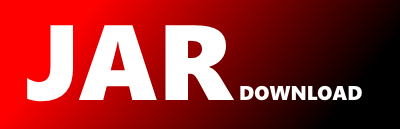
software.amazon.awssdk.services.neptunegraph.model.GetGraphResponse Maven / Gradle / Ivy
Show all versions of neptunegraph Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptunegraph.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetGraphResponse extends NeptuneGraphResponse implements
ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("id")
.getter(getter(GetGraphResponse::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("id").build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(GetGraphResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(GetGraphResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(GetGraphResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("statusReason").getter(getter(GetGraphResponse::statusReason)).setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("statusReason").build()).build();
private static final SdkField CREATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createTime").getter(getter(GetGraphResponse::createTime)).setter(setter(Builder::createTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createTime").build()).build();
private static final SdkField PROVISIONED_MEMORY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("provisionedMemory").getter(getter(GetGraphResponse::provisionedMemory))
.setter(setter(Builder::provisionedMemory))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("provisionedMemory").build()).build();
private static final SdkField ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("endpoint").getter(getter(GetGraphResponse::endpoint)).setter(setter(Builder::endpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endpoint").build()).build();
private static final SdkField PUBLIC_CONNECTIVITY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("publicConnectivity").getter(getter(GetGraphResponse::publicConnectivity))
.setter(setter(Builder::publicConnectivity))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("publicConnectivity").build())
.build();
private static final SdkField VECTOR_SEARCH_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("vectorSearchConfiguration")
.getter(getter(GetGraphResponse::vectorSearchConfiguration)).setter(setter(Builder::vectorSearchConfiguration))
.constructor(VectorSearchConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("vectorSearchConfiguration").build())
.build();
private static final SdkField REPLICA_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("replicaCount").getter(getter(GetGraphResponse::replicaCount)).setter(setter(Builder::replicaCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("replicaCount").build()).build();
private static final SdkField KMS_KEY_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyIdentifier").getter(getter(GetGraphResponse::kmsKeyIdentifier))
.setter(setter(Builder::kmsKeyIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyIdentifier").build()).build();
private static final SdkField SOURCE_SNAPSHOT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sourceSnapshotId").getter(getter(GetGraphResponse::sourceSnapshotId))
.setter(setter(Builder::sourceSnapshotId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sourceSnapshotId").build()).build();
private static final SdkField DELETION_PROTECTION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("deletionProtection").getter(getter(GetGraphResponse::deletionProtection))
.setter(setter(Builder::deletionProtection))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deletionProtection").build())
.build();
private static final SdkField BUILD_NUMBER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("buildNumber").getter(getter(GetGraphResponse::buildNumber)).setter(setter(Builder::buildNumber))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("buildNumber").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, NAME_FIELD,
ARN_FIELD, STATUS_FIELD, STATUS_REASON_FIELD, CREATE_TIME_FIELD, PROVISIONED_MEMORY_FIELD, ENDPOINT_FIELD,
PUBLIC_CONNECTIVITY_FIELD, VECTOR_SEARCH_CONFIGURATION_FIELD, REPLICA_COUNT_FIELD, KMS_KEY_IDENTIFIER_FIELD,
SOURCE_SNAPSHOT_ID_FIELD, DELETION_PROTECTION_FIELD, BUILD_NUMBER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String id;
private final String name;
private final String arn;
private final String status;
private final String statusReason;
private final Instant createTime;
private final Integer provisionedMemory;
private final String endpoint;
private final Boolean publicConnectivity;
private final VectorSearchConfiguration vectorSearchConfiguration;
private final Integer replicaCount;
private final String kmsKeyIdentifier;
private final String sourceSnapshotId;
private final Boolean deletionProtection;
private final String buildNumber;
private GetGraphResponse(BuilderImpl builder) {
super(builder);
this.id = builder.id;
this.name = builder.name;
this.arn = builder.arn;
this.status = builder.status;
this.statusReason = builder.statusReason;
this.createTime = builder.createTime;
this.provisionedMemory = builder.provisionedMemory;
this.endpoint = builder.endpoint;
this.publicConnectivity = builder.publicConnectivity;
this.vectorSearchConfiguration = builder.vectorSearchConfiguration;
this.replicaCount = builder.replicaCount;
this.kmsKeyIdentifier = builder.kmsKeyIdentifier;
this.sourceSnapshotId = builder.sourceSnapshotId;
this.deletionProtection = builder.deletionProtection;
this.buildNumber = builder.buildNumber;
}
/**
*
* The unique identifier of the graph.
*
*
* @return The unique identifier of the graph.
*/
public final String id() {
return id;
}
/**
*
* The name of the graph.
*
*
* @return The name of the graph.
*/
public final String name() {
return name;
}
/**
*
* The ARN associated with the graph.
*
*
* @return The ARN associated with the graph.
*/
public final String arn() {
return arn;
}
/**
*
* The status of the graph.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link GraphStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the graph.
* @see GraphStatus
*/
public final GraphStatus status() {
return GraphStatus.fromValue(status);
}
/**
*
* The status of the graph.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link GraphStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the graph.
* @see GraphStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The reason that the graph has this status.
*
*
* @return The reason that the graph has this status.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* The time at which the graph was created.
*
*
* @return The time at which the graph was created.
*/
public final Instant createTime() {
return createTime;
}
/**
*
* The number of memory-optimized Neptune Capacity Units (m-NCUs) allocated to the graph.
*
*
* @return The number of memory-optimized Neptune Capacity Units (m-NCUs) allocated to the graph.
*/
public final Integer provisionedMemory() {
return provisionedMemory;
}
/**
*
* The graph endpoint.
*
*
* @return The graph endpoint.
*/
public final String endpoint() {
return endpoint;
}
/**
*
* If true
, the graph has a public endpoint, otherwise not.
*
*
* @return If true
, the graph has a public endpoint, otherwise not.
*/
public final Boolean publicConnectivity() {
return publicConnectivity;
}
/**
* Returns the value of the VectorSearchConfiguration property for this object.
*
* @return The value of the VectorSearchConfiguration property for this object.
*/
public final VectorSearchConfiguration vectorSearchConfiguration() {
return vectorSearchConfiguration;
}
/**
*
* The number of replicas for the graph.
*
*
* @return The number of replicas for the graph.
*/
public final Integer replicaCount() {
return replicaCount;
}
/**
*
* The ID of the KMS key used to encrypt and decrypt graph data.
*
*
* @return The ID of the KMS key used to encrypt and decrypt graph data.
*/
public final String kmsKeyIdentifier() {
return kmsKeyIdentifier;
}
/**
*
* The ID of the snapshot from which the graph was created, if it was created from a snapshot.
*
*
* @return The ID of the snapshot from which the graph was created, if it was created from a snapshot.
*/
public final String sourceSnapshotId() {
return sourceSnapshotId;
}
/**
*
* If true
, deletion protection is enabled for the graph.
*
*
* @return If true
, deletion protection is enabled for the graph.
*/
public final Boolean deletionProtection() {
return deletionProtection;
}
/**
*
* The build number of the graph.
*
*
* @return The build number of the graph.
*/
public final String buildNumber() {
return buildNumber;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(createTime());
hashCode = 31 * hashCode + Objects.hashCode(provisionedMemory());
hashCode = 31 * hashCode + Objects.hashCode(endpoint());
hashCode = 31 * hashCode + Objects.hashCode(publicConnectivity());
hashCode = 31 * hashCode + Objects.hashCode(vectorSearchConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(replicaCount());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(sourceSnapshotId());
hashCode = 31 * hashCode + Objects.hashCode(deletionProtection());
hashCode = 31 * hashCode + Objects.hashCode(buildNumber());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetGraphResponse)) {
return false;
}
GetGraphResponse other = (GetGraphResponse) obj;
return Objects.equals(id(), other.id()) && Objects.equals(name(), other.name()) && Objects.equals(arn(), other.arn())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(statusReason(), other.statusReason()) && Objects.equals(createTime(), other.createTime())
&& Objects.equals(provisionedMemory(), other.provisionedMemory()) && Objects.equals(endpoint(), other.endpoint())
&& Objects.equals(publicConnectivity(), other.publicConnectivity())
&& Objects.equals(vectorSearchConfiguration(), other.vectorSearchConfiguration())
&& Objects.equals(replicaCount(), other.replicaCount())
&& Objects.equals(kmsKeyIdentifier(), other.kmsKeyIdentifier())
&& Objects.equals(sourceSnapshotId(), other.sourceSnapshotId())
&& Objects.equals(deletionProtection(), other.deletionProtection())
&& Objects.equals(buildNumber(), other.buildNumber());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetGraphResponse").add("Id", id()).add("Name", name()).add("Arn", arn())
.add("Status", statusAsString()).add("StatusReason", statusReason()).add("CreateTime", createTime())
.add("ProvisionedMemory", provisionedMemory()).add("Endpoint", endpoint())
.add("PublicConnectivity", publicConnectivity()).add("VectorSearchConfiguration", vectorSearchConfiguration())
.add("ReplicaCount", replicaCount()).add("KmsKeyIdentifier", kmsKeyIdentifier())
.add("SourceSnapshotId", sourceSnapshotId()).add("DeletionProtection", deletionProtection())
.add("BuildNumber", buildNumber()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "id":
return Optional.ofNullable(clazz.cast(id()));
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "statusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "createTime":
return Optional.ofNullable(clazz.cast(createTime()));
case "provisionedMemory":
return Optional.ofNullable(clazz.cast(provisionedMemory()));
case "endpoint":
return Optional.ofNullable(clazz.cast(endpoint()));
case "publicConnectivity":
return Optional.ofNullable(clazz.cast(publicConnectivity()));
case "vectorSearchConfiguration":
return Optional.ofNullable(clazz.cast(vectorSearchConfiguration()));
case "replicaCount":
return Optional.ofNullable(clazz.cast(replicaCount()));
case "kmsKeyIdentifier":
return Optional.ofNullable(clazz.cast(kmsKeyIdentifier()));
case "sourceSnapshotId":
return Optional.ofNullable(clazz.cast(sourceSnapshotId()));
case "deletionProtection":
return Optional.ofNullable(clazz.cast(deletionProtection()));
case "buildNumber":
return Optional.ofNullable(clazz.cast(buildNumber()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("id", ID_FIELD);
map.put("name", NAME_FIELD);
map.put("arn", ARN_FIELD);
map.put("status", STATUS_FIELD);
map.put("statusReason", STATUS_REASON_FIELD);
map.put("createTime", CREATE_TIME_FIELD);
map.put("provisionedMemory", PROVISIONED_MEMORY_FIELD);
map.put("endpoint", ENDPOINT_FIELD);
map.put("publicConnectivity", PUBLIC_CONNECTIVITY_FIELD);
map.put("vectorSearchConfiguration", VECTOR_SEARCH_CONFIGURATION_FIELD);
map.put("replicaCount", REPLICA_COUNT_FIELD);
map.put("kmsKeyIdentifier", KMS_KEY_IDENTIFIER_FIELD);
map.put("sourceSnapshotId", SOURCE_SNAPSHOT_ID_FIELD);
map.put("deletionProtection", DELETION_PROTECTION_FIELD);
map.put("buildNumber", BUILD_NUMBER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function