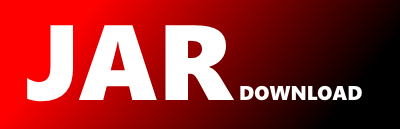
software.amazon.awssdk.services.opsworks.model.Recipes Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import javax.annotation.Generated;
import software.amazon.awssdk.annotation.SdkInternalApi;
import software.amazon.awssdk.protocol.ProtocolMarshaller;
import software.amazon.awssdk.protocol.StructuredPojo;
import software.amazon.awssdk.services.opsworks.transform.RecipesMarshaller;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* AWS OpsWorks Stacks supports five lifecycle events: setup, configuration, deploy,
* undeploy, and shutdown. For each layer, AWS OpsWorks Stacks runs a set of standard recipes for each
* event. In addition, you can provide custom recipes for any or all layers and events. AWS OpsWorks Stacks runs custom
* event recipes after the standard recipes. LayerCustomRecipes
specifies the custom recipes for a
* particular layer to be run in response to each of the five events.
*
*
* To specify a recipe, use the cookbook's directory name in the repository followed by two colons and the recipe name,
* which is the recipe's file name without the .rb extension. For example: phpapp2::dbsetup specifies the dbsetup.rb
* recipe in the repository's phpapp2 folder.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class Recipes implements StructuredPojo, ToCopyableBuilder {
private final List setup;
private final List configure;
private final List deploy;
private final List undeploy;
private final List shutdown;
private Recipes(BuilderImpl builder) {
this.setup = builder.setup;
this.configure = builder.configure;
this.deploy = builder.deploy;
this.undeploy = builder.undeploy;
this.shutdown = builder.shutdown;
}
/**
*
* An array of custom recipe names to be run following a setup
event.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of custom recipe names to be run following a setup
event.
*/
public List setup() {
return setup;
}
/**
*
* An array of custom recipe names to be run following a configure
event.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of custom recipe names to be run following a configure
event.
*/
public List configure() {
return configure;
}
/**
*
* An array of custom recipe names to be run following a deploy
event.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of custom recipe names to be run following a deploy
event.
*/
public List deploy() {
return deploy;
}
/**
*
* An array of custom recipe names to be run following a undeploy
event.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of custom recipe names to be run following a undeploy
event.
*/
public List undeploy() {
return undeploy;
}
/**
*
* An array of custom recipe names to be run following a shutdown
event.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of custom recipe names to be run following a shutdown
event.
*/
public List shutdown() {
return shutdown;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + ((setup() == null) ? 0 : setup().hashCode());
hashCode = 31 * hashCode + ((configure() == null) ? 0 : configure().hashCode());
hashCode = 31 * hashCode + ((deploy() == null) ? 0 : deploy().hashCode());
hashCode = 31 * hashCode + ((undeploy() == null) ? 0 : undeploy().hashCode());
hashCode = 31 * hashCode + ((shutdown() == null) ? 0 : shutdown().hashCode());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Recipes)) {
return false;
}
Recipes other = (Recipes) obj;
if (other.setup() == null ^ this.setup() == null) {
return false;
}
if (other.setup() != null && !other.setup().equals(this.setup())) {
return false;
}
if (other.configure() == null ^ this.configure() == null) {
return false;
}
if (other.configure() != null && !other.configure().equals(this.configure())) {
return false;
}
if (other.deploy() == null ^ this.deploy() == null) {
return false;
}
if (other.deploy() != null && !other.deploy().equals(this.deploy())) {
return false;
}
if (other.undeploy() == null ^ this.undeploy() == null) {
return false;
}
if (other.undeploy() != null && !other.undeploy().equals(this.undeploy())) {
return false;
}
if (other.shutdown() == null ^ this.shutdown() == null) {
return false;
}
if (other.shutdown() != null && !other.shutdown().equals(this.shutdown())) {
return false;
}
return true;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (setup() != null) {
sb.append("Setup: ").append(setup()).append(",");
}
if (configure() != null) {
sb.append("Configure: ").append(configure()).append(",");
}
if (deploy() != null) {
sb.append("Deploy: ").append(deploy()).append(",");
}
if (undeploy() != null) {
sb.append("Undeploy: ").append(undeploy()).append(",");
}
if (shutdown() != null) {
sb.append("Shutdown: ").append(shutdown()).append(",");
}
sb.append("}");
return sb.toString();
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
RecipesMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* An array of custom recipe names to be run following a setup
event.
*
*
* @param setup
* An array of custom recipe names to be run following a setup
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder setup(Collection setup);
/**
*
* An array of custom recipe names to be run following a setup
event.
*
*
* @param setup
* An array of custom recipe names to be run following a setup
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder setup(String... setup);
/**
*
* An array of custom recipe names to be run following a configure
event.
*
*
* @param configure
* An array of custom recipe names to be run following a configure
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder configure(Collection configure);
/**
*
* An array of custom recipe names to be run following a configure
event.
*
*
* @param configure
* An array of custom recipe names to be run following a configure
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder configure(String... configure);
/**
*
* An array of custom recipe names to be run following a deploy
event.
*
*
* @param deploy
* An array of custom recipe names to be run following a deploy
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deploy(Collection deploy);
/**
*
* An array of custom recipe names to be run following a deploy
event.
*
*
* @param deploy
* An array of custom recipe names to be run following a deploy
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deploy(String... deploy);
/**
*
* An array of custom recipe names to be run following a undeploy
event.
*
*
* @param undeploy
* An array of custom recipe names to be run following a undeploy
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder undeploy(Collection undeploy);
/**
*
* An array of custom recipe names to be run following a undeploy
event.
*
*
* @param undeploy
* An array of custom recipe names to be run following a undeploy
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder undeploy(String... undeploy);
/**
*
* An array of custom recipe names to be run following a shutdown
event.
*
*
* @param shutdown
* An array of custom recipe names to be run following a shutdown
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder shutdown(Collection shutdown);
/**
*
* An array of custom recipe names to be run following a shutdown
event.
*
*
* @param shutdown
* An array of custom recipe names to be run following a shutdown
event.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder shutdown(String... shutdown);
}
private static final class BuilderImpl implements Builder {
private List setup;
private List configure;
private List deploy;
private List undeploy;
private List shutdown;
private BuilderImpl() {
}
private BuilderImpl(Recipes model) {
setSetup(model.setup);
setConfigure(model.configure);
setDeploy(model.deploy);
setUndeploy(model.undeploy);
setShutdown(model.shutdown);
}
public final Collection getSetup() {
return setup;
}
@Override
public final Builder setup(Collection setup) {
this.setup = StringsCopier.copy(setup);
return this;
}
@Override
@SafeVarargs
public final Builder setup(String... setup) {
setup(Arrays.asList(setup));
return this;
}
public final void setSetup(Collection setup) {
this.setup = StringsCopier.copy(setup);
}
@SafeVarargs
public final void setSetup(String... setup) {
setup(Arrays.asList(setup));
}
public final Collection getConfigure() {
return configure;
}
@Override
public final Builder configure(Collection configure) {
this.configure = StringsCopier.copy(configure);
return this;
}
@Override
@SafeVarargs
public final Builder configure(String... configure) {
configure(Arrays.asList(configure));
return this;
}
public final void setConfigure(Collection configure) {
this.configure = StringsCopier.copy(configure);
}
@SafeVarargs
public final void setConfigure(String... configure) {
configure(Arrays.asList(configure));
}
public final Collection getDeploy() {
return deploy;
}
@Override
public final Builder deploy(Collection deploy) {
this.deploy = StringsCopier.copy(deploy);
return this;
}
@Override
@SafeVarargs
public final Builder deploy(String... deploy) {
deploy(Arrays.asList(deploy));
return this;
}
public final void setDeploy(Collection deploy) {
this.deploy = StringsCopier.copy(deploy);
}
@SafeVarargs
public final void setDeploy(String... deploy) {
deploy(Arrays.asList(deploy));
}
public final Collection getUndeploy() {
return undeploy;
}
@Override
public final Builder undeploy(Collection undeploy) {
this.undeploy = StringsCopier.copy(undeploy);
return this;
}
@Override
@SafeVarargs
public final Builder undeploy(String... undeploy) {
undeploy(Arrays.asList(undeploy));
return this;
}
public final void setUndeploy(Collection undeploy) {
this.undeploy = StringsCopier.copy(undeploy);
}
@SafeVarargs
public final void setUndeploy(String... undeploy) {
undeploy(Arrays.asList(undeploy));
}
public final Collection getShutdown() {
return shutdown;
}
@Override
public final Builder shutdown(Collection shutdown) {
this.shutdown = StringsCopier.copy(shutdown);
return this;
}
@Override
@SafeVarargs
public final Builder shutdown(String... shutdown) {
shutdown(Arrays.asList(shutdown));
return this;
}
public final void setShutdown(Collection shutdown) {
this.shutdown = StringsCopier.copy(shutdown);
}
@SafeVarargs
public final void setShutdown(String... shutdown) {
shutdown(Arrays.asList(shutdown));
}
@Override
public Recipes build() {
return new Recipes(this);
}
}
}