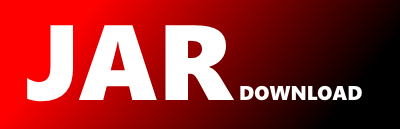
software.amazon.awssdk.services.nimble.model.LaunchProfileInitializationActiveDirectory Maven / Gradle / Ivy
Show all versions of nimble Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.nimble.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The launch profile initialization Active Directory contains information required for the launch profile to connect to
* the Active Directory.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LaunchProfileInitializationActiveDirectory implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField> COMPUTER_ATTRIBUTES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("computerAttributes")
.getter(getter(LaunchProfileInitializationActiveDirectory::computerAttributes))
.setter(setter(Builder::computerAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("computerAttributes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ActiveDirectoryComputerAttribute::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DIRECTORY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("directoryId").getter(getter(LaunchProfileInitializationActiveDirectory::directoryId))
.setter(setter(Builder::directoryId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directoryId").build()).build();
private static final SdkField DIRECTORY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("directoryName").getter(getter(LaunchProfileInitializationActiveDirectory::directoryName))
.setter(setter(Builder::directoryName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("directoryName").build()).build();
private static final SdkField> DNS_IP_ADDRESSES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("dnsIpAddresses")
.getter(getter(LaunchProfileInitializationActiveDirectory::dnsIpAddresses))
.setter(setter(Builder::dnsIpAddresses))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dnsIpAddresses").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField ORGANIZATIONAL_UNIT_DISTINGUISHED_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("organizationalUnitDistinguishedName")
.getter(getter(LaunchProfileInitializationActiveDirectory::organizationalUnitDistinguishedName))
.setter(setter(Builder::organizationalUnitDistinguishedName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("organizationalUnitDistinguishedName").build()).build();
private static final SdkField STUDIO_COMPONENT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("studioComponentId").getter(getter(LaunchProfileInitializationActiveDirectory::studioComponentId))
.setter(setter(Builder::studioComponentId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("studioComponentId").build()).build();
private static final SdkField STUDIO_COMPONENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("studioComponentName").getter(getter(LaunchProfileInitializationActiveDirectory::studioComponentName))
.setter(setter(Builder::studioComponentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("studioComponentName").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPUTER_ATTRIBUTES_FIELD,
DIRECTORY_ID_FIELD, DIRECTORY_NAME_FIELD, DNS_IP_ADDRESSES_FIELD, ORGANIZATIONAL_UNIT_DISTINGUISHED_NAME_FIELD,
STUDIO_COMPONENT_ID_FIELD, STUDIO_COMPONENT_NAME_FIELD));
private static final long serialVersionUID = 1L;
private final List computerAttributes;
private final String directoryId;
private final String directoryName;
private final List dnsIpAddresses;
private final String organizationalUnitDistinguishedName;
private final String studioComponentId;
private final String studioComponentName;
private LaunchProfileInitializationActiveDirectory(BuilderImpl builder) {
this.computerAttributes = builder.computerAttributes;
this.directoryId = builder.directoryId;
this.directoryName = builder.directoryName;
this.dnsIpAddresses = builder.dnsIpAddresses;
this.organizationalUnitDistinguishedName = builder.organizationalUnitDistinguishedName;
this.studioComponentId = builder.studioComponentId;
this.studioComponentName = builder.studioComponentName;
}
/**
* For responses, this returns true if the service returned a value for the ComputerAttributes property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasComputerAttributes() {
return computerAttributes != null && !(computerAttributes instanceof SdkAutoConstructList);
}
/**
*
* A collection of custom attributes for an Active Directory computer.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasComputerAttributes} method.
*
*
* @return A collection of custom attributes for an Active Directory computer.
*/
public final List computerAttributes() {
return computerAttributes;
}
/**
*
* The directory ID of the Directory Service for Microsoft Active Directory to access using this launch profile.
*
*
* @return The directory ID of the Directory Service for Microsoft Active Directory to access using this launch
* profile.
*/
public final String directoryId() {
return directoryId;
}
/**
*
* The directory name.
*
*
* @return The directory name.
*/
public final String directoryName() {
return directoryName;
}
/**
* For responses, this returns true if the service returned a value for the DnsIpAddresses property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDnsIpAddresses() {
return dnsIpAddresses != null && !(dnsIpAddresses instanceof SdkAutoConstructList);
}
/**
*
* The DNS IP address.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDnsIpAddresses} method.
*
*
* @return The DNS IP address.
*/
public final List dnsIpAddresses() {
return dnsIpAddresses;
}
/**
*
* The name for the organizational unit distinguished name.
*
*
* @return The name for the organizational unit distinguished name.
*/
public final String organizationalUnitDistinguishedName() {
return organizationalUnitDistinguishedName;
}
/**
*
* The unique identifier for a studio component resource.
*
*
* @return The unique identifier for a studio component resource.
*/
public final String studioComponentId() {
return studioComponentId;
}
/**
*
* The name for the studio component.
*
*
* @return The name for the studio component.
*/
public final String studioComponentName() {
return studioComponentName;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(hasComputerAttributes() ? computerAttributes() : null);
hashCode = 31 * hashCode + Objects.hashCode(directoryId());
hashCode = 31 * hashCode + Objects.hashCode(directoryName());
hashCode = 31 * hashCode + Objects.hashCode(hasDnsIpAddresses() ? dnsIpAddresses() : null);
hashCode = 31 * hashCode + Objects.hashCode(organizationalUnitDistinguishedName());
hashCode = 31 * hashCode + Objects.hashCode(studioComponentId());
hashCode = 31 * hashCode + Objects.hashCode(studioComponentName());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LaunchProfileInitializationActiveDirectory)) {
return false;
}
LaunchProfileInitializationActiveDirectory other = (LaunchProfileInitializationActiveDirectory) obj;
return hasComputerAttributes() == other.hasComputerAttributes()
&& Objects.equals(computerAttributes(), other.computerAttributes())
&& Objects.equals(directoryId(), other.directoryId()) && Objects.equals(directoryName(), other.directoryName())
&& hasDnsIpAddresses() == other.hasDnsIpAddresses() && Objects.equals(dnsIpAddresses(), other.dnsIpAddresses())
&& Objects.equals(organizationalUnitDistinguishedName(), other.organizationalUnitDistinguishedName())
&& Objects.equals(studioComponentId(), other.studioComponentId())
&& Objects.equals(studioComponentName(), other.studioComponentName());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LaunchProfileInitializationActiveDirectory")
.add("ComputerAttributes", computerAttributes() == null ? null : "*** Sensitive Data Redacted ***")
.add("DirectoryId", directoryId()).add("DirectoryName", directoryName())
.add("DnsIpAddresses", hasDnsIpAddresses() ? dnsIpAddresses() : null)
.add("OrganizationalUnitDistinguishedName", organizationalUnitDistinguishedName())
.add("StudioComponentId", studioComponentId())
.add("StudioComponentName", studioComponentName() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "computerAttributes":
return Optional.ofNullable(clazz.cast(computerAttributes()));
case "directoryId":
return Optional.ofNullable(clazz.cast(directoryId()));
case "directoryName":
return Optional.ofNullable(clazz.cast(directoryName()));
case "dnsIpAddresses":
return Optional.ofNullable(clazz.cast(dnsIpAddresses()));
case "organizationalUnitDistinguishedName":
return Optional.ofNullable(clazz.cast(organizationalUnitDistinguishedName()));
case "studioComponentId":
return Optional.ofNullable(clazz.cast(studioComponentId()));
case "studioComponentName":
return Optional.ofNullable(clazz.cast(studioComponentName()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function