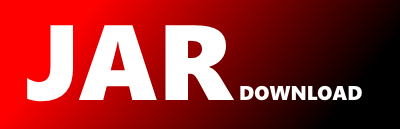
software.amazon.awssdk.services.opensearch.DefaultOpenSearchAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opensearch;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.opensearch.internal.OpenSearchServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.opensearch.model.AcceptInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.AcceptInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.AccessDeniedException;
import software.amazon.awssdk.services.opensearch.model.AddDataSourceRequest;
import software.amazon.awssdk.services.opensearch.model.AddDataSourceResponse;
import software.amazon.awssdk.services.opensearch.model.AddTagsRequest;
import software.amazon.awssdk.services.opensearch.model.AddTagsResponse;
import software.amazon.awssdk.services.opensearch.model.AssociatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.AssociatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.AuthorizeVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.AuthorizeVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.BaseException;
import software.amazon.awssdk.services.opensearch.model.CancelDomainConfigChangeRequest;
import software.amazon.awssdk.services.opensearch.model.CancelDomainConfigChangeResponse;
import software.amazon.awssdk.services.opensearch.model.CancelServiceSoftwareUpdateRequest;
import software.amazon.awssdk.services.opensearch.model.CancelServiceSoftwareUpdateResponse;
import software.amazon.awssdk.services.opensearch.model.ConflictException;
import software.amazon.awssdk.services.opensearch.model.CreateDomainRequest;
import software.amazon.awssdk.services.opensearch.model.CreateDomainResponse;
import software.amazon.awssdk.services.opensearch.model.CreateOutboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.CreateOutboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.CreatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.CreatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.CreateVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.CreateVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteDataSourceRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteDataSourceResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteDomainRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteDomainResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteOutboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteOutboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.DeletePackageRequest;
import software.amazon.awssdk.services.opensearch.model.DeletePackageResponse;
import software.amazon.awssdk.services.opensearch.model.DeleteVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.DeleteVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.DependencyFailureException;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainAutoTunesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainChangeProgressRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainChangeProgressResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainConfigRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainConfigResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainHealthRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainHealthResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainNodesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainNodesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDomainsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeDryRunProgressRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeDryRunProgressResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeInboundConnectionsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeInstanceTypeLimitsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeInstanceTypeLimitsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeOutboundConnectionsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribePackagesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribePackagesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstanceOfferingsResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeReservedInstancesResponse;
import software.amazon.awssdk.services.opensearch.model.DescribeVpcEndpointsRequest;
import software.amazon.awssdk.services.opensearch.model.DescribeVpcEndpointsResponse;
import software.amazon.awssdk.services.opensearch.model.DisabledOperationException;
import software.amazon.awssdk.services.opensearch.model.DissociatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.DissociatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.GetCompatibleVersionsRequest;
import software.amazon.awssdk.services.opensearch.model.GetCompatibleVersionsResponse;
import software.amazon.awssdk.services.opensearch.model.GetDataSourceRequest;
import software.amazon.awssdk.services.opensearch.model.GetDataSourceResponse;
import software.amazon.awssdk.services.opensearch.model.GetDomainMaintenanceStatusRequest;
import software.amazon.awssdk.services.opensearch.model.GetDomainMaintenanceStatusResponse;
import software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryRequest;
import software.amazon.awssdk.services.opensearch.model.GetPackageVersionHistoryResponse;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryRequest;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeHistoryResponse;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeStatusRequest;
import software.amazon.awssdk.services.opensearch.model.GetUpgradeStatusResponse;
import software.amazon.awssdk.services.opensearch.model.InternalException;
import software.amazon.awssdk.services.opensearch.model.InvalidPaginationTokenException;
import software.amazon.awssdk.services.opensearch.model.InvalidTypeException;
import software.amazon.awssdk.services.opensearch.model.LimitExceededException;
import software.amazon.awssdk.services.opensearch.model.ListDataSourcesRequest;
import software.amazon.awssdk.services.opensearch.model.ListDataSourcesResponse;
import software.amazon.awssdk.services.opensearch.model.ListDomainMaintenancesRequest;
import software.amazon.awssdk.services.opensearch.model.ListDomainMaintenancesResponse;
import software.amazon.awssdk.services.opensearch.model.ListDomainNamesRequest;
import software.amazon.awssdk.services.opensearch.model.ListDomainNamesResponse;
import software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageRequest;
