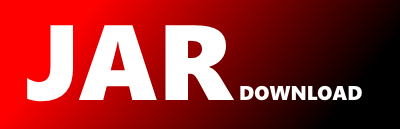
software.amazon.awssdk.services.neptune.model.PendingModifiedValues Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This data type is used as a response element in the ModifyDBInstance action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PendingModifiedValues implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DB_INSTANCE_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceClass").getter(getter(PendingModifiedValues::dbInstanceClass))
.setter(setter(Builder::dbInstanceClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceClass").build()).build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(PendingModifiedValues::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(PendingModifiedValues::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(PendingModifiedValues::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(PendingModifiedValues::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField MULTI_AZ_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiAZ").getter(getter(PendingModifiedValues::multiAZ)).setter(setter(Builder::multiAZ))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiAZ").build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(PendingModifiedValues::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField LICENSE_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LicenseModel").getter(getter(PendingModifiedValues::licenseModel)).setter(setter(Builder::licenseModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LicenseModel").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(PendingModifiedValues::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final SdkField DB_INSTANCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBInstanceIdentifier").getter(getter(PendingModifiedValues::dbInstanceIdentifier))
.setter(setter(Builder::dbInstanceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceIdentifier").build())
.build();
private static final SdkField STORAGE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StorageType").getter(getter(PendingModifiedValues::storageType)).setter(setter(Builder::storageType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageType").build()).build();
private static final SdkField CA_CERTIFICATE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CACertificateIdentifier").getter(getter(PendingModifiedValues::caCertificateIdentifier))
.setter(setter(Builder::caCertificateIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CACertificateIdentifier").build())
.build();
private static final SdkField DB_SUBNET_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBSubnetGroupName").getter(getter(PendingModifiedValues::dbSubnetGroupName))
.setter(setter(Builder::dbSubnetGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBSubnetGroupName").build()).build();
private static final SdkField PENDING_CLOUDWATCH_LOGS_EXPORTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("PendingCloudwatchLogsExports")
.getter(getter(PendingModifiedValues::pendingCloudwatchLogsExports))
.setter(setter(Builder::pendingCloudwatchLogsExports))
.constructor(PendingCloudwatchLogsExports::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingCloudwatchLogsExports")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_INSTANCE_CLASS_FIELD,
ALLOCATED_STORAGE_FIELD, MASTER_USER_PASSWORD_FIELD, PORT_FIELD, BACKUP_RETENTION_PERIOD_FIELD, MULTI_AZ_FIELD,
ENGINE_VERSION_FIELD, LICENSE_MODEL_FIELD, IOPS_FIELD, DB_INSTANCE_IDENTIFIER_FIELD, STORAGE_TYPE_FIELD,
CA_CERTIFICATE_IDENTIFIER_FIELD, DB_SUBNET_GROUP_NAME_FIELD, PENDING_CLOUDWATCH_LOGS_EXPORTS_FIELD));
private static final long serialVersionUID = 1L;
private final String dbInstanceClass;
private final Integer allocatedStorage;
private final String masterUserPassword;
private final Integer port;
private final Integer backupRetentionPeriod;
private final Boolean multiAZ;
private final String engineVersion;
private final String licenseModel;
private final Integer iops;
private final String dbInstanceIdentifier;
private final String storageType;
private final String caCertificateIdentifier;
private final String dbSubnetGroupName;
private final PendingCloudwatchLogsExports pendingCloudwatchLogsExports;
private PendingModifiedValues(BuilderImpl builder) {
this.dbInstanceClass = builder.dbInstanceClass;
this.allocatedStorage = builder.allocatedStorage;
this.masterUserPassword = builder.masterUserPassword;
this.port = builder.port;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.multiAZ = builder.multiAZ;
this.engineVersion = builder.engineVersion;
this.licenseModel = builder.licenseModel;
this.iops = builder.iops;
this.dbInstanceIdentifier = builder.dbInstanceIdentifier;
this.storageType = builder.storageType;
this.caCertificateIdentifier = builder.caCertificateIdentifier;
this.dbSubnetGroupName = builder.dbSubnetGroupName;
this.pendingCloudwatchLogsExports = builder.pendingCloudwatchLogsExports;
}
/**
*
* Contains the new DBInstanceClass
for the DB instance that will be applied or is currently being
* applied.
*
*
* @return Contains the new DBInstanceClass
for the DB instance that will be applied or is currently
* being applied.
*/
public final String dbInstanceClass() {
return dbInstanceClass;
}
/**
*
* Contains the new AllocatedStorage
size for the DB instance that will be applied or is currently
* being applied.
*
*
* @return Contains the new AllocatedStorage
size for the DB instance that will be applied or is
* currently being applied.
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
*
* Specifies the pending port for the DB instance.
*
*
* @return Specifies the pending port for the DB instance.
*/
public final Integer port() {
return port;
}
/**
*
* Specifies the pending number of days for which automated backups are retained.
