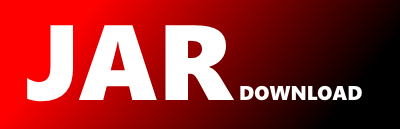
software.amazon.awssdk.services.mturk.model.CreateHitRequest Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateHitRequest extends MTurkRequest implements ToCopyableBuilder {
private static final SdkField MAX_ASSIGNMENTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxAssignments").getter(getter(CreateHitRequest::maxAssignments))
.setter(setter(Builder::maxAssignments))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxAssignments").build()).build();
private static final SdkField AUTO_APPROVAL_DELAY_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("AutoApprovalDelayInSeconds")
.getter(getter(CreateHitRequest::autoApprovalDelayInSeconds))
.setter(setter(Builder::autoApprovalDelayInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoApprovalDelayInSeconds").build())
.build();
private static final SdkField LIFETIME_IN_SECONDS_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("LifetimeInSeconds").getter(getter(CreateHitRequest::lifetimeInSeconds))
.setter(setter(Builder::lifetimeInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LifetimeInSeconds").build()).build();
private static final SdkField ASSIGNMENT_DURATION_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("AssignmentDurationInSeconds")
.getter(getter(CreateHitRequest::assignmentDurationInSeconds))
.setter(setter(Builder::assignmentDurationInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssignmentDurationInSeconds")
.build()).build();
private static final SdkField REWARD_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Reward")
.getter(getter(CreateHitRequest::reward)).setter(setter(Builder::reward))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Reward").build()).build();
private static final SdkField TITLE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Title")
.getter(getter(CreateHitRequest::title)).setter(setter(Builder::title))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Title").build()).build();
private static final SdkField KEYWORDS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Keywords").getter(getter(CreateHitRequest::keywords)).setter(setter(Builder::keywords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Keywords").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateHitRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField QUESTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Question").getter(getter(CreateHitRequest::question)).setter(setter(Builder::question))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Question").build()).build();
private static final SdkField REQUESTER_ANNOTATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RequesterAnnotation").getter(getter(CreateHitRequest::requesterAnnotation))
.setter(setter(Builder::requesterAnnotation))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RequesterAnnotation").build())
.build();
private static final SdkField> QUALIFICATION_REQUIREMENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("QualificationRequirements")
.getter(getter(CreateHitRequest::qualificationRequirements))
.setter(setter(Builder::qualificationRequirements))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QualificationRequirements").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(QualificationRequirement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField UNIQUE_REQUEST_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UniqueRequestToken").getter(getter(CreateHitRequest::uniqueRequestToken))
.setter(setter(Builder::uniqueRequestToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UniqueRequestToken").build())
.build();
private static final SdkField ASSIGNMENT_REVIEW_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("AssignmentReviewPolicy")
.getter(getter(CreateHitRequest::assignmentReviewPolicy)).setter(setter(Builder::assignmentReviewPolicy))
.constructor(ReviewPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssignmentReviewPolicy").build())
.build();
private static final SdkField HIT_REVIEW_POLICY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("HITReviewPolicy")
.getter(getter(CreateHitRequest::hitReviewPolicy)).setter(setter(Builder::hitReviewPolicy))
.constructor(ReviewPolicy::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HITReviewPolicy").build()).build();
private static final SdkField HIT_LAYOUT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HITLayoutId").getter(getter(CreateHitRequest::hitLayoutId)).setter(setter(Builder::hitLayoutId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HITLayoutId").build()).build();
private static final SdkField> HIT_LAYOUT_PARAMETERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("HITLayoutParameters")
.getter(getter(CreateHitRequest::hitLayoutParameters))
.setter(setter(Builder::hitLayoutParameters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HITLayoutParameters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(HITLayoutParameter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MAX_ASSIGNMENTS_FIELD,
AUTO_APPROVAL_DELAY_IN_SECONDS_FIELD, LIFETIME_IN_SECONDS_FIELD, ASSIGNMENT_DURATION_IN_SECONDS_FIELD, REWARD_FIELD,
TITLE_FIELD, KEYWORDS_FIELD, DESCRIPTION_FIELD, QUESTION_FIELD, REQUESTER_ANNOTATION_FIELD,
QUALIFICATION_REQUIREMENTS_FIELD, UNIQUE_REQUEST_TOKEN_FIELD, ASSIGNMENT_REVIEW_POLICY_FIELD,
HIT_REVIEW_POLICY_FIELD, HIT_LAYOUT_ID_FIELD, HIT_LAYOUT_PARAMETERS_FIELD));
private final Integer maxAssignments;
private final Long autoApprovalDelayInSeconds;
private final Long lifetimeInSeconds;
private final Long assignmentDurationInSeconds;
private final String reward;
private final String title;
private final String keywords;
private final String description;
private final String question;
private final String requesterAnnotation;
private final List qualificationRequirements;
private final String uniqueRequestToken;
private final ReviewPolicy assignmentReviewPolicy;
private final ReviewPolicy hitReviewPolicy;
private final String hitLayoutId;
private final List hitLayoutParameters;
private CreateHitRequest(BuilderImpl builder) {
super(builder);
this.maxAssignments = builder.maxAssignments;
this.autoApprovalDelayInSeconds = builder.autoApprovalDelayInSeconds;
this.lifetimeInSeconds = builder.lifetimeInSeconds;
this.assignmentDurationInSeconds = builder.assignmentDurationInSeconds;
this.reward = builder.reward;
this.title = builder.title;
this.keywords = builder.keywords;
this.description = builder.description;
this.question = builder.question;
this.requesterAnnotation = builder.requesterAnnotation;
this.qualificationRequirements = builder.qualificationRequirements;
this.uniqueRequestToken = builder.uniqueRequestToken;
this.assignmentReviewPolicy = builder.assignmentReviewPolicy;
this.hitReviewPolicy = builder.hitReviewPolicy;
this.hitLayoutId = builder.hitLayoutId;
this.hitLayoutParameters = builder.hitLayoutParameters;
}
/**
*
* The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*
*
* @return The number of times the HIT can be accepted and completed before the HIT becomes unavailable.
*/
public final Integer maxAssignments() {
return maxAssignments;
}
/**
*
* The number of seconds after an assignment for the HIT has been submitted, after which the assignment is
* considered Approved automatically unless the Requester explicitly rejects it.
*
*
* @return The number of seconds after an assignment for the HIT has been submitted, after which the assignment is
* considered Approved automatically unless the Requester explicitly rejects it.
*/
public final Long autoApprovalDelayInSeconds() {
return autoApprovalDelayInSeconds;
}
/**
*
* An amount of time, in seconds, after which the HIT is no longer available for users to accept. After the lifetime
* of the HIT elapses, the HIT no longer appears in HIT searches, even if not all of the assignments for the HIT
* have been accepted.
*
*
* @return An amount of time, in seconds, after which the HIT is no longer available for users to accept. After the
* lifetime of the HIT elapses, the HIT no longer appears in HIT searches, even if not all of the
* assignments for the HIT have been accepted.
*/
public final Long lifetimeInSeconds() {
return lifetimeInSeconds;
}
/**
*
* The amount of time, in seconds, that a Worker has to complete the HIT after accepting it. If a Worker does not
* complete the assignment within the specified duration, the assignment is considered abandoned. If the HIT is
* still active (that is, its lifetime has not elapsed), the assignment becomes available for other users to find
* and accept.
*
*
* @return The amount of time, in seconds, that a Worker has to complete the HIT after accepting it. If a Worker
* does not complete the assignment within the specified duration, the assignment is considered abandoned.
* If the HIT is still active (that is, its lifetime has not elapsed), the assignment becomes available for
* other users to find and accept.
*/
public final Long assignmentDurationInSeconds() {
return assignmentDurationInSeconds;
}
/**
*
* The amount of money the Requester will pay a Worker for successfully completing the HIT.
*
*
* @return The amount of money the Requester will pay a Worker for successfully completing the HIT.
*/
public final String reward() {
return reward;
}
/**
*
* The title of the HIT. A title should be short and descriptive about the kind of task the HIT contains. On the
* Amazon Mechanical Turk web site, the HIT title appears in search results, and everywhere the HIT is mentioned.
*
*
* @return The title of the HIT. A title should be short and descriptive about the kind of task the HIT contains. On
* the Amazon Mechanical Turk web site, the HIT title appears in search results, and everywhere the HIT is
* mentioned.
*/
public final String title() {
return title;
}
/**
*
* One or more words or phrases that describe the HIT, separated by commas. These words are used in searches to find
* HITs.
*
*
* @return One or more words or phrases that describe the HIT, separated by commas. These words are used in searches
* to find HITs.
*/
public final String keywords() {
return keywords;
}
/**
*
* A general description of the HIT. A description includes detailed information about the kind of task the HIT
* contains. On the Amazon Mechanical Turk web site, the HIT description appears in the expanded view of search
* results, and in the HIT and assignment screens. A good description gives the user enough information to evaluate
* the HIT before accepting it.
*
*
* @return A general description of the HIT. A description includes detailed information about the kind of task the
* HIT contains. On the Amazon Mechanical Turk web site, the HIT description appears in the expanded view of
* search results, and in the HIT and assignment screens. A good description gives the user enough
* information to evaluate the HIT before accepting it.
*/
public final String description() {
return description;
}
/**
*
* The data the person completing the HIT uses to produce the results.
*
*
* Constraints: Must be a QuestionForm data structure, an ExternalQuestion data structure, or an HTMLQuestion data
* structure. The XML question data must not be larger than 64 kilobytes (65,535 bytes) in size, including
* whitespace.
*
*
* Either a Question parameter or a HITLayoutId parameter must be provided.
*
*
* @return The data the person completing the HIT uses to produce the results.
*
* Constraints: Must be a QuestionForm data structure, an ExternalQuestion data structure, or an
* HTMLQuestion data structure. The XML question data must not be larger than 64 kilobytes (65,535 bytes) in
* size, including whitespace.
*
*
* Either a Question parameter or a HITLayoutId parameter must be provided.
*/
public final String question() {
return question;
}
/**
*
* An arbitrary data field. The RequesterAnnotation parameter lets your application attach arbitrary data to the HIT
* for tracking purposes. For example, this parameter could be an identifier internal to the Requester's application
* that corresponds with the HIT.
*
*
* The RequesterAnnotation parameter for a HIT is only visible to the Requester who created the HIT. It is not shown
* to the Worker, or any other Requester.
*
*
* The RequesterAnnotation parameter may be different for each HIT you submit. It does not affect how your HITs are
* grouped.
*
*
* @return An arbitrary data field. The RequesterAnnotation parameter lets your application attach arbitrary data to
* the HIT for tracking purposes. For example, this parameter could be an identifier internal to the
* Requester's application that corresponds with the HIT.
*
* The RequesterAnnotation parameter for a HIT is only visible to the Requester who created the HIT. It is
* not shown to the Worker, or any other Requester.
*
*
* The RequesterAnnotation parameter may be different for each HIT you submit. It does not affect how your
* HITs are grouped.
*/
public final String requesterAnnotation() {
return requesterAnnotation;
}
/**
* For responses, this returns true if the service returned a value for the QualificationRequirements property. This
* DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the
* property). This is useful because the SDK will never return a null collection or map, but you may need to
* differentiate between the service returning nothing (or null) and the service returning an empty collection or
* map. For requests, this returns true if a value for the property was specified in the request builder, and false
* if a value was not specified.
*/
public final boolean hasQualificationRequirements() {
return qualificationRequirements != null && !(qualificationRequirements instanceof SdkAutoConstructList);
}
/**
*
* Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between zero and
* ten Qualification requirements. All requirements must be met in order for a Worker to accept the HIT.
* Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasQualificationRequirements} method.
*
*
* @return Conditions that a Worker's Qualifications must meet in order to accept the HIT. A HIT can have between
* zero and ten Qualification requirements. All requirements must be met in order for a Worker to accept the
* HIT. Additionally, other actions can be restricted using the ActionsGuarded
field on each
* QualificationRequirement
structure.
*/
public final List qualificationRequirements() {
return qualificationRequirements;
}
/**
*
* A unique identifier for this request which allows you to retry the call on error without creating duplicate HITs.
* This is useful in cases such as network timeouts where it is unclear whether or not the call succeeded on the
* server. If the HIT already exists in the system from a previous call using the same UniqueRequestToken,
* subsequent calls will return a AWS.MechanicalTurk.HitAlreadyExists error with a message containing the HITId.
*
*
*
* Note: It is your responsibility to ensure uniqueness of the token. The unique token expires after 24 hours.
* Subsequent calls using the same UniqueRequestToken made after the 24 hour limit could create duplicate HITs.
*
*
*
* @return A unique identifier for this request which allows you to retry the call on error without creating
* duplicate HITs. This is useful in cases such as network timeouts where it is unclear whether or not the
* call succeeded on the server. If the HIT already exists in the system from a previous call using the same
* UniqueRequestToken, subsequent calls will return a AWS.MechanicalTurk.HitAlreadyExists error with a
* message containing the HITId.
*
* Note: It is your responsibility to ensure uniqueness of the token. The unique token expires after 24
* hours. Subsequent calls using the same UniqueRequestToken made after the 24 hour limit could create
* duplicate HITs.
*
*/
public final String uniqueRequestToken() {
return uniqueRequestToken;
}
/**
*
* The Assignment-level Review Policy applies to the assignments under the HIT. You can specify for Mechanical Turk
* to take various actions based on the policy.
*
*
* @return The Assignment-level Review Policy applies to the assignments under the HIT. You can specify for
* Mechanical Turk to take various actions based on the policy.
*/
public final ReviewPolicy assignmentReviewPolicy() {
return assignmentReviewPolicy;
}
/**
*
* The HIT-level Review Policy applies to the HIT. You can specify for Mechanical Turk to take various actions based
* on the policy.
*
*
* @return The HIT-level Review Policy applies to the HIT. You can specify for Mechanical Turk to take various
* actions based on the policy.
*/
public final ReviewPolicy hitReviewPolicy() {
return hitReviewPolicy;
}
/**
*
* The HITLayoutId allows you to use a pre-existing HIT design with placeholder values and create an additional HIT
* by providing those values as HITLayoutParameters.
*
*
* Constraints: Either a Question parameter or a HITLayoutId parameter must be provided.
*
*
* @return The HITLayoutId allows you to use a pre-existing HIT design with placeholder values and create an
* additional HIT by providing those values as HITLayoutParameters.
*
* Constraints: Either a Question parameter or a HITLayoutId parameter must be provided.
*/
public final String hitLayoutId() {
return hitLayoutId;
}
/**
* For responses, this returns true if the service returned a value for the HITLayoutParameters property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasHitLayoutParameters() {
return hitLayoutParameters != null && !(hitLayoutParameters instanceof SdkAutoConstructList);
}
/**
*
* If the HITLayoutId is provided, any placeholder values must be filled in with values using the HITLayoutParameter
* structure. For more information, see HITLayout.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasHitLayoutParameters} method.
*
*
* @return If the HITLayoutId is provided, any placeholder values must be filled in with values using the
* HITLayoutParameter structure. For more information, see HITLayout.
*/
public final List hitLayoutParameters() {
return hitLayoutParameters;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(maxAssignments());
hashCode = 31 * hashCode + Objects.hashCode(autoApprovalDelayInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(lifetimeInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(assignmentDurationInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(reward());
hashCode = 31 * hashCode + Objects.hashCode(title());
hashCode = 31 * hashCode + Objects.hashCode(keywords());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(question());
hashCode = 31 * hashCode + Objects.hashCode(requesterAnnotation());
hashCode = 31 * hashCode + Objects.hashCode(hasQualificationRequirements() ? qualificationRequirements() : null);
hashCode = 31 * hashCode + Objects.hashCode(uniqueRequestToken());
hashCode = 31 * hashCode + Objects.hashCode(assignmentReviewPolicy());
hashCode = 31 * hashCode + Objects.hashCode(hitReviewPolicy());
hashCode = 31 * hashCode + Objects.hashCode(hitLayoutId());
hashCode = 31 * hashCode + Objects.hashCode(hasHitLayoutParameters() ? hitLayoutParameters() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateHitRequest)) {
return false;
}
CreateHitRequest other = (CreateHitRequest) obj;
return Objects.equals(maxAssignments(), other.maxAssignments())
&& Objects.equals(autoApprovalDelayInSeconds(), other.autoApprovalDelayInSeconds())
&& Objects.equals(lifetimeInSeconds(), other.lifetimeInSeconds())
&& Objects.equals(assignmentDurationInSeconds(), other.assignmentDurationInSeconds())
&& Objects.equals(reward(), other.reward()) && Objects.equals(title(), other.title())
&& Objects.equals(keywords(), other.keywords()) && Objects.equals(description(), other.description())
&& Objects.equals(question(), other.question())
&& Objects.equals(requesterAnnotation(), other.requesterAnnotation())
&& hasQualificationRequirements() == other.hasQualificationRequirements()
&& Objects.equals(qualificationRequirements(), other.qualificationRequirements())
&& Objects.equals(uniqueRequestToken(), other.uniqueRequestToken())
&& Objects.equals(assignmentReviewPolicy(), other.assignmentReviewPolicy())
&& Objects.equals(hitReviewPolicy(), other.hitReviewPolicy())
&& Objects.equals(hitLayoutId(), other.hitLayoutId())
&& hasHitLayoutParameters() == other.hasHitLayoutParameters()
&& Objects.equals(hitLayoutParameters(), other.hitLayoutParameters());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateHitRequest").add("MaxAssignments", maxAssignments())
.add("AutoApprovalDelayInSeconds", autoApprovalDelayInSeconds()).add("LifetimeInSeconds", lifetimeInSeconds())
.add("AssignmentDurationInSeconds", assignmentDurationInSeconds()).add("Reward", reward()).add("Title", title())
.add("Keywords", keywords()).add("Description", description()).add("Question", question())
.add("RequesterAnnotation", requesterAnnotation())
.add("QualificationRequirements", hasQualificationRequirements() ? qualificationRequirements() : null)
.add("UniqueRequestToken", uniqueRequestToken()).add("AssignmentReviewPolicy", assignmentReviewPolicy())
.add("HITReviewPolicy", hitReviewPolicy()).add("HITLayoutId", hitLayoutId())
.add("HITLayoutParameters", hasHitLayoutParameters() ? hitLayoutParameters() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MaxAssignments":
return Optional.ofNullable(clazz.cast(maxAssignments()));
case "AutoApprovalDelayInSeconds":
return Optional.ofNullable(clazz.cast(autoApprovalDelayInSeconds()));
case "LifetimeInSeconds":
return Optional.ofNullable(clazz.cast(lifetimeInSeconds()));
case "AssignmentDurationInSeconds":
return Optional.ofNullable(clazz.cast(assignmentDurationInSeconds()));
case "Reward":
return Optional.ofNullable(clazz.cast(reward()));
case "Title":
return Optional.ofNullable(clazz.cast(title()));
case "Keywords":
return Optional.ofNullable(clazz.cast(keywords()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Question":
return Optional.ofNullable(clazz.cast(question()));
case "RequesterAnnotation":
return Optional.ofNullable(clazz.cast(requesterAnnotation()));
case "QualificationRequirements":
return Optional.ofNullable(clazz.cast(qualificationRequirements()));
case "UniqueRequestToken":
return Optional.ofNullable(clazz.cast(uniqueRequestToken()));
case "AssignmentReviewPolicy":
return Optional.ofNullable(clazz.cast(assignmentReviewPolicy()));
case "HITReviewPolicy":
return Optional.ofNullable(clazz.cast(hitReviewPolicy()));
case "HITLayoutId":
return Optional.ofNullable(clazz.cast(hitLayoutId()));
case "HITLayoutParameters":
return Optional.ofNullable(clazz.cast(hitLayoutParameters()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function