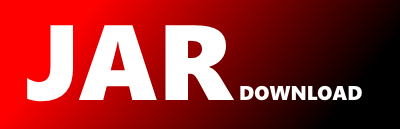
software.amazon.awssdk.services.neptune.model.ModifyDbInstanceRequest Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyDbInstanceRequest extends NeptuneRequest implements
ToCopyableBuilder {
private static final SdkField DB_INSTANCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceIdentifier").getter(getter(ModifyDbInstanceRequest::dbInstanceIdentifier))
.setter(setter(Builder::dbInstanceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceIdentifier").build())
.build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(ModifyDbInstanceRequest::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField DB_INSTANCE_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceClass").getter(getter(ModifyDbInstanceRequest::dbInstanceClass))
.setter(setter(Builder::dbInstanceClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceClass").build()).build();
private static final SdkField DB_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBSubnetGroupName").getter(getter(ModifyDbInstanceRequest::dbSubnetGroupName))
.setter(setter(Builder::dbSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBSubnetGroupName").build()).build();
private static final SdkField> DB_SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DBSecurityGroups")
.getter(getter(ModifyDbInstanceRequest::dbSecurityGroups))
.setter(setter(Builder::dbSecurityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBSecurityGroups").build(),
ListTrait
.builder()
.memberLocationName("DBSecurityGroupName")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("DBSecurityGroupName").build()).build()).build()).build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroupIds")
.getter(getter(ModifyDbInstanceRequest::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroupId").build()).build()).build()).build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyDbInstanceRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(ModifyDbInstanceRequest::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField DB_PARAMETER_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBParameterGroupName").getter(getter(ModifyDbInstanceRequest::dbParameterGroupName))
.setter(setter(Builder::dbParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBParameterGroupName").build())
.build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(ModifyDbInstanceRequest::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField PREFERRED_BACKUP_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredBackupWindow").getter(getter(ModifyDbInstanceRequest::preferredBackupWindow))
.setter(setter(Builder::preferredBackupWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredBackupWindow").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyDbInstanceRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZ").getter(getter(ModifyDbInstanceRequest::multiAZ)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ModifyDbInstanceRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField ALLOW_MAJOR_VERSION_UPGRADE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("AllowMajorVersionUpgrade")
.getter(getter(ModifyDbInstanceRequest::allowMajorVersionUpgrade)).setter(setter(Builder::allowMajorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowMajorVersionUpgrade").build())
.build();
private static final SdkField AUTO_MINOR_VERSION_UPGRADE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("AutoMinorVersionUpgrade").getter(getter(ModifyDbInstanceRequest::autoMinorVersionUpgrade))
.setter(setter(Builder::autoMinorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoMinorVersionUpgrade").build())
.build();
private static final SdkField LICENSE_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LicenseModel").getter(getter(ModifyDbInstanceRequest::licenseModel))
.setter(setter(Builder::licenseModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LicenseModel").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(ModifyDbInstanceRequest::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final SdkField OPTION_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OptionGroupName").getter(getter(ModifyDbInstanceRequest::optionGroupName))
.setter(setter(Builder::optionGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionGroupName").build()).build();
private static final SdkField NEW_DB_INSTANCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewDBInstanceIdentifier").getter(getter(ModifyDbInstanceRequest::newDBInstanceIdentifier))
.setter(setter(Builder::newDBInstanceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewDBInstanceIdentifier").build())
.build();
private static final SdkField STORAGE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StorageType").getter(getter(ModifyDbInstanceRequest::storageType)).setter(setter(Builder::storageType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageType").build()).build();
private static final SdkField TDE_CREDENTIAL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TdeCredentialArn").getter(getter(ModifyDbInstanceRequest::tdeCredentialArn))
.setter(setter(Builder::tdeCredentialArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TdeCredentialArn").build()).build();
private static final SdkField TDE_CREDENTIAL_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TdeCredentialPassword").getter(getter(ModifyDbInstanceRequest::tdeCredentialPassword))
.setter(setter(Builder::tdeCredentialPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TdeCredentialPassword").build())
