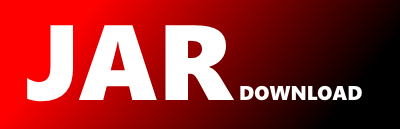
software.amazon.awssdk.services.neptune.model.DBCluster Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the details of an Amazon Neptune DB cluster.
*
*
* This data type is used as a response element in the DescribeDBClusters action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DBCluster implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(DBCluster::allocatedStorage)).setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField> AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AvailabilityZones")
.getter(getter(DBCluster::availabilityZones))
.setter(setter(Builder::availabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName("AvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AvailabilityZone").build()).build()).build()).build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(DBCluster::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField CHARACTER_SET_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CharacterSetName").getter(getter(DBCluster::characterSetName)).setter(setter(Builder::characterSetName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CharacterSetName").build()).build();
private static final SdkField DATABASE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatabaseName").getter(getter(DBCluster::databaseName)).setter(setter(Builder::databaseName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatabaseName").build()).build();
private static final SdkField DB_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterIdentifier").getter(getter(DBCluster::dbClusterIdentifier))
.setter(setter(Builder::dbClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterIdentifier").build())
.build();
private static final SdkField DB_CLUSTER_PARAMETER_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterParameterGroup").getter(getter(DBCluster::dbClusterParameterGroup))
.setter(setter(Builder::dbClusterParameterGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterParameterGroup").build())
.build();
private static final SdkField DB_SUBNET_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBSubnetGroup").getter(getter(DBCluster::dbSubnetGroup)).setter(setter(Builder::dbSubnetGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBSubnetGroup").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DBCluster::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PERCENT_PROGRESS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PercentProgress").getter(getter(DBCluster::percentProgress)).setter(setter(Builder::percentProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentProgress").build()).build();
private static final SdkField EARLIEST_RESTORABLE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EarliestRestorableTime").getter(getter(DBCluster::earliestRestorableTime))
.setter(setter(Builder::earliestRestorableTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EarliestRestorableTime").build())
.build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Endpoint").getter(getter(DBCluster::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Endpoint").build()).build();
private static final SdkField READER_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReaderEndpoint").getter(getter(DBCluster::readerEndpoint)).setter(setter(Builder::readerEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReaderEndpoint").build()).build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZ").getter(getter(DBCluster::multiAZ)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(DBCluster::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(DBCluster::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField LATEST_RESTORABLE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LatestRestorableTime").getter(getter(DBCluster::latestRestorableTime))
.setter(setter(Builder::latestRestorableTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LatestRestorableTime").build())
.build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(DBCluster::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField MASTER_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUsername").getter(getter(DBCluster::masterUsername)).setter(setter(Builder::masterUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUsername").build()).build();
private static final SdkField> DB_CLUSTER_OPTION_GROUP_MEMBERSHIPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DBClusterOptionGroupMemberships")
.getter(getter(DBCluster::dbClusterOptionGroupMemberships))
.setter(setter(Builder::dbClusterOptionGroupMemberships))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterOptionGroupMemberships")
.build(),
ListTrait
.builder()
.memberLocationName("DBClusterOptionGroup")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DBClusterOptionGroupStatus::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBClusterOptionGroup").build()).build()).build()).build();
private static final SdkField PREFERRED_BACKUP_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredBackupWindow").getter(getter(DBCluster::preferredBackupWindow))
