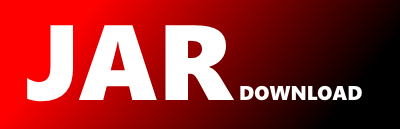
software.amazon.awssdk.services.mturk.model.UpdateNotificationSettingsRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mturk Show documentation
Show all versions of mturk Show documentation
The AWS Java SDK for Amazon Mechanical Turk Requester module holds the client classes that are used
for communicating with Amazon Mechanical Turk Requester Service.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateNotificationSettingsRequest extends MTurkRequest implements
ToCopyableBuilder {
private static final SdkField HIT_TYPE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HITTypeId").getter(getter(UpdateNotificationSettingsRequest::hitTypeId))
.setter(setter(Builder::hitTypeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HITTypeId").build()).build();
private static final SdkField NOTIFICATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Notification")
.getter(getter(UpdateNotificationSettingsRequest::notification)).setter(setter(Builder::notification))
.constructor(NotificationSpecification::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Notification").build()).build();
private static final SdkField ACTIVE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Active").getter(getter(UpdateNotificationSettingsRequest::active)).setter(setter(Builder::active))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Active").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(HIT_TYPE_ID_FIELD,
NOTIFICATION_FIELD, ACTIVE_FIELD));
private final String hitTypeId;
private final NotificationSpecification notification;
private final Boolean active;
private UpdateNotificationSettingsRequest(BuilderImpl builder) {
super(builder);
this.hitTypeId = builder.hitTypeId;
this.notification = builder.notification;
this.active = builder.active;
}
/**
*
* The ID of the HIT type whose notification specification is being updated.
*
*
* @return The ID of the HIT type whose notification specification is being updated.
*/
public final String hitTypeId() {
return hitTypeId;
}
/**
*
* The notification specification for the HIT type.
*
*
* @return The notification specification for the HIT type.
*/
public final NotificationSpecification notification() {
return notification;
}
/**
*
* Specifies whether notifications are sent for HITs of this HIT type, according to the notification specification.
* You must specify either the Notification parameter or the Active parameter for the call to
* UpdateNotificationSettings to succeed.
*
*
* @return Specifies whether notifications are sent for HITs of this HIT type, according to the notification
* specification. You must specify either the Notification parameter or the Active parameter for the call to
* UpdateNotificationSettings to succeed.
*/
public final Boolean active() {
return active;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(hitTypeId());
hashCode = 31 * hashCode + Objects.hashCode(notification());
hashCode = 31 * hashCode + Objects.hashCode(active());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateNotificationSettingsRequest)) {
return false;
}
UpdateNotificationSettingsRequest other = (UpdateNotificationSettingsRequest) obj;
return Objects.equals(hitTypeId(), other.hitTypeId()) && Objects.equals(notification(), other.notification())
&& Objects.equals(active(), other.active());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateNotificationSettingsRequest").add("HITTypeId", hitTypeId())
.add("Notification", notification()).add("Active", active()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "HITTypeId":
return Optional.ofNullable(clazz.cast(hitTypeId()));
case "Notification":
return Optional.ofNullable(clazz.cast(notification()));
case "Active":
return Optional.ofNullable(clazz.cast(active()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy