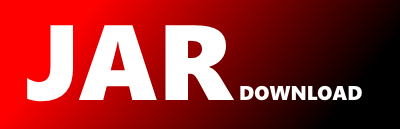
software.amazon.awssdk.services.opsworks.DefaultOpsWorksClient Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks;
import java.util.Collections;
import java.util.List;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.CloneStackRequest;
import software.amazon.awssdk.services.opsworks.model.CloneStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateAppRequest;
import software.amazon.awssdk.services.opsworks.model.CreateAppResponse;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.CreateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.CreateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.CreateStackRequest;
import software.amazon.awssdk.services.opsworks.model.CreateStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteAppRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteAppResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteStackRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteStackResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesResponse;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionRequest;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionResponse;
import software.amazon.awssdk.services.opsworks.model.GrantAccessRequest;
import software.amazon.awssdk.services.opsworks.model.GrantAccessResponse;
import software.amazon.awssdk.services.opsworks.model.ListTagsRequest;
import software.amazon.awssdk.services.opsworks.model.ListTagsResponse;
import software.amazon.awssdk.services.opsworks.model.OpsWorksException;
import software.amazon.awssdk.services.opsworks.model.OpsWorksRequest;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ResourceNotFoundException;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.SetPermissionRequest;
import software.amazon.awssdk.services.opsworks.model.SetPermissionResponse;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.StartInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StartInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StartStackRequest;
import software.amazon.awssdk.services.opsworks.model.StartStackResponse;
import software.amazon.awssdk.services.opsworks.model.StopInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StopInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StopStackRequest;
import software.amazon.awssdk.services.opsworks.model.StopStackResponse;
import software.amazon.awssdk.services.opsworks.model.TagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.TagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.UntagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.UntagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateAppRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateAppResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateStackRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateStackResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ValidationException;
import software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable;
import software.amazon.awssdk.services.opsworks.transform.AssignInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AssignVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AssociateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AttachElasticLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CloneStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateDeploymentRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterEcsClusterRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeAgentVersionsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeAppsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeCommandsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeEcsClustersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeElasticIpsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeElasticLoadBalancersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeInstancesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeLayersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeLoadBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeMyUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeOperatingSystemsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribePermissionsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeRaidArraysRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeRdsDbInstancesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeServiceErrorsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStackProvisioningParametersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStackSummaryRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStacksRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeTimeBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeUserProfilesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeVolumesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DetachElasticLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DisassociateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.GetHostnameSuggestionRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.GrantAccessRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.ListTagsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RebootInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterEcsClusterRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetLoadBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetPermissionRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetTimeBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StartInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StartStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StopInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StopStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UnassignInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UnassignVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateMyUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.waiters.OpsWorksWaiter;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link OpsWorksClient}.
*
* @see OpsWorksClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultOpsWorksClient implements OpsWorksClient {
private static final Logger log = Logger.loggerFor(DefaultOpsWorksClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultOpsWorksClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Assign a registered instance to a layer.
*
*
* -
*
* You can assign registered on-premises instances to any layer type.
*
*
* -
*
* You can assign registered Amazon EC2 instances only to custom layers.
*
*
* -
*
* You cannot use this action with instances that were created with AWS OpsWorks Stacks.
*
*
*
*
* Required Permissions: To use this action, an AWS Identity and Access Management (IAM) user must have a
* Manage permissions level for the stack or an attached policy that explicitly grants permissions. For more
* information on user permissions, see Managing User
* Permissions.
*
*
* @param assignInstanceRequest
* @return Result of the AssignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignInstance
* @see AWS API
* Documentation
*/
@Override
public AssignInstanceResponse assignInstance(AssignInstanceRequest assignInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssignInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, assignInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssignInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssignInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(assignInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssignInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a specified instance. The volume must first be
* registered with the stack by calling RegisterVolume. After you register the volume, you must call
* UpdateVolume to specify a mount point before calling AssignVolume
. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param assignVolumeRequest
* @return Result of the AssignVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignVolume
* @see AWS API
* Documentation
*/
@Override
public AssignVolumeResponse assignVolume(AssignVolumeRequest assignVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssignVolumeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, assignVolumeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssignVolume");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssignVolume").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(assignVolumeRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssignVolumeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a specified instance. The address must first
* be registered with the stack by calling RegisterElasticIp. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param associateElasticIpRequest
* @return Result of the AssociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssociateElasticIp
* @see AWS
* API Documentation
*/
@Override
public AssociateElasticIpResponse associateElasticIp(AssociateElasticIpRequest associateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateElasticIp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateElasticIp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateElasticIpRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateElasticIpRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer. AWS OpsWorks Stacks does not support
* Application Load Balancer. You can only use Classic Load Balancer with AWS OpsWorks Stacks. For more information,
* see Elastic Load Balancing.
