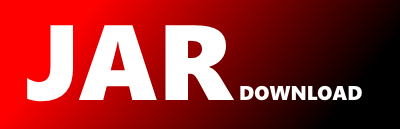
software.amazon.awssdk.services.opensearch.model.AvailabilityZoneInfo Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about an Availability Zone on a domain.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class AvailabilityZoneInfo implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField AVAILABILITY_ZONE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZoneName").getter(getter(AvailabilityZoneInfo::availabilityZoneName))
.setter(setter(Builder::availabilityZoneName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZoneName").build())
.build();
private static final SdkField ZONE_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ZoneStatus").getter(getter(AvailabilityZoneInfo::zoneStatusAsString))
.setter(setter(Builder::zoneStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ZoneStatus").build()).build();
private static final SdkField CONFIGURED_DATA_NODE_COUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ConfiguredDataNodeCount").getter(getter(AvailabilityZoneInfo::configuredDataNodeCount))
.setter(setter(Builder::configuredDataNodeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConfiguredDataNodeCount").build())
.build();
private static final SdkField AVAILABLE_DATA_NODE_COUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailableDataNodeCount").getter(getter(AvailabilityZoneInfo::availableDataNodeCount))
.setter(setter(Builder::availableDataNodeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailableDataNodeCount").build())
.build();
private static final SdkField TOTAL_SHARDS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalShards").getter(getter(AvailabilityZoneInfo::totalShards)).setter(setter(Builder::totalShards))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalShards").build()).build();
private static final SdkField TOTAL_UN_ASSIGNED_SHARDS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TotalUnAssignedShards").getter(getter(AvailabilityZoneInfo::totalUnAssignedShards))
.setter(setter(Builder::totalUnAssignedShards))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TotalUnAssignedShards").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AVAILABILITY_ZONE_NAME_FIELD,
ZONE_STATUS_FIELD, CONFIGURED_DATA_NODE_COUNT_FIELD, AVAILABLE_DATA_NODE_COUNT_FIELD, TOTAL_SHARDS_FIELD,
TOTAL_UN_ASSIGNED_SHARDS_FIELD));
private static final long serialVersionUID = 1L;
private final String availabilityZoneName;
private final String zoneStatus;
private final String configuredDataNodeCount;
private final String availableDataNodeCount;
private final String totalShards;
private final String totalUnAssignedShards;
private AvailabilityZoneInfo(BuilderImpl builder) {
this.availabilityZoneName = builder.availabilityZoneName;
this.zoneStatus = builder.zoneStatus;
this.configuredDataNodeCount = builder.configuredDataNodeCount;
this.availableDataNodeCount = builder.availableDataNodeCount;
this.totalShards = builder.totalShards;
this.totalUnAssignedShards = builder.totalUnAssignedShards;
}
/**
*
* The name of the Availability Zone.
*
*
* @return The name of the Availability Zone.
*/
public final String availabilityZoneName() {
return availabilityZoneName;
}
/**
*
* The current state of the Availability Zone. Current options are Active
and StandBy
.
*
*
* -
*
* Active
- Data nodes in the Availability Zone are in use.
*
*
* -
*
* StandBy
- Data nodes in the Availability Zone are in a standby state.
*
*
* -
*
* NotAvailable
- Unable to retrieve information.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #zoneStatus} will
* return {@link ZoneStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #zoneStatusAsString}.
*
*
* @return The current state of the Availability Zone. Current options are Active
and
* StandBy
.
*
* -
*
* Active
- Data nodes in the Availability Zone are in use.
*
*
* -
*
* StandBy
- Data nodes in the Availability Zone are in a standby state.
*
*
* -
*
* NotAvailable
- Unable to retrieve information.
*
*
* @see ZoneStatus
*/
public final ZoneStatus zoneStatus() {
return ZoneStatus.fromValue(zoneStatus);
}
/**
*
* The current state of the Availability Zone. Current options are Active
and StandBy
.
*
*
* -
*
* Active
- Data nodes in the Availability Zone are in use.
*
*
* -
*
* StandBy
- Data nodes in the Availability Zone are in a standby state.
*
*
* -
*
* NotAvailable
- Unable to retrieve information.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #zoneStatus} will
* return {@link ZoneStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #zoneStatusAsString}.
*
*
* @return The current state of the Availability Zone. Current options are Active
and
* StandBy
.
*
* -
*
* Active
- Data nodes in the Availability Zone are in use.
*
*
* -
*
* StandBy
- Data nodes in the Availability Zone are in a standby state.
*
*
* -
*
* NotAvailable
- Unable to retrieve information.
*
*
* @see ZoneStatus
*/
public final String zoneStatusAsString() {
return zoneStatus;
}
/**
*
* The total number of data nodes configured in the Availability Zone.
*
*
* @return The total number of data nodes configured in the Availability Zone.
*/
public final String configuredDataNodeCount() {
return configuredDataNodeCount;
}
/**
*
* The number of data nodes active in the Availability Zone.
*
*
* @return The number of data nodes active in the Availability Zone.
*/
public final String availableDataNodeCount() {
return availableDataNodeCount;
}
/**
*
* The total number of primary and replica shards in the Availability Zone.
*
*
* @return The total number of primary and replica shards in the Availability Zone.
*/
public final String totalShards() {
return totalShards;
}
/**
*
* The total number of primary and replica shards that aren't allocated to any of the nodes in the Availability
* Zone.
*
*
* @return The total number of primary and replica shards that aren't allocated to any of the nodes in the
* Availability Zone.
*/
public final String totalUnAssignedShards() {
return totalUnAssignedShards;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(availabilityZoneName());
hashCode = 31 * hashCode + Objects.hashCode(zoneStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(configuredDataNodeCount());
hashCode = 31 * hashCode + Objects.hashCode(availableDataNodeCount());
hashCode = 31 * hashCode + Objects.hashCode(totalShards());
hashCode = 31 * hashCode + Objects.hashCode(totalUnAssignedShards());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof AvailabilityZoneInfo)) {
return false;
}
AvailabilityZoneInfo other = (AvailabilityZoneInfo) obj;
return Objects.equals(availabilityZoneName(), other.availabilityZoneName())
&& Objects.equals(zoneStatusAsString(), other.zoneStatusAsString())
&& Objects.equals(configuredDataNodeCount(), other.configuredDataNodeCount())
&& Objects.equals(availableDataNodeCount(), other.availableDataNodeCount())
&& Objects.equals(totalShards(), other.totalShards())
&& Objects.equals(totalUnAssignedShards(), other.totalUnAssignedShards());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("AvailabilityZoneInfo").add("AvailabilityZoneName", availabilityZoneName())
.add("ZoneStatus", zoneStatusAsString()).add("ConfiguredDataNodeCount", configuredDataNodeCount())
.add("AvailableDataNodeCount", availableDataNodeCount()).add("TotalShards", totalShards())
.add("TotalUnAssignedShards", totalUnAssignedShards()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AvailabilityZoneName":
return Optional.ofNullable(clazz.cast(availabilityZoneName()));
case "ZoneStatus":
return Optional.ofNullable(clazz.cast(zoneStatusAsString()));
case "ConfiguredDataNodeCount":
return Optional.ofNullable(clazz.cast(configuredDataNodeCount()));
case "AvailableDataNodeCount":
return Optional.ofNullable(clazz.cast(availableDataNodeCount()));
case "TotalShards":
return Optional.ofNullable(clazz.cast(totalShards()));
case "TotalUnAssignedShards":
return Optional.ofNullable(clazz.cast(totalUnAssignedShards()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function