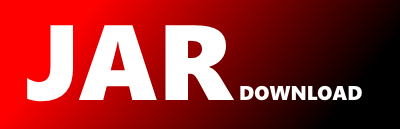
software.amazon.awssdk.services.neptunegraph.model.StartExportTaskResponse Maven / Gradle / Ivy
Show all versions of neptunegraph Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.neptunegraph.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartExportTaskResponse extends NeptuneGraphResponse implements
ToCopyableBuilder {
private static final SdkField GRAPH_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("graphId").getter(getter(StartExportTaskResponse::graphId)).setter(setter(Builder::graphId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("graphId").build()).build();
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleArn").getter(getter(StartExportTaskResponse::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField TASK_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("taskId")
.getter(getter(StartExportTaskResponse::taskId)).setter(setter(Builder::taskId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(StartExportTaskResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField FORMAT_FIELD = SdkField. builder(MarshallingType.STRING).memberName("format")
.getter(getter(StartExportTaskResponse::formatAsString)).setter(setter(Builder::format))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("format").build()).build();
private static final SdkField DESTINATION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("destination").getter(getter(StartExportTaskResponse::destination)).setter(setter(Builder::destination))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("destination").build()).build();
private static final SdkField KMS_KEY_IDENTIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("kmsKeyIdentifier").getter(getter(StartExportTaskResponse::kmsKeyIdentifier))
.setter(setter(Builder::kmsKeyIdentifier))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("kmsKeyIdentifier").build()).build();
private static final SdkField PARQUET_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("parquetType").getter(getter(StartExportTaskResponse::parquetTypeAsString))
.setter(setter(Builder::parquetType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parquetType").build()).build();
private static final SdkField STATUS_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("statusReason").getter(getter(StartExportTaskResponse::statusReason))
.setter(setter(Builder::statusReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("statusReason").build()).build();
private static final SdkField EXPORT_FILTER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("exportFilter").getter(getter(StartExportTaskResponse::exportFilter))
.setter(setter(Builder::exportFilter)).constructor(ExportFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("exportFilter").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(GRAPH_ID_FIELD,
ROLE_ARN_FIELD, TASK_ID_FIELD, STATUS_FIELD, FORMAT_FIELD, DESTINATION_FIELD, KMS_KEY_IDENTIFIER_FIELD,
PARQUET_TYPE_FIELD, STATUS_REASON_FIELD, EXPORT_FILTER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String graphId;
private final String roleArn;
private final String taskId;
private final String status;
private final String format;
private final String destination;
private final String kmsKeyIdentifier;
private final String parquetType;
private final String statusReason;
private final ExportFilter exportFilter;
private StartExportTaskResponse(BuilderImpl builder) {
super(builder);
this.graphId = builder.graphId;
this.roleArn = builder.roleArn;
this.taskId = builder.taskId;
this.status = builder.status;
this.format = builder.format;
this.destination = builder.destination;
this.kmsKeyIdentifier = builder.kmsKeyIdentifier;
this.parquetType = builder.parquetType;
this.statusReason = builder.statusReason;
this.exportFilter = builder.exportFilter;
}
/**
*
* The source graph identifier of the export task.
*
*
* @return The source graph identifier of the export task.
*/
public final String graphId() {
return graphId;
}
/**
*
* The ARN of the IAM role that will allow data to be exported to the destination.
*
*
* @return The ARN of the IAM role that will allow data to be exported to the destination.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The unique identifier of the export task.
*
*
* @return The unique identifier of the export task.
*/
public final String taskId() {
return taskId;
}
/**
*
* The current status of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExportTaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the export task.
* @see ExportTaskStatus
*/
public final ExportTaskStatus status() {
return ExportTaskStatus.fromValue(status);
}
/**
*
* The current status of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link ExportTaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The current status of the export task.
* @see ExportTaskStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The format of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link ExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the export task.
* @see ExportFormat
*/
public final ExportFormat format() {
return ExportFormat.fromValue(format);
}
/**
*
* The format of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #format} will
* return {@link ExportFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #formatAsString}.
*
*
* @return The format of the export task.
* @see ExportFormat
*/
public final String formatAsString() {
return format;
}
/**
*
* The Amazon S3 URI of the export task where data will be exported to.
*
*
* @return The Amazon S3 URI of the export task where data will be exported to.
*/
public final String destination() {
return destination;
}
/**
*
* The KMS key identifier of the export task.
*
*
* @return The KMS key identifier of the export task.
*/
public final String kmsKeyIdentifier() {
return kmsKeyIdentifier;
}
/**
*
* The parquet type of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #parquetType} will
* return {@link ParquetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #parquetTypeAsString}.
*
*
* @return The parquet type of the export task.
* @see ParquetType
*/
public final ParquetType parquetType() {
return ParquetType.fromValue(parquetType);
}
/**
*
* The parquet type of the export task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #parquetType} will
* return {@link ParquetType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #parquetTypeAsString}.
*
*
* @return The parquet type of the export task.
* @see ParquetType
*/
public final String parquetTypeAsString() {
return parquetType;
}
/**
*
* The reason that the export task has this status value.
*
*
* @return The reason that the export task has this status value.
*/
public final String statusReason() {
return statusReason;
}
/**
*
* The export filter of the export task.
*
*
* @return The export filter of the export task.
*/
public final ExportFilter exportFilter() {
return exportFilter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(graphId());
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(taskId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(formatAsString());
hashCode = 31 * hashCode + Objects.hashCode(destination());
hashCode = 31 * hashCode + Objects.hashCode(kmsKeyIdentifier());
hashCode = 31 * hashCode + Objects.hashCode(parquetTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusReason());
hashCode = 31 * hashCode + Objects.hashCode(exportFilter());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartExportTaskResponse)) {
return false;
}
StartExportTaskResponse other = (StartExportTaskResponse) obj;
return Objects.equals(graphId(), other.graphId()) && Objects.equals(roleArn(), other.roleArn())
&& Objects.equals(taskId(), other.taskId()) && Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(formatAsString(), other.formatAsString()) && Objects.equals(destination(), other.destination())
&& Objects.equals(kmsKeyIdentifier(), other.kmsKeyIdentifier())
&& Objects.equals(parquetTypeAsString(), other.parquetTypeAsString())
&& Objects.equals(statusReason(), other.statusReason()) && Objects.equals(exportFilter(), other.exportFilter());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartExportTaskResponse").add("GraphId", graphId()).add("RoleArn", roleArn())
.add("TaskId", taskId()).add("Status", statusAsString()).add("Format", formatAsString())
.add("Destination", destination()).add("KmsKeyIdentifier", kmsKeyIdentifier())
.add("ParquetType", parquetTypeAsString()).add("StatusReason", statusReason())
.add("ExportFilter", exportFilter()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "graphId":
return Optional.ofNullable(clazz.cast(graphId()));
case "roleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "taskId":
return Optional.ofNullable(clazz.cast(taskId()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "format":
return Optional.ofNullable(clazz.cast(formatAsString()));
case "destination":
return Optional.ofNullable(clazz.cast(destination()));
case "kmsKeyIdentifier":
return Optional.ofNullable(clazz.cast(kmsKeyIdentifier()));
case "parquetType":
return Optional.ofNullable(clazz.cast(parquetTypeAsString()));
case "statusReason":
return Optional.ofNullable(clazz.cast(statusReason()));
case "exportFilter":
return Optional.ofNullable(clazz.cast(exportFilter()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("graphId", GRAPH_ID_FIELD);
map.put("roleArn", ROLE_ARN_FIELD);
map.put("taskId", TASK_ID_FIELD);
map.put("status", STATUS_FIELD);
map.put("format", FORMAT_FIELD);
map.put("destination", DESTINATION_FIELD);
map.put("kmsKeyIdentifier", KMS_KEY_IDENTIFIER_FIELD);
map.put("parquetType", PARQUET_TYPE_FIELD);
map.put("statusReason", STATUS_REASON_FIELD);
map.put("exportFilter", EXPORT_FILTER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function