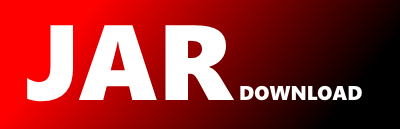
software.amazon.awssdk.services.mturk.model.QualificationRequirement Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The QualificationRequirement data structure describes a Qualification that a Worker must have before the Worker is
* allowed to accept a HIT. A requirement may optionally state that a Worker must have the Qualification in order to
* preview the HIT, or see the HIT in search results.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class QualificationRequirement implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField QUALIFICATION_TYPE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QualificationTypeId").getter(getter(QualificationRequirement::qualificationTypeId))
.setter(setter(Builder::qualificationTypeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QualificationTypeId").build())
.build();
private static final SdkField COMPARATOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Comparator").getter(getter(QualificationRequirement::comparatorAsString))
.setter(setter(Builder::comparator))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Comparator").build()).build();
private static final SdkField> INTEGER_VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("IntegerValues")
.getter(getter(QualificationRequirement::integerValues))
.setter(setter(Builder::integerValues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IntegerValues").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.INTEGER)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> LOCALE_VALUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LocaleValues")
.getter(getter(QualificationRequirement::localeValues))
.setter(setter(Builder::localeValues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocaleValues").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Locale::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField REQUIRED_TO_PREVIEW_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("RequiredToPreview").getter(getter(QualificationRequirement::requiredToPreview))
.setter(setter(Builder::requiredToPreview))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequiredToPreview").build()).build();
private static final SdkField ACTIONS_GUARDED_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionsGuarded").getter(getter(QualificationRequirement::actionsGuardedAsString))
.setter(setter(Builder::actionsGuarded))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionsGuarded").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(QUALIFICATION_TYPE_ID_FIELD,
COMPARATOR_FIELD, INTEGER_VALUES_FIELD, LOCALE_VALUES_FIELD, REQUIRED_TO_PREVIEW_FIELD, ACTIONS_GUARDED_FIELD));
private static final long serialVersionUID = 1L;
private final String qualificationTypeId;
private final String comparator;
private final List integerValues;
private final List localeValues;
private final Boolean requiredToPreview;
private final String actionsGuarded;
private QualificationRequirement(BuilderImpl builder) {
this.qualificationTypeId = builder.qualificationTypeId;
this.comparator = builder.comparator;
this.integerValues = builder.integerValues;
this.localeValues = builder.localeValues;
this.requiredToPreview = builder.requiredToPreview;
this.actionsGuarded = builder.actionsGuarded;
}
/**
*
* The ID of the Qualification type for the requirement.
*
*
* @return The ID of the Qualification type for the requirement.
*/
public final String qualificationTypeId() {
return qualificationTypeId;
}
/**
*
* The kind of comparison to make against a Qualification's value. You can compare a Qualification's value to an
* IntegerValue to see if it is LessThan, LessThanOrEqualTo, GreaterThan, GreaterThanOrEqualTo, EqualTo, or
* NotEqualTo the IntegerValue. You can compare it to a LocaleValue to see if it is EqualTo, or NotEqualTo the
* LocaleValue. You can check to see if the value is In or NotIn a set of IntegerValue or LocaleValue values.
* Lastly, a Qualification requirement can also test if a Qualification Exists or DoesNotExist in the user's
* profile, regardless of its value.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #comparator} will
* return {@link Comparator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #comparatorAsString}.
*
*
* @return The kind of comparison to make against a Qualification's value. You can compare a Qualification's value
* to an IntegerValue to see if it is LessThan, LessThanOrEqualTo, GreaterThan, GreaterThanOrEqualTo,
* EqualTo, or NotEqualTo the IntegerValue. You can compare it to a LocaleValue to see if it is EqualTo, or
* NotEqualTo the LocaleValue. You can check to see if the value is In or NotIn a set of IntegerValue or
* LocaleValue values. Lastly, a Qualification requirement can also test if a Qualification Exists or
* DoesNotExist in the user's profile, regardless of its value.
