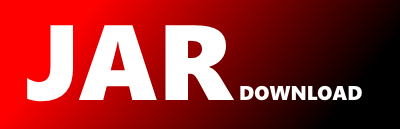
software.amazon.awssdk.services.neptune.model.Parameter Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Specifies a parameter.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Parameter implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField PARAMETER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParameterName").getter(getter(Parameter::parameterName)).setter(setter(Builder::parameterName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParameterName").build()).build();
private static final SdkField PARAMETER_VALUE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ParameterValue").getter(getter(Parameter::parameterValue)).setter(setter(Builder::parameterValue))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ParameterValue").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(Parameter::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField SOURCE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Source")
.getter(getter(Parameter::source)).setter(setter(Builder::source))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField APPLY_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplyType").getter(getter(Parameter::applyType)).setter(setter(Builder::applyType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyType").build()).build();
private static final SdkField DATA_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataType").getter(getter(Parameter::dataType)).setter(setter(Builder::dataType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataType").build()).build();
private static final SdkField ALLOWED_VALUES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AllowedValues").getter(getter(Parameter::allowedValues)).setter(setter(Builder::allowedValues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AllowedValues").build()).build();
private static final SdkField IS_MODIFIABLE_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IsModifiable").getter(getter(Parameter::isModifiable)).setter(setter(Builder::isModifiable))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IsModifiable").build()).build();
private static final SdkField MINIMUM_ENGINE_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MinimumEngineVersion").getter(getter(Parameter::minimumEngineVersion))
.setter(setter(Builder::minimumEngineVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MinimumEngineVersion").build())
.build();
private static final SdkField APPLY_METHOD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplyMethod").getter(getter(Parameter::applyMethodAsString)).setter(setter(Builder::applyMethod))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplyMethod").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(PARAMETER_NAME_FIELD,
PARAMETER_VALUE_FIELD, DESCRIPTION_FIELD, SOURCE_FIELD, APPLY_TYPE_FIELD, DATA_TYPE_FIELD, ALLOWED_VALUES_FIELD,
IS_MODIFIABLE_FIELD, MINIMUM_ENGINE_VERSION_FIELD, APPLY_METHOD_FIELD));
private static final long serialVersionUID = 1L;
private final String parameterName;
private final String parameterValue;
private final String description;
private final String source;
private final String applyType;
private final String dataType;
private final String allowedValues;
private final Boolean isModifiable;
private final String minimumEngineVersion;
private final String applyMethod;
private Parameter(BuilderImpl builder) {
this.parameterName = builder.parameterName;
this.parameterValue = builder.parameterValue;
this.description = builder.description;
this.source = builder.source;
this.applyType = builder.applyType;
this.dataType = builder.dataType;
this.allowedValues = builder.allowedValues;
this.isModifiable = builder.isModifiable;
this.minimumEngineVersion = builder.minimumEngineVersion;
this.applyMethod = builder.applyMethod;
}
/**
*
* Specifies the name of the parameter.
*
*
* @return Specifies the name of the parameter.
*/
public final String parameterName() {
return parameterName;
}
/**
*
* Specifies the value of the parameter.
*
*
* @return Specifies the value of the parameter.
*/
public final String parameterValue() {
return parameterValue;
}
/**
*
* Provides a description of the parameter.
*
*
* @return Provides a description of the parameter.
*/
public final String description() {
return description;
}
/**
*
* Indicates the source of the parameter value.
*
*
* @return Indicates the source of the parameter value.
*/
public final String source() {
return source;
}
/**
*
* Specifies the engine specific parameters type.
*
*
* @return Specifies the engine specific parameters type.
*/
public final String applyType() {
return applyType;
}
/**
*
* Specifies the valid data type for the parameter.
*
*
* @return Specifies the valid data type for the parameter.
*/
public final String dataType() {
return dataType;
}
/**
*
* Specifies the valid range of values for the parameter.
*
*
* @return Specifies the valid range of values for the parameter.
*/
public final String allowedValues() {
return allowedValues;
}
/**
*
* Indicates whether (true
) or not (false
) the parameter can be modified. Some parameters
* have security or operational implications that prevent them from being changed.
*
*
* @return Indicates whether (true
) or not (false
) the parameter can be modified. Some
* parameters have security or operational implications that prevent them from being changed.
*/
public final Boolean isModifiable() {
return isModifiable;
}
/**
*
* The earliest engine version to which the parameter can apply.
*
*
* @return The earliest engine version to which the parameter can apply.
*/
public final String minimumEngineVersion() {
return minimumEngineVersion;
}
/**
*
* Indicates when to apply parameter updates.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #applyMethod} will
* return {@link ApplyMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #applyMethodAsString}.
*
*
* @return Indicates when to apply parameter updates.
* @see ApplyMethod
*/
public final ApplyMethod applyMethod() {
return ApplyMethod.fromValue(applyMethod);
}
/**
*
* Indicates when to apply parameter updates.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #applyMethod} will
* return {@link ApplyMethod#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #applyMethodAsString}.
*
*
* @return Indicates when to apply parameter updates.
* @see ApplyMethod
*/
public final String applyMethodAsString() {
return applyMethod;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(parameterName());
hashCode = 31 * hashCode + Objects.hashCode(parameterValue());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(applyType());
hashCode = 31 * hashCode + Objects.hashCode(dataType());
hashCode = 31 * hashCode + Objects.hashCode(allowedValues());
hashCode = 31 * hashCode + Objects.hashCode(isModifiable());
hashCode = 31 * hashCode + Objects.hashCode(minimumEngineVersion());
hashCode = 31 * hashCode + Objects.hashCode(applyMethodAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Parameter)) {
return false;
}
Parameter other = (Parameter) obj;
return Objects.equals(parameterName(), other.parameterName()) && Objects.equals(parameterValue(), other.parameterValue())
&& Objects.equals(description(), other.description()) && Objects.equals(source(), other.source())
&& Objects.equals(applyType(), other.applyType()) && Objects.equals(dataType(), other.dataType())
&& Objects.equals(allowedValues(), other.allowedValues()) && Objects.equals(isModifiable(), other.isModifiable())
&& Objects.equals(minimumEngineVersion(), other.minimumEngineVersion())
&& Objects.equals(applyMethodAsString(), other.applyMethodAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Parameter").add("ParameterName", parameterName()).add("ParameterValue", parameterValue())
.add("Description", description()).add("Source", source()).add("ApplyType", applyType())
.add("DataType", dataType()).add("AllowedValues", allowedValues()).add("IsModifiable", isModifiable())
.add("MinimumEngineVersion", minimumEngineVersion()).add("ApplyMethod", applyMethodAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ParameterName":
return Optional.ofNullable(clazz.cast(parameterName()));
case "ParameterValue":
return Optional.ofNullable(clazz.cast(parameterValue()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "ApplyType":
return Optional.ofNullable(clazz.cast(applyType()));
case "DataType":
return Optional.ofNullable(clazz.cast(dataType()));
case "AllowedValues":
return Optional.ofNullable(clazz.cast(allowedValues()));
case "IsModifiable":
return Optional.ofNullable(clazz.cast(isModifiable()));
case "MinimumEngineVersion":
return Optional.ofNullable(clazz.cast(minimumEngineVersion()));
case "ApplyMethod":
return Optional.ofNullable(clazz.cast(applyMethodAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function