrorException, ServiceUnavailableException, DryRunOperationException,
UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException, AwsServiceException,
SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateSourceResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateSourceResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateSourceResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateSourceResource");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateSourceResource").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(disassociateSourceResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateSourceResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers a new migration task which represents a server, database, etc., being migrated to AWS by a migration
* tool.
*
*
* This API is a prerequisite to calling the NotifyMigrationTaskState
API as the migration tool must
* first register the migration task with Migration Hub.
*
*
* @param importMigrationTaskRequest
* @return Result of the ImportMigrationTask operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ImportMigrationTask
* @see AWS API Documentation
*/
@Override
public ImportMigrationTaskResponse importMigrationTask(ImportMigrationTaskRequest importMigrationTaskRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
DryRunOperationException, UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException,
HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportMigrationTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(importMigrationTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importMigrationTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportMigrationTask");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ImportMigrationTask").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(importMigrationTaskRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ImportMigrationTaskRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the migration statuses for your applications. If you use the optional ApplicationIds
* parameter, only the migration statuses for those applications will be returned.
*
*
* @param listApplicationStatesRequest
* @return Result of the ListApplicationStates operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListApplicationStates
* @see AWS API Documentation
*/
@Override
public ListApplicationStatesResponse listApplicationStates(ListApplicationStatesRequest listApplicationStatesRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListApplicationStatesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listApplicationStatesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listApplicationStatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListApplicationStates");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListApplicationStates").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listApplicationStatesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListApplicationStatesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the created artifacts attached to a given migration task in an update stream. This API has the following
* traits:
*
*
* -
*
* Gets the list of the created artifacts while migration is taking place.
*
*
* -
*
* Shows the artifacts created by the migration tool that was associated by the
* AssociateCreatedArtifact
API.
*
*
* -
*
* Lists created artifacts in a paginated interface.
*
*
*
*
* @param listCreatedArtifactsRequest
* @return Result of the ListCreatedArtifacts operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListCreatedArtifacts
* @see AWS API Documentation
*/
@Override
public ListCreatedArtifactsResponse listCreatedArtifacts(ListCreatedArtifactsRequest listCreatedArtifactsRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException,
MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCreatedArtifactsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCreatedArtifactsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCreatedArtifactsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCreatedArtifacts");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListCreatedArtifacts").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listCreatedArtifactsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListCreatedArtifactsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists discovered resources associated with the given MigrationTask
.
*
*
* @param listDiscoveredResourcesRequest
* @return Result of the ListDiscoveredResources operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListDiscoveredResources
* @see AWS API Documentation
*/
@Override
public ListDiscoveredResourcesResponse listDiscoveredResources(ListDiscoveredResourcesRequest listDiscoveredResourcesRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException,
MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDiscoveredResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listDiscoveredResourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDiscoveredResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDiscoveredResources");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDiscoveredResources").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listDiscoveredResourcesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDiscoveredResourcesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* This is a paginated API that returns all the migration-task states for the specified
* MigrationTaskName
and ProgressUpdateStream
.
*
*
* @param listMigrationTaskUpdatesRequest
* @return Result of the ListMigrationTaskUpdates operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListMigrationTaskUpdates
* @see AWS API Documentation
*/
@Override
public ListMigrationTaskUpdatesResponse listMigrationTaskUpdates(
ListMigrationTaskUpdatesRequest listMigrationTaskUpdatesRequest) throws AccessDeniedException, ThrottlingException,
InternalServerErrorException, ServiceUnavailableException, InvalidInputException, ResourceNotFoundException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMigrationTaskUpdatesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMigrationTaskUpdatesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMigrationTaskUpdatesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMigrationTaskUpdates");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListMigrationTaskUpdates").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listMigrationTaskUpdatesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMigrationTaskUpdatesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all, or filtered by resource name, migration tasks associated with the user account making this call. This
* API has the following traits:
*
*
* -
*
* Can show a summary list of the most recent migration tasks.
