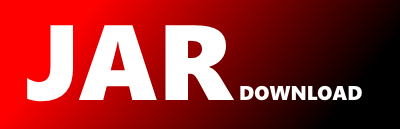
software.amazon.awssdk.services.mturk.model.NotifyWorkersFailureStatus Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* When MTurk encounters an issue with notifying the Workers you specified, it returns back this object with failure
* details.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NotifyWorkersFailureStatus implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField NOTIFY_WORKERS_FAILURE_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NotifyWorkersFailureCode").getter(getter(NotifyWorkersFailureStatus::notifyWorkersFailureCodeAsString))
.setter(setter(Builder::notifyWorkersFailureCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotifyWorkersFailureCode").build())
.build();
private static final SdkField NOTIFY_WORKERS_FAILURE_MESSAGE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("NotifyWorkersFailureMessage")
.getter(getter(NotifyWorkersFailureStatus::notifyWorkersFailureMessage))
.setter(setter(Builder::notifyWorkersFailureMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NotifyWorkersFailureMessage")
.build()).build();
private static final SdkField WORKER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkerId").getter(getter(NotifyWorkersFailureStatus::workerId)).setter(setter(Builder::workerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkerId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
NOTIFY_WORKERS_FAILURE_CODE_FIELD, NOTIFY_WORKERS_FAILURE_MESSAGE_FIELD, WORKER_ID_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String notifyWorkersFailureCode;
private final String notifyWorkersFailureMessage;
private final String workerId;
private NotifyWorkersFailureStatus(BuilderImpl builder) {
this.notifyWorkersFailureCode = builder.notifyWorkersFailureCode;
this.notifyWorkersFailureMessage = builder.notifyWorkersFailureMessage;
this.workerId = builder.workerId;
}
/**
*
* Encoded value for the failure type.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #notifyWorkersFailureCode} will return {@link NotifyWorkersFailureCode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #notifyWorkersFailureCodeAsString}.
*
*
* @return Encoded value for the failure type.
* @see NotifyWorkersFailureCode
*/
public final NotifyWorkersFailureCode notifyWorkersFailureCode() {
return NotifyWorkersFailureCode.fromValue(notifyWorkersFailureCode);
}
/**
*
* Encoded value for the failure type.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #notifyWorkersFailureCode} will return {@link NotifyWorkersFailureCode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #notifyWorkersFailureCodeAsString}.
*
*
* @return Encoded value for the failure type.
* @see NotifyWorkersFailureCode
*/
public final String notifyWorkersFailureCodeAsString() {
return notifyWorkersFailureCode;
}
/**
*
* A message detailing the reason the Worker could not be notified.
*
*
* @return A message detailing the reason the Worker could not be notified.
*/
public final String notifyWorkersFailureMessage() {
return notifyWorkersFailureMessage;
}
/**
*
* The ID of the Worker.
*
*
* @return The ID of the Worker.
*/
public final String workerId() {
return workerId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(notifyWorkersFailureCodeAsString());
hashCode = 31 * hashCode + Objects.hashCode(notifyWorkersFailureMessage());
hashCode = 31 * hashCode + Objects.hashCode(workerId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NotifyWorkersFailureStatus)) {
return false;
}
NotifyWorkersFailureStatus other = (NotifyWorkersFailureStatus) obj;
return Objects.equals(notifyWorkersFailureCodeAsString(), other.notifyWorkersFailureCodeAsString())
&& Objects.equals(notifyWorkersFailureMessage(), other.notifyWorkersFailureMessage())
&& Objects.equals(workerId(), other.workerId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NotifyWorkersFailureStatus").add("NotifyWorkersFailureCode", notifyWorkersFailureCodeAsString())
.add("NotifyWorkersFailureMessage", notifyWorkersFailureMessage()).add("WorkerId", workerId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "NotifyWorkersFailureCode":
return Optional.ofNullable(clazz.cast(notifyWorkersFailureCodeAsString()));
case "NotifyWorkersFailureMessage":
return Optional.ofNullable(clazz.cast(notifyWorkersFailureMessage()));
case "WorkerId":
return Optional.ofNullable(clazz.cast(workerId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("NotifyWorkersFailureCode", NOTIFY_WORKERS_FAILURE_CODE_FIELD);
map.put("NotifyWorkersFailureMessage", NOTIFY_WORKERS_FAILURE_MESSAGE_FIELD);
map.put("WorkerId", WORKER_ID_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function