* @see Comparator
*/
public final Comparator comparator() {
return Comparator.fromValue(comparator);
}
/**
*
* The kind of comparison to make against a Qualification's value. You can compare a Qualification's value to an
* IntegerValue to see if it is LessThan, LessThanOrEqualTo, GreaterThan, GreaterThanOrEqualTo, EqualTo, or
* NotEqualTo the IntegerValue. You can compare it to a LocaleValue to see if it is EqualTo, or NotEqualTo the
* LocaleValue. You can check to see if the value is In or NotIn a set of IntegerValue or LocaleValue values.
* Lastly, a Qualification requirement can also test if a Qualification Exists or DoesNotExist in the user's
* profile, regardless of its value.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #comparator} will
* return {@link Comparator#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #comparatorAsString}.
*
*
* @return The kind of comparison to make against a Qualification's value. You can compare a Qualification's value
* to an IntegerValue to see if it is LessThan, LessThanOrEqualTo, GreaterThan, GreaterThanOrEqualTo,
* EqualTo, or NotEqualTo the IntegerValue. You can compare it to a LocaleValue to see if it is EqualTo, or
* NotEqualTo the LocaleValue. You can check to see if the value is In or NotIn a set of IntegerValue or
* LocaleValue values. Lastly, a Qualification requirement can also test if a Qualification Exists or
* DoesNotExist in the user's profile, regardless of its value.
* @see Comparator
*/
public final String comparatorAsString() {
return comparator;
}
/**
* For responses, this returns true if the service returned a value for the IntegerValues property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasIntegerValues() {
return integerValues != null && !(integerValues instanceof SdkAutoConstructList);
}
/**
*
* The integer value to compare against the Qualification's value. IntegerValue must not be present if Comparator is
* Exists or DoesNotExist. IntegerValue can only be used if the Qualification type has an integer value; it cannot
* be used with the Worker_Locale QualificationType ID. When performing a set comparison by using the In or the
* NotIn comparator, you can use up to 15 IntegerValue elements in a QualificationRequirement data structure.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIntegerValues} method.
*
*
* @return The integer value to compare against the Qualification's value. IntegerValue must not be present if
* Comparator is Exists or DoesNotExist. IntegerValue can only be used if the Qualification type has an
* integer value; it cannot be used with the Worker_Locale QualificationType ID. When performing a set
* comparison by using the In or the NotIn comparator, you can use up to 15 IntegerValue elements in a
* QualificationRequirement data structure.
*/
public final List integerValues() {
return integerValues;
}
/**
* For responses, this returns true if the service returned a value for the LocaleValues property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasLocaleValues() {
return localeValues != null && !(localeValues instanceof SdkAutoConstructList);
}
/**
*
* The locale value to compare against the Qualification's value. The local value must be a valid ISO 3166 country
* code or supports ISO 3166-2 subdivisions. LocaleValue can only be used with a Worker_Locale QualificationType ID.
* LocaleValue can only be used with the EqualTo, NotEqualTo, In, and NotIn comparators. You must only use a single
* LocaleValue element when using the EqualTo or NotEqualTo comparators. When performing a set comparison by using
* the In or the NotIn comparator, you can use up to 30 LocaleValue elements in a QualificationRequirement data
* structure.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLocaleValues} method.
*
*
* @return The locale value to compare against the Qualification's value. The local value must be a valid ISO 3166
* country code or supports ISO 3166-2 subdivisions. LocaleValue can only be used with a Worker_Locale
* QualificationType ID. LocaleValue can only be used with the EqualTo, NotEqualTo, In, and NotIn
* comparators. You must only use a single LocaleValue element when using the EqualTo or NotEqualTo
* comparators. When performing a set comparison by using the In or the NotIn comparator, you can use up to
* 30 LocaleValue elements in a QualificationRequirement data structure.
