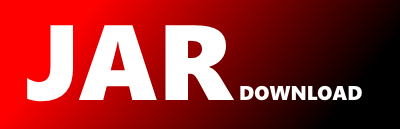
software.amazon.awssdk.services.migrationhubstrategy.model.SourceCodeRepository Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.migrationhubstrategy.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Object containing source code information that is linked to an application component.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SourceCodeRepository implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BRANCH_FIELD = SdkField. builder(MarshallingType.STRING).memberName("branch")
.getter(getter(SourceCodeRepository::branch)).setter(setter(Builder::branch))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("branch").build()).build();
private static final SdkField PROJECT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("projectName").getter(getter(SourceCodeRepository::projectName)).setter(setter(Builder::projectName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("projectName").build()).build();
private static final SdkField REPOSITORY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("repository").getter(getter(SourceCodeRepository::repository)).setter(setter(Builder::repository))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("repository").build()).build();
private static final SdkField VERSION_CONTROL_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("versionControlType").getter(getter(SourceCodeRepository::versionControlType))
.setter(setter(Builder::versionControlType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("versionControlType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BRANCH_FIELD,
PROJECT_NAME_FIELD, REPOSITORY_FIELD, VERSION_CONTROL_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String branch;
private final String projectName;
private final String repository;
private final String versionControlType;
private SourceCodeRepository(BuilderImpl builder) {
this.branch = builder.branch;
this.projectName = builder.projectName;
this.repository = builder.repository;
this.versionControlType = builder.versionControlType;
}
/**
*
* The branch of the source code.
*
*
* @return The branch of the source code.
*/
public final String branch() {
return branch;
}
/**
*
* The name of the project.
*
*
* @return The name of the project.
*/
public final String projectName() {
return projectName;
}
/**
*
* The repository name for the source code.
*
*
* @return The repository name for the source code.
*/
public final String repository() {
return repository;
}
/**
*
* The type of repository to use for the source code.
*
*
* @return The type of repository to use for the source code.
*/
public final String versionControlType() {
return versionControlType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(branch());
hashCode = 31 * hashCode + Objects.hashCode(projectName());
hashCode = 31 * hashCode + Objects.hashCode(repository());
hashCode = 31 * hashCode + Objects.hashCode(versionControlType());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SourceCodeRepository)) {
return false;
}
SourceCodeRepository other = (SourceCodeRepository) obj;
return Objects.equals(branch(), other.branch()) && Objects.equals(projectName(), other.projectName())
&& Objects.equals(repository(), other.repository())
&& Objects.equals(versionControlType(), other.versionControlType());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SourceCodeRepository").add("Branch", branch()).add("ProjectName", projectName())
.add("Repository", repository()).add("VersionControlType", versionControlType()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "branch":
return Optional.ofNullable(clazz.cast(branch()));
case "projectName":
return Optional.ofNullable(clazz.cast(projectName()));
case "repository":
return Optional.ofNullable(clazz.cast(repository()));
case "versionControlType":
return Optional.ofNullable(clazz.cast(versionControlType()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("branch", BRANCH_FIELD);
map.put("projectName", PROJECT_NAME_FIELD);
map.put("repository", REPOSITORY_FIELD);
map.put("versionControlType", VERSION_CONTROL_TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy