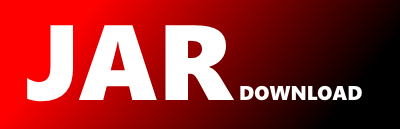
software.amazon.awssdk.services.neptune.model.EventSubscription Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the results of a successful invocation of the DescribeEventSubscriptions action.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class EventSubscription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CUSTOMER_AWS_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomerAwsId").getter(getter(EventSubscription::customerAwsId)).setter(setter(Builder::customerAwsId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomerAwsId").build()).build();
private static final SdkField CUST_SUBSCRIPTION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustSubscriptionId").getter(getter(EventSubscription::custSubscriptionId))
.setter(setter(Builder::custSubscriptionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustSubscriptionId").build())
.build();
private static final SdkField SNS_TOPIC_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SnsTopicArn").getter(getter(EventSubscription::snsTopicArn)).setter(setter(Builder::snsTopicArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SnsTopicArn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(EventSubscription::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField SUBSCRIPTION_CREATION_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubscriptionCreationTime").getter(getter(EventSubscription::subscriptionCreationTime))
.setter(setter(Builder::subscriptionCreationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubscriptionCreationTime").build())
.build();
private static final SdkField SOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SourceType").getter(getter(EventSubscription::sourceType)).setter(setter(Builder::sourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceType").build()).build();
private static final SdkField> SOURCE_IDS_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SourceIdsList")
.getter(getter(EventSubscription::sourceIdsList))
.setter(setter(Builder::sourceIdsList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SourceIdsList").build(),
ListTrait
.builder()
.memberLocationName("SourceId")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("SourceId").build()).build()).build()).build();
private static final SdkField> EVENT_CATEGORIES_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EventCategoriesList")
.getter(getter(EventSubscription::eventCategoriesList))
.setter(setter(Builder::eventCategoriesList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventCategoriesList").build(),
ListTrait
.builder()
.memberLocationName("EventCategory")
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("EventCategory").build()).build()).build()).build();
private static final SdkField ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Enabled").getter(getter(EventSubscription::enabled)).setter(setter(Builder::enabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Enabled").build()).build();
private static final SdkField EVENT_SUBSCRIPTION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EventSubscriptionArn").getter(getter(EventSubscription::eventSubscriptionArn))
.setter(setter(Builder::eventSubscriptionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EventSubscriptionArn").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CUSTOMER_AWS_ID_FIELD,
CUST_SUBSCRIPTION_ID_FIELD, SNS_TOPIC_ARN_FIELD, STATUS_FIELD, SUBSCRIPTION_CREATION_TIME_FIELD, SOURCE_TYPE_FIELD,
SOURCE_IDS_LIST_FIELD, EVENT_CATEGORIES_LIST_FIELD, ENABLED_FIELD, EVENT_SUBSCRIPTION_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final String customerAwsId;
private final String custSubscriptionId;
private final String snsTopicArn;
private final String status;
private final String subscriptionCreationTime;
private final String sourceType;
private final List sourceIdsList;
private final List eventCategoriesList;
private final Boolean enabled;
private final String eventSubscriptionArn;
private EventSubscription(BuilderImpl builder) {
this.customerAwsId = builder.customerAwsId;
this.custSubscriptionId = builder.custSubscriptionId;
this.snsTopicArn = builder.snsTopicArn;
this.status = builder.status;
this.subscriptionCreationTime = builder.subscriptionCreationTime;
this.sourceType = builder.sourceType;
this.sourceIdsList = builder.sourceIdsList;
this.eventCategoriesList = builder.eventCategoriesList;
this.enabled = builder.enabled;
this.eventSubscriptionArn = builder.eventSubscriptionArn;
}
/**
*
* The Amazon customer account associated with the event notification subscription.
*
*
* @return The Amazon customer account associated with the event notification subscription.
*/
public final String customerAwsId() {
return customerAwsId;
}
/**
*
* The event notification subscription Id.
*
*
* @return The event notification subscription Id.
*/
public final String custSubscriptionId() {
return custSubscriptionId;
}
/**
*
* The topic ARN of the event notification subscription.
*
*
* @return The topic ARN of the event notification subscription.
*/
public final String snsTopicArn() {
return snsTopicArn;
}
/**
*
* The status of the event notification subscription.
*
*
* Constraints:
*
*
* Can be one of the following: creating | modifying | deleting | active | no-permission | topic-not-exist
*
*
* The status "no-permission" indicates that Neptune no longer has permission to post to the SNS topic. The status
* "topic-not-exist" indicates that the topic was deleted after the subscription was created.
*
*
* @return The status of the event notification subscription.
*
* Constraints:
*
*
* Can be one of the following: creating | modifying | deleting | active | no-permission | topic-not-exist
*
*
* The status "no-permission" indicates that Neptune no longer has permission to post to the SNS topic. The
* status "topic-not-exist" indicates that the topic was deleted after the subscription was created.
*/
public final String status() {
return status;
}
/**
*
* The time the event notification subscription was created.
*
*
* @return The time the event notification subscription was created.
*/
public final String subscriptionCreationTime() {
return subscriptionCreationTime;
}
/**
*
* The source type for the event notification subscription.
*
*
* @return The source type for the event notification subscription.
*/
public final String sourceType() {
return sourceType;
}
/**
* For responses, this returns true if the service returned a value for the SourceIdsList property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSourceIdsList() {
return sourceIdsList != null && !(sourceIdsList instanceof SdkAutoConstructList);
}
/**
*
* A list of source IDs for the event notification subscription.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSourceIdsList} method.
*
*
* @return A list of source IDs for the event notification subscription.
*/
public final List sourceIdsList() {
return sourceIdsList;
}
/**
* For responses, this returns true if the service returned a value for the EventCategoriesList property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEventCategoriesList() {
return eventCategoriesList != null && !(eventCategoriesList instanceof SdkAutoConstructList);
}
/**
*
* A list of event categories for the event notification subscription.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEventCategoriesList} method.
*
*
* @return A list of event categories for the event notification subscription.
*/
public final List eventCategoriesList() {
return eventCategoriesList;
}
/**
*
* A Boolean value indicating if the subscription is enabled. True indicates the subscription is enabled.
*
*
* @return A Boolean value indicating if the subscription is enabled. True indicates the subscription is enabled.
*/
public final Boolean enabled() {
return enabled;
}
/**
*
* The Amazon Resource Name (ARN) for the event subscription.
*
*
* @return The Amazon Resource Name (ARN) for the event subscription.
*/
public final String eventSubscriptionArn() {
return eventSubscriptionArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(customerAwsId());
hashCode = 31 * hashCode + Objects.hashCode(custSubscriptionId());
hashCode = 31 * hashCode + Objects.hashCode(snsTopicArn());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(subscriptionCreationTime());
hashCode = 31 * hashCode + Objects.hashCode(sourceType());
hashCode = 31 * hashCode + Objects.hashCode(hasSourceIdsList() ? sourceIdsList() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasEventCategoriesList() ? eventCategoriesList() : null);
hashCode = 31 * hashCode + Objects.hashCode(enabled());
hashCode = 31 * hashCode + Objects.hashCode(eventSubscriptionArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof EventSubscription)) {
return false;
}
EventSubscription other = (EventSubscription) obj;
return Objects.equals(customerAwsId(), other.customerAwsId())
&& Objects.equals(custSubscriptionId(), other.custSubscriptionId())
&& Objects.equals(snsTopicArn(), other.snsTopicArn()) && Objects.equals(status(), other.status())
&& Objects.equals(subscriptionCreationTime(), other.subscriptionCreationTime())
&& Objects.equals(sourceType(), other.sourceType()) && hasSourceIdsList() == other.hasSourceIdsList()
&& Objects.equals(sourceIdsList(), other.sourceIdsList())
&& hasEventCategoriesList() == other.hasEventCategoriesList()
&& Objects.equals(eventCategoriesList(), other.eventCategoriesList())
&& Objects.equals(enabled(), other.enabled())
&& Objects.equals(eventSubscriptionArn(), other.eventSubscriptionArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("EventSubscription").add("CustomerAwsId", customerAwsId())
.add("CustSubscriptionId", custSubscriptionId()).add("SnsTopicArn", snsTopicArn()).add("Status", status())
.add("SubscriptionCreationTime", subscriptionCreationTime()).add("SourceType", sourceType())
.add("SourceIdsList", hasSourceIdsList() ? sourceIdsList() : null)
.add("EventCategoriesList", hasEventCategoriesList() ? eventCategoriesList() : null).add("Enabled", enabled())
.add("EventSubscriptionArn", eventSubscriptionArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CustomerAwsId":
return Optional.ofNullable(clazz.cast(customerAwsId()));
case "CustSubscriptionId":
return Optional.ofNullable(clazz.cast(custSubscriptionId()));
case "SnsTopicArn":
return Optional.ofNullable(clazz.cast(snsTopicArn()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "SubscriptionCreationTime":
return Optional.ofNullable(clazz.cast(subscriptionCreationTime()));
case "SourceType":
return Optional.ofNullable(clazz.cast(sourceType()));
case "SourceIdsList":
return Optional.ofNullable(clazz.cast(sourceIdsList()));
case "EventCategoriesList":
return Optional.ofNullable(clazz.cast(eventCategoriesList()));
case "Enabled":
return Optional.ofNullable(clazz.cast(enabled()));
case "EventSubscriptionArn":
return Optional.ofNullable(clazz.cast(eventSubscriptionArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function