*
*
* -
*
* Can show a summary list of migration tasks associated with a given discovered resource.
*
*
* -
*
* Lists migration tasks in a paginated interface.
*
*
*
*
* @param listMigrationTasksRequest
* @return Result of the ListMigrationTasks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws PolicyErrorException
* Exception raised when there are problems accessing Application Discovery Service (Application Discovery
* Service); most likely due to a misconfigured policy or the migrationhub-discovery
role is
* missing or not configured correctly.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListMigrationTasks
* @see AWS API Documentation
*/
@Override
public ListMigrationTasksResponse listMigrationTasks(ListMigrationTasksRequest listMigrationTasksRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, PolicyErrorException, ResourceNotFoundException, HomeRegionNotSetException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListMigrationTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listMigrationTasksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listMigrationTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListMigrationTasks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListMigrationTasks").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listMigrationTasksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListMigrationTasksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists progress update streams associated with the user account making this call.
*
*
* @param listProgressUpdateStreamsRequest
* @return Result of the ListProgressUpdateStreams operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListProgressUpdateStreams
* @see AWS API Documentation
*/
@Override
public ListProgressUpdateStreamsResponse listProgressUpdateStreams(
ListProgressUpdateStreamsRequest listProgressUpdateStreamsRequest) throws AccessDeniedException, ThrottlingException,
InternalServerErrorException, ServiceUnavailableException, InvalidInputException, HomeRegionNotSetException,
AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListProgressUpdateStreamsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listProgressUpdateStreamsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listProgressUpdateStreamsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListProgressUpdateStreams");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListProgressUpdateStreams").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listProgressUpdateStreamsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListProgressUpdateStreamsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the source resource that are associated with the specified MigrationTaskName
and
* ProgressUpdateStream
.
*
*
* @param listSourceResourcesRequest
* @return Result of the ListSourceResources operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.ListSourceResources
* @see AWS API Documentation
*/
@Override
public ListSourceResourcesResponse listSourceResources(ListSourceResourcesRequest listSourceResourcesRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
InvalidInputException, ResourceNotFoundException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSourceResourcesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSourceResourcesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSourceResourcesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSourceResources");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSourceResources").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(listSourceResourcesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSourceResourcesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Sets the migration state of an application. For a given application identified by the value passed to
* ApplicationId
, its status is set or updated by passing one of three values to Status
:
* NOT_STARTED | IN_PROGRESS | COMPLETED
.
*
*
* @param notifyApplicationStateRequest
* @return Result of the NotifyApplicationState operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws PolicyErrorException
* Exception raised when there are problems accessing Application Discovery Service (Application Discovery
* Service); most likely due to a misconfigured policy or the migrationhub-discovery
role is
* missing or not configured correctly.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.NotifyApplicationState
* @see AWS API Documentation
*/
@Override
public NotifyApplicationStateResponse notifyApplicationState(NotifyApplicationStateRequest notifyApplicationStateRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
DryRunOperationException, UnauthorizedOperationException, InvalidInputException, PolicyErrorException,
ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, NotifyApplicationStateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(notifyApplicationStateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, notifyApplicationStateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "NotifyApplicationState");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("NotifyApplicationState").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(notifyApplicationStateRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new NotifyApplicationStateRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Notifies Migration Hub of the current status, progress, or other detail regarding a migration task. This API has
* the following traits:
*
*
* -
*
* Migration tools will call the NotifyMigrationTaskState
API to share the latest progress and status.
*
*
* -
*
* MigrationTaskName
is used for addressing updates to the correct target.
*
*
* -
*
* ProgressUpdateStream
is used for access control and to provide a namespace for each migration tool.
