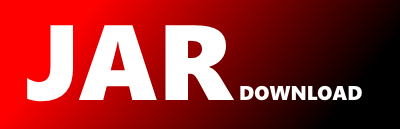
software.amazon.awssdk.services.opensearch.model.DomainEndpointOptions Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Options to configure the endpoint for the domain.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DomainEndpointOptions implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField ENFORCE_HTTPS_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EnforceHTTPS").getter(getter(DomainEndpointOptions::enforceHTTPS)).setter(setter(Builder::enforceHTTPS))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EnforceHTTPS").build()).build();
private static final SdkField TLS_SECURITY_POLICY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TLSSecurityPolicy").getter(getter(DomainEndpointOptions::tlsSecurityPolicyAsString))
.setter(setter(Builder::tlsSecurityPolicy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TLSSecurityPolicy").build()).build();
private static final SdkField CUSTOM_ENDPOINT_ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("CustomEndpointEnabled").getter(getter(DomainEndpointOptions::customEndpointEnabled))
.setter(setter(Builder::customEndpointEnabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomEndpointEnabled").build())
.build();
private static final SdkField CUSTOM_ENDPOINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomEndpoint").getter(getter(DomainEndpointOptions::customEndpoint))
.setter(setter(Builder::customEndpoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomEndpoint").build()).build();
private static final SdkField CUSTOM_ENDPOINT_CERTIFICATE_ARN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CustomEndpointCertificateArn")
.getter(getter(DomainEndpointOptions::customEndpointCertificateArn))
.setter(setter(Builder::customEndpointCertificateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomEndpointCertificateArn")
.build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ENFORCE_HTTPS_FIELD,
TLS_SECURITY_POLICY_FIELD, CUSTOM_ENDPOINT_ENABLED_FIELD, CUSTOM_ENDPOINT_FIELD,
CUSTOM_ENDPOINT_CERTIFICATE_ARN_FIELD));
private static final long serialVersionUID = 1L;
private final Boolean enforceHTTPS;
private final String tlsSecurityPolicy;
private final Boolean customEndpointEnabled;
private final String customEndpoint;
private final String customEndpointCertificateArn;
private DomainEndpointOptions(BuilderImpl builder) {
this.enforceHTTPS = builder.enforceHTTPS;
this.tlsSecurityPolicy = builder.tlsSecurityPolicy;
this.customEndpointEnabled = builder.customEndpointEnabled;
this.customEndpoint = builder.customEndpoint;
this.customEndpointCertificateArn = builder.customEndpointCertificateArn;
}
/**
*
* Whether only HTTPS endpoint should be enabled for the domain.
*
*
* @return Whether only HTTPS endpoint should be enabled for the domain.
*/
public final Boolean enforceHTTPS() {
return enforceHTTPS;
}
/**
*
* Specify the TLS security policy to apply to the HTTPS endpoint of the domain.
* Can be one of the following values:
*
* - Policy-Min-TLS-1-0-2019-07: TLS security policy which supports TLSv1.0 and higher.
* - Policy-Min-TLS-1-2-2019-07: TLS security policy which supports only TLSv1.2
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tlsSecurityPolicy}
* will return {@link TLSSecurityPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #tlsSecurityPolicyAsString}.
*
*
* @return Specify the TLS security policy to apply to the HTTPS endpoint of the domain.
* Can be one of the following values:
*
* - Policy-Min-TLS-1-0-2019-07: TLS security policy which supports TLSv1.0 and higher.
* - Policy-Min-TLS-1-2-2019-07: TLS security policy which supports only TLSv1.2
*
* @see TLSSecurityPolicy
*/
public final TLSSecurityPolicy tlsSecurityPolicy() {
return TLSSecurityPolicy.fromValue(tlsSecurityPolicy);
}
/**
*
* Specify the TLS security policy to apply to the HTTPS endpoint of the domain.
* Can be one of the following values:
*
* - Policy-Min-TLS-1-0-2019-07: TLS security policy which supports TLSv1.0 and higher.
* - Policy-Min-TLS-1-2-2019-07: TLS security policy which supports only TLSv1.2
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #tlsSecurityPolicy}
* will return {@link TLSSecurityPolicy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #tlsSecurityPolicyAsString}.
*
*
* @return Specify the TLS security policy to apply to the HTTPS endpoint of the domain.
* Can be one of the following values:
*
* - Policy-Min-TLS-1-0-2019-07: TLS security policy which supports TLSv1.0 and higher.
* - Policy-Min-TLS-1-2-2019-07: TLS security policy which supports only TLSv1.2
*
* @see TLSSecurityPolicy
*/
public final String tlsSecurityPolicyAsString() {
return tlsSecurityPolicy;
}
/**
*
* Whether to enable a custom endpoint for the domain.
*
*
* @return Whether to enable a custom endpoint for the domain.
*/
public final Boolean customEndpointEnabled() {
return customEndpointEnabled;
}
/**
*
* The fully qualified domain for your custom endpoint.
*
*
* @return The fully qualified domain for your custom endpoint.
*/
public final String customEndpoint() {
return customEndpoint;
}
/**
*
* The ACM certificate ARN for your custom endpoint.
*
*
* @return The ACM certificate ARN for your custom endpoint.
*/
public final String customEndpointCertificateArn() {
return customEndpointCertificateArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(enforceHTTPS());
hashCode = 31 * hashCode + Objects.hashCode(tlsSecurityPolicyAsString());
hashCode = 31 * hashCode + Objects.hashCode(customEndpointEnabled());
hashCode = 31 * hashCode + Objects.hashCode(customEndpoint());
hashCode = 31 * hashCode + Objects.hashCode(customEndpointCertificateArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DomainEndpointOptions)) {
return false;
}
DomainEndpointOptions other = (DomainEndpointOptions) obj;
return Objects.equals(enforceHTTPS(), other.enforceHTTPS())
&& Objects.equals(tlsSecurityPolicyAsString(), other.tlsSecurityPolicyAsString())
&& Objects.equals(customEndpointEnabled(), other.customEndpointEnabled())
&& Objects.equals(customEndpoint(), other.customEndpoint())
&& Objects.equals(customEndpointCertificateArn(), other.customEndpointCertificateArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DomainEndpointOptions").add("EnforceHTTPS", enforceHTTPS())
.add("TLSSecurityPolicy", tlsSecurityPolicyAsString()).add("CustomEndpointEnabled", customEndpointEnabled())
.add("CustomEndpoint", customEndpoint()).add("CustomEndpointCertificateArn", customEndpointCertificateArn())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "EnforceHTTPS":
return Optional.ofNullable(clazz.cast(enforceHTTPS()));
case "TLSSecurityPolicy":
return Optional.ofNullable(clazz.cast(tlsSecurityPolicyAsString()));
case "CustomEndpointEnabled":
return Optional.ofNullable(clazz.cast(customEndpointEnabled()));
case "CustomEndpoint":
return Optional.ofNullable(clazz.cast(customEndpoint()));
case "CustomEndpointCertificateArn":
return Optional.ofNullable(clazz.cast(customEndpointCertificateArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function