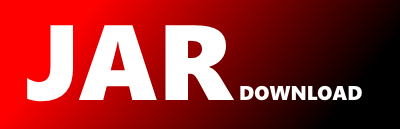
software.amazon.awssdk.services.networkmanager.NetworkManagerAsyncClient Maven / Gradle / Ivy
Show all versions of networkmanager Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.networkmanager;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.networkmanager.model.AssociateCustomerGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateCustomerGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.AssociateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteSiteResponse;
import software.amazon.awssdk.services.networkmanager.model.DeregisterTransitGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.DeregisterTransitGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest;
import software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateCustomerGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateCustomerGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetLinksRequest;
import software.amazon.awssdk.services.networkmanager.model.GetLinksResponse;
import software.amazon.awssdk.services.networkmanager.model.GetSitesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetSitesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse;
import software.amazon.awssdk.services.networkmanager.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.RegisterTransitGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.RegisterTransitGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.TagResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.TagResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.UntagResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.UntagResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateSiteResponse;
import software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher;
/**
* Service client for accessing NetworkManager asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* Transit Gateway Network Manager (Network Manager) enables you to create a global network, in which you can monitor
* your AWS and on-premises networks that are built around transit gateways.
*
*/
@Generated("software.amazon.awssdk:codegen")
public interface NetworkManagerAsyncClient extends SdkClient {
String SERVICE_NAME = "networkmanager";
/**
* Create a {@link NetworkManagerAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static NetworkManagerAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link NetworkManagerAsyncClient}.
*/
static NetworkManagerAsyncClientBuilder builder() {
return new DefaultNetworkManagerAsyncClientBuilder();
}
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway. The transit
* gateway must be registered in your global network. When you register a transit gateway, customer gateways that
* are connected to the transit gateway are automatically included in the global network. To list customer gateways
* that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
* @param associateCustomerGatewayRequest
* @return A Java Future containing the result of the AssociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture associateCustomerGateway(
AssociateCustomerGatewayRequest associateCustomerGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway. The transit
* gateway must be registered in your global network. When you register a transit gateway, customer gateways that
* are connected to the transit gateway are automatically included in the global network. To list customer gateways
* that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateCustomerGatewayRequest.Builder} avoiding
* the need to create one manually via {@link AssociateCustomerGatewayRequest#builder()}
*
*
* @param associateCustomerGatewayRequest
* A {@link Consumer} that will call methods on {@link AssociateCustomerGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture associateCustomerGateway(
Consumer associateCustomerGatewayRequest) {
return associateCustomerGateway(AssociateCustomerGatewayRequest.builder().applyMutation(associateCustomerGatewayRequest)
.build());
}
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
* @param associateLinkRequest
* @return A Java Future containing the result of the AssociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateLink
* @see AWS
* API Documentation
*/
default CompletableFuture associateLink(AssociateLinkRequest associateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateLinkRequest.Builder} avoiding the need to
* create one manually via {@link AssociateLinkRequest#builder()}
*
*
* @param associateLinkRequest
* A {@link Consumer} that will call methods on {@link AssociateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateLink
* @see AWS
* API Documentation
*/
default CompletableFuture associateLink(Consumer associateLinkRequest) {
return associateLink(AssociateLinkRequest.builder().applyMutation(associateLinkRequest).build());
}
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
* @param createDeviceRequest
* @return A Java Future containing the result of the CreateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture createDevice(CreateDeviceRequest createDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDeviceRequest.Builder} avoiding the need to
* create one manually via {@link CreateDeviceRequest#builder()}
*
*
* @param createDeviceRequest
* A {@link Consumer} that will call methods on {@link CreateDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture createDevice(Consumer createDeviceRequest) {
return createDevice(CreateDeviceRequest.builder().applyMutation(createDeviceRequest).build());
}
/**
*
* Creates a new, empty global network.
*
*
* @param createGlobalNetworkRequest
* @return A Java Future containing the result of the CreateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture createGlobalNetwork(
CreateGlobalNetworkRequest createGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new, empty global network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link CreateGlobalNetworkRequest#builder()}
*
*
* @param createGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link CreateGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture createGlobalNetwork(
Consumer createGlobalNetworkRequest) {
return createGlobalNetwork(CreateGlobalNetworkRequest.builder().applyMutation(createGlobalNetworkRequest).build());
}
/**
*
* Creates a new link for a specified site.
*
*
* @param createLinkRequest
* @return A Java Future containing the result of the CreateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateLink
* @see AWS API
* Documentation
*/
default CompletableFuture createLink(CreateLinkRequest createLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new link for a specified site.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLinkRequest.Builder} avoiding the need to
* create one manually via {@link CreateLinkRequest#builder()}
*
*
* @param createLinkRequest
* A {@link Consumer} that will call methods on {@link CreateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateLink
* @see AWS API
* Documentation
*/
default CompletableFuture createLink(Consumer createLinkRequest) {
return createLink(CreateLinkRequest.builder().applyMutation(createLinkRequest).build());
}
/**
*
* Creates a new site in a global network.
*
*
* @param createSiteRequest
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(CreateSiteRequest createSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new site in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSiteRequest.Builder} avoiding the need to
* create one manually via {@link CreateSiteRequest#builder()}
*
*
* @param createSiteRequest
* A {@link Consumer} that will call methods on {@link CreateSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(Consumer createSiteRequest) {
return createSite(CreateSiteRequest.builder().applyMutation(createSiteRequest).build());
}
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
* @param deleteDeviceRequest
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(DeleteDeviceRequest deleteDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDeviceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDeviceRequest#builder()}
*
*
* @param deleteDeviceRequest
* A {@link Consumer} that will call methods on {@link DeleteDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(Consumer deleteDeviceRequest) {
return deleteDevice(DeleteDeviceRequest.builder().applyMutation(deleteDeviceRequest).build());
}
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites)
* and deregister all transit gateways.
*
*
* @param deleteGlobalNetworkRequest
* @return A Java Future containing the result of the DeleteGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalNetwork(
DeleteGlobalNetworkRequest deleteGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites)
* and deregister all transit gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link DeleteGlobalNetworkRequest#builder()}
*
*
* @param deleteGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link DeleteGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalNetwork(
Consumer deleteGlobalNetworkRequest) {
return deleteGlobalNetwork(DeleteGlobalNetworkRequest.builder().applyMutation(deleteGlobalNetworkRequest).build());
}
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
* @param deleteLinkRequest
* @return A Java Future containing the result of the DeleteLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteLink
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLink(DeleteLinkRequest deleteLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLinkRequest.Builder} avoiding the need to
* create one manually via {@link DeleteLinkRequest#builder()}
*
*
* @param deleteLinkRequest
* A {@link Consumer} that will call methods on {@link DeleteLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteLink
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLink(Consumer deleteLinkRequest) {
return deleteLink(DeleteLinkRequest.builder().applyMutation(deleteLinkRequest).build());
}
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
* @param deleteSiteRequest
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(DeleteSiteRequest deleteSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSiteRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSiteRequest#builder()}
*
*
* @param deleteSiteRequest
* A {@link Consumer} that will call methods on {@link DeleteSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(Consumer deleteSiteRequest) {
return deleteSite(DeleteSiteRequest.builder().applyMutation(deleteSiteRequest).build());
}
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
* @param deregisterTransitGatewayRequest
* @return A Java Future containing the result of the DeregisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeregisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture deregisterTransitGateway(
DeregisterTransitGatewayRequest deregisterTransitGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterTransitGatewayRequest.Builder} avoiding
* the need to create one manually via {@link DeregisterTransitGatewayRequest#builder()}
*
*
* @param deregisterTransitGatewayRequest
* A {@link Consumer} that will call methods on {@link DeregisterTransitGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeregisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeregisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture deregisterTransitGateway(
Consumer deregisterTransitGatewayRequest) {
return deregisterTransitGateway(DeregisterTransitGatewayRequest.builder().applyMutation(deregisterTransitGatewayRequest)
.build());
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
* @param describeGlobalNetworksRequest
* @return A Java Future containing the result of the DescribeGlobalNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalNetworks(
DescribeGlobalNetworksRequest describeGlobalNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalNetworksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeGlobalNetworksRequest#builder()}
*
*
* @param describeGlobalNetworksRequest
* A {@link Consumer} that will call methods on {@link DescribeGlobalNetworksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeGlobalNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalNetworks(
Consumer describeGlobalNetworksRequest) {
return describeGlobalNetworks(DescribeGlobalNetworksRequest.builder().applyMutation(describeGlobalNetworksRequest)
.build());
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a variant of
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation.
*
*
* @param describeGlobalNetworksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default DescribeGlobalNetworksPublisher describeGlobalNetworksPaginator(
DescribeGlobalNetworksRequest describeGlobalNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a variant of
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalNetworksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeGlobalNetworksRequest#builder()}
*
*
* @param describeGlobalNetworksRequest
* A {@link Consumer} that will call methods on {@link DescribeGlobalNetworksRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default DescribeGlobalNetworksPublisher describeGlobalNetworksPaginator(
Consumer describeGlobalNetworksRequest) {
return describeGlobalNetworksPaginator(DescribeGlobalNetworksRequest.builder()
.applyMutation(describeGlobalNetworksRequest).build());
}
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
* @param disassociateCustomerGatewayRequest
* @return A Java Future containing the result of the DisassociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture disassociateCustomerGateway(
DisassociateCustomerGatewayRequest disassociateCustomerGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateCustomerGatewayRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateCustomerGatewayRequest#builder()}
*
*
* @param disassociateCustomerGatewayRequest
* A {@link Consumer} that will call methods on {@link DisassociateCustomerGatewayRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DisassociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture disassociateCustomerGateway(
Consumer disassociateCustomerGatewayRequest) {
return disassociateCustomerGateway(DisassociateCustomerGatewayRequest.builder()
.applyMutation(disassociateCustomerGatewayRequest).build());
}
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
* @param disassociateLinkRequest
* @return A Java Future containing the result of the DisassociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateLink
* @see AWS API Documentation
*/
default CompletableFuture disassociateLink(DisassociateLinkRequest disassociateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateLinkRequest.Builder} avoiding the need
* to create one manually via {@link DisassociateLinkRequest#builder()}
*
*
* @param disassociateLinkRequest
* A {@link Consumer} that will call methods on {@link DisassociateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisassociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateLink
* @see AWS API Documentation
*/
default CompletableFuture disassociateLink(
Consumer disassociateLinkRequest) {
return disassociateLink(DisassociateLinkRequest.builder().applyMutation(disassociateLinkRequest).build());
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return A Java Future containing the result of the GetCustomerGatewayAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default CompletableFuture getCustomerGatewayAssociations(
GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a convenience which creates an instance of the {@link GetCustomerGatewayAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetCustomerGatewayAssociationsRequest#builder()}
*
*
* @param getCustomerGatewayAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetCustomerGatewayAssociationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetCustomerGatewayAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default CompletableFuture getCustomerGatewayAssociations(
Consumer getCustomerGatewayAssociationsRequest) {
return getCustomerGatewayAssociations(GetCustomerGatewayAssociationsRequest.builder()
.applyMutation(getCustomerGatewayAssociationsRequest).build());
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a variant of
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default GetCustomerGatewayAssociationsPublisher getCustomerGatewayAssociationsPaginator(
GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a variant of
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetCustomerGatewayAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetCustomerGatewayAssociationsRequest#builder()}
*
*
* @param getCustomerGatewayAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetCustomerGatewayAssociationsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default GetCustomerGatewayAssociationsPublisher getCustomerGatewayAssociationsPaginator(
Consumer getCustomerGatewayAssociationsRequest) {
return getCustomerGatewayAssociationsPaginator(GetCustomerGatewayAssociationsRequest.builder()
.applyMutation(getCustomerGatewayAssociationsRequest).build());
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
* @param getDevicesRequest
* @return A Java Future containing the result of the GetDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default CompletableFuture getDevices(GetDevicesRequest getDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetDevicesRequest.Builder} avoiding the need to
* create one manually via {@link GetDevicesRequest#builder()}
*
*
* @param getDevicesRequest
* A {@link Consumer} that will call methods on {@link GetDevicesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default CompletableFuture getDevices(Consumer getDevicesRequest) {
return getDevices(GetDevicesRequest.builder().applyMutation(getDevicesRequest).build());
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a variant of {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)} operation.