*/
public final List localeValues() {
return localeValues;
}
/**
*
* DEPRECATED: Use the ActionsGuarded
field instead. If RequiredToPreview is true, the question data
* for the HIT will not be shown when a Worker whose Qualifications do not meet this requirement tries to preview
* the HIT. That is, a Worker's Qualifications must meet all of the requirements for which RequiredToPreview is true
* in order to preview the HIT. If a Worker meets all of the requirements where RequiredToPreview is true (or if
* there are no such requirements), but does not meet all of the requirements for the HIT, the Worker will be
* allowed to preview the HIT's question data, but will not be allowed to accept and complete the HIT. The default
* is false. This should not be used in combination with the ActionsGuarded
field.
*
*
* @return DEPRECATED: Use the ActionsGuarded
field instead. If RequiredToPreview is true, the question
* data for the HIT will not be shown when a Worker whose Qualifications do not meet this requirement tries
* to preview the HIT. That is, a Worker's Qualifications must meet all of the requirements for which
* RequiredToPreview is true in order to preview the HIT. If a Worker meets all of the requirements where
* RequiredToPreview is true (or if there are no such requirements), but does not meet all of the
* requirements for the HIT, the Worker will be allowed to preview the HIT's question data, but will not be
* allowed to accept and complete the HIT. The default is false. This should not be used in combination with
* the ActionsGuarded
field.
* @deprecated
*/
@Deprecated
public final Boolean requiredToPreview() {
return requiredToPreview;
}
/**
*
* Setting this attribute prevents Workers whose Qualifications do not meet this QualificationRequirement from
* taking the specified action. Valid arguments include "Accept" (Worker cannot accept the HIT, but can preview the
* HIT and see it in their search results), "PreviewAndAccept" (Worker cannot accept or preview the HIT, but can see
* the HIT in their search results), and "DiscoverPreviewAndAccept" (Worker cannot accept, preview, or see the HIT
* in their search results). It's possible for you to create a HIT with multiple QualificationRequirements (which
* can have different values for the ActionGuarded attribute). In this case, the Worker is only permitted to perform
* an action when they have met all QualificationRequirements guarding the action. The actions in the order of least
* restrictive to most restrictive are Discover, Preview and Accept. For example, if a Worker meets all
* QualificationRequirements that are set to DiscoverPreviewAndAccept, but do not meet all requirements that are set
* with PreviewAndAccept, then the Worker will be able to Discover, i.e. see the HIT in their search result, but
* will not be able to Preview or Accept the HIT. ActionsGuarded should not be used in combination with the
* RequiredToPreview
field.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #actionsGuarded}
* will return {@link HITAccessActions#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionsGuardedAsString}.
*
*
* @return Setting this attribute prevents Workers whose Qualifications do not meet this QualificationRequirement
* from taking the specified action. Valid arguments include "Accept" (Worker cannot accept the HIT, but can
* preview the HIT and see it in their search results), "PreviewAndAccept" (Worker cannot accept or preview
* the HIT, but can see the HIT in their search results), and "DiscoverPreviewAndAccept" (Worker cannot
* accept, preview, or see the HIT in their search results). It's possible for you to create a HIT with
* multiple QualificationRequirements (which can have different values for the ActionGuarded attribute). In
* this case, the Worker is only permitted to perform an action when they have met all
* QualificationRequirements guarding the action. The actions in the order of least restrictive to most
* restrictive are Discover, Preview and Accept. For example, if a Worker meets all
* QualificationRequirements that are set to DiscoverPreviewAndAccept, but do not meet all requirements that
* are set with PreviewAndAccept, then the Worker will be able to Discover, i.e. see the HIT in their search
* result, but will not be able to Preview or Accept the HIT. ActionsGuarded should not be used in
* combination with the RequiredToPreview
field.