*
*
*
*
* @param notifyMigrationTaskStateRequest
* @return Result of the NotifyMigrationTaskState operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.NotifyMigrationTaskState
* @see AWS API Documentation
*/
@Override
public NotifyMigrationTaskStateResponse notifyMigrationTaskState(
NotifyMigrationTaskStateRequest notifyMigrationTaskStateRequest) throws AccessDeniedException, ThrottlingException,
InternalServerErrorException, ServiceUnavailableException, DryRunOperationException, UnauthorizedOperationException,
InvalidInputException, ResourceNotFoundException, HomeRegionNotSetException, AwsServiceException, SdkClientException,
MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, NotifyMigrationTaskStateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(notifyMigrationTaskStateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, notifyMigrationTaskStateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "NotifyMigrationTaskState");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("NotifyMigrationTaskState").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(notifyMigrationTaskStateRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new NotifyMigrationTaskStateRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Provides identifying details of the resource being migrated so that it can be associated in the Application
* Discovery Service repository. This association occurs asynchronously after PutResourceAttributes
* returns.
*
*
*
* -
*
* Keep in mind that subsequent calls to PutResourceAttributes will override previously stored attributes. For
* example, if it is first called with a MAC address, but later, it is desired to add an IP address, it will
* then be required to call it with both the IP and MAC addresses to prevent overriding the MAC address.
*
*
* -
*
* Note the instructions regarding the special use case of the ResourceAttributeList
parameter when specifying any "VM" related value.
*
*
*
*
*
* Because this is an asynchronous call, it will always return 200, whether an association occurs or not. To confirm
* if an association was found based on the provided details, call ListDiscoveredResources
.
*
*
*
* @param putResourceAttributesRequest
* @return Result of the PutResourceAttributes operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ThrottlingException
* The request was denied due to request throttling.
* @throws InternalServerErrorException
* Exception raised when an internal, configuration, or dependency error is encountered.
* @throws ServiceUnavailableException
* Exception raised when there is an internal, configuration, or dependency error encountered.
* @throws DryRunOperationException
* Exception raised to indicate a successfully authorized action when the DryRun
flag is set to
* "true".
* @throws UnauthorizedOperationException
* Exception raised to indicate a request was not authorized when the DryRun
flag is set to
* "true".
* @throws InvalidInputException
* Exception raised when the provided input violates a policy constraint or is entered in the wrong format
* or data type.
* @throws ResourceNotFoundException
* Exception raised when the request references a resource (Application Discovery Service configuration,
* update stream, migration task, etc.) that does not exist in Application Discovery Service (Application
* Discovery Service) or in Migration Hub's repository.
* @throws HomeRegionNotSetException
* The home region is not set. Set the home region to continue.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws MigrationHubException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample MigrationHubClient.PutResourceAttributes
* @see AWS API Documentation
*/
@Override
public PutResourceAttributesResponse putResourceAttributes(PutResourceAttributesRequest putResourceAttributesRequest)
throws AccessDeniedException, ThrottlingException, InternalServerErrorException, ServiceUnavailableException,
DryRunOperationException, UnauthorizedOperationException, InvalidInputException, ResourceNotFoundException,
HomeRegionNotSetException, AwsServiceException, SdkClientException, MigrationHubException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, PutResourceAttributesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(putResourceAttributesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putResourceAttributesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Migration Hub");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutResourceAttributes");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutResourceAttributes").withProtocolMetadata(protocolMetadata)
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withInput(putResourceAttributesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutResourceAttributesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
MigrationHubServiceClientConfigurationBuilder serviceConfigBuilder = new MigrationHubServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(MigrationHubException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("UnauthorizedOperation")
.exceptionBuilderSupplier(UnauthorizedOperationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidInputException")
.exceptionBuilderSupplier(InvalidInputException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PolicyErrorException")
.exceptionBuilderSupplier(PolicyErrorException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceUnavailableException")
.exceptionBuilderSupplier(ServiceUnavailableException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("HomeRegionNotSetException")
.exceptionBuilderSupplier(HomeRegionNotSetException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerError")
.exceptionBuilderSupplier(InternalServerErrorException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DryRunOperation")
.exceptionBuilderSupplier(DryRunOperationException::builder).httpStatusCode(400).build());
}
@Override
public final MigrationHubServiceClientConfiguration serviceClientConfiguration() {
return new MigrationHubServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public void close() {
clientHandler.close();
}
}