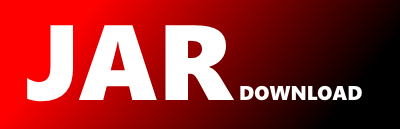
software.amazon.awssdk.services.neptune.model.ClusterPendingModifiedValues Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* This data type is used as a response element in the ModifyDBCluster
operation and contains changes that
* will be applied during the next maintenance window.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ClusterPendingModifiedValues implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField PENDING_CLOUDWATCH_LOGS_EXPORTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("PendingCloudwatchLogsExports")
.getter(getter(ClusterPendingModifiedValues::pendingCloudwatchLogsExports))
.setter(setter(Builder::pendingCloudwatchLogsExports))
.constructor(PendingCloudwatchLogsExports::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PendingCloudwatchLogsExports")
.build()).build();
private static final SdkField DB_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterIdentifier").getter(getter(ClusterPendingModifiedValues::dbClusterIdentifier))
.setter(setter(Builder::dbClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterIdentifier").build())
.build();
private static final SdkField IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("IAMDatabaseAuthenticationEnabled")
.getter(getter(ClusterPendingModifiedValues::iamDatabaseAuthenticationEnabled))
.setter(setter(Builder::iamDatabaseAuthenticationEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IAMDatabaseAuthenticationEnabled")
.build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ClusterPendingModifiedValues::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(ClusterPendingModifiedValues::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField ALLOCATED_STORAGE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("AllocatedStorage").getter(getter(ClusterPendingModifiedValues::allocatedStorage))
.setter(setter(Builder::allocatedStorage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllocatedStorage").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(ClusterPendingModifiedValues::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
PENDING_CLOUDWATCH_LOGS_EXPORTS_FIELD, DB_CLUSTER_IDENTIFIER_FIELD, IAM_DATABASE_AUTHENTICATION_ENABLED_FIELD,
ENGINE_VERSION_FIELD, BACKUP_RETENTION_PERIOD_FIELD, ALLOCATED_STORAGE_FIELD, IOPS_FIELD));
private static final long serialVersionUID = 1L;
private final PendingCloudwatchLogsExports pendingCloudwatchLogsExports;
private final String dbClusterIdentifier;
private final Boolean iamDatabaseAuthenticationEnabled;
private final String engineVersion;
private final Integer backupRetentionPeriod;
private final Integer allocatedStorage;
private final Integer iops;
private ClusterPendingModifiedValues(BuilderImpl builder) {
this.pendingCloudwatchLogsExports = builder.pendingCloudwatchLogsExports;
this.dbClusterIdentifier = builder.dbClusterIdentifier;
this.iamDatabaseAuthenticationEnabled = builder.iamDatabaseAuthenticationEnabled;
this.engineVersion = builder.engineVersion;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.allocatedStorage = builder.allocatedStorage;
this.iops = builder.iops;
}
/**
*
* This PendingCloudwatchLogsExports
structure specifies pending changes to which CloudWatch logs are
* enabled and which are disabled.
*
*
* @return This PendingCloudwatchLogsExports
structure specifies pending changes to which CloudWatch
* logs are enabled and which are disabled.
*/
public final PendingCloudwatchLogsExports pendingCloudwatchLogsExports() {
return pendingCloudwatchLogsExports;
}
/**
*
* The DBClusterIdentifier value for the DB cluster.
*
*
* @return The DBClusterIdentifier value for the DB cluster.
*/
public final String dbClusterIdentifier() {
return dbClusterIdentifier;
}
/**
*
* A value that indicates whether mapping of Amazon Web Services Identity and Access Management (IAM) accounts to
* database accounts is enabled.
*
*
* @return A value that indicates whether mapping of Amazon Web Services Identity and Access Management (IAM)
* accounts to database accounts is enabled.
*/
public final Boolean iamDatabaseAuthenticationEnabled() {
return iamDatabaseAuthenticationEnabled;
}
/**
*
* The database engine version.
*
*
* @return The database engine version.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* The number of days for which automatic DB snapshots are retained.
*
*
* @return The number of days for which automatic DB snapshots are retained.
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* The allocated storage size in gibibytes (GiB) for database engines. For Neptune, AllocatedStorage
* always returns 1, because Neptune DB cluster storage size isn't fixed, but instead automatically adjusts as
* needed.
*
*
* @return The allocated storage size in gibibytes (GiB) for database engines. For Neptune,
* AllocatedStorage
always returns 1, because Neptune DB cluster storage size isn't fixed, but
* instead automatically adjusts as needed.
*/
public final Integer allocatedStorage() {
return allocatedStorage;
}
/**
*
* The Provisioned IOPS (I/O operations per second) value. This setting is only for non-Aurora Multi-AZ DB clusters.
*
*
* @return The Provisioned IOPS (I/O operations per second) value. This setting is only for non-Aurora Multi-AZ DB
* clusters.
*/
public final Integer iops() {
return iops;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(pendingCloudwatchLogsExports());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(iamDatabaseAuthenticationEnabled());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(allocatedStorage());
hashCode = 31 * hashCode + Objects.hashCode(iops());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ClusterPendingModifiedValues)) {
return false;
}
ClusterPendingModifiedValues other = (ClusterPendingModifiedValues) obj;
return Objects.equals(pendingCloudwatchLogsExports(), other.pendingCloudwatchLogsExports())
&& Objects.equals(dbClusterIdentifier(), other.dbClusterIdentifier())
&& Objects.equals(iamDatabaseAuthenticationEnabled(), other.iamDatabaseAuthenticationEnabled())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(allocatedStorage(), other.allocatedStorage()) && Objects.equals(iops(), other.iops());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ClusterPendingModifiedValues")
.add("PendingCloudwatchLogsExports", pendingCloudwatchLogsExports())
.add("DBClusterIdentifier", dbClusterIdentifier())
.add("IAMDatabaseAuthenticationEnabled", iamDatabaseAuthenticationEnabled())
.add("EngineVersion", engineVersion()).add("BackupRetentionPeriod", backupRetentionPeriod())
.add("AllocatedStorage", allocatedStorage()).add("Iops", iops()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "PendingCloudwatchLogsExports":
return Optional.ofNullable(clazz.cast(pendingCloudwatchLogsExports()));
case "DBClusterIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterIdentifier()));
case "IAMDatabaseAuthenticationEnabled":
return Optional.ofNullable(clazz.cast(iamDatabaseAuthenticationEnabled()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "AllocatedStorage":
return Optional.ofNullable(clazz.cast(allocatedStorage()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function