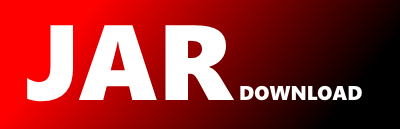
software.amazon.awssdk.services.neptune.model.ModifyDbClusterRequest Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyDbClusterRequest extends NeptuneRequest implements
ToCopyableBuilder {
private static final SdkField DB_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DBClusterIdentifier").getter(getter(ModifyDbClusterRequest::dbClusterIdentifier))
.setter(setter(Builder::dbClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterIdentifier").build())
.build();
private static final SdkField NEW_DB_CLUSTER_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NewDBClusterIdentifier").getter(getter(ModifyDbClusterRequest::newDBClusterIdentifier))
.setter(setter(Builder::newDBClusterIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NewDBClusterIdentifier").build())
.build();
private static final SdkField APPLY_IMMEDIATELY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("ApplyImmediately").getter(getter(ModifyDbClusterRequest::applyImmediately))
.setter(setter(Builder::applyImmediately))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyImmediately").build()).build();
private static final SdkField BACKUP_RETENTION_PERIOD_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BackupRetentionPeriod").getter(getter(ModifyDbClusterRequest::backupRetentionPeriod))
.setter(setter(Builder::backupRetentionPeriod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BackupRetentionPeriod").build())
.build();
private static final SdkField DB_CLUSTER_PARAMETER_GROUP_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DBClusterParameterGroupName")
.getter(getter(ModifyDbClusterRequest::dbClusterParameterGroupName))
.setter(setter(Builder::dbClusterParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterParameterGroupName")
.build()).build();
private static final SdkField> VPC_SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("VpcSecurityGroupIds")
.getter(getter(ModifyDbClusterRequest::vpcSecurityGroupIds))
.setter(setter(Builder::vpcSecurityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VpcSecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName("VpcSecurityGroupId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("VpcSecurityGroupId").build()).build()).build()).build();
private static final SdkField PORT_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Port")
.getter(getter(ModifyDbClusterRequest::port)).setter(setter(Builder::port))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Port").build()).build();
private static final SdkField MASTER_USER_PASSWORD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterUserPassword").getter(getter(ModifyDbClusterRequest::masterUserPassword))
.setter(setter(Builder::masterUserPassword))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterUserPassword").build())
.build();
private static final SdkField OPTION_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OptionGroupName").getter(getter(ModifyDbClusterRequest::optionGroupName))
.setter(setter(Builder::optionGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptionGroupName").build()).build();
private static final SdkField PREFERRED_BACKUP_WINDOW_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PreferredBackupWindow").getter(getter(ModifyDbClusterRequest::preferredBackupWindow))
.setter(setter(Builder::preferredBackupWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredBackupWindow").build())
.build();
private static final SdkField PREFERRED_MAINTENANCE_WINDOW_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("PreferredMaintenanceWindow")
.getter(getter(ModifyDbClusterRequest::preferredMaintenanceWindow))
.setter(setter(Builder::preferredMaintenanceWindow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PreferredMaintenanceWindow").build())
.build();
private static final SdkField ENABLE_IAM_DATABASE_AUTHENTICATION_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("EnableIAMDatabaseAuthentication")
.getter(getter(ModifyDbClusterRequest::enableIAMDatabaseAuthentication))
.setter(setter(Builder::enableIAMDatabaseAuthentication))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnableIAMDatabaseAuthentication")
.build()).build();
private static final SdkField CLOUDWATCH_LOGS_EXPORT_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("CloudwatchLogsExportConfiguration")
.getter(getter(ModifyDbClusterRequest::cloudwatchLogsExportConfiguration))
.setter(setter(Builder::cloudwatchLogsExportConfiguration))
.constructor(CloudwatchLogsExportConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CloudwatchLogsExportConfiguration")
.build()).build();
private static final SdkField ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EngineVersion").getter(getter(ModifyDbClusterRequest::engineVersion))
.setter(setter(Builder::engineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EngineVersion").build()).build();
private static final SdkField ALLOW_MAJOR_VERSION_UPGRADE_FIELD = SdkField
. builder(MarshallingType.BOOLEAN).memberName("AllowMajorVersionUpgrade")
.getter(getter(ModifyDbClusterRequest::allowMajorVersionUpgrade)).setter(setter(Builder::allowMajorVersionUpgrade))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowMajorVersionUpgrade").build())
.build();
private static final SdkField DB_INSTANCE_PARAMETER_GROUP_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DBInstanceParameterGroupName")
.getter(getter(ModifyDbClusterRequest::dbInstanceParameterGroupName))
.setter(setter(Builder::dbInstanceParameterGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBInstanceParameterGroupName")
.build()).build();
private static final SdkField DELETION_PROTECTION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DeletionProtection").getter(getter(ModifyDbClusterRequest::deletionProtection))
.setter(setter(Builder::deletionProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeletionProtection").build())
.build();
private static final SdkField COPY_TAGS_TO_SNAPSHOT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("CopyTagsToSnapshot").getter(getter(ModifyDbClusterRequest::copyTagsToSnapshot))
.setter(setter(Builder::copyTagsToSnapshot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CopyTagsToSnapshot").build())
.build();
private static final SdkField SERVERLESS_V2_SCALING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("ServerlessV2ScalingConfiguration")
.getter(getter(ModifyDbClusterRequest::serverlessV2ScalingConfiguration))
.setter(setter(Builder::serverlessV2ScalingConfiguration))
.constructor(ServerlessV2ScalingConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ServerlessV2ScalingConfiguration")
.build()).build();
private static final SdkField STORAGE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StorageType").getter(getter(ModifyDbClusterRequest::storageType)).setter(setter(Builder::storageType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StorageType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DB_CLUSTER_IDENTIFIER_FIELD,
NEW_DB_CLUSTER_IDENTIFIER_FIELD, APPLY_IMMEDIATELY_FIELD, BACKUP_RETENTION_PERIOD_FIELD,
DB_CLUSTER_PARAMETER_GROUP_NAME_FIELD, VPC_SECURITY_GROUP_IDS_FIELD, PORT_FIELD, MASTER_USER_PASSWORD_FIELD,
OPTION_GROUP_NAME_FIELD, PREFERRED_BACKUP_WINDOW_FIELD, PREFERRED_MAINTENANCE_WINDOW_FIELD,
ENABLE_IAM_DATABASE_AUTHENTICATION_FIELD, CLOUDWATCH_LOGS_EXPORT_CONFIGURATION_FIELD, ENGINE_VERSION_FIELD,
ALLOW_MAJOR_VERSION_UPGRADE_FIELD, DB_INSTANCE_PARAMETER_GROUP_NAME_FIELD, DELETION_PROTECTION_FIELD,
COPY_TAGS_TO_SNAPSHOT_FIELD, SERVERLESS_V2_SCALING_CONFIGURATION_FIELD, STORAGE_TYPE_FIELD));
private final String dbClusterIdentifier;
private final String newDBClusterIdentifier;
private final Boolean applyImmediately;
private final Integer backupRetentionPeriod;
private final String dbClusterParameterGroupName;
private final List vpcSecurityGroupIds;
private final Integer port;
private final String masterUserPassword;
private final String optionGroupName;
private final String preferredBackupWindow;
private final String preferredMaintenanceWindow;
private final Boolean enableIAMDatabaseAuthentication;
private final CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration;
private final String engineVersion;
private final Boolean allowMajorVersionUpgrade;
private final String dbInstanceParameterGroupName;
private final Boolean deletionProtection;
private final Boolean copyTagsToSnapshot;
private final ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration;
private final String storageType;
private ModifyDbClusterRequest(BuilderImpl builder) {
super(builder);
this.dbClusterIdentifier = builder.dbClusterIdentifier;
this.newDBClusterIdentifier = builder.newDBClusterIdentifier;
this.applyImmediately = builder.applyImmediately;
this.backupRetentionPeriod = builder.backupRetentionPeriod;
this.dbClusterParameterGroupName = builder.dbClusterParameterGroupName;
this.vpcSecurityGroupIds = builder.vpcSecurityGroupIds;
this.port = builder.port;
this.masterUserPassword = builder.masterUserPassword;
this.optionGroupName = builder.optionGroupName;
this.preferredBackupWindow = builder.preferredBackupWindow;
this.preferredMaintenanceWindow = builder.preferredMaintenanceWindow;
this.enableIAMDatabaseAuthentication = builder.enableIAMDatabaseAuthentication;
this.cloudwatchLogsExportConfiguration = builder.cloudwatchLogsExportConfiguration;
this.engineVersion = builder.engineVersion;
this.allowMajorVersionUpgrade = builder.allowMajorVersionUpgrade;
this.dbInstanceParameterGroupName = builder.dbInstanceParameterGroupName;
this.deletionProtection = builder.deletionProtection;
this.copyTagsToSnapshot = builder.copyTagsToSnapshot;
this.serverlessV2ScalingConfiguration = builder.serverlessV2ScalingConfiguration;
this.storageType = builder.storageType;
}
/**
*
* The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*
*
* @return The DB cluster identifier for the cluster being modified. This parameter is not case-sensitive.
