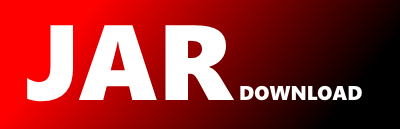
software.amazon.awssdk.services.networkmanager.model.RouteAnalysis Maven / Gradle / Ivy
Show all versions of networkmanager Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.networkmanager.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a route analysis.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class RouteAnalysis implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField GLOBAL_NETWORK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GlobalNetworkId").getter(getter(RouteAnalysis::globalNetworkId))
.setter(setter(Builder::globalNetworkId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GlobalNetworkId").build()).build();
private static final SdkField OWNER_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OwnerAccountId").getter(getter(RouteAnalysis::ownerAccountId)).setter(setter(Builder::ownerAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OwnerAccountId").build()).build();
private static final SdkField ROUTE_ANALYSIS_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RouteAnalysisId").getter(getter(RouteAnalysis::routeAnalysisId))
.setter(setter(Builder::routeAnalysisId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RouteAnalysisId").build()).build();
private static final SdkField START_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTimestamp").getter(getter(RouteAnalysis::startTimestamp)).setter(setter(Builder::startTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTimestamp").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(RouteAnalysis::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField SOURCE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Source")
.getter(getter(RouteAnalysis::source)).setter(setter(Builder::source))
.constructor(RouteAnalysisEndpointOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Source").build()).build();
private static final SdkField DESTINATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Destination")
.getter(getter(RouteAnalysis::destination)).setter(setter(Builder::destination))
.constructor(RouteAnalysisEndpointOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Destination").build()).build();
private static final SdkField INCLUDE_RETURN_PATH_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeReturnPath").getter(getter(RouteAnalysis::includeReturnPath))
.setter(setter(Builder::includeReturnPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeReturnPath").build()).build();
private static final SdkField USE_MIDDLEBOXES_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("UseMiddleboxes").getter(getter(RouteAnalysis::useMiddleboxes)).setter(setter(Builder::useMiddleboxes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UseMiddleboxes").build()).build();
private static final SdkField FORWARD_PATH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ForwardPath")
.getter(getter(RouteAnalysis::forwardPath)).setter(setter(Builder::forwardPath))
.constructor(RouteAnalysisPath::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ForwardPath").build()).build();
private static final SdkField RETURN_PATH_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ReturnPath")
.getter(getter(RouteAnalysis::returnPath)).setter(setter(Builder::returnPath))
.constructor(RouteAnalysisPath::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReturnPath").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(GLOBAL_NETWORK_ID_FIELD,
OWNER_ACCOUNT_ID_FIELD, ROUTE_ANALYSIS_ID_FIELD, START_TIMESTAMP_FIELD, STATUS_FIELD, SOURCE_FIELD,
DESTINATION_FIELD, INCLUDE_RETURN_PATH_FIELD, USE_MIDDLEBOXES_FIELD, FORWARD_PATH_FIELD, RETURN_PATH_FIELD));
private static final long serialVersionUID = 1L;
private final String globalNetworkId;
private final String ownerAccountId;
private final String routeAnalysisId;
private final Instant startTimestamp;
private final String status;
private final RouteAnalysisEndpointOptions source;
private final RouteAnalysisEndpointOptions destination;
private final Boolean includeReturnPath;
private final Boolean useMiddleboxes;
private final RouteAnalysisPath forwardPath;
private final RouteAnalysisPath returnPath;
private RouteAnalysis(BuilderImpl builder) {
this.globalNetworkId = builder.globalNetworkId;
this.ownerAccountId = builder.ownerAccountId;
this.routeAnalysisId = builder.routeAnalysisId;
this.startTimestamp = builder.startTimestamp;
this.status = builder.status;
this.source = builder.source;
this.destination = builder.destination;
this.includeReturnPath = builder.includeReturnPath;
this.useMiddleboxes = builder.useMiddleboxes;
this.forwardPath = builder.forwardPath;
this.returnPath = builder.returnPath;
}
/**
*
* The ID of the global network.
*
*
* @return The ID of the global network.
*/
public final String globalNetworkId() {
return globalNetworkId;
}
/**
*
* The ID of the AWS account that created the route analysis.
*
*
* @return The ID of the AWS account that created the route analysis.
*/
public final String ownerAccountId() {
return ownerAccountId;
}
/**
*
* The ID of the route analysis.
*
*
* @return The ID of the route analysis.