.build();
private static final SdkField CA_CERTIFICATE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CACertificateIdentifier").getter(getter(ModifyDbInstanceRequest::caCertificateIdentifier))
.setter(setter(Builder::caCertificateIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CACertificateIdentifier").build())
.build();
private static final SdkField DOMAIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Domain")
.getter(getter(ModifyDbInstanceRequest::domain)).setter(setter(Builder::domain))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Domain").build()).build();
private static final SdkField COPY_TAGS_TO_SNAPSHOT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("CopyTagsToSnapshot").getter(getter(ModifyDbInstanceRequest::copyTagsToSnapshot))
.setter(setter(Builder::copyTagsToSnapshot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CopyTagsToSnapshot").build())
.build();
private static final SdkField MONITORING_INTERVAL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MonitoringInterval").getter(getter(ModifyDbInstanceRequest::monitoringInterval))
.setter(setter(Builder::monitoringInterval))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MonitoringInterval").build())
.build();
private static final SdkField DB_PORT_NUMBER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("DBPortNumber").getter(getter(ModifyDbInstanceRequest::dbPortNumber))
.setter(setter(Builder::dbPortNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBPortNumber").build()).build();
private static final SdkField PUBLICLY_ACCESSIBLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("PubliclyAccessible").getter(getter(ModifyDbInstanceRequest::publiclyAccessible))
.setter(setter(Builder::publiclyAccessible))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PubliclyAccessible").build())
.build();
private static final SdkField MONITORING_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MonitoringRoleArn").getter(getter(ModifyDbInstanceRequest::monitoringRoleArn))
.setter(setter(Builder::monitoringRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MonitoringRoleArn").build()).build();
private static final SdkField DOMAIN_IAM_ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DomainIAMRoleName").getter(getter(ModifyDbInstanceRequest::domainIAMRoleName))
.setter(setter(Builder::domainIAMRoleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DomainIAMRoleName").build()).build();
private static final SdkField PROMOTION_TIER_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("PromotionTier").getter(getter(ModifyDbInstanceRequest::promotionTier))
.setter(setter(Builder::promotionTier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PromotionTier").build()).build();
private static final SdkField ENABLE_IAM_DATABASE_AUTHENTICATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableIAMDatabaseAuthentication")
.getter(getter(ModifyDbInstanceRequest::enableIAMDatabaseAuthentication))
.setter(setter(Builder::enableIAMDatabaseAuthentication))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableIAMDatabaseAuthentication")
.build()).build();
private static final SdkField ENABLE_PERFORMANCE_INSIGHTS_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("EnablePerformanceInsights")
.getter(getter(ModifyDbInstanceRequest::enablePerformanceInsights))
.setter(setter(Builder::enablePerformanceInsights))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnablePerformanceInsights").build())
.build();
private static final SdkField PERFORMANCE_INSIGHTS_KMS_KEY_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PerformanceInsightsKMSKeyId")
.getter(getter(ModifyDbInstanceRequest::performanceInsightsKMSKeyId))
.setter(setter(Builder::performanceInsightsKMSKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PerformanceInsightsKMSKeyId")
.build()).build();
private static final SdkField CLOUDWATCH_LOGS_EXPORT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("CloudwatchLogsExportConfiguration")
.getter(getter(ModifyDbInstanceRequest::cloudwatchLogsExportConfiguration))
.setter(setter(Builder::cloudwatchLogsExportConfiguration))
.constructor(CloudwatchLogsExportConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CloudwatchLogsExportConfiguration")
.build()).build();
private static final SdkField DELETION_PROTECTION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DeletionProtection").getter(getter(ModifyDbInstanceRequest::deletionProtection))
.setter(setter(Builder::deletionProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeletionProtection").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_INSTANCE_IDENTIFIER_FIELD,
ALLOCATED_STORAGE_FIELD, DB_INSTANCE_CLASS_FIELD, DB_SUBNET_GROUP_NAME_FIELD, DB_SECURITY_GROUPS_FIELD,
VPC_SECURITY_GROUP_IDS_FIELD, APPLY_IMMEDIATELY_FIELD, MASTER_USER_PASSWORD_FIELD, DB_PARAMETER_GROUP_NAME_FIELD,
BACKUP_RETENTION_PERIOD_FIELD, PREFERRED_BACKUP_WINDOW_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD, MULTI_AZ_FIELD,
ENGINE_VERSION_FIELD, ALLOW_MAJOR_VERSION_UPGRADE_FIELD, AUTO_MINOR_VERSION_UPGRADE_FIELD, LICENSE_MODEL_FIELD,
IOPS_FIELD, OPTION_GROUP_NAME_FIELD, NEW_DB_INSTANCE_IDENTIFIER_FIELD, STORAGE_TYPE_FIELD, TDE_CREDENTIAL_ARN_FIELD,
TDE_CREDENTIAL_PASSWORD_FIELD, CA_CERTIFICATE_IDENTIFIER_FIELD, DOMAIN_FIELD, COPY_TAGS_TO_SNAPSHOT_FIELD,
MONITORING_INTERVAL_FIELD, DB_PORT_NUMBER_FIELD, PUBLICLY_ACCESSIBLE_FIELD, MONITORING_ROLE_ARN_FIELD,
DOMAIN_IAM_ROLE_NAME_FIELD, PROMOTION_TIER_FIELD, ENABLE_IAM_DATABASE_AUTHENTICATION_FIELD,
ENABLE_PERFORMANCE_INSIGHTS_FIELD, PERFORMANCE_INSIGHTS_KMS_KEY_ID_FIELD, CLOUDWATCH_LOGS_EXPORT_CONFIGURATION_FIELD,
DELETION_PROTECTION_FIELD));
private final String dbInstanceIdentifier;
private final Integer allocatedStorage;
private final String dbInstanceClass;
private final String dbSubnetGroupName;
private final List dbSecurityGroups;
private final List vpcSecurityGroupIds;
private final Boolean applyImmediately;
private final String masterUserPassword;
private final String dbParameterGroupName;
private final Integer backupRetentionPeriod;
private final String preferredBackupWindow;
private final String preferredMaintenanceWindow;
private final Boolean multiAZ;
private final String engineVersion;
private final Boolean allowMajorVersionUpgrade;
private final Boolean autoMinorVersionUpgrade;
private final String licenseModel;
private final Integer iops;
private final String optionGroupName;
private final String newDBInstanceIdentifier;
private final String storageType;
private final String tdeCredentialArn;
private final String tdeCredentialPassword;
private final String caCertificateIdentifier;
private final String domain;
private final Boolean copyTagsToSnapshot;
private final Integer monitoringInterval;
private final Integer dbPortNumber;
private final Boolean publiclyAccessible;
private final String monitoringRoleArn;
private final String domainIAMRoleName;
private final Integer promotionTier;
private final Boolean enableIAMDatabaseAuthentication;
private final Boolean enablePerformanceInsights;
private final String performanceInsightsKMSKeyId;
private final CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration;
private final Boolean deletionProtection;
private ModifyDbInstanceRequest(BuilderImpl builder) {
super(builder);
this.dbInstanceIdentifier = builder.dbInstanceIdentifier;
this.allocatedStorage = builder.allocatedStorage;
this.dbInstanceClass = builder.dbInstanceClass;
this.dbSubnetGroupName = builder.dbSubnetGroupName;
this.dbSecurityGroups = builder.dbSecurityGroups;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.applyImmediately = builder.applyImmediately;
this.masterUserPassword = builder.masterUserPassword;
this.dbParameterGroupName = builder.dbParameterGroupName;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.preferredBackupWindow = builder.preferredBackupWindow;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.multiAZ = builder.multiAZ;
this.engineVersion = builder.engineVersion;
this.allowMajorVersionUpgrade = builder.allowMajorVersionUpgrade;
this.autoMinorVersionUpgrade = builder.autoMinorVersionUpgrade;
this.licenseModel = builder.licenseModel;
this.iops = builder.iops;
this.optionGroupName = builder.optionGroupName;
this.newDBInstanceIdentifier = builder.newDBInstanceIdentifier;
this.storageType = builder.storageType;
this.tdeCredentialArn = builder.tdeCredentialArn;
this.tdeCredentialPassword = builder.tdeCredentialPassword;
this.caCertificateIdentifier = builder.caCertificateIdentifier;
this.domain = builder.domain;
this.copyTagsToSnapshot = builder.copyTagsToSnapshot;
this.monitoringInterval = builder.monitoringInterval;
this.dbPortNumber = builder.dbPortNumber;
this.publiclyAccessible = builder.publiclyAccessible;
this.monitoringRoleArn = builder.monitoringRoleArn;
this.domainIAMRoleName = builder.domainIAMRoleName;
this.promotionTier = builder.promotionTier;
this.enableIAMDatabaseAuthentication = builder.enableIAMDatabaseAuthentication;
this.enablePerformanceInsights = builder.enablePerformanceInsights;
this.performanceInsightsKMSKeyId = builder.performanceInsightsKMSKeyId;
this.cloudwatchLogsExportConfiguration = builder.cloudwatchLogsExportConfiguration;
this.deletionProtection = builder.deletionProtection;
}
/**
*
* The DB instance identifier. This value is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBInstance.
*
*
*
*
* @return The DB instance identifier. This value is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBInstance.
*
*
*/
public final String dbInstanceIdentifier() {
return dbInstanceIdentifier;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* The new compute and memory capacity of the DB instance, for example, db.m4.large
. Not all DB
* instance classes are available in all Amazon Regions.
*
*
* If you modify the DB instance class, an outage occurs during the change. The change is applied during the next
* maintenance window, unless ApplyImmediately
is specified as true
for this request.
*
*
* Default: Uses existing setting
*
*
* @return The new compute and memory capacity of the DB instance, for example, db.m4.large
. Not all DB
* instance classes are available in all Amazon Regions.
*
* If you modify the DB instance class, an outage occurs during the change. The change is applied during the
* next maintenance window, unless ApplyImmediately
is specified as true
for this
* request.
*
*
* Default: Uses existing setting
*/
public final String dbInstanceClass() {
return dbInstanceClass;
}
/**
*
* The new DB subnet group for the DB instance. You can use this parameter to move your DB instance to a different
* VPC.
*
*
* Changing the subnet group causes an outage during the change. The change is applied during the next maintenance
* window, unless you specify true
for the ApplyImmediately
parameter.
*
*
* Constraints: If supplied, must match the name of an existing DBSubnetGroup.
*
*
* Example: mySubnetGroup
*
*
* @return The new DB subnet group for the DB instance. You can use this parameter to move your DB instance to a
* different VPC.
*
* Changing the subnet group causes an outage during the change. The change is applied during the next
* maintenance window, unless you specify true
for the ApplyImmediately
parameter.
*
*
* Constraints: If supplied, must match the name of an existing DBSubnetGroup.
*
*
* Example: mySubnetGroup
*/
public final String dbSubnetGroupName() {
return dbSubnetGroupName;
}
/**
* For responses, this returns true if the service returned a value for the DBSecurityGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDbSecurityGroups() {
return dbSecurityGroups != null && !(dbSecurityGroups instanceof SdkAutoConstructList);
}
/**
*
* A list of DB security groups to authorize on this DB instance. Changing this setting doesn't result in an outage
* and the change is asynchronously applied as soon as possible.
*
*
* Constraints:
*
*
* -
*
* If supplied, must match existing DBSecurityGroups.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDbSecurityGroups} method.
*
*
* @return A list of DB security groups to authorize on this DB instance. Changing this setting doesn't result in an
* outage and the change is asynchronously applied as soon as possible.
*
* Constraints:
*
*
* -
*
* If supplied, must match existing DBSecurityGroups.
*
*
*/
public final List dbSecurityGroups() {
return dbSecurityGroups;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroupIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of EC2 VPC security groups to authorize on this DB instance. This change is asynchronously applied as soon
* as possible.
*
*
* Not applicable. The associated list of EC2 VPC security groups is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
*
* Constraints:
*
*
* -
*
* If supplied, must match existing VpcSecurityGroupIds.
*
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroupIds} method.
*
*
* @return A list of EC2 VPC security groups to authorize on this DB instance. This change is asynchronously applied
* as soon as possible.
*
* Not applicable. The associated list of EC2 VPC security groups is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
*
* Constraints:
*
*
* -
*
* If supplied, must match existing VpcSecurityGroupIds.
*
*
*/
public final List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* Specifies whether the modifications in this request and any pending modifications are asynchronously applied as
* soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB instance.
*
*
* If this parameter is set to false
, changes to the DB instance are applied during the next
* maintenance window. Some parameter changes can cause an outage and are applied on the next call to
* RebootDBInstance, or the next failure reboot.
*
*
* Default: false
*
*
* @return Specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* instance.
*
* If this parameter is set to false
, changes to the DB instance are applied during the next
* maintenance window. Some parameter changes can cause an outage and are applied on the next call to
* RebootDBInstance, or the next failure reboot.
*
*
* Default: false
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
*
* The name of the DB parameter group to apply to the DB instance. Changing this setting doesn't result in an
* outage. The parameter group name itself is changed immediately, but the actual parameter changes are not applied
* until you reboot the instance without failover. The db instance will NOT be rebooted automatically and the
* parameter changes will NOT be applied during the next maintenance window.
*
*
* Default: Uses existing setting
*
*
* Constraints: The DB parameter group must be in the same DB parameter group family as this DB instance.
*
*
* @return The name of the DB parameter group to apply to the DB instance. Changing this setting doesn't result in
* an outage. The parameter group name itself is changed immediately, but the actual parameter changes are
* not applied until you reboot the instance without failover. The db instance will NOT be rebooted
* automatically and the parameter changes will NOT be applied during the next maintenance window.
*
* Default: Uses existing setting
*
*
* Constraints: The DB parameter group must be in the same DB parameter group family as this DB instance.
*/
public final String dbParameterGroupName() {
return dbParameterGroupName;
}
/**
*
* Not applicable. The retention period for automated backups is managed by the DB cluster. For more information,
* see ModifyDBCluster.
*
*
* Default: Uses existing setting
*
*
* @return Not applicable. The retention period for automated backups is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
* Default: Uses existing setting
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled.
*
*
* Not applicable. The daily time range for creating automated backups is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
*
*
* -
*
* Must be in Universal Time Coordinated (UTC)
*
*
* -
*
* Must not conflict with the preferred maintenance window
*
*
* -
*
* Must be at least 30 minutes
*
*
*
*
* @return The daily time range during which automated backups are created if automated backups are enabled.
*
* Not applicable. The daily time range for creating automated backups is managed by the DB cluster. For
* more information, see ModifyDBCluster.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
*
*
* -
*
* Must be in Universal Time Coordinated (UTC)
*
*
* -
*
* Must not conflict with the preferred maintenance window
*
*
* -
*
* Must be at least 30 minutes
*
*
*/
public final String preferredBackupWindow() {
return preferredBackupWindow;
}
/**
*
* The weekly time range (in UTC) during which system maintenance can occur, which might result in an outage.
* Changing this parameter doesn't result in an outage, except in the following situation, and the change is
* asynchronously applied as soon as possible. If there are pending actions that cause a reboot, and the maintenance
* window is changed to include the current time, then changing this parameter will cause a reboot of the DB
* instance. If moving this window to the current time, there must be at least 30 minutes between the current time
* and end of the window to ensure pending changes are applied.
*
*
* Default: Uses existing setting
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes
*
*
* @return The weekly time range (in UTC) during which system maintenance can occur, which might result in an
* outage. Changing this parameter doesn't result in an outage, except in the following situation, and the
* change is asynchronously applied as soon as possible. If there are pending actions that cause a reboot,
* and the maintenance window is changed to include the current time, then changing this parameter will
* cause a reboot of the DB instance. If moving this window to the current time, there must be at least 30
* minutes between the current time and end of the window to ensure pending changes are applied.
*
* Default: Uses existing setting
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Valid Days: Mon | Tue | Wed | Thu | Fri | Sat | Sun
*
*
* Constraints: Must be at least 30 minutes
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* Specifies if the DB instance is a Multi-AZ deployment. Changing this parameter doesn't result in an outage and
* the change is applied during the next maintenance window unless the ApplyImmediately
parameter is
* set to true
for this request.
*
*
* @return Specifies if the DB instance is a Multi-AZ deployment. Changing this parameter doesn't result in an
* outage and the change is applied during the next maintenance window unless the
* ApplyImmediately
parameter is set to true
for this request.
*/
public final Boolean multiAZ() {
return multiAZ;
}
/**
*
* The version number of the database engine to upgrade to. Currently, setting this parameter has no effect. To
* upgrade your database engine to the most recent release, use the ApplyPendingMaintenanceAction API.
*
*
* @return The version number of the database engine to upgrade to. Currently, setting this parameter has no effect.
* To upgrade your database engine to the most recent release, use the ApplyPendingMaintenanceAction
* API.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* Indicates that major version upgrades are allowed. Changing this parameter doesn't result in an outage and the
* change is asynchronously applied as soon as possible.
*
*
* @return Indicates that major version upgrades are allowed. Changing this parameter doesn't result in an outage
* and the change is asynchronously applied as soon as possible.
*/
public final Boolean allowMajorVersionUpgrade() {
return allowMajorVersionUpgrade;
}
/**
*
* Indicates that minor version upgrades are applied automatically to the DB instance during the maintenance window.
* Changing this parameter doesn't result in an outage except in the following case and the change is asynchronously
* applied as soon as possible. An outage will result if this parameter is set to true
during the
* maintenance window, and a newer minor version is available, and Neptune has enabled auto patching for that engine
* version.
*
*
* @return Indicates that minor version upgrades are applied automatically to the DB instance during the maintenance
* window. Changing this parameter doesn't result in an outage except in the following case and the change
* is asynchronously applied as soon as possible. An outage will result if this parameter is set to
* true
during the maintenance window, and a newer minor version is available, and Neptune has
* enabled auto patching for that engine version.
*/
public final Boolean autoMinorVersionUpgrade() {
return autoMinorVersionUpgrade;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String licenseModel() {
return licenseModel;
}
/**
*
* The new Provisioned IOPS (I/O operations per second) value for the instance.
*
*
* Changing this setting doesn't result in an outage and the change is applied during the next maintenance window
* unless the ApplyImmediately
parameter is set to true
for this request.
*
*
* Default: Uses existing setting
*
*
* @return The new Provisioned IOPS (I/O operations per second) value for the instance.
*
* Changing this setting doesn't result in an outage and the change is applied during the next maintenance
* window unless the ApplyImmediately
parameter is set to true
for this request.
*
*
* Default: Uses existing setting
*/
public final Integer iops() {
return iops;
}
/**
*
* (Not supported by Neptune)
*
*
* @return (Not supported by Neptune)
*/
public final String optionGroupName() {
return optionGroupName;
}
/**
*
* The new DB instance identifier for the DB instance when renaming a DB instance. When you change the DB instance
* identifier, an instance reboot will occur immediately if you set Apply Immediately
to true, or will
* occur during the next maintenance window if Apply Immediately
to false. This value is stored as a
* lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: mydbinstance
*
*
* @return The new DB instance identifier for the DB instance when renaming a DB instance. When you change the DB
* instance identifier, an instance reboot will occur immediately if you set Apply Immediately
* to true, or will occur during the next maintenance window if Apply Immediately
to false.
* This value is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: mydbinstance
*/
public final String newDBInstanceIdentifier() {
return newDBInstanceIdentifier;
}
/**
*
* Not supported.
*
*
* @return Not supported.
*/
public final String storageType() {
return storageType;
}
/**
*
* The ARN from the key store with which to associate the instance for TDE encryption.
*
*
* @return The ARN from the key store with which to associate the instance for TDE encryption.
*/
public final String tdeCredentialArn() {
return tdeCredentialArn;
}
/**
*
* The password for the given ARN from the key store in order to access the device.
*
*
* @return The password for the given ARN from the key store in order to access the device.
*/
public final String tdeCredentialPassword() {
return tdeCredentialPassword;
}
/**
*
* Indicates the certificate that needs to be associated with the instance.
*
*
* @return Indicates the certificate that needs to be associated with the instance.
*/
public final String caCertificateIdentifier() {
return caCertificateIdentifier;
}
/**
*
* Not supported.
*
*
* @return Not supported.
*/
public final String domain() {
return domain;
}
/**
*
* True to copy all tags from the DB instance to snapshots of the DB instance, and otherwise false. The default is
* false.
*
*
* @return True to copy all tags from the DB instance to snapshots of the DB instance, and otherwise false. The
* default is false.
*/
public final Boolean copyTagsToSnapshot() {
return copyTagsToSnapshot;
}
/**
*
* The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB instance. To
* disable collecting Enhanced Monitoring metrics, specify 0. The default is 0.
*
*
* If MonitoringRoleArn
is specified, then you must also set MonitoringInterval
to a value
* other than 0.
*
*
* Valid Values: 0, 1, 5, 10, 15, 30, 60
*
*
* @return The interval, in seconds, between points when Enhanced Monitoring metrics are collected for the DB
* instance. To disable collecting Enhanced Monitoring metrics, specify 0. The default is 0.
*
* If MonitoringRoleArn
is specified, then you must also set MonitoringInterval
to
* a value other than 0.
*
*
* Valid Values: 0, 1, 5, 10, 15, 30, 60
*/
public final Integer monitoringInterval() {
return monitoringInterval;
}
/**
*
* The port number on which the database accepts connections.
*
*
* The value of the DBPortNumber
parameter must not match any of the port values specified for options
* in the option group for the DB instance.
*
*
* Your database will restart when you change the DBPortNumber
value regardless of the value of the
* ApplyImmediately
parameter.
*
*
* Default: 8182
*
*
* @return The port number on which the database accepts connections.
*
* The value of the DBPortNumber
parameter must not match any of the port values specified for
* options in the option group for the DB instance.
*
*
* Your database will restart when you change the DBPortNumber
value regardless of the value of
* the ApplyImmediately
parameter.
*
*
* Default: 8182
*/
public final Integer dbPortNumber() {
return dbPortNumber;
}
/**
*
* This flag should no longer be used.
*
*
* @return This flag should no longer be used.
* @deprecated
*/
@Deprecated
public final Boolean publiclyAccessible() {
return publiclyAccessible;
}
/**
*
* The ARN for the IAM role that permits Neptune to send enhanced monitoring metrics to Amazon CloudWatch Logs. For
* example, arn:aws:iam:123456789012:role/emaccess
.
*
*
* If MonitoringInterval
is set to a value other than 0, then you must supply a
* MonitoringRoleArn
value.
*
*
* @return The ARN for the IAM role that permits Neptune to send enhanced monitoring metrics to Amazon CloudWatch
* Logs. For example, arn:aws:iam:123456789012:role/emaccess
.
*
* If MonitoringInterval
is set to a value other than 0, then you must supply a
* MonitoringRoleArn
value.
*/
public final String monitoringRoleArn() {
return monitoringRoleArn;
}
/**
*
* Not supported
*
*
* @return Not supported
*/
public final String domainIAMRoleName() {
return domainIAMRoleName;
}
/**
*
* A value that specifies the order in which a Read Replica is promoted to the primary instance after a failure of
* the existing primary instance.
*
*
* Default: 1
*
*
* Valid Values: 0 - 15
*
*
* @return A value that specifies the order in which a Read Replica is promoted to the primary instance after a
* failure of the existing primary instance.
*
* Default: 1
*
*
* Valid Values: 0 - 15
*/
public final Integer promotionTier() {
return promotionTier;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* You can enable IAM database authentication for the following database engines
*
*
* Not applicable. Mapping Amazon IAM accounts to database accounts is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
*
* Default: false
*
*
* @return True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* You can enable IAM database authentication for the following database engines
*
*
* Not applicable. Mapping Amazon IAM accounts to database accounts is managed by the DB cluster. For more
* information, see ModifyDBCluster.
*
*
* Default: false
*/
public final Boolean enableIAMDatabaseAuthentication() {
return enableIAMDatabaseAuthentication;
}
/**
*
* (Not supported by Neptune)
*
*
* @return (Not supported by Neptune)
*/
public final Boolean enablePerformanceInsights() {
return enablePerformanceInsights;
}
/**
*
* (Not supported by Neptune)
*
*
* @return (Not supported by Neptune)
*/
public final String performanceInsightsKMSKeyId() {
return performanceInsightsKMSKeyId;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* instance or DB cluster.
*
*
* @return The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* instance or DB cluster.
*/
public final CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration() {
return cloudwatchLogsExportConfiguration;
}
/**
*
* A value that indicates whether the DB instance has deletion protection enabled. The database can't be deleted
* when deletion protection is enabled. By default, deletion protection is disabled. See Deleting a DB
* Instance.
*
*
* @return A value that indicates whether the DB instance has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled. See Deleting
* a DB Instance.
*/
public final Boolean deletionProtection() {
return deletionProtection;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceClass());
hashCode = 31 * hashCode + Objects.hashCode(dbSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(hasDbSecurityGroups() ? dbSecurityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(dbParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(preferredBackupWindow());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(allowMajorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(autoMinorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(licenseModel());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(optionGroupName());
hashCode = 31 * hashCode + Objects.hashCode(newDBInstanceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(storageType());
hashCode = 31 * hashCode + Objects.hashCode(tdeCredentialArn());
hashCode = 31 * hashCode + Objects.hashCode(tdeCredentialPassword());
hashCode = 31 * hashCode + Objects.hashCode(caCertificateIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(domain());
hashCode = 31 * hashCode + Objects.hashCode(copyTagsToSnapshot());
hashCode = 31 * hashCode + Objects.hashCode(monitoringInterval());
hashCode = 31 * hashCode + Objects.hashCode(dbPortNumber());
hashCode = 31 * hashCode + Objects.hashCode(publiclyAccessible());
hashCode = 31 * hashCode + Objects.hashCode(monitoringRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(domainIAMRoleName());
hashCode = 31 * hashCode + Objects.hashCode(promotionTier());
hashCode = 31 * hashCode + Objects.hashCode(enableIAMDatabaseAuthentication());
hashCode = 31 * hashCode + Objects.hashCode(enablePerformanceInsights());
hashCode = 31 * hashCode + Objects.hashCode(performanceInsightsKMSKeyId());
hashCode = 31 * hashCode + Objects.hashCode(cloudwatchLogsExportConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(deletionProtection());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyDbInstanceRequest)) {
return false;
}
ModifyDbInstanceRequest other = (ModifyDbInstanceRequest) obj;
return Objects.equals(dbInstanceIdentifier(), other.dbInstanceIdentifier())
&& Objects.equals(allocatedStorage(), other.allocatedStorage())
&& Objects.equals(dbInstanceClass(), other.dbInstanceClass())
&& Objects.equals(dbSubnetGroupName(), other.dbSubnetGroupName())
&& hasDbSecurityGroups() == other.hasDbSecurityGroups()
&& Objects.equals(dbSecurityGroups(), other.dbSecurityGroups())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& Objects.equals(masterUserPassword(), other.masterUserPassword())
&& Objects.equals(dbParameterGroupName(), other.dbParameterGroupName())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(preferredBackupWindow(), other.preferredBackupWindow())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(multiAZ(), other.multiAZ()) && Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(allowMajorVersionUpgrade(), other.allowMajorVersionUpgrade())
&& Objects.equals(autoMinorVersionUpgrade(), other.autoMinorVersionUpgrade())
&& Objects.equals(licenseModel(), other.licenseModel()) && Objects.equals(iops(), other.iops())
&& Objects.equals(optionGroupName(), other.optionGroupName())
&& Objects.equals(newDBInstanceIdentifier(), other.newDBInstanceIdentifier())
&& Objects.equals(storageType(), other.storageType())
&& Objects.equals(tdeCredentialArn(), other.tdeCredentialArn())
&& Objects.equals(tdeCredentialPassword(), other.tdeCredentialPassword())
&& Objects.equals(caCertificateIdentifier(), other.caCertificateIdentifier())
&& Objects.equals(domain(), other.domain()) && Objects.equals(copyTagsToSnapshot(), other.copyTagsToSnapshot())
&& Objects.equals(monitoringInterval(), other.monitoringInterval())
&& Objects.equals(dbPortNumber(), other.dbPortNumber())
&& Objects.equals(publiclyAccessible(), other.publiclyAccessible())
&& Objects.equals(monitoringRoleArn(), other.monitoringRoleArn())
&& Objects.equals(domainIAMRoleName(), other.domainIAMRoleName())
&& Objects.equals(promotionTier(), other.promotionTier())
&& Objects.equals(enableIAMDatabaseAuthentication(), other.enableIAMDatabaseAuthentication())
&& Objects.equals(enablePerformanceInsights(), other.enablePerformanceInsights())
&& Objects.equals(performanceInsightsKMSKeyId(), other.performanceInsightsKMSKeyId())
&& Objects.equals(cloudwatchLogsExportConfiguration(), other.cloudwatchLogsExportConfiguration())
&& Objects.equals(deletionProtection(), other.deletionProtection());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyDbInstanceRequest").add("DBInstanceIdentifier", dbInstanceIdentifier())
.add("AllocatedStorage", allocatedStorage()).add("DBInstanceClass", dbInstanceClass())
.add("DBSubnetGroupName", dbSubnetGroupName())
.add("DBSecurityGroups", hasDbSecurityGroups() ? dbSecurityGroups() : null)
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null)
.add("ApplyImmediately", applyImmediately()).add("MasterUserPassword", masterUserPassword())
.add("DBParameterGroupName", dbParameterGroupName()).add("BackupRetentionPeriod", backupRetentionPeriod())
.add("PreferredBackupWindow", preferredBackupWindow())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow()).add("MultiAZ", multiAZ())
.add("EngineVersion", engineVersion()).add("AllowMajorVersionUpgrade", allowMajorVersionUpgrade())
.add("AutoMinorVersionUpgrade", autoMinorVersionUpgrade()).add("LicenseModel", licenseModel())
.add("Iops", iops()).add("OptionGroupName", optionGroupName())
.add("NewDBInstanceIdentifier", newDBInstanceIdentifier()).add("StorageType", storageType())
.add("TdeCredentialArn", tdeCredentialArn()).add("TdeCredentialPassword", tdeCredentialPassword())
.add("CACertificateIdentifier", caCertificateIdentifier()).add("Domain", domain())
.add("CopyTagsToSnapshot", copyTagsToSnapshot()).add("MonitoringInterval", monitoringInterval())
.add("DBPortNumber", dbPortNumber()).add("PubliclyAccessible", publiclyAccessible())
.add("MonitoringRoleArn", monitoringRoleArn()).add("DomainIAMRoleName", domainIAMRoleName())
.add("PromotionTier", promotionTier()).add("EnableIAMDatabaseAuthentication", enableIAMDatabaseAuthentication())
.add("EnablePerformanceInsights", enablePerformanceInsights())
.add("PerformanceInsightsKMSKeyId", performanceInsightsKMSKeyId())
.add("CloudwatchLogsExportConfiguration", cloudwatchLogsExportConfiguration())
.add("DeletionProtection", deletionProtection()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBInstanceIdentifier":
return Optional.ofNullable(clazz.cast(dbInstanceIdentifier()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "DBInstanceClass":
return Optional.ofNullable(clazz.cast(dbInstanceClass()));
case "DBSubnetGroupName":
return Optional.ofNullable(clazz.cast(dbSubnetGroupName()));
case "DBSecurityGroups":
return Optional.ofNullable(clazz.cast(dbSecurityGroups()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "DBParameterGroupName":
return Optional.ofNullable(clazz.cast(dbParameterGroupName()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "PreferredBackupWindow":
return Optional.ofNullable(clazz.cast(preferredBackupWindow()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AllowMajorVersionUpgrade":
return Optional.ofNullable(clazz.cast(allowMajorVersionUpgrade()));
case "AutoMinorVersionUpgrade":
return Optional.ofNullable(clazz.cast(autoMinorVersionUpgrade()));
case "LicenseModel":
return Optional.ofNullable(clazz.cast(licenseModel()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "OptionGroupName":
return Optional.ofNullable(clazz.cast(optionGroupName()));
case "NewDBInstanceIdentifier":
return Optional.ofNullable(clazz.cast(newDBInstanceIdentifier()));
case "StorageType":
return Optional.ofNullable(clazz.cast(storageType()));
case "TdeCredentialArn":
return Optional.ofNullable(clazz.cast(tdeCredentialArn()));
case "TdeCredentialPassword":
return Optional.ofNullable(clazz.cast(tdeCredentialPassword()));
case "CACertificateIdentifier":
return Optional.ofNullable(clazz.cast(caCertificateIdentifier()));
case "Domain":
return Optional.ofNullable(clazz.cast(domain()));
case "CopyTagsToSnapshot":
return Optional.ofNullable(clazz.cast(copyTagsToSnapshot()));
case "MonitoringInterval":
return Optional.ofNullable(clazz.cast(monitoringInterval()));
case "DBPortNumber":
return Optional.ofNullable(clazz.cast(dbPortNumber()));
case "PubliclyAccessible":
return Optional.ofNullable(clazz.cast(publiclyAccessible()));
case "MonitoringRoleArn":
return Optional.ofNullable(clazz.cast(monitoringRoleArn()));
case "DomainIAMRoleName":
return Optional.ofNullable(clazz.cast(domainIAMRoleName()));
case "PromotionTier":
return Optional.ofNullable(clazz.cast(promotionTier()));
case "EnableIAMDatabaseAuthentication":
return Optional.ofNullable(clazz.cast(enableIAMDatabaseAuthentication()));
case "EnablePerformanceInsights":
return Optional.ofNullable(clazz.cast(enablePerformanceInsights()));
case "PerformanceInsightsKMSKeyId":
return Optional.ofNullable(clazz.cast(performanceInsightsKMSKeyId()));
case "CloudwatchLogsExportConfiguration":
return Optional.ofNullable(clazz.cast(cloudwatchLogsExportConfiguration()));
case "DeletionProtection":
return Optional.ofNullable(clazz.cast(deletionProtection()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function