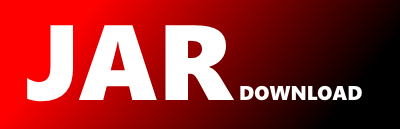
software.amazon.awssdk.services.migrationhubrefactorspaces.model.ApiGatewayProxySummary Maven / Gradle / Ivy
Show all versions of migrationhubrefactorspaces Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.migrationhubrefactorspaces.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A wrapper object holding the Amazon API Gateway proxy summary.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ApiGatewayProxySummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField API_GATEWAY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApiGatewayId").getter(getter(ApiGatewayProxySummary::apiGatewayId))
.setter(setter(Builder::apiGatewayId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApiGatewayId").build()).build();
private static final SdkField ENDPOINT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EndpointType").getter(getter(ApiGatewayProxySummary::endpointTypeAsString))
.setter(setter(Builder::endpointType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndpointType").build()).build();
private static final SdkField NLB_ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("NlbArn")
.getter(getter(ApiGatewayProxySummary::nlbArn)).setter(setter(Builder::nlbArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NlbArn").build()).build();
private static final SdkField NLB_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NlbName").getter(getter(ApiGatewayProxySummary::nlbName)).setter(setter(Builder::nlbName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NlbName").build()).build();
private static final SdkField PROXY_URL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ProxyUrl").getter(getter(ApiGatewayProxySummary::proxyUrl)).setter(setter(Builder::proxyUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProxyUrl").build()).build();
private static final SdkField STAGE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StageName").getter(getter(ApiGatewayProxySummary::stageName)).setter(setter(Builder::stageName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StageName").build()).build();
private static final SdkField VPC_LINK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VpcLinkId").getter(getter(ApiGatewayProxySummary::vpcLinkId)).setter(setter(Builder::vpcLinkId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcLinkId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(API_GATEWAY_ID_FIELD,
ENDPOINT_TYPE_FIELD, NLB_ARN_FIELD, NLB_NAME_FIELD, PROXY_URL_FIELD, STAGE_NAME_FIELD, VPC_LINK_ID_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ApiGatewayId", API_GATEWAY_ID_FIELD);
put("EndpointType", ENDPOINT_TYPE_FIELD);
put("NlbArn", NLB_ARN_FIELD);
put("NlbName", NLB_NAME_FIELD);
put("ProxyUrl", PROXY_URL_FIELD);
put("StageName", STAGE_NAME_FIELD);
put("VpcLinkId", VPC_LINK_ID_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String apiGatewayId;
private final String endpointType;
private final String nlbArn;
private final String nlbName;
private final String proxyUrl;
private final String stageName;
private final String vpcLinkId;
private ApiGatewayProxySummary(BuilderImpl builder) {
this.apiGatewayId = builder.apiGatewayId;
this.endpointType = builder.endpointType;
this.nlbArn = builder.nlbArn;
this.nlbName = builder.nlbName;
this.proxyUrl = builder.proxyUrl;
this.stageName = builder.stageName;
this.vpcLinkId = builder.vpcLinkId;
}
/**
*
* The resource ID of the API Gateway for the proxy.
*
*
* @return The resource ID of the API Gateway for the proxy.
*/
public final String apiGatewayId() {
return apiGatewayId;
}
/**
*
* The type of API Gateway endpoint created.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointType} will
* return {@link ApiGatewayEndpointType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #endpointTypeAsString}.
*
*
* @return The type of API Gateway endpoint created.
* @see ApiGatewayEndpointType
*/
public final ApiGatewayEndpointType endpointType() {
return ApiGatewayEndpointType.fromValue(endpointType);
}
/**
*
* The type of API Gateway endpoint created.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #endpointType} will
* return {@link ApiGatewayEndpointType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #endpointTypeAsString}.
*
*
* @return The type of API Gateway endpoint created.
* @see ApiGatewayEndpointType
*/
public final String endpointTypeAsString() {
return endpointType;
}
/**
*
* The Amazon Resource Name (ARN) of the Network Load Balancer configured by the API Gateway proxy.
*
*
* @return The Amazon Resource Name (ARN) of the Network Load Balancer configured by the API Gateway proxy.
*/
public final String nlbArn() {
return nlbArn;
}
/**
*
* The name of the Network Load Balancer that is configured by the API Gateway proxy.
*
*
* @return The name of the Network Load Balancer that is configured by the API Gateway proxy.
*/
public final String nlbName() {
return nlbName;
}
/**
*
* The endpoint URL of the API Gateway proxy.
*
*
* @return The endpoint URL of the API Gateway proxy.
*/
public final String proxyUrl() {
return proxyUrl;
}
/**
*
* The name of the API Gateway stage. The name defaults to prod
.
*
*
* @return The name of the API Gateway stage. The name defaults to prod
.
*/
public final String stageName() {
return stageName;
}
/**
*
* The VpcLink
ID of the API Gateway proxy.
*
*
* @return The VpcLink
ID of the API Gateway proxy.
*/
public final String vpcLinkId() {
return vpcLinkId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(apiGatewayId());
hashCode = 31 * hashCode + Objects.hashCode(endpointTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(nlbArn());
hashCode = 31 * hashCode + Objects.hashCode(nlbName());
hashCode = 31 * hashCode + Objects.hashCode(proxyUrl());
hashCode = 31 * hashCode + Objects.hashCode(stageName());
hashCode = 31 * hashCode + Objects.hashCode(vpcLinkId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ApiGatewayProxySummary)) {
return false;
}
ApiGatewayProxySummary other = (ApiGatewayProxySummary) obj;
return Objects.equals(apiGatewayId(), other.apiGatewayId())
&& Objects.equals(endpointTypeAsString(), other.endpointTypeAsString())
&& Objects.equals(nlbArn(), other.nlbArn()) && Objects.equals(nlbName(), other.nlbName())
&& Objects.equals(proxyUrl(), other.proxyUrl()) && Objects.equals(stageName(), other.stageName())
&& Objects.equals(vpcLinkId(), other.vpcLinkId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ApiGatewayProxySummary").add("ApiGatewayId", apiGatewayId())
.add("EndpointType", endpointTypeAsString()).add("NlbArn", nlbArn()).add("NlbName", nlbName())
.add("ProxyUrl", proxyUrl()).add("StageName", stageName()).add("VpcLinkId", vpcLinkId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApiGatewayId":
return Optional.ofNullable(clazz.cast(apiGatewayId()));
case "EndpointType":
return Optional.ofNullable(clazz.cast(endpointTypeAsString()));
case "NlbArn":
return Optional.ofNullable(clazz.cast(nlbArn()));
case "NlbName":
return Optional.ofNullable(clazz.cast(nlbName()));
case "ProxyUrl":
return Optional.ofNullable(clazz.cast(proxyUrl()));
case "StageName":
return Optional.ofNullable(clazz.cast(stageName()));
case "VpcLinkId":
return Optional.ofNullable(clazz.cast(vpcLinkId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function