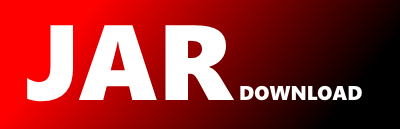
software.amazon.awssdk.services.mturk.model.SendBonusRequest Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class SendBonusRequest extends MTurkRequest implements ToCopyableBuilder {
private static final SdkField WORKER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkerId").getter(getter(SendBonusRequest::workerId)).setter(setter(Builder::workerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkerId").build()).build();
private static final SdkField BONUS_AMOUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BonusAmount").getter(getter(SendBonusRequest::bonusAmount)).setter(setter(Builder::bonusAmount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BonusAmount").build()).build();
private static final SdkField ASSIGNMENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AssignmentId").getter(getter(SendBonusRequest::assignmentId)).setter(setter(Builder::assignmentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssignmentId").build()).build();
private static final SdkField REASON_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Reason")
.getter(getter(SendBonusRequest::reason)).setter(setter(Builder::reason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Reason").build()).build();
private static final SdkField UNIQUE_REQUEST_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UniqueRequestToken").getter(getter(SendBonusRequest::uniqueRequestToken))
.setter(setter(Builder::uniqueRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UniqueRequestToken").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKER_ID_FIELD,
BONUS_AMOUNT_FIELD, ASSIGNMENT_ID_FIELD, REASON_FIELD, UNIQUE_REQUEST_TOKEN_FIELD));
private final String workerId;
private final String bonusAmount;
private final String assignmentId;
private final String reason;
private final String uniqueRequestToken;
private SendBonusRequest(BuilderImpl builder) {
super(builder);
this.workerId = builder.workerId;
this.bonusAmount = builder.bonusAmount;
this.assignmentId = builder.assignmentId;
this.reason = builder.reason;
this.uniqueRequestToken = builder.uniqueRequestToken;
}
/**
*
* The ID of the Worker being paid the bonus.
*
*
* @return The ID of the Worker being paid the bonus.
*/
public final String workerId() {
return workerId;
}
/**
*
* The Bonus amount is a US Dollar amount specified using a string (for example, "5" represents $5.00 USD and
* "101.42" represents $101.42 USD). Do not include currency symbols or currency codes.
*
*
* @return The Bonus amount is a US Dollar amount specified using a string (for example, "5" represents $5.00 USD
* and "101.42" represents $101.42 USD). Do not include currency symbols or currency codes.
*/
public final String bonusAmount() {
return bonusAmount;
}
/**
*
* The ID of the assignment for which this bonus is paid.
*
*
* @return The ID of the assignment for which this bonus is paid.
*/
public final String assignmentId() {
return assignmentId;
}
/**
*
* A message that explains the reason for the bonus payment. The Worker receiving the bonus can see this message.
*
*
* @return A message that explains the reason for the bonus payment. The Worker receiving the bonus can see this
* message.
*/
public final String reason() {
return reason;
}
/**
*
* A unique identifier for this request, which allows you to retry the call on error without granting multiple
* bonuses. This is useful in cases such as network timeouts where it is unclear whether or not the call succeeded
* on the server. If the bonus already exists in the system from a previous call using the same UniqueRequestToken,
* subsequent calls will return an error with a message containing the request ID.
*
*
* @return A unique identifier for this request, which allows you to retry the call on error without granting
* multiple bonuses. This is useful in cases such as network timeouts where it is unclear whether or not the
* call succeeded on the server. If the bonus already exists in the system from a previous call using the
* same UniqueRequestToken, subsequent calls will return an error with a message containing the request ID.
*/
public final String uniqueRequestToken() {
return uniqueRequestToken;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(workerId());
hashCode = 31 * hashCode + Objects.hashCode(bonusAmount());
hashCode = 31 * hashCode + Objects.hashCode(assignmentId());
hashCode = 31 * hashCode + Objects.hashCode(reason());
hashCode = 31 * hashCode + Objects.hashCode(uniqueRequestToken());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SendBonusRequest)) {
return false;
}
SendBonusRequest other = (SendBonusRequest) obj;
return Objects.equals(workerId(), other.workerId()) && Objects.equals(bonusAmount(), other.bonusAmount())
&& Objects.equals(assignmentId(), other.assignmentId()) && Objects.equals(reason(), other.reason())
&& Objects.equals(uniqueRequestToken(), other.uniqueRequestToken());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SendBonusRequest").add("WorkerId", workerId()).add("BonusAmount", bonusAmount())
.add("AssignmentId", assignmentId()).add("Reason", reason()).add("UniqueRequestToken", uniqueRequestToken())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "WorkerId":
return Optional.ofNullable(clazz.cast(workerId()));
case "BonusAmount":
return Optional.ofNullable(clazz.cast(bonusAmount()));
case "AssignmentId":
return Optional.ofNullable(clazz.cast(assignmentId()));
case "Reason":
return Optional.ofNullable(clazz.cast(reason()));
case "UniqueRequestToken":
return Optional.ofNullable(clazz.cast(uniqueRequestToken()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function