.setter(setter(Builder::preferredBackupWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredBackupWindow").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(DBCluster::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField REPLICATION_SOURCE_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("ReplicationSourceIdentifier")
.getter(getter(DBCluster::replicationSourceIdentifier))
.setter(setter(Builder::replicationSourceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReplicationSourceIdentifier")
.build()).build();
private static final SdkField> READ_REPLICA_IDENTIFIERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReadReplicaIdentifiers")
.getter(getter(DBCluster::readReplicaIdentifiers))
.setter(setter(Builder::readReplicaIdentifiers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReadReplicaIdentifiers").build(),
ListTrait
.builder()
.memberLocationName("ReadReplicaIdentifier")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("ReadReplicaIdentifier").build()).build()).build()).build();
private static final SdkField> DB_CLUSTER_MEMBERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DBClusterMembers")
.getter(getter(DBCluster::dbClusterMembers))
.setter(setter(Builder::dbClusterMembers))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterMembers").build(),
ListTrait
.builder()
.memberLocationName("DBClusterMember")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DBClusterMember::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBClusterMember").build()).build()).build()).build();
private static final SdkField> VPC_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroups")
.getter(getter(DBCluster::vpcSecurityGroups))
.setter(setter(Builder::vpcSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroupMembership")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(VpcSecurityGroupMembership::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroupMembership").build()).build()).build())
.build();
private static final SdkField HOSTED_ZONE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("HostedZoneId").getter(getter(DBCluster::hostedZoneId)).setter(setter(Builder::hostedZoneId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("HostedZoneId").build()).build();
private static final SdkField STORAGE_ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("StorageEncrypted").getter(getter(DBCluster::storageEncrypted)).setter(setter(Builder::storageEncrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageEncrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(DBCluster::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField DB_CLUSTER_RESOURCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DbClusterResourceId").getter(getter(DBCluster::dbClusterResourceId))
.setter(setter(Builder::dbClusterResourceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DbClusterResourceId").build())
.build();
private static final SdkField DB_CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterArn").getter(getter(DBCluster::dbClusterArn)).setter(setter(Builder::dbClusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterArn").build()).build();
private static final SdkField> ASSOCIATED_ROLES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AssociatedRoles")
.getter(getter(DBCluster::associatedRoles))
.setter(setter(Builder::associatedRoles))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AssociatedRoles").build(),
ListTrait
.builder()
.memberLocationName("DBClusterRole")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DBClusterRole::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBClusterRole").build()).build()).build()).build();
private static final SdkField IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IAMDatabaseAuthenticationEnabled")
.getter(getter(DBCluster::iamDatabaseAuthenticationEnabled))
.setter(setter(Builder::iamDatabaseAuthenticationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IAMDatabaseAuthenticationEnabled")
.build()).build();
private static final SdkField CLONE_GROUP_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CloneGroupId").getter(getter(DBCluster::cloneGroupId)).setter(setter(Builder::cloneGroupId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CloneGroupId").build()).build();
private static final SdkField CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ClusterCreateTime").getter(getter(DBCluster::clusterCreateTime))
.setter(setter(Builder::clusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterCreateTime").build()).build();
private static final SdkField> ENABLED_CLOUDWATCH_LOGS_EXPORTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EnabledCloudwatchLogsExports")
.getter(getter(DBCluster::enabledCloudwatchLogsExports))
.setter(setter(Builder::enabledCloudwatchLogsExports))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnabledCloudwatchLogsExports")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DELETION_PROTECTION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DeletionProtection").getter(getter(DBCluster::deletionProtection))
.setter(setter(Builder::deletionProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeletionProtection").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ALLOCATED_STORAGE_FIELD,
AVAILABILITY_ZONES_FIELD, BACKUP_RETENTION_PERIOD_FIELD, CHARACTER_SET_NAME_FIELD, DATABASE_NAME_FIELD,
DB_CLUSTER_IDENTIFIER_FIELD, DB_CLUSTER_PARAMETER_GROUP_FIELD, DB_SUBNET_GROUP_FIELD, STATUS_FIELD,
PERCENT_PROGRESS_FIELD, EARLIEST_RESTORABLE_TIME_FIELD, ENDPOINT_FIELD, READER_ENDPOINT_FIELD, MULTI_AZ_FIELD,
ENGINE_FIELD, ENGINE_VERSION_FIELD, LATEST_RESTORABLE_TIME_FIELD, PORT_FIELD, MASTER_USERNAME_FIELD,
DB_CLUSTER_OPTION_GROUP_MEMBERSHIPS_FIELD, PREFERRED_BACKUP_WINDOW_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD,
REPLICATION_SOURCE_IDENTIFIER_FIELD, READ_REPLICA_IDENTIFIERS_FIELD, DB_CLUSTER_MEMBERS_FIELD,
VPC_SECURITY_GROUPS_FIELD, HOSTED_ZONE_ID_FIELD, STORAGE_ENCRYPTED_FIELD, KMS_KEY_ID_FIELD,
DB_CLUSTER_RESOURCE_ID_FIELD, DB_CLUSTER_ARN_FIELD, ASSOCIATED_ROLES_FIELD,
IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD, CLONE_GROUP_ID_FIELD, CLUSTER_CREATE_TIME_FIELD,
ENABLED_CLOUDWATCH_LOGS_EXPORTS_FIELD, DELETION_PROTECTION_FIELD));
private static final long serialVersionUID = 1L;
private final Integer allocatedStorage;
private final List availabilityZones;
private final Integer backupRetentionPeriod;
private final String characterSetName;
private final String databaseName;
private final String dbClusterIdentifier;
private final String dbClusterParameterGroup;
private final String dbSubnetGroup;
private final String status;
private final String percentProgress;
private final Instant earliestRestorableTime;
private final String endpoint;
private final String readerEndpoint;
private final Boolean multiAZ;
private final String engine;
private final String engineVersion;
private final Instant latestRestorableTime;
private final Integer port;
private final String masterUsername;
private final List dbClusterOptionGroupMemberships;
private final String preferredBackupWindow;
private final String preferredMaintenanceWindow;
private final String replicationSourceIdentifier;
private final List readReplicaIdentifiers;
private final List dbClusterMembers;
private final List vpcSecurityGroups;
private final String hostedZoneId;
private final Boolean storageEncrypted;
private final String kmsKeyId;
private final String dbClusterResourceId;
private final String dbClusterArn;
private final List associatedRoles;
private final Boolean iamDatabaseAuthenticationEnabled;
private final String cloneGroupId;
private final Instant clusterCreateTime;
private final List enabledCloudwatchLogsExports;
private final Boolean deletionProtection;
private DBCluster(BuilderImpl builder) {
this.allocatedStorage = builder.allocatedStorage;
this.availabilityZones = builder.availabilityZones;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.characterSetName = builder.characterSetName;
this.databaseName = builder.databaseName;
this.dbClusterIdentifier = builder.dbClusterIdentifier;
this.dbClusterParameterGroup = builder.dbClusterParameterGroup;
this.dbSubnetGroup = builder.dbSubnetGroup;
this.status = builder.status;
this.percentProgress = builder.percentProgress;
this.earliestRestorableTime = builder.earliestRestorableTime;
this.endpoint = builder.endpoint;
this.readerEndpoint = builder.readerEndpoint;
this.multiAZ = builder.multiAZ;
this.engine = builder.engine;
this.engineVersion = builder.engineVersion;
this.latestRestorableTime = builder.latestRestorableTime;
this.port = builder.port;
this.masterUsername = builder.masterUsername;
this.dbClusterOptionGroupMemberships = builder.dbClusterOptionGroupMemberships;
this.preferredBackupWindow = builder.preferredBackupWindow;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.replicationSourceIdentifier = builder.replicationSourceIdentifier;
this.readReplicaIdentifiers = builder.readReplicaIdentifiers;
this.dbClusterMembers = builder.dbClusterMembers;
this.vpcSecurityGroups = builder.vpcSecurityGroups;
this.hostedZoneId = builder.hostedZoneId;
this.storageEncrypted = builder.storageEncrypted;
this.kmsKeyId = builder.kmsKeyId;
this.dbClusterResourceId = builder.dbClusterResourceId;
this.dbClusterArn = builder.dbClusterArn;
this.associatedRoles = builder.associatedRoles;
this.iamDatabaseAuthenticationEnabled = builder.iamDatabaseAuthenticationEnabled;
this.cloneGroupId = builder.cloneGroupId;
this.clusterCreateTime = builder.clusterCreateTime;
this.enabledCloudwatchLogsExports = builder.enabledCloudwatchLogsExports;
this.deletionProtection = builder.deletionProtection;
}
/**
*
* AllocatedStorage
always returns 1, because Neptune DB cluster storage size is not fixed, but instead
* automatically adjusts as needed.
*
*
* @return AllocatedStorage
always returns 1, because Neptune DB cluster storage size is not fixed, but
* instead automatically adjusts as needed.
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
* Returns true if the AvailabilityZones property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasAvailabilityZones() {
return availabilityZones != null && !(availabilityZones instanceof SdkAutoConstructList);
}
/**
*
* Provides the list of EC2 Availability Zones that instances in the DB cluster can be created in.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAvailabilityZones()} to see if a value was sent in this field.
*
*
* @return Provides the list of EC2 Availability Zones that instances in the DB cluster can be created in.
*/
public final List availabilityZones() {
return availabilityZones;
}
/**
*
* Specifies the number of days for which automatic DB snapshots are retained.
*
*
* @return Specifies the number of days for which automatic DB snapshots are retained.
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* (Not supported by Neptune)
*
*
* @return (Not supported by Neptune)
*/
public final String characterSetName() {
return characterSetName;
}
/**
*
* Contains the name of the initial database of this DB cluster that was provided at create time, if one was
* specified when the DB cluster was created. This same name is returned for the life of the DB cluster.
*
*
* @return Contains the name of the initial database of this DB cluster that was provided at create time, if one was
* specified when the DB cluster was created. This same name is returned for the life of the DB cluster.
*/
public final String databaseName() {
return databaseName;
}
/**
*
* Contains a user-supplied DB cluster identifier. This identifier is the unique key that identifies a DB cluster.
*
*
* @return Contains a user-supplied DB cluster identifier. This identifier is the unique key that identifies a DB
* cluster.
*/
public final String dbClusterIdentifier() {
return dbClusterIdentifier;
}
/**
*
* Specifies the name of the DB cluster parameter group for the DB cluster.
*
*
* @return Specifies the name of the DB cluster parameter group for the DB cluster.
*/
public final String dbClusterParameterGroup() {
return dbClusterParameterGroup;
}
/**
*
* Specifies information on the subnet group associated with the DB cluster, including the name, description, and
* subnets in the subnet group.
*
*
* @return Specifies information on the subnet group associated with the DB cluster, including the name,
* description, and subnets in the subnet group.
*/
public final String dbSubnetGroup() {
return dbSubnetGroup;
}
/**
*
* Specifies the current state of this DB cluster.
*
*
* @return Specifies the current state of this DB cluster.
*/
public final String status() {
return status;
}
/**
*
* Specifies the progress of the operation as a percentage.
*
*
* @return Specifies the progress of the operation as a percentage.
*/
public final String percentProgress() {
return percentProgress;
}
/**
*
* Specifies the earliest time to which a database can be restored with point-in-time restore.
*
*
* @return Specifies the earliest time to which a database can be restored with point-in-time restore.
*/
public final Instant earliestRestorableTime() {
return earliestRestorableTime;
}
/**
*
* Specifies the connection endpoint for the primary instance of the DB cluster.
*
*
* @return Specifies the connection endpoint for the primary instance of the DB cluster.
*/
public final String endpoint() {
return endpoint;
}
/**
*
* The reader endpoint for the DB cluster. The reader endpoint for a DB cluster load-balances connections across the
* Read Replicas that are available in a DB cluster. As clients request new connections to the reader endpoint,
* Neptune distributes the connection requests among the Read Replicas in the DB cluster. This functionality can
* help balance your read workload across multiple Read Replicas in your DB cluster.
*
*
* If a failover occurs, and the Read Replica that you are connected to is promoted to be the primary instance, your
* connection is dropped. To continue sending your read workload to other Read Replicas in the cluster, you can then
* reconnect to the reader endpoint.
*
*
* @return The reader endpoint for the DB cluster. The reader endpoint for a DB cluster load-balances connections
* across the Read Replicas that are available in a DB cluster. As clients request new connections to the
* reader endpoint, Neptune distributes the connection requests among the Read Replicas in the DB cluster.
* This functionality can help balance your read workload across multiple Read Replicas in your DB
* cluster.
*
* If a failover occurs, and the Read Replica that you are connected to is promoted to be the primary
* instance, your connection is dropped. To continue sending your read workload to other Read Replicas in
* the cluster, you can then reconnect to the reader endpoint.
*/
public final String readerEndpoint() {
return readerEndpoint;
}
/**
*
* Specifies whether the DB cluster has instances in multiple Availability Zones.
*
*
* @return Specifies whether the DB cluster has instances in multiple Availability Zones.
*/
public final Boolean multiAZ() {
return multiAZ;
}
/**
*
* Provides the name of the database engine to be used for this DB cluster.
*
*
* @return Provides the name of the database engine to be used for this DB cluster.
