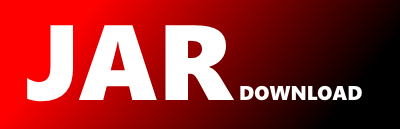
software.amazon.awssdk.services.opensearch.OpenSearchClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.opensearch.model.AcceptInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.AcceptInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.AccessDeniedException;
import software.amazon.awssdk.services.opensearch.model.AddTagsRequest;
import software.amazon.awssdk.services.opensearch.model.AddTagsResponse;
import software.amazon.awssdk.services.opensearch.model.AssociatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.AssociatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.AuthorizeVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.AuthorizeVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.BaseException;
import software.amazon.awssdk.services.opensearch.model.CancelServiceSoftwareUpdateRequest;
import software.amazon.awssdk.services.opensearch.model.CancelServiceSoftwareUpdateResponse;
import software.amazon.awssdk.services.opensearch.model.ConflictException;
import software.amazon.awssdk.services.opensearch.model.CreateDomainRequest;
import software.amazon.awssdk.services.opensearch.model.CreateDomainResponse;
import software.amazon.awssdk.services.opensearch.model.CreateOutboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.CreateOutboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.CreatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.CreatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.CreateVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.CreateVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteDomainRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteDomainResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteOutboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteOutboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.DeletePackageRequest;
import software.amazon.awssdk.services.opensearch.model.DeletePackageResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainChangeProgressRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainChangeProgressResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainConfigRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainConfigResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDryRunProgressRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDryRunProgressResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeInstanceTypeLimitsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeInstanceTypeLimitsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribePackagesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeVpcEndpointsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeVpcEndpointsResponse;
import software.amazon.awssdk.services.opensearch.model.DisabledOperationException;
import software.amazon.awssdk.services.opensearch.model.DissociatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.DissociatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.GetCompatibleVersionsRequest;
import software.amazon.awssdk.services.opensearch.model.GetCompatibleVersionsResponse;
import software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest;
import software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryResponse;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryResponse;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeStatusRequest;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeStatusResponse;
import software.amazon.awssdk.services.opensearch.model.InternalException;
import software.amazon.awssdk.services.opensearch.model.InvalidPaginationTokenException;
import software.amazon.awssdk.services.opensearch.model.InvalidTypeException;
import software.amazon.awssdk.services.opensearch.model.LimitExceededException;
import software.amazon.awssdk.services.opensearch.model.ListDomainNamesRequest;
import software.amazon.awssdk.services.opensearch.model.ListDomainNamesResponse;
import software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest;
import software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageResponse;
import software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest;
import software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsResponse;
import software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest;
import software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest;
import software.amazon.awssdk.services.opensearch.model.ListScheduledActionsResponse;
import software.amazon.awssdk.services.opensearch.model.ListTagsRequest;
import software.amazon.awssdk.services.opensearch.model.ListTagsResponse;
import software.amazon.awssdk.services.opensearch.model.ListVersionsRequest;
import software.amazon.awssdk.services.opensearch.model.ListVersionsResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsForDomainRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsForDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsResponse;
import software.amazon.awssdk.services.opensearch.model.OpenSearchException;
import software.amazon.awssdk.services.opensearch.model.PurchaseReservedInstanceOfferingRequest;
import software.amazon.awssdk.services.opensearch.model.PurchaseReservedInstanceOfferingResponse;
import software.amazon.awssdk.services.opensearch.model.RejectInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.RejectInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.RemoveTagsRequest;
import software.amazon.awssdk.services.opensearch.model.RemoveTagsResponse;
import software.amazon.awssdk.services.opensearch.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.opensearch.model.ResourceNotFoundException;
import software.amazon.awssdk.services.opensearch.model.RevokeVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.RevokeVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.SlotNotAvailableException;
import software.amazon.awssdk.services.opensearch.model.StartServiceSoftwareUpdateRequest;
import software.amazon.awssdk.services.opensearch.model.StartServiceSoftwareUpdateResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateDomainConfigRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateDomainConfigResponse;
import software.amazon.awssdk.services.opensearch.model.UpdatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.UpdatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateScheduledActionRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateScheduledActionResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.UpgradeDomainRequest;
import software.amazon.awssdk.services.opensearch.model.UpgradeDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ValidationException;
import software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable;
import software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable;
import software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable;
import software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable;
import software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable;
import software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable;
import software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable;
import software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable;
import software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable;
import software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable;
import software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable;
import software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable;
import software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable;
/**
* Service client for accessing Amazon OpenSearch Service. This can be created using the static {@link #builder()}
* method.
*
*
* Use the Amazon OpenSearch Service configuration API to create, configure, and manage OpenSearch Service domains.
*
*
* For sample code that uses the configuration API, see the
* Amazon OpenSearch Service Developer Guide . The guide also contains sample code for
* sending signed HTTP requests to the OpenSearch APIs. The endpoint for configuration service requests is Region
* specific: es.region.amazonaws.com. For example, es.us-east-1.amazonaws.com. For a current list of supported
* Regions and endpoints, see Amazon
* Web Services service endpoints.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface OpenSearchClient extends SdkClient {
String SERVICE_NAME = "es";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "es";
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to accept an inbound cross-cluster search
* connection request. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param acceptInboundConnectionRequest
* Container for the parameters to the AcceptInboundConnection
operation.
* @return Result of the AcceptInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AcceptInboundConnection
*/
default AcceptInboundConnectionResponse acceptInboundConnection(AcceptInboundConnectionRequest acceptInboundConnectionRequest)
throws ResourceNotFoundException, LimitExceededException, DisabledOperationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to accept an inbound cross-cluster search
* connection request. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link AcceptInboundConnectionRequest.Builder} avoiding
* the need to create one manually via {@link AcceptInboundConnectionRequest#builder()}
*
*
* @param acceptInboundConnectionRequest
* A {@link Consumer} that will call methods on {@link AcceptInboundConnectionRequest.Builder} to create a
* request. Container for the parameters to the AcceptInboundConnection
operation.
* @return Result of the AcceptInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AcceptInboundConnection
*/
default AcceptInboundConnectionResponse acceptInboundConnection(
Consumer acceptInboundConnectionRequest) throws ResourceNotFoundException,
LimitExceededException, DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
return acceptInboundConnection(AcceptInboundConnectionRequest.builder().applyMutation(acceptInboundConnectionRequest)
.build());
}
/**
*
* Attaches tags to an existing Amazon OpenSearch Service domain. Tags are a set of case-sensitive key-value pairs.
* A domain can have up to 10 tags. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
operation. Specifies the tags to attach to the
* domain.
* @return Result of the AddTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AddTags
*/
default AddTagsResponse addTags(AddTagsRequest addTagsRequest) throws BaseException, LimitExceededException,
ValidationException, InternalException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches tags to an existing Amazon OpenSearch Service domain. Tags are a set of case-sensitive key-value pairs.
* A domain can have up to 10 tags. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
*
* This is a convenience which creates an instance of the {@link AddTagsRequest.Builder} avoiding the need to create
* one manually via {@link AddTagsRequest#builder()}
*
*
* @param addTagsRequest
* A {@link Consumer} that will call methods on {@link AddTagsRequest.Builder} to create a request. Container
* for the parameters to the AddTags
operation. Specifies the tags to attach to the domain.
* @return Result of the AddTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AddTags
*/
default AddTagsResponse addTags(Consumer addTagsRequest) throws BaseException,
LimitExceededException, ValidationException, InternalException, AwsServiceException, SdkClientException,
OpenSearchException {
return addTags(AddTagsRequest.builder().applyMutation(addTagsRequest).build());
}
/**
*
* Associates a package with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param associatePackageRequest
* Container for the request parameters to the AssociatePackage
operation.
* @return Result of the AssociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AssociatePackage
*/
default AssociatePackageResponse associatePackage(AssociatePackageRequest associatePackageRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, ConflictException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Associates a package with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link AssociatePackageRequest.Builder} avoiding the need
* to create one manually via {@link AssociatePackageRequest#builder()}
*
*
* @param associatePackageRequest
* A {@link Consumer} that will call methods on {@link AssociatePackageRequest.Builder} to create a request.
* Container for the request parameters to the AssociatePackage
operation.
* @return Result of the AssociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AssociatePackage
*/
default AssociatePackageResponse associatePackage(Consumer associatePackageRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ConflictException, AwsServiceException, SdkClientException, OpenSearchException {
return associatePackage(AssociatePackageRequest.builder().applyMutation(associatePackageRequest).build());
}
/**
*
* Provides access to an Amazon OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
* @param authorizeVpcEndpointAccessRequest
* @return Result of the AuthorizeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AuthorizeVpcEndpointAccess
*/
default AuthorizeVpcEndpointAccessResponse authorizeVpcEndpointAccess(
AuthorizeVpcEndpointAccessRequest authorizeVpcEndpointAccessRequest) throws ResourceNotFoundException,
DisabledOperationException, LimitExceededException, ValidationException, InternalException, BaseException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Provides access to an Amazon OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link AuthorizeVpcEndpointAccessRequest.Builder} avoiding
* the need to create one manually via {@link AuthorizeVpcEndpointAccessRequest#builder()}
*
*
* @param authorizeVpcEndpointAccessRequest
* A {@link Consumer} that will call methods on {@link AuthorizeVpcEndpointAccessRequest.Builder} to create a
* request.
* @return Result of the AuthorizeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.AuthorizeVpcEndpointAccess
*/
default AuthorizeVpcEndpointAccessResponse authorizeVpcEndpointAccess(
Consumer authorizeVpcEndpointAccessRequest)
throws ResourceNotFoundException, DisabledOperationException, LimitExceededException, ValidationException,
InternalException, BaseException, AwsServiceException, SdkClientException, OpenSearchException {
return authorizeVpcEndpointAccess(AuthorizeVpcEndpointAccessRequest.builder()
.applyMutation(authorizeVpcEndpointAccessRequest).build());
}
/**
*
* Cancels a scheduled service software update for an Amazon OpenSearch Service domain. You can only perform this
* operation before the AutomatedUpdateDate
and when the domain's UpdateStatus
is
* PENDING_UPDATE
. For more information, see Service
* software updates in Amazon OpenSearch Service.
*
*
* @param cancelServiceSoftwareUpdateRequest
* Container for the request parameters to cancel a service software update.
* @return Result of the CancelServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CancelServiceSoftwareUpdate
*/
default CancelServiceSoftwareUpdateResponse cancelServiceSoftwareUpdate(
CancelServiceSoftwareUpdateRequest cancelServiceSoftwareUpdateRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Cancels a scheduled service software update for an Amazon OpenSearch Service domain. You can only perform this
* operation before the AutomatedUpdateDate
and when the domain's UpdateStatus
is
* PENDING_UPDATE
. For more information, see Service
* software updates in Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link CancelServiceSoftwareUpdateRequest.Builder}
* avoiding the need to create one manually via {@link CancelServiceSoftwareUpdateRequest#builder()}
*
*
* @param cancelServiceSoftwareUpdateRequest
* A {@link Consumer} that will call methods on {@link CancelServiceSoftwareUpdateRequest.Builder} to create
* a request. Container for the request parameters to cancel a service software update.