*
*
*
* You must create the Elastic Load Balancing instance separately, by using the Elastic Load Balancing console, API,
* or CLI. For more information, see Elastic Load
* Balancing Developer Guide.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param attachElasticLoadBalancerRequest
* @return Result of the AttachElasticLoadBalancer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AttachElasticLoadBalancer
* @see AWS API Documentation
*/
@Override
public AttachElasticLoadBalancerResponse attachElasticLoadBalancer(
AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AttachElasticLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachElasticLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachElasticLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachElasticLoadBalancer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(attachElasticLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AttachElasticLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a clone of a specified stack. For more information, see Clone a Stack. By
* default, all parameters are set to the values used by the parent stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param cloneStackRequest
* @return Result of the CloneStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CloneStack
* @see AWS API
* Documentation
*/
@Override
public CloneStackResponse cloneStack(CloneStackRequest cloneStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CloneStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cloneStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CloneStack");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("CloneStack")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(cloneStackRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CloneStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an app for a specified stack. For more information, see Creating Apps.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateApp
* @see AWS API
* Documentation
*/
@Override
public CreateAppResponse createApp(CreateAppRequest createAppRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new CreateAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Runs deployment or stack commands. For more information, see Deploying Apps and Run Stack Commands.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
* @param createDeploymentRequest
* @return Result of the CreateDeployment operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateDeployment
* @see AWS API
* Documentation
*/
@Override
public CreateDeploymentResponse createDeployment(CreateDeploymentRequest createDeploymentRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeployment");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDeployment").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createDeploymentRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDeploymentRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an instance in a specified stack. For more information, see Adding an Instance to a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createInstanceRequest
* @return Result of the CreateInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateInstance
* @see AWS API
* Documentation
*/
@Override
public CreateInstanceResponse createInstance(CreateInstanceRequest createInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a layer. For more information, see How to Create a
* Layer.
*
*
*
* You should use CreateLayer for noncustom layer types such as PHP App Server only if the stack does not
* have an existing layer of that type. A stack can have at most one instance of each noncustom layer; if you
* attempt to create a second instance, CreateLayer fails. A stack can have an arbitrary number of custom
* layers, so you can call CreateLayer as many times as you like for that layer type.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createLayerRequest
* @return Result of the CreateLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateLayer
* @see AWS API
* Documentation
*/
@Override
public CreateLayerResponse createLayer(CreateLayerRequest createLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateLayerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLayerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLayer");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateLayer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createLayerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateLayerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new stack. For more information, see Create a New Stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createStackRequest
* @return Result of the CreateStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateStack
* @see AWS API
* Documentation
*/
@Override
public CreateStackResponse createStack(CreateStackRequest createStackRequest) throws ValidationException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateStack").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createUserProfileRequest
* @return Result of the CreateUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateUserProfile
* @see AWS API
* Documentation
*/
@Override
public CreateUserProfileResponse createUserProfile(CreateUserProfileRequest createUserProfileRequest)
throws ValidationException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUserProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateUserProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createUserProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateUserProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteApp
* @see AWS API
* Documentation
*/
@Override
public DeleteAppResponse deleteApp(DeleteAppRequest deleteAppRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteApp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteAppRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new DeleteAppRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a specified instance, which terminates the associated Amazon EC2 instance. You must stop an instance
* before you can delete it.
*
*
* For more information, see Deleting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteInstanceRequest
* @return Result of the DeleteInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteInstance
* @see AWS API
* Documentation
*/
@Override
public DeleteInstanceResponse deleteInstance(DeleteInstanceRequest deleteInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a specified layer. You must first stop and then delete all associated instances or unassign registered
* instances. For more information, see How to Delete a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteLayerRequest
* @return Result of the DeleteLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteLayer
* @see AWS API
* Documentation
*/
@Override
public DeleteLayerResponse deleteLayer(DeleteLayerRequest deleteLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteLayerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLayerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLayer");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteLayer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteLayerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteLayerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a specified stack. You must first delete all instances, layers, and apps or deregister registered
* instances. For more information, see Shut Down a Stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteStackRequest
* @return Result of the DeleteStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteStack
* @see AWS API
* Documentation
*/
@Override
public DeleteStackResponse deleteStack(DeleteStackRequest deleteStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteStack").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteStackRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param deleteUserProfileRequest
* @return Result of the DeleteUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteUserProfile
* @see AWS API
* Documentation
*/
@Override
public DeleteUserProfileResponse deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteUserProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteUserProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteUserProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters a specified Amazon ECS cluster from a stack. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see https://docs.aws.amazon.com/opsworks/latest/userguide/opsworks-security-users.html.
*
*
* @param deregisterEcsClusterRequest
* @return Result of the DeregisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterEcsCluster
* @see AWS
* API Documentation
*/
@Override
public DeregisterEcsClusterResponse deregisterEcsCluster(DeregisterEcsClusterRequest deregisterEcsClusterRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterEcsClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterEcsClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterEcsCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeregisterEcsCluster").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterEcsClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterEcsClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be registered by another stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterElasticIpRequest
* @return Result of the DeregisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterElasticIp
* @see AWS
* API Documentation
*/
@Override
public DeregisterElasticIpResponse deregisterElasticIp(DeregisterElasticIpRequest deregisterElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterElasticIp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeregisterElasticIp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterElasticIpRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterElasticIpRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregister a registered Amazon EC2 or on-premises instance. This action removes the instance from the stack and
* returns it to your control. This action cannot be used with instances that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterInstanceRequest
* @return Result of the DeregisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterInstance
* @see AWS
* API Documentation
*/
@Override
public DeregisterInstanceResponse deregisterInstance(DeregisterInstanceRequest deregisterInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeregisterInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterRdsDbInstanceRequest
* @return Result of the DeregisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterRdsDbInstance
* @see AWS API Documentation
*/
@Override
public DeregisterRdsDbInstanceResponse deregisterRdsDbInstance(DeregisterRdsDbInstanceRequest deregisterRdsDbInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterRdsDbInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterRdsDbInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterRdsDbInstance");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterRdsDbInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterRdsDbInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterRdsDbInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by another stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterVolumeRequest
* @return Result of the DeregisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterVolume
* @see AWS API
* Documentation
*/
@Override
public DeregisterVolumeResponse deregisterVolume(DeregisterVolumeRequest deregisterVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeregisterVolumeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterVolumeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterVolume");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeregisterVolume").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deregisterVolumeRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeregisterVolumeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the available AWS OpsWorks Stacks agent versions. You must specify a stack ID or a configuration
* manager. DescribeAgentVersions
returns a list of available agent versions for the specified stack or
* configuration manager.
*
*
* @param describeAgentVersionsRequest
* @return Result of the DescribeAgentVersions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeAgentVersions
* @see AWS
* API Documentation
*/
@Override
public DescribeAgentVersionsResponse describeAgentVersions(DescribeAgentVersionsRequest describeAgentVersionsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAgentVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAgentVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAgentVersions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeAgentVersions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeAgentVersionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAgentVersionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of a specified set of apps.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeAppsRequest
* @return Result of the DescribeApps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeApps
* @see AWS API
* Documentation
*/
@Override
public DescribeAppsResponse describeApps(DescribeAppsRequest describeAppsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeAppsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAppsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeApps").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeAppsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeAppsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the results of specified commands.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeCommandsRequest
* @return Result of the DescribeCommands operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeCommands
* @see AWS API
* Documentation
*/
@Override
public DescribeCommandsResponse describeCommands(DescribeCommandsRequest describeCommandsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeCommandsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCommandsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCommands");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeCommands").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeCommandsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeCommandsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of a specified set of deployments.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeDeploymentsRequest
* @return Result of the DescribeDeployments operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeDeployments
* @see AWS
* API Documentation
*/
@Override
public DescribeDeploymentsResponse describeDeployments(DescribeDeploymentsRequest describeDeploymentsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDeployments");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeDeployments").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeDeploymentsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeDeploymentsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeEcsClustersRequest
* @return Result of the DescribeEcsClusters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
@Override
public DescribeEcsClustersResponse describeEcsClusters(DescribeEcsClustersRequest describeEcsClustersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEcsClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEcsClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEcsClusters");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeEcsClusters").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeEcsClustersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeEcsClustersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a variant of
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client
* .describeEcsClustersPaginator(request);
* for (software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation.
*
*
* @param describeEcsClustersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
@Override
public DescribeEcsClustersIterable describeEcsClustersPaginator(DescribeEcsClustersRequest describeEcsClustersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return new DescribeEcsClustersIterable(this, applyPaginatorUserAgent(describeEcsClustersRequest));
}
/**
*
* Describes Elastic IP
* addresses.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticIpsRequest
* @return Result of the DescribeElasticIps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticIps
* @see AWS
* API Documentation
*/
@Override
public DescribeElasticIpsResponse describeElasticIps(DescribeElasticIpsRequest describeElasticIpsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeElasticIpsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeElasticIpsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeElasticIps");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeElasticIps").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeElasticIpsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeElasticIpsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticLoadBalancersRequest
* @return Result of the DescribeElasticLoadBalancers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticLoadBalancers
* @see AWS API Documentation
*/
@Override
public DescribeElasticLoadBalancersResponse describeElasticLoadBalancers(
DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeElasticLoadBalancersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeElasticLoadBalancersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeElasticLoadBalancers");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeElasticLoadBalancers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeElasticLoadBalancersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeElasticLoadBalancersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of a set of instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeInstancesRequest
* @return Result of the DescribeInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeInstances
* @see AWS API
* Documentation
*/
@Override
public DescribeInstancesResponse describeInstances(DescribeInstancesRequest describeInstancesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstances");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeInstances").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLayersRequest
* @return Result of the DescribeLayers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLayers
* @see AWS API
* Documentation
*/
@Override
public DescribeLayersResponse describeLayers(DescribeLayersRequest describeLayersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeLayersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLayersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLayers");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeLayers").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeLayersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLayersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLoadBasedAutoScalingRequest
* @return Result of the DescribeLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLoadBasedAutoScaling
* @see AWS API Documentation
*/
@Override
public DescribeLoadBasedAutoScalingResponse describeLoadBasedAutoScaling(
DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeLoadBasedAutoScalingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLoadBasedAutoScalingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBasedAutoScaling");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBasedAutoScaling").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeLoadBasedAutoScalingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeLoadBasedAutoScalingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param describeMyUserProfileRequest
* @return Result of the DescribeMyUserProfile operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeMyUserProfile
* @see AWS
* API Documentation
*/
@Override
public DescribeMyUserProfileResponse describeMyUserProfile(DescribeMyUserProfileRequest describeMyUserProfileRequest)
throws AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeMyUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeMyUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeMyUserProfile");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeMyUserProfile").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeMyUserProfileRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeMyUserProfileRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the operating systems that are supported by AWS OpsWorks Stacks.
*
*
* @param describeOperatingSystemsRequest
* @return Result of the DescribeOperatingSystems operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeOperatingSystems
* @see AWS API Documentation
*/
@Override
public DescribeOperatingSystemsResponse describeOperatingSystems(
DescribeOperatingSystemsRequest describeOperatingSystemsRequest) throws AwsServiceException, SdkClientException,
OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOperatingSystemsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeOperatingSystemsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOperatingSystems");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOperatingSystems").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeOperatingSystemsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeOperatingSystemsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param describePermissionsRequest
* @return Result of the DescribePermissions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribePermissions
* @see AWS
* API Documentation
*/
@Override
public DescribePermissionsResponse describePermissions(DescribePermissionsRequest describePermissionsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePermissions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribePermissions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describePermissionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribePermissionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describe an instance's RAID arrays.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeRaidArraysRequest
* @return Result of the DescribeRaidArrays operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRaidArrays
* @see AWS
* API Documentation
*/
@Override
public DescribeRaidArraysResponse describeRaidArrays(DescribeRaidArraysRequest describeRaidArraysRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRaidArraysResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRaidArraysRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRaidArrays");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeRaidArrays").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRaidArraysRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRaidArraysRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes Amazon RDS instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeRdsDbInstancesRequest
* @return Result of the DescribeRdsDbInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRdsDbInstances
* @see AWS API Documentation
*/
@Override
public DescribeRdsDbInstancesResponse describeRdsDbInstances(DescribeRdsDbInstancesRequest describeRdsDbInstancesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRdsDbInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRdsDbInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRdsDbInstances");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRdsDbInstances").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeRdsDbInstancesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeRdsDbInstancesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes AWS OpsWorks Stacks service errors.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeServiceErrorsRequest
* @return Result of the DescribeServiceErrors operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeServiceErrors
* @see AWS
* API Documentation
*/
@Override
public DescribeServiceErrorsResponse describeServiceErrors(DescribeServiceErrorsRequest describeServiceErrorsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeServiceErrorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeServiceErrorsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeServiceErrors");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeServiceErrors").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeServiceErrorsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeServiceErrorsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of a stack's provisioning parameters.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStackProvisioningParametersRequest
* @return Result of the DescribeStackProvisioningParameters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackProvisioningParameters
* @see AWS API Documentation
*/
@Override
public DescribeStackProvisioningParametersResponse describeStackProvisioningParameters(
DescribeStackProvisioningParametersRequest describeStackProvisioningParametersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeStackProvisioningParametersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
describeStackProvisioningParametersRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackProvisioningParameters");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeStackProvisioningParameters").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeStackProvisioningParametersRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStackProvisioningParametersRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes the number of layers and apps in a specified stack, and the number of instances in each state, such as
* running_setup
or online
.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStackSummaryRequest
* @return Result of the DescribeStackSummary operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackSummary
* @see AWS
* API Documentation
*/
@Override
public DescribeStackSummaryResponse describeStackSummary(DescribeStackSummaryRequest describeStackSummaryRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeStackSummaryResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStackSummaryRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStackSummary");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeStackSummary").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeStackSummaryRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStackSummaryRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStacksRequest
* @return Result of the DescribeStacks operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStacks
* @see AWS API
* Documentation
*/
@Override
public DescribeStacksResponse describeStacks(DescribeStacksRequest describeStacksRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeStacksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeStacksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeStacks");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeStacks").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeStacksRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeStacksRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes time-based auto scaling configurations for specified instances.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeTimeBasedAutoScalingRequest
* @return Result of the DescribeTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeTimeBasedAutoScaling
* @see AWS API Documentation
*/
@Override
public DescribeTimeBasedAutoScalingResponse describeTimeBasedAutoScaling(
DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeTimeBasedAutoScalingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeTimeBasedAutoScalingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeTimeBasedAutoScaling");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeTimeBasedAutoScaling").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeTimeBasedAutoScalingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeTimeBasedAutoScalingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describe specified users.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param describeUserProfilesRequest
* @return Result of the DescribeUserProfiles operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeUserProfiles
* @see AWS
* API Documentation
*/
@Override
public DescribeUserProfilesResponse describeUserProfiles(DescribeUserProfilesRequest describeUserProfilesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeUserProfilesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeUserProfilesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeUserProfiles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeUserProfiles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeUserProfilesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeUserProfilesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeVolumesRequest
* @return Result of the DescribeVolumes operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeVolumes
* @see AWS API
* Documentation
*/
@Override
public DescribeVolumesResponse describeVolumes(DescribeVolumesRequest describeVolumesRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeVolumesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeVolumesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeVolumes");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DescribeVolumes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(describeVolumesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DescribeVolumesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param detachElasticLoadBalancerRequest
* @return Result of the DetachElasticLoadBalancer operation returned by the service.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DetachElasticLoadBalancer
* @see AWS API Documentation
*/
@Override
public DetachElasticLoadBalancerResponse detachElasticLoadBalancer(
DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DetachElasticLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, detachElasticLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DetachElasticLoadBalancer");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DetachElasticLoadBalancer").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(detachElasticLoadBalancerRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DetachElasticLoadBalancerRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Disassociates an Elastic IP address from its instance. The address remains registered with the stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param disassociateElasticIpRequest
* @return Result of the DisassociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DisassociateElasticIp
* @see AWS
* API Documentation
*/
@Override
public DisassociateElasticIpResponse disassociateElasticIp(DisassociateElasticIpRequest disassociateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateElasticIp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DisassociateElasticIp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateElasticIpRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateElasticIpRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Gets a generated host name for the specified layer, based on the current host name theme.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param getHostnameSuggestionRequest
* @return Result of the GetHostnameSuggestion operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GetHostnameSuggestion
* @see AWS
* API Documentation
*/
@Override
public GetHostnameSuggestionResponse getHostnameSuggestion(GetHostnameSuggestionRequest getHostnameSuggestionRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetHostnameSuggestionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getHostnameSuggestionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetHostnameSuggestion");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetHostnameSuggestion").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getHostnameSuggestionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetHostnameSuggestionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This action can be used only with Windows stacks.
*
*
*
* Grants RDP access to a Windows instance for a specified time period.
*
*
* @param grantAccessRequest
* @return Result of the GrantAccess operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GrantAccess
* @see AWS API
* Documentation
*/
@Override
public GrantAccessResponse grantAccess(GrantAccessRequest grantAccessRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GrantAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, grantAccessRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GrantAccess");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GrantAccess").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(grantAccessRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GrantAccessRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Returns a list of tags that are applied to the specified stack or layer.
*
*
* @param listTagsRequest
* @return Result of the ListTags operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.ListTags
* @see AWS API
* Documentation
*/
@Override
public ListTagsResponse listTags(ListTagsRequest listTagsRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListTagsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTags");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTags").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new ListTagsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Reboots a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param rebootInstanceRequest
* @return Result of the RebootInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RebootInstance
* @see AWS API
* Documentation
*/
@Override
public RebootInstanceResponse rebootInstance(RebootInstanceRequest rebootInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RebootInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, rebootInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RebootInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RebootInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(rebootInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RebootInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers a specified Amazon ECS cluster with a stack. You can register only one cluster with a stack. A cluster
* can be registered with only one stack. For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerEcsClusterRequest
* @return Result of the RegisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterEcsCluster
* @see AWS
* API Documentation
*/
@Override
public RegisterEcsClusterResponse registerEcsCluster(RegisterEcsClusterRequest registerEcsClusterRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RegisterEcsClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerEcsClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterEcsCluster");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterEcsCluster").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerEcsClusterRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterEcsClusterRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can be registered with only one stack at a
* time. If the address is already registered, you must first deregister it by calling DeregisterElasticIp.
* For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerElasticIpRequest
* @return Result of the RegisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterElasticIp
* @see AWS API
* Documentation
*/
@Override
public RegisterElasticIpResponse registerElasticIp(RegisterElasticIpRequest registerElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RegisterElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterElasticIp");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterElasticIp").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerElasticIpRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterElasticIpRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers instances that were created outside of AWS OpsWorks Stacks with a specified stack.
*
*
*
* We do not recommend using this action to register instances. The complete registration operation includes two
* tasks: installing the AWS OpsWorks Stacks agent on the instance, and registering the instance with the stack.
* RegisterInstance
handles only the second step. You should instead use the AWS CLI
* register
command, which performs the entire registration operation. For more information, see Registering an
* Instance with an AWS OpsWorks Stacks Stack.
*
*
*
* Registered instances have the same requirements as instances that are created by using the CreateInstance
* API. For example, registered instances must be running a supported Linux-based operating system, and they must
* have a supported instance type. For more information about requirements for instances that you want to register,
* see
* Preparing the Instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerInstanceRequest
* @return Result of the RegisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterInstance
* @see AWS API
* Documentation
*/
@Override
public RegisterInstanceResponse registerInstance(RegisterInstanceRequest registerInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RegisterInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers an Amazon RDS instance with a stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerRdsDbInstanceRequest
* @return Result of the RegisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterRdsDbInstance
* @see AWS
* API Documentation
*/
@Override
public RegisterRdsDbInstanceResponse registerRdsDbInstance(RegisterRdsDbInstanceRequest registerRdsDbInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RegisterRdsDbInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerRdsDbInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterRdsDbInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterRdsDbInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerRdsDbInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterRdsDbInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be registered with only one stack at a time.
* If the volume is already registered, you must first deregister it by calling DeregisterVolume. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerVolumeRequest
* @return Result of the RegisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterVolume
* @see AWS API
* Documentation
*/
@Override
public RegisterVolumeResponse registerVolume(RegisterVolumeRequest registerVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RegisterVolumeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerVolumeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterVolume");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterVolume").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerVolumeRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterVolumeRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Specify the load-based auto scaling configuration for a specified layer. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
*
* To use load-based auto scaling, you must create a set of load-based auto scaling instances. Load-based auto
* scaling operates only on the instances from that set, so you must ensure that you have created enough instances
* to handle the maximum anticipated load.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setLoadBasedAutoScalingRequest
* @return Result of the SetLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetLoadBasedAutoScaling
* @see AWS API Documentation
*/
@Override
public SetLoadBasedAutoScalingResponse setLoadBasedAutoScaling(SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetLoadBasedAutoScalingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setLoadBasedAutoScalingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetLoadBasedAutoScaling");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetLoadBasedAutoScaling").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setLoadBasedAutoScalingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetLoadBasedAutoScalingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Specifies a user's permissions. For more information, see Security and Permissions.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setPermissionRequest
* @return Result of the SetPermission operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetPermission
* @see AWS API
* Documentation
*/
@Override
public SetPermissionResponse setPermission(SetPermissionRequest setPermissionRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SetPermissionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setPermissionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetPermission");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("SetPermission").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setPermissionRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetPermissionRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Specify the time-based auto scaling configuration for a specified instance. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setTimeBasedAutoScalingRequest
* @return Result of the SetTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetTimeBasedAutoScaling
* @see AWS API Documentation
*/
@Override
public SetTimeBasedAutoScalingResponse setTimeBasedAutoScaling(SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, SetTimeBasedAutoScalingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, setTimeBasedAutoScalingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SetTimeBasedAutoScaling");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("SetTimeBasedAutoScaling").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(setTimeBasedAutoScalingRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new SetTimeBasedAutoScalingRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param startInstanceRequest
* @return Result of the StartInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartInstance
* @see AWS API
* Documentation
*/
@Override
public StartInstanceResponse startInstance(StartInstanceRequest startInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StartInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(startInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Starts a stack's instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param startStackRequest
* @return Result of the StartStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartStack
* @see AWS API
* Documentation
*/
@Override
public StartStackResponse startStack(StartStackRequest startStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StartStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartStack");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("StartStack")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(startStackRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StartStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Stops a specified instance. When you stop a standard instance, the data disappears and must be reinstalled when
* you restart the instance. You can stop an Amazon EBS-backed instance without losing data. For more information,
* see Starting,
* Stopping, and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param stopInstanceRequest
* @return Result of the StopInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopInstance
* @see AWS API
* Documentation
*/
@Override
public StopInstanceResponse stopInstance(StopInstanceRequest stopInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StopInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopInstance");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StopInstance").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(stopInstanceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new StopInstanceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Stops a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param stopStackRequest
* @return Result of the StopStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopStack
* @see AWS API
* Documentation
*/
@Override
public StopStackResponse stopStack(StopStackRequest stopStackRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
StopStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, stopStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StopStack");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("StopStack").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(stopStackRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new StopStackRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Apply cost-allocation tags to a specified stack or layer in AWS OpsWorks Stacks. For more information about how
* tagging works, see Tags in the
* AWS OpsWorks User Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Unassigns a registered instance from all layers that are using the instance. The instance remains in the stack as
* an unassigned instance, and can be assigned to another layer as needed. You cannot use this action with instances
* that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param unassignInstanceRequest
* @return Result of the UnassignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UnassignInstance
* @see AWS API
* Documentation
*/
@Override
public UnassignInstanceResponse unassignInstance(UnassignInstanceRequest unassignInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UnassignInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List