* @see HITAccessActions
*/
public final HITAccessActions actionsGuarded() {
return HITAccessActions.fromValue(actionsGuarded);
}
/**
*
* Setting this attribute prevents Workers whose Qualifications do not meet this QualificationRequirement from
* taking the specified action. Valid arguments include "Accept" (Worker cannot accept the HIT, but can preview the
* HIT and see it in their search results), "PreviewAndAccept" (Worker cannot accept or preview the HIT, but can see
* the HIT in their search results), and "DiscoverPreviewAndAccept" (Worker cannot accept, preview, or see the HIT
* in their search results). It's possible for you to create a HIT with multiple QualificationRequirements (which
* can have different values for the ActionGuarded attribute). In this case, the Worker is only permitted to perform
* an action when they have met all QualificationRequirements guarding the action. The actions in the order of least
* restrictive to most restrictive are Discover, Preview and Accept. For example, if a Worker meets all
* QualificationRequirements that are set to DiscoverPreviewAndAccept, but do not meet all requirements that are set
* with PreviewAndAccept, then the Worker will be able to Discover, i.e. see the HIT in their search result, but
* will not be able to Preview or Accept the HIT. ActionsGuarded should not be used in combination with the
* RequiredToPreview
field.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #actionsGuarded}
* will return {@link HITAccessActions#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #actionsGuardedAsString}.
*
*
* @return Setting this attribute prevents Workers whose Qualifications do not meet this QualificationRequirement
* from taking the specified action. Valid arguments include "Accept" (Worker cannot accept the HIT, but can
* preview the HIT and see it in their search results), "PreviewAndAccept" (Worker cannot accept or preview
* the HIT, but can see the HIT in their search results), and "DiscoverPreviewAndAccept" (Worker cannot
* accept, preview, or see the HIT in their search results). It's possible for you to create a HIT with
* multiple QualificationRequirements (which can have different values for the ActionGuarded attribute). In
* this case, the Worker is only permitted to perform an action when they have met all
* QualificationRequirements guarding the action. The actions in the order of least restrictive to most
* restrictive are Discover, Preview and Accept. For example, if a Worker meets all
* QualificationRequirements that are set to DiscoverPreviewAndAccept, but do not meet all requirements that
* are set with PreviewAndAccept, then the Worker will be able to Discover, i.e. see the HIT in their search
* result, but will not be able to Preview or Accept the HIT. ActionsGuarded should not be used in
* combination with the RequiredToPreview
field.
* @see HITAccessActions
*/
public final String actionsGuardedAsString() {
return actionsGuarded;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(qualificationTypeId());
hashCode = 31 * hashCode + Objects.hashCode(comparatorAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasIntegerValues() ? integerValues() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasLocaleValues() ? localeValues() : null);
hashCode = 31 * hashCode + Objects.hashCode(requiredToPreview());
hashCode = 31 * hashCode + Objects.hashCode(actionsGuardedAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof QualificationRequirement)) {
return false;
}
QualificationRequirement other = (QualificationRequirement) obj;
return Objects.equals(qualificationTypeId(), other.qualificationTypeId())
&& Objects.equals(comparatorAsString(), other.comparatorAsString())
&& hasIntegerValues() == other.hasIntegerValues() && Objects.equals(integerValues(), other.integerValues())
&& hasLocaleValues() == other.hasLocaleValues() && Objects.equals(localeValues(), other.localeValues())
&& Objects.equals(requiredToPreview(), other.requiredToPreview())
&& Objects.equals(actionsGuardedAsString(), other.actionsGuardedAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("QualificationRequirement").add("QualificationTypeId", qualificationTypeId())
.add("Comparator", comparatorAsString()).add("IntegerValues", hasIntegerValues() ? integerValues() : null)
.add("LocaleValues", hasLocaleValues() ? localeValues() : null).add("RequiredToPreview", requiredToPreview())
.add("ActionsGuarded", actionsGuardedAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "QualificationTypeId":
return Optional.ofNullable(clazz.cast(qualificationTypeId()));
case "Comparator":
return Optional.ofNullable(clazz.cast(comparatorAsString()));
case "IntegerValues":
return Optional.ofNullable(clazz.cast(integerValues()));
case "LocaleValues":
return Optional.ofNullable(clazz.cast(localeValues()));
case "RequiredToPreview":
return Optional.ofNullable(clazz.cast(requiredToPreview()));
case "ActionsGuarded":
return Optional.ofNullable(clazz.cast(actionsGuardedAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function