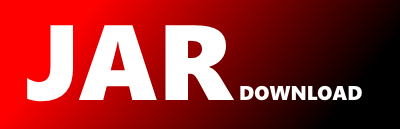
software.amazon.awssdk.services.neptune.model.PendingMaintenanceAction Maven / Gradle / Ivy
Show all versions of neptune Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptune.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides information about a pending maintenance action for a resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PendingMaintenanceAction implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ACTION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Action")
.getter(getter(PendingMaintenanceAction::action)).setter(setter(Builder::action))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Action").build()).build();
private static final SdkField AUTO_APPLIED_AFTER_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("AutoAppliedAfterDate").getter(getter(PendingMaintenanceAction::autoAppliedAfterDate))
.setter(setter(Builder::autoAppliedAfterDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoAppliedAfterDate").build())
.build();
private static final SdkField FORCED_APPLY_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("ForcedApplyDate").getter(getter(PendingMaintenanceAction::forcedApplyDate))
.setter(setter(Builder::forcedApplyDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForcedApplyDate").build()).build();
private static final SdkField OPT_IN_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OptInStatus").getter(getter(PendingMaintenanceAction::optInStatus)).setter(setter(Builder::optInStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OptInStatus").build()).build();
private static final SdkField CURRENT_APPLY_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CurrentApplyDate").getter(getter(PendingMaintenanceAction::currentApplyDate))
.setter(setter(Builder::currentApplyDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentApplyDate").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(PendingMaintenanceAction::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ACTION_FIELD,
AUTO_APPLIED_AFTER_DATE_FIELD, FORCED_APPLY_DATE_FIELD, OPT_IN_STATUS_FIELD, CURRENT_APPLY_DATE_FIELD,
DESCRIPTION_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("Action", ACTION_FIELD);
put("AutoAppliedAfterDate", AUTO_APPLIED_AFTER_DATE_FIELD);
put("ForcedApplyDate", FORCED_APPLY_DATE_FIELD);
put("OptInStatus", OPT_IN_STATUS_FIELD);
put("CurrentApplyDate", CURRENT_APPLY_DATE_FIELD);
put("Description", DESCRIPTION_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String action;
private final Instant autoAppliedAfterDate;
private final Instant forcedApplyDate;
private final String optInStatus;
private final Instant currentApplyDate;
private final String description;
private PendingMaintenanceAction(BuilderImpl builder) {
this.action = builder.action;
this.autoAppliedAfterDate = builder.autoAppliedAfterDate;
this.forcedApplyDate = builder.forcedApplyDate;
this.optInStatus = builder.optInStatus;
this.currentApplyDate = builder.currentApplyDate;
this.description = builder.description;
}
/**
*
* The type of pending maintenance action that is available for the resource.
*
*
* @return The type of pending maintenance action that is available for the resource.
*/
public final String action() {
return action;
}
/**
*
* The date of the maintenance window when the action is applied. The maintenance action is applied to the resource
* during its first maintenance window after this date. If this date is specified, any next-maintenance
* opt-in requests are ignored.
*
*
* @return The date of the maintenance window when the action is applied. The maintenance action is applied to the
* resource during its first maintenance window after this date. If this date is specified, any
* next-maintenance
opt-in requests are ignored.
*/
public final Instant autoAppliedAfterDate() {
return autoAppliedAfterDate;
}
/**
*
* The date when the maintenance action is automatically applied. The maintenance action is applied to the resource
* on this date regardless of the maintenance window for the resource. If this date is specified, any
* immediate
opt-in requests are ignored.
*
*
* @return The date when the maintenance action is automatically applied. The maintenance action is applied to the
* resource on this date regardless of the maintenance window for the resource. If this date is specified,
* any immediate
opt-in requests are ignored.
*/
public final Instant forcedApplyDate() {
return forcedApplyDate;
}
/**
*
* Indicates the type of opt-in request that has been received for the resource.
*
*
* @return Indicates the type of opt-in request that has been received for the resource.
*/
public final String optInStatus() {
return optInStatus;
}
/**
*
* The effective date when the pending maintenance action is applied to the resource. This date takes into account
* opt-in requests received from the ApplyPendingMaintenanceAction API, the AutoAppliedAfterDate
* , and the ForcedApplyDate
. This value is blank if an opt-in request has not been received and
* nothing has been specified as AutoAppliedAfterDate
or ForcedApplyDate
.
*
*
* @return The effective date when the pending maintenance action is applied to the resource. This date takes into
* account opt-in requests received from the ApplyPendingMaintenanceAction API, the
* AutoAppliedAfterDate
, and the ForcedApplyDate
. This value is blank if an opt-in
* request has not been received and nothing has been specified as AutoAppliedAfterDate
or
* ForcedApplyDate
.
*/
public final Instant currentApplyDate() {
return currentApplyDate;
}
/**
*
* A description providing more detail about the maintenance action.
*
*
* @return A description providing more detail about the maintenance action.
*/
public final String description() {
return description;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(action());
hashCode = 31 * hashCode + Objects.hashCode(autoAppliedAfterDate());
hashCode = 31 * hashCode + Objects.hashCode(forcedApplyDate());
hashCode = 31 * hashCode + Objects.hashCode(optInStatus());
hashCode = 31 * hashCode + Objects.hashCode(currentApplyDate());
hashCode = 31 * hashCode + Objects.hashCode(description());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PendingMaintenanceAction)) {
return false;
}
PendingMaintenanceAction other = (PendingMaintenanceAction) obj;
return Objects.equals(action(), other.action()) && Objects.equals(autoAppliedAfterDate(), other.autoAppliedAfterDate())
&& Objects.equals(forcedApplyDate(), other.forcedApplyDate())
&& Objects.equals(optInStatus(), other.optInStatus())
&& Objects.equals(currentApplyDate(), other.currentApplyDate())
&& Objects.equals(description(), other.description());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PendingMaintenanceAction").add("Action", action())
.add("AutoAppliedAfterDate", autoAppliedAfterDate()).add("ForcedApplyDate", forcedApplyDate())
.add("OptInStatus", optInStatus()).add("CurrentApplyDate", currentApplyDate()).add("Description", description())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Action":
return Optional.ofNullable(clazz.cast(action()));
case "AutoAppliedAfterDate":
return Optional.ofNullable(clazz.cast(autoAppliedAfterDate()));
case "ForcedApplyDate":
return Optional.ofNullable(clazz.cast(forcedApplyDate()));
case "OptInStatus":
return Optional.ofNullable(clazz.cast(optInStatus()));
case "CurrentApplyDate":
return Optional.ofNullable(clazz.cast(currentApplyDate()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function