*/
public final String engine() {
return engine;
}
/**
*
* Indicates the database engine version.
*
*
* @return Indicates the database engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* Specifies the latest time to which a database can be restored with point-in-time restore.
*
*
* @return Specifies the latest time to which a database can be restored with point-in-time restore.
*/
public final Instant latestRestorableTime() {
return latestRestorableTime;
}
/**
*
* Specifies the port that the database engine is listening on.
*
*
* @return Specifies the port that the database engine is listening on.
*/
public final Integer port() {
return port;
}
/**
*
* Contains the master username for the DB cluster.
*
*
* @return Contains the master username for the DB cluster.
*/
public final String masterUsername() {
return masterUsername;
}
/**
* Returns true if the DBClusterOptionGroupMemberships property was specified by the sender (it may be empty), or
* false if the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender
* is the AWS service.
*/
public final boolean hasDbClusterOptionGroupMemberships() {
return dbClusterOptionGroupMemberships != null && !(dbClusterOptionGroupMemberships instanceof SdkAutoConstructList);
}
/**
*
* (Not supported by Neptune)
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasDbClusterOptionGroupMemberships()} to see if a value was sent in this field.
*
*
* @return (Not supported by Neptune)
*/
public final List dbClusterOptionGroupMemberships() {
return dbClusterOptionGroupMemberships;
}
/**
*
* Specifies the daily time range during which automated backups are created if automated backups are enabled, as
* determined by the BackupRetentionPeriod
.
*
*
* @return Specifies the daily time range during which automated backups are created if automated backups are
* enabled, as determined by the BackupRetentionPeriod
.
*/
public final String preferredBackupWindow() {
return preferredBackupWindow;
}
/**
*
* Specifies the weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* @return Specifies the weekly time range during which system maintenance can occur, in Universal Coordinated Time
* (UTC).
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String replicationSourceIdentifier() {
return replicationSourceIdentifier;
}
/**
* Returns true if the ReadReplicaIdentifiers property was specified by the sender (it may be empty), or false if
* the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasReadReplicaIdentifiers() {
return readReplicaIdentifiers != null && !(readReplicaIdentifiers instanceof SdkAutoConstructList);
}
/**
*
* Contains one or more identifiers of the Read Replicas associated with this DB cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasReadReplicaIdentifiers()} to see if a value was sent in this field.
*
*
* @return Contains one or more identifiers of the Read Replicas associated with this DB cluster.
*/
public final List readReplicaIdentifiers() {
return readReplicaIdentifiers;
}
/**
* Returns true if the DBClusterMembers property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasDbClusterMembers() {
return dbClusterMembers != null && !(dbClusterMembers instanceof SdkAutoConstructList);
}
/**
*
* Provides the list of instances that make up the DB cluster.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasDbClusterMembers()} to see if a value was sent in this field.
*
*
* @return Provides the list of instances that make up the DB cluster.
*/
public final List dbClusterMembers() {
return dbClusterMembers;
}
/**
* Returns true if the VpcSecurityGroups property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasVpcSecurityGroups() {
return vpcSecurityGroups != null && !(vpcSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* Provides a list of VPC security groups that the DB cluster belongs to.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasVpcSecurityGroups()} to see if a value was sent in this field.
*
*
* @return Provides a list of VPC security groups that the DB cluster belongs to.
*/
public final List vpcSecurityGroups() {
return vpcSecurityGroups;
}
/**
*
* Specifies the ID that Amazon Route 53 assigns when you create a hosted zone.
*
*
* @return Specifies the ID that Amazon Route 53 assigns when you create a hosted zone.
*/
public final String hostedZoneId() {
return hostedZoneId;
}
/**
*
* Specifies whether the DB cluster is encrypted.
*
*
* @return Specifies whether the DB cluster is encrypted.
*/
public final Boolean storageEncrypted() {
return storageEncrypted;
}
/**
*
* If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB cluster.
*
*
* @return If StorageEncrypted
is true, the AWS KMS key identifier for the encrypted DB cluster.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The AWS Region-unique, immutable identifier for the DB cluster. This identifier is found in AWS CloudTrail log
* entries whenever the AWS KMS key for the DB cluster is accessed.