*/
public final String routeAnalysisId() {
return routeAnalysisId;
}
/**
*
* The time that the analysis started.
*
*
* @return The time that the analysis started.
*/
public final Instant startTimestamp() {
return startTimestamp;
}
/**
*
* The status of the route analysis.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RouteAnalysisStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the route analysis.
* @see RouteAnalysisStatus
*/
public final RouteAnalysisStatus status() {
return RouteAnalysisStatus.fromValue(status);
}
/**
*
* The status of the route analysis.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link RouteAnalysisStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return The status of the route analysis.
* @see RouteAnalysisStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The source.
*
*
* @return The source.
*/
public final RouteAnalysisEndpointOptions source() {
return source;
}
/**
*
* The destination.
*
*
* @return The destination.
*/
public final RouteAnalysisEndpointOptions destination() {
return destination;
}
/**
*
* Indicates whether to analyze the return path. The return path is not analyzed if the forward path analysis does
* not succeed.
*
*
* @return Indicates whether to analyze the return path. The return path is not analyzed if the forward path
* analysis does not succeed.
*/
public final Boolean includeReturnPath() {
return includeReturnPath;
}
/**
*
* Indicates whether to include the location of middlebox appliances in the route analysis.
*
*
* @return Indicates whether to include the location of middlebox appliances in the route analysis.
*/
public final Boolean useMiddleboxes() {
return useMiddleboxes;
}
/**
*
* The forward path.
*
*
* @return The forward path.
*/
public final RouteAnalysisPath forwardPath() {
return forwardPath;
}
/**
*
* The return path.
*
*
* @return The return path.
*/
public final RouteAnalysisPath returnPath() {
return returnPath;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(globalNetworkId());
hashCode = 31 * hashCode + Objects.hashCode(ownerAccountId());
hashCode = 31 * hashCode + Objects.hashCode(routeAnalysisId());
hashCode = 31 * hashCode + Objects.hashCode(startTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(source());
hashCode = 31 * hashCode + Objects.hashCode(destination());
hashCode = 31 * hashCode + Objects.hashCode(includeReturnPath());
hashCode = 31 * hashCode + Objects.hashCode(useMiddleboxes());
hashCode = 31 * hashCode + Objects.hashCode(forwardPath());
hashCode = 31 * hashCode + Objects.hashCode(returnPath());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof RouteAnalysis)) {
return false;
}
RouteAnalysis other = (RouteAnalysis) obj;
return Objects.equals(globalNetworkId(), other.globalNetworkId())
&& Objects.equals(ownerAccountId(), other.ownerAccountId())
&& Objects.equals(routeAnalysisId(), other.routeAnalysisId())
&& Objects.equals(startTimestamp(), other.startTimestamp())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(source(), other.source())
&& Objects.equals(destination(), other.destination())
&& Objects.equals(includeReturnPath(), other.includeReturnPath())
&& Objects.equals(useMiddleboxes(), other.useMiddleboxes()) && Objects.equals(forwardPath(), other.forwardPath())
&& Objects.equals(returnPath(), other.returnPath());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("RouteAnalysis").add("GlobalNetworkId", globalNetworkId())
.add("OwnerAccountId", ownerAccountId()).add("RouteAnalysisId", routeAnalysisId())
.add("StartTimestamp", startTimestamp()).add("Status", statusAsString()).add("Source", source())
.add("Destination", destination()).add("IncludeReturnPath", includeReturnPath())
.add("UseMiddleboxes", useMiddleboxes()).add("ForwardPath", forwardPath()).add("ReturnPath", returnPath())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "GlobalNetworkId":
return Optional.ofNullable(clazz.cast(globalNetworkId()));
case "OwnerAccountId":
return Optional.ofNullable(clazz.cast(ownerAccountId()));
case "RouteAnalysisId":
return Optional.ofNullable(clazz.cast(routeAnalysisId()));
case "StartTimestamp":
return Optional.ofNullable(clazz.cast(startTimestamp()));
case "Status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "Source":
return Optional.ofNullable(clazz.cast(source()));
case "Destination":
return Optional.ofNullable(clazz.cast(destination()));
case "IncludeReturnPath":
return Optional.ofNullable(clazz.cast(includeReturnPath()));
case "UseMiddleboxes":
return Optional.ofNullable(clazz.cast(useMiddleboxes()));
case "ForwardPath":
return Optional.ofNullable(clazz.cast(forwardPath()));
case "ReturnPath":
return Optional.ofNullable(clazz.cast(returnPath()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function