*
*
* @param getDevicesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default GetDevicesPublisher getDevicesPaginator(GetDevicesRequest getDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a variant of {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetDevicesRequest.Builder} avoiding the need to
* create one manually via {@link GetDevicesRequest#builder()}
*
*
* @param getDevicesRequest
* A {@link Consumer} that will call methods on {@link GetDevicesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default GetDevicesPublisher getDevicesPaginator(Consumer getDevicesRequest) {
return getDevicesPaginator(GetDevicesRequest.builder().applyMutation(getDevicesRequest).build());
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
* @param getLinkAssociationsRequest
* @return A Java Future containing the result of the GetLinkAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default CompletableFuture getLinkAssociations(
GetLinkAssociationsRequest getLinkAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a convenience which creates an instance of the {@link GetLinkAssociationsRequest.Builder} avoiding the
* need to create one manually via {@link GetLinkAssociationsRequest#builder()}
*
*
* @param getLinkAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetLinkAssociationsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLinkAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default CompletableFuture getLinkAssociations(
Consumer getLinkAssociationsRequest) {
return getLinkAssociations(GetLinkAssociationsRequest.builder().applyMutation(getLinkAssociationsRequest).build());
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a variant of
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation.
*
*
* @param getLinkAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default GetLinkAssociationsPublisher getLinkAssociationsPaginator(GetLinkAssociationsRequest getLinkAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a variant of
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetLinkAssociationsRequest.Builder} avoiding the
* need to create one manually via {@link GetLinkAssociationsRequest#builder()}
*
*
* @param getLinkAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetLinkAssociationsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default GetLinkAssociationsPublisher getLinkAssociationsPaginator(
Consumer getLinkAssociationsRequest) {
return getLinkAssociationsPaginator(GetLinkAssociationsRequest.builder().applyMutation(getLinkAssociationsRequest)
.build());
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
* @param getLinksRequest
* @return A Java Future containing the result of the GetLinks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default CompletableFuture getLinks(GetLinksRequest getLinksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a convenience which creates an instance of the {@link GetLinksRequest.Builder} avoiding the need to
* create one manually via {@link GetLinksRequest#builder()}
*
*
* @param getLinksRequest
* A {@link Consumer} that will call methods on {@link GetLinksRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetLinks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default CompletableFuture getLinks(Consumer getLinksRequest) {
return getLinks(GetLinksRequest.builder().applyMutation(getLinksRequest).build());
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a variant of {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)} operation.
*
*
* @param getLinksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default GetLinksPublisher getLinksPaginator(GetLinksRequest getLinksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a variant of {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetLinksRequest.Builder} avoiding the need to
* create one manually via {@link GetLinksRequest#builder()}
*
*
* @param getLinksRequest
* A {@link Consumer} that will call methods on {@link GetLinksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default GetLinksPublisher getLinksPaginator(Consumer getLinksRequest) {
return getLinksPaginator(GetLinksRequest.builder().applyMutation(getLinksRequest).build());
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
* @param getSitesRequest
* @return A Java Future containing the result of the GetSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default CompletableFuture getSites(GetSitesRequest getSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetSitesRequest.Builder} avoiding the need to
* create one manually via {@link GetSitesRequest#builder()}
*
*
* @param getSitesRequest
* A {@link Consumer} that will call methods on {@link GetSitesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default CompletableFuture getSites(Consumer getSitesRequest) {
return getSites(GetSitesRequest.builder().applyMutation(getSitesRequest).build());
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a variant of {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)} operation.
*
*
* @param getSitesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default GetSitesPublisher getSitesPaginator(GetSitesRequest getSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a variant of {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetSitesRequest.Builder} avoiding the need to
* create one manually via {@link GetSitesRequest#builder()}
*
*
* @param getSitesRequest
* A {@link Consumer} that will call methods on {@link GetSitesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default GetSitesPublisher getSitesPaginator(Consumer getSitesRequest) {
return getSitesPaginator(GetSitesRequest.builder().applyMutation(getSitesRequest).build());
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return A Java Future containing the result of the GetTransitGatewayRegistrations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayRegistrations(
GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetTransitGatewayRegistrationsRequest.Builder}
* avoiding the need to create one manually via {@link GetTransitGatewayRegistrationsRequest#builder()}
*
*
* @param getTransitGatewayRegistrationsRequest
* A {@link Consumer} that will call methods on {@link GetTransitGatewayRegistrationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetTransitGatewayRegistrations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayRegistrations(
Consumer getTransitGatewayRegistrationsRequest) {
return getTransitGatewayRegistrations(GetTransitGatewayRegistrationsRequest.builder()
.applyMutation(getTransitGatewayRegistrationsRequest).build());
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default GetTransitGatewayRegistrationsPublisher getTransitGatewayRegistrationsPaginator(
GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetTransitGatewayRegistrationsRequest.Builder}
* avoiding the need to create one manually via {@link GetTransitGatewayRegistrationsRequest#builder()}
*
*
* @param getTransitGatewayRegistrationsRequest
* A {@link Consumer} that will call methods on {@link GetTransitGatewayRegistrationsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default GetTransitGatewayRegistrationsPublisher getTransitGatewayRegistrationsPaginator(
Consumer getTransitGatewayRegistrationsRequest) {
return getTransitGatewayRegistrationsPaginator(GetTransitGatewayRegistrationsRequest.builder()
.applyMutation(getTransitGatewayRegistrationsRequest).build());
}
/**
*
* Lists the tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Registers a transit gateway in your global network. The transit gateway can be in any AWS Region, but it must be
* owned by the same AWS account that owns the global network. You cannot register a transit gateway in more than
* one global network.
*
*
* @param registerTransitGatewayRequest
* @return A Java Future containing the result of the RegisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RegisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture registerTransitGateway(
RegisterTransitGatewayRequest registerTransitGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Registers a transit gateway in your global network. The transit gateway can be in any AWS Region, but it must be
* owned by the same AWS account that owns the global network. You cannot register a transit gateway in more than
* one global network.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterTransitGatewayRequest.Builder} avoiding the
* need to create one manually via {@link RegisterTransitGatewayRequest#builder()}
*
*
* @param registerTransitGatewayRequest
* A {@link Consumer} that will call methods on {@link RegisterTransitGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RegisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RegisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture registerTransitGateway(
Consumer registerTransitGatewayRequest) {
return registerTransitGateway(RegisterTransitGatewayRequest.builder().applyMutation(registerTransitGatewayRequest)
.build());
}
/**
*
* Tags a specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Tags a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateDeviceRequest
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture updateDevice(UpdateDeviceRequest updateDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDeviceRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDeviceRequest#builder()}
*
*
* @param updateDeviceRequest
* A {@link Consumer} that will call methods on {@link UpdateDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture updateDevice(Consumer updateDeviceRequest) {
return updateDevice(UpdateDeviceRequest.builder().applyMutation(updateDeviceRequest).build());
}
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
* @param updateGlobalNetworkRequest
* @return A Java Future containing the result of the UpdateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateGlobalNetwork(
UpdateGlobalNetworkRequest updateGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link UpdateGlobalNetworkRequest#builder()}
*
*
* @param updateGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link UpdateGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateGlobalNetwork(
Consumer updateGlobalNetworkRequest) {
return updateGlobalNetwork(UpdateGlobalNetworkRequest.builder().applyMutation(updateGlobalNetworkRequest).build());
}
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateLinkRequest
* @return A Java Future containing the result of the UpdateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateLink
* @see AWS API
* Documentation
*/
default CompletableFuture updateLink(UpdateLinkRequest updateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLinkRequest.Builder} avoiding the need to
* create one manually via {@link UpdateLinkRequest#builder()}
*
*
* @param updateLinkRequest
* A {@link Consumer} that will call methods on {@link UpdateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateLink
* @see AWS API
* Documentation
*/
default CompletableFuture updateLink(Consumer updateLinkRequest) {
return updateLink(UpdateLinkRequest.builder().applyMutation(updateLinkRequest).build());
}
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateSiteRequest
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(UpdateSiteRequest updateSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSiteRequest.Builder} avoiding the need to
* create one manually via {@link UpdateSiteRequest#builder()}
*
*
* @param updateSiteRequest
* A {@link Consumer} that will call methods on {@link UpdateSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(Consumer updateSiteRequest) {
return updateSite(UpdateSiteRequest.builder().applyMutation(updateSiteRequest).build());
}
}