*
*
* @return The AWS Region-unique, immutable identifier for the DB cluster. This identifier is found in AWS
* CloudTrail log entries whenever the AWS KMS key for the DB cluster is accessed.
*/
public final String dbClusterResourceId() {
return dbClusterResourceId;
}
/**
*
* The Amazon Resource Name (ARN) for the DB cluster.
*
*
* @return The Amazon Resource Name (ARN) for the DB cluster.
*/
public final String dbClusterArn() {
return dbClusterArn;
}
/**
* Returns true if the AssociatedRoles property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public final boolean hasAssociatedRoles() {
return associatedRoles != null && !(associatedRoles instanceof SdkAutoConstructList);
}
/**
*
* Provides a list of the AWS Identity and Access Management (IAM) roles that are associated with the DB cluster.
* IAM roles that are associated with a DB cluster grant permission for the DB cluster to access other AWS services
* on your behalf.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasAssociatedRoles()} to see if a value was sent in this field.
*
*
* @return Provides a list of the AWS Identity and Access Management (IAM) roles that are associated with the DB
* cluster. IAM roles that are associated with a DB cluster grant permission for the DB cluster to access
* other AWS services on your behalf.
*/
public final List associatedRoles() {
return associatedRoles;
}
/**
*
* True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* @return True if mapping of AWS Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*/
public final Boolean iamDatabaseAuthenticationEnabled() {
return iamDatabaseAuthenticationEnabled;
}
/**
*
* Identifies the clone group to which the DB cluster is associated.
*
*
* @return Identifies the clone group to which the DB cluster is associated.
*/
public final String cloneGroupId() {
return cloneGroupId;
}
/**
*
* Specifies the time when the DB cluster was created, in Universal Coordinated Time (UTC).
*
*
* @return Specifies the time when the DB cluster was created, in Universal Coordinated Time (UTC).
*/
public final Instant clusterCreateTime() {
return clusterCreateTime;
}
/**
* Returns true if the EnabledCloudwatchLogsExports property was specified by the sender (it may be empty), or false
* if the sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the
* AWS service.
*/
public final boolean hasEnabledCloudwatchLogsExports() {
return enabledCloudwatchLogsExports != null && !(enabledCloudwatchLogsExports instanceof SdkAutoConstructList);
}
/**
*
* A list of log types that this DB cluster is configured to export to CloudWatch Logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasEnabledCloudwatchLogsExports()} to see if a value was sent in this field.
*
*
* @return A list of log types that this DB cluster is configured to export to CloudWatch Logs.
*/
public final List enabledCloudwatchLogsExports() {
return enabledCloudwatchLogsExports;
}
/**
*
* Indicates whether or not the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled.
*
*
* @return Indicates whether or not the DB cluster has deletion protection enabled. The database can't be deleted
* when deletion protection is enabled.
*/
public final Boolean deletionProtection() {
return deletionProtection;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(hasAvailabilityZones() ? availabilityZones() : null);
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(characterSetName());
hashCode = 31 * hashCode + Objects.hashCode(databaseName());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterParameterGroup());
hashCode = 31 * hashCode + Objects.hashCode(dbSubnetGroup());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(percentProgress());
hashCode = 31 * hashCode + Objects.hashCode(earliestRestorableTime());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(readerEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(latestRestorableTime());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(masterUsername());
hashCode = 31 * hashCode
+ Objects.hashCode(hasDbClusterOptionGroupMemberships() ? dbClusterOptionGroupMemberships() : null);
hashCode = 31 * hashCode + Objects.hashCode(preferredBackupWindow());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(replicationSourceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(hasReadReplicaIdentifiers() ? readReplicaIdentifiers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasDbClusterMembers() ? dbClusterMembers() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroups() ? vpcSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hostedZoneId());
hashCode = 31 * hashCode + Objects.hashCode(storageEncrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterResourceId());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterArn());
hashCode = 31 * hashCode + Objects.hashCode(hasAssociatedRoles() ? associatedRoles() : null);
hashCode = 31 * hashCode + Objects.hashCode(iamDatabaseAuthenticationEnabled());
hashCode = 31 * hashCode + Objects.hashCode(cloneGroupId());
hashCode = 31 * hashCode + Objects.hashCode(clusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(hasEnabledCloudwatchLogsExports() ? enabledCloudwatchLogsExports() : null);
hashCode = 31 * hashCode + Objects.hashCode(deletionProtection());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DBCluster)) {
return false;
}
DBCluster other = (DBCluster) obj;
return Objects.equals(allocatedStorage(), other.allocatedStorage())
&& hasAvailabilityZones() == other.hasAvailabilityZones()
&& Objects.equals(availabilityZones(), other.availabilityZones())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(characterSetName(), other.characterSetName())
&& Objects.equals(databaseName(), other.databaseName())
&& Objects.equals(dbClusterIdentifier(), other.dbClusterIdentifier())
&& Objects.equals(dbClusterParameterGroup(), other.dbClusterParameterGroup())
&& Objects.equals(dbSubnetGroup(), other.dbSubnetGroup()) && Objects.equals(status(), other.status())
&& Objects.equals(percentProgress(), other.percentProgress())
&& Objects.equals(earliestRestorableTime(), other.earliestRestorableTime())
&& Objects.equals(endpoint(), other.endpoint()) && Objects.equals(readerEndpoint(), other.readerEndpoint())
&& Objects.equals(multiAZ(), other.multiAZ()) && Objects.equals(engine(), other.engine())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(latestRestorableTime(), other.latestRestorableTime()) && Objects.equals(port(), other.port())
&& Objects.equals(masterUsername(), other.masterUsername())
&& hasDbClusterOptionGroupMemberships() == other.hasDbClusterOptionGroupMemberships()
&& Objects.equals(dbClusterOptionGroupMemberships(), other.dbClusterOptionGroupMemberships())
&& Objects.equals(preferredBackupWindow(), other.preferredBackupWindow())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(replicationSourceIdentifier(), other.replicationSourceIdentifier())
&& hasReadReplicaIdentifiers() == other.hasReadReplicaIdentifiers()
&& Objects.equals(readReplicaIdentifiers(), other.readReplicaIdentifiers())
&& hasDbClusterMembers() == other.hasDbClusterMembers()
&& Objects.equals(dbClusterMembers(), other.dbClusterMembers())
&& hasVpcSecurityGroups() == other.hasVpcSecurityGroups()
&& Objects.equals(vpcSecurityGroups(), other.vpcSecurityGroups())
&& Objects.equals(hostedZoneId(), other.hostedZoneId())
&& Objects.equals(storageEncrypted(), other.storageEncrypted()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(dbClusterResourceId(), other.dbClusterResourceId())
&& Objects.equals(dbClusterArn(), other.dbClusterArn()) && hasAssociatedRoles() == other.hasAssociatedRoles()
&& Objects.equals(associatedRoles(), other.associatedRoles())
&& Objects.equals(iamDatabaseAuthenticationEnabled(), other.iamDatabaseAuthenticationEnabled())
&& Objects.equals(cloneGroupId(), other.cloneGroupId())
&& Objects.equals(clusterCreateTime(), other.clusterCreateTime())
&& hasEnabledCloudwatchLogsExports() == other.hasEnabledCloudwatchLogsExports()
&& Objects.equals(enabledCloudwatchLogsExports(), other.enabledCloudwatchLogsExports())
&& Objects.equals(deletionProtection(), other.deletionProtection());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("DBCluster")
.add("AllocatedStorage", allocatedStorage())
.add("AvailabilityZones", hasAvailabilityZones() ? availabilityZones() : null)
.add("BackupRetentionPeriod", backupRetentionPeriod())
.add("CharacterSetName", characterSetName())
.add("DatabaseName", databaseName())
.add("DBClusterIdentifier", dbClusterIdentifier())
.add("DBClusterParameterGroup", dbClusterParameterGroup())
.add("DBSubnetGroup", dbSubnetGroup())
.add("Status", status())
.add("PercentProgress", percentProgress())
.add("EarliestRestorableTime", earliestRestorableTime())
.add("Endpoint", endpoint())
.add("ReaderEndpoint", readerEndpoint())
.add("MultiAZ", multiAZ())
.add("Engine", engine())
.add("EngineVersion", engineVersion())
.add("LatestRestorableTime", latestRestorableTime())
.add("Port", port())
.add("MasterUsername", masterUsername())
.add("DBClusterOptionGroupMemberships",
hasDbClusterOptionGroupMemberships() ? dbClusterOptionGroupMemberships() : null)
.add("PreferredBackupWindow", preferredBackupWindow())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("ReplicationSourceIdentifier", replicationSourceIdentifier())
.add("ReadReplicaIdentifiers", hasReadReplicaIdentifiers() ? readReplicaIdentifiers() : null)
.add("DBClusterMembers", hasDbClusterMembers() ? dbClusterMembers() : null)
.add("VpcSecurityGroups", hasVpcSecurityGroups() ? vpcSecurityGroups() : null)
.add("HostedZoneId", hostedZoneId()).add("StorageEncrypted", storageEncrypted()).add("KmsKeyId", kmsKeyId())
.add("DbClusterResourceId", dbClusterResourceId()).add("DBClusterArn", dbClusterArn())
.add("AssociatedRoles", hasAssociatedRoles() ? associatedRoles() : null)
.add("IAMDatabaseAuthenticationEnabled", iamDatabaseAuthenticationEnabled()).add("CloneGroupId", cloneGroupId())
.add("ClusterCreateTime", clusterCreateTime())
.add("EnabledCloudwatchLogsExports", hasEnabledCloudwatchLogsExports() ? enabledCloudwatchLogsExports() : null)
.add("DeletionProtection", deletionProtection()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "AvailabilityZones":
return Optional.ofNullable(clazz.cast(availabilityZones()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "CharacterSetName":
return Optional.ofNullable(clazz.cast(characterSetName()));
case "DatabaseName":
return Optional.ofNullable(clazz.cast(databaseName()));
case "DBClusterIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterIdentifier()));
case "DBClusterParameterGroup":
return Optional.ofNullable(clazz.cast(dbClusterParameterGroup()));
case "DBSubnetGroup":
return Optional.ofNullable(clazz.cast(dbSubnetGroup()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "PercentProgress":
return Optional.ofNullable(clazz.cast(percentProgress()));
case "EarliestRestorableTime":
return Optional.ofNullable(clazz.cast(earliestRestorableTime()));
case "Endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "ReaderEndpoint":
return Optional.ofNullable(clazz.cast(readerEndpoint()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "LatestRestorableTime":
return Optional.ofNullable(clazz.cast(latestRestorableTime()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "MasterUsername":
return Optional.ofNullable(clazz.cast(masterUsername()));
case "DBClusterOptionGroupMemberships":
return Optional.ofNullable(clazz.cast(dbClusterOptionGroupMemberships()));
case "PreferredBackupWindow":
return Optional.ofNullable(clazz.cast(preferredBackupWindow()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "ReplicationSourceIdentifier":
return Optional.ofNullable(clazz.cast(replicationSourceIdentifier()));
case "ReadReplicaIdentifiers":
return Optional.ofNullable(clazz.cast(readReplicaIdentifiers()));
case "DBClusterMembers":
return Optional.ofNullable(clazz.cast(dbClusterMembers()));
case "VpcSecurityGroups":
return Optional.ofNullable(clazz.cast(vpcSecurityGroups()));
case "HostedZoneId":
return Optional.ofNullable(clazz.cast(hostedZoneId()));
case "StorageEncrypted":
return Optional.ofNullable(clazz.cast(storageEncrypted()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "DbClusterResourceId":
return Optional.ofNullable(clazz.cast(dbClusterResourceId()));
case "DBClusterArn":
return Optional.ofNullable(clazz.cast(dbClusterArn()));
case "AssociatedRoles":
return Optional.ofNullable(clazz.cast(associatedRoles()));
case "IAMDatabaseAuthenticationEnabled":
return Optional.ofNullable(clazz.cast(iamDatabaseAuthenticationEnabled()));
case "CloneGroupId":
return Optional.ofNullable(clazz.cast(cloneGroupId()));
case "ClusterCreateTime":
return Optional.ofNullable(clazz.cast(clusterCreateTime()));
case "EnabledCloudwatchLogsExports":
return Optional.ofNullable(clazz.cast(enabledCloudwatchLogsExports()));
case "DeletionProtection":
return Optional.ofNullable(clazz.cast(deletionProtection()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function