import software.amazon.awssdk.services.opensearch.model.ListDomainsForPackageResponse;
import software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsRequest;
import software.amazon.awssdk.services.opensearch.model.ListInstanceTypeDetailsResponse;
import software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainRequest;
import software.amazon.awssdk.services.opensearch.model.ListPackagesForDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ListScheduledActionsRequest;
import software.amazon.awssdk.services.opensearch.model.ListScheduledActionsResponse;
import software.amazon.awssdk.services.opensearch.model.ListTagsRequest;
import software.amazon.awssdk.services.opensearch.model.ListTagsResponse;
import software.amazon.awssdk.services.opensearch.model.ListVersionsRequest;
import software.amazon.awssdk.services.opensearch.model.ListVersionsResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsForDomainRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsForDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsRequest;
import software.amazon.awssdk.services.opensearch.model.ListVpcEndpointsResponse;
import software.amazon.awssdk.services.opensearch.model.OpenSearchException;
import software.amazon.awssdk.services.opensearch.model.PurchaseReservedInstanceOfferingRequest;
import software.amazon.awssdk.services.opensearch.model.PurchaseReservedInstanceOfferingResponse;
import software.amazon.awssdk.services.opensearch.model.RejectInboundConnectionRequest;
import software.amazon.awssdk.services.opensearch.model.RejectInboundConnectionResponse;
import software.amazon.awssdk.services.opensearch.model.RemoveTagsRequest;
import software.amazon.awssdk.services.opensearch.model.RemoveTagsResponse;
import software.amazon.awssdk.services.opensearch.model.ResourceAlreadyExistsException;
import software.amazon.awssdk.services.opensearch.model.ResourceNotFoundException;
import software.amazon.awssdk.services.opensearch.model.RevokeVpcEndpointAccessRequest;
import software.amazon.awssdk.services.opensearch.model.RevokeVpcEndpointAccessResponse;
import software.amazon.awssdk.services.opensearch.model.SlotNotAvailableException;
import software.amazon.awssdk.services.opensearch.model.StartDomainMaintenanceRequest;
import software.amazon.awssdk.services.opensearch.model.StartDomainMaintenanceResponse;
import software.amazon.awssdk.services.opensearch.model.StartServiceSoftwareUpdateRequest;
import software.amazon.awssdk.services.opensearch.model.StartServiceSoftwareUpdateResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateDataSourceRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateDataSourceResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateDomainConfigRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateDomainConfigResponse;
import software.amazon.awssdk.services.opensearch.model.UpdatePackageRequest;
import software.amazon.awssdk.services.opensearch.model.UpdatePackageResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateScheduledActionRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateScheduledActionResponse;
import software.amazon.awssdk.services.opensearch.model.UpdateVpcEndpointRequest;
import software.amazon.awssdk.services.opensearch.model.UpdateVpcEndpointResponse;
import software.amazon.awssdk.services.opensearch.model.UpgradeDomainRequest;
import software.amazon.awssdk.services.opensearch.model.UpgradeDomainResponse;
import software.amazon.awssdk.services.opensearch.model.ValidationException;
import software.amazon.awssdk.services.opensearch.transform.AcceptInboundConnectionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.AddDataSourceRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.AddTagsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.AssociatePackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.AuthorizeVpcEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CancelDomainConfigChangeRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CancelServiceSoftwareUpdateRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CreateDomainRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CreateOutboundConnectionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CreatePackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.CreateVpcEndpointRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeleteDataSourceRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeleteDomainRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeleteInboundConnectionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeleteOutboundConnectionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeletePackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DeleteVpcEndpointRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainAutoTunesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainChangeProgressRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainConfigRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainHealthRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainNodesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDomainsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeDryRunProgressRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeInboundConnectionsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeInstanceTypeLimitsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeOutboundConnectionsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribePackagesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeReservedInstanceOfferingsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeReservedInstancesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DescribeVpcEndpointsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.DissociatePackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetCompatibleVersionsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetDataSourceRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetDomainMaintenanceStatusRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetPackageVersionHistoryRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetUpgradeHistoryRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.GetUpgradeStatusRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListDataSourcesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListDomainMaintenancesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListDomainNamesRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListDomainsForPackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListInstanceTypeDetailsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListPackagesForDomainRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListScheduledActionsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListTagsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListVersionsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListVpcEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListVpcEndpointsForDomainRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.ListVpcEndpointsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.PurchaseReservedInstanceOfferingRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.RejectInboundConnectionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.RemoveTagsRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.RevokeVpcEndpointAccessRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.StartDomainMaintenanceRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.StartServiceSoftwareUpdateRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpdateDataSourceRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpdateDomainConfigRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpdatePackageRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpdateScheduledActionRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpdateVpcEndpointRequestMarshaller;
import software.amazon.awssdk.services.opensearch.transform.UpgradeDomainRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link OpenSearchAsyncClient}.
*
* @see OpenSearchAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultOpenSearchAsyncClient implements OpenSearchAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultOpenSearchAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultOpenSearchAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to accept an inbound cross-cluster search
* connection request. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param acceptInboundConnectionRequest
* Container for the parameters to the AcceptInboundConnection
operation.
* @return A Java Future containing the result of the AcceptInboundConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.AcceptInboundConnection
*/
@Override
public CompletableFuture acceptInboundConnection(
AcceptInboundConnectionRequest acceptInboundConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(acceptInboundConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, acceptInboundConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AcceptInboundConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AcceptInboundConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AcceptInboundConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AcceptInboundConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(acceptInboundConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new direct-query data source to the specified domain. For more information, see Creating Amazon OpenSearch Service data source integrations with Amazon S3.
*
*
* @param addDataSourceRequest
* Container for the parameters to the AddDataSource
operation.
* @return A Java Future containing the result of the AddDataSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - DependencyFailureException An exception for when a failure in one of the dependencies results in the
* service being unable to fetch details about the resource.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.AddDataSource
*/
@Override
public CompletableFuture addDataSource(AddDataSourceRequest addDataSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addDataSourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addDataSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddDataSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddDataSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AddDataSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AddDataSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addDataSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches tags to an existing Amazon OpenSearch Service domain. Tags are a set of case-sensitive key-value pairs.
* A domain can have up to 10 tags. For more information, see Tagging Amazon OpenSearch Service domains.
*
*
* @param addTagsRequest
* Container for the parameters to the AddTags
operation. Specifies the tags to attach to the
* domain.
* @return A Java Future containing the result of the AddTags operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.AddTags
*/
@Override
public CompletableFuture addTags(AddTagsRequest addTagsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(addTagsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, addTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AddTags");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AddTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("AddTags")
.withProtocolMetadata(protocolMetadata).withMarshaller(new AddTagsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(addTagsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associates a package with an Amazon OpenSearch Service domain. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param associatePackageRequest
* Container for the request parameters to the AssociatePackage
operation.
* @return A Java Future containing the result of the AssociatePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - AccessDeniedException An error occurred because you don't have permissions to access the resource.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - ConflictException An error occurred because the client attempts to remove a resource that is
* currently in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.AssociatePackage
*/
@Override
public CompletableFuture associatePackage(AssociatePackageRequest associatePackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associatePackageRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associatePackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociatePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociatePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociatePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociatePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associatePackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Provides access to an Amazon OpenSearch Service domain through the use of an interface VPC endpoint.
*
*
* @param authorizeVpcEndpointAccessRequest
* @return A Java Future containing the result of the AuthorizeVpcEndpointAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - BaseException An error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.AuthorizeVpcEndpointAccess
*/
@Override
public CompletableFuture authorizeVpcEndpointAccess(
AuthorizeVpcEndpointAccessRequest authorizeVpcEndpointAccessRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(authorizeVpcEndpointAccessRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, authorizeVpcEndpointAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AuthorizeVpcEndpointAccess");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AuthorizeVpcEndpointAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AuthorizeVpcEndpointAccess").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AuthorizeVpcEndpointAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(authorizeVpcEndpointAccessRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a pending configuration change on an Amazon OpenSearch Service domain.
*
*
* @param cancelDomainConfigChangeRequest
* @return A Java Future containing the result of the CancelDomainConfigChange operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CancelDomainConfigChange
*/
@Override
public CompletableFuture cancelDomainConfigChange(
CancelDomainConfigChangeRequest cancelDomainConfigChangeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelDomainConfigChangeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelDomainConfigChangeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelDomainConfigChange");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelDomainConfigChangeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelDomainConfigChange").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelDomainConfigChangeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelDomainConfigChangeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a scheduled service software update for an Amazon OpenSearch Service domain. You can only perform this
* operation before the AutomatedUpdateDate
and when the domain's UpdateStatus
is
* PENDING_UPDATE
. For more information, see Service
* software updates in Amazon OpenSearch Service.
*
*
* @param cancelServiceSoftwareUpdateRequest
* Container for the request parameters to cancel a service software update.
* @return A Java Future containing the result of the CancelServiceSoftwareUpdate operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CancelServiceSoftwareUpdate
*/
@Override
public CompletableFuture cancelServiceSoftwareUpdate(
CancelServiceSoftwareUpdateRequest cancelServiceSoftwareUpdateRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelServiceSoftwareUpdateRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelServiceSoftwareUpdateRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelServiceSoftwareUpdate");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelServiceSoftwareUpdateResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelServiceSoftwareUpdate").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelServiceSoftwareUpdateRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelServiceSoftwareUpdateRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon OpenSearch Service domain. For more information, see Creating and
* managing Amazon OpenSearch Service domains.
*
*
* @param createDomainRequest
* @return A Java Future containing the result of the CreateDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - InvalidTypeException An exception for trying to create or access a sub-resource that's either invalid
* or not supported.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - ResourceAlreadyExistsException An exception for creating a resource that already exists.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CreateDomain
*/
@Override
public CompletableFuture createDomain(CreateDomainRequest createDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new cross-cluster search connection from a source Amazon OpenSearch Service domain to a destination
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param createOutboundConnectionRequest
* Container for the parameters to the CreateOutboundConnection
operation.
* @return A Java Future containing the result of the CreateOutboundConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceAlreadyExistsException An exception for creating a resource that already exists.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CreateOutboundConnection
*/
@Override
public CompletableFuture createOutboundConnection(
CreateOutboundConnectionRequest createOutboundConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createOutboundConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createOutboundConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateOutboundConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateOutboundConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOutboundConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateOutboundConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createOutboundConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a package for use with Amazon OpenSearch Service domains. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param createPackageRequest
* Container for request parameters to the CreatePackage
operation.
* @return A Java Future containing the result of the CreatePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - InvalidTypeException An exception for trying to create or access a sub-resource that's either invalid
* or not supported.
* - ResourceAlreadyExistsException An exception for creating a resource that already exists.
* - AccessDeniedException An error occurred because you don't have permissions to access the resource.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CreatePackage
*/
@Override
public CompletableFuture createPackage(CreatePackageRequest createPackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createPackageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createPackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreatePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreatePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreatePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createPackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Amazon OpenSearch Service-managed VPC endpoint.
*
*
* @param createVpcEndpointRequest
* @return A Java Future containing the result of the CreateVpcEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException An error occurred because the client attempts to remove a resource that is
* currently in use.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - BaseException An error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.CreateVpcEndpoint
*/
@Override
public CompletableFuture createVpcEndpoint(CreateVpcEndpointRequest createVpcEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createVpcEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVpcEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVpcEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateVpcEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateVpcEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateVpcEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createVpcEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a direct-query data source. For more information, see Deleting
* an Amazon OpenSearch Service data source with Amazon S3.
*
*
* @param deleteDataSourceRequest
* Container for the parameters to the DeleteDataSource
operation.
* @return A Java Future containing the result of the DeleteDataSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - DependencyFailureException An exception for when a failure in one of the dependencies results in the
* service being unable to fetch details about the resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeleteDataSource
*/
@Override
public CompletableFuture deleteDataSource(DeleteDataSourceRequest deleteDataSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDataSourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDataSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDataSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDataSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDataSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteDataSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteDataSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon OpenSearch Service domain and all of its data. You can't recover a domain after you delete it.
*
*
* @param deleteDomainRequest
* Container for the parameters to the DeleteDomain
operation.
* @return A Java Future containing the result of the DeleteDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeleteDomain
*/
@Override
public CompletableFuture deleteDomain(DeleteDomainRequest deleteDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Allows the destination Amazon OpenSearch Service domain owner to delete an existing inbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param deleteInboundConnectionRequest
* Container for the parameters to the DeleteInboundConnection
operation.
* @return A Java Future containing the result of the DeleteInboundConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeleteInboundConnection
*/
@Override
public CompletableFuture deleteInboundConnection(
DeleteInboundConnectionRequest deleteInboundConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteInboundConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInboundConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInboundConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteInboundConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInboundConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteInboundConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteInboundConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Allows the source Amazon OpenSearch Service domain owner to delete an existing outbound cross-cluster search
* connection. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param deleteOutboundConnectionRequest
* Container for the parameters to the DeleteOutboundConnection
operation.
* @return A Java Future containing the result of the DeleteOutboundConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeleteOutboundConnection
*/
@Override
public CompletableFuture deleteOutboundConnection(
DeleteOutboundConnectionRequest deleteOutboundConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteOutboundConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteOutboundConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteOutboundConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteOutboundConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOutboundConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteOutboundConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteOutboundConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon OpenSearch Service package. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param deletePackageRequest
* Deletes a package from OpenSearch Service. The package can't be associated with any OpenSearch Service
* domain.
* @return A Java Future containing the result of the DeletePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - AccessDeniedException An error occurred because you don't have permissions to access the resource.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - ConflictException An error occurred because the client attempts to remove a resource that is
* currently in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeletePackage
*/
@Override
public CompletableFuture deletePackage(DeletePackageRequest deletePackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deletePackageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deletePackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeletePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeletePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeletePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deletePackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an Amazon OpenSearch Service-managed interface VPC endpoint.
*
*
* @param deleteVpcEndpointRequest
* @return A Java Future containing the result of the DeleteVpcEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - BaseException An error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DeleteVpcEndpoint
*/
@Override
public CompletableFuture deleteVpcEndpoint(DeleteVpcEndpointRequest deleteVpcEndpointRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteVpcEndpointRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVpcEndpointRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVpcEndpoint");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteVpcEndpointResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteVpcEndpoint").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteVpcEndpointRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteVpcEndpointRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the domain configuration for the specified Amazon OpenSearch Service domain, including the domain ID,
* domain service endpoint, and domain ARN.
*
*
* @param describeDomainRequest
* Container for the parameters to the DescribeDomain
operation.
* @return A Java Future containing the result of the DescribeDomain operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomain
*/
@Override
public CompletableFuture describeDomain(DescribeDomainRequest describeDomainRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomain");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomain").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the list of optimizations that Auto-Tune has made to an Amazon OpenSearch Service domain. For more
* information, see Auto-Tune for Amazon
* OpenSearch Service.
*
*
* @param describeDomainAutoTunesRequest
* Container for the parameters to the DescribeDomainAutoTunes
operation.
* @return A Java Future containing the result of the DescribeDomainAutoTunes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomainAutoTunes
*/
@Override
public CompletableFuture describeDomainAutoTunes(
DescribeDomainAutoTunesRequest describeDomainAutoTunesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainAutoTunesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainAutoTunesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomainAutoTunes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainAutoTunesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomainAutoTunes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainAutoTunesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainAutoTunesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about the current blue/green deployment happening on an Amazon OpenSearch Service domain. For
* more information, see Making configuration changes in Amazon OpenSearch Service.
*
*
* @param describeDomainChangeProgressRequest
* Container for the parameters to the DescribeDomainChangeProgress
operation.
* @return A Java Future containing the result of the DescribeDomainChangeProgress operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomainChangeProgress
*/
@Override
public CompletableFuture describeDomainChangeProgress(
DescribeDomainChangeProgressRequest describeDomainChangeProgressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainChangeProgressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainChangeProgressRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomainChangeProgress");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainChangeProgressResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomainChangeProgress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainChangeProgressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainChangeProgressRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the configuration of an Amazon OpenSearch Service domain.
*
*
* @param describeDomainConfigRequest
* Container for the parameters to the DescribeDomainConfig
operation.
* @return A Java Future containing the result of the DescribeDomainConfig operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomainConfig
*/
@Override
public CompletableFuture describeDomainConfig(
DescribeDomainConfigRequest describeDomainConfigRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainConfigRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainConfigRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomainConfig");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainConfigResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomainConfig").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainConfigRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainConfigRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about domain and node health, the standby Availability Zone, number of nodes per Availability
* Zone, and shard count per node.
*
*
* @param describeDomainHealthRequest
* Container for the parameters to the DescribeDomainHealth
operation.
* @return A Java Future containing the result of the DescribeDomainHealth operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomainHealth
*/
@Override
public CompletableFuture describeDomainHealth(
DescribeDomainHealthRequest describeDomainHealthRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainHealthRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainHealthRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomainHealth");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainHealthResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomainHealth").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainHealthRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainHealthRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns information about domain and nodes, including data nodes, master nodes, ultrawarm nodes, Availability
* Zone(s), standby nodes, node configurations, and node states.
*
*
* @param describeDomainNodesRequest
* Container for the parameters to the DescribeDomainNodes
operation.
* @return A Java Future containing the result of the DescribeDomainNodes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - DependencyFailureException An exception for when a failure in one of the dependencies results in the
* service being unable to fetch details about the resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomainNodes
*/
@Override
public CompletableFuture describeDomainNodes(
DescribeDomainNodesRequest describeDomainNodesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainNodesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainNodesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomainNodes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainNodesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomainNodes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainNodesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainNodesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns domain configuration information about the specified Amazon OpenSearch Service domains.
*
*
* @param describeDomainsRequest
* Container for the parameters to the DescribeDomains
operation.
* @return A Java Future containing the result of the DescribeDomains operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDomains
*/
@Override
public CompletableFuture describeDomains(DescribeDomainsRequest describeDomainsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDomainsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDomainsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDomains");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDomainsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDomains").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDomainsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDomainsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the progress of a pre-update dry run analysis on an Amazon OpenSearch Service domain. For more
* information, see Determining whether a change will cause a blue/green deployment.
*
*
* @param describeDryRunProgressRequest
* @return A Java Future containing the result of the DescribeDryRunProgress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeDryRunProgress
*/
@Override
public CompletableFuture describeDryRunProgress(
DescribeDryRunProgressRequest describeDryRunProgressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDryRunProgressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDryRunProgressRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDryRunProgress");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDryRunProgressResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDryRunProgress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDryRunProgressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDryRunProgressRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the inbound cross-cluster search connections for a destination (remote) Amazon OpenSearch Service
* domain. For more information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param describeInboundConnectionsRequest
* Container for the parameters to the DescribeInboundConnections
operation.
* @return A Java Future containing the result of the DescribeInboundConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidPaginationTokenException Request processing failed because you provided an invalid pagination
* token.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeInboundConnections
*/
@Override
public CompletableFuture describeInboundConnections(
DescribeInboundConnectionsRequest describeInboundConnectionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeInboundConnectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInboundConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInboundConnections");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInboundConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInboundConnections").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeInboundConnectionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeInboundConnectionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the instance count, storage, and master node limits for a given OpenSearch or Elasticsearch version and
* instance type.
*
*
* @param describeInstanceTypeLimitsRequest
* Container for the parameters to the DescribeInstanceTypeLimits
operation.
* @return A Java Future containing the result of the DescribeInstanceTypeLimits operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - InvalidTypeException An exception for trying to create or access a sub-resource that's either invalid
* or not supported.
* - LimitExceededException An exception for trying to create more than the allowed number of resources or
* sub-resources.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeInstanceTypeLimits
*/
@Override
public CompletableFuture describeInstanceTypeLimits(
DescribeInstanceTypeLimitsRequest describeInstanceTypeLimitsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeInstanceTypeLimitsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstanceTypeLimitsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstanceTypeLimits");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstanceTypeLimitsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstanceTypeLimits").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeInstanceTypeLimitsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeInstanceTypeLimitsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the outbound cross-cluster connections for a local (source) Amazon OpenSearch Service domain. For more
* information, see Cross-cluster search for Amazon OpenSearch Service.
*
*
* @param describeOutboundConnectionsRequest
* Container for the parameters to the DescribeOutboundConnections
operation.
* @return A Java Future containing the result of the DescribeOutboundConnections operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InvalidPaginationTokenException Request processing failed because you provided an invalid pagination
* token.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeOutboundConnections
*/
@Override
public CompletableFuture describeOutboundConnections(
DescribeOutboundConnectionsRequest describeOutboundConnectionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeOutboundConnectionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeOutboundConnectionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOutboundConnections");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOutboundConnectionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOutboundConnections").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeOutboundConnectionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeOutboundConnectionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes all packages available to OpenSearch Service. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param describePackagesRequest
* Container for the request parameters to the DescribePackage
operation.
* @return A Java Future containing the result of the DescribePackages operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - AccessDeniedException An error occurred because you don't have permissions to access the resource.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribePackages
*/
@Override
public CompletableFuture describePackages(DescribePackagesRequest describePackagesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePackagesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePackagesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePackages");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePackagesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePackages").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePackagesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePackagesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the available Amazon OpenSearch Service Reserved Instance offerings for a given Region. For more
* information, see Reserved
* Instances in Amazon OpenSearch Service.
*
*
* @param describeReservedInstanceOfferingsRequest
* Container for the request parameters to a DescribeReservedInstanceOfferings
operation.
* @return A Java Future containing the result of the DescribeReservedInstanceOfferings operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeReservedInstanceOfferings
*/
@Override
public CompletableFuture describeReservedInstanceOfferings(
DescribeReservedInstanceOfferingsRequest describeReservedInstanceOfferingsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReservedInstanceOfferingsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeReservedInstanceOfferingsRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReservedInstanceOfferings");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DescribeReservedInstanceOfferingsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReservedInstanceOfferings").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeReservedInstanceOfferingsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeReservedInstanceOfferingsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the Amazon OpenSearch Service instances that you have reserved in a given Region. For more information,
* see Reserved Instances in
* Amazon OpenSearch Service.
*
*
* @param describeReservedInstancesRequest
* Container for the request parameters to the DescribeReservedInstances
operation.
* @return A Java Future containing the result of the DescribeReservedInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeReservedInstances
*/
@Override
public CompletableFuture describeReservedInstances(
DescribeReservedInstancesRequest describeReservedInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeReservedInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeReservedInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeReservedInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeReservedInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeReservedInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeReservedInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeReservedInstancesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes one or more Amazon OpenSearch Service-managed VPC endpoints.
*
*
* @param describeVpcEndpointsRequest
* @return A Java Future containing the result of the DescribeVpcEndpoints operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - BaseException An error occurred while processing the request.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DescribeVpcEndpoints
*/
@Override
public CompletableFuture describeVpcEndpoints(
DescribeVpcEndpointsRequest describeVpcEndpointsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeVpcEndpointsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeVpcEndpointsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeVpcEndpoints");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeVpcEndpointsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeVpcEndpoints").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeVpcEndpointsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeVpcEndpointsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes a package from the specified Amazon OpenSearch Service domain. The package can't be in use with any
* OpenSearch index for the dissociation to succeed. The package is still available in OpenSearch Service for
* association later. For more information, see Custom packages
* for Amazon OpenSearch Service.
*
*
* @param dissociatePackageRequest
* Container for the request parameters to the DissociatePackage
operation.
* @return A Java Future containing the result of the DissociatePackage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - AccessDeniedException An error occurred because you don't have permissions to access the resource.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - ConflictException An error occurred because the client attempts to remove a resource that is
* currently in use.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.DissociatePackage
*/
@Override
public CompletableFuture dissociatePackage(DissociatePackageRequest dissociatePackageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(dissociatePackageRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, dissociatePackageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DissociatePackage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DissociatePackageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DissociatePackage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DissociatePackageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(dissociatePackageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a map of OpenSearch or Elasticsearch versions and the versions you can upgrade them to.
*
*
* @param getCompatibleVersionsRequest
* Container for the request parameters to GetCompatibleVersions
operation.
* @return A Java Future containing the result of the GetCompatibleVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.GetCompatibleVersions
*/
@Override
public CompletableFuture getCompatibleVersions(
GetCompatibleVersionsRequest getCompatibleVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCompatibleVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCompatibleVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCompatibleVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCompatibleVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCompatibleVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCompatibleVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCompatibleVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about a direct query data source.
*
*
* @param getDataSourceRequest
* Container for the parameters to the GetDataSource
operation.
* @return A Java Future containing the result of the GetDataSource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - DependencyFailureException An exception for when a failure in one of the dependencies results in the
* service being unable to fetch details about the resource.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.GetDataSource
*/
@Override
public CompletableFuture getDataSource(GetDataSourceRequest getDataSourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDataSourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDataSourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDataSource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetDataSourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetDataSource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetDataSourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getDataSourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* The status of the maintenance action.
*
*
* @param getDomainMaintenanceStatusRequest
* Container for the parameters to the GetDomainMaintenanceStatus
operation.
* @return A Java Future containing the result of the GetDomainMaintenanceStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - BaseException An error occurred while processing the request.
* - InternalException Request processing failed because of an unknown error, exception, or internal
* failure.
* - ResourceNotFoundException An exception for accessing or deleting a resource that doesn't exist.
* - ValidationException An exception for accessing or deleting a resource that doesn't exist.
* - DisabledOperationException An error occured because the client wanted to access an unsupported
* operation.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpenSearchException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpenSearchAsyncClient.GetDomainMaintenanceStatus
*/
@Override
public CompletableFuture getDomainMaintenanceStatus(
GetDomainMaintenanceStatusRequest getDomainMaintenanceStatusRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getDomainMaintenanceStatusRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDomainMaintenanceStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpenSearch");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDomainMaintenanceStatus");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDomainMaintenanceStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams