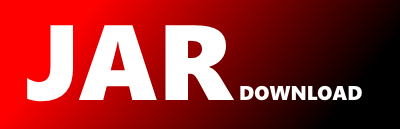
software.amazon.awssdk.services.mturk.DefaultMTurkAsyncClient Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.mturk.internal.MTurkServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.mturk.model.AcceptQualificationRequestRequest;
import software.amazon.awssdk.services.mturk.model.AcceptQualificationRequestResponse;
import software.amazon.awssdk.services.mturk.model.ApproveAssignmentRequest;
import software.amazon.awssdk.services.mturk.model.ApproveAssignmentResponse;
import software.amazon.awssdk.services.mturk.model.AssociateQualificationWithWorkerRequest;
import software.amazon.awssdk.services.mturk.model.AssociateQualificationWithWorkerResponse;
import software.amazon.awssdk.services.mturk.model.CreateAdditionalAssignmentsForHitRequest;
import software.amazon.awssdk.services.mturk.model.CreateAdditionalAssignmentsForHitResponse;
import software.amazon.awssdk.services.mturk.model.CreateHitRequest;
import software.amazon.awssdk.services.mturk.model.CreateHitResponse;
import software.amazon.awssdk.services.mturk.model.CreateHitTypeRequest;
import software.amazon.awssdk.services.mturk.model.CreateHitTypeResponse;
import software.amazon.awssdk.services.mturk.model.CreateHitWithHitTypeRequest;
import software.amazon.awssdk.services.mturk.model.CreateHitWithHitTypeResponse;
import software.amazon.awssdk.services.mturk.model.CreateQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.CreateQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.model.CreateWorkerBlockRequest;
import software.amazon.awssdk.services.mturk.model.CreateWorkerBlockResponse;
import software.amazon.awssdk.services.mturk.model.DeleteHitRequest;
import software.amazon.awssdk.services.mturk.model.DeleteHitResponse;
import software.amazon.awssdk.services.mturk.model.DeleteQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.DeleteQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.model.DeleteWorkerBlockRequest;
import software.amazon.awssdk.services.mturk.model.DeleteWorkerBlockResponse;
import software.amazon.awssdk.services.mturk.model.DisassociateQualificationFromWorkerRequest;
import software.amazon.awssdk.services.mturk.model.DisassociateQualificationFromWorkerResponse;
import software.amazon.awssdk.services.mturk.model.GetAccountBalanceRequest;
import software.amazon.awssdk.services.mturk.model.GetAccountBalanceResponse;
import software.amazon.awssdk.services.mturk.model.GetAssignmentRequest;
import software.amazon.awssdk.services.mturk.model.GetAssignmentResponse;
import software.amazon.awssdk.services.mturk.model.GetFileUploadUrlRequest;
import software.amazon.awssdk.services.mturk.model.GetFileUploadUrlResponse;
import software.amazon.awssdk.services.mturk.model.GetHitRequest;
import software.amazon.awssdk.services.mturk.model.GetHitResponse;
import software.amazon.awssdk.services.mturk.model.GetQualificationScoreRequest;
import software.amazon.awssdk.services.mturk.model.GetQualificationScoreResponse;
import software.amazon.awssdk.services.mturk.model.GetQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.GetQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.model.ListAssignmentsForHitRequest;
import software.amazon.awssdk.services.mturk.model.ListAssignmentsForHitResponse;
import software.amazon.awssdk.services.mturk.model.ListBonusPaymentsRequest;
import software.amazon.awssdk.services.mturk.model.ListBonusPaymentsResponse;
import software.amazon.awssdk.services.mturk.model.ListHiTsForQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.ListHiTsForQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.model.ListHiTsRequest;
import software.amazon.awssdk.services.mturk.model.ListHiTsResponse;
import software.amazon.awssdk.services.mturk.model.ListQualificationRequestsRequest;
import software.amazon.awssdk.services.mturk.model.ListQualificationRequestsResponse;
import software.amazon.awssdk.services.mturk.model.ListQualificationTypesRequest;
import software.amazon.awssdk.services.mturk.model.ListQualificationTypesResponse;
import software.amazon.awssdk.services.mturk.model.ListReviewPolicyResultsForHitRequest;
import software.amazon.awssdk.services.mturk.model.ListReviewPolicyResultsForHitResponse;
import software.amazon.awssdk.services.mturk.model.ListReviewableHiTsRequest;
import software.amazon.awssdk.services.mturk.model.ListReviewableHiTsResponse;
import software.amazon.awssdk.services.mturk.model.ListWorkerBlocksRequest;
import software.amazon.awssdk.services.mturk.model.ListWorkerBlocksResponse;
import software.amazon.awssdk.services.mturk.model.ListWorkersWithQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.ListWorkersWithQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.model.MTurkException;
import software.amazon.awssdk.services.mturk.model.NotifyWorkersRequest;
import software.amazon.awssdk.services.mturk.model.NotifyWorkersResponse;
import software.amazon.awssdk.services.mturk.model.RejectAssignmentRequest;
import software.amazon.awssdk.services.mturk.model.RejectAssignmentResponse;
import software.amazon.awssdk.services.mturk.model.RejectQualificationRequestRequest;
import software.amazon.awssdk.services.mturk.model.RejectQualificationRequestResponse;
import software.amazon.awssdk.services.mturk.model.RequestErrorException;
import software.amazon.awssdk.services.mturk.model.SendBonusRequest;
import software.amazon.awssdk.services.mturk.model.SendBonusResponse;
import software.amazon.awssdk.services.mturk.model.SendTestEventNotificationRequest;
import software.amazon.awssdk.services.mturk.model.SendTestEventNotificationResponse;
import software.amazon.awssdk.services.mturk.model.ServiceException;
import software.amazon.awssdk.services.mturk.model.UpdateExpirationForHitRequest;
import software.amazon.awssdk.services.mturk.model.UpdateExpirationForHitResponse;
import software.amazon.awssdk.services.mturk.model.UpdateHitReviewStatusRequest;
import software.amazon.awssdk.services.mturk.model.UpdateHitReviewStatusResponse;
import software.amazon.awssdk.services.mturk.model.UpdateHitTypeOfHitRequest;
import software.amazon.awssdk.services.mturk.model.UpdateHitTypeOfHitResponse;
import software.amazon.awssdk.services.mturk.model.UpdateNotificationSettingsRequest;
import software.amazon.awssdk.services.mturk.model.UpdateNotificationSettingsResponse;
import software.amazon.awssdk.services.mturk.model.UpdateQualificationTypeRequest;
import software.amazon.awssdk.services.mturk.model.UpdateQualificationTypeResponse;
import software.amazon.awssdk.services.mturk.transform.AcceptQualificationRequestRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ApproveAssignmentRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.AssociateQualificationWithWorkerRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateAdditionalAssignmentsForHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateHitTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateHitWithHitTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateQualificationTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.CreateWorkerBlockRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.DeleteHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.DeleteQualificationTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.DeleteWorkerBlockRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.DisassociateQualificationFromWorkerRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetAccountBalanceRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetAssignmentRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetFileUploadUrlRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetQualificationScoreRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.GetQualificationTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListAssignmentsForHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListBonusPaymentsRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListHiTsForQualificationTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListHiTsRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListQualificationRequestsRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListQualificationTypesRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListReviewPolicyResultsForHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListReviewableHiTsRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListWorkerBlocksRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.ListWorkersWithQualificationTypeRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.NotifyWorkersRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.RejectAssignmentRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.RejectQualificationRequestRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.SendBonusRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.SendTestEventNotificationRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.UpdateExpirationForHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.UpdateHitReviewStatusRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.UpdateHitTypeOfHitRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.UpdateNotificationSettingsRequestMarshaller;
import software.amazon.awssdk.services.mturk.transform.UpdateQualificationTypeRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link MTurkAsyncClient}.
*
* @see MTurkAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultMTurkAsyncClient implements MTurkAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultMTurkAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultMTurkAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* The AcceptQualificationRequest
operation approves a Worker's request for a Qualification.
*
*
* Only the owner of the Qualification type can grant a Qualification request for that type.
*
*
* A successful request for the AcceptQualificationRequest
operation returns with no errors and an
* empty body.
*
*
* @param acceptQualificationRequestRequest
* @return A Java Future containing the result of the AcceptQualificationRequest operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.AcceptQualificationRequest
* @see AWS API Documentation
*/
@Override
public CompletableFuture acceptQualificationRequest(
AcceptQualificationRequestRequest acceptQualificationRequestRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(acceptQualificationRequestRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, acceptQualificationRequestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AcceptQualificationRequest");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AcceptQualificationRequestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AcceptQualificationRequest").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AcceptQualificationRequestRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(acceptQualificationRequestRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ApproveAssignment
operation approves the results of a completed assignment.
*
*
* Approving an assignment initiates two payments from the Requester's Amazon.com account
*
*
* -
*
* The Worker who submitted the results is paid the reward specified in the HIT.
*
*
* -
*
* Amazon Mechanical Turk fees are debited.
*
*
*
*
* If the Requester's account does not have adequate funds for these payments, the call to ApproveAssignment returns
* an exception, and the approval is not processed. You can include an optional feedback message with the approval,
* which the Worker can see in the Status section of the web site.
*
*
* You can also call this operation for assignments that were previous rejected and approve them by explicitly
* overriding the previous rejection. This only works on rejected assignments that were submitted within the
* previous 30 days and only if the assignment's related HIT has not been deleted.
*
*
* @param approveAssignmentRequest
* @return A Java Future containing the result of the ApproveAssignment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ApproveAssignment
* @see AWS API Documentation
*/
@Override
public CompletableFuture approveAssignment(ApproveAssignmentRequest approveAssignmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(approveAssignmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, approveAssignmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ApproveAssignment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ApproveAssignmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ApproveAssignment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ApproveAssignmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(approveAssignmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The AssociateQualificationWithWorker
operation gives a Worker a Qualification.
* AssociateQualificationWithWorker
does not require that the Worker submit a Qualification request. It
* gives the Qualification directly to the Worker.
*
*
* You can only assign a Qualification of a Qualification type that you created (using the
* CreateQualificationType
operation).
*
*
*
* Note: AssociateQualificationWithWorker
does not affect any pending Qualification requests for the
* Qualification by the Worker. If you assign a Qualification to a Worker, then later grant a Qualification request
* made by the Worker, the granting of the request may modify the Qualification score. To resolve a pending
* Qualification request without affecting the Qualification the Worker already has, reject the request with the
* RejectQualificationRequest
operation.
*
*
*
* @param associateQualificationWithWorkerRequest
* @return A Java Future containing the result of the AssociateQualificationWithWorker operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.AssociateQualificationWithWorker
* @see AWS API Documentation
*/
@Override
public CompletableFuture associateQualificationWithWorker(
AssociateQualificationWithWorkerRequest associateQualificationWithWorkerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateQualificationWithWorkerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
associateQualificationWithWorkerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateQualificationWithWorker");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, AssociateQualificationWithWorkerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateQualificationWithWorker").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateQualificationWithWorkerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateQualificationWithWorkerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateAdditionalAssignmentsForHIT
operation increases the maximum number of assignments of an
* existing HIT.
*
*
* To extend the maximum number of assignments, specify the number of additional assignments.
*
*
*
* -
*
* HITs created with fewer than 10 assignments cannot be extended to have 10 or more assignments. Attempting to add
* assignments in a way that brings the total number of assignments for a HIT from fewer than 10 assignments to 10
* or more assignments will result in an AWS.MechanicalTurk.InvalidMaximumAssignmentsIncrease
* exception.
*
*
* -
*
* HITs that were created before July 22, 2015 cannot be extended. Attempting to extend HITs that were created
* before July 22, 2015 will result in an AWS.MechanicalTurk.HITTooOldForExtension
exception.
*
*
*
*
*
* @param createAdditionalAssignmentsForHitRequest
* @return A Java Future containing the result of the CreateAdditionalAssignmentsForHIT operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateAdditionalAssignmentsForHIT
* @see AWS API Documentation
*/
@Override
public CompletableFuture createAdditionalAssignmentsForHIT(
CreateAdditionalAssignmentsForHitRequest createAdditionalAssignmentsForHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAdditionalAssignmentsForHitRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
createAdditionalAssignmentsForHitRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateAdditionalAssignmentsForHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, CreateAdditionalAssignmentsForHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAdditionalAssignmentsForHIT").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAdditionalAssignmentsForHitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createAdditionalAssignmentsForHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateHIT
operation creates a new Human Intelligence Task (HIT). The new HIT is made available
* for Workers to find and accept on the Amazon Mechanical Turk website.
*
*
* This operation allows you to specify a new HIT by passing in values for the properties of the HIT, such as its
* title, reward amount and number of assignments. When you pass these values to CreateHIT
, a new HIT
* is created for you, with a new HITTypeID
. The HITTypeID can be used to create additional HITs in the
* future without needing to specify common parameters such as the title, description and reward amount each time.
*
*
* An alternative way to create HITs is to first generate a HITTypeID using the CreateHITType
operation
* and then call the CreateHITWithHITType
operation. This is the recommended best practice for
* Requesters who are creating large numbers of HITs.
*
*
* CreateHIT also supports several ways to provide question data: by providing a value for the Question
* parameter that fully specifies the contents of the HIT, or by providing a HitLayoutId
and associated
* HitLayoutParameters
.
*
*
*
* If a HIT is created with 10 or more maximum assignments, there is an additional fee. For more information, see Amazon Mechanical Turk Pricing.
*
*
*
* @param createHitRequest
* @return A Java Future containing the result of the CreateHIT operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateHIT
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createHIT(CreateHitRequest createHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHitRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateHIT")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHitRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(createHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateHITType
operation creates a new HIT type. This operation allows you to define a standard
* set of HIT properties to use when creating HITs. If you register a HIT type with values that match an existing
* HIT type, the HIT type ID of the existing type will be returned.
*
*
* @param createHitTypeRequest
* @return A Java Future containing the result of the CreateHITType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateHITType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createHITType(CreateHitTypeRequest createHitTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHitTypeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHitTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHITType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateHitTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHITType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHitTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createHitTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateHITWithHITType
operation creates a new Human Intelligence Task (HIT) using an existing
* HITTypeID generated by the CreateHITType
operation.
*
*
* This is an alternative way to create HITs from the CreateHIT
operation. This is the recommended best
* practice for Requesters who are creating large numbers of HITs.
*
*
* CreateHITWithHITType also supports several ways to provide question data: by providing a value for the
* Question
parameter that fully specifies the contents of the HIT, or by providing a
* HitLayoutId
and associated HitLayoutParameters
.
*
*
*
* If a HIT is created with 10 or more maximum assignments, there is an additional fee. For more information, see Amazon Mechanical Turk Pricing.
*
*
*
* @param createHitWithHitTypeRequest
* @return A Java Future containing the result of the CreateHITWithHITType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateHITWithHITType
* @see AWS API Documentation
*/
@Override
public CompletableFuture createHITWithHITType(
CreateHitWithHitTypeRequest createHitWithHitTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createHitWithHitTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createHitWithHitTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateHITWithHITType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateHitWithHitTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateHITWithHITType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateHitWithHitTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createHitWithHitTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateQualificationType
operation creates a new Qualification type, which is represented by a
* QualificationType
data structure.
*
*
* @param createQualificationTypeRequest
* @return A Java Future containing the result of the CreateQualificationType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateQualificationType
* @see AWS API Documentation
*/
@Override
public CompletableFuture createQualificationType(
CreateQualificationTypeRequest createQualificationTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createQualificationTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createQualificationTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateQualificationType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateQualificationTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateQualificationType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateQualificationTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createQualificationTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The CreateWorkerBlock
operation allows you to prevent a Worker from working on your HITs. For
* example, you can block a Worker who is producing poor quality work. You can block up to 100,000 Workers.
*
*
* @param createWorkerBlockRequest
* @return A Java Future containing the result of the CreateWorkerBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.CreateWorkerBlock
* @see AWS API Documentation
*/
@Override
public CompletableFuture createWorkerBlock(CreateWorkerBlockRequest createWorkerBlockRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createWorkerBlockRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createWorkerBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateWorkerBlock");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateWorkerBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateWorkerBlock").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateWorkerBlockRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createWorkerBlockRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The DeleteHIT
operation is used to delete HIT that is no longer needed. Only the Requester who
* created the HIT can delete it.
*
*
* You can only dispose of HITs that are in the Reviewable
state, with all of their submitted
* assignments already either approved or rejected. If you call the DeleteHIT operation on a HIT that is not in the
* Reviewable
state (for example, that has not expired, or still has active assignments), or on a HIT
* that is Reviewable but without all of its submitted assignments already approved or rejected, the service will
* return an error.
*
*
*
* -
*
* HITs are automatically disposed of after 120 days.
*
*
* -
*
* After you dispose of a HIT, you can no longer approve the HIT's rejected assignments.
*
*
* -
*
* Disposed HITs are not returned in results for the ListHITs operation.
*
*
* -
*
* Disposing HITs can improve the performance of operations such as ListReviewableHITs and ListHITs.
*
*
*
*
*
* @param deleteHitRequest
* @return A Java Future containing the result of the DeleteHIT operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.DeleteHIT
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteHIT(DeleteHitRequest deleteHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteHitRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteHitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteHIT")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteHitRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(deleteHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The DeleteQualificationType
deletes a Qualification type and deletes any HIT types that are
* associated with the Qualification type.
*
*
* This operation does not revoke Qualifications already assigned to Workers because the Qualifications might be
* needed for active HITs. If there are any pending requests for the Qualification type, Amazon Mechanical Turk
* rejects those requests. After you delete a Qualification type, you can no longer use it to create HITs or HIT
* types.
*
*
*
* DeleteQualificationType must wait for all the HITs that use the deleted Qualification type to be deleted before
* completing. It may take up to 48 hours before DeleteQualificationType completes and the unique name of the
* Qualification type is available for reuse with CreateQualificationType.
*
*
*
* @param deleteQualificationTypeRequest
* @return A Java Future containing the result of the DeleteQualificationType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.DeleteQualificationType
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteQualificationType(
DeleteQualificationTypeRequest deleteQualificationTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteQualificationTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteQualificationTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteQualificationType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteQualificationTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteQualificationType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteQualificationTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteQualificationTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The DeleteWorkerBlock
operation allows you to reinstate a blocked Worker to work on your HITs. This
* operation reverses the effects of the CreateWorkerBlock operation. You need the Worker ID to use this operation.
* If the Worker ID is missing or invalid, this operation fails and returns the message “WorkerId is invalid.” If
* the specified Worker is not blocked, this operation returns successfully.
*
*
* @param deleteWorkerBlockRequest
* @return A Java Future containing the result of the DeleteWorkerBlock operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.DeleteWorkerBlock
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteWorkerBlock(DeleteWorkerBlockRequest deleteWorkerBlockRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteWorkerBlockRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteWorkerBlockRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteWorkerBlock");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteWorkerBlockResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteWorkerBlock").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteWorkerBlockRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteWorkerBlockRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The DisassociateQualificationFromWorker
revokes a previously granted Qualification from a user.
*
*
* You can provide a text message explaining why the Qualification was revoked. The user who had the Qualification
* can see this message.
*
*
* @param disassociateQualificationFromWorkerRequest
* @return A Java Future containing the result of the DisassociateQualificationFromWorker operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.DisassociateQualificationFromWorker
* @see AWS API Documentation
*/
@Override
public CompletableFuture disassociateQualificationFromWorker(
DisassociateQualificationFromWorkerRequest disassociateQualificationFromWorkerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disassociateQualificationFromWorkerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
disassociateQualificationFromWorkerRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateQualificationFromWorker");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DisassociateQualificationFromWorkerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateQualificationFromWorker").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisassociateQualificationFromWorkerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disassociateQualificationFromWorkerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetAccountBalance
operation retrieves the Prepaid HITs balance in your Amazon Mechanical Turk
* account if you are a Prepaid Requester. Alternatively, this operation will retrieve the remaining available AWS
* Billing usage if you have enabled AWS Billing. Note: If you have enabled AWS Billing and still have a remaining
* Prepaid HITs balance, this balance can be viewed on the My Account page in the Requester console.
*
*
* @param getAccountBalanceRequest
* @return A Java Future containing the result of the GetAccountBalance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetAccountBalance
* @see AWS API Documentation
*/
@Override
public CompletableFuture getAccountBalance(GetAccountBalanceRequest getAccountBalanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAccountBalanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAccountBalanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAccountBalance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetAccountBalanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAccountBalance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAccountBalanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAccountBalanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetAssignment
operation retrieves the details of the specified Assignment.
*
*
* @param getAssignmentRequest
* @return A Java Future containing the result of the GetAssignment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetAssignment
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture getAssignment(GetAssignmentRequest getAssignmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getAssignmentRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getAssignmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetAssignment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetAssignmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetAssignment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetAssignmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getAssignmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetFileUploadURL
operation generates and returns a temporary URL. You use the temporary URL to
* retrieve a file uploaded by a Worker as an answer to a FileUploadAnswer question for a HIT. The temporary URL is
* generated the instant the GetFileUploadURL operation is called, and is valid for 60 seconds. You can get a
* temporary file upload URL any time until the HIT is disposed. After the HIT is disposed, any uploaded files are
* deleted, and cannot be retrieved. Pending Deprecation on December 12, 2017. The Answer Specification structure
* will no longer support the FileUploadAnswer
element to be used for the QuestionForm data structure.
* Instead, we recommend that Requesters who want to create HITs asking Workers to upload files to use Amazon S3.
*
*
* @param getFileUploadUrlRequest
* @return A Java Future containing the result of the GetFileUploadURL operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetFileUploadURL
* @see AWS API Documentation
*/
@Override
public CompletableFuture getFileUploadURL(GetFileUploadUrlRequest getFileUploadUrlRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getFileUploadUrlRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getFileUploadUrlRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetFileUploadURL");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetFileUploadUrlResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetFileUploadURL").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetFileUploadUrlRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getFileUploadUrlRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetHIT
operation retrieves the details of the specified HIT.
*
*
* @param getHitRequest
* @return A Java Future containing the result of the GetHIT operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetHIT
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getHIT(GetHitRequest getHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getHitRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getHitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetHIT")
.withProtocolMetadata(protocolMetadata).withMarshaller(new GetHitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetQualificationScore
operation returns the value of a Worker's Qualification for a given
* Qualification type.
*
*
* To get a Worker's Qualification, you must know the Worker's ID. The Worker's ID is included in the assignment
* data returned by the ListAssignmentsForHIT
operation.
*
*
* Only the owner of a Qualification type can query the value of a Worker's Qualification of that type.
*
*
* @param getQualificationScoreRequest
* @return A Java Future containing the result of the GetQualificationScore operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetQualificationScore
* @see AWS API Documentation
*/
@Override
public CompletableFuture getQualificationScore(
GetQualificationScoreRequest getQualificationScoreRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getQualificationScoreRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getQualificationScoreRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetQualificationScore");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetQualificationScoreResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetQualificationScore").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetQualificationScoreRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getQualificationScoreRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The GetQualificationType
operation retrieves information about a Qualification type using its ID.
*
*
* @param getQualificationTypeRequest
* @return A Java Future containing the result of the GetQualificationType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.GetQualificationType
* @see AWS API Documentation
*/
@Override
public CompletableFuture getQualificationType(
GetQualificationTypeRequest getQualificationTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getQualificationTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getQualificationTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetQualificationType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetQualificationTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetQualificationType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetQualificationTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getQualificationTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListAssignmentsForHIT
operation retrieves completed assignments for a HIT. You can use this
* operation to retrieve the results for a HIT.
*
*
* You can get assignments for a HIT at any time, even if the HIT is not yet Reviewable. If a HIT requested multiple
* assignments, and has received some results but has not yet become Reviewable, you can still retrieve the partial
* results with this operation.
*
*
* Use the AssignmentStatus parameter to control which set of assignments for a HIT are returned. The
* ListAssignmentsForHIT operation can return submitted assignments awaiting approval, or it can return assignments
* that have already been approved or rejected. You can set AssignmentStatus=Approved,Rejected to get assignments
* that have already been approved and rejected together in one result set.
*
*
* Only the Requester who created the HIT can retrieve the assignments for that HIT.
*
*
* Results are sorted and divided into numbered pages and the operation returns a single page of results. You can
* use the parameters of the operation to control sorting and pagination.
*
*
* @param listAssignmentsForHitRequest
* @return A Java Future containing the result of the ListAssignmentsForHIT operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListAssignmentsForHIT
* @see AWS API Documentation
*/
@Override
public CompletableFuture listAssignmentsForHIT(
ListAssignmentsForHitRequest listAssignmentsForHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssignmentsForHitRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssignmentsForHitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssignmentsForHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAssignmentsForHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAssignmentsForHIT").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAssignmentsForHitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAssignmentsForHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListBonusPayments
operation retrieves the amounts of bonuses you have paid to Workers for a
* given HIT or assignment.
*
*
* @param listBonusPaymentsRequest
* @return A Java Future containing the result of the ListBonusPayments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListBonusPayments
* @see AWS API Documentation
*/
@Override
public CompletableFuture listBonusPayments(ListBonusPaymentsRequest listBonusPaymentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listBonusPaymentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listBonusPaymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListBonusPayments");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListBonusPaymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListBonusPayments").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListBonusPaymentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listBonusPaymentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListHITs
operation returns all of a Requester's HITs. The operation returns HITs of any status,
* except for HITs that have been deleted of with the DeleteHIT operation or that have been auto-deleted.
*
*
* @param listHiTsRequest
* @return A Java Future containing the result of the ListHITs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListHITs
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listHITs(ListHiTsRequest listHiTsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listHiTsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listHiTsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListHITs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListHiTsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListHITs")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListHiTsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(listHiTsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListHITsForQualificationType
operation returns the HITs that use the given Qualification type
* for a Qualification requirement. The operation returns HITs of any status, except for HITs that have been deleted
* with the DeleteHIT
operation or that have been auto-deleted.
*
*
* @param listHiTsForQualificationTypeRequest
* @return A Java Future containing the result of the ListHITsForQualificationType operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListHITsForQualificationType
* @see AWS API Documentation
*/
@Override
public CompletableFuture listHITsForQualificationType(
ListHiTsForQualificationTypeRequest listHiTsForQualificationTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listHiTsForQualificationTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listHiTsForQualificationTypeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListHITsForQualificationType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListHiTsForQualificationTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListHITsForQualificationType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListHiTsForQualificationTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listHiTsForQualificationTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListQualificationRequests
operation retrieves requests for Qualifications of a particular
* Qualification type. The owner of the Qualification type calls this operation to poll for pending requests, and
* accepts them using the AcceptQualification operation.
*
*
* @param listQualificationRequestsRequest
* @return A Java Future containing the result of the ListQualificationRequests operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListQualificationRequests
* @see AWS API Documentation
*/
@Override
public CompletableFuture listQualificationRequests(
ListQualificationRequestsRequest listQualificationRequestsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listQualificationRequestsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listQualificationRequestsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListQualificationRequests");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListQualificationRequestsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListQualificationRequests").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListQualificationRequestsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listQualificationRequestsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListQualificationTypes
operation returns a list of Qualification types, filtered by an optional
* search term.
*
*
* @param listQualificationTypesRequest
* @return A Java Future containing the result of the ListQualificationTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListQualificationTypes
* @see AWS API Documentation
*/
@Override
public CompletableFuture listQualificationTypes(
ListQualificationTypesRequest listQualificationTypesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listQualificationTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listQualificationTypesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListQualificationTypes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListQualificationTypesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListQualificationTypes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListQualificationTypesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listQualificationTypesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListReviewPolicyResultsForHIT
operation retrieves the computed results and the actions taken in
* the course of executing your Review Policies for a given HIT. For information about how to specify Review
* Policies when you call CreateHIT, see Review Policies. The ListReviewPolicyResultsForHIT operation can return
* results for both Assignment-level and HIT-level review results.
*
*
* @param listReviewPolicyResultsForHitRequest
* @return A Java Future containing the result of the ListReviewPolicyResultsForHIT operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListReviewPolicyResultsForHIT
* @see AWS API Documentation
*/
@Override
public CompletableFuture listReviewPolicyResultsForHIT(
ListReviewPolicyResultsForHitRequest listReviewPolicyResultsForHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listReviewPolicyResultsForHitRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listReviewPolicyResultsForHitRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReviewPolicyResultsForHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListReviewPolicyResultsForHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReviewPolicyResultsForHIT").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListReviewPolicyResultsForHitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listReviewPolicyResultsForHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListReviewableHITs
operation retrieves the HITs with Status equal to Reviewable or Status equal
* to Reviewing that belong to the Requester calling the operation.
*
*
* @param listReviewableHiTsRequest
* @return A Java Future containing the result of the ListReviewableHITs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListReviewableHITs
* @see AWS API Documentation
*/
@Override
public CompletableFuture listReviewableHITs(ListReviewableHiTsRequest listReviewableHiTsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listReviewableHiTsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listReviewableHiTsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListReviewableHITs");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListReviewableHiTsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListReviewableHITs").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListReviewableHiTsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listReviewableHiTsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListWorkersBlocks
operation retrieves a list of Workers who are blocked from working on your
* HITs.
*
*
* @param listWorkerBlocksRequest
* @return A Java Future containing the result of the ListWorkerBlocks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListWorkerBlocks
* @see AWS API Documentation
*/
@Override
public CompletableFuture listWorkerBlocks(ListWorkerBlocksRequest listWorkerBlocksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listWorkerBlocksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listWorkerBlocksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListWorkerBlocks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListWorkerBlocksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListWorkerBlocks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListWorkerBlocksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listWorkerBlocksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The ListWorkersWithQualificationType
operation returns all of the Workers that have been associated
* with a given Qualification type.
*
*
* @param listWorkersWithQualificationTypeRequest
* @return A Java Future containing the result of the ListWorkersWithQualificationType operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.ListWorkersWithQualificationType
* @see AWS API Documentation
*/
@Override
public CompletableFuture listWorkersWithQualificationType(
ListWorkersWithQualificationTypeRequest listWorkersWithQualificationTypeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listWorkersWithQualificationTypeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listWorkersWithQualificationTypeRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListWorkersWithQualificationType");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListWorkersWithQualificationTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListWorkersWithQualificationType").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListWorkersWithQualificationTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listWorkersWithQualificationTypeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The NotifyWorkers
operation sends an email to one or more Workers that you specify with the Worker
* ID. You can specify up to 100 Worker IDs to send the same message with a single call to the NotifyWorkers
* operation. The NotifyWorkers operation will send a notification email to a Worker only if you have previously
* approved or rejected work from the Worker.
*
*
* @param notifyWorkersRequest
* @return A Java Future containing the result of the NotifyWorkers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.NotifyWorkers
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture notifyWorkers(NotifyWorkersRequest notifyWorkersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(notifyWorkersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, notifyWorkersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "NotifyWorkers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
NotifyWorkersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("NotifyWorkers").withProtocolMetadata(protocolMetadata)
.withMarshaller(new NotifyWorkersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(notifyWorkersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The RejectAssignment
operation rejects the results of a completed assignment.
