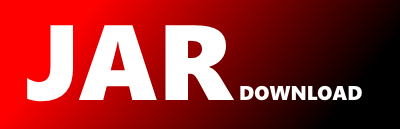
software.amazon.awssdk.services.mturk.model.Qualification Maven / Gradle / Ivy
Show all versions of mturk Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.mturk.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Qualification data structure represents a Qualification assigned to a user, including the Qualification type and
* the value (score).
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Qualification implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField QUALIFICATION_TYPE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("QualificationTypeId").getter(getter(Qualification::qualificationTypeId))
.setter(setter(Builder::qualificationTypeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("QualificationTypeId").build())
.build();
private static final SdkField WORKER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("WorkerId").getter(getter(Qualification::workerId)).setter(setter(Builder::workerId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WorkerId").build()).build();
private static final SdkField GRANT_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("GrantTime").getter(getter(Qualification::grantTime)).setter(setter(Builder::grantTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrantTime").build()).build();
private static final SdkField INTEGER_VALUE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("IntegerValue").getter(getter(Qualification::integerValue)).setter(setter(Builder::integerValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IntegerValue").build()).build();
private static final SdkField LOCALE_VALUE_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("LocaleValue").getter(getter(Qualification::localeValue)).setter(setter(Builder::localeValue))
.constructor(Locale::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LocaleValue").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(Qualification::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(QUALIFICATION_TYPE_ID_FIELD,
WORKER_ID_FIELD, GRANT_TIME_FIELD, INTEGER_VALUE_FIELD, LOCALE_VALUE_FIELD, STATUS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String qualificationTypeId;
private final String workerId;
private final Instant grantTime;
private final Integer integerValue;
private final Locale localeValue;
private final String status;
private Qualification(BuilderImpl builder) {
this.qualificationTypeId = builder.qualificationTypeId;
this.workerId = builder.workerId;
this.grantTime = builder.grantTime;
this.integerValue = builder.integerValue;
this.localeValue = builder.localeValue;
this.status = builder.status;
}
/**
*
* The ID of the Qualification type for the Qualification.
*
*
* @return The ID of the Qualification type for the Qualification.
*/
public final String qualificationTypeId() {
return qualificationTypeId;
}
/**
*
* The ID of the Worker who possesses the Qualification.
*
*
* @return The ID of the Worker who possesses the Qualification.
*/
public final String workerId() {
return workerId;
}
/**
*
* The date and time the Qualification was granted to the Worker. If the Worker's Qualification was revoked, and
* then re-granted based on a new Qualification request, GrantTime is the date and time of the last call to the
* AcceptQualificationRequest operation.
*
*
* @return The date and time the Qualification was granted to the Worker. If the Worker's Qualification was revoked,
* and then re-granted based on a new Qualification request, GrantTime is the date and time of the last call
* to the AcceptQualificationRequest operation.
*/
public final Instant grantTime() {
return grantTime;
}
/**
*
* The value (score) of the Qualification, if the Qualification has an integer value.
*
*
* @return The value (score) of the Qualification, if the Qualification has an integer value.
*/
public final Integer integerValue() {
return integerValue;
}
/**
* Returns the value of the LocaleValue property for this object.
*
* @return The value of the LocaleValue property for this object.
*/
public final Locale localeValue() {
return localeValue;
}
/**
*
* The status of the Qualification. Valid values are Granted | Revoked.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link QualificationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the Qualification. Valid values are Granted | Revoked.
* @see QualificationStatus
*/
public final QualificationStatus status() {
return QualificationStatus.fromValue(status);
}
/**
*
* The status of the Qualification. Valid values are Granted | Revoked.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link QualificationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the Qualification. Valid values are Granted | Revoked.
* @see QualificationStatus
*/
public final String statusAsString() {
return status;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(qualificationTypeId());
hashCode = 31 * hashCode + Objects.hashCode(workerId());
hashCode = 31 * hashCode + Objects.hashCode(grantTime());
hashCode = 31 * hashCode + Objects.hashCode(integerValue());
hashCode = 31 * hashCode + Objects.hashCode(localeValue());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Qualification)) {
return false;
}
Qualification other = (Qualification) obj;
return Objects.equals(qualificationTypeId(), other.qualificationTypeId()) && Objects.equals(workerId(), other.workerId())
&& Objects.equals(grantTime(), other.grantTime()) && Objects.equals(integerValue(), other.integerValue())
&& Objects.equals(localeValue(), other.localeValue()) && Objects.equals(statusAsString(), other.statusAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Qualification").add("QualificationTypeId", qualificationTypeId()).add("WorkerId", workerId())
.add("GrantTime", grantTime()).add("IntegerValue", integerValue()).add("LocaleValue", localeValue())
.add("Status", statusAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "QualificationTypeId":
return Optional.ofNullable(clazz.cast(qualificationTypeId()));
case "WorkerId":
return Optional.ofNullable(clazz.cast(workerId()));
case "GrantTime":
return Optional.ofNullable(clazz.cast(grantTime()));
case "IntegerValue":
return Optional.ofNullable(clazz.cast(integerValue()));
case "LocaleValue":
return Optional.ofNullable(clazz.cast(localeValue()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("QualificationTypeId", QUALIFICATION_TYPE_ID_FIELD);
map.put("WorkerId", WORKER_ID_FIELD);
map.put("GrantTime", GRANT_TIME_FIELD);
map.put("IntegerValue", INTEGER_VALUE_FIELD);
map.put("LocaleValue", LOCALE_VALUE_FIELD);
map.put("Status", STATUS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function