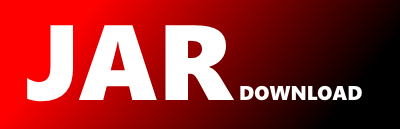
software.amazon.awssdk.services.networkmanager.NetworkManagerAsyncClient Maven / Gradle / Ivy
Show all versions of networkmanager Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.networkmanager;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.networkmanager.model.AcceptAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.AcceptAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.AssociateConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.AssociateCustomerGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateCustomerGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.AssociateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.AssociateTransitGatewayConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.AssociateTransitGatewayConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectionRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateConnectionResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateCoreNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateCoreNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteToSiteVpnAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateSiteToSiteVpnAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.CreateVpcAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.CreateVpcAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteConnectionRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteConnectionResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteCoreNetworkPolicyVersionRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteCoreNetworkPolicyVersionResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteCoreNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteCoreNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteResourcePolicyRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteResourcePolicyResponse;
import software.amazon.awssdk.services.networkmanager.model.DeleteSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.DeleteSiteResponse;
import software.amazon.awssdk.services.networkmanager.model.DeregisterTransitGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.DeregisterTransitGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest;
import software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateCustomerGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateCustomerGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.DisassociateTransitGatewayConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.DisassociateTransitGatewayConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.ExecuteCoreNetworkChangeSetRequest;
import software.amazon.awssdk.services.networkmanager.model.ExecuteCoreNetworkChangeSetResponse;
import software.amazon.awssdk.services.networkmanager.model.GetConnectAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.GetConnectAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetConnectPeerRequest;
import software.amazon.awssdk.services.networkmanager.model.GetConnectPeerResponse;
import software.amazon.awssdk.services.networkmanager.model.GetConnectionsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetConnectionsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetRequest;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetResponse;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkPolicyRequest;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkPolicyResponse;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetLinksRequest;
import software.amazon.awssdk.services.networkmanager.model.GetLinksResponse;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkRoutesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkRoutesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryRequest;
import software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryResponse;
import software.amazon.awssdk.services.networkmanager.model.GetResourcePolicyRequest;
import software.amazon.awssdk.services.networkmanager.model.GetResourcePolicyResponse;
import software.amazon.awssdk.services.networkmanager.model.GetRouteAnalysisRequest;
import software.amazon.awssdk.services.networkmanager.model.GetRouteAnalysisResponse;
import software.amazon.awssdk.services.networkmanager.model.GetSiteToSiteVpnAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.GetSiteToSiteVpnAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.GetSitesRequest;
import software.amazon.awssdk.services.networkmanager.model.GetSitesResponse;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest;
import software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse;
import software.amazon.awssdk.services.networkmanager.model.GetVpcAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.GetVpcAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.ListAttachmentsRequest;
import software.amazon.awssdk.services.networkmanager.model.ListAttachmentsResponse;
import software.amazon.awssdk.services.networkmanager.model.ListConnectPeersRequest;
import software.amazon.awssdk.services.networkmanager.model.ListConnectPeersResponse;
import software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsRequest;
import software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsResponse;
import software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksRequest;
import software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksResponse;
import software.amazon.awssdk.services.networkmanager.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.PutCoreNetworkPolicyRequest;
import software.amazon.awssdk.services.networkmanager.model.PutCoreNetworkPolicyResponse;
import software.amazon.awssdk.services.networkmanager.model.PutResourcePolicyRequest;
import software.amazon.awssdk.services.networkmanager.model.PutResourcePolicyResponse;
import software.amazon.awssdk.services.networkmanager.model.RegisterTransitGatewayRequest;
import software.amazon.awssdk.services.networkmanager.model.RegisterTransitGatewayResponse;
import software.amazon.awssdk.services.networkmanager.model.RejectAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.RejectAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.model.RestoreCoreNetworkPolicyVersionRequest;
import software.amazon.awssdk.services.networkmanager.model.RestoreCoreNetworkPolicyVersionResponse;
import software.amazon.awssdk.services.networkmanager.model.StartRouteAnalysisRequest;
import software.amazon.awssdk.services.networkmanager.model.StartRouteAnalysisResponse;
import software.amazon.awssdk.services.networkmanager.model.TagResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.TagResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.UntagResourceRequest;
import software.amazon.awssdk.services.networkmanager.model.UntagResourceResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateConnectionRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateConnectionResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateCoreNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateCoreNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateDeviceRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateDeviceResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateGlobalNetworkRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateGlobalNetworkResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateLinkRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateLinkResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateNetworkResourceMetadataRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateNetworkResourceMetadataResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateSiteRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateSiteResponse;
import software.amazon.awssdk.services.networkmanager.model.UpdateVpcAttachmentRequest;
import software.amazon.awssdk.services.networkmanager.model.UpdateVpcAttachmentResponse;
import software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetConnectPeerAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetConnectionsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetCoreNetworkChangeSetPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceCountsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceRelationshipsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourcesPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetNetworkTelemetryPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayConnectPeerAssociationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.ListAttachmentsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.ListConnectPeersPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworkPolicyVersionsPublisher;
import software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworksPublisher;
/**
* Service client for accessing NetworkManager asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* Transit Gateway Network Manager (Network Manager) enables you to create a global network, in which you can monitor
* your Amazon Web Services and on-premises networks that are built around transit gateways.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface NetworkManagerAsyncClient extends SdkClient {
String SERVICE_NAME = "networkmanager";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "networkmanager";
/**
* Create a {@link NetworkManagerAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static NetworkManagerAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link NetworkManagerAsyncClient}.
*/
static NetworkManagerAsyncClientBuilder builder() {
return new DefaultNetworkManagerAsyncClientBuilder();
}
/**
*
* Accepts a core network attachment request.
*
*
* Once the attachment request is accepted by a core network owner, the attachment is created and connected to a
* core network.
*
*
* @param acceptAttachmentRequest
* @return A Java Future containing the result of the AcceptAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AcceptAttachment
* @see AWS API Documentation
*/
default CompletableFuture acceptAttachment(AcceptAttachmentRequest acceptAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Accepts a core network attachment request.
*
*
* Once the attachment request is accepted by a core network owner, the attachment is created and connected to a
* core network.
*
*
*
* This is a convenience which creates an instance of the {@link AcceptAttachmentRequest.Builder} avoiding the need
* to create one manually via {@link AcceptAttachmentRequest#builder()}
*
*
* @param acceptAttachmentRequest
* A {@link Consumer} that will call methods on {@link AcceptAttachmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the AcceptAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AcceptAttachment
* @see AWS API Documentation
*/
default CompletableFuture acceptAttachment(
Consumer acceptAttachmentRequest) {
return acceptAttachment(AcceptAttachmentRequest.builder().applyMutation(acceptAttachmentRequest).build());
}
/**
*
* Associates a core network Connect peer with a device and optionally, with a link.
*
*
* If you specify a link, it must be associated with the specified device. You can only associate core network
* Connect peers that have been created on a core network Connect attachment on a core network.
*
*
* @param associateConnectPeerRequest
* @return A Java Future containing the result of the AssociateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture associateConnectPeer(
AssociateConnectPeerRequest associateConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a core network Connect peer with a device and optionally, with a link.
*
*
* If you specify a link, it must be associated with the specified device. You can only associate core network
* Connect peers that have been created on a core network Connect attachment on a core network.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateConnectPeerRequest.Builder} avoiding the
* need to create one manually via {@link AssociateConnectPeerRequest#builder()}
*
*
* @param associateConnectPeerRequest
* A {@link Consumer} that will call methods on {@link AssociateConnectPeerRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture associateConnectPeer(
Consumer associateConnectPeerRequest) {
return associateConnectPeer(AssociateConnectPeerRequest.builder().applyMutation(associateConnectPeerRequest).build());
}
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway. The transit
* gateway must be registered in your global network. When you register a transit gateway, customer gateways that
* are connected to the transit gateway are automatically included in the global network. To list customer gateways
* that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
* @param associateCustomerGatewayRequest
* @return A Java Future containing the result of the AssociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture associateCustomerGateway(
AssociateCustomerGatewayRequest associateCustomerGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a customer gateway with a device and optionally, with a link. If you specify a link, it must be
* associated with the specified device.
*
*
* You can only associate customer gateways that are connected to a VPN attachment on a transit gateway. The transit
* gateway must be registered in your global network. When you register a transit gateway, customer gateways that
* are connected to the transit gateway are automatically included in the global network. To list customer gateways
* that are connected to a transit gateway, use the DescribeVpnConnections EC2 API and filter by transit-gateway-id
.
*
*
* You cannot associate a customer gateway with more than one device and link.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateCustomerGatewayRequest.Builder} avoiding
* the need to create one manually via {@link AssociateCustomerGatewayRequest#builder()}
*
*
* @param associateCustomerGatewayRequest
* A {@link Consumer} that will call methods on {@link AssociateCustomerGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the AssociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture associateCustomerGateway(
Consumer associateCustomerGatewayRequest) {
return associateCustomerGateway(AssociateCustomerGatewayRequest.builder().applyMutation(associateCustomerGatewayRequest)
.build());
}
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
* @param associateLinkRequest
* @return A Java Future containing the result of the AssociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateLink
* @see AWS
* API Documentation
*/
default CompletableFuture associateLink(AssociateLinkRequest associateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a link to a device. A device can be associated to multiple links and a link can be associated to
* multiple devices. The device and link must be in the same global network and the same site.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateLinkRequest.Builder} avoiding the need to
* create one manually via {@link AssociateLinkRequest#builder()}
*
*
* @param associateLinkRequest
* A {@link Consumer} that will call methods on {@link AssociateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the AssociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateLink
* @see AWS
* API Documentation
*/
default CompletableFuture associateLink(Consumer associateLinkRequest) {
return associateLink(AssociateLinkRequest.builder().applyMutation(associateLinkRequest).build());
}
/**
*
* Associates a transit gateway Connect peer with a device, and optionally, with a link. If you specify a link, it
* must be associated with the specified device.
*
*
* You can only associate transit gateway Connect peers that have been created on a transit gateway that's
* registered in your global network.
*
*
* You cannot associate a transit gateway Connect peer with more than one device and link.
*
*
* @param associateTransitGatewayConnectPeerRequest
* @return A Java Future containing the result of the AssociateTransitGatewayConnectPeer operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture associateTransitGatewayConnectPeer(
AssociateTransitGatewayConnectPeerRequest associateTransitGatewayConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Associates a transit gateway Connect peer with a device, and optionally, with a link. If you specify a link, it
* must be associated with the specified device.
*
*
* You can only associate transit gateway Connect peers that have been created on a transit gateway that's
* registered in your global network.
*
*
* You cannot associate a transit gateway Connect peer with more than one device and link.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateTransitGatewayConnectPeerRequest.Builder}
* avoiding the need to create one manually via {@link AssociateTransitGatewayConnectPeerRequest#builder()}
*
*
* @param associateTransitGatewayConnectPeerRequest
* A {@link Consumer} that will call methods on {@link AssociateTransitGatewayConnectPeerRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the AssociateTransitGatewayConnectPeer operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.AssociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture associateTransitGatewayConnectPeer(
Consumer associateTransitGatewayConnectPeerRequest) {
return associateTransitGatewayConnectPeer(AssociateTransitGatewayConnectPeerRequest.builder()
.applyMutation(associateTransitGatewayConnectPeerRequest).build());
}
/**
*
* Creates a core network Connect attachment from a specified core network attachment.