* @return Result of the CancelServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CancelServiceSoftwareUpdate
*/
default CancelServiceSoftwareUpdateResponse cancelServiceSoftwareUpdate(
Consumer cancelServiceSoftwareUpdateRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return cancelServiceSoftwareUpdate(CancelServiceSoftwareUpdateRequest.builder()
.applyMutation(cancelServiceSoftwareUpdateRequest).build());
}
/**
*
* Creates an Amazon OpenSearch Service domain. For more information, see Creating and
* managing Amazon OpenSearch Service domains.
*
*
* @param createDomainRequest
* @return Result of the CreateDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateDomain
*/
default CreateDomainResponse createDomain(CreateDomainRequest createDomainRequest) throws BaseException,
DisabledOperationException, InternalException, InvalidTypeException, LimitExceededException,
ResourceAlreadyExistsException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Amazon OpenSearch Service domain. For more information, see Creating and
* managing Amazon OpenSearch Service domains.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDomainRequest.Builder} avoiding the need to
* create one manually via {@link CreateDomainRequest#builder()}
*
*
* @param createDomainRequest
* A {@link Consumer} that will call methods on {@link CreateDomainRequest.Builder} to create a request.
* @return Result of the CreateDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateDomain
*/
default CreateDomainResponse createDomain(Consumer createDomainRequest) throws BaseException,
DisabledOperationException, InternalException, InvalidTypeException, LimitExceededException,
ResourceAlreadyExistsException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
return createDomain(CreateDomainRequest.builder().applyMutation(createDomainRequest).build());
}
/**
*
* Creates a new cross-cluster search connection from a source Amazon OpenSearch Service domain to a destination
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param createOutboundConnectionRequest
* Container for the parameters to the CreateOutboundConnection
operation.
* @return Result of the CreateOutboundConnection operation returned by the service.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateOutboundConnection
*/
default CreateOutboundConnectionResponse createOutboundConnection(
CreateOutboundConnectionRequest createOutboundConnectionRequest) throws LimitExceededException, InternalException,
ResourceAlreadyExistsException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new cross-cluster search connection from a source Amazon OpenSearch Service domain to a destination
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOutboundConnectionRequest.Builder} avoiding
* the need to create one manually via {@link CreateOutboundConnectionRequest#builder()}
*
*
* @param createOutboundConnectionRequest
* A {@link Consumer} that will call methods on {@link CreateOutboundConnectionRequest.Builder} to create a
* request. Container for the parameters to the CreateOutboundConnection
operation.
* @return Result of the CreateOutboundConnection operation returned by the service.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateOutboundConnection
*/
default CreateOutboundConnectionResponse createOutboundConnection(
Consumer createOutboundConnectionRequest) throws LimitExceededException,
InternalException, ResourceAlreadyExistsException, DisabledOperationException, AwsServiceException,
SdkClientException, OpenSearchException {
return createOutboundConnection(CreateOutboundConnectionRequest.builder().applyMutation(createOutboundConnectionRequest)
.build());
}
/**
*
* Creates a package for use with Amazon OpenSearch Service domains. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param createPackageRequest
* Container for request parameters to the CreatePackage
operation.
* @return Result of the CreatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreatePackage
*/
default CreatePackageResponse createPackage(CreatePackageRequest createPackageRequest) throws BaseException,
InternalException, LimitExceededException, InvalidTypeException, ResourceAlreadyExistsException,
AccessDeniedException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a package for use with Amazon OpenSearch Service domains. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link CreatePackageRequest.Builder} avoiding the need to
* create one manually via {@link CreatePackageRequest#builder()}
*
*
* @param createPackageRequest
* A {@link Consumer} that will call methods on {@link CreatePackageRequest.Builder} to create a request.
* Container for request parameters to the CreatePackage
operation.
* @return Result of the CreatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreatePackage
*/
default CreatePackageResponse createPackage(Consumer createPackageRequest)
throws BaseException, InternalException, LimitExceededException, InvalidTypeException,
ResourceAlreadyExistsException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return createPackage(CreatePackageRequest.builder().applyMutation(createPackageRequest).build());
}
/**
*
* Creates an Amazon OpenSearch Service-managed VPC endpoint.
*
*
* @param createVpcEndpointRequest
* @return Result of the CreateVpcEndpoint operation returned by the service.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateVpcEndpoint
*/
default CreateVpcEndpointResponse createVpcEndpoint(CreateVpcEndpointRequest createVpcEndpointRequest)
throws ConflictException, ValidationException, LimitExceededException, InternalException, DisabledOperationException,
BaseException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Amazon OpenSearch Service-managed VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link CreateVpcEndpointRequest.Builder} avoiding the need
* to create one manually via {@link CreateVpcEndpointRequest#builder()}
*
*
* @param createVpcEndpointRequest
* A {@link Consumer} that will call methods on {@link CreateVpcEndpointRequest.Builder} to create a request.
* @return Result of the CreateVpcEndpoint operation returned by the service.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.CreateVpcEndpoint
*/
default CreateVpcEndpointResponse createVpcEndpoint(Consumer createVpcEndpointRequest)
throws ConflictException, ValidationException, LimitExceededException, InternalException, DisabledOperationException,
BaseException, AwsServiceException, SdkClientException, OpenSearchException {
return createVpcEndpoint(CreateVpcEndpointRequest.builder().applyMutation(createVpcEndpointRequest).build());
}
/**
*
* Deletes an Amazon OpenSearch Service domain and all of its data. You can't recover a domain after you delete it.
*
*
* @param deleteDomainRequest
* Container for the parameters to the DeleteDomain
operation.
* @return Result of the DeleteDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteDomain
*/
default DeleteDomainResponse deleteDomain(DeleteDomainRequest deleteDomainRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon OpenSearch Service domain and all of its data. You can't recover a domain after you delete it.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteDomainRequest.Builder} avoiding the need to
* create one manually via {@link DeleteDomainRequest#builder()}
*
*
* @param deleteDomainRequest
* A {@link Consumer} that will call methods on {@link DeleteDomainRequest.Builder} to create a request.
* Container for the parameters to the DeleteDomain
operation.
* @return Result of the DeleteDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteDomain
*/
default DeleteDomainResponse deleteDomain(Consumer deleteDomainRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return deleteDomain(DeleteDomainRequest.builder().applyMutation(deleteDomainRequest).build());
}
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to delete an existing inbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param deleteInboundConnectionRequest
* Container for the parameters to the DeleteInboundConnection
operation.
* @return Result of the DeleteInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteInboundConnection
*/
default DeleteInboundConnectionResponse deleteInboundConnection(DeleteInboundConnectionRequest deleteInboundConnectionRequest)
throws ResourceNotFoundException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to delete an existing inbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInboundConnectionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteInboundConnectionRequest#builder()}
*
*
* @param deleteInboundConnectionRequest
* A {@link Consumer} that will call methods on {@link DeleteInboundConnectionRequest.Builder} to create a
* request. Container for the parameters to the DeleteInboundConnection
operation.
* @return Result of the DeleteInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteInboundConnection
*/
default DeleteInboundConnectionResponse deleteInboundConnection(
Consumer deleteInboundConnectionRequest) throws ResourceNotFoundException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
return deleteInboundConnection(DeleteInboundConnectionRequest.builder().applyMutation(deleteInboundConnectionRequest)
.build());
}
/**
*
* Allows the source Amazon OpenSearch Service domain owner to delete an existing outbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param deleteOutboundConnectionRequest
* Container for the parameters to the DeleteOutboundConnection
operation.
* @return Result of the DeleteOutboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteOutboundConnection
*/
default DeleteOutboundConnectionResponse deleteOutboundConnection(
DeleteOutboundConnectionRequest deleteOutboundConnectionRequest) throws ResourceNotFoundException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows the source Amazon OpenSearch Service domain owner to delete an existing outbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteOutboundConnectionRequest.Builder} avoiding
* the need to create one manually via {@link DeleteOutboundConnectionRequest#builder()}
*
*
* @param deleteOutboundConnectionRequest
* A {@link Consumer} that will call methods on {@link DeleteOutboundConnectionRequest.Builder} to create a
* request. Container for the parameters to the DeleteOutboundConnection
operation.
* @return Result of the DeleteOutboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteOutboundConnection
*/
default DeleteOutboundConnectionResponse deleteOutboundConnection(
Consumer deleteOutboundConnectionRequest) throws ResourceNotFoundException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
return deleteOutboundConnection(DeleteOutboundConnectionRequest.builder().applyMutation(deleteOutboundConnectionRequest)
.build());
}
/**
*
* Deletes an Amazon OpenSearch Service package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param deletePackageRequest
* Deletes a package from OpenSearch Service. The package can't be associated with any OpenSearch Service
* domain.
* @return Result of the DeletePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeletePackage
*/
default DeletePackageResponse deletePackage(DeletePackageRequest deletePackageRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, ConflictException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon OpenSearch Service package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePackageRequest.Builder} avoiding the need to
* create one manually via {@link DeletePackageRequest#builder()}
*
*
* @param deletePackageRequest
* A {@link Consumer} that will call methods on {@link DeletePackageRequest.Builder} to create a request.
* Deletes a package from OpenSearch Service. The package can't be associated with any OpenSearch Service
* domain.
* @return Result of the DeletePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeletePackage
*/
default DeletePackageResponse deletePackage(Consumer deletePackageRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ConflictException, AwsServiceException, SdkClientException, OpenSearchException {
return deletePackage(DeletePackageRequest.builder().applyMutation(deletePackageRequest).build());
}
/**
*
* Deletes an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
* @param deleteVpcEndpointRequest
* @return Result of the DeleteVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteVpcEndpoint
*/
default DeleteVpcEndpointResponse deleteVpcEndpoint(DeleteVpcEndpointRequest deleteVpcEndpointRequest)
throws ResourceNotFoundException, DisabledOperationException, InternalException, BaseException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteVpcEndpointRequest.Builder} avoiding the need
* to create one manually via {@link DeleteVpcEndpointRequest#builder()}
*
*
* @param deleteVpcEndpointRequest
* A {@link Consumer} that will call methods on {@link DeleteVpcEndpointRequest.Builder} to create a request.
* @return Result of the DeleteVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DeleteVpcEndpoint
*/
default DeleteVpcEndpointResponse deleteVpcEndpoint(Consumer deleteVpcEndpointRequest)
throws ResourceNotFoundException, DisabledOperationException, InternalException, BaseException, AwsServiceException,
SdkClientException, OpenSearchException {
return deleteVpcEndpoint(DeleteVpcEndpointRequest.builder().applyMutation(deleteVpcEndpointRequest).build());
}
/**
*
* Describes the domain configuration for the specified Amazon OpenSearch Service domain, including the domain ID,
* domain service endpoint, and domain ARN.
*
*
* @param describeDomainRequest
* Container for the parameters to the DescribeDomain
operation.
* @return Result of the DescribeDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomain
*/
default DescribeDomainResponse describeDomain(DescribeDomainRequest describeDomainRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the domain configuration for the specified Amazon OpenSearch Service domain, including the domain ID,
* domain service endpoint, and domain ARN.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainRequest.Builder} avoiding the need to
* create one manually via {@link DescribeDomainRequest#builder()}
*
*
* @param describeDomainRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainRequest.Builder} to create a request.
* Container for the parameters to the DescribeDomain
operation.