*
* Constraints:
*
*
* -
*
* Must match the identifier of an existing DBCluster.
*
*
*/
public final String dbClusterIdentifier() {
return dbClusterIdentifier;
}
/**
*
* The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a lowercase
* string.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*
*
* @return The new DB cluster identifier for the DB cluster when renaming a DB cluster. This value is stored as a
* lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens
*
*
* -
*
* The first character must be a letter
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens
*
*
*
*
* Example: my-cluster2
*/
public final String newDBClusterIdentifier() {
return newDBClusterIdentifier;
}
/**
*
* A value that specifies whether the modifications in this request and any pending modifications are asynchronously
* applied as soon as possible, regardless of the PreferredMaintenanceWindow
setting for the DB
* cluster. If this parameter is set to false
, changes to the DB cluster are applied during the next
* maintenance window.
*
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If you set
* the ApplyImmediately
parameter value to false, then changes to NewDBClusterIdentifier
* values are applied during the next maintenance window. All other changes are applied immediately, regardless of
* the value of the ApplyImmediately
parameter.
*
*
* Default: false
*
*
* @return A value that specifies whether the modifications in this request and any pending modifications are
* asynchronously applied as soon as possible, regardless of the PreferredMaintenanceWindow
* setting for the DB cluster. If this parameter is set to false
, changes to the DB cluster are
* applied during the next maintenance window.
*
* The ApplyImmediately
parameter only affects NewDBClusterIdentifier
values. If
* you set the ApplyImmediately
parameter value to false, then changes to
* NewDBClusterIdentifier
values are applied during the next maintenance window. All other
* changes are applied immediately, regardless of the value of the ApplyImmediately
parameter.
*
*
* Default: false
*/
public final Boolean applyImmediately() {
return applyImmediately;
}
/**
*
* The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*
*
* @return The number of days for which automated backups are retained. You must specify a minimum value of 1.
*
* Default: 1
*
*
* Constraints:
*
*
* -
*
* Must be a value from 1 to 35
*
*
*/
public final Integer backupRetentionPeriod() {
return backupRetentionPeriod;
}
/**
*
* The name of the DB cluster parameter group to use for the DB cluster.
*
*
* @return The name of the DB cluster parameter group to use for the DB cluster.
*/
public final String dbClusterParameterGroupName() {
return dbClusterParameterGroupName;
}
/**
* For responses, this returns true if the service returned a value for the VpcSecurityGroupIds property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasVpcSecurityGroupIds() {
return vpcSecurityGroupIds != null && !(vpcSecurityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* A list of VPC security groups that the DB cluster will belong to.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasVpcSecurityGroupIds} method.
*
*
* @return A list of VPC security groups that the DB cluster will belong to.
*/
public final List vpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* The port number on which the DB cluster accepts connections.
*
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*
*
* @return The port number on which the DB cluster accepts connections.
*
* Constraints: Value must be 1150-65535
*
*
* Default: The same port as the original DB cluster.
*/
public final Integer port() {
return port;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String masterUserPassword() {
return masterUserPassword;
}
/**
*
* Not supported by Neptune.
*
*
* @return Not supported by Neptune.
*/
public final String optionGroupName() {
return optionGroupName;
}
/**
*
* The daily time range during which automated backups are created if automated backups are enabled, using the
* BackupRetentionPeriod
parameter.
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*
*
* @return The daily time range during which automated backups are created if automated backups are enabled, using
* the BackupRetentionPeriod
parameter.
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region.
*
*
* Constraints:
*
*
* -
*
* Must be in the format hh24:mi-hh24:mi
.
*
*
* -
*
* Must be in Universal Coordinated Time (UTC).
*
*
* -
*
* Must not conflict with the preferred maintenance window.
*
*
* -
*
* Must be at least 30 minutes.
*
*
*/
public final String preferredBackupWindow() {
return preferredBackupWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* The default is a 30-minute window selected at random from an 8-hour block of time for each Amazon Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun.
*
*
* Constraints: Minimum 30-minute window.
*/
public final String preferredMaintenanceWindow() {
return preferredMaintenanceWindow;
}
/**
*
* True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
*
* Default: false
*
*
* @return True to enable mapping of Amazon Identity and Access Management (IAM) accounts to database accounts, and
* otherwise false.
*
* Default: false
*/
public final Boolean enableIAMDatabaseAuthentication() {
return enableIAMDatabaseAuthentication;
}
/**
*
* The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the
* CLI to publish Neptune audit logs to CloudWatch Logs.
*
*
* @return The configuration setting for the log types to be enabled for export to CloudWatch Logs for a specific DB
* cluster. See Using the CLI to publish Neptune audit logs to CloudWatch Logs.
*/
public final CloudwatchLogsExportConfiguration cloudwatchLogsExportConfiguration() {
return cloudwatchLogsExportConfiguration;
}
/**
*
* The version number of the database engine to which you want to upgrade. Changing this parameter results in an
* outage. The change is applied during the next maintenance window unless the ApplyImmediately
* parameter is set to true.
*
*
* For a list of valid engine versions, see Engine Releases for Amazon
* Neptune, or call DescribeDBEngineVersions.
*
*
* @return The version number of the database engine to which you want to upgrade. Changing this parameter results
* in an outage. The change is applied during the next maintenance window unless the
* ApplyImmediately
parameter is set to true.
*
* For a list of valid engine versions, see Engine Releases for
* Amazon Neptune, or call DescribeDBEngineVersions.
*/
public final String engineVersion() {
return engineVersion;
}
/**
*
* A value that indicates whether upgrades between different major versions are allowed.
*
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an EngineVersion
* parameter that uses a different major version than the DB cluster's current version.
*
*
* @return A value that indicates whether upgrades between different major versions are allowed.
*
* Constraints: You must set the allow-major-version-upgrade flag when providing an
* EngineVersion
parameter that uses a different major version than the DB cluster's current
* version.
*/
public final Boolean allowMajorVersionUpgrade() {
return allowMajorVersionUpgrade;
}
/**
*
* The name of the DB parameter group to apply to all instances of the DB cluster.
*
*
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes aren't
* applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*
*
* @return The name of the DB parameter group to apply to all instances of the DB cluster.
*
* When you apply a parameter group using DBInstanceParameterGroupName
, parameter changes
* aren't applied during the next maintenance window but instead are applied immediately.
*
*
*
* Default: The existing name setting
*
*
* Constraints:
*
*
* -
*
* The DB parameter group must be in the same DB parameter group family as the target DB cluster version.
*
*
* -
*
* The DBInstanceParameterGroupName
parameter is only valid in combination with the
* AllowMajorVersionUpgrade
parameter.
*
*
*/
public final String dbInstanceParameterGroupName() {
return dbInstanceParameterGroupName;
}
/**
*
* A value that indicates whether the DB cluster has deletion protection enabled. The database can't be deleted when
* deletion protection is enabled. By default, deletion protection is disabled.
*
*
* @return A value that indicates whether the DB cluster has deletion protection enabled. The database can't be
* deleted when deletion protection is enabled. By default, deletion protection is disabled.
*/
public final Boolean deletionProtection() {
return deletionProtection;
}
/**
*
* If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*
*
* @return If set to true
, tags are copied to any snapshot of the DB cluster that is created.
*/
public final Boolean copyTagsToSnapshot() {
return copyTagsToSnapshot;
}
/**
*
* Contains the scaling configuration of a Neptune Serverless DB cluster.
*
*
* For more information, see Using Amazon Neptune
* Serverless in the Amazon Neptune User Guide.
*
*
* @return Contains the scaling configuration of a Neptune Serverless DB cluster.
*
* For more information, see Using Amazon
* Neptune Serverless in the Amazon Neptune User Guide.
*/
public final ServerlessV2ScalingConfiguration serverlessV2ScalingConfiguration() {
return serverlessV2ScalingConfiguration;
}
/**
*
* The storage type to associate with the DB cluster.
*
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*
*
* @return The storage type to associate with the DB cluster.
*
* Valid Values:
*
*
* -
*
* standard | iopt1
*
*
*
*
* Default:
*
*
* -
*
* standard
*
*
*/
public final String storageType() {
return storageType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dbClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(newDBClusterIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(applyImmediately());
hashCode = 31 * hashCode + Objects.hashCode(backupRetentionPeriod());
hashCode = 31 * hashCode + Objects.hashCode(dbClusterParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(port());
hashCode = 31 * hashCode + Objects.hashCode(masterUserPassword());
hashCode = 31 * hashCode + Objects.hashCode(optionGroupName());
hashCode = 31 * hashCode + Objects.hashCode(preferredBackupWindow());
hashCode = 31 * hashCode + Objects.hashCode(preferredMaintenanceWindow());
hashCode = 31 * hashCode + Objects.hashCode(enableIAMDatabaseAuthentication());
hashCode = 31 * hashCode + Objects.hashCode(cloudwatchLogsExportConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(engineVersion());
hashCode = 31 * hashCode + Objects.hashCode(allowMajorVersionUpgrade());
hashCode = 31 * hashCode + Objects.hashCode(dbInstanceParameterGroupName());
hashCode = 31 * hashCode + Objects.hashCode(deletionProtection());
hashCode = 31 * hashCode + Objects.hashCode(copyTagsToSnapshot());
hashCode = 31 * hashCode + Objects.hashCode(serverlessV2ScalingConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(storageType());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyDbClusterRequest)) {
return false;
}
ModifyDbClusterRequest other = (ModifyDbClusterRequest) obj;
return Objects.equals(dbClusterIdentifier(), other.dbClusterIdentifier())
&& Objects.equals(newDBClusterIdentifier(), other.newDBClusterIdentifier())
&& Objects.equals(applyImmediately(), other.applyImmediately())
&& Objects.equals(backupRetentionPeriod(), other.backupRetentionPeriod())
&& Objects.equals(dbClusterParameterGroupName(), other.dbClusterParameterGroupName())
&& hasVpcSecurityGroupIds() == other.hasVpcSecurityGroupIds()
&& Objects.equals(vpcSecurityGroupIds(), other.vpcSecurityGroupIds()) && Objects.equals(port(), other.port())
&& Objects.equals(masterUserPassword(), other.masterUserPassword())
&& Objects.equals(optionGroupName(), other.optionGroupName())
&& Objects.equals(preferredBackupWindow(), other.preferredBackupWindow())
&& Objects.equals(preferredMaintenanceWindow(), other.preferredMaintenanceWindow())
&& Objects.equals(enableIAMDatabaseAuthentication(), other.enableIAMDatabaseAuthentication())
&& Objects.equals(cloudwatchLogsExportConfiguration(), other.cloudwatchLogsExportConfiguration())
&& Objects.equals(engineVersion(), other.engineVersion())
&& Objects.equals(allowMajorVersionUpgrade(), other.allowMajorVersionUpgrade())
&& Objects.equals(dbInstanceParameterGroupName(), other.dbInstanceParameterGroupName())
&& Objects.equals(deletionProtection(), other.deletionProtection())
&& Objects.equals(copyTagsToSnapshot(), other.copyTagsToSnapshot())
&& Objects.equals(serverlessV2ScalingConfiguration(), other.serverlessV2ScalingConfiguration())
&& Objects.equals(storageType(), other.storageType());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyDbClusterRequest").add("DBClusterIdentifier", dbClusterIdentifier())
.add("NewDBClusterIdentifier", newDBClusterIdentifier()).add("ApplyImmediately", applyImmediately())
.add("BackupRetentionPeriod", backupRetentionPeriod())
.add("DBClusterParameterGroupName", dbClusterParameterGroupName())
.add("VpcSecurityGroupIds", hasVpcSecurityGroupIds() ? vpcSecurityGroupIds() : null).add("Port", port())
.add("MasterUserPassword", masterUserPassword()).add("OptionGroupName", optionGroupName())
.add("PreferredBackupWindow", preferredBackupWindow())
.add("PreferredMaintenanceWindow", preferredMaintenanceWindow())
.add("EnableIAMDatabaseAuthentication", enableIAMDatabaseAuthentication())
.add("CloudwatchLogsExportConfiguration", cloudwatchLogsExportConfiguration())
.add("EngineVersion", engineVersion()).add("AllowMajorVersionUpgrade", allowMajorVersionUpgrade())
.add("DBInstanceParameterGroupName", dbInstanceParameterGroupName())
.add("DeletionProtection", deletionProtection()).add("CopyTagsToSnapshot", copyTagsToSnapshot())
.add("ServerlessV2ScalingConfiguration", serverlessV2ScalingConfiguration()).add("StorageType", storageType())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBClusterIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterIdentifier()));
case "NewDBClusterIdentifier":
return Optional.ofNullable(clazz.cast(newDBClusterIdentifier()));
case "ApplyImmediately":
return Optional.ofNullable(clazz.cast(applyImmediately()));
case "BackupRetentionPeriod":
return Optional.ofNullable(clazz.cast(backupRetentionPeriod()));
case "DBClusterParameterGroupName":
return Optional.ofNullable(clazz.cast(dbClusterParameterGroupName()));
case "VpcSecurityGroupIds":
return Optional.ofNullable(clazz.cast(vpcSecurityGroupIds()));
case "Port":
return Optional.ofNullable(clazz.cast(port()));
case "MasterUserPassword":
return Optional.ofNullable(clazz.cast(masterUserPassword()));
case "OptionGroupName":
return Optional.ofNullable(clazz.cast(optionGroupName()));
case "PreferredBackupWindow":
return Optional.ofNullable(clazz.cast(preferredBackupWindow()));
case "PreferredMaintenanceWindow":
return Optional.ofNullable(clazz.cast(preferredMaintenanceWindow()));
case "EnableIAMDatabaseAuthentication":
return Optional.ofNullable(clazz.cast(enableIAMDatabaseAuthentication()));
case "CloudwatchLogsExportConfiguration":
return Optional.ofNullable(clazz.cast(cloudwatchLogsExportConfiguration()));
case "EngineVersion":
return Optional.ofNullable(clazz.cast(engineVersion()));
case "AllowMajorVersionUpgrade":
return Optional.ofNullable(clazz.cast(allowMajorVersionUpgrade()));
case "DBInstanceParameterGroupName":
return Optional.ofNullable(clazz.cast(dbInstanceParameterGroupName()));
case "DeletionProtection":
return Optional.ofNullable(clazz.cast(deletionProtection()));
case "CopyTagsToSnapshot":
return Optional.ofNullable(clazz.cast(copyTagsToSnapshot()));
case "ServerlessV2ScalingConfiguration":
return Optional.ofNullable(clazz.cast(serverlessV2ScalingConfiguration()));
case "StorageType":
return Optional.ofNullable(clazz.cast(storageType()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function