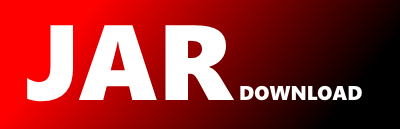
software.amazon.awssdk.services.mturk.model.ReviewActionDetail Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Both the AssignmentReviewReport and the HITReviewReport elements contains the ReviewActionDetail data structure. This
* structure is returned multiple times for each action specified in the Review Policy.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ReviewActionDetail implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ACTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionId").getter(getter(ReviewActionDetail::actionId)).setter(setter(Builder::actionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionId").build()).build();
private static final SdkField ACTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ActionName").getter(getter(ReviewActionDetail::actionName)).setter(setter(Builder::actionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ActionName").build()).build();
private static final SdkField TARGET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetId").getter(getter(ReviewActionDetail::targetId)).setter(setter(Builder::targetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetId").build()).build();
private static final SdkField TARGET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TargetType").getter(getter(ReviewActionDetail::targetType)).setter(setter(Builder::targetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TargetType").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(ReviewActionDetail::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField COMPLETE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CompleteTime").getter(getter(ReviewActionDetail::completeTime)).setter(setter(Builder::completeTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CompleteTime").build()).build();
private static final SdkField RESULT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Result")
.getter(getter(ReviewActionDetail::result)).setter(setter(Builder::result))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Result").build()).build();
private static final SdkField ERROR_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ErrorCode").getter(getter(ReviewActionDetail::errorCode)).setter(setter(Builder::errorCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ErrorCode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTION_ID_FIELD,
ACTION_NAME_FIELD, TARGET_ID_FIELD, TARGET_TYPE_FIELD, STATUS_FIELD, COMPLETE_TIME_FIELD, RESULT_FIELD,
ERROR_CODE_FIELD));
private static final long serialVersionUID = 1L;
private final String actionId;
private final String actionName;
private final String targetId;
private final String targetType;
private final String status;
private final Instant completeTime;
private final String result;
private final String errorCode;
private ReviewActionDetail(BuilderImpl builder) {
this.actionId = builder.actionId;
this.actionName = builder.actionName;
this.targetId = builder.targetId;
this.targetType = builder.targetType;
this.status = builder.status;
this.completeTime = builder.completeTime;
this.result = builder.result;
this.errorCode = builder.errorCode;
}
/**
*
* The unique identifier for the action.
*
*
* @return The unique identifier for the action.
*/
public final String actionId() {
return actionId;
}
/**
*
* The nature of the action itself. The Review Policy is responsible for examining the HIT and Assignments, emitting
* results, and deciding which other actions will be necessary.
*
*
* @return The nature of the action itself. The Review Policy is responsible for examining the HIT and Assignments,
* emitting results, and deciding which other actions will be necessary.
*/
public final String actionName() {
return actionName;
}
/**
*
* The specific HITId or AssignmentID targeted by the action.
*
*
* @return The specific HITId or AssignmentID targeted by the action.
*/
public final String targetId() {
return targetId;
}
/**
*
* The type of object in TargetId.
*
*
* @return The type of object in TargetId.
*/
public final String targetType() {
return targetType;
}
/**
*
* The current disposition of the action: INTENDED, SUCCEEDED, FAILED, or CANCELLED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ReviewActionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current disposition of the action: INTENDED, SUCCEEDED, FAILED, or CANCELLED.
* @see ReviewActionStatus
*/
public final ReviewActionStatus status() {
return ReviewActionStatus.fromValue(status);
}
/**
*
* The current disposition of the action: INTENDED, SUCCEEDED, FAILED, or CANCELLED.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ReviewActionStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current disposition of the action: INTENDED, SUCCEEDED, FAILED, or CANCELLED.
* @see ReviewActionStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The date when the action was completed.
*
*
* @return The date when the action was completed.
*/
public final Instant completeTime() {
return completeTime;
}
/**
*
* A description of the outcome of the review.
*
*
* @return A description of the outcome of the review.
*/
public final String result() {
return result;
}
/**
*
* Present only when the Results have a FAILED Status.
*
*
* @return Present only when the Results have a FAILED Status.
*/
public final String errorCode() {
return errorCode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(actionId());
hashCode = 31 * hashCode + Objects.hashCode(actionName());
hashCode = 31 * hashCode + Objects.hashCode(targetId());
hashCode = 31 * hashCode + Objects.hashCode(targetType());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(completeTime());
hashCode = 31 * hashCode + Objects.hashCode(result());
hashCode = 31 * hashCode + Objects.hashCode(errorCode());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ReviewActionDetail)) {
return false;
}
ReviewActionDetail other = (ReviewActionDetail) obj;
return Objects.equals(actionId(), other.actionId()) && Objects.equals(actionName(), other.actionName())
&& Objects.equals(targetId(), other.targetId()) && Objects.equals(targetType(), other.targetType())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(completeTime(), other.completeTime()) && Objects.equals(result(), other.result())
&& Objects.equals(errorCode(), other.errorCode());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ReviewActionDetail").add("ActionId", actionId()).add("ActionName", actionName())
.add("TargetId", targetId()).add("TargetType", targetType()).add("Status", statusAsString())
.add("CompleteTime", completeTime()).add("Result", result()).add("ErrorCode", errorCode()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ActionId":
return Optional.ofNullable(clazz.cast(actionId()));
case "ActionName":
return Optional.ofNullable(clazz.cast(actionName()));
case "TargetId":
return Optional.ofNullable(clazz.cast(targetId()));
case "TargetType":
return Optional.ofNullable(clazz.cast(targetType()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "CompleteTime":
return Optional.ofNullable(clazz.cast(completeTime()));
case "Result":
return Optional.ofNullable(clazz.cast(result()));
case "ErrorCode":
return Optional.ofNullable(clazz.cast(errorCode()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function