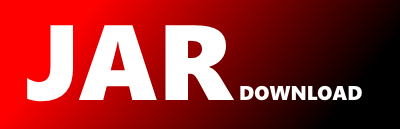
software.amazon.awssdk.services.opensearch.model.UpgradeHistory Maven / Gradle / Ivy
Show all versions of opensearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch.model;
import java.beans.Transient;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* History of the last 10 upgrades and upgrade eligibility checks.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpgradeHistory implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UPGRADE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpgradeName").getter(getter(UpgradeHistory::upgradeName)).setter(setter(Builder::upgradeName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeName").build()).build();
private static final SdkField START_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("StartTimestamp").getter(getter(UpgradeHistory::startTimestamp)).setter(setter(Builder::startTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartTimestamp").build()).build();
private static final SdkField UPGRADE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpgradeStatus").getter(getter(UpgradeHistory::upgradeStatusAsString))
.setter(setter(Builder::upgradeStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeStatus").build()).build();
private static final SdkField> STEPS_LIST_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StepsList")
.getter(getter(UpgradeHistory::stepsList))
.setter(setter(Builder::stepsList))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StepsList").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(UpgradeStepItem::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UPGRADE_NAME_FIELD,
START_TIMESTAMP_FIELD, UPGRADE_STATUS_FIELD, STEPS_LIST_FIELD));
private static final long serialVersionUID = 1L;
private final String upgradeName;
private final Instant startTimestamp;
private final String upgradeStatus;
private final List stepsList;
private UpgradeHistory(BuilderImpl builder) {
this.upgradeName = builder.upgradeName;
this.startTimestamp = builder.startTimestamp;
this.upgradeStatus = builder.upgradeStatus;
this.stepsList = builder.stepsList;
}
/**
*
* A string that briefly describes the upgrade.
*
*
* @return A string that briefly describes the upgrade.
*/
public final String upgradeName() {
return upgradeName;
}
/**
*
* UTC timestamp at which the upgrade API call was made in "yyyy-MM-ddTHH:mm:ssZ" format.
*
*
* @return UTC timestamp at which the upgrade API call was made in "yyyy-MM-ddTHH:mm:ssZ" format.
*/
public final Instant startTimestamp() {
return startTimestamp;
}
/**
*
* The current status of the upgrade. The status can take one of the following values:
*
* - In Progress
* - Succeeded
* - Succeeded with Issues
* - Failed
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStatus}
* will return {@link UpgradeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStatusAsString}.
*
*
* @return The current status of the upgrade. The status can take one of the following values:
*
* - In Progress
* - Succeeded
* - Succeeded with Issues
* - Failed
*
* @see UpgradeStatus
*/
public final UpgradeStatus upgradeStatus() {
return UpgradeStatus.fromValue(upgradeStatus);
}
/**
*
* The current status of the upgrade. The status can take one of the following values:
*
* - In Progress
* - Succeeded
* - Succeeded with Issues
* - Failed
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStatus}
* will return {@link UpgradeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStatusAsString}.
*
*
* @return The current status of the upgrade. The status can take one of the following values:
*
* - In Progress
* - Succeeded
* - Succeeded with Issues
* - Failed
*
* @see UpgradeStatus
*/
public final String upgradeStatusAsString() {
return upgradeStatus;
}
/**
* For responses, this returns true if the service returned a value for the StepsList property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasStepsList() {
return stepsList != null && !(stepsList instanceof SdkAutoConstructList);
}
/**
*
* A list of UpgradeStepItem
s representing information about each step performed as part of a
* specific upgrade or upgrade eligibility check.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStepsList} method.
*
*
* @return A list of UpgradeStepItem
s representing information about each step performed as
* part of a specific upgrade or upgrade eligibility check.
*/
public final List stepsList() {
return stepsList;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(upgradeName());
hashCode = 31 * hashCode + Objects.hashCode(startTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(upgradeStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasStepsList() ? stepsList() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpgradeHistory)) {
return false;
}
UpgradeHistory other = (UpgradeHistory) obj;
return Objects.equals(upgradeName(), other.upgradeName()) && Objects.equals(startTimestamp(), other.startTimestamp())
&& Objects.equals(upgradeStatusAsString(), other.upgradeStatusAsString())
&& hasStepsList() == other.hasStepsList() && Objects.equals(stepsList(), other.stepsList());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpgradeHistory").add("UpgradeName", upgradeName()).add("StartTimestamp", startTimestamp())
.add("UpgradeStatus", upgradeStatusAsString()).add("StepsList", hasStepsList() ? stepsList() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UpgradeName":
return Optional.ofNullable(clazz.cast(upgradeName()));
case "StartTimestamp":
return Optional.ofNullable(clazz.cast(startTimestamp()));
case "UpgradeStatus":
return Optional.ofNullable(clazz.cast(upgradeStatusAsString()));
case "StepsList":
return Optional.ofNullable(clazz.cast(stepsList()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function