*
*
* A core network Connect attachment is a GRE-based tunnel attachment that you can use to establish a connection
* between a core network and an appliance. A core network Connect attachment uses an existing VPC attachment as the
* underlying transport mechanism.
*
*
* @param createConnectAttachmentRequest
* @return A Java Future containing the result of the CreateConnectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnectAttachment
* @see AWS API Documentation
*/
default CompletableFuture createConnectAttachment(
CreateConnectAttachmentRequest createConnectAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a core network Connect attachment from a specified core network attachment.
*
*
* A core network Connect attachment is a GRE-based tunnel attachment that you can use to establish a connection
* between a core network and an appliance. A core network Connect attachment uses an existing VPC attachment as the
* underlying transport mechanism.
*
*
*
* This is a convenience which creates an instance of the {@link CreateConnectAttachmentRequest.Builder} avoiding
* the need to create one manually via {@link CreateConnectAttachmentRequest#builder()}
*
*
* @param createConnectAttachmentRequest
* A {@link Consumer} that will call methods on {@link CreateConnectAttachmentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateConnectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnectAttachment
* @see AWS API Documentation
*/
default CompletableFuture createConnectAttachment(
Consumer createConnectAttachmentRequest) {
return createConnectAttachment(CreateConnectAttachmentRequest.builder().applyMutation(createConnectAttachmentRequest)
.build());
}
/**
*
* Creates a core network connect peer for a specified core network connect attachment between a core network and an
* appliance. The peer address and transit gateway address must be the same IP address family (IPv4 or IPv6).
*
*
* @param createConnectPeerRequest
* @return A Java Future containing the result of the CreateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture createConnectPeer(CreateConnectPeerRequest createConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a core network connect peer for a specified core network connect attachment between a core network and an
* appliance. The peer address and transit gateway address must be the same IP address family (IPv4 or IPv6).
*
*
*
* This is a convenience which creates an instance of the {@link CreateConnectPeerRequest.Builder} avoiding the need
* to create one manually via {@link CreateConnectPeerRequest#builder()}
*
*
* @param createConnectPeerRequest
* A {@link Consumer} that will call methods on {@link CreateConnectPeerRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture createConnectPeer(
Consumer createConnectPeerRequest) {
return createConnectPeer(CreateConnectPeerRequest.builder().applyMutation(createConnectPeerRequest).build());
}
/**
*
* Creates a connection between two devices. The devices can be a physical or virtual appliance that connects to a
* third-party appliance in a VPC, or a physical appliance that connects to another physical appliance in an
* on-premises network.
*
*
* @param createConnectionRequest
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnection
* @see AWS API Documentation
*/
default CompletableFuture createConnection(CreateConnectionRequest createConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a connection between two devices. The devices can be a physical or virtual appliance that connects to a
* third-party appliance in a VPC, or a physical appliance that connects to another physical appliance in an
* on-premises network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateConnectionRequest.Builder} avoiding the need
* to create one manually via {@link CreateConnectionRequest#builder()}
*
*
* @param createConnectionRequest
* A {@link Consumer} that will call methods on {@link CreateConnectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateConnection
* @see AWS API Documentation
*/
default CompletableFuture createConnection(
Consumer createConnectionRequest) {
return createConnection(CreateConnectionRequest.builder().applyMutation(createConnectionRequest).build());
}
/**
*
* Creates a core network as part of your global network, and optionally, with a core network policy.
*
*
* @param createCoreNetworkRequest
* @return A Java Future containing the result of the CreateCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CoreNetworkPolicyException Describes a core network policy exception.
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture createCoreNetwork(CreateCoreNetworkRequest createCoreNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a core network as part of your global network, and optionally, with a core network policy.
*
*
*
* This is a convenience which creates an instance of the {@link CreateCoreNetworkRequest.Builder} avoiding the need
* to create one manually via {@link CreateCoreNetworkRequest#builder()}
*
*
* @param createCoreNetworkRequest
* A {@link Consumer} that will call methods on {@link CreateCoreNetworkRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CoreNetworkPolicyException Describes a core network policy exception.
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture createCoreNetwork(
Consumer createCoreNetworkRequest) {
return createCoreNetwork(CreateCoreNetworkRequest.builder().applyMutation(createCoreNetworkRequest).build());
}
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
* @param createDeviceRequest
* @return A Java Future containing the result of the CreateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture createDevice(CreateDeviceRequest createDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new device in a global network. If you specify both a site ID and a location, the location of the site
* is used for visualization in the Network Manager console.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDeviceRequest.Builder} avoiding the need to
* create one manually via {@link CreateDeviceRequest#builder()}
*
*
* @param createDeviceRequest
* A {@link Consumer} that will call methods on {@link CreateDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture createDevice(Consumer createDeviceRequest) {
return createDevice(CreateDeviceRequest.builder().applyMutation(createDeviceRequest).build());
}
/**
*
* Creates a new, empty global network.
*
*
* @param createGlobalNetworkRequest
* @return A Java Future containing the result of the CreateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture createGlobalNetwork(
CreateGlobalNetworkRequest createGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new, empty global network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link CreateGlobalNetworkRequest#builder()}
*
*
* @param createGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link CreateGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture createGlobalNetwork(
Consumer createGlobalNetworkRequest) {
return createGlobalNetwork(CreateGlobalNetworkRequest.builder().applyMutation(createGlobalNetworkRequest).build());
}
/**
*
* Creates a new link for a specified site.
*
*
* @param createLinkRequest
* @return A Java Future containing the result of the CreateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateLink
* @see AWS API
* Documentation
*/
default CompletableFuture createLink(CreateLinkRequest createLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new link for a specified site.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLinkRequest.Builder} avoiding the need to
* create one manually via {@link CreateLinkRequest#builder()}
*
*
* @param createLinkRequest
* A {@link Consumer} that will call methods on {@link CreateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateLink
* @see AWS API
* Documentation
*/
default CompletableFuture createLink(Consumer createLinkRequest) {
return createLink(CreateLinkRequest.builder().applyMutation(createLinkRequest).build());
}
/**
*
* Creates a new site in a global network.
*
*
* @param createSiteRequest
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(CreateSiteRequest createSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new site in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSiteRequest.Builder} avoiding the need to
* create one manually via {@link CreateSiteRequest#builder()}
*
*
* @param createSiteRequest
* A {@link Consumer} that will call methods on {@link CreateSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(Consumer createSiteRequest) {
return createSite(CreateSiteRequest.builder().applyMutation(createSiteRequest).build());
}
/**
*
* Creates a site-to-site VPN attachment on an edge location of a core network.
*
*
* @param createSiteToSiteVpnAttachmentRequest
* @return A Java Future containing the result of the CreateSiteToSiteVpnAttachment operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
default CompletableFuture createSiteToSiteVpnAttachment(
CreateSiteToSiteVpnAttachmentRequest createSiteToSiteVpnAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a site-to-site VPN attachment on an edge location of a core network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSiteToSiteVpnAttachmentRequest.Builder}
* avoiding the need to create one manually via {@link CreateSiteToSiteVpnAttachmentRequest#builder()}
*
*
* @param createSiteToSiteVpnAttachmentRequest
* A {@link Consumer} that will call methods on {@link CreateSiteToSiteVpnAttachmentRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateSiteToSiteVpnAttachment operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
default CompletableFuture createSiteToSiteVpnAttachment(
Consumer createSiteToSiteVpnAttachmentRequest) {
return createSiteToSiteVpnAttachment(CreateSiteToSiteVpnAttachmentRequest.builder()
.applyMutation(createSiteToSiteVpnAttachmentRequest).build());
}
/**
*
* Creates a VPC attachment on an edge location of a core network.
*
*
* @param createVpcAttachmentRequest
* @return A Java Future containing the result of the CreateVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture createVpcAttachment(
CreateVpcAttachmentRequest createVpcAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a VPC attachment on an edge location of a core network.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVpcAttachmentRequest.Builder} avoiding the
* need to create one manually via {@link CreateVpcAttachmentRequest#builder()}
*
*
* @param createVpcAttachmentRequest
* A {@link Consumer} that will call methods on {@link CreateVpcAttachmentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.CreateVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture createVpcAttachment(
Consumer createVpcAttachmentRequest) {
return createVpcAttachment(CreateVpcAttachmentRequest.builder().applyMutation(createVpcAttachmentRequest).build());
}
/**
*
* Deletes an attachment. Supports all attachment types.
*
*
* @param deleteAttachmentRequest
* @return A Java Future containing the result of the DeleteAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteAttachment
* @see AWS API Documentation
*/
default CompletableFuture deleteAttachment(DeleteAttachmentRequest deleteAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an attachment. Supports all attachment types.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAttachmentRequest.Builder} avoiding the need
* to create one manually via {@link DeleteAttachmentRequest#builder()}
*
*
* @param deleteAttachmentRequest
* A {@link Consumer} that will call methods on {@link DeleteAttachmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteAttachment
* @see AWS API Documentation
*/
default CompletableFuture deleteAttachment(
Consumer deleteAttachmentRequest) {
return deleteAttachment(DeleteAttachmentRequest.builder().applyMutation(deleteAttachmentRequest).build());
}
/**
*
* Deletes a Connect peer.
*
*
* @param deleteConnectPeerRequest
* @return A Java Future containing the result of the DeleteConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture deleteConnectPeer(DeleteConnectPeerRequest deleteConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Connect peer.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConnectPeerRequest.Builder} avoiding the need
* to create one manually via {@link DeleteConnectPeerRequest#builder()}
*
*
* @param deleteConnectPeerRequest
* A {@link Consumer} that will call methods on {@link DeleteConnectPeerRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture deleteConnectPeer(
Consumer deleteConnectPeerRequest) {
return deleteConnectPeer(DeleteConnectPeerRequest.builder().applyMutation(deleteConnectPeerRequest).build());
}
/**
*
* Deletes the specified connection in your global network.
*
*
* @param deleteConnectionRequest
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteConnection
* @see AWS API Documentation
*/
default CompletableFuture deleteConnection(DeleteConnectionRequest deleteConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified connection in your global network.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteConnectionRequest.Builder} avoiding the need
* to create one manually via {@link DeleteConnectionRequest#builder()}
*
*
* @param deleteConnectionRequest
* A {@link Consumer} that will call methods on {@link DeleteConnectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteConnection
* @see AWS API Documentation
*/
default CompletableFuture deleteConnection(
Consumer deleteConnectionRequest) {
return deleteConnection(DeleteConnectionRequest.builder().applyMutation(deleteConnectionRequest).build());
}
/**
*
* Deletes a core network along with all core network policies. This can only be done if there are no attachments on
* a core network.
*
*
* @param deleteCoreNetworkRequest
* @return A Java Future containing the result of the DeleteCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteCoreNetwork(DeleteCoreNetworkRequest deleteCoreNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a core network along with all core network policies. This can only be done if there are no attachments on
* a core network.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCoreNetworkRequest.Builder} avoiding the need
* to create one manually via {@link DeleteCoreNetworkRequest#builder()}
*
*
* @param deleteCoreNetworkRequest
* A {@link Consumer} that will call methods on {@link DeleteCoreNetworkRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteCoreNetwork(
Consumer deleteCoreNetworkRequest) {
return deleteCoreNetwork(DeleteCoreNetworkRequest.builder().applyMutation(deleteCoreNetworkRequest).build());
}
/**
*
* Deletes a policy version from a core network. You can't delete the current LIVE policy.
*
*
* @param deleteCoreNetworkPolicyVersionRequest
* @return A Java Future containing the result of the DeleteCoreNetworkPolicyVersion operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
default CompletableFuture deleteCoreNetworkPolicyVersion(
DeleteCoreNetworkPolicyVersionRequest deleteCoreNetworkPolicyVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a policy version from a core network. You can't delete the current LIVE policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteCoreNetworkPolicyVersionRequest.Builder}
* avoiding the need to create one manually via {@link DeleteCoreNetworkPolicyVersionRequest#builder()}
*
*
* @param deleteCoreNetworkPolicyVersionRequest
* A {@link Consumer} that will call methods on {@link DeleteCoreNetworkPolicyVersionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteCoreNetworkPolicyVersion operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
default CompletableFuture deleteCoreNetworkPolicyVersion(
Consumer deleteCoreNetworkPolicyVersionRequest) {
return deleteCoreNetworkPolicyVersion(DeleteCoreNetworkPolicyVersionRequest.builder()
.applyMutation(deleteCoreNetworkPolicyVersionRequest).build());
}
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
* @param deleteDeviceRequest
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(DeleteDeviceRequest deleteDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing device. You must first disassociate the device from any links and customer gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDeviceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDeviceRequest#builder()}
*
*
* @param deleteDeviceRequest
* A {@link Consumer} that will call methods on {@link DeleteDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteDevice
* @see AWS
* API Documentation
*/
default CompletableFuture deleteDevice(Consumer deleteDeviceRequest) {
return deleteDevice(DeleteDeviceRequest.builder().applyMutation(deleteDeviceRequest).build());
}
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites)
* and deregister all transit gateways.
*
*
* @param deleteGlobalNetworkRequest
* @return A Java Future containing the result of the DeleteGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalNetwork(
DeleteGlobalNetworkRequest deleteGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing global network. You must first delete all global network objects (devices, links, and sites)
* and deregister all transit gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link DeleteGlobalNetworkRequest#builder()}
*
*
* @param deleteGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link DeleteGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture deleteGlobalNetwork(
Consumer deleteGlobalNetworkRequest) {
return deleteGlobalNetwork(DeleteGlobalNetworkRequest.builder().applyMutation(deleteGlobalNetworkRequest).build());
}
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
* @param deleteLinkRequest
* @return A Java Future containing the result of the DeleteLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteLink
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLink(DeleteLinkRequest deleteLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing link. You must first disassociate the link from any devices and customer gateways.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLinkRequest.Builder} avoiding the need to
* create one manually via {@link DeleteLinkRequest#builder()}
*
*
* @param deleteLinkRequest
* A {@link Consumer} that will call methods on {@link DeleteLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteLink
* @see AWS API
* Documentation
*/
default CompletableFuture deleteLink(Consumer deleteLinkRequest) {
return deleteLink(DeleteLinkRequest.builder().applyMutation(deleteLinkRequest).build());
}
/**
*
* Deletes a resource policy for the specified resource. This revokes the access of the principals specified in the
* resource policy.
*
*
* @param deleteResourcePolicyRequest
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteResourcePolicy(
DeleteResourcePolicyRequest deleteResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a resource policy for the specified resource. This revokes the access of the principals specified in the
* resource policy.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteResourcePolicyRequest.Builder} avoiding the
* need to create one manually via {@link DeleteResourcePolicyRequest#builder()}
*
*
* @param deleteResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link DeleteResourcePolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture deleteResourcePolicy(
Consumer deleteResourcePolicyRequest) {
return deleteResourcePolicy(DeleteResourcePolicyRequest.builder().applyMutation(deleteResourcePolicyRequest).build());
}
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
* @param deleteSiteRequest
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(DeleteSiteRequest deleteSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an existing site. The site cannot be associated with any device or link.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSiteRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSiteRequest#builder()}
*
*
* @param deleteSiteRequest
* A {@link Consumer} that will call methods on {@link DeleteSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(Consumer deleteSiteRequest) {
return deleteSite(DeleteSiteRequest.builder().applyMutation(deleteSiteRequest).build());
}
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
* @param deregisterTransitGatewayRequest
* @return A Java Future containing the result of the DeregisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeregisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture deregisterTransitGateway(
DeregisterTransitGatewayRequest deregisterTransitGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a transit gateway from your global network. This action does not delete your transit gateway, or
* modify any of its attachments. This action removes any customer gateway associations.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterTransitGatewayRequest.Builder} avoiding
* the need to create one manually via {@link DeregisterTransitGatewayRequest#builder()}
*
*
* @param deregisterTransitGatewayRequest
* A {@link Consumer} that will call methods on {@link DeregisterTransitGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeregisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DeregisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture deregisterTransitGateway(
Consumer deregisterTransitGatewayRequest) {
return deregisterTransitGateway(DeregisterTransitGatewayRequest.builder().applyMutation(deregisterTransitGatewayRequest)
.build());
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
* @param describeGlobalNetworksRequest
* @return A Java Future containing the result of the DescribeGlobalNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalNetworks(
DescribeGlobalNetworksRequest describeGlobalNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalNetworksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeGlobalNetworksRequest#builder()}
*
*
* @param describeGlobalNetworksRequest
* A {@link Consumer} that will call methods on {@link DescribeGlobalNetworksRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DescribeGlobalNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default CompletableFuture describeGlobalNetworks(
Consumer describeGlobalNetworksRequest) {
return describeGlobalNetworks(DescribeGlobalNetworksRequest.builder().applyMutation(describeGlobalNetworksRequest)
.build());
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a variant of
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation.
*
*
* @param describeGlobalNetworksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default DescribeGlobalNetworksPublisher describeGlobalNetworksPaginator(
DescribeGlobalNetworksRequest describeGlobalNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more global networks. By default, all global networks are described. To describe the objects in
* your global network, you must use the appropriate Get*
action. For example, to list the transit
* gateways in your global network, use GetTransitGatewayRegistrations.
*
*
*
* This is a variant of
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.DescribeGlobalNetworksPublisher publisher = client.describeGlobalNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeGlobalNetworks(software.amazon.awssdk.services.networkmanager.model.DescribeGlobalNetworksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeGlobalNetworksRequest.Builder} avoiding the
* need to create one manually via {@link DescribeGlobalNetworksRequest#builder()}
*
*
* @param describeGlobalNetworksRequest
* A {@link Consumer} that will call methods on {@link DescribeGlobalNetworksRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DescribeGlobalNetworks
* @see AWS API Documentation
*/
default DescribeGlobalNetworksPublisher describeGlobalNetworksPaginator(
Consumer describeGlobalNetworksRequest) {
return describeGlobalNetworksPaginator(DescribeGlobalNetworksRequest.builder()
.applyMutation(describeGlobalNetworksRequest).build());
}
/**
*
* Disassociates a core network Connect peer from a device and a link.
*
*
* @param disassociateConnectPeerRequest
* @return A Java Future containing the result of the DisassociateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture disassociateConnectPeer(
DisassociateConnectPeerRequest disassociateConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a core network Connect peer from a device and a link.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateConnectPeerRequest.Builder} avoiding
* the need to create one manually via {@link DisassociateConnectPeerRequest#builder()}
*
*
* @param disassociateConnectPeerRequest
* A {@link Consumer} that will call methods on {@link DisassociateConnectPeerRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DisassociateConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture disassociateConnectPeer(
Consumer disassociateConnectPeerRequest) {
return disassociateConnectPeer(DisassociateConnectPeerRequest.builder().applyMutation(disassociateConnectPeerRequest)
.build());
}
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
* @param disassociateCustomerGatewayRequest
* @return A Java Future containing the result of the DisassociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture disassociateCustomerGateway(
DisassociateCustomerGatewayRequest disassociateCustomerGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a customer gateway from a device and a link.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateCustomerGatewayRequest.Builder}
* avoiding the need to create one manually via {@link DisassociateCustomerGatewayRequest#builder()}
*
*
* @param disassociateCustomerGatewayRequest
* A {@link Consumer} that will call methods on {@link DisassociateCustomerGatewayRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DisassociateCustomerGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateCustomerGateway
* @see AWS API Documentation
*/
default CompletableFuture disassociateCustomerGateway(
Consumer disassociateCustomerGatewayRequest) {
return disassociateCustomerGateway(DisassociateCustomerGatewayRequest.builder()
.applyMutation(disassociateCustomerGatewayRequest).build());
}
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
* @param disassociateLinkRequest
* @return A Java Future containing the result of the DisassociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateLink
* @see AWS API Documentation
*/
default CompletableFuture disassociateLink(DisassociateLinkRequest disassociateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an existing device from a link. You must first disassociate any customer gateways that are
* associated with the link.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateLinkRequest.Builder} avoiding the need
* to create one manually via {@link DisassociateLinkRequest#builder()}
*
*
* @param disassociateLinkRequest
* A {@link Consumer} that will call methods on {@link DisassociateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisassociateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateLink
* @see AWS API Documentation
*/
default CompletableFuture disassociateLink(
Consumer disassociateLinkRequest) {
return disassociateLink(DisassociateLinkRequest.builder().applyMutation(disassociateLinkRequest).build());
}
/**
*
* Disassociates a transit gateway Connect peer from a device and link.
*
*
* @param disassociateTransitGatewayConnectPeerRequest
* @return A Java Future containing the result of the DisassociateTransitGatewayConnectPeer operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture disassociateTransitGatewayConnectPeer(
DisassociateTransitGatewayConnectPeerRequest disassociateTransitGatewayConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates a transit gateway Connect peer from a device and link.
*
*
*
* This is a convenience which creates an instance of the
* {@link DisassociateTransitGatewayConnectPeerRequest.Builder} avoiding the need to create one manually via
* {@link DisassociateTransitGatewayConnectPeerRequest#builder()}
*
*
* @param disassociateTransitGatewayConnectPeerRequest
* A {@link Consumer} that will call methods on {@link DisassociateTransitGatewayConnectPeerRequest.Builder}
* to create a request.
* @return A Java Future containing the result of the DisassociateTransitGatewayConnectPeer operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.DisassociateTransitGatewayConnectPeer
* @see AWS API Documentation
*/
default CompletableFuture disassociateTransitGatewayConnectPeer(
Consumer disassociateTransitGatewayConnectPeerRequest) {
return disassociateTransitGatewayConnectPeer(DisassociateTransitGatewayConnectPeerRequest.builder()
.applyMutation(disassociateTransitGatewayConnectPeerRequest).build());
}
/**
*
* Executes a change set on your core network. Deploys changes globally based on the policy submitted..
*
*
* @param executeCoreNetworkChangeSetRequest
* @return A Java Future containing the result of the ExecuteCoreNetworkChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ExecuteCoreNetworkChangeSet
* @see AWS API Documentation
*/
default CompletableFuture executeCoreNetworkChangeSet(
ExecuteCoreNetworkChangeSetRequest executeCoreNetworkChangeSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Executes a change set on your core network. Deploys changes globally based on the policy submitted..
*
*
*
* This is a convenience which creates an instance of the {@link ExecuteCoreNetworkChangeSetRequest.Builder}
* avoiding the need to create one manually via {@link ExecuteCoreNetworkChangeSetRequest#builder()}
*
*
* @param executeCoreNetworkChangeSetRequest
* A {@link Consumer} that will call methods on {@link ExecuteCoreNetworkChangeSetRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ExecuteCoreNetworkChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ExecuteCoreNetworkChangeSet
* @see AWS API Documentation
*/
default CompletableFuture executeCoreNetworkChangeSet(
Consumer executeCoreNetworkChangeSetRequest) {
return executeCoreNetworkChangeSet(ExecuteCoreNetworkChangeSetRequest.builder()
.applyMutation(executeCoreNetworkChangeSetRequest).build());
}
/**
*
* Returns information about a core network Connect attachment.
*
*
* @param getConnectAttachmentRequest
* @return A Java Future containing the result of the GetConnectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectAttachment
* @see AWS API Documentation
*/
default CompletableFuture getConnectAttachment(
GetConnectAttachmentRequest getConnectAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a core network Connect attachment.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectAttachmentRequest.Builder} avoiding the
* need to create one manually via {@link GetConnectAttachmentRequest#builder()}
*
*
* @param getConnectAttachmentRequest
* A {@link Consumer} that will call methods on {@link GetConnectAttachmentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetConnectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectAttachment
* @see AWS API Documentation
*/
default CompletableFuture getConnectAttachment(
Consumer getConnectAttachmentRequest) {
return getConnectAttachment(GetConnectAttachmentRequest.builder().applyMutation(getConnectAttachmentRequest).build());
}
/**
*
* Returns information about a core network Connect peer.
*
*
* @param getConnectPeerRequest
* @return A Java Future containing the result of the GetConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeer
* @see AWS
* API Documentation
*/
default CompletableFuture getConnectPeer(GetConnectPeerRequest getConnectPeerRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a core network Connect peer.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectPeerRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectPeerRequest#builder()}
*
*
* @param getConnectPeerRequest
* A {@link Consumer} that will call methods on {@link GetConnectPeerRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetConnectPeer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeer
* @see AWS
* API Documentation
*/
default CompletableFuture getConnectPeer(Consumer getConnectPeerRequest) {
return getConnectPeer(GetConnectPeerRequest.builder().applyMutation(getConnectPeerRequest).build());
}
/**
*
* Returns information about a core network Connect peer associations.
*
*
* @param getConnectPeerAssociationsRequest
* @return A Java Future containing the result of the GetConnectPeerAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeerAssociations
* @see AWS API Documentation
*/
default CompletableFuture getConnectPeerAssociations(
GetConnectPeerAssociationsRequest getConnectPeerAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a core network Connect peer associations.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectPeerAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link GetConnectPeerAssociationsRequest#builder()}
*
*
* @param getConnectPeerAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetConnectPeerAssociationsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetConnectPeerAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeerAssociations
* @see AWS API Documentation
*/
default CompletableFuture getConnectPeerAssociations(
Consumer getConnectPeerAssociationsRequest) {
return getConnectPeerAssociations(GetConnectPeerAssociationsRequest.builder()
.applyMutation(getConnectPeerAssociationsRequest).build());
}
/**
*
* Returns information about a core network Connect peer associations.
*
*
*
* This is a variant of
* {@link #getConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectPeerAssociationsPublisher publisher = client.getConnectPeerAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectPeerAssociationsPublisher publisher = client.getConnectPeerAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsRequest)}
* operation.
*
*
* @param getConnectPeerAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeerAssociations
* @see AWS API Documentation
*/
default GetConnectPeerAssociationsPublisher getConnectPeerAssociationsPaginator(
GetConnectPeerAssociationsRequest getConnectPeerAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a core network Connect peer associations.
*
*
*
* This is a variant of
* {@link #getConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectPeerAssociationsPublisher publisher = client.getConnectPeerAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectPeerAssociationsPublisher publisher = client.getConnectPeerAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetConnectPeerAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetConnectPeerAssociationsRequest.Builder} avoiding
* the need to create one manually via {@link GetConnectPeerAssociationsRequest#builder()}
*
*
* @param getConnectPeerAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetConnectPeerAssociationsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnectPeerAssociations
* @see AWS API Documentation
*/
default GetConnectPeerAssociationsPublisher getConnectPeerAssociationsPaginator(
Consumer getConnectPeerAssociationsRequest) {
return getConnectPeerAssociationsPaginator(GetConnectPeerAssociationsRequest.builder()
.applyMutation(getConnectPeerAssociationsRequest).build());
}
/**
*
* Gets information about one or more of your connections in a global network.
*
*
* @param getConnectionsRequest
* @return A Java Future containing the result of the GetConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnections
* @see AWS
* API Documentation
*/
default CompletableFuture getConnections(GetConnectionsRequest getConnectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your connections in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetConnectionsRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectionsRequest#builder()}
*
*
* @param getConnectionsRequest
* A {@link Consumer} that will call methods on {@link GetConnectionsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnections
* @see AWS
* API Documentation
*/
default CompletableFuture getConnections(Consumer getConnectionsRequest) {
return getConnections(GetConnectionsRequest.builder().applyMutation(getConnectionsRequest).build());
}
/**
*
* Gets information about one or more of your connections in a global network.
*
*
*
* This is a variant of
* {@link #getConnections(software.amazon.awssdk.services.networkmanager.model.GetConnectionsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectionsPublisher publisher = client.getConnectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectionsPublisher publisher = client.getConnectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetConnectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnections(software.amazon.awssdk.services.networkmanager.model.GetConnectionsRequest)}
* operation.
*
*
* @param getConnectionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnections
* @see AWS
* API Documentation
*/
default GetConnectionsPublisher getConnectionsPaginator(GetConnectionsRequest getConnectionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your connections in a global network.
*
*
*
* This is a variant of
* {@link #getConnections(software.amazon.awssdk.services.networkmanager.model.GetConnectionsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectionsPublisher publisher = client.getConnectionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetConnectionsPublisher publisher = client.getConnectionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetConnectionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getConnections(software.amazon.awssdk.services.networkmanager.model.GetConnectionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetConnectionsRequest.Builder} avoiding the need to
* create one manually via {@link GetConnectionsRequest#builder()}
*
*
* @param getConnectionsRequest
* A {@link Consumer} that will call methods on {@link GetConnectionsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetConnections
* @see AWS
* API Documentation
*/
default GetConnectionsPublisher getConnectionsPaginator(Consumer getConnectionsRequest) {
return getConnectionsPaginator(GetConnectionsRequest.builder().applyMutation(getConnectionsRequest).build());
}
/**
*
* Returns information about a core network. By default it returns the LIVE policy.
*
*
* @param getCoreNetworkRequest
* @return A Java Future containing the result of the GetCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetwork
* @see AWS
* API Documentation
*/
default CompletableFuture getCoreNetwork(GetCoreNetworkRequest getCoreNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a core network. By default it returns the LIVE policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetCoreNetworkRequest.Builder} avoiding the need to
* create one manually via {@link GetCoreNetworkRequest#builder()}
*
*
* @param getCoreNetworkRequest
* A {@link Consumer} that will call methods on {@link GetCoreNetworkRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetwork
* @see AWS
* API Documentation
*/
default CompletableFuture getCoreNetwork(Consumer getCoreNetworkRequest) {
return getCoreNetwork(GetCoreNetworkRequest.builder().applyMutation(getCoreNetworkRequest).build());
}
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
* @param getCoreNetworkChangeSetRequest
* @return A Java Future containing the result of the GetCoreNetworkChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
default CompletableFuture getCoreNetworkChangeSet(
GetCoreNetworkChangeSetRequest getCoreNetworkChangeSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetCoreNetworkChangeSetRequest.Builder} avoiding
* the need to create one manually via {@link GetCoreNetworkChangeSetRequest#builder()}
*
*
* @param getCoreNetworkChangeSetRequest
* A {@link Consumer} that will call methods on {@link GetCoreNetworkChangeSetRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCoreNetworkChangeSet operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
default CompletableFuture getCoreNetworkChangeSet(
Consumer getCoreNetworkChangeSetRequest) {
return getCoreNetworkChangeSet(GetCoreNetworkChangeSetRequest.builder().applyMutation(getCoreNetworkChangeSetRequest)
.build());
}
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
*
* This is a variant of
* {@link #getCoreNetworkChangeSet(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCoreNetworkChangeSetPublisher publisher = client.getCoreNetworkChangeSetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCoreNetworkChangeSetPublisher publisher = client.getCoreNetworkChangeSetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCoreNetworkChangeSet(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetRequest)}
* operation.
*
*
* @param getCoreNetworkChangeSetRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
default GetCoreNetworkChangeSetPublisher getCoreNetworkChangeSetPaginator(
GetCoreNetworkChangeSetRequest getCoreNetworkChangeSetRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a change set between the LIVE core network policy and a submitted policy.
*
*
*
* This is a variant of
* {@link #getCoreNetworkChangeSet(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCoreNetworkChangeSetPublisher publisher = client.getCoreNetworkChangeSetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCoreNetworkChangeSetPublisher publisher = client.getCoreNetworkChangeSetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCoreNetworkChangeSet(software.amazon.awssdk.services.networkmanager.model.GetCoreNetworkChangeSetRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetCoreNetworkChangeSetRequest.Builder} avoiding
* the need to create one manually via {@link GetCoreNetworkChangeSetRequest#builder()}
*
*
* @param getCoreNetworkChangeSetRequest
* A {@link Consumer} that will call methods on {@link GetCoreNetworkChangeSetRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkChangeSet
* @see AWS API Documentation
*/
default GetCoreNetworkChangeSetPublisher getCoreNetworkChangeSetPaginator(
Consumer getCoreNetworkChangeSetRequest) {
return getCoreNetworkChangeSetPaginator(GetCoreNetworkChangeSetRequest.builder()
.applyMutation(getCoreNetworkChangeSetRequest).build());
}
/**
*
* Gets details about a core network policy. You can get details about your current live policy or any previous
* policy version.
*
*
* @param getCoreNetworkPolicyRequest
* @return A Java Future containing the result of the GetCoreNetworkPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkPolicy
* @see AWS API Documentation
*/
default CompletableFuture getCoreNetworkPolicy(
GetCoreNetworkPolicyRequest getCoreNetworkPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets details about a core network policy. You can get details about your current live policy or any previous
* policy version.
*
*
*
* This is a convenience which creates an instance of the {@link GetCoreNetworkPolicyRequest.Builder} avoiding the
* need to create one manually via {@link GetCoreNetworkPolicyRequest#builder()}
*
*
* @param getCoreNetworkPolicyRequest
* A {@link Consumer} that will call methods on {@link GetCoreNetworkPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetCoreNetworkPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCoreNetworkPolicy
* @see AWS API Documentation
*/
default CompletableFuture getCoreNetworkPolicy(
Consumer getCoreNetworkPolicyRequest) {
return getCoreNetworkPolicy(GetCoreNetworkPolicyRequest.builder().applyMutation(getCoreNetworkPolicyRequest).build());
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return A Java Future containing the result of the GetCustomerGatewayAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default CompletableFuture getCustomerGatewayAssociations(
GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a convenience which creates an instance of the {@link GetCustomerGatewayAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetCustomerGatewayAssociationsRequest#builder()}
*
*
* @param getCustomerGatewayAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetCustomerGatewayAssociationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetCustomerGatewayAssociations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default CompletableFuture getCustomerGatewayAssociations(
Consumer getCustomerGatewayAssociationsRequest) {
return getCustomerGatewayAssociations(GetCustomerGatewayAssociationsRequest.builder()
.applyMutation(getCustomerGatewayAssociationsRequest).build());
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a variant of
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation.
*
*
* @param getCustomerGatewayAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default GetCustomerGatewayAssociationsPublisher getCustomerGatewayAssociationsPaginator(
GetCustomerGatewayAssociationsRequest getCustomerGatewayAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the association information for customer gateways that are associated with devices and links in your global
* network.
*
*
*
* This is a variant of
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetCustomerGatewayAssociationsPublisher publisher = client.getCustomerGatewayAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getCustomerGatewayAssociations(software.amazon.awssdk.services.networkmanager.model.GetCustomerGatewayAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetCustomerGatewayAssociationsRequest.Builder}
* avoiding the need to create one manually via {@link GetCustomerGatewayAssociationsRequest#builder()}
*
*
* @param getCustomerGatewayAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetCustomerGatewayAssociationsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetCustomerGatewayAssociations
* @see AWS API Documentation
*/
default GetCustomerGatewayAssociationsPublisher getCustomerGatewayAssociationsPaginator(
Consumer getCustomerGatewayAssociationsRequest) {
return getCustomerGatewayAssociationsPaginator(GetCustomerGatewayAssociationsRequest.builder()
.applyMutation(getCustomerGatewayAssociationsRequest).build());
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
* @param getDevicesRequest
* @return A Java Future containing the result of the GetDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default CompletableFuture getDevices(GetDevicesRequest getDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetDevicesRequest.Builder} avoiding the need to
* create one manually via {@link GetDevicesRequest#builder()}
*
*
* @param getDevicesRequest
* A {@link Consumer} that will call methods on {@link GetDevicesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetDevices operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default CompletableFuture getDevices(Consumer getDevicesRequest) {
return getDevices(GetDevicesRequest.builder().applyMutation(getDevicesRequest).build());
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a variant of {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)} operation.
*
*
* @param getDevicesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default GetDevicesPublisher getDevicesPaginator(GetDevicesRequest getDevicesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your devices in a global network.
*
*
*
* This is a variant of {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetDevicesPublisher publisher = client.getDevicesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetDevicesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getDevices(software.amazon.awssdk.services.networkmanager.model.GetDevicesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetDevicesRequest.Builder} avoiding the need to
* create one manually via {@link GetDevicesRequest#builder()}
*
*
* @param getDevicesRequest
* A {@link Consumer} that will call methods on {@link GetDevicesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetDevices
* @see AWS API
* Documentation
*/
default GetDevicesPublisher getDevicesPaginator(Consumer getDevicesRequest) {
return getDevicesPaginator(GetDevicesRequest.builder().applyMutation(getDevicesRequest).build());
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
* @param getLinkAssociationsRequest
* @return A Java Future containing the result of the GetLinkAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default CompletableFuture getLinkAssociations(
GetLinkAssociationsRequest getLinkAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a convenience which creates an instance of the {@link GetLinkAssociationsRequest.Builder} avoiding the
* need to create one manually via {@link GetLinkAssociationsRequest#builder()}
*
*
* @param getLinkAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetLinkAssociationsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLinkAssociations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default CompletableFuture getLinkAssociations(
Consumer getLinkAssociationsRequest) {
return getLinkAssociations(GetLinkAssociationsRequest.builder().applyMutation(getLinkAssociationsRequest).build());
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a variant of
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation.
*
*
* @param getLinkAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default GetLinkAssociationsPublisher getLinkAssociationsPaginator(GetLinkAssociationsRequest getLinkAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the link associations for a device or a link. Either the device ID or the link ID must be specified.
*
*
*
* This is a variant of
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinkAssociationsPublisher publisher = client.getLinkAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinkAssociations(software.amazon.awssdk.services.networkmanager.model.GetLinkAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetLinkAssociationsRequest.Builder} avoiding the
* need to create one manually via {@link GetLinkAssociationsRequest#builder()}
*
*
* @param getLinkAssociationsRequest
* A {@link Consumer} that will call methods on {@link GetLinkAssociationsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinkAssociations
* @see AWS API Documentation
*/
default GetLinkAssociationsPublisher getLinkAssociationsPaginator(
Consumer getLinkAssociationsRequest) {
return getLinkAssociationsPaginator(GetLinkAssociationsRequest.builder().applyMutation(getLinkAssociationsRequest)
.build());
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
* @param getLinksRequest
* @return A Java Future containing the result of the GetLinks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default CompletableFuture getLinks(GetLinksRequest getLinksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a convenience which creates an instance of the {@link GetLinksRequest.Builder} avoiding the need to
* create one manually via {@link GetLinksRequest#builder()}
*
*
* @param getLinksRequest
* A {@link Consumer} that will call methods on {@link GetLinksRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetLinks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default CompletableFuture getLinks(Consumer getLinksRequest) {
return getLinks(GetLinksRequest.builder().applyMutation(getLinksRequest).build());
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a variant of {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)} operation.
*
*
* @param getLinksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default GetLinksPublisher getLinksPaginator(GetLinksRequest getLinksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more links in a specified global network.
*
*
* If you specify the site ID, you cannot specify the type or provider in the same request. You can specify the type
* and provider in the same request.
*
*
*
* This is a variant of {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetLinksPublisher publisher = client.getLinksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetLinksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getLinks(software.amazon.awssdk.services.networkmanager.model.GetLinksRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetLinksRequest.Builder} avoiding the need to
* create one manually via {@link GetLinksRequest#builder()}
*
*
* @param getLinksRequest
* A {@link Consumer} that will call methods on {@link GetLinksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetLinks
* @see AWS API
* Documentation
*/
default GetLinksPublisher getLinksPaginator(Consumer getLinksRequest) {
return getLinksPaginator(GetLinksRequest.builder().applyMutation(getLinksRequest).build());
}
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
* @param getNetworkResourceCountsRequest
* @return A Java Future containing the result of the GetNetworkResourceCounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceCounts
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResourceCounts(
GetNetworkResourceCountsRequest getNetworkResourceCountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourceCountsRequest.Builder} avoiding
* the need to create one manually via {@link GetNetworkResourceCountsRequest#builder()}
*
*
* @param getNetworkResourceCountsRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourceCountsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetNetworkResourceCounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceCounts
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResourceCounts(
Consumer getNetworkResourceCountsRequest) {
return getNetworkResourceCounts(GetNetworkResourceCountsRequest.builder().applyMutation(getNetworkResourceCountsRequest)
.build());
}
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkResourceCounts(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceCountsPublisher publisher = client.getNetworkResourceCountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceCountsPublisher publisher = client.getNetworkResourceCountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResourceCounts(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsRequest)}
* operation.
*
*
* @param getNetworkResourceCountsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceCounts
* @see AWS API Documentation
*/
default GetNetworkResourceCountsPublisher getNetworkResourceCountsPaginator(
GetNetworkResourceCountsRequest getNetworkResourceCountsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the count of network resources, by resource type, for the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkResourceCounts(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceCountsPublisher publisher = client.getNetworkResourceCountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceCountsPublisher publisher = client.getNetworkResourceCountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResourceCounts(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceCountsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourceCountsRequest.Builder} avoiding
* the need to create one manually via {@link GetNetworkResourceCountsRequest#builder()}
*
*
* @param getNetworkResourceCountsRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourceCountsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceCounts
* @see AWS API Documentation
*/
default GetNetworkResourceCountsPublisher getNetworkResourceCountsPaginator(
Consumer getNetworkResourceCountsRequest) {
return getNetworkResourceCountsPaginator(GetNetworkResourceCountsRequest.builder()
.applyMutation(getNetworkResourceCountsRequest).build());
}
/**
*
* Gets the network resource relationships for the specified global network.
*
*
* @param getNetworkResourceRelationshipsRequest
* @return A Java Future containing the result of the GetNetworkResourceRelationships operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResourceRelationships(
GetNetworkResourceRelationshipsRequest getNetworkResourceRelationshipsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network resource relationships for the specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourceRelationshipsRequest.Builder}
* avoiding the need to create one manually via {@link GetNetworkResourceRelationshipsRequest#builder()}
*
*
* @param getNetworkResourceRelationshipsRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourceRelationshipsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetNetworkResourceRelationships operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResourceRelationships(
Consumer getNetworkResourceRelationshipsRequest) {
return getNetworkResourceRelationships(GetNetworkResourceRelationshipsRequest.builder()
.applyMutation(getNetworkResourceRelationshipsRequest).build());
}
/**
*
* Gets the network resource relationships for the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkResourceRelationships(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceRelationshipsPublisher publisher = client.getNetworkResourceRelationshipsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceRelationshipsPublisher publisher = client.getNetworkResourceRelationshipsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResourceRelationships(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsRequest)}
* operation.
*
*
* @param getNetworkResourceRelationshipsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
default GetNetworkResourceRelationshipsPublisher getNetworkResourceRelationshipsPaginator(
GetNetworkResourceRelationshipsRequest getNetworkResourceRelationshipsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network resource relationships for the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkResourceRelationships(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceRelationshipsPublisher publisher = client.getNetworkResourceRelationshipsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourceRelationshipsPublisher publisher = client.getNetworkResourceRelationshipsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResourceRelationships(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourceRelationshipsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourceRelationshipsRequest.Builder}
* avoiding the need to create one manually via {@link GetNetworkResourceRelationshipsRequest#builder()}
*
*
* @param getNetworkResourceRelationshipsRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourceRelationshipsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResourceRelationships
* @see AWS API Documentation
*/
default GetNetworkResourceRelationshipsPublisher getNetworkResourceRelationshipsPaginator(
Consumer getNetworkResourceRelationshipsRequest) {
return getNetworkResourceRelationshipsPaginator(GetNetworkResourceRelationshipsRequest.builder()
.applyMutation(getNetworkResourceRelationshipsRequest).build());
}
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
* @param getNetworkResourcesRequest
* @return A Java Future containing the result of the GetNetworkResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResources
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResources(
GetNetworkResourcesRequest getNetworkResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourcesRequest.Builder} avoiding the
* need to create one manually via {@link GetNetworkResourcesRequest#builder()}
*
*
* @param getNetworkResourcesRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourcesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetNetworkResources operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResources
* @see AWS API Documentation
*/
default CompletableFuture getNetworkResources(
Consumer getNetworkResourcesRequest) {
return getNetworkResources(GetNetworkResourcesRequest.builder().applyMutation(getNetworkResourcesRequest).build());
}
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
*
* This is a variant of
* {@link #getNetworkResources(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourcesPublisher publisher = client.getNetworkResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourcesPublisher publisher = client.getNetworkResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResources(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesRequest)}
* operation.
*
*
* @param getNetworkResourcesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResources
* @see AWS API Documentation
*/
default GetNetworkResourcesPublisher getNetworkResourcesPaginator(GetNetworkResourcesRequest getNetworkResourcesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Describes the network resources for the specified global network.
*
*
* The results include information from the corresponding Describe call for the resource, minus any sensitive
* information such as pre-shared keys.
*
*
*
* This is a variant of
* {@link #getNetworkResources(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourcesPublisher publisher = client.getNetworkResourcesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkResourcesPublisher publisher = client.getNetworkResourcesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkResources(software.amazon.awssdk.services.networkmanager.model.GetNetworkResourcesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetNetworkResourcesRequest.Builder} avoiding the
* need to create one manually via {@link GetNetworkResourcesRequest#builder()}
*
*
* @param getNetworkResourcesRequest
* A {@link Consumer} that will call methods on {@link GetNetworkResourcesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkResources
* @see AWS API Documentation
*/
default GetNetworkResourcesPublisher getNetworkResourcesPaginator(
Consumer getNetworkResourcesRequest) {
return getNetworkResourcesPaginator(GetNetworkResourcesRequest.builder().applyMutation(getNetworkResourcesRequest)
.build());
}
/**
*
* Gets the network routes of the specified global network.
*
*
* @param getNetworkRoutesRequest
* @return A Java Future containing the result of the GetNetworkRoutes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkRoutes
* @see AWS API Documentation
*/
default CompletableFuture getNetworkRoutes(GetNetworkRoutesRequest getNetworkRoutesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network routes of the specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkRoutesRequest.Builder} avoiding the need
* to create one manually via {@link GetNetworkRoutesRequest#builder()}
*
*
* @param getNetworkRoutesRequest
* A {@link Consumer} that will call methods on {@link GetNetworkRoutesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetNetworkRoutes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkRoutes
* @see AWS API Documentation
*/
default CompletableFuture getNetworkRoutes(
Consumer getNetworkRoutesRequest) {
return getNetworkRoutes(GetNetworkRoutesRequest.builder().applyMutation(getNetworkRoutesRequest).build());
}
/**
*
* Gets the network telemetry of the specified global network.
*
*
* @param getNetworkTelemetryRequest
* @return A Java Future containing the result of the GetNetworkTelemetry operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkTelemetry
* @see AWS API Documentation
*/
default CompletableFuture getNetworkTelemetry(
GetNetworkTelemetryRequest getNetworkTelemetryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network telemetry of the specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetNetworkTelemetryRequest.Builder} avoiding the
* need to create one manually via {@link GetNetworkTelemetryRequest#builder()}
*
*
* @param getNetworkTelemetryRequest
* A {@link Consumer} that will call methods on {@link GetNetworkTelemetryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetNetworkTelemetry operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkTelemetry
* @see AWS API Documentation
*/
default CompletableFuture getNetworkTelemetry(
Consumer getNetworkTelemetryRequest) {
return getNetworkTelemetry(GetNetworkTelemetryRequest.builder().applyMutation(getNetworkTelemetryRequest).build());
}
/**
*
* Gets the network telemetry of the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkTelemetry(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkTelemetryPublisher publisher = client.getNetworkTelemetryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkTelemetryPublisher publisher = client.getNetworkTelemetryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkTelemetry(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryRequest)}
* operation.
*
*
* @param getNetworkTelemetryRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkTelemetry
* @see AWS API Documentation
*/
default GetNetworkTelemetryPublisher getNetworkTelemetryPaginator(GetNetworkTelemetryRequest getNetworkTelemetryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the network telemetry of the specified global network.
*
*
*
* This is a variant of
* {@link #getNetworkTelemetry(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkTelemetryPublisher publisher = client.getNetworkTelemetryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetNetworkTelemetryPublisher publisher = client.getNetworkTelemetryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getNetworkTelemetry(software.amazon.awssdk.services.networkmanager.model.GetNetworkTelemetryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetNetworkTelemetryRequest.Builder} avoiding the
* need to create one manually via {@link GetNetworkTelemetryRequest#builder()}
*
*
* @param getNetworkTelemetryRequest
* A {@link Consumer} that will call methods on {@link GetNetworkTelemetryRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetNetworkTelemetry
* @see AWS API Documentation
*/
default GetNetworkTelemetryPublisher getNetworkTelemetryPaginator(
Consumer getNetworkTelemetryRequest) {
return getNetworkTelemetryPaginator(GetNetworkTelemetryRequest.builder().applyMutation(getNetworkTelemetryRequest)
.build());
}
/**
*
* Returns information about a resource policy.
*
*
* @param getResourcePolicyRequest
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture getResourcePolicy(GetResourcePolicyRequest getResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a resource policy.
*
*
*
* This is a convenience which creates an instance of the {@link GetResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link GetResourcePolicyRequest#builder()}
*
*
* @param getResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link GetResourcePolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture getResourcePolicy(
Consumer getResourcePolicyRequest) {
return getResourcePolicy(GetResourcePolicyRequest.builder().applyMutation(getResourcePolicyRequest).build());
}
/**
*
* Gets information about the specified route analysis.
*
*
* @param getRouteAnalysisRequest
* @return A Java Future containing the result of the GetRouteAnalysis operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetRouteAnalysis
* @see AWS API Documentation
*/
default CompletableFuture getRouteAnalysis(GetRouteAnalysisRequest getRouteAnalysisRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified route analysis.
*
*
*
* This is a convenience which creates an instance of the {@link GetRouteAnalysisRequest.Builder} avoiding the need
* to create one manually via {@link GetRouteAnalysisRequest#builder()}
*
*
* @param getRouteAnalysisRequest
* A {@link Consumer} that will call methods on {@link GetRouteAnalysisRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetRouteAnalysis operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetRouteAnalysis
* @see AWS API Documentation
*/
default CompletableFuture getRouteAnalysis(
Consumer getRouteAnalysisRequest) {
return getRouteAnalysis(GetRouteAnalysisRequest.builder().applyMutation(getRouteAnalysisRequest).build());
}
/**
*
* Returns information about a site-to-site VPN attachment.
*
*
* @param getSiteToSiteVpnAttachmentRequest
* @return A Java Future containing the result of the GetSiteToSiteVpnAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
default CompletableFuture getSiteToSiteVpnAttachment(
GetSiteToSiteVpnAttachmentRequest getSiteToSiteVpnAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a site-to-site VPN attachment.
*
*
*
* This is a convenience which creates an instance of the {@link GetSiteToSiteVpnAttachmentRequest.Builder} avoiding
* the need to create one manually via {@link GetSiteToSiteVpnAttachmentRequest#builder()}
*
*
* @param getSiteToSiteVpnAttachmentRequest
* A {@link Consumer} that will call methods on {@link GetSiteToSiteVpnAttachmentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetSiteToSiteVpnAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSiteToSiteVpnAttachment
* @see AWS API Documentation
*/
default CompletableFuture getSiteToSiteVpnAttachment(
Consumer getSiteToSiteVpnAttachmentRequest) {
return getSiteToSiteVpnAttachment(GetSiteToSiteVpnAttachmentRequest.builder()
.applyMutation(getSiteToSiteVpnAttachmentRequest).build());
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
* @param getSitesRequest
* @return A Java Future containing the result of the GetSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default CompletableFuture getSites(GetSitesRequest getSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetSitesRequest.Builder} avoiding the need to
* create one manually via {@link GetSitesRequest#builder()}
*
*
* @param getSitesRequest
* A {@link Consumer} that will call methods on {@link GetSitesRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default CompletableFuture getSites(Consumer getSitesRequest) {
return getSites(GetSitesRequest.builder().applyMutation(getSitesRequest).build());
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a variant of {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)} operation.
*
*
* @param getSitesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default GetSitesPublisher getSitesPaginator(GetSitesRequest getSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your sites in a global network.
*
*
*
* This is a variant of {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetSitesPublisher publisher = client.getSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getSites(software.amazon.awssdk.services.networkmanager.model.GetSitesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link GetSitesRequest.Builder} avoiding the need to
* create one manually via {@link GetSitesRequest#builder()}
*
*
* @param getSitesRequest
* A {@link Consumer} that will call methods on {@link GetSitesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetSites
* @see AWS API
* Documentation
*/
default GetSitesPublisher getSitesPaginator(Consumer getSitesRequest) {
return getSitesPaginator(GetSitesRequest.builder().applyMutation(getSitesRequest).build());
}
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* @return A Java Future containing the result of the GetTransitGatewayConnectPeerAssociations operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayConnectPeerAssociations(
GetTransitGatewayConnectPeerAssociationsRequest getTransitGatewayConnectPeerAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
*
* This is a convenience which creates an instance of the
* {@link GetTransitGatewayConnectPeerAssociationsRequest.Builder} avoiding the need to create one manually via
* {@link GetTransitGatewayConnectPeerAssociationsRequest#builder()}
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link GetTransitGatewayConnectPeerAssociationsRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetTransitGatewayConnectPeerAssociations operation returned by
* the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayConnectPeerAssociations(
Consumer getTransitGatewayConnectPeerAssociationsRequest) {
return getTransitGatewayConnectPeerAssociations(GetTransitGatewayConnectPeerAssociationsRequest.builder()
.applyMutation(getTransitGatewayConnectPeerAssociationsRequest).build());
}
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayConnectPeerAssociationsPublisher publisher = client.getTransitGatewayConnectPeerAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayConnectPeerAssociationsPublisher publisher = client.getTransitGatewayConnectPeerAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsRequest)}
* operation.
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
default GetTransitGatewayConnectPeerAssociationsPublisher getTransitGatewayConnectPeerAssociationsPaginator(
GetTransitGatewayConnectPeerAssociationsRequest getTransitGatewayConnectPeerAssociationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about one or more of your transit gateway Connect peer associations in a global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayConnectPeerAssociationsPublisher publisher = client.getTransitGatewayConnectPeerAssociationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayConnectPeerAssociationsPublisher publisher = client.getTransitGatewayConnectPeerAssociationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayConnectPeerAssociations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayConnectPeerAssociationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the
* {@link GetTransitGatewayConnectPeerAssociationsRequest.Builder} avoiding the need to create one manually via
* {@link GetTransitGatewayConnectPeerAssociationsRequest#builder()}
*
*
* @param getTransitGatewayConnectPeerAssociationsRequest
* A {@link Consumer} that will call methods on
* {@link GetTransitGatewayConnectPeerAssociationsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayConnectPeerAssociations
* @see AWS API Documentation
*/
default GetTransitGatewayConnectPeerAssociationsPublisher getTransitGatewayConnectPeerAssociationsPaginator(
Consumer getTransitGatewayConnectPeerAssociationsRequest) {
return getTransitGatewayConnectPeerAssociationsPaginator(GetTransitGatewayConnectPeerAssociationsRequest.builder()
.applyMutation(getTransitGatewayConnectPeerAssociationsRequest).build());
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return A Java Future containing the result of the GetTransitGatewayRegistrations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayRegistrations(
GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link GetTransitGatewayRegistrationsRequest.Builder}
* avoiding the need to create one manually via {@link GetTransitGatewayRegistrationsRequest#builder()}
*
*
* @param getTransitGatewayRegistrationsRequest
* A {@link Consumer} that will call methods on {@link GetTransitGatewayRegistrationsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the GetTransitGatewayRegistrations operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default CompletableFuture getTransitGatewayRegistrations(
Consumer getTransitGatewayRegistrationsRequest) {
return getTransitGatewayRegistrations(GetTransitGatewayRegistrationsRequest.builder()
.applyMutation(getTransitGatewayRegistrationsRequest).build());
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation.
*
*
* @param getTransitGatewayRegistrationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default GetTransitGatewayRegistrationsPublisher getTransitGatewayRegistrationsPaginator(
GetTransitGatewayRegistrationsRequest getTransitGatewayRegistrationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the transit gateway registrations in a specified global network.
*
*
*
* This is a variant of
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.GetTransitGatewayRegistrationsPublisher publisher = client.getTransitGatewayRegistrationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getTransitGatewayRegistrations(software.amazon.awssdk.services.networkmanager.model.GetTransitGatewayRegistrationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetTransitGatewayRegistrationsRequest.Builder}
* avoiding the need to create one manually via {@link GetTransitGatewayRegistrationsRequest#builder()}
*
*
* @param getTransitGatewayRegistrationsRequest
* A {@link Consumer} that will call methods on {@link GetTransitGatewayRegistrationsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetTransitGatewayRegistrations
* @see AWS API Documentation
*/
default GetTransitGatewayRegistrationsPublisher getTransitGatewayRegistrationsPaginator(
Consumer getTransitGatewayRegistrationsRequest) {
return getTransitGatewayRegistrationsPaginator(GetTransitGatewayRegistrationsRequest.builder()
.applyMutation(getTransitGatewayRegistrationsRequest).build());
}
/**
*
* Returns information about a VPC attachment.
*
*
* @param getVpcAttachmentRequest
* @return A Java Future containing the result of the GetVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture getVpcAttachment(GetVpcAttachmentRequest getVpcAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about a VPC attachment.
*
*
*
* This is a convenience which creates an instance of the {@link GetVpcAttachmentRequest.Builder} avoiding the need
* to create one manually via {@link GetVpcAttachmentRequest#builder()}
*
*
* @param getVpcAttachmentRequest
* A {@link Consumer} that will call methods on {@link GetVpcAttachmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.GetVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture getVpcAttachment(
Consumer getVpcAttachmentRequest) {
return getVpcAttachment(GetVpcAttachmentRequest.builder().applyMutation(getVpcAttachmentRequest).build());
}
/**
*
* Returns a list of core network attachments.
*
*
* @param listAttachmentsRequest
* @return A Java Future containing the result of the ListAttachments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListAttachments
* @see AWS API Documentation
*/
default CompletableFuture listAttachments(ListAttachmentsRequest listAttachmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network attachments.
*
*
*
* This is a convenience which creates an instance of the {@link ListAttachmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListAttachmentsRequest#builder()}
*
*
* @param listAttachmentsRequest
* A {@link Consumer} that will call methods on {@link ListAttachmentsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListAttachments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListAttachments
* @see AWS API Documentation
*/
default CompletableFuture listAttachments(
Consumer listAttachmentsRequest) {
return listAttachments(ListAttachmentsRequest.builder().applyMutation(listAttachmentsRequest).build());
}
/**
*
* Returns a list of core network attachments.
*
*
*
* This is a variant of
* {@link #listAttachments(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListAttachmentsPublisher publisher = client.listAttachmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListAttachmentsPublisher publisher = client.listAttachmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachments(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsRequest)}
* operation.
*
*
* @param listAttachmentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListAttachments
* @see AWS API Documentation
*/
default ListAttachmentsPublisher listAttachmentsPaginator(ListAttachmentsRequest listAttachmentsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network attachments.
*
*
*
* This is a variant of
* {@link #listAttachments(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListAttachmentsPublisher publisher = client.listAttachmentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListAttachmentsPublisher publisher = client.listAttachmentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAttachments(software.amazon.awssdk.services.networkmanager.model.ListAttachmentsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListAttachmentsRequest.Builder} avoiding the need
* to create one manually via {@link ListAttachmentsRequest#builder()}
*
*
* @param listAttachmentsRequest
* A {@link Consumer} that will call methods on {@link ListAttachmentsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListAttachments
* @see AWS API Documentation
*/
default ListAttachmentsPublisher listAttachmentsPaginator(Consumer listAttachmentsRequest) {
return listAttachmentsPaginator(ListAttachmentsRequest.builder().applyMutation(listAttachmentsRequest).build());
}
/**
*
* Returns a list of core network Connect peers.
*
*
* @param listConnectPeersRequest
* @return A Java Future containing the result of the ListConnectPeers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListConnectPeers
* @see AWS API Documentation
*/
default CompletableFuture listConnectPeers(ListConnectPeersRequest listConnectPeersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network Connect peers.
*
*
*
* This is a convenience which creates an instance of the {@link ListConnectPeersRequest.Builder} avoiding the need
* to create one manually via {@link ListConnectPeersRequest#builder()}
*
*
* @param listConnectPeersRequest
* A {@link Consumer} that will call methods on {@link ListConnectPeersRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListConnectPeers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListConnectPeers
* @see AWS API Documentation
*/
default CompletableFuture listConnectPeers(
Consumer listConnectPeersRequest) {
return listConnectPeers(ListConnectPeersRequest.builder().applyMutation(listConnectPeersRequest).build());
}
/**
*
* Returns a list of core network Connect peers.
*
*
*
* This is a variant of
* {@link #listConnectPeers(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListConnectPeersPublisher publisher = client.listConnectPeersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListConnectPeersPublisher publisher = client.listConnectPeersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnectPeers(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersRequest)}
* operation.
*
*
* @param listConnectPeersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListConnectPeers
* @see AWS API Documentation
*/
default ListConnectPeersPublisher listConnectPeersPaginator(ListConnectPeersRequest listConnectPeersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network Connect peers.
*
*
*
* This is a variant of
* {@link #listConnectPeers(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListConnectPeersPublisher publisher = client.listConnectPeersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListConnectPeersPublisher publisher = client.listConnectPeersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listConnectPeers(software.amazon.awssdk.services.networkmanager.model.ListConnectPeersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListConnectPeersRequest.Builder} avoiding the need
* to create one manually via {@link ListConnectPeersRequest#builder()}
*
*
* @param listConnectPeersRequest
* A {@link Consumer} that will call methods on {@link ListConnectPeersRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListConnectPeers
* @see AWS API Documentation
*/
default ListConnectPeersPublisher listConnectPeersPaginator(Consumer listConnectPeersRequest) {
return listConnectPeersPaginator(ListConnectPeersRequest.builder().applyMutation(listConnectPeersRequest).build());
}
/**
*
* Returns a list of core network policy versions.
*
*
* @param listCoreNetworkPolicyVersionsRequest
* @return A Java Future containing the result of the ListCoreNetworkPolicyVersions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
default CompletableFuture listCoreNetworkPolicyVersions(
ListCoreNetworkPolicyVersionsRequest listCoreNetworkPolicyVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network policy versions.
*
*
*
* This is a convenience which creates an instance of the {@link ListCoreNetworkPolicyVersionsRequest.Builder}
* avoiding the need to create one manually via {@link ListCoreNetworkPolicyVersionsRequest#builder()}
*
*
* @param listCoreNetworkPolicyVersionsRequest
* A {@link Consumer} that will call methods on {@link ListCoreNetworkPolicyVersionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListCoreNetworkPolicyVersions operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
default CompletableFuture listCoreNetworkPolicyVersions(
Consumer listCoreNetworkPolicyVersionsRequest) {
return listCoreNetworkPolicyVersions(ListCoreNetworkPolicyVersionsRequest.builder()
.applyMutation(listCoreNetworkPolicyVersionsRequest).build());
}
/**
*
* Returns a list of core network policy versions.
*
*
*
* This is a variant of
* {@link #listCoreNetworkPolicyVersions(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworkPolicyVersionsPublisher publisher = client.listCoreNetworkPolicyVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworkPolicyVersionsPublisher publisher = client.listCoreNetworkPolicyVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCoreNetworkPolicyVersions(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsRequest)}
* operation.
*
*
* @param listCoreNetworkPolicyVersionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
default ListCoreNetworkPolicyVersionsPublisher listCoreNetworkPolicyVersionsPaginator(
ListCoreNetworkPolicyVersionsRequest listCoreNetworkPolicyVersionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of core network policy versions.
*
*
*
* This is a variant of
* {@link #listCoreNetworkPolicyVersions(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworkPolicyVersionsPublisher publisher = client.listCoreNetworkPolicyVersionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworkPolicyVersionsPublisher publisher = client.listCoreNetworkPolicyVersionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCoreNetworkPolicyVersions(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworkPolicyVersionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListCoreNetworkPolicyVersionsRequest.Builder}
* avoiding the need to create one manually via {@link ListCoreNetworkPolicyVersionsRequest#builder()}
*
*
* @param listCoreNetworkPolicyVersionsRequest
* A {@link Consumer} that will call methods on {@link ListCoreNetworkPolicyVersionsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworkPolicyVersions
* @see AWS API Documentation
*/
default ListCoreNetworkPolicyVersionsPublisher listCoreNetworkPolicyVersionsPaginator(
Consumer listCoreNetworkPolicyVersionsRequest) {
return listCoreNetworkPolicyVersionsPaginator(ListCoreNetworkPolicyVersionsRequest.builder()
.applyMutation(listCoreNetworkPolicyVersionsRequest).build());
}
/**
*
* Returns a list of owned and shared core networks.
*
*
* @param listCoreNetworksRequest
* @return A Java Future containing the result of the ListCoreNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworks
* @see AWS API Documentation
*/
default CompletableFuture listCoreNetworks(ListCoreNetworksRequest listCoreNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of owned and shared core networks.
*
*
*
* This is a convenience which creates an instance of the {@link ListCoreNetworksRequest.Builder} avoiding the need
* to create one manually via {@link ListCoreNetworksRequest#builder()}
*
*
* @param listCoreNetworksRequest
* A {@link Consumer} that will call methods on {@link ListCoreNetworksRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListCoreNetworks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworks
* @see AWS API Documentation
*/
default CompletableFuture listCoreNetworks(
Consumer listCoreNetworksRequest) {
return listCoreNetworks(ListCoreNetworksRequest.builder().applyMutation(listCoreNetworksRequest).build());
}
/**
*
* Returns a list of owned and shared core networks.
*
*
*
* This is a variant of
* {@link #listCoreNetworks(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworksPublisher publisher = client.listCoreNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworksPublisher publisher = client.listCoreNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCoreNetworks(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksRequest)}
* operation.
*
*
* @param listCoreNetworksRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworks
* @see AWS API Documentation
*/
default ListCoreNetworksPublisher listCoreNetworksPaginator(ListCoreNetworksRequest listCoreNetworksRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of owned and shared core networks.
*
*
*
* This is a variant of
* {@link #listCoreNetworks(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworksPublisher publisher = client.listCoreNetworksPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.networkmanager.paginators.ListCoreNetworksPublisher publisher = client.listCoreNetworksPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCoreNetworks(software.amazon.awssdk.services.networkmanager.model.ListCoreNetworksRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListCoreNetworksRequest.Builder} avoiding the need
* to create one manually via {@link ListCoreNetworksRequest#builder()}
*
*
* @param listCoreNetworksRequest
* A {@link Consumer} that will call methods on {@link ListCoreNetworksRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListCoreNetworks
* @see AWS API Documentation
*/
default ListCoreNetworksPublisher listCoreNetworksPaginator(Consumer listCoreNetworksRequest) {
return listCoreNetworksPaginator(ListCoreNetworksRequest.builder().applyMutation(listCoreNetworksRequest).build());
}
/**
*
* Lists the tags for a specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Creates a new, immutable version of a core network policy. A subsequent change set is created showing the
* differences between the LIVE policy and the submitted policy.
*
*
* @param putCoreNetworkPolicyRequest
* @return A Java Future containing the result of the PutCoreNetworkPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CoreNetworkPolicyException Describes a core network policy exception.
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.PutCoreNetworkPolicy
* @see AWS API Documentation
*/
default CompletableFuture putCoreNetworkPolicy(
PutCoreNetworkPolicyRequest putCoreNetworkPolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new, immutable version of a core network policy. A subsequent change set is created showing the
* differences between the LIVE policy and the submitted policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutCoreNetworkPolicyRequest.Builder} avoiding the
* need to create one manually via {@link PutCoreNetworkPolicyRequest#builder()}
*
*
* @param putCoreNetworkPolicyRequest
* A {@link Consumer} that will call methods on {@link PutCoreNetworkPolicyRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the PutCoreNetworkPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - CoreNetworkPolicyException Describes a core network policy exception.
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.PutCoreNetworkPolicy
* @see AWS API Documentation
*/
default CompletableFuture putCoreNetworkPolicy(
Consumer putCoreNetworkPolicyRequest) {
return putCoreNetworkPolicy(PutCoreNetworkPolicyRequest.builder().applyMutation(putCoreNetworkPolicyRequest).build());
}
/**
*
* Creates or updates a resource policy.
*
*
* @param putResourcePolicyRequest
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.PutResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture putResourcePolicy(PutResourcePolicyRequest putResourcePolicyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates or updates a resource policy.
*
*
*
* This is a convenience which creates an instance of the {@link PutResourcePolicyRequest.Builder} avoiding the need
* to create one manually via {@link PutResourcePolicyRequest#builder()}
*
*
* @param putResourcePolicyRequest
* A {@link Consumer} that will call methods on {@link PutResourcePolicyRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutResourcePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.PutResourcePolicy
* @see AWS API Documentation
*/
default CompletableFuture putResourcePolicy(
Consumer putResourcePolicyRequest) {
return putResourcePolicy(PutResourcePolicyRequest.builder().applyMutation(putResourcePolicyRequest).build());
}
/**
*
* Registers a transit gateway in your global network. The transit gateway can be in any Amazon Web Services Region,
* but it must be owned by the same Amazon Web Services account that owns the global network. You cannot register a
* transit gateway in more than one global network.
*
*
* @param registerTransitGatewayRequest
* @return A Java Future containing the result of the RegisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RegisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture registerTransitGateway(
RegisterTransitGatewayRequest registerTransitGatewayRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Registers a transit gateway in your global network. The transit gateway can be in any Amazon Web Services Region,
* but it must be owned by the same Amazon Web Services account that owns the global network. You cannot register a
* transit gateway in more than one global network.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterTransitGatewayRequest.Builder} avoiding the
* need to create one manually via {@link RegisterTransitGatewayRequest#builder()}
*
*
* @param registerTransitGatewayRequest
* A {@link Consumer} that will call methods on {@link RegisterTransitGatewayRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the RegisterTransitGateway operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RegisterTransitGateway
* @see AWS API Documentation
*/
default CompletableFuture registerTransitGateway(
Consumer registerTransitGatewayRequest) {
return registerTransitGateway(RegisterTransitGatewayRequest.builder().applyMutation(registerTransitGatewayRequest)
.build());
}
/**
*
* Rejects a core network attachment request.
*
*
* @param rejectAttachmentRequest
* @return A Java Future containing the result of the RejectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RejectAttachment
* @see AWS API Documentation
*/
default CompletableFuture rejectAttachment(RejectAttachmentRequest rejectAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Rejects a core network attachment request.
*
*
*
* This is a convenience which creates an instance of the {@link RejectAttachmentRequest.Builder} avoiding the need
* to create one manually via {@link RejectAttachmentRequest#builder()}
*
*
* @param rejectAttachmentRequest
* A {@link Consumer} that will call methods on {@link RejectAttachmentRequest.Builder} to create a request.
* @return A Java Future containing the result of the RejectAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RejectAttachment
* @see AWS API Documentation
*/
default CompletableFuture rejectAttachment(
Consumer rejectAttachmentRequest) {
return rejectAttachment(RejectAttachmentRequest.builder().applyMutation(rejectAttachmentRequest).build());
}
/**
*
* Restores a previous policy version as a new, immutable version of a core network policy. A subsequent change set
* is created showing the differences between the LIVE policy and restored policy.
*
*
* @param restoreCoreNetworkPolicyVersionRequest
* @return A Java Future containing the result of the RestoreCoreNetworkPolicyVersion operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RestoreCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
default CompletableFuture restoreCoreNetworkPolicyVersion(
RestoreCoreNetworkPolicyVersionRequest restoreCoreNetworkPolicyVersionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Restores a previous policy version as a new, immutable version of a core network policy. A subsequent change set
* is created showing the differences between the LIVE policy and restored policy.
*
*
*
* This is a convenience which creates an instance of the {@link RestoreCoreNetworkPolicyVersionRequest.Builder}
* avoiding the need to create one manually via {@link RestoreCoreNetworkPolicyVersionRequest#builder()}
*
*
* @param restoreCoreNetworkPolicyVersionRequest
* A {@link Consumer} that will call methods on {@link RestoreCoreNetworkPolicyVersionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the RestoreCoreNetworkPolicyVersion operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.RestoreCoreNetworkPolicyVersion
* @see AWS API Documentation
*/
default CompletableFuture restoreCoreNetworkPolicyVersion(
Consumer restoreCoreNetworkPolicyVersionRequest) {
return restoreCoreNetworkPolicyVersion(RestoreCoreNetworkPolicyVersionRequest.builder()
.applyMutation(restoreCoreNetworkPolicyVersionRequest).build());
}
/**
*
* Starts analyzing the routing path between the specified source and destination. For more information, see Route Analyzer.
*
*
* @param startRouteAnalysisRequest
* @return A Java Future containing the result of the StartRouteAnalysis operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.StartRouteAnalysis
* @see AWS API Documentation
*/
default CompletableFuture startRouteAnalysis(StartRouteAnalysisRequest startRouteAnalysisRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts analyzing the routing path between the specified source and destination. For more information, see Route Analyzer.
*
*
*
* This is a convenience which creates an instance of the {@link StartRouteAnalysisRequest.Builder} avoiding the
* need to create one manually via {@link StartRouteAnalysisRequest#builder()}
*
*
* @param startRouteAnalysisRequest
* A {@link Consumer} that will call methods on {@link StartRouteAnalysisRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartRouteAnalysis operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.StartRouteAnalysis
* @see AWS API Documentation
*/
default CompletableFuture startRouteAnalysis(
Consumer startRouteAnalysisRequest) {
return startRouteAnalysis(StartRouteAnalysisRequest.builder().applyMutation(startRouteAnalysisRequest).build());
}
/**
*
* Tags a specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Tags a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates the information for an existing connection. To remove information for any of the parameters, specify an
* empty string.
*
*
* @param updateConnectionRequest
* @return A Java Future containing the result of the UpdateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateConnection
* @see AWS API Documentation
*/
default CompletableFuture updateConnection(UpdateConnectionRequest updateConnectionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the information for an existing connection. To remove information for any of the parameters, specify an
* empty string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateConnectionRequest.Builder} avoiding the need
* to create one manually via {@link UpdateConnectionRequest#builder()}
*
*
* @param updateConnectionRequest
* A {@link Consumer} that will call methods on {@link UpdateConnectionRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateConnection
* @see AWS API Documentation
*/
default CompletableFuture updateConnection(
Consumer updateConnectionRequest) {
return updateConnection(UpdateConnectionRequest.builder().applyMutation(updateConnectionRequest).build());
}
/**
*
* Updates the description of a core network.
*
*
* @param updateCoreNetworkRequest
* @return A Java Future containing the result of the UpdateCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateCoreNetwork(UpdateCoreNetworkRequest updateCoreNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the description of a core network.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateCoreNetworkRequest.Builder} avoiding the need
* to create one manually via {@link UpdateCoreNetworkRequest#builder()}
*
*
* @param updateCoreNetworkRequest
* A {@link Consumer} that will call methods on {@link UpdateCoreNetworkRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateCoreNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateCoreNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateCoreNetwork(
Consumer updateCoreNetworkRequest) {
return updateCoreNetwork(UpdateCoreNetworkRequest.builder().applyMutation(updateCoreNetworkRequest).build());
}
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateDeviceRequest
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture updateDevice(UpdateDeviceRequest updateDeviceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details for an existing device. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDeviceRequest.Builder} avoiding the need to
* create one manually via {@link UpdateDeviceRequest#builder()}
*
*
* @param updateDeviceRequest
* A {@link Consumer} that will call methods on {@link UpdateDeviceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateDevice operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateDevice
* @see AWS
* API Documentation
*/
default CompletableFuture updateDevice(Consumer updateDeviceRequest) {
return updateDevice(UpdateDeviceRequest.builder().applyMutation(updateDeviceRequest).build());
}
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
* @param updateGlobalNetworkRequest
* @return A Java Future containing the result of the UpdateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateGlobalNetwork(
UpdateGlobalNetworkRequest updateGlobalNetworkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an existing global network. To remove information for any of the parameters, specify an empty string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateGlobalNetworkRequest.Builder} avoiding the
* need to create one manually via {@link UpdateGlobalNetworkRequest#builder()}
*
*
* @param updateGlobalNetworkRequest
* A {@link Consumer} that will call methods on {@link UpdateGlobalNetworkRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateGlobalNetwork operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateGlobalNetwork
* @see AWS API Documentation
*/
default CompletableFuture updateGlobalNetwork(
Consumer updateGlobalNetworkRequest) {
return updateGlobalNetwork(UpdateGlobalNetworkRequest.builder().applyMutation(updateGlobalNetworkRequest).build());
}
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateLinkRequest
* @return A Java Future containing the result of the UpdateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateLink
* @see AWS API
* Documentation
*/
default CompletableFuture updateLink(UpdateLinkRequest updateLinkRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the details for an existing link. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLinkRequest.Builder} avoiding the need to
* create one manually via {@link UpdateLinkRequest#builder()}
*
*
* @param updateLinkRequest
* A {@link Consumer} that will call methods on {@link UpdateLinkRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateLink operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - ServiceQuotaExceededException A service limit was exceeded.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateLink
* @see AWS API
* Documentation
*/
default CompletableFuture updateLink(Consumer updateLinkRequest) {
return updateLink(UpdateLinkRequest.builder().applyMutation(updateLinkRequest).build());
}
/**
*
* Updates the resource metadata for the specified global network.
*
*
* @param updateNetworkResourceMetadataRequest
* @return A Java Future containing the result of the UpdateNetworkResourceMetadata operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateNetworkResourceMetadata
* @see AWS API Documentation
*/
default CompletableFuture updateNetworkResourceMetadata(
UpdateNetworkResourceMetadataRequest updateNetworkResourceMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the resource metadata for the specified global network.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateNetworkResourceMetadataRequest.Builder}
* avoiding the need to create one manually via {@link UpdateNetworkResourceMetadataRequest#builder()}
*
*
* @param updateNetworkResourceMetadataRequest
* A {@link Consumer} that will call methods on {@link UpdateNetworkResourceMetadataRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateNetworkResourceMetadata operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateNetworkResourceMetadata
* @see AWS API Documentation
*/
default CompletableFuture updateNetworkResourceMetadata(
Consumer updateNetworkResourceMetadataRequest) {
return updateNetworkResourceMetadata(UpdateNetworkResourceMetadataRequest.builder()
.applyMutation(updateNetworkResourceMetadataRequest).build());
}
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
* @param updateSiteRequest
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(UpdateSiteRequest updateSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the information for an existing site. To remove information for any of the parameters, specify an empty
* string.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSiteRequest.Builder} avoiding the need to
* create one manually via {@link UpdateSiteRequest#builder()}
*
*
* @param updateSiteRequest
* A {@link Consumer} that will call methods on {@link UpdateSiteRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(Consumer updateSiteRequest) {
return updateSite(UpdateSiteRequest.builder().applyMutation(updateSiteRequest).build());
}
/**
*
* Updates a VPC attachment.
*
*
* @param updateVpcAttachmentRequest
* @return A Java Future containing the result of the UpdateVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture updateVpcAttachment(
UpdateVpcAttachmentRequest updateVpcAttachmentRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a VPC attachment.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVpcAttachmentRequest.Builder} avoiding the
* need to create one manually via {@link UpdateVpcAttachmentRequest#builder()}
*
*
* @param updateVpcAttachmentRequest
* A {@link Consumer} that will call methods on {@link UpdateVpcAttachmentRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateVpcAttachment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException The input fails to satisfy the constraints.
* - AccessDeniedException You do not have sufficient access to perform this action.
* - ResourceNotFoundException The specified resource could not be found.
* - ConflictException There was a conflict processing the request. Updating or deleting the resource can
* cause an inconsistent state.
* - ThrottlingException The request was denied due to request throttling.
* - InternalServerException The request has failed due to an internal error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - NetworkManagerException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample NetworkManagerAsyncClient.UpdateVpcAttachment
* @see AWS API Documentation
*/
default CompletableFuture updateVpcAttachment(
Consumer updateVpcAttachmentRequest) {
return updateVpcAttachment(UpdateVpcAttachmentRequest.builder().applyMutation(updateVpcAttachmentRequest).build());
}
}