*
*
* @return Specifies the pending number of days for which automated backups are retained.
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* Indicates that the Single-AZ DB instance is to change to a Multi-AZ deployment.
*
*
* @return Indicates that the Single-AZ DB instance is to change to a Multi-AZ deployment.
*/
public final Boolean multiAZ() {
return multiAZ;
}
/**
*
* Indicates the database engine version.
*
*
* @return Indicates the database engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String licenseModel() {
return licenseModel;
}
/**
*
* Specifies the new Provisioned IOPS value for the DB instance that will be applied or is currently being applied.
*
*
* @return Specifies the new Provisioned IOPS value for the DB instance that will be applied or is currently being
* applied.
*/
public final Integer iops() {
return iops;
}
/**
*
* Contains the new DBInstanceIdentifier
for the DB instance that will be applied or is currently being
* applied.
*
*
* @return Contains the new DBInstanceIdentifier
for the DB instance that will be applied or is
* currently being applied.
*/
public final String dbInstanceIdentifier() {
return dbInstanceIdentifier;
}
/**
*
* Specifies the storage type to be associated with the DB instance.
*
*
* @return Specifies the storage type to be associated with the DB instance.
*/
public final String storageType() {
return storageType;
}
/**
*
* Specifies the identifier of the CA certificate for the DB instance.
*
*
* @return Specifies the identifier of the CA certificate for the DB instance.
*/
public final String caCertificateIdentifier() {
return caCertificateIdentifier;
}
/**
*
* The new DB subnet group for the DB instance.
*
*
* @return The new DB subnet group for the DB instance.
*/
public final String dbSubnetGroupName() {
return dbSubnetGroupName;
}
/**
*
* This PendingCloudwatchLogsExports
structure specifies pending changes to which CloudWatch logs are
* enabled and which are disabled.
*
*
* @return This PendingCloudwatchLogsExports
structure specifies pending changes to which CloudWatch
* logs are enabled and which are disabled.
*/
public final PendingCloudwatchLogsExports pendingCloudwatchLogsExports() {
return pendingCloudwatchLogsExports;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceClass());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(multiAZ());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(licenseModel());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(storageType());
hashCode = 31 * hashCode + Objects.hashCode(caCertificateIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(dbSubnetGroupName());
hashCode = 31 * hashCode + Objects.hashCode(pendingCloudwatchLogsExports());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PendingModifiedValues)) {
return false;
}
PendingModifiedValues other = (PendingModifiedValues) obj;
return Objects.equals(dbInstanceClass(), other.dbInstanceClass())
&& Objects.equals(allocatedStorage(), other.allocatedStorage())
&& Objects.equals(masterUserPassword(), other.masterUserPassword()) && Objects.equals(port(), other.port())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(multiAZ(), other.multiAZ()) && Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(licenseModel(), other.licenseModel()) && Objects.equals(iops(), other.iops())
&& Objects.equals(dbInstanceIdentifier(), other.dbInstanceIdentifier())
&& Objects.equals(storageType(), other.storageType())
&& Objects.equals(caCertificateIdentifier(), other.caCertificateIdentifier())
&& Objects.equals(dbSubnetGroupName(), other.dbSubnetGroupName())
&& Objects.equals(pendingCloudwatchLogsExports(), other.pendingCloudwatchLogsExports());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PendingModifiedValues").add("DBInstanceClass", dbInstanceClass())
.add("AllocatedStorage", allocatedStorage()).add("MasterUserPassword", masterUserPassword()).add("Port", port())
.add("BackupRetentionPeriod", backupRetentionPeriod()).add("MultiAZ", multiAZ())
.add("EngineVersion", engineVersion()).add("LicenseModel", licenseModel()).add("Iops", iops())
.add("DBInstanceIdentifier", dbInstanceIdentifier()).add("StorageType", storageType())
.add("CACertificateIdentifier", caCertificateIdentifier()).add("DBSubnetGroupName", dbSubnetGroupName())
.add("PendingCloudwatchLogsExports", pendingCloudwatchLogsExports()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBInstanceClass":
return Optional.ofNullable(clazz.cast(dbInstanceClass()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "MultiAZ":
return Optional.ofNullable(clazz.cast(multiAZ()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "LicenseModel":
return Optional.ofNullable(clazz.cast(licenseModel()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "DBInstanceIdentifier":
return Optional.ofNullable(clazz.cast(dbInstanceIdentifier()));
case "StorageType":
return Optional.ofNullable(clazz.cast(storageType()));
case "CACertificateIdentifier":
return Optional.ofNullable(clazz.cast(caCertificateIdentifier()));
case "DBSubnetGroupName":
return Optional.ofNullable(clazz.cast(dbSubnetGroupName()));
case "PendingCloudwatchLogsExports":
return Optional.ofNullable(clazz.cast(pendingCloudwatchLogsExports()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function