*
*
* You can include an optional feedback message with the rejection, which the Worker can see in the Status section
* of the web site. When you include a feedback message with the rejection, it helps the Worker understand why the
* assignment was rejected, and can improve the quality of the results the Worker submits in the future.
*
*
* Only the Requester who created the HIT can reject an assignment for the HIT.
*
*
* @param rejectAssignmentRequest
* @return A Java Future containing the result of the RejectAssignment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.RejectAssignment
* @see AWS API Documentation
*/
@Override
public CompletableFuture rejectAssignment(RejectAssignmentRequest rejectAssignmentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(rejectAssignmentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rejectAssignmentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RejectAssignment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RejectAssignmentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RejectAssignment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RejectAssignmentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(rejectAssignmentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The RejectQualificationRequest
operation rejects a user's request for a Qualification.
*
*
* You can provide a text message explaining why the request was rejected. The Worker who made the request can see
* this message.
*
*
* @param rejectQualificationRequestRequest
* @return A Java Future containing the result of the RejectQualificationRequest operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.RejectQualificationRequest
* @see AWS API Documentation
*/
@Override
public CompletableFuture rejectQualificationRequest(
RejectQualificationRequestRequest rejectQualificationRequestRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(rejectQualificationRequestRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rejectQualificationRequestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RejectQualificationRequest");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RejectQualificationRequestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RejectQualificationRequest").withProtocolMetadata(protocolMetadata)
.withMarshaller(new RejectQualificationRequestRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(rejectQualificationRequestRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The SendBonus
operation issues a payment of money from your account to a Worker. This payment
* happens separately from the reward you pay to the Worker when you approve the Worker's assignment. The SendBonus
* operation requires the Worker's ID and the assignment ID as parameters to initiate payment of the bonus. You must
* include a message that explains the reason for the bonus payment, as the Worker may not be expecting the payment.
* Amazon Mechanical Turk collects a fee for bonus payments, similar to the HIT listing fee. This operation fails if
* your account does not have enough funds to pay for both the bonus and the fees.
*
*
* @param sendBonusRequest
* @return A Java Future containing the result of the SendBonus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.SendBonus
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture sendBonus(SendBonusRequest sendBonusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendBonusRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendBonusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendBonus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SendBonusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("SendBonus")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new SendBonusRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(sendBonusRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The SendTestEventNotification
operation causes Amazon Mechanical Turk to send a notification message
* as if a HIT event occurred, according to the provided notification specification. This allows you to test
* notifications without setting up notifications for a real HIT type and trying to trigger them using the website.
* When you call this operation, the service attempts to send the test notification immediately.
*
*
* @param sendTestEventNotificationRequest
* @return A Java Future containing the result of the SendTestEventNotification operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.SendTestEventNotification
* @see AWS API Documentation
*/
@Override
public CompletableFuture sendTestEventNotification(
SendTestEventNotificationRequest sendTestEventNotificationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendTestEventNotificationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendTestEventNotificationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendTestEventNotification");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SendTestEventNotificationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SendTestEventNotification").withProtocolMetadata(protocolMetadata)
.withMarshaller(new SendTestEventNotificationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(sendTestEventNotificationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The UpdateExpirationForHIT
operation allows you update the expiration time of a HIT. If you update
* it to a time in the past, the HIT will be immediately expired.
*
*
* @param updateExpirationForHitRequest
* @return A Java Future containing the result of the UpdateExpirationForHIT operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.UpdateExpirationForHIT
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateExpirationForHIT(
UpdateExpirationForHitRequest updateExpirationForHitRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateExpirationForHitRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateExpirationForHitRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateExpirationForHIT");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateExpirationForHitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateExpirationForHIT").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateExpirationForHitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateExpirationForHitRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The UpdateHITReviewStatus
operation updates the status of a HIT. If the status is Reviewable, this
* operation can update the status to Reviewing, or it can revert a Reviewing HIT back to the Reviewable status.
*
*
* @param updateHitReviewStatusRequest
* @return A Java Future containing the result of the UpdateHITReviewStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ServiceException Amazon Mechanical Turk is temporarily unable to process your request. Try your call
* again.
* - RequestErrorException Your request is invalid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - MTurkException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample MTurkAsyncClient.UpdateHITReviewStatus
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateHITReviewStatus(
UpdateHitReviewStatusRequest updateHitReviewStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateHitReviewStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateHitReviewStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "MTurk");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateHITReviewStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateHitReviewStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams