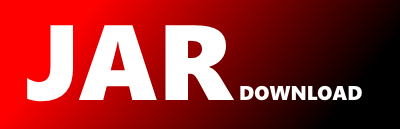
software.amazon.awssdk.services.opsworks.OpsWorksClient Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.CloneStackRequest;
import software.amazon.awssdk.services.opsworks.model.CloneStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateAppRequest;
import software.amazon.awssdk.services.opsworks.model.CreateAppResponse;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.CreateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.CreateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.CreateStackRequest;
import software.amazon.awssdk.services.opsworks.model.CreateStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteAppRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteAppResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteStackRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteStackResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesResponse;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionRequest;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionResponse;
import software.amazon.awssdk.services.opsworks.model.GrantAccessRequest;
import software.amazon.awssdk.services.opsworks.model.GrantAccessResponse;
import software.amazon.awssdk.services.opsworks.model.ListTagsRequest;
import software.amazon.awssdk.services.opsworks.model.ListTagsResponse;
import software.amazon.awssdk.services.opsworks.model.OpsWorksException;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ResourceNotFoundException;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.SetPermissionRequest;
import software.amazon.awssdk.services.opsworks.model.SetPermissionResponse;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.StartInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StartInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StartStackRequest;
import software.amazon.awssdk.services.opsworks.model.StartStackResponse;
import software.amazon.awssdk.services.opsworks.model.StopInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StopInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StopStackRequest;
import software.amazon.awssdk.services.opsworks.model.StopStackResponse;
import software.amazon.awssdk.services.opsworks.model.TagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.TagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.UntagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.UntagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateAppRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateAppResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateStackRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateStackResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ValidationException;
import software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable;
import software.amazon.awssdk.services.opsworks.waiters.OpsWorksWaiter;
/**
* Service client for accessing AWS OpsWorks. This can be created using the static {@link #builder()} method.
*
* AWS OpsWorks
*
* Welcome to the AWS OpsWorks Stacks API Reference. This guide provides descriptions, syntax, and usage examples
* for AWS OpsWorks Stacks actions and data types, including common parameters and error codes.
*
*
* AWS OpsWorks Stacks is an application management service that provides an integrated experience for overseeing the
* complete application lifecycle. For information about this product, go to the AWS OpsWorks details page.
*
*
* SDKs and CLI
*
*
* The most common way to use the AWS OpsWorks Stacks API is by using the AWS Command Line Interface (CLI) or by using
* one of the AWS SDKs to implement applications in your preferred language. For more information, see:
*
*
* -
*
* AWS CLI
*
*
* -
*
* AWS SDK for Java
*
*
* -
*
* AWS SDK for .NET
*
*
* -
*
*
* -
*
* AWS SDK for Ruby
*
*
* -
*
*
* -
*
*
*
*
* Endpoints
*
*
* AWS OpsWorks Stacks supports the following endpoints, all HTTPS. You must connect to one of the following endpoints.
* Stacks can only be accessed or managed within the endpoint in which they are created.
*
*
* -
*
* opsworks.us-east-1.amazonaws.com
*
*
* -
*
* opsworks.us-east-2.amazonaws.com
*
*
* -
*
* opsworks.us-west-1.amazonaws.com
*
*
* -
*
* opsworks.us-west-2.amazonaws.com
*
*
* -
*
* opsworks.ca-central-1.amazonaws.com (API only; not available in the AWS console)
*
*
* -
*
* opsworks.eu-west-1.amazonaws.com
*
*
* -
*
* opsworks.eu-west-2.amazonaws.com
*
*
* -
*
* opsworks.eu-west-3.amazonaws.com
*
*
* -
*
* opsworks.eu-central-1.amazonaws.com
*
*
* -
*
* opsworks.ap-northeast-1.amazonaws.com
*
*
* -
*
* opsworks.ap-northeast-2.amazonaws.com
*
*
* -
*
* opsworks.ap-south-1.amazonaws.com
*
*
* -
*
* opsworks.ap-southeast-1.amazonaws.com
*
*
* -
*
* opsworks.ap-southeast-2.amazonaws.com
*
*
* -
*
* opsworks.sa-east-1.amazonaws.com
*
*
*
*
* Chef Versions
*
*
* When you call CreateStack, CloneStack, or UpdateStack we recommend you use the
* ConfigurationManager
parameter to specify the Chef version. The recommended and default value for Linux
* stacks is currently 12. Windows stacks use Chef 12.2. For more information, see Chef Versions.
*
*
*
* You can specify Chef 12, 11.10, or 11.4 for your Linux stack. We recommend migrating your existing Linux stacks to
* Chef 12 as soon as possible.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface OpsWorksClient extends AwsClient {
String SERVICE_NAME = "opsworks";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "opsworks";
/**
*
* Assign a registered instance to a layer.
*
*
* -
*
* You can assign registered on-premises instances to any layer type.
*
*
* -
*
* You can assign registered Amazon EC2 instances only to custom layers.
*
*
* -
*
* You cannot use this action with instances that were created with AWS OpsWorks Stacks.
*
*
*
*
* Required Permissions: To use this action, an AWS Identity and Access Management (IAM) user must have a
* Manage permissions level for the stack or an attached policy that explicitly grants permissions. For more
* information on user permissions, see Managing User
* Permissions.
*
*
* @param assignInstanceRequest
* @return Result of the AssignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignInstance
* @see AWS API
* Documentation
*/
default AssignInstanceResponse assignInstance(AssignInstanceRequest assignInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Assign a registered instance to a layer.
*
*
* -
*
* You can assign registered on-premises instances to any layer type.
*
*
* -
*
* You can assign registered Amazon EC2 instances only to custom layers.
*
*
* -
*
* You cannot use this action with instances that were created with AWS OpsWorks Stacks.
*
*
*
*
* Required Permissions: To use this action, an AWS Identity and Access Management (IAM) user must have a
* Manage permissions level for the stack or an attached policy that explicitly grants permissions. For more
* information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AssignInstanceRequest.Builder} avoiding the need to
* create one manually via {@link AssignInstanceRequest#builder()}
*
*
* @param assignInstanceRequest
* A {@link Consumer} that will call methods on {@link AssignInstanceRequest.Builder} to create a request.
* @return Result of the AssignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignInstance
* @see AWS API
* Documentation
*/
default AssignInstanceResponse assignInstance(Consumer assignInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return assignInstance(AssignInstanceRequest.builder().applyMutation(assignInstanceRequest).build());
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a specified instance. The volume must first be
* registered with the stack by calling RegisterVolume. After you register the volume, you must call
* UpdateVolume to specify a mount point before calling AssignVolume
. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param assignVolumeRequest
* @return Result of the AssignVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignVolume
* @see AWS API
* Documentation
*/
default AssignVolumeResponse assignVolume(AssignVolumeRequest assignVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a specified instance. The volume must first be
* registered with the stack by calling RegisterVolume. After you register the volume, you must call
* UpdateVolume to specify a mount point before calling AssignVolume
. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AssignVolumeRequest.Builder} avoiding the need to
* create one manually via {@link AssignVolumeRequest#builder()}
*
*
* @param assignVolumeRequest
* A {@link Consumer} that will call methods on {@link AssignVolumeRequest.Builder} to create a request.
* @return Result of the AssignVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssignVolume
* @see AWS API
* Documentation
*/
default AssignVolumeResponse assignVolume(Consumer assignVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return assignVolume(AssignVolumeRequest.builder().applyMutation(assignVolumeRequest).build());
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a specified instance. The address must first
* be registered with the stack by calling RegisterElasticIp. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param associateElasticIpRequest
* @return Result of the AssociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssociateElasticIp
* @see AWS
* API Documentation
*/
default AssociateElasticIpResponse associateElasticIp(AssociateElasticIpRequest associateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a specified instance. The address must first
* be registered with the stack by calling RegisterElasticIp. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AssociateElasticIpRequest.Builder} avoiding the
* need to create one manually via {@link AssociateElasticIpRequest#builder()}
*
*
* @param associateElasticIpRequest
* A {@link Consumer} that will call methods on {@link AssociateElasticIpRequest.Builder} to create a
* request.
* @return Result of the AssociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AssociateElasticIp
* @see AWS
* API Documentation
*/
default AssociateElasticIpResponse associateElasticIp(Consumer associateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return associateElasticIp(AssociateElasticIpRequest.builder().applyMutation(associateElasticIpRequest).build());
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer. AWS OpsWorks Stacks does not support
* Application Load Balancer. You can only use Classic Load Balancer with AWS OpsWorks Stacks. For more information,
* see Elastic Load Balancing.
*
*
*
* You must create the Elastic Load Balancing instance separately, by using the Elastic Load Balancing console, API,
* or CLI. For more information, see Elastic Load
* Balancing Developer Guide.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param attachElasticLoadBalancerRequest
* @return Result of the AttachElasticLoadBalancer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AttachElasticLoadBalancer
* @see AWS API Documentation
*/
default AttachElasticLoadBalancerResponse attachElasticLoadBalancer(
AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer. AWS OpsWorks Stacks does not support
* Application Load Balancer. You can only use Classic Load Balancer with AWS OpsWorks Stacks. For more information,
* see Elastic Load Balancing.
*
*
*
* You must create the Elastic Load Balancing instance separately, by using the Elastic Load Balancing console, API,
* or CLI. For more information, see Elastic Load
* Balancing Developer Guide.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link AttachElasticLoadBalancerRequest.Builder} avoiding
* the need to create one manually via {@link AttachElasticLoadBalancerRequest#builder()}
*
*
* @param attachElasticLoadBalancerRequest
* A {@link Consumer} that will call methods on {@link AttachElasticLoadBalancerRequest.Builder} to create a
* request.
* @return Result of the AttachElasticLoadBalancer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.AttachElasticLoadBalancer
* @see AWS API Documentation
*/
default AttachElasticLoadBalancerResponse attachElasticLoadBalancer(
Consumer attachElasticLoadBalancerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return attachElasticLoadBalancer(AttachElasticLoadBalancerRequest.builder()
.applyMutation(attachElasticLoadBalancerRequest).build());
}
/**
*
* Creates a clone of a specified stack. For more information, see Clone a Stack. By
* default, all parameters are set to the values used by the parent stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param cloneStackRequest
* @return Result of the CloneStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CloneStack
* @see AWS API
* Documentation
*/
default CloneStackResponse cloneStack(CloneStackRequest cloneStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a clone of a specified stack. For more information, see Clone a Stack. By
* default, all parameters are set to the values used by the parent stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CloneStackRequest.Builder} avoiding the need to
* create one manually via {@link CloneStackRequest#builder()}
*
*
* @param cloneStackRequest
* A {@link Consumer} that will call methods on {@link CloneStackRequest.Builder} to create a request.
* @return Result of the CloneStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CloneStack
* @see AWS API
* Documentation
*/
default CloneStackResponse cloneStack(Consumer cloneStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return cloneStack(CloneStackRequest.builder().applyMutation(cloneStackRequest).build());
}
/**
*
* Creates an app for a specified stack. For more information, see Creating Apps.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createAppRequest
* @return Result of the CreateApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateApp
* @see AWS API
* Documentation
*/
default CreateAppResponse createApp(CreateAppRequest createAppRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an app for a specified stack. For more information, see Creating Apps.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateAppRequest.Builder} avoiding the need to
* create one manually via {@link CreateAppRequest#builder()}
*
*
* @param createAppRequest
* A {@link Consumer} that will call methods on {@link CreateAppRequest.Builder} to create a request.
* @return Result of the CreateApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateApp
* @see AWS API
* Documentation
*/
default CreateAppResponse createApp(Consumer createAppRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return createApp(CreateAppRequest.builder().applyMutation(createAppRequest).build());
}
/**
*
* Runs deployment or stack commands. For more information, see Deploying Apps and Run Stack Commands.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
* @param createDeploymentRequest
* @return Result of the CreateDeployment operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateDeployment
* @see AWS API
* Documentation
*/
default CreateDeploymentResponse createDeployment(CreateDeploymentRequest createDeploymentRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Runs deployment or stack commands. For more information, see Deploying Apps and Run Stack Commands.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateDeploymentRequest.Builder} avoiding the need
* to create one manually via {@link CreateDeploymentRequest#builder()}
*
*
* @param createDeploymentRequest
* A {@link Consumer} that will call methods on {@link CreateDeploymentRequest.Builder} to create a request.
* @return Result of the CreateDeployment operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateDeployment
* @see AWS API
* Documentation
*/
default CreateDeploymentResponse createDeployment(Consumer createDeploymentRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return createDeployment(CreateDeploymentRequest.builder().applyMutation(createDeploymentRequest).build());
}
/**
*
* Creates an instance in a specified stack. For more information, see Adding an Instance to a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createInstanceRequest
* @return Result of the CreateInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateInstance
* @see AWS API
* Documentation
*/
default CreateInstanceResponse createInstance(CreateInstanceRequest createInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an instance in a specified stack. For more information, see Adding an Instance to a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateInstanceRequest.Builder} avoiding the need to
* create one manually via {@link CreateInstanceRequest#builder()}
*
*
* @param createInstanceRequest
* A {@link Consumer} that will call methods on {@link CreateInstanceRequest.Builder} to create a request.
* @return Result of the CreateInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateInstance
* @see AWS API
* Documentation
*/
default CreateInstanceResponse createInstance(Consumer createInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return createInstance(CreateInstanceRequest.builder().applyMutation(createInstanceRequest).build());
}
/**
*
* Creates a layer. For more information, see How to Create a
* Layer.
*
*
*
* You should use CreateLayer for noncustom layer types such as PHP App Server only if the stack does not
* have an existing layer of that type. A stack can have at most one instance of each noncustom layer; if you
* attempt to create a second instance, CreateLayer fails. A stack can have an arbitrary number of custom
* layers, so you can call CreateLayer as many times as you like for that layer type.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createLayerRequest
* @return Result of the CreateLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateLayer
* @see AWS API
* Documentation
*/
default CreateLayerResponse createLayer(CreateLayerRequest createLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a layer. For more information, see How to Create a
* Layer.
*
*
*
* You should use CreateLayer for noncustom layer types such as PHP App Server only if the stack does not
* have an existing layer of that type. A stack can have at most one instance of each noncustom layer; if you
* attempt to create a second instance, CreateLayer fails. A stack can have an arbitrary number of custom
* layers, so you can call CreateLayer as many times as you like for that layer type.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLayerRequest.Builder} avoiding the need to
* create one manually via {@link CreateLayerRequest#builder()}
*
*
* @param createLayerRequest
* A {@link Consumer} that will call methods on {@link CreateLayerRequest.Builder} to create a request.
* @return Result of the CreateLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateLayer
* @see AWS API
* Documentation
*/
default CreateLayerResponse createLayer(Consumer createLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return createLayer(CreateLayerRequest.builder().applyMutation(createLayerRequest).build());
}
/**
*
* Creates a new stack. For more information, see Create a New Stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createStackRequest
* @return Result of the CreateStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateStack
* @see AWS API
* Documentation
*/
default CreateStackResponse createStack(CreateStackRequest createStackRequest) throws ValidationException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new stack. For more information, see Create a New Stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStackRequest.Builder} avoiding the need to
* create one manually via {@link CreateStackRequest#builder()}
*
*
* @param createStackRequest
* A {@link Consumer} that will call methods on {@link CreateStackRequest.Builder} to create a request.
* @return Result of the CreateStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateStack
* @see AWS API
* Documentation
*/
default CreateStackResponse createStack(Consumer createStackRequest) throws ValidationException,
AwsServiceException, SdkClientException, OpsWorksException {
return createStack(CreateStackRequest.builder().applyMutation(createStackRequest).build());
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createUserProfileRequest
* @return Result of the CreateUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateUserProfile
* @see AWS
* API Documentation
*/
default CreateUserProfileResponse createUserProfile(CreateUserProfileRequest createUserProfileRequest)
throws ValidationException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link CreateUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link CreateUserProfileRequest#builder()}
*
*
* @param createUserProfileRequest
* A {@link Consumer} that will call methods on {@link CreateUserProfileRequest.Builder} to create a request.
* @return Result of the CreateUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.CreateUserProfile
* @see AWS
* API Documentation
*/
default CreateUserProfileResponse createUserProfile(Consumer createUserProfileRequest)
throws ValidationException, AwsServiceException, SdkClientException, OpsWorksException {
return createUserProfile(CreateUserProfileRequest.builder().applyMutation(createUserProfileRequest).build());
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteAppRequest
* @return Result of the DeleteApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteApp
* @see AWS API
* Documentation
*/
default DeleteAppResponse deleteApp(DeleteAppRequest deleteAppRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteAppRequest.Builder} avoiding the need to
* create one manually via {@link DeleteAppRequest#builder()}
*
*
* @param deleteAppRequest
* A {@link Consumer} that will call methods on {@link DeleteAppRequest.Builder} to create a request.
* @return Result of the DeleteApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteApp
* @see AWS API
* Documentation
*/
default DeleteAppResponse deleteApp(Consumer deleteAppRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deleteApp(DeleteAppRequest.builder().applyMutation(deleteAppRequest).build());
}
/**
*
* Deletes a specified instance, which terminates the associated Amazon EC2 instance. You must stop an instance
* before you can delete it.
*
*
* For more information, see Deleting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteInstanceRequest
* @return Result of the DeleteInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteInstance
* @see AWS API
* Documentation
*/
default DeleteInstanceResponse deleteInstance(DeleteInstanceRequest deleteInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified instance, which terminates the associated Amazon EC2 instance. You must stop an instance
* before you can delete it.
*
*
* For more information, see Deleting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteInstanceRequest.Builder} avoiding the need to
* create one manually via {@link DeleteInstanceRequest#builder()}
*
*
* @param deleteInstanceRequest
* A {@link Consumer} that will call methods on {@link DeleteInstanceRequest.Builder} to create a request.
* @return Result of the DeleteInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteInstance
* @see AWS API
* Documentation
*/
default DeleteInstanceResponse deleteInstance(Consumer deleteInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deleteInstance(DeleteInstanceRequest.builder().applyMutation(deleteInstanceRequest).build());
}
/**
*
* Deletes a specified layer. You must first stop and then delete all associated instances or unassign registered
* instances. For more information, see How to Delete a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteLayerRequest
* @return Result of the DeleteLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteLayer
* @see AWS API
* Documentation
*/
default DeleteLayerResponse deleteLayer(DeleteLayerRequest deleteLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified layer. You must first stop and then delete all associated instances or unassign registered
* instances. For more information, see How to Delete a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLayerRequest.Builder} avoiding the need to
* create one manually via {@link DeleteLayerRequest#builder()}
*
*
* @param deleteLayerRequest
* A {@link Consumer} that will call methods on {@link DeleteLayerRequest.Builder} to create a request.
* @return Result of the DeleteLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteLayer
* @see AWS API
* Documentation
*/
default DeleteLayerResponse deleteLayer(Consumer deleteLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deleteLayer(DeleteLayerRequest.builder().applyMutation(deleteLayerRequest).build());
}
/**
*
* Deletes a specified stack. You must first delete all instances, layers, and apps or deregister registered
* instances. For more information, see Shut Down a Stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteStackRequest
* @return Result of the DeleteStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteStack
* @see AWS API
* Documentation
*/
default DeleteStackResponse deleteStack(DeleteStackRequest deleteStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified stack. You must first delete all instances, layers, and apps or deregister registered
* instances. For more information, see Shut Down a Stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStackRequest.Builder} avoiding the need to
* create one manually via {@link DeleteStackRequest#builder()}
*
*
* @param deleteStackRequest
* A {@link Consumer} that will call methods on {@link DeleteStackRequest.Builder} to create a request.
* @return Result of the DeleteStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteStack
* @see AWS API
* Documentation
*/
default DeleteStackResponse deleteStack(Consumer deleteStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deleteStack(DeleteStackRequest.builder().applyMutation(deleteStackRequest).build());
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param deleteUserProfileRequest
* @return Result of the DeleteUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteUserProfile
* @see AWS
* API Documentation
*/
default DeleteUserProfileResponse deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link DeleteUserProfileRequest#builder()}
*
*
* @param deleteUserProfileRequest
* A {@link Consumer} that will call methods on {@link DeleteUserProfileRequest.Builder} to create a request.
* @return Result of the DeleteUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeleteUserProfile
* @see AWS
* API Documentation
*/
default DeleteUserProfileResponse deleteUserProfile(Consumer deleteUserProfileRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deleteUserProfile(DeleteUserProfileRequest.builder().applyMutation(deleteUserProfileRequest).build());
}
/**
*
* Deregisters a specified Amazon ECS cluster from a stack. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see https://docs.aws.amazon.com/opsworks/latest/userguide/opsworks-security-users.html.
*
*
* @param deregisterEcsClusterRequest
* @return Result of the DeregisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterEcsCluster
* @see AWS
* API Documentation
*/
default DeregisterEcsClusterResponse deregisterEcsCluster(DeregisterEcsClusterRequest deregisterEcsClusterRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a specified Amazon ECS cluster from a stack. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see https://docs.aws.amazon.com/opsworks/latest/userguide/opsworks-security-users.html.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterEcsClusterRequest.Builder} avoiding the
* need to create one manually via {@link DeregisterEcsClusterRequest#builder()}
*
*
* @param deregisterEcsClusterRequest
* A {@link Consumer} that will call methods on {@link DeregisterEcsClusterRequest.Builder} to create a
* request.
* @return Result of the DeregisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterEcsCluster
* @see AWS
* API Documentation
*/
default DeregisterEcsClusterResponse deregisterEcsCluster(
Consumer deregisterEcsClusterRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deregisterEcsCluster(DeregisterEcsClusterRequest.builder().applyMutation(deregisterEcsClusterRequest).build());
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be registered by another stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterElasticIpRequest
* @return Result of the DeregisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterElasticIp
* @see AWS
* API Documentation
*/
default DeregisterElasticIpResponse deregisterElasticIp(DeregisterElasticIpRequest deregisterElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters a specified Elastic IP address. The address can then be registered by another stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterElasticIpRequest.Builder} avoiding the
* need to create one manually via {@link DeregisterElasticIpRequest#builder()}
*
*
* @param deregisterElasticIpRequest
* A {@link Consumer} that will call methods on {@link DeregisterElasticIpRequest.Builder} to create a
* request.
* @return Result of the DeregisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterElasticIp
* @see AWS
* API Documentation
*/
default DeregisterElasticIpResponse deregisterElasticIp(
Consumer deregisterElasticIpRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deregisterElasticIp(DeregisterElasticIpRequest.builder().applyMutation(deregisterElasticIpRequest).build());
}
/**
*
* Deregister a registered Amazon EC2 or on-premises instance. This action removes the instance from the stack and
* returns it to your control. This action cannot be used with instances that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterInstanceRequest
* @return Result of the DeregisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterInstance
* @see AWS
* API Documentation
*/
default DeregisterInstanceResponse deregisterInstance(DeregisterInstanceRequest deregisterInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregister a registered Amazon EC2 or on-premises instance. This action removes the instance from the stack and
* returns it to your control. This action cannot be used with instances that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterInstanceRequest.Builder} avoiding the
* need to create one manually via {@link DeregisterInstanceRequest#builder()}
*
*
* @param deregisterInstanceRequest
* A {@link Consumer} that will call methods on {@link DeregisterInstanceRequest.Builder} to create a
* request.
* @return Result of the DeregisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterInstance
* @see AWS
* API Documentation
*/
default DeregisterInstanceResponse deregisterInstance(Consumer deregisterInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deregisterInstance(DeregisterInstanceRequest.builder().applyMutation(deregisterInstanceRequest).build());
}
/**
*
* Deregisters an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterRdsDbInstanceRequest
* @return Result of the DeregisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterRdsDbInstance
* @see AWS API Documentation
*/
default DeregisterRdsDbInstanceResponse deregisterRdsDbInstance(DeregisterRdsDbInstanceRequest deregisterRdsDbInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterRdsDbInstanceRequest.Builder} avoiding
* the need to create one manually via {@link DeregisterRdsDbInstanceRequest#builder()}
*
*
* @param deregisterRdsDbInstanceRequest
* A {@link Consumer} that will call methods on {@link DeregisterRdsDbInstanceRequest.Builder} to create a
* request.
* @return Result of the DeregisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterRdsDbInstance
* @see AWS API Documentation
*/
default DeregisterRdsDbInstanceResponse deregisterRdsDbInstance(
Consumer deregisterRdsDbInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deregisterRdsDbInstance(DeregisterRdsDbInstanceRequest.builder().applyMutation(deregisterