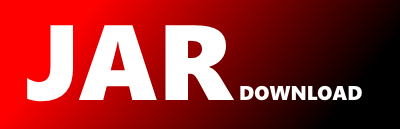
software.amazon.awssdk.services.neptune.model.DBClusterSnapshot Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the details for an Amazon Neptune DB cluster snapshot
*
*
* This data type is used as a response element in the DescribeDBClusterSnapshots action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DBClusterSnapshot implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> AVAILABILITY_ZONES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AvailabilityZones")
.getter(getter(DBClusterSnapshot::availabilityZones))
.setter(setter(Builder::availabilityZones))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZones").build(),
ListTrait
.builder()
.memberLocationName("AvailabilityZone")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AvailabilityZone").build()).build()).build()).build();
private static final SdkField DB_CLUSTER_SNAPSHOT_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DBClusterSnapshotIdentifier")
.getter(getter(DBClusterSnapshot::dbClusterSnapshotIdentifier))
.setter(setter(Builder::dbClusterSnapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterSnapshotIdentifier")
.build()).build();
private static final SdkField DB_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterIdentifier").getter(getter(DBClusterSnapshot::dbClusterIdentifier))
.setter(setter(Builder::dbClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterIdentifier").build())
.build();
private static final SdkField SNAPSHOT_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("SnapshotCreateTime").getter(getter(DBClusterSnapshot::snapshotCreateTime))
.setter(setter(Builder::snapshotCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotCreateTime").build())
.build();
private static final SdkField ENGINE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Engine")
.getter(getter(DBClusterSnapshot::engine)).setter(setter(Builder::engine))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Engine").build()).build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(DBClusterSnapshot::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(DBClusterSnapshot::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(DBClusterSnapshot::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField VPC_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("VpcId")
.getter(getter(DBClusterSnapshot::vpcId)).setter(setter(Builder::vpcId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcId").build()).build();
private static final SdkField CLUSTER_CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ClusterCreateTime").getter(getter(DBClusterSnapshot::clusterCreateTime))
.setter(setter(Builder::clusterCreateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ClusterCreateTime").build()).build();
private static final SdkField MASTER_USERNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUsername").getter(getter(DBClusterSnapshot::masterUsername))
.setter(setter(Builder::masterUsername))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUsername").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(DBClusterSnapshot::engineVersion)).setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField LICENSE_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LicenseModel").getter(getter(DBClusterSnapshot::licenseModel)).setter(setter(Builder::licenseModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LicenseModel").build()).build();
private static final SdkField SNAPSHOT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnapshotType").getter(getter(DBClusterSnapshot::snapshotType)).setter(setter(Builder::snapshotType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnapshotType").build()).build();
private static final SdkField PERCENT_PROGRESS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PercentProgress").getter(getter(DBClusterSnapshot::percentProgress))
.setter(setter(Builder::percentProgress))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PercentProgress").build()).build();
private static final SdkField STORAGE_ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("StorageEncrypted").getter(getter(DBClusterSnapshot::storageEncrypted))
.setter(setter(Builder::storageEncrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageEncrypted").build()).build();
private static final SdkField KMS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KmsKeyId").getter(getter(DBClusterSnapshot::kmsKeyId)).setter(setter(Builder::kmsKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KmsKeyId").build()).build();
private static final SdkField DB_CLUSTER_SNAPSHOT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterSnapshotArn").getter(getter(DBClusterSnapshot::dbClusterSnapshotArn))
.setter(setter(Builder::dbClusterSnapshotArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterSnapshotArn").build())
.build();
private static final SdkField SOURCE_DB_CLUSTER_SNAPSHOT_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("SourceDBClusterSnapshotArn")
.getter(getter(DBClusterSnapshot::sourceDBClusterSnapshotArn))
.setter(setter(Builder::sourceDBClusterSnapshotArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceDBClusterSnapshotArn").build())
.build();
private static final SdkField IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IAMDatabaseAuthenticationEnabled")
.getter(getter(DBClusterSnapshot::iamDatabaseAuthenticationEnabled))
.setter(setter(Builder::iamDatabaseAuthenticationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IAMDatabaseAuthenticationEnabled")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AVAILABILITY_ZONES_FIELD,
DB_CLUSTER_SNAPSHOT_IDENTIFIER_FIELD, DB_CLUSTER_IDENTIFIER_FIELD, SNAPSHOT_CREATE_TIME_FIELD, ENGINE_FIELD,
ALLOCATED_STORAGE_FIELD, STATUS_FIELD, PORT_FIELD, VPC_ID_FIELD, CLUSTER_CREATE_TIME_FIELD, MASTER_USERNAME_FIELD,
ENGINE_VERSION_FIELD, LICENSE_MODEL_FIELD, SNAPSHOT_TYPE_FIELD, PERCENT_PROGRESS_FIELD, STORAGE_ENCRYPTED_FIELD,
KMS_KEY_ID_FIELD, DB_CLUSTER_SNAPSHOT_ARN_FIELD, SOURCE_DB_CLUSTER_SNAPSHOT_ARN_FIELD,
IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD));
private static final long serialVersionUID = 1L;
private final List availabilityZones;
private final String dbClusterSnapshotIdentifier;
private final String dbClusterIdentifier;
private final Instant snapshotCreateTime;
private final String engine;
private final Integer allocatedStorage;
private final String status;
private final Integer port;
private final String vpcId;
private final Instant clusterCreateTime;
private final String masterUsername;
private final String engineVersion;
private final String licenseModel;
private final String snapshotType;
private final Integer percentProgress;
private final Boolean storageEncrypted;
private final String kmsKeyId;
private final String dbClusterSnapshotArn;
private final String sourceDBClusterSnapshotArn;
private final Boolean iamDatabaseAuthenticationEnabled;
private DBClusterSnapshot(BuilderImpl builder) {
this.availabilityZones = builder.availabilityZones;
this.dbClusterSnapshotIdentifier = builder.dbClusterSnapshotIdentifier;
this.dbClusterIdentifier = builder.dbClusterIdentifier;
this.snapshotCreateTime = builder.snapshotCreateTime;
this.engine = builder.engine;
this.allocatedStorage = builder.allocatedStorage;
this.status = builder.status;
this.port = builder.port;
this.vpcId = builder.vpcId;
this.clusterCreateTime = builder.clusterCreateTime;
this.masterUsername = builder.masterUsername;
this.engineVersion = builder.engineVersion;
this.licenseModel = builder.licenseModel;
this.snapshotType = builder.snapshotType;
this.percentProgress = builder.percentProgress;
this.storageEncrypted = builder.storageEncrypted;
this.kmsKeyId = builder.kmsKeyId;
this.dbClusterSnapshotArn = builder.dbClusterSnapshotArn;
this.sourceDBClusterSnapshotArn = builder.sourceDBClusterSnapshotArn;
this.iamDatabaseAuthenticationEnabled = builder.iamDatabaseAuthenticationEnabled;
}
/**
* For responses, this returns true if the service returned a value for the AvailabilityZones property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAvailabilityZones() {
return availabilityZones != null && !(availabilityZones instanceof SdkAutoConstructList);
}
/**
*
* Provides the list of EC2 Availability Zones that instances in the DB cluster snapshot can be restored in.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAvailabilityZones} method.
*
*
* @return Provides the list of EC2 Availability Zones that instances in the DB cluster snapshot can be restored in.
*/
public final List availabilityZones() {
return availabilityZones;
}
/**
*
* Specifies the identifier for a DB cluster snapshot. Must match the identifier of an existing snapshot.
*
*
* After you restore a DB cluster using a DBClusterSnapshotIdentifier
, you must specify the same
* DBClusterSnapshotIdentifier
for any future updates to the DB cluster. When you specify this property
* for an update, the DB cluster is not restored from the snapshot again, and the data in the database is not
* changed.
*
*
* However, if you don't specify the DBClusterSnapshotIdentifier
, an empty DB cluster is created, and
* the original DB cluster is deleted. If you specify a property that is different from the previous snapshot
* restore property, the DB cluster is restored from the snapshot specified by the
* DBClusterSnapshotIdentifier
, and the original DB cluster is deleted.
*
*
* @return Specifies the identifier for a DB cluster snapshot. Must match the identifier of an existing
* snapshot.
*
* After you restore a DB cluster using a DBClusterSnapshotIdentifier
, you must specify the
* same DBClusterSnapshotIdentifier
for any future updates to the DB cluster. When you specify
* this property for an update, the DB cluster is not restored from the snapshot again, and the data in the
* database is not changed.
*
*
* However, if you don't specify the DBClusterSnapshotIdentifier
, an empty DB cluster is
* created, and the original DB cluster is deleted. If you specify a property that is different from the
* previous snapshot restore property, the DB cluster is restored from the snapshot specified by the
* DBClusterSnapshotIdentifier
, and the original DB cluster is deleted.
*/
public final String dbClusterSnapshotIdentifier() {
return dbClusterSnapshotIdentifier;
}
/**
*
* Specifies the DB cluster identifier of the DB cluster that this DB cluster snapshot was created from.
*
*
* @return Specifies the DB cluster identifier of the DB cluster that this DB cluster snapshot was created from.
*/
public final String dbClusterIdentifier() {
return dbClusterIdentifier;
}
/**
*
* Provides the time when the snapshot was taken, in Universal Coordinated Time (UTC).
*
*
* @return Provides the time when the snapshot was taken, in Universal Coordinated Time (UTC).
*/
public final Instant snapshotCreateTime() {
return snapshotCreateTime;
}
/**
*
* Specifies the name of the database engine.
*
*
* @return Specifies the name of the database engine.
*/
public final String engine() {
return engine;
}
/**
*
* Specifies the allocated storage size in gibibytes (GiB).
*
*
* @return Specifies the allocated storage size in gibibytes (GiB).
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* Specifies the status of this DB cluster snapshot.
*
*
* @return Specifies the status of this DB cluster snapshot.
*/
public final String status() {
return status;
}
/**
*
* Specifies the port that the DB cluster was listening on at the time of the snapshot.
*
*
* @return Specifies the port that the DB cluster was listening on at the time of the snapshot.
*/
public final Integer port() {
return port;
}
/**
*
* Provides the VPC ID associated with the DB cluster snapshot.
*
*
* @return Provides the VPC ID associated with the DB cluster snapshot.
*/
public final String vpcId() {
return vpcId;
}
/**
*
* Specifies the time when the DB cluster was created, in Universal Coordinated Time (UTC).
*
*
* @return Specifies the time when the DB cluster was created, in Universal Coordinated Time (UTC).
*/
public final Instant clusterCreateTime() {
return clusterCreateTime;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String masterUsername() {
return masterUsername;
}
/**
*
* Provides the version of the database engine for this DB cluster snapshot.
*
*
* @return Provides the version of the database engine for this DB cluster snapshot.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* Provides the license model information for this DB cluster snapshot.
*
*
* @return Provides the license model information for this DB cluster snapshot.
*/
public final String licenseModel() {
return licenseModel;
}
/**
*
* Provides the type of the DB cluster snapshot.
*
*
* @return Provides the type of the DB cluster snapshot.
*/
public final String snapshotType() {
return snapshotType;
}
/**
*
* Specifies the percentage of the estimated data that has been transferred.
*
*
* @return Specifies the percentage of the estimated data that has been transferred.
*/
public final Integer percentProgress() {
return percentProgress;
}
/**
*
* Specifies whether the DB cluster snapshot is encrypted.
*
*
* @return Specifies whether the DB cluster snapshot is encrypted.
*/
public final Boolean storageEncrypted() {
return storageEncrypted;
}
/**
*
* If StorageEncrypted
is true, the Amazon KMS key identifier for the encrypted DB cluster snapshot.
*
*
* @return If StorageEncrypted
is true, the Amazon KMS key identifier for the encrypted DB cluster
* snapshot.
*/
public final String kmsKeyId() {
return kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) for the DB cluster snapshot.
*
*
* @return The Amazon Resource Name (ARN) for the DB cluster snapshot.
*/
public final String dbClusterSnapshotArn() {
return dbClusterSnapshotArn;
}
/**
*
* If the DB cluster snapshot was copied from a source DB cluster snapshot, the Amazon Resource Name (ARN) for the
* source DB cluster snapshot, otherwise, a null value.
*
*
* @return If the DB cluster snapshot was copied from a source DB cluster snapshot, the Amazon Resource Name (ARN)
* for the source DB cluster snapshot, otherwise, a null value.
*/
public final String sourceDBClusterSnapshotArn() {
return sourceDBClusterSnapshotArn;
}
/**
*
* True if mapping of Amazon Identity and Access Management (IAM) accounts to database accounts is enabled, and
* otherwise false.
*
*
* @return True if mapping of Amazon Identity and Access Management (IAM) accounts to database accounts is enabled,
* and otherwise false.
*/
public final Boolean iamDatabaseAuthenticationEnabled() {
return iamDatabaseAuthenticationEnabled;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasAvailabilityZones() ? availabilityZones() : null);
hashCode = 31 * hashCode + Objects.hashCode(dbClusterSnapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(snapshotCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(engine());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(vpcId());
hashCode = 31 * hashCode + Objects.hashCode(clusterCreateTime());
hashCode = 31 * hashCode + Objects.hashCode(masterUsername());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(licenseModel());
hashCode = 31 * hashCode + Objects.hashCode(snapshotType());
hashCode = 31 * hashCode + Objects.hashCode(percentProgress());
hashCode = 31 * hashCode + Objects.hashCode(storageEncrypted());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyId());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterSnapshotArn());
hashCode = 31 * hashCode + Objects.hashCode(sourceDBClusterSnapshotArn());
hashCode = 31 * hashCode + Objects.hashCode(iamDatabaseAuthenticationEnabled());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DBClusterSnapshot)) {
return false;
}
DBClusterSnapshot other = (DBClusterSnapshot) obj;
return hasAvailabilityZones() == other.hasAvailabilityZones()
&& Objects.equals(availabilityZones(), other.availabilityZones())
&& Objects.equals(dbClusterSnapshotIdentifier(), other.dbClusterSnapshotIdentifier())
&& Objects.equals(dbClusterIdentifier(), other.dbClusterIdentifier())
&& Objects.equals(snapshotCreateTime(), other.snapshotCreateTime()) && Objects.equals(engine(), other.engine())
&& Objects.equals(allocatedStorage(), other.allocatedStorage()) && Objects.equals(status(), other.status())
&& Objects.equals(port(), other.port()) && Objects.equals(vpcId(), other.vpcId())
&& Objects.equals(clusterCreateTime(), other.clusterCreateTime())
&& Objects.equals(masterUsername(), other.masterUsername())
&& Objects.equals(engineVersion(), other.engineVersion()) && Objects.equals(licenseModel(), other.licenseModel())
&& Objects.equals(snapshotType(), other.snapshotType())
&& Objects.equals(percentProgress(), other.percentProgress())
&& Objects.equals(storageEncrypted(), other.storageEncrypted()) && Objects.equals(kmsKeyId(), other.kmsKeyId())
&& Objects.equals(dbClusterSnapshotArn(), other.dbClusterSnapshotArn())
&& Objects.equals(sourceDBClusterSnapshotArn(), other.sourceDBClusterSnapshotArn())
&& Objects.equals(iamDatabaseAuthenticationEnabled(), other.iamDatabaseAuthenticationEnabled());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DBClusterSnapshot")
.add("AvailabilityZones", hasAvailabilityZones() ? availabilityZones() : null)
.add("DBClusterSnapshotIdentifier", dbClusterSnapshotIdentifier())
.add("DBClusterIdentifier", dbClusterIdentifier()).add("SnapshotCreateTime", snapshotCreateTime())
.add("Engine", engine()).add("AllocatedStorage", allocatedStorage()).add("Status", status()).add("Port", port())
.add("VpcId", vpcId()).add("ClusterCreateTime", clusterCreateTime()).add("MasterUsername", masterUsername())
.add("EngineVersion", engineVersion()).add("LicenseModel", licenseModel()).add("SnapshotType", snapshotType())
.add("PercentProgress", percentProgress()).add("StorageEncrypted", storageEncrypted())
.add("KmsKeyId", kmsKeyId()).add("DBClusterSnapshotArn", dbClusterSnapshotArn())
.add("SourceDBClusterSnapshotArn", sourceDBClusterSnapshotArn())
.add("IAMDatabaseAuthenticationEnabled", iamDatabaseAuthenticationEnabled()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AvailabilityZones":
return Optional.ofNullable(clazz.cast(availabilityZones()));
case "DBClusterSnapshotIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterSnapshotIdentifier()));
case "DBClusterIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterIdentifier()));
case "SnapshotCreateTime":
return Optional.ofNullable(clazz.cast(snapshotCreateTime()));
case "Engine":
return Optional.ofNullable(clazz.cast(engine()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "VpcId":
return Optional.ofNullable(clazz.cast(vpcId()));
case "ClusterCreateTime":
return Optional.ofNullable(clazz.cast(clusterCreateTime()));
case "MasterUsername":
return Optional.ofNullable(clazz.cast(masterUsername()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "LicenseModel":
return Optional.ofNullable(clazz.cast(licenseModel()));
case "SnapshotType":
return Optional.ofNullable(clazz.cast(snapshotType()));
case "PercentProgress":
return Optional.ofNullable(clazz.cast(percentProgress()));
case "StorageEncrypted":
return Optional.ofNullable(clazz.cast(storageEncrypted()));
case "KmsKeyId":
return Optional.ofNullable(clazz.cast(kmsKeyId()));
case "DBClusterSnapshotArn":
return Optional.ofNullable(clazz.cast(dbClusterSnapshotArn()));
case "SourceDBClusterSnapshotArn":
return Optional.ofNullable(clazz.cast(sourceDBClusterSnapshotArn()));
case "IAMDatabaseAuthenticationEnabled":
return Optional.ofNullable(clazz.cast(iamDatabaseAuthenticationEnabled()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function