* @return Result of the DescribeDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomain
*/
default DescribeDomainResponse describeDomain(Consumer describeDomainRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return describeDomain(DescribeDomainRequest.builder().applyMutation(describeDomainRequest).build());
}
/**
*
* Returns the list of optimizations that Auto-Tune has made to an Amazon OpenSearch Service domain. For more
* information, see Auto-Tune for Amazon
* OpenSearch Service.
*
*
* @param describeDomainAutoTunesRequest
* Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return Result of the DescribeDomainAutoTunes operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainAutoTunes
*/
default DescribeDomainAutoTunesResponse describeDomainAutoTunes(DescribeDomainAutoTunesRequest describeDomainAutoTunesRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the list of optimizations that Auto-Tune has made to an Amazon OpenSearch Service domain. For more
* information, see Auto-Tune for Amazon
* OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainAutoTunesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDomainAutoTunesRequest#builder()}
*
*
* @param describeDomainAutoTunesRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainAutoTunesRequest.Builder} to create a
* request. Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return Result of the DescribeDomainAutoTunes operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainAutoTunes
*/
default DescribeDomainAutoTunesResponse describeDomainAutoTunes(
Consumer describeDomainAutoTunesRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeDomainAutoTunes(DescribeDomainAutoTunesRequest.builder().applyMutation(describeDomainAutoTunesRequest)
.build());
}
/**
*
* Returns the list of optimizations that Auto-Tune has made to an Amazon OpenSearch Service domain. For more
* information, see Auto-Tune for Amazon
* OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeDomainAutoTunes(software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client.describeDomainAutoTunesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client
* .describeDomainAutoTunesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client.describeDomainAutoTunesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDomainAutoTunes(software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest)}
* operation.
*
*
* @param describeDomainAutoTunesRequest
* Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainAutoTunes
*/
default DescribeDomainAutoTunesIterable describeDomainAutoTunesPaginator(
DescribeDomainAutoTunesRequest describeDomainAutoTunesRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the list of optimizations that Auto-Tune has made to an Amazon OpenSearch Service domain. For more
* information, see Auto-Tune for Amazon
* OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeDomainAutoTunes(software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client.describeDomainAutoTunesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client
* .describeDomainAutoTunesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeDomainAutoTunesIterable responses = client.describeDomainAutoTunesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeDomainAutoTunes(software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainAutoTunesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeDomainAutoTunesRequest#builder()}
*
*
* @param describeDomainAutoTunesRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainAutoTunesRequest.Builder} to create a
* request. Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainAutoTunes
*/
default DescribeDomainAutoTunesIterable describeDomainAutoTunesPaginator(
Consumer describeDomainAutoTunesRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeDomainAutoTunesPaginator(DescribeDomainAutoTunesRequest.builder()
.applyMutation(describeDomainAutoTunesRequest).build());
}
/**
*
* Returns information about the current blue/green deployment happening on an Amazon OpenSearch Service domain. For
* more information, see Making configuration changes in Amazon OpenSearch Service.
*
*
* @param describeDomainChangeProgressRequest
* Container for the parameters to the DescribeDomainChangeProgress
operation.
* @return Result of the DescribeDomainChangeProgress operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainChangeProgress
*/
default DescribeDomainChangeProgressResponse describeDomainChangeProgress(
DescribeDomainChangeProgressRequest describeDomainChangeProgressRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns information about the current blue/green deployment happening on an Amazon OpenSearch Service domain. For
* more information, see Making configuration changes in Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainChangeProgressRequest.Builder}
* avoiding the need to create one manually via {@link DescribeDomainChangeProgressRequest#builder()}
*
*
* @param describeDomainChangeProgressRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainChangeProgressRequest.Builder} to create
* a request. Container for the parameters to the DescribeDomainChangeProgress
operation.
* @return Result of the DescribeDomainChangeProgress operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainChangeProgress
*/
default DescribeDomainChangeProgressResponse describeDomainChangeProgress(
Consumer describeDomainChangeProgressRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeDomainChangeProgress(DescribeDomainChangeProgressRequest.builder()
.applyMutation(describeDomainChangeProgressRequest).build());
}
/**
*
* Returns the configuration of an Amazon OpenSearch Service domain.
*
*
* @param describeDomainConfigRequest
* Container for the parameters to the DescribeDomainConfig
operation.
* @return Result of the DescribeDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainConfig
*/
default DescribeDomainConfigResponse describeDomainConfig(DescribeDomainConfigRequest describeDomainConfigRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the configuration of an Amazon OpenSearch Service domain.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainConfigRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDomainConfigRequest#builder()}
*
*
* @param describeDomainConfigRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainConfigRequest.Builder} to create a
* request. Container for the parameters to the DescribeDomainConfig
operation.
* @return Result of the DescribeDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomainConfig
*/
default DescribeDomainConfigResponse describeDomainConfig(
Consumer describeDomainConfigRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
return describeDomainConfig(DescribeDomainConfigRequest.builder().applyMutation(describeDomainConfigRequest).build());
}
/**
*
* Returns domain configuration information about the specified Amazon OpenSearch Service domains.
*
*
* @param describeDomainsRequest
* Container for the parameters to the DescribeDomains
operation.
* @return Result of the DescribeDomains operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomains
*/
default DescribeDomainsResponse describeDomains(DescribeDomainsRequest describeDomainsRequest) throws BaseException,
InternalException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns domain configuration information about the specified Amazon OpenSearch Service domains.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDomainsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeDomainsRequest#builder()}
*
*
* @param describeDomainsRequest
* A {@link Consumer} that will call methods on {@link DescribeDomainsRequest.Builder} to create a request.
* Container for the parameters to the DescribeDomains
operation.
* @return Result of the DescribeDomains operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDomains
*/
default DescribeDomainsResponse describeDomains(Consumer describeDomainsRequest)
throws BaseException, InternalException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeDomains(DescribeDomainsRequest.builder().applyMutation(describeDomainsRequest).build());
}
/**
*
* Describes the progress of a pre-update dry run analysis on an Amazon OpenSearch Service domain. For more
* information, see Determining whether a change will cause a blue/green deployment.
*
*
* @param describeDryRunProgressRequest
* @return Result of the DescribeDryRunProgress operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDryRunProgress
*/
default DescribeDryRunProgressResponse describeDryRunProgress(DescribeDryRunProgressRequest describeDryRunProgressRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, DisabledOperationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the progress of a pre-update dry run analysis on an Amazon OpenSearch Service domain. For more
* information, see Determining whether a change will cause a blue/green deployment.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDryRunProgressRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDryRunProgressRequest#builder()}
*
*
* @param describeDryRunProgressRequest
* A {@link Consumer} that will call methods on {@link DescribeDryRunProgressRequest.Builder} to create a
* request.
* @return Result of the DescribeDryRunProgress operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeDryRunProgress
*/
default DescribeDryRunProgressResponse describeDryRunProgress(
Consumer describeDryRunProgressRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, DisabledOperationException, AwsServiceException,
SdkClientException, OpenSearchException {
return describeDryRunProgress(DescribeDryRunProgressRequest.builder().applyMutation(describeDryRunProgressRequest)
.build());
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination (remote) Amazon OpenSearch Service
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param describeInboundConnectionsRequest
* Container for the parameters to the DescribeInboundConnections
operation.
* @return Result of the DescribeInboundConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInboundConnections
*/
default DescribeInboundConnectionsResponse describeInboundConnections(
DescribeInboundConnectionsRequest describeInboundConnectionsRequest) throws InvalidPaginationTokenException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination (remote) Amazon OpenSearch Service
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInboundConnectionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeInboundConnectionsRequest#builder()}
*
*
* @param describeInboundConnectionsRequest
* A {@link Consumer} that will call methods on {@link DescribeInboundConnectionsRequest.Builder} to create a
* request. Container for the parameters to the DescribeInboundConnections
operation.
* @return Result of the DescribeInboundConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInboundConnections
*/
default DescribeInboundConnectionsResponse describeInboundConnections(
Consumer describeInboundConnectionsRequest)
throws InvalidPaginationTokenException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeInboundConnections(DescribeInboundConnectionsRequest.builder()
.applyMutation(describeInboundConnectionsRequest).build());
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination (remote) Amazon OpenSearch Service
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeInboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client.describeInboundConnectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client
* .describeInboundConnectionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client.describeInboundConnectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeInboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest)}
* operation.
*
*
* @param describeInboundConnectionsRequest
* Container for the parameters to the DescribeInboundConnections
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInboundConnections
*/
default DescribeInboundConnectionsIterable describeInboundConnectionsPaginator(
DescribeInboundConnectionsRequest describeInboundConnectionsRequest) throws InvalidPaginationTokenException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination (remote) Amazon OpenSearch Service
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeInboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client.describeInboundConnectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client
* .describeInboundConnectionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeInboundConnectionsIterable responses = client.describeInboundConnectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeInboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeInboundConnectionsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeInboundConnectionsRequest#builder()}
*
*
* @param describeInboundConnectionsRequest
* A {@link Consumer} that will call methods on {@link DescribeInboundConnectionsRequest.Builder} to create a
* request. Container for the parameters to the DescribeInboundConnections
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInboundConnections
*/
default DescribeInboundConnectionsIterable describeInboundConnectionsPaginator(
Consumer describeInboundConnectionsRequest)
throws InvalidPaginationTokenException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeInboundConnectionsPaginator(DescribeInboundConnectionsRequest.builder()
.applyMutation(describeInboundConnectionsRequest).build());
}
/**
*
* Describes the instance count, storage, and master node limits for a given OpenSearch or Elasticsearch version and
* instance type.
*
*
* @param describeInstanceTypeLimitsRequest
* Container for the parameters to the DescribeInstanceTypeLimits
operation.
* @return Result of the DescribeInstanceTypeLimits operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInstanceTypeLimits
*/
default DescribeInstanceTypeLimitsResponse describeInstanceTypeLimits(
DescribeInstanceTypeLimitsRequest describeInstanceTypeLimitsRequest) throws BaseException, InternalException,
InvalidTypeException, LimitExceededException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the instance count, storage, and master node limits for a given OpenSearch or Elasticsearch version and
* instance type.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstanceTypeLimitsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeInstanceTypeLimitsRequest#builder()}
*
*
* @param describeInstanceTypeLimitsRequest
* A {@link Consumer} that will call methods on {@link DescribeInstanceTypeLimitsRequest.Builder} to create a
* request. Container for the parameters to the DescribeInstanceTypeLimits
operation.
* @return Result of the DescribeInstanceTypeLimits operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeInstanceTypeLimits
*/
default DescribeInstanceTypeLimitsResponse describeInstanceTypeLimits(
Consumer describeInstanceTypeLimitsRequest) throws BaseException,
InternalException, InvalidTypeException, LimitExceededException, ResourceNotFoundException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
return describeInstanceTypeLimits(DescribeInstanceTypeLimitsRequest.builder()
.applyMutation(describeInstanceTypeLimitsRequest).build());
}
/**
*
* Lists all the outbound cross-cluster connections for a local (source) Amazon OpenSearch Service domain. For more
* information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param describeOutboundConnectionsRequest
* Container for the parameters to the DescribeOutboundConnections
operation.
* @return Result of the DescribeOutboundConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeOutboundConnections
*/
default DescribeOutboundConnectionsResponse describeOutboundConnections(
DescribeOutboundConnectionsRequest describeOutboundConnectionsRequest) throws InvalidPaginationTokenException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the outbound cross-cluster connections for a local (source) Amazon OpenSearch Service domain. For more
* information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeOutboundConnectionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeOutboundConnectionsRequest#builder()}
*
*
* @param describeOutboundConnectionsRequest
* A {@link Consumer} that will call methods on {@link DescribeOutboundConnectionsRequest.Builder} to create
* a request. Container for the parameters to the DescribeOutboundConnections
operation.
* @return Result of the DescribeOutboundConnections operation returned by the service.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeOutboundConnections
*/
default DescribeOutboundConnectionsResponse describeOutboundConnections(
Consumer describeOutboundConnectionsRequest)
throws InvalidPaginationTokenException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeOutboundConnections(DescribeOutboundConnectionsRequest.builder()
.applyMutation(describeOutboundConnectionsRequest).build());
}
/**
*
* Lists all the outbound cross-cluster connections for a local (source) Amazon OpenSearch Service domain. For more
* information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeOutboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client.describeOutboundConnectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client
* .describeOutboundConnectionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client.describeOutboundConnectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeOutboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest)}
* operation.
*
*
* @param describeOutboundConnectionsRequest
* Container for the parameters to the DescribeOutboundConnections
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeOutboundConnections
*/
default DescribeOutboundConnectionsIterable describeOutboundConnectionsPaginator(
DescribeOutboundConnectionsRequest describeOutboundConnectionsRequest) throws InvalidPaginationTokenException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all the outbound cross-cluster connections for a local (source) Amazon OpenSearch Service domain. For more
* information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeOutboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client.describeOutboundConnectionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client
* .describeOutboundConnectionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeOutboundConnectionsIterable responses = client.describeOutboundConnectionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeOutboundConnections(software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeOutboundConnectionsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeOutboundConnectionsRequest#builder()}
*
*
* @param describeOutboundConnectionsRequest
* A {@link Consumer} that will call methods on {@link DescribeOutboundConnectionsRequest.Builder} to create
* a request. Container for the parameters to the DescribeOutboundConnections
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeOutboundConnections
*/
default DescribeOutboundConnectionsIterable describeOutboundConnectionsPaginator(
Consumer describeOutboundConnectionsRequest)
throws InvalidPaginationTokenException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeOutboundConnectionsPaginator(DescribeOutboundConnectionsRequest.builder()
.applyMutation(describeOutboundConnectionsRequest).build());
}
/**
*
* Describes all packages available to OpenSearch Service. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param describePackagesRequest
* Container for the request parameters to the DescribePackage
operation.
* @return Result of the DescribePackages operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribePackages
*/
default DescribePackagesResponse describePackages(DescribePackagesRequest describePackagesRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes all packages available to OpenSearch Service. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePackagesRequest.Builder} avoiding the need
* to create one manually via {@link DescribePackagesRequest#builder()}
*
*
* @param describePackagesRequest
* A {@link Consumer} that will call methods on {@link DescribePackagesRequest.Builder} to create a request.
* Container for the request parameters to the DescribePackage
operation.
* @return Result of the DescribePackages operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribePackages
*/
default DescribePackagesResponse describePackages(Consumer describePackagesRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
return describePackages(DescribePackagesRequest.builder().applyMutation(describePackagesRequest).build());
}
/**
*
* Describes all packages available to OpenSearch Service. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describePackages(software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client.describePackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client
* .describePackagesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribePackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client.describePackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePackages(software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest)}
* operation.
*
*
* @param describePackagesRequest
* Container for the request parameters to the DescribePackage
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribePackages
*/
default DescribePackagesIterable describePackagesPaginator(DescribePackagesRequest describePackagesRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes all packages available to OpenSearch Service. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describePackages(software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client.describePackagesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client
* .describePackagesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribePackagesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribePackagesIterable responses = client.describePackagesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describePackages(software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribePackagesRequest.Builder} avoiding the need
* to create one manually via {@link DescribePackagesRequest#builder()}
*
*
* @param describePackagesRequest
* A {@link Consumer} that will call methods on {@link DescribePackagesRequest.Builder} to create a request.
* Container for the request parameters to the DescribePackage
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribePackages
*/
default DescribePackagesIterable describePackagesPaginator(Consumer describePackagesRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
return describePackagesPaginator(DescribePackagesRequest.builder().applyMutation(describePackagesRequest).build());
}
/**
*
* Describes the available Amazon OpenSearch Service Reserved Instance offerings for a given Region. For more
* information, see Reserved
* Instances in Amazon OpenSearch Service.
*
*
* @param describeReservedInstanceOfferingsRequest
* Container for the request parameters to a DescribeReservedInstanceOfferings
operation.
* @return Result of the DescribeReservedInstanceOfferings operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstanceOfferings
*/
default DescribeReservedInstanceOfferingsResponse describeReservedInstanceOfferings(
DescribeReservedInstanceOfferingsRequest describeReservedInstanceOfferingsRequest) throws ResourceNotFoundException,
ValidationException, DisabledOperationException, InternalException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the available Amazon OpenSearch Service Reserved Instance offerings for a given Region. For more
* information, see Reserved
* Instances in Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedInstanceOfferingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeReservedInstanceOfferingsRequest#builder()}
*
*
* @param describeReservedInstanceOfferingsRequest
* A {@link Consumer} that will call methods on {@link DescribeReservedInstanceOfferingsRequest.Builder} to
* create a request. Container for the request parameters to a DescribeReservedInstanceOfferings
* operation.
* @return Result of the DescribeReservedInstanceOfferings operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstanceOfferings
*/
default DescribeReservedInstanceOfferingsResponse describeReservedInstanceOfferings(
Consumer describeReservedInstanceOfferingsRequest)
throws ResourceNotFoundException, ValidationException, DisabledOperationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
return describeReservedInstanceOfferings(DescribeReservedInstanceOfferingsRequest.builder()
.applyMutation(describeReservedInstanceOfferingsRequest).build());
}
/**
*
* Describes the available Amazon OpenSearch Service Reserved Instance offerings for a given Region. For more
* information, see Reserved
* Instances in Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeReservedInstanceOfferings(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client.describeReservedInstanceOfferingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client
* .describeReservedInstanceOfferingsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client.describeReservedInstanceOfferingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedInstanceOfferings(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest)}
* operation.
*
*
* @param describeReservedInstanceOfferingsRequest
* Container for the request parameters to a DescribeReservedInstanceOfferings
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstanceOfferings
*/
default DescribeReservedInstanceOfferingsIterable describeReservedInstanceOfferingsPaginator(
DescribeReservedInstanceOfferingsRequest describeReservedInstanceOfferingsRequest) throws ResourceNotFoundException,
ValidationException, DisabledOperationException, InternalException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the available Amazon OpenSearch Service Reserved Instance offerings for a given Region. For more
* information, see Reserved
* Instances in Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeReservedInstanceOfferings(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client.describeReservedInstanceOfferingsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client
* .describeReservedInstanceOfferingsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstanceOfferingsIterable responses = client.describeReservedInstanceOfferingsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedInstanceOfferings(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedInstanceOfferingsRequest.Builder}
* avoiding the need to create one manually via {@link DescribeReservedInstanceOfferingsRequest#builder()}
*
*
* @param describeReservedInstanceOfferingsRequest
* A {@link Consumer} that will call methods on {@link DescribeReservedInstanceOfferingsRequest.Builder} to
* create a request. Container for the request parameters to a DescribeReservedInstanceOfferings
* operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstanceOfferings
*/
default DescribeReservedInstanceOfferingsIterable describeReservedInstanceOfferingsPaginator(
Consumer describeReservedInstanceOfferingsRequest)
throws ResourceNotFoundException, ValidationException, DisabledOperationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
return describeReservedInstanceOfferingsPaginator(DescribeReservedInstanceOfferingsRequest.builder()
.applyMutation(describeReservedInstanceOfferingsRequest).build());
}
/**
*
* Describes the Amazon OpenSearch Service instances that you have reserved in a given Region. For more information,
* see Reserved Instances in
* Amazon OpenSearch Service.
*
*
* @param describeReservedInstancesRequest
* Container for the request parameters to the DescribeReservedInstances
operation.
* @return Result of the DescribeReservedInstances operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstances
*/
default DescribeReservedInstancesResponse describeReservedInstances(
DescribeReservedInstancesRequest describeReservedInstancesRequest) throws ResourceNotFoundException,
InternalException, ValidationException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the Amazon OpenSearch Service instances that you have reserved in a given Region. For more information,
* see Reserved Instances in
* Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedInstancesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReservedInstancesRequest#builder()}
*
*
* @param describeReservedInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeReservedInstancesRequest.Builder} to create a
* request. Container for the request parameters to the DescribeReservedInstances
operation.
* @return Result of the DescribeReservedInstances operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstances
*/
default DescribeReservedInstancesResponse describeReservedInstances(
Consumer describeReservedInstancesRequest)
throws ResourceNotFoundException, InternalException, ValidationException, DisabledOperationException,
AwsServiceException, SdkClientException, OpenSearchException {
return describeReservedInstances(DescribeReservedInstancesRequest.builder()
.applyMutation(describeReservedInstancesRequest).build());
}
/**
*
* Describes the Amazon OpenSearch Service instances that you have reserved in a given Region. For more information,
* see Reserved Instances in
* Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeReservedInstances(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client.describeReservedInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client
* .describeReservedInstancesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client.describeReservedInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedInstances(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest)}
* operation.
*
*
* @param describeReservedInstancesRequest
* Container for the request parameters to the DescribeReservedInstances
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstances
*/
default DescribeReservedInstancesIterable describeReservedInstancesPaginator(
DescribeReservedInstancesRequest describeReservedInstancesRequest) throws ResourceNotFoundException,
InternalException, ValidationException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the Amazon OpenSearch Service instances that you have reserved in a given Region. For more information,
* see Reserved Instances in
* Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #describeReservedInstances(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client.describeReservedInstancesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client
* .describeReservedInstancesPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.DescribeReservedInstancesIterable responses = client.describeReservedInstancesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeReservedInstances(software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeReservedInstancesRequest.Builder} avoiding
* the need to create one manually via {@link DescribeReservedInstancesRequest#builder()}
*
*
* @param describeReservedInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeReservedInstancesRequest.Builder} to create a
* request. Container for the request parameters to the DescribeReservedInstances
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeReservedInstances
*/
default DescribeReservedInstancesIterable describeReservedInstancesPaginator(
Consumer describeReservedInstancesRequest)
throws ResourceNotFoundException, InternalException, ValidationException, DisabledOperationException,
AwsServiceException, SdkClientException, OpenSearchException {
return describeReservedInstancesPaginator(DescribeReservedInstancesRequest.builder()
.applyMutation(describeReservedInstancesRequest).build());
}
/**
*
* Describes one or more Amazon OpenSearch Service-managed VPC endpoints.
*
*
* @param describeVpcEndpointsRequest
* @return Result of the DescribeVpcEndpoints operation returned by the service.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeVpcEndpoints
*/
default DescribeVpcEndpointsResponse describeVpcEndpoints(DescribeVpcEndpointsRequest describeVpcEndpointsRequest)
throws ValidationException, InternalException, DisabledOperationException, BaseException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Describes one or more Amazon OpenSearch Service-managed VPC endpoints.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeVpcEndpointsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeVpcEndpointsRequest#builder()}
*
*
* @param describeVpcEndpointsRequest
* A {@link Consumer} that will call methods on {@link DescribeVpcEndpointsRequest.Builder} to create a
* request.
* @return Result of the DescribeVpcEndpoints operation returned by the service.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DescribeVpcEndpoints
*/
default DescribeVpcEndpointsResponse describeVpcEndpoints(
Consumer describeVpcEndpointsRequest) throws ValidationException,
InternalException, DisabledOperationException, BaseException, AwsServiceException, SdkClientException,
OpenSearchException {
return describeVpcEndpoints(DescribeVpcEndpointsRequest.builder().applyMutation(describeVpcEndpointsRequest).build());
}
/**
*
* Removes a package from the specified Amazon OpenSearch Service domain. The package can't be in use with any
* OpenSearch index for the dissociation to succeed. The package is still available in OpenSearch Service for
* association later. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param dissociatePackageRequest
* Container for the request parameters to the DissociatePackage
operation.
* @return Result of the DissociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DissociatePackage
*/
default DissociatePackageResponse dissociatePackage(DissociatePackageRequest dissociatePackageRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, ConflictException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Removes a package from the specified Amazon OpenSearch Service domain. The package can't be in use with any
* OpenSearch index for the dissociation to succeed. The package is still available in OpenSearch Service for
* association later. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link DissociatePackageRequest.Builder} avoiding the need
* to create one manually via {@link DissociatePackageRequest#builder()}
*
*
* @param dissociatePackageRequest
* A {@link Consumer} that will call methods on {@link DissociatePackageRequest.Builder} to create a request.
* Container for the request parameters to the DissociatePackage
operation.
* @return Result of the DissociatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.DissociatePackage
*/
default DissociatePackageResponse dissociatePackage(Consumer dissociatePackageRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
ConflictException, AwsServiceException, SdkClientException, OpenSearchException {
return dissociatePackage(DissociatePackageRequest.builder().applyMutation(dissociatePackageRequest).build());
}
/**
*
* Returns a map of OpenSearch or Elasticsearch versions and the versions you can upgrade them to.
*
*
* @param getCompatibleVersionsRequest
* Container for the request parameters to GetCompatibleVersions
operation.
* @return Result of the GetCompatibleVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetCompatibleVersions
*/
default GetCompatibleVersionsResponse getCompatibleVersions(GetCompatibleVersionsRequest getCompatibleVersionsRequest)
throws BaseException, ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a map of OpenSearch or Elasticsearch versions and the versions you can upgrade them to.
*
*
*
* This is a convenience which creates an instance of the {@link GetCompatibleVersionsRequest.Builder} avoiding the
* need to create one manually via {@link GetCompatibleVersionsRequest#builder()}
*
*
* @param getCompatibleVersionsRequest
* A {@link Consumer} that will call methods on {@link GetCompatibleVersionsRequest.Builder} to create a
* request. Container for the request parameters to GetCompatibleVersions
operation.
* @return Result of the GetCompatibleVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetCompatibleVersions
*/
default GetCompatibleVersionsResponse getCompatibleVersions(
Consumer getCompatibleVersionsRequest) throws BaseException,
ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException, AwsServiceException,
SdkClientException, OpenSearchException {
return getCompatibleVersions(GetCompatibleVersionsRequest.builder().applyMutation(getCompatibleVersionsRequest).build());
}
/**
*
* Returns a list of Amazon OpenSearch Service package versions, along with their creation time and commit message.
* For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param getPackageVersionHistoryRequest
* Container for the request parameters to the GetPackageVersionHistory
operation.
* @return Result of the GetPackageVersionHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetPackageVersionHistory
*/
default GetPackageVersionHistoryResponse getPackageVersionHistory(
GetPackageVersionHistoryRequest getPackageVersionHistoryRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of Amazon OpenSearch Service package versions, along with their creation time and commit message.
* For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link GetPackageVersionHistoryRequest.Builder} avoiding
* the need to create one manually via {@link GetPackageVersionHistoryRequest#builder()}
*
*
* @param getPackageVersionHistoryRequest
* A {@link Consumer} that will call methods on {@link GetPackageVersionHistoryRequest.Builder} to create a
* request. Container for the request parameters to the GetPackageVersionHistory
operation.
* @return Result of the GetPackageVersionHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetPackageVersionHistory
*/
default GetPackageVersionHistoryResponse getPackageVersionHistory(
Consumer getPackageVersionHistoryRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return getPackageVersionHistory(GetPackageVersionHistoryRequest.builder().applyMutation(getPackageVersionHistoryRequest)
.build());
}
/**
*
* Returns a list of Amazon OpenSearch Service package versions, along with their creation time and commit message.
* For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #getPackageVersionHistory(software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client.getPackageVersionHistoryPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client
* .getPackageVersionHistoryPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client.getPackageVersionHistoryPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getPackageVersionHistory(software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest)}
* operation.
*
*
* @param getPackageVersionHistoryRequest
* Container for the request parameters to the GetPackageVersionHistory
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetPackageVersionHistory
*/
default GetPackageVersionHistoryIterable getPackageVersionHistoryPaginator(
GetPackageVersionHistoryRequest getPackageVersionHistoryRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of Amazon OpenSearch Service package versions, along with their creation time and commit message.
* For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #getPackageVersionHistory(software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client.getPackageVersionHistoryPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client
* .getPackageVersionHistoryPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetPackageVersionHistoryIterable responses = client.getPackageVersionHistoryPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getPackageVersionHistory(software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetPackageVersionHistoryRequest.Builder} avoiding
* the need to create one manually via {@link GetPackageVersionHistoryRequest#builder()}
*
*
* @param getPackageVersionHistoryRequest
* A {@link Consumer} that will call methods on {@link GetPackageVersionHistoryRequest.Builder} to create a
* request. Container for the request parameters to the GetPackageVersionHistory
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetPackageVersionHistory
*/
default GetPackageVersionHistoryIterable getPackageVersionHistoryPaginator(
Consumer getPackageVersionHistoryRequest) throws BaseException,
InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return getPackageVersionHistoryPaginator(GetPackageVersionHistoryRequest.builder()
.applyMutation(getPackageVersionHistoryRequest).build());
}
/**
*
* Retrieves the complete history of the last 10 upgrades performed on an Amazon OpenSearch Service domain.
*
*
* @param getUpgradeHistoryRequest
* Container for the request parameters to the GetUpgradeHistory
operation.
* @return Result of the GetUpgradeHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeHistory
*/
default GetUpgradeHistoryResponse getUpgradeHistory(GetUpgradeHistoryRequest getUpgradeHistoryRequest) throws BaseException,
ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the complete history of the last 10 upgrades performed on an Amazon OpenSearch Service domain.
*
*
*
* This is a convenience which creates an instance of the {@link GetUpgradeHistoryRequest.Builder} avoiding the need
* to create one manually via {@link GetUpgradeHistoryRequest#builder()}
*
*
* @param getUpgradeHistoryRequest
* A {@link Consumer} that will call methods on {@link GetUpgradeHistoryRequest.Builder} to create a request.
* Container for the request parameters to the GetUpgradeHistory
operation.
* @return Result of the GetUpgradeHistory operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeHistory
*/
default GetUpgradeHistoryResponse getUpgradeHistory(Consumer getUpgradeHistoryRequest)
throws BaseException, ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
return getUpgradeHistory(GetUpgradeHistoryRequest.builder().applyMutation(getUpgradeHistoryRequest).build());
}
/**
*
* Retrieves the complete history of the last 10 upgrades performed on an Amazon OpenSearch Service domain.
*
*
*
* This is a variant of
* {@link #getUpgradeHistory(software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client.getUpgradeHistoryPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client
* .getUpgradeHistoryPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client.getUpgradeHistoryPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getUpgradeHistory(software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest)}
* operation.
*
*
* @param getUpgradeHistoryRequest
* Container for the request parameters to the GetUpgradeHistory
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeHistory
*/
default GetUpgradeHistoryIterable getUpgradeHistoryPaginator(GetUpgradeHistoryRequest getUpgradeHistoryRequest)
throws BaseException, ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves the complete history of the last 10 upgrades performed on an Amazon OpenSearch Service domain.
*
*
*
* This is a variant of
* {@link #getUpgradeHistory(software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest)} operation.
* The return type is a custom iterable that can be used to iterate through all the pages. SDK will internally
* handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client.getUpgradeHistoryPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client
* .getUpgradeHistoryPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.GetUpgradeHistoryIterable responses = client.getUpgradeHistoryPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getUpgradeHistory(software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetUpgradeHistoryRequest.Builder} avoiding the need
* to create one manually via {@link GetUpgradeHistoryRequest#builder()}
*
*
* @param getUpgradeHistoryRequest
* A {@link Consumer} that will call methods on {@link GetUpgradeHistoryRequest.Builder} to create a request.
* Container for the request parameters to the GetUpgradeHistory
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeHistory
*/
default GetUpgradeHistoryIterable getUpgradeHistoryPaginator(
Consumer getUpgradeHistoryRequest) throws BaseException, ResourceNotFoundException,
DisabledOperationException, ValidationException, InternalException, AwsServiceException, SdkClientException,
OpenSearchException {
return getUpgradeHistoryPaginator(GetUpgradeHistoryRequest.builder().applyMutation(getUpgradeHistoryRequest).build());
}
/**
*
* Returns the most recent status of the last upgrade or upgrade eligibility check performed on an Amazon OpenSearch
* Service domain.
*
*
* @param getUpgradeStatusRequest
* Container for the request parameters to the GetUpgradeStatus
operation.
* @return Result of the GetUpgradeStatus operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeStatus
*/
default GetUpgradeStatusResponse getUpgradeStatus(GetUpgradeStatusRequest getUpgradeStatusRequest) throws BaseException,
ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the most recent status of the last upgrade or upgrade eligibility check performed on an Amazon OpenSearch
* Service domain.
*
*
*
* This is a convenience which creates an instance of the {@link GetUpgradeStatusRequest.Builder} avoiding the need
* to create one manually via {@link GetUpgradeStatusRequest#builder()}
*
*
* @param getUpgradeStatusRequest
* A {@link Consumer} that will call methods on {@link GetUpgradeStatusRequest.Builder} to create a request.
* Container for the request parameters to the GetUpgradeStatus
operation.
* @return Result of the GetUpgradeStatus operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.GetUpgradeStatus
*/
default GetUpgradeStatusResponse getUpgradeStatus(Consumer getUpgradeStatusRequest)
throws BaseException, ResourceNotFoundException, DisabledOperationException, ValidationException, InternalException,
AwsServiceException, SdkClientException, OpenSearchException {
return getUpgradeStatus(GetUpgradeStatusRequest.builder().applyMutation(getUpgradeStatusRequest).build());
}
/**
*
* Returns the names of all Amazon OpenSearch Service domains owned by the current user in the active Region.
*
*
* @param listDomainNamesRequest
* Container for the parameters to the ListDomainNames
operation.
* @return Result of the ListDomainNames operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainNames
*/
default ListDomainNamesResponse listDomainNames(ListDomainNamesRequest listDomainNamesRequest) throws BaseException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns the names of all Amazon OpenSearch Service domains owned by the current user in the active Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListDomainNamesRequest.Builder} avoiding the need
* to create one manually via {@link ListDomainNamesRequest#builder()}
*
*
* @param listDomainNamesRequest
* A {@link Consumer} that will call methods on {@link ListDomainNamesRequest.Builder} to create a request.
* Container for the parameters to the ListDomainNames
operation.
* @return Result of the ListDomainNames operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainNames
*/
default ListDomainNamesResponse listDomainNames(Consumer listDomainNamesRequest)
throws BaseException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
return listDomainNames(ListDomainNamesRequest.builder().applyMutation(listDomainNamesRequest).build());
}
/**
*
* Lists all Amazon OpenSearch Service domains associated with a given package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param listDomainsForPackageRequest
* Container for the request parameters to the ListDomainsForPackage
operation.
* @return Result of the ListDomainsForPackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainsForPackage
*/
default ListDomainsForPackageResponse listDomainsForPackage(ListDomainsForPackageRequest listDomainsForPackageRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all Amazon OpenSearch Service domains associated with a given package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link ListDomainsForPackageRequest.Builder} avoiding the
* need to create one manually via {@link ListDomainsForPackageRequest#builder()}
*
*
* @param listDomainsForPackageRequest
* A {@link Consumer} that will call methods on {@link ListDomainsForPackageRequest.Builder} to create a
* request. Container for the request parameters to the ListDomainsForPackage
operation.
* @return Result of the ListDomainsForPackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainsForPackage
*/
default ListDomainsForPackageResponse listDomainsForPackage(
Consumer listDomainsForPackageRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listDomainsForPackage(ListDomainsForPackageRequest.builder().applyMutation(listDomainsForPackageRequest).build());
}
/**
*
* Lists all Amazon OpenSearch Service domains associated with a given package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #listDomainsForPackage(software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client.listDomainsForPackagePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client
* .listDomainsForPackagePaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client.listDomainsForPackagePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDomainsForPackage(software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest)}
* operation.
*
*
* @param listDomainsForPackageRequest
* Container for the request parameters to the ListDomainsForPackage
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainsForPackage
*/
default ListDomainsForPackageIterable listDomainsForPackagePaginator(ListDomainsForPackageRequest listDomainsForPackageRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all Amazon OpenSearch Service domains associated with a given package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #listDomainsForPackage(software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client.listDomainsForPackagePaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client
* .listDomainsForPackagePaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListDomainsForPackageIterable responses = client.listDomainsForPackagePaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDomainsForPackage(software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListDomainsForPackageRequest.Builder} avoiding the
* need to create one manually via {@link ListDomainsForPackageRequest#builder()}
*
*
* @param listDomainsForPackageRequest
* A {@link Consumer} that will call methods on {@link ListDomainsForPackageRequest.Builder} to create a
* request. Container for the request parameters to the ListDomainsForPackage
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListDomainsForPackage
*/
default ListDomainsForPackageIterable listDomainsForPackagePaginator(
Consumer listDomainsForPackageRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listDomainsForPackagePaginator(ListDomainsForPackageRequest.builder().applyMutation(listDomainsForPackageRequest)
.build());
}
/**
*
* Lists all instance types and available features for a given OpenSearch or Elasticsearch version.
*
*
* @param listInstanceTypeDetailsRequest
* @return Result of the ListInstanceTypeDetails operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListInstanceTypeDetails
*/
default ListInstanceTypeDetailsResponse listInstanceTypeDetails(ListInstanceTypeDetailsRequest listInstanceTypeDetailsRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all instance types and available features for a given OpenSearch or Elasticsearch version.
*
*
*
* This is a convenience which creates an instance of the {@link ListInstanceTypeDetailsRequest.Builder} avoiding
* the need to create one manually via {@link ListInstanceTypeDetailsRequest#builder()}
*
*
* @param listInstanceTypeDetailsRequest
* A {@link Consumer} that will call methods on {@link ListInstanceTypeDetailsRequest.Builder} to create a
* request.
* @return Result of the ListInstanceTypeDetails operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListInstanceTypeDetails
*/
default ListInstanceTypeDetailsResponse listInstanceTypeDetails(
Consumer listInstanceTypeDetailsRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listInstanceTypeDetails(ListInstanceTypeDetailsRequest.builder().applyMutation(listInstanceTypeDetailsRequest)
.build());
}
/**
*
* Lists all instance types and available features for a given OpenSearch or Elasticsearch version.
*
*
*
* This is a variant of
* {@link #listInstanceTypeDetails(software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client.listInstanceTypeDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client
* .listInstanceTypeDetailsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client.listInstanceTypeDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceTypeDetails(software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest)}
* operation.
*
*
* @param listInstanceTypeDetailsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListInstanceTypeDetails
*/
default ListInstanceTypeDetailsIterable listInstanceTypeDetailsPaginator(
ListInstanceTypeDetailsRequest listInstanceTypeDetailsRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all instance types and available features for a given OpenSearch or Elasticsearch version.
*
*
*
* This is a variant of
* {@link #listInstanceTypeDetails(software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client.listInstanceTypeDetailsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client
* .listInstanceTypeDetailsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListInstanceTypeDetailsIterable responses = client.listInstanceTypeDetailsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listInstanceTypeDetails(software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListInstanceTypeDetailsRequest.Builder} avoiding
* the need to create one manually via {@link ListInstanceTypeDetailsRequest#builder()}
*
*
* @param listInstanceTypeDetailsRequest
* A {@link Consumer} that will call methods on {@link ListInstanceTypeDetailsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListInstanceTypeDetails
*/
default ListInstanceTypeDetailsIterable listInstanceTypeDetailsPaginator(
Consumer listInstanceTypeDetailsRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listInstanceTypeDetailsPaginator(ListInstanceTypeDetailsRequest.builder()
.applyMutation(listInstanceTypeDetailsRequest).build());
}
/**
*
* Lists all packages associated with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param listPackagesForDomainRequest
* Container for the request parameters to the ListPackagesForDomain
operation.
* @return Result of the ListPackagesForDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListPackagesForDomain
*/
default ListPackagesForDomainResponse listPackagesForDomain(ListPackagesForDomainRequest listPackagesForDomainRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all packages associated with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link ListPackagesForDomainRequest.Builder} avoiding the
* need to create one manually via {@link ListPackagesForDomainRequest#builder()}
*
*
* @param listPackagesForDomainRequest
* A {@link Consumer} that will call methods on {@link ListPackagesForDomainRequest.Builder} to create a
* request. Container for the request parameters to the ListPackagesForDomain
operation.
* @return Result of the ListPackagesForDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListPackagesForDomain
*/
default ListPackagesForDomainResponse listPackagesForDomain(
Consumer listPackagesForDomainRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listPackagesForDomain(ListPackagesForDomainRequest.builder().applyMutation(listPackagesForDomainRequest).build());
}
/**
*
* Lists all packages associated with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #listPackagesForDomain(software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client.listPackagesForDomainPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client
* .listPackagesForDomainPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client.listPackagesForDomainPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackagesForDomain(software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest)}
* operation.
*
*
* @param listPackagesForDomainRequest
* Container for the request parameters to the ListPackagesForDomain
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListPackagesForDomain
*/
default ListPackagesForDomainIterable listPackagesForDomainPaginator(ListPackagesForDomainRequest listPackagesForDomainRequest)
throws BaseException, InternalException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all packages associated with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a variant of
* {@link #listPackagesForDomain(software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client.listPackagesForDomainPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client
* .listPackagesForDomainPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListPackagesForDomainIterable responses = client.listPackagesForDomainPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPackagesForDomain(software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPackagesForDomainRequest.Builder} avoiding the
* need to create one manually via {@link ListPackagesForDomainRequest#builder()}
*
*
* @param listPackagesForDomainRequest
* A {@link Consumer} that will call methods on {@link ListPackagesForDomainRequest.Builder} to create a
* request. Container for the request parameters to the ListPackagesForDomain
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListPackagesForDomain
*/
default ListPackagesForDomainIterable listPackagesForDomainPaginator(
Consumer listPackagesForDomainRequest) throws BaseException, InternalException,
ResourceNotFoundException, AccessDeniedException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listPackagesForDomainPaginator(ListPackagesForDomainRequest.builder().applyMutation(listPackagesForDomainRequest)
.build());
}
/**
*
* Retrieves a list of configuration changes that are scheduled for a domain. These changes can be service
* software updates or blue/green Auto-Tune enhancements.
*
*
* @param listScheduledActionsRequest
* @return Result of the ListScheduledActions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListScheduledActions
*/
default ListScheduledActionsResponse listScheduledActions(ListScheduledActionsRequest listScheduledActionsRequest)
throws BaseException, InternalException, ResourceNotFoundException, InvalidPaginationTokenException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of configuration changes that are scheduled for a domain. These changes can be service
* software updates or blue/green Auto-Tune enhancements.
*
*
*
* This is a convenience which creates an instance of the {@link ListScheduledActionsRequest.Builder} avoiding the
* need to create one manually via {@link ListScheduledActionsRequest#builder()}
*
*
* @param listScheduledActionsRequest
* A {@link Consumer} that will call methods on {@link ListScheduledActionsRequest.Builder} to create a
* request.
* @return Result of the ListScheduledActions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListScheduledActions
*/
default ListScheduledActionsResponse listScheduledActions(
Consumer listScheduledActionsRequest) throws BaseException, InternalException,
ResourceNotFoundException, InvalidPaginationTokenException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return listScheduledActions(ListScheduledActionsRequest.builder().applyMutation(listScheduledActionsRequest).build());
}
/**
*
* Retrieves a list of configuration changes that are scheduled for a domain. These changes can be service
* software updates or blue/green Auto-Tune enhancements.
*
*
*
* This is a variant of
* {@link #listScheduledActions(software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client.listScheduledActionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client
* .listScheduledActionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListScheduledActionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client.listScheduledActionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScheduledActions(software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest)}
* operation.
*
*
* @param listScheduledActionsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListScheduledActions
*/
default ListScheduledActionsIterable listScheduledActionsPaginator(ListScheduledActionsRequest listScheduledActionsRequest)
throws BaseException, InternalException, ResourceNotFoundException, InvalidPaginationTokenException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves a list of configuration changes that are scheduled for a domain. These changes can be service
* software updates or blue/green Auto-Tune enhancements.
*
*
*
* This is a variant of
* {@link #listScheduledActions(software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client.listScheduledActionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client
* .listScheduledActionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListScheduledActionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListScheduledActionsIterable responses = client.listScheduledActionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScheduledActions(software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListScheduledActionsRequest.Builder} avoiding the
* need to create one manually via {@link ListScheduledActionsRequest#builder()}
*
*
* @param listScheduledActionsRequest
* A {@link Consumer} that will call methods on {@link ListScheduledActionsRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws InvalidPaginationTokenException
* The request processing has failed because you provided an invalid pagination token.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListScheduledActions
*/
default ListScheduledActionsIterable listScheduledActionsPaginator(
Consumer listScheduledActionsRequest) throws BaseException, InternalException,
ResourceNotFoundException, InvalidPaginationTokenException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return listScheduledActionsPaginator(ListScheduledActionsRequest.builder().applyMutation(listScheduledActionsRequest)
.build());
}
/**
*
* Returns all resource tags for an Amazon OpenSearch Service domain. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
* @param listTagsRequest
* Container for the parameters to the ListTags
operation.
* @return Result of the ListTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListTags
*/
default ListTagsResponse listTags(ListTagsRequest listTagsRequest) throws BaseException, ResourceNotFoundException,
ValidationException, InternalException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Returns all resource tags for an Amazon OpenSearch Service domain. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on {@link ListTagsRequest.Builder} to create a request.
* Container for the parameters to the ListTags
operation.
* @return Result of the ListTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListTags
*/
default ListTagsResponse listTags(Consumer listTagsRequest) throws BaseException,
ResourceNotFoundException, ValidationException, InternalException, AwsServiceException, SdkClientException,
OpenSearchException {
return listTags(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* Lists all versions of OpenSearch and Elasticsearch that Amazon OpenSearch Service supports.
*
*
* @param listVersionsRequest
* Container for the request parameters to the ListVersions
operation.
* @return Result of the ListVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVersions
*/
default ListVersionsResponse listVersions(ListVersionsRequest listVersionsRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all versions of OpenSearch and Elasticsearch that Amazon OpenSearch Service supports.
*
*
*
* This is a convenience which creates an instance of the {@link ListVersionsRequest.Builder} avoiding the need to
* create one manually via {@link ListVersionsRequest#builder()}
*
*
* @param listVersionsRequest
* A {@link Consumer} that will call methods on {@link ListVersionsRequest.Builder} to create a request.
* Container for the request parameters to the ListVersions
operation.
* @return Result of the ListVersions operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVersions
*/
default ListVersionsResponse listVersions(Consumer listVersionsRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return listVersions(ListVersionsRequest.builder().applyMutation(listVersionsRequest).build());
}
/**
*
* Lists all versions of OpenSearch and Elasticsearch that Amazon OpenSearch Service supports.
*
*
*
* This is a variant of {@link #listVersions(software.amazon.awssdk.services.opensearch.model.ListVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVersions(software.amazon.awssdk.services.opensearch.model.ListVersionsRequest)} operation.
*
*
* @param listVersionsRequest
* Container for the request parameters to the ListVersions
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVersions
*/
default ListVersionsIterable listVersionsPaginator(ListVersionsRequest listVersionsRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Lists all versions of OpenSearch and Elasticsearch that Amazon OpenSearch Service supports.
*
*
*
* This is a variant of {@link #listVersions(software.amazon.awssdk.services.opensearch.model.ListVersionsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* for (software.amazon.awssdk.services.opensearch.model.ListVersionsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opensearch.paginators.ListVersionsIterable responses = client.listVersionsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listVersions(software.amazon.awssdk.services.opensearch.model.ListVersionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListVersionsRequest.Builder} avoiding the need to
* create one manually via {@link ListVersionsRequest#builder()}
*
*
* @param listVersionsRequest
* A {@link Consumer} that will call methods on {@link ListVersionsRequest.Builder} to create a request.
* Container for the request parameters to the ListVersions
operation.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVersions
*/
default ListVersionsIterable listVersionsPaginator(Consumer listVersionsRequest)
throws BaseException, InternalException, ResourceNotFoundException, ValidationException, AwsServiceException,
SdkClientException, OpenSearchException {
return listVersionsPaginator(ListVersionsRequest.builder().applyMutation(listVersionsRequest).build());
}
/**
*
* Retrieves information about each Amazon Web Services principal that is allowed to access a given Amazon
* OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
* @param listVpcEndpointAccessRequest
* @return Result of the ListVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpointAccess
*/
default ListVpcEndpointAccessResponse listVpcEndpointAccess(ListVpcEndpointAccessRequest listVpcEndpointAccessRequest)
throws ResourceNotFoundException, DisabledOperationException, InternalException, BaseException, AwsServiceException,
SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about each Amazon Web Services principal that is allowed to access a given Amazon
* OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link ListVpcEndpointAccessRequest.Builder} avoiding the
* need to create one manually via {@link ListVpcEndpointAccessRequest#builder()}
*
*
* @param listVpcEndpointAccessRequest
* A {@link Consumer} that will call methods on {@link ListVpcEndpointAccessRequest.Builder} to create a
* request.
* @return Result of the ListVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpointAccess
*/
default ListVpcEndpointAccessResponse listVpcEndpointAccess(
Consumer listVpcEndpointAccessRequest) throws ResourceNotFoundException,
DisabledOperationException, InternalException, BaseException, AwsServiceException, SdkClientException,
OpenSearchException {
return listVpcEndpointAccess(ListVpcEndpointAccessRequest.builder().applyMutation(listVpcEndpointAccessRequest).build());
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints in the current Amazon Web Services account and
* Region.
*
*
* @param listVpcEndpointsRequest
* @return Result of the ListVpcEndpoints operation returned by the service.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpoints
*/
default ListVpcEndpointsResponse listVpcEndpoints(ListVpcEndpointsRequest listVpcEndpointsRequest) throws InternalException,
DisabledOperationException, BaseException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints in the current Amazon Web Services account and
* Region.
*
*
*
* This is a convenience which creates an instance of the {@link ListVpcEndpointsRequest.Builder} avoiding the need
* to create one manually via {@link ListVpcEndpointsRequest#builder()}
*
*
* @param listVpcEndpointsRequest
* A {@link Consumer} that will call methods on {@link ListVpcEndpointsRequest.Builder} to create a request.
* @return Result of the ListVpcEndpoints operation returned by the service.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpoints
*/
default ListVpcEndpointsResponse listVpcEndpoints(Consumer listVpcEndpointsRequest)
throws InternalException, DisabledOperationException, BaseException, AwsServiceException, SdkClientException,
OpenSearchException {
return listVpcEndpoints(ListVpcEndpointsRequest.builder().applyMutation(listVpcEndpointsRequest).build());
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints associated with a particular domain.
*
*
* @param listVpcEndpointsForDomainRequest
* @return Result of the ListVpcEndpointsForDomain operation returned by the service.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpointsForDomain
*/
default ListVpcEndpointsForDomainResponse listVpcEndpointsForDomain(
ListVpcEndpointsForDomainRequest listVpcEndpointsForDomainRequest) throws InternalException,
DisabledOperationException, ResourceNotFoundException, BaseException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves all Amazon OpenSearch Service-managed VPC endpoints associated with a particular domain.
*
*
*
* This is a convenience which creates an instance of the {@link ListVpcEndpointsForDomainRequest.Builder} avoiding
* the need to create one manually via {@link ListVpcEndpointsForDomainRequest#builder()}
*
*
* @param listVpcEndpointsForDomainRequest
* A {@link Consumer} that will call methods on {@link ListVpcEndpointsForDomainRequest.Builder} to create a
* request.
* @return Result of the ListVpcEndpointsForDomain operation returned by the service.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.ListVpcEndpointsForDomain
*/
default ListVpcEndpointsForDomainResponse listVpcEndpointsForDomain(
Consumer listVpcEndpointsForDomainRequest) throws InternalException,
DisabledOperationException, ResourceNotFoundException, BaseException, AwsServiceException, SdkClientException,
OpenSearchException {
return listVpcEndpointsForDomain(ListVpcEndpointsForDomainRequest.builder()
.applyMutation(listVpcEndpointsForDomainRequest).build());
}
/**
*
* Allows you to purchase Amazon OpenSearch Service Reserved Instances.
*
*
* @param purchaseReservedInstanceOfferingRequest
* Container for request parameters to the PurchaseReservedInstanceOffering
operation.
* @return Result of the PurchaseReservedInstanceOffering operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.PurchaseReservedInstanceOffering
*/
default PurchaseReservedInstanceOfferingResponse purchaseReservedInstanceOffering(
PurchaseReservedInstanceOfferingRequest purchaseReservedInstanceOfferingRequest) throws ResourceNotFoundException,
ResourceAlreadyExistsException, LimitExceededException, DisabledOperationException, ValidationException,
InternalException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to purchase Amazon OpenSearch Service Reserved Instances.
*
*
*
* This is a convenience which creates an instance of the {@link PurchaseReservedInstanceOfferingRequest.Builder}
* avoiding the need to create one manually via {@link PurchaseReservedInstanceOfferingRequest#builder()}
*
*
* @param purchaseReservedInstanceOfferingRequest
* A {@link Consumer} that will call methods on {@link PurchaseReservedInstanceOfferingRequest.Builder} to
* create a request. Container for request parameters to the PurchaseReservedInstanceOffering
* operation.
* @return Result of the PurchaseReservedInstanceOffering operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.PurchaseReservedInstanceOffering
*/
default PurchaseReservedInstanceOfferingResponse purchaseReservedInstanceOffering(
Consumer purchaseReservedInstanceOfferingRequest)
throws ResourceNotFoundException, ResourceAlreadyExistsException, LimitExceededException, DisabledOperationException,
ValidationException, InternalException, AwsServiceException, SdkClientException, OpenSearchException {
return purchaseReservedInstanceOffering(PurchaseReservedInstanceOfferingRequest.builder()
.applyMutation(purchaseReservedInstanceOfferingRequest).build());
}
/**
*
* Allows the remote Amazon OpenSearch Service domain owner to reject an inbound cross-cluster connection request.
*
*
* @param rejectInboundConnectionRequest
* Container for the request parameters to the RejectInboundConnection
operation.
* @return Result of the RejectInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RejectInboundConnection
*/
default RejectInboundConnectionResponse rejectInboundConnection(RejectInboundConnectionRequest rejectInboundConnectionRequest)
throws ResourceNotFoundException, DisabledOperationException, AwsServiceException, SdkClientException,
OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows the remote Amazon OpenSearch Service domain owner to reject an inbound cross-cluster connection request.
*
*
*
* This is a convenience which creates an instance of the {@link RejectInboundConnectionRequest.Builder} avoiding
* the need to create one manually via {@link RejectInboundConnectionRequest#builder()}
*
*
* @param rejectInboundConnectionRequest
* A {@link Consumer} that will call methods on {@link RejectInboundConnectionRequest.Builder} to create a
* request. Container for the request parameters to the RejectInboundConnection
operation.
* @return Result of the RejectInboundConnection operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RejectInboundConnection
*/
default RejectInboundConnectionResponse rejectInboundConnection(
Consumer rejectInboundConnectionRequest) throws ResourceNotFoundException,
DisabledOperationException, AwsServiceException, SdkClientException, OpenSearchException {
return rejectInboundConnection(RejectInboundConnectionRequest.builder().applyMutation(rejectInboundConnectionRequest)
.build());
}
/**
*
* Removes the specified set of tags from an Amazon OpenSearch Service domain. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
* @param removeTagsRequest
* Container for the request parameters to the RemoveTags
operation.
* @return Result of the RemoveTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RemoveTags
*/
default RemoveTagsResponse removeTags(RemoveTagsRequest removeTagsRequest) throws BaseException, ValidationException,
InternalException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Removes the specified set of tags from an Amazon OpenSearch Service domain. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
*
* This is a convenience which creates an instance of the {@link RemoveTagsRequest.Builder} avoiding the need to
* create one manually via {@link RemoveTagsRequest#builder()}
*
*
* @param removeTagsRequest
* A {@link Consumer} that will call methods on {@link RemoveTagsRequest.Builder} to create a request.
* Container for the request parameters to the RemoveTags
operation.
* @return Result of the RemoveTags operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RemoveTags
*/
default RemoveTagsResponse removeTags(Consumer removeTagsRequest) throws BaseException,
ValidationException, InternalException, AwsServiceException, SdkClientException, OpenSearchException {
return removeTags(RemoveTagsRequest.builder().applyMutation(removeTagsRequest).build());
}
/**
*
* Revokes access to an Amazon OpenSearch Service domain that was provided through an interface VPC endpoint.
*
*
* @param revokeVpcEndpointAccessRequest
* @return Result of the RevokeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RevokeVpcEndpointAccess
*/
default RevokeVpcEndpointAccessResponse revokeVpcEndpointAccess(RevokeVpcEndpointAccessRequest revokeVpcEndpointAccessRequest)
throws ResourceNotFoundException, ValidationException, DisabledOperationException, InternalException, BaseException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Revokes access to an Amazon OpenSearch Service domain that was provided through an interface VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link RevokeVpcEndpointAccessRequest.Builder} avoiding
* the need to create one manually via {@link RevokeVpcEndpointAccessRequest#builder()}
*
*
* @param revokeVpcEndpointAccessRequest
* A {@link Consumer} that will call methods on {@link RevokeVpcEndpointAccessRequest.Builder} to create a
* request.
* @return Result of the RevokeVpcEndpointAccess operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.RevokeVpcEndpointAccess
*/
default RevokeVpcEndpointAccessResponse revokeVpcEndpointAccess(
Consumer revokeVpcEndpointAccessRequest) throws ResourceNotFoundException,
ValidationException, DisabledOperationException, InternalException, BaseException, AwsServiceException,
SdkClientException, OpenSearchException {
return revokeVpcEndpointAccess(RevokeVpcEndpointAccessRequest.builder().applyMutation(revokeVpcEndpointAccessRequest)
.build());
}
/**
*
* Schedules a service software update for an Amazon OpenSearch Service domain. For more information, see Service
* software updates in Amazon OpenSearch Service.
*
*
* @param startServiceSoftwareUpdateRequest
* Container for the request parameters to the StartServiceSoftwareUpdate
operation.
* @return Result of the StartServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.StartServiceSoftwareUpdate
*/
default StartServiceSoftwareUpdateResponse startServiceSoftwareUpdate(
StartServiceSoftwareUpdateRequest startServiceSoftwareUpdateRequest) throws BaseException, InternalException,
ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Schedules a service software update for an Amazon OpenSearch Service domain. For more information, see Service
* software updates in Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link StartServiceSoftwareUpdateRequest.Builder} avoiding
* the need to create one manually via {@link StartServiceSoftwareUpdateRequest#builder()}
*
*
* @param startServiceSoftwareUpdateRequest
* A {@link Consumer} that will call methods on {@link StartServiceSoftwareUpdateRequest.Builder} to create a
* request. Container for the request parameters to the StartServiceSoftwareUpdate
operation.
* @return Result of the StartServiceSoftwareUpdate operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.StartServiceSoftwareUpdate
*/
default StartServiceSoftwareUpdateResponse startServiceSoftwareUpdate(
Consumer startServiceSoftwareUpdateRequest) throws BaseException,
InternalException, ResourceNotFoundException, ValidationException, AwsServiceException, SdkClientException,
OpenSearchException {
return startServiceSoftwareUpdate(StartServiceSoftwareUpdateRequest.builder()
.applyMutation(startServiceSoftwareUpdateRequest).build());
}
/**
*
* Modifies the cluster configuration of the specified Amazon OpenSearch Service domain.
*
*
* @param updateDomainConfigRequest
* Container for the request parameters to the UpdateDomain
operation.
* @return Result of the UpdateDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateDomainConfig
*/
default UpdateDomainConfigResponse updateDomainConfig(UpdateDomainConfigRequest updateDomainConfigRequest)
throws BaseException, InternalException, InvalidTypeException, LimitExceededException, ResourceNotFoundException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Modifies the cluster configuration of the specified Amazon OpenSearch Service domain.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateDomainConfigRequest.Builder} avoiding the
* need to create one manually via {@link UpdateDomainConfigRequest#builder()}
*
*
* @param updateDomainConfigRequest
* A {@link Consumer} that will call methods on {@link UpdateDomainConfigRequest.Builder} to create a
* request. Container for the request parameters to the UpdateDomain
operation.
* @return Result of the UpdateDomainConfig operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws InvalidTypeException
* An exception for trying to create or access a sub-resource that's either invalid or not supported.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateDomainConfig
*/
default UpdateDomainConfigResponse updateDomainConfig(Consumer updateDomainConfigRequest)
throws BaseException, InternalException, InvalidTypeException, LimitExceededException, ResourceNotFoundException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
return updateDomainConfig(UpdateDomainConfigRequest.builder().applyMutation(updateDomainConfigRequest).build());
}
/**
*
* Updates a package for use with Amazon OpenSearch Service domains. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param updatePackageRequest
* Container for request parameters to the UpdatePackage
operation.
* @return Result of the UpdatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdatePackage
*/
default UpdatePackageResponse updatePackage(UpdatePackageRequest updatePackageRequest) throws BaseException,
InternalException, LimitExceededException, ResourceNotFoundException, AccessDeniedException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a package for use with Amazon OpenSearch Service domains. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
*
* This is a convenience which creates an instance of the {@link UpdatePackageRequest.Builder} avoiding the need to
* create one manually via {@link UpdatePackageRequest#builder()}
*
*
* @param updatePackageRequest
* A {@link Consumer} that will call methods on {@link UpdatePackageRequest.Builder} to create a request.
* Container for request parameters to the UpdatePackage
operation.
* @return Result of the UpdatePackage operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws AccessDeniedException
* An error occurred because you don't have permissions to access the resource.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdatePackage
*/
default UpdatePackageResponse updatePackage(Consumer updatePackageRequest)
throws BaseException, InternalException, LimitExceededException, ResourceNotFoundException, AccessDeniedException,
ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
return updatePackage(UpdatePackageRequest.builder().applyMutation(updatePackageRequest).build());
}
/**
*
* Reschedules a planned domain configuration change for a later time. This change can be a scheduled service
* software update or a blue/green Auto-Tune enhancement.
*
*
* @param updateScheduledActionRequest
* @return Result of the UpdateScheduledAction operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws SlotNotAvailableException
* An exception for attempting to schedule a domain action during an unavailable time slot.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateScheduledAction
*/
default UpdateScheduledActionResponse updateScheduledAction(UpdateScheduledActionRequest updateScheduledActionRequest)
throws BaseException, InternalException, ResourceNotFoundException, SlotNotAvailableException, ConflictException,
LimitExceededException, ValidationException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Reschedules a planned domain configuration change for a later time. This change can be a scheduled service
* software update or a blue/green Auto-Tune enhancement.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateScheduledActionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateScheduledActionRequest#builder()}
*
*
* @param updateScheduledActionRequest
* A {@link Consumer} that will call methods on {@link UpdateScheduledActionRequest.Builder} to create a
* request.
* @return Result of the UpdateScheduledAction operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws SlotNotAvailableException
* An exception for attempting to schedule a domain action during an unavailable time slot.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws LimitExceededException
* An exception for trying to create more than the allowed number of resources or sub-resources.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateScheduledAction
*/
default UpdateScheduledActionResponse updateScheduledAction(
Consumer updateScheduledActionRequest) throws BaseException, InternalException,
ResourceNotFoundException, SlotNotAvailableException, ConflictException, LimitExceededException, ValidationException,
AwsServiceException, SdkClientException, OpenSearchException {
return updateScheduledAction(UpdateScheduledActionRequest.builder().applyMutation(updateScheduledActionRequest).build());
}
/**
*
* Modifies an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
* @param updateVpcEndpointRequest
* @return Result of the UpdateVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateVpcEndpoint
*/
default UpdateVpcEndpointResponse updateVpcEndpoint(UpdateVpcEndpointRequest updateVpcEndpointRequest)
throws ResourceNotFoundException, DisabledOperationException, InternalException, ValidationException,
ConflictException, BaseException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Modifies an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVpcEndpointRequest.Builder} avoiding the need
* to create one manually via {@link UpdateVpcEndpointRequest#builder()}
*
*
* @param updateVpcEndpointRequest
* A {@link Consumer} that will call methods on {@link UpdateVpcEndpointRequest.Builder} to create a request.
* @return Result of the UpdateVpcEndpoint operation returned by the service.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws ConflictException
* An error occurred because the client attempts to remove a resource that is currently in use.
* @throws BaseException
* An error occurred while processing the request.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpdateVpcEndpoint
*/
default UpdateVpcEndpointResponse updateVpcEndpoint(Consumer updateVpcEndpointRequest)
throws ResourceNotFoundException, DisabledOperationException, InternalException, ValidationException,
ConflictException, BaseException, AwsServiceException, SdkClientException, OpenSearchException {
return updateVpcEndpoint(UpdateVpcEndpointRequest.builder().applyMutation(updateVpcEndpointRequest).build());
}
/**
*
* Allows you to either upgrade your Amazon OpenSearch Service domain or perform an upgrade eligibility check to a
* compatible version of OpenSearch or Elasticsearch.
*
*
* @param upgradeDomainRequest
* Container for the request parameters to the UpgradeDomain
operation.
* @return Result of the UpgradeDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpgradeDomain
*/
default UpgradeDomainResponse upgradeDomain(UpgradeDomainRequest upgradeDomainRequest) throws BaseException,
ResourceNotFoundException, ResourceAlreadyExistsException, DisabledOperationException, ValidationException,
InternalException, AwsServiceException, SdkClientException, OpenSearchException {
throw new UnsupportedOperationException();
}
/**
*
* Allows you to either upgrade your Amazon OpenSearch Service domain or perform an upgrade eligibility check to a
* compatible version of OpenSearch or Elasticsearch.
*
*
*
* This is a convenience which creates an instance of the {@link UpgradeDomainRequest.Builder} avoiding the need to
* create one manually via {@link UpgradeDomainRequest#builder()}
*
*
* @param upgradeDomainRequest
* A {@link Consumer} that will call methods on {@link UpgradeDomainRequest.Builder} to create a request.
* Container for the request parameters to the UpgradeDomain
operation.
* @return Result of the UpgradeDomain operation returned by the service.
* @throws BaseException
* An error occurred while processing the request.
* @throws ResourceNotFoundException
* An exception for accessing or deleting a resource that does not exist..
* @throws ResourceAlreadyExistsException
* An exception for creating a resource that already exists.
* @throws DisabledOperationException
* An error occured because the client wanted to access a not supported operation.
* @throws ValidationException
* An exception for accessing or deleting a resource that doesn't exist.
* @throws InternalException
* Request processing failed because of an unknown error, exception, or internal failure.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpenSearchException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpenSearchClient.UpgradeDomain
*/
default UpgradeDomainResponse upgradeDomain(Consumer upgradeDomainRequest)
throws BaseException, ResourceNotFoundException, ResourceAlreadyExistsException, DisabledOperationException,
ValidationException, InternalException, AwsServiceException, SdkClientException, OpenSearchException {
return upgradeDomain(UpgradeDomainRequest.builder().applyMutation(upgradeDomainRequest).build());
}
/**
* Create a {@link OpenSearchClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static OpenSearchClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link OpenSearchClient}.
*/
static OpenSearchClientBuilder builder() {
return new DefaultOpenSearchClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}