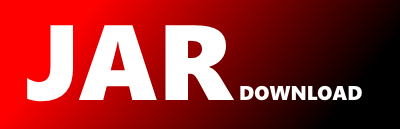
software.amazon.awssdk.services.opensearch.model.UpgradeStepItem Maven / Gradle / Ivy
Show all versions of opensearch Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Represents a single step of an upgrade or upgrade eligibility check workflow.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpgradeStepItem implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField UPGRADE_STEP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpgradeStep").getter(getter(UpgradeStepItem::upgradeStepAsString)).setter(setter(Builder::upgradeStep))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeStep").build()).build();
private static final SdkField UPGRADE_STEP_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpgradeStepStatus").getter(getter(UpgradeStepItem::upgradeStepStatusAsString))
.setter(setter(Builder::upgradeStepStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpgradeStepStatus").build()).build();
private static final SdkField> ISSUES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Issues")
.getter(getter(UpgradeStepItem::issues))
.setter(setter(Builder::issues))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Issues").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField PROGRESS_PERCENT_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("ProgressPercent").getter(getter(UpgradeStepItem::progressPercent))
.setter(setter(Builder::progressPercent))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ProgressPercent").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(UPGRADE_STEP_FIELD,
UPGRADE_STEP_STATUS_FIELD, ISSUES_FIELD, PROGRESS_PERCENT_FIELD));
private static final long serialVersionUID = 1L;
private final String upgradeStep;
private final String upgradeStepStatus;
private final List issues;
private final Double progressPercent;
private UpgradeStepItem(BuilderImpl builder) {
this.upgradeStep = builder.upgradeStep;
this.upgradeStepStatus = builder.upgradeStepStatus;
this.issues = builder.issues;
this.progressPercent = builder.progressPercent;
}
/**
*
* One of three steps that an upgrade or upgrade eligibility check goes through:
*
*
* -
*
* PreUpgradeCheck
*
*
* -
*
* Snapshot
*
*
* -
*
* Upgrade
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStep} will
* return {@link UpgradeStep#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStepAsString}.
*
*
* @return One of three steps that an upgrade or upgrade eligibility check goes through:
*
* -
*
* PreUpgradeCheck
*
*
* -
*
* Snapshot
*
*
* -
*
* Upgrade
*
*
* @see UpgradeStep
*/
public final UpgradeStep upgradeStep() {
return UpgradeStep.fromValue(upgradeStep);
}
/**
*
* One of three steps that an upgrade or upgrade eligibility check goes through:
*
*
* -
*
* PreUpgradeCheck
*
*
* -
*
* Snapshot
*
*
* -
*
* Upgrade
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStep} will
* return {@link UpgradeStep#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStepAsString}.
*
*
* @return One of three steps that an upgrade or upgrade eligibility check goes through:
*
* -
*
* PreUpgradeCheck
*
*
* -
*
* Snapshot
*
*
* -
*
* Upgrade
*
*
* @see UpgradeStep
*/
public final String upgradeStepAsString() {
return upgradeStep;
}
/**
*
* The current status of the upgrade. The status can take one of the following values:
*
*
* -
*
* In Progress
*
*
* -
*
* Succeeded
*
*
* -
*
* Succeeded with Issues
*
*
* -
*
* Failed
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStepStatus}
* will return {@link UpgradeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStepStatusAsString}.
*
*
* @return The current status of the upgrade. The status can take one of the following values:
*
* -
*
* In Progress
*
*
* -
*
* Succeeded
*
*
* -
*
* Succeeded with Issues
*
*
* -
*
* Failed
*
*
* @see UpgradeStatus
*/
public final UpgradeStatus upgradeStepStatus() {
return UpgradeStatus.fromValue(upgradeStepStatus);
}
/**
*
* The current status of the upgrade. The status can take one of the following values:
*
*
* -
*
* In Progress
*
*
* -
*
* Succeeded
*
*
* -
*
* Succeeded with Issues
*
*
* -
*
* Failed
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #upgradeStepStatus}
* will return {@link UpgradeStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #upgradeStepStatusAsString}.
*
*
* @return The current status of the upgrade. The status can take one of the following values:
*
* -
*
* In Progress
*
*
* -
*
* Succeeded
*
*
* -
*
* Succeeded with Issues
*
*
* -
*
* Failed
*
*
* @see UpgradeStatus
*/
public final String upgradeStepStatusAsString() {
return upgradeStepStatus;
}
/**
* For responses, this returns true if the service returned a value for the Issues property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasIssues() {
return issues != null && !(issues instanceof SdkAutoConstructList);
}
/**
*
* A list of strings containing detailed information about the errors encountered in a particular step.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasIssues} method.
*
*
* @return A list of strings containing detailed information about the errors encountered in a particular step.
*/
public final List issues() {
return issues;
}
/**
*
* The floating point value representing the progress percentage of a particular step.
*
*
* @return The floating point value representing the progress percentage of a particular step.
*/
public final Double progressPercent() {
return progressPercent;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(upgradeStepAsString());
hashCode = 31 * hashCode + Objects.hashCode(upgradeStepStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasIssues() ? issues() : null);
hashCode = 31 * hashCode + Objects.hashCode(progressPercent());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpgradeStepItem)) {
return false;
}
UpgradeStepItem other = (UpgradeStepItem) obj;
return Objects.equals(upgradeStepAsString(), other.upgradeStepAsString())
&& Objects.equals(upgradeStepStatusAsString(), other.upgradeStepStatusAsString())
&& hasIssues() == other.hasIssues() && Objects.equals(issues(), other.issues())
&& Objects.equals(progressPercent(), other.progressPercent());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpgradeStepItem").add("UpgradeStep", upgradeStepAsString())
.add("UpgradeStepStatus", upgradeStepStatusAsString()).add("Issues", hasIssues() ? issues() : null)
.add("ProgressPercent", progressPercent()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "UpgradeStep":
return Optional.ofNullable(clazz.cast(upgradeStepAsString()));
case "UpgradeStepStatus":
return Optional.ofNullable(clazz.cast(upgradeStepStatusAsString()));
case "Issues":
return Optional.ofNullable(clazz.cast(issues()));
case "ProgressPercent":
return Optional.ofNullable(clazz.cast(progressPercent()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function