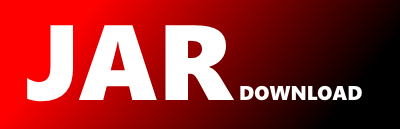
software.amazon.awssdk.services.neptune.model.ModifyDbClusterSnapshotAttributeRequest Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ModifyDbClusterSnapshotAttributeRequest extends NeptuneRequest implements
ToCopyableBuilder {
private static final SdkField DB_CLUSTER_SNAPSHOT_IDENTIFIER_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("DBClusterSnapshotIdentifier")
.getter(getter(ModifyDbClusterSnapshotAttributeRequest::dbClusterSnapshotIdentifier))
.setter(setter(Builder::dbClusterSnapshotIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DBClusterSnapshotIdentifier")
.build()).build();
private static final SdkField ATTRIBUTE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AttributeName").getter(getter(ModifyDbClusterSnapshotAttributeRequest::attributeName))
.setter(setter(Builder::attributeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AttributeName").build()).build();
private static final SdkField> VALUES_TO_ADD_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ValuesToAdd")
.getter(getter(ModifyDbClusterSnapshotAttributeRequest::valuesToAdd))
.setter(setter(Builder::valuesToAdd))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValuesToAdd").build(),
ListTrait
.builder()
.memberLocationName("AttributeValue")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AttributeValue").build()).build()).build()).build();
private static final SdkField> VALUES_TO_REMOVE_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ValuesToRemove")
.getter(getter(ModifyDbClusterSnapshotAttributeRequest::valuesToRemove))
.setter(setter(Builder::valuesToRemove))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValuesToRemove").build(),
ListTrait
.builder()
.memberLocationName("AttributeValue")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("AttributeValue").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(
DB_CLUSTER_SNAPSHOT_IDENTIFIER_FIELD, ATTRIBUTE_NAME_FIELD, VALUES_TO_ADD_FIELD, VALUES_TO_REMOVE_FIELD));
private final String dbClusterSnapshotIdentifier;
private final String attributeName;
private final List valuesToAdd;
private final List valuesToRemove;
private ModifyDbClusterSnapshotAttributeRequest(BuilderImpl builder) {
super(builder);
this.dbClusterSnapshotIdentifier = builder.dbClusterSnapshotIdentifier;
this.attributeName = builder.attributeName;
this.valuesToAdd = builder.valuesToAdd;
this.valuesToRemove = builder.valuesToRemove;
}
/**
*
* The identifier for the DB cluster snapshot to modify the attributes for.
*
*
* @return The identifier for the DB cluster snapshot to modify the attributes for.
*/
public final String dbClusterSnapshotIdentifier() {
return dbClusterSnapshotIdentifier;
}
/**
*
* The name of the DB cluster snapshot attribute to modify.
*
*
* To manage authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set this value
* to restore
.
*
*
* @return The name of the DB cluster snapshot attribute to modify.
*
* To manage authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set
* this value to restore
.
*/
public final String attributeName() {
return attributeName;
}
/**
* For responses, this returns true if the service returned a value for the ValuesToAdd property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasValuesToAdd() {
return valuesToAdd != null && !(valuesToAdd instanceof SdkAutoConstructList);
}
/**
*
* A list of DB cluster snapshot attributes to add to the attribute specified by AttributeName
.
*
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to include one
* or more Amazon account IDs, or all
to make the manual DB cluster snapshot restorable by any Amazon
* account. Do not add the all
value for any manual DB cluster snapshots that contain private
* information that you don't want available to all Amazon accounts.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasValuesToAdd} method.
*
*
* @return A list of DB cluster snapshot attributes to add to the attribute specified by AttributeName
* .
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to
* include one or more Amazon account IDs, or all
to make the manual DB cluster snapshot
* restorable by any Amazon account. Do not add the all
value for any manual DB cluster
* snapshots that contain private information that you don't want available to all Amazon accounts.
*/
public final List valuesToAdd() {
return valuesToAdd;
}
/**
* For responses, this returns true if the service returned a value for the ValuesToRemove property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasValuesToRemove() {
return valuesToRemove != null && !(valuesToRemove instanceof SdkAutoConstructList);
}
/**
*
* A list of DB cluster snapshot attributes to remove from the attribute specified by AttributeName
.
*
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list
* to include one or more Amazon account identifiers, or all
to remove authorization for any Amazon
* account to copy or restore the DB cluster snapshot. If you specify all
, an Amazon account whose
* account ID is explicitly added to the restore
attribute can still copy or restore a manual DB
* cluster snapshot.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasValuesToRemove} method.
*
*
* @return A list of DB cluster snapshot attributes to remove from the attribute specified by
* AttributeName
.
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set
* this list to include one or more Amazon account identifiers, or all
to remove authorization
* for any Amazon account to copy or restore the DB cluster snapshot. If you specify all
, an
* Amazon account whose account ID is explicitly added to the restore
attribute can still copy
* or restore a manual DB cluster snapshot.
*/
public final List valuesToRemove() {
return valuesToRemove;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(dbClusterSnapshotIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(attributeName());
hashCode = 31 * hashCode + Objects.hashCode(hasValuesToAdd() ? valuesToAdd() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasValuesToRemove() ? valuesToRemove() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ModifyDbClusterSnapshotAttributeRequest)) {
return false;
}
ModifyDbClusterSnapshotAttributeRequest other = (ModifyDbClusterSnapshotAttributeRequest) obj;
return Objects.equals(dbClusterSnapshotIdentifier(), other.dbClusterSnapshotIdentifier())
&& Objects.equals(attributeName(), other.attributeName()) && hasValuesToAdd() == other.hasValuesToAdd()
&& Objects.equals(valuesToAdd(), other.valuesToAdd()) && hasValuesToRemove() == other.hasValuesToRemove()
&& Objects.equals(valuesToRemove(), other.valuesToRemove());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ModifyDbClusterSnapshotAttributeRequest")
.add("DBClusterSnapshotIdentifier", dbClusterSnapshotIdentifier()).add("AttributeName", attributeName())
.add("ValuesToAdd", hasValuesToAdd() ? valuesToAdd() : null)
.add("ValuesToRemove", hasValuesToRemove() ? valuesToRemove() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DBClusterSnapshotIdentifier":
return Optional.ofNullable(clazz.cast(dbClusterSnapshotIdentifier()));
case "AttributeName":
return Optional.ofNullable(clazz.cast(attributeName()));
case "ValuesToAdd":
return Optional.ofNullable(clazz.cast(valuesToAdd()));
case "ValuesToRemove":
return Optional.ofNullable(clazz.cast(valuesToRemove()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* To manage authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set
* this value to restore
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder attributeName(String attributeName);
/**
*
* A list of DB cluster snapshot attributes to add to the attribute specified by AttributeName
.
*
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to include
* one or more Amazon account IDs, or all
to make the manual DB cluster snapshot restorable by any
* Amazon account. Do not add the all
value for any manual DB cluster snapshots that contain
* private information that you don't want available to all Amazon accounts.
*
*
* @param valuesToAdd
* A list of DB cluster snapshot attributes to add to the attribute specified by
* AttributeName
.
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to
* include one or more Amazon account IDs, or all
to make the manual DB cluster snapshot
* restorable by any Amazon account. Do not add the all
value for any manual DB cluster
* snapshots that contain private information that you don't want available to all Amazon accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder valuesToAdd(Collection valuesToAdd);
/**
*
* A list of DB cluster snapshot attributes to add to the attribute specified by AttributeName
.
*
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to include
* one or more Amazon account IDs, or all
to make the manual DB cluster snapshot restorable by any
* Amazon account. Do not add the all
value for any manual DB cluster snapshots that contain
* private information that you don't want available to all Amazon accounts.
*
*
* @param valuesToAdd
* A list of DB cluster snapshot attributes to add to the attribute specified by
* AttributeName
.
*
* To authorize other Amazon accounts to copy or restore a manual DB cluster snapshot, set this list to
* include one or more Amazon account IDs, or all
to make the manual DB cluster snapshot
* restorable by any Amazon account. Do not add the all
value for any manual DB cluster
* snapshots that contain private information that you don't want available to all Amazon accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder valuesToAdd(String... valuesToAdd);
/**
*
* A list of DB cluster snapshot attributes to remove from the attribute specified by AttributeName
* .
*
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set this
* list to include one or more Amazon account identifiers, or all
to remove authorization for any
* Amazon account to copy or restore the DB cluster snapshot. If you specify all
, an Amazon account
* whose account ID is explicitly added to the restore
attribute can still copy or restore a manual
* DB cluster snapshot.
*
*
* @param valuesToRemove
* A list of DB cluster snapshot attributes to remove from the attribute specified by
* AttributeName
.
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set
* this list to include one or more Amazon account identifiers, or all
to remove
* authorization for any Amazon account to copy or restore the DB cluster snapshot. If you specify
* all
, an Amazon account whose account ID is explicitly added to the restore
* attribute can still copy or restore a manual DB cluster snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder valuesToRemove(Collection valuesToRemove);
/**
*
* A list of DB cluster snapshot attributes to remove from the attribute specified by AttributeName
* .
*
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set this
* list to include one or more Amazon account identifiers, or all
to remove authorization for any
* Amazon account to copy or restore the DB cluster snapshot. If you specify all
, an Amazon account
* whose account ID is explicitly added to the restore
attribute can still copy or restore a manual
* DB cluster snapshot.
*
*
* @param valuesToRemove
* A list of DB cluster snapshot attributes to remove from the attribute specified by
* AttributeName
.
*
* To remove authorization for other Amazon accounts to copy or restore a manual DB cluster snapshot, set
* this list to include one or more Amazon account identifiers, or all
to remove
* authorization for any Amazon account to copy or restore the DB cluster snapshot. If you specify
* all
, an Amazon account whose account ID is explicitly added to the restore
* attribute can still copy or restore a manual DB cluster snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder valuesToRemove(String... valuesToRemove);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends NeptuneRequest.BuilderImpl implements Builder {
private String dbClusterSnapshotIdentifier;
private String attributeName;
private List valuesToAdd = DefaultSdkAutoConstructList.getInstance();
private List valuesToRemove = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(ModifyDbClusterSnapshotAttributeRequest model) {
super(model);
dbClusterSnapshotIdentifier(model.dbClusterSnapshotIdentifier);
attributeName(model.attributeName);
valuesToAdd(model.valuesToAdd);
valuesToRemove(model.valuesToRemove);
}
public final String getDbClusterSnapshotIdentifier() {
return dbClusterSnapshotIdentifier;
}
public final void setDbClusterSnapshotIdentifier(String dbClusterSnapshotIdentifier) {
this.dbClusterSnapshotIdentifier = dbClusterSnapshotIdentifier;
}
@Override
public final Builder dbClusterSnapshotIdentifier(String dbClusterSnapshotIdentifier) {
this.dbClusterSnapshotIdentifier = dbClusterSnapshotIdentifier;
return this;
}
public final String getAttributeName() {
return attributeName;
}
public final void setAttributeName(String attributeName) {
this.attributeName = attributeName;
}
@Override
public final Builder attributeName(String attributeName) {
this.attributeName = attributeName;
return this;
}
public final Collection getValuesToAdd() {
if (valuesToAdd instanceof SdkAutoConstructList) {
return null;
}
return valuesToAdd;
}
public final void setValuesToAdd(Collection valuesToAdd) {
this.valuesToAdd = AttributeValueListCopier.copy(valuesToAdd);
}
@Override
public final Builder valuesToAdd(Collection valuesToAdd) {
this.valuesToAdd = AttributeValueListCopier.copy(valuesToAdd);
return this;
}
@Override
@SafeVarargs
public final Builder valuesToAdd(String... valuesToAdd) {
valuesToAdd(Arrays.asList(valuesToAdd));
return this;
}
public final Collection getValuesToRemove() {
if (valuesToRemove instanceof SdkAutoConstructList) {
return null;
}
return valuesToRemove;
}
public final void setValuesToRemove(Collection valuesToRemove) {
this.valuesToRemove = AttributeValueListCopier.copy(valuesToRemove);
}
@Override
public final Builder valuesToRemove(Collection valuesToRemove) {
this.valuesToRemove = AttributeValueListCopier.copy(valuesToRemove);
return this;
}
@Override
@SafeVarargs
public final Builder valuesToRemove(String... valuesToRemove) {
valuesToRemove(Arrays.asList(valuesToRemove));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public ModifyDbClusterSnapshotAttributeRequest build() {
return new ModifyDbClusterSnapshotAttributeRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}