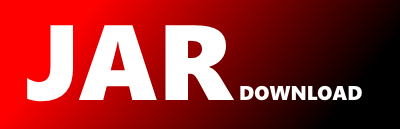
software.amazon.awssdk.services.neptune.model.DescribeEventsRequest Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeEventsRequest extends NeptuneRequest implements
ToCopyableBuilder {
private static final SdkField SOURCE_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceIdentifier").getter(getter(DescribeEventsRequest::sourceIdentifier))
.setter(setter(Builder::sourceIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceIdentifier").build()).build();
private static final SdkField SOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceType").getter(getter(DescribeEventsRequest::sourceTypeAsString))
.setter(setter(Builder::sourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceType").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTime").getter(getter(DescribeEventsRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("EndTime").getter(getter(DescribeEventsRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndTime").build()).build();
private static final SdkField DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Duration").getter(getter(DescribeEventsRequest::duration)).setter(setter(Builder::duration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Duration").build()).build();
private static final SdkField> EVENT_CATEGORIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EventCategories")
.getter(getter(DescribeEventsRequest::eventCategories))
.setter(setter(Builder::eventCategories))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventCategories").build(),
ListTrait
.builder()
.memberLocationName("EventCategory")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EventCategory").build()).build()).build()).build();
private static final SdkField> FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Filters")
.getter(getter(DescribeEventsRequest::filters))
.setter(setter(Builder::filters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Filters").build(),
ListTrait
.builder()
.memberLocationName("Filter")
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Filter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("Filter").build()).build()).build()).build();
private static final SdkField MAX_RECORDS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxRecords").getter(getter(DescribeEventsRequest::maxRecords)).setter(setter(Builder::maxRecords))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaxRecords").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Marker")
.getter(getter(DescribeEventsRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Marker").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SOURCE_IDENTIFIER_FIELD,
SOURCE_TYPE_FIELD, START_TIME_FIELD, END_TIME_FIELD, DURATION_FIELD, EVENT_CATEGORIES_FIELD, FILTERS_FIELD,
MAX_RECORDS_FIELD, MARKER_FIELD));
private final String sourceIdentifier;
private final String sourceType;
private final Instant startTime;
private final Instant endTime;
private final Integer duration;
private final List eventCategories;
private final List filters;
private final Integer maxRecords;
private final String marker;
private DescribeEventsRequest(BuilderImpl builder) {
super(builder);
this.sourceIdentifier = builder.sourceIdentifier;
this.sourceType = builder.sourceType;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.duration = builder.duration;
this.eventCategories = builder.eventCategories;
this.filters = builder.filters;
this.maxRecords = builder.maxRecords;
this.marker = builder.marker;
}
/**
*
* The identifier of the event source for which events are returned. If not specified, then all sources are included
* in the response.
*
*
* Constraints:
*
*
* -
*
* If SourceIdentifier is supplied, SourceType must also be provided.
*
*
* -
*
* If the source type is DBInstance
, then a DBInstanceIdentifier
must be supplied.
*
*
* -
*
* If the source type is DBSecurityGroup
, a DBSecurityGroupName
must be supplied.
*
*
* -
*
* If the source type is DBParameterGroup
, a DBParameterGroupName
must be supplied.
*
*
* -
*
* If the source type is DBSnapshot
, a DBSnapshotIdentifier
must be supplied.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* @return The identifier of the event source for which events are returned. If not specified, then all sources are
* included in the response.
*
* Constraints:
*
*
* -
*
* If SourceIdentifier is supplied, SourceType must also be provided.
*
*
* -
*
* If the source type is DBInstance
, then a DBInstanceIdentifier
must be supplied.
*
*
* -
*
* If the source type is DBSecurityGroup
, a DBSecurityGroupName
must be supplied.
*
*
* -
*
* If the source type is DBParameterGroup
, a DBParameterGroupName
must be
* supplied.
*
*
* -
*
* If the source type is DBSnapshot
, a DBSnapshotIdentifier
must be supplied.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*/
public final String sourceIdentifier() {
return sourceIdentifier;
}
/**
*
* The event source to retrieve events for. If no value is specified, all events are returned.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The event source to retrieve events for. If no value is specified, all events are returned.
* @see SourceType
*/
public final SourceType sourceType() {
return SourceType.fromValue(sourceType);
}
/**
*
* The event source to retrieve events for. If no value is specified, all events are returned.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #sourceType} will
* return {@link SourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #sourceTypeAsString}.
*
*
* @return The event source to retrieve events for. If no value is specified, all events are returned.
* @see SourceType
*/
public final String sourceTypeAsString() {
return sourceType;
}
/**
*
* The beginning of the time interval to retrieve events for, specified in ISO 8601 format. For more information
* about ISO 8601, go to the ISO8601 Wikipedia page.
*
*
* Example: 2009-07-08T18:00Z
*
*
* @return The beginning of the time interval to retrieve events for, specified in ISO 8601 format. For more
* information about ISO 8601, go to the ISO8601 Wikipedia
* page.
*
* Example: 2009-07-08T18:00Z
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The end of the time interval for which to retrieve events, specified in ISO 8601 format. For more information
* about ISO 8601, go to the ISO8601 Wikipedia page.
*
*
* Example: 2009-07-08T18:00Z
*
*
* @return The end of the time interval for which to retrieve events, specified in ISO 8601 format. For more
* information about ISO 8601, go to the ISO8601 Wikipedia
* page.
*
* Example: 2009-07-08T18:00Z
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The number of minutes to retrieve events for.
*
*
* Default: 60
*
*
* @return The number of minutes to retrieve events for.
*
* Default: 60
*/
public final Integer duration() {
return duration;
}
/**
* For responses, this returns true if the service returned a value for the EventCategories property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEventCategories() {
return eventCategories != null && !(eventCategories instanceof SdkAutoConstructList);
}
/**
*
* A list of event categories that trigger notifications for a event notification subscription.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEventCategories} method.
*
*
* @return A list of event categories that trigger notifications for a event notification subscription.
*/
public final List eventCategories() {
return eventCategories;
}
/**
* For responses, this returns true if the service returned a value for the Filters property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasFilters() {
return filters != null && !(filters instanceof SdkAutoConstructList);
}
/**
*
* This parameter is not currently supported.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFilters} method.
*
*
* @return This parameter is not currently supported.
*/
public final List filters() {
return filters;
}
/**
*
* The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a pagination token called a marker is included in the response so that the
* remaining results can be retrieved.
*
*
* Default: 100
*
*
* Constraints: Minimum 20, maximum 100.
*
*
* @return The maximum number of records to include in the response. If more records exist than the specified
* MaxRecords
value, a pagination token called a marker is included in the response so that the
* remaining results can be retrieved.
*
* Default: 100
*
*
* Constraints: Minimum 20, maximum 100.
*/
public final Integer maxRecords() {
return maxRecords;
}
/**
*
* An optional pagination token provided by a previous DescribeEvents request. If this parameter is specified, the
* response includes only records beyond the marker, up to the value specified by MaxRecords
.
*
*
* @return An optional pagination token provided by a previous DescribeEvents request. If this parameter is
* specified, the response includes only records beyond the marker, up to the value specified by
* MaxRecords
.
*/
public final String marker() {
return marker;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(sourceIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(duration());
hashCode = 31 * hashCode + Objects.hashCode(hasEventCategories() ? eventCategories() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasFilters() ? filters() : null);
hashCode = 31 * hashCode + Objects.hashCode(maxRecords());
hashCode = 31 * hashCode + Objects.hashCode(marker());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeEventsRequest)) {
return false;
}
DescribeEventsRequest other = (DescribeEventsRequest) obj;
return Objects.equals(sourceIdentifier(), other.sourceIdentifier())
&& Objects.equals(sourceTypeAsString(), other.sourceTypeAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(duration(), other.duration()) && hasEventCategories() == other.hasEventCategories()
&& Objects.equals(eventCategories(), other.eventCategories()) && hasFilters() == other.hasFilters()
&& Objects.equals(filters(), other.filters()) && Objects.equals(maxRecords(), other.maxRecords())
&& Objects.equals(marker(), other.marker());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeEventsRequest").add("SourceIdentifier", sourceIdentifier())
.add("SourceType", sourceTypeAsString()).add("StartTime", startTime()).add("EndTime", endTime())
.add("Duration", duration()).add("EventCategories", hasEventCategories() ? eventCategories() : null)
.add("Filters", hasFilters() ? filters() : null).add("MaxRecords", maxRecords()).add("Marker", marker()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SourceIdentifier":
return Optional.ofNullable(clazz.cast(sourceIdentifier()));
case "SourceType":
return Optional.ofNullable(clazz.cast(sourceTypeAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "Duration":
return Optional.ofNullable(clazz.cast(duration()));
case "EventCategories":
return Optional.ofNullable(clazz.cast(eventCategories()));
case "Filters":
return Optional.ofNullable(clazz.cast(filters()));
case "MaxRecords":
return Optional.ofNullable(clazz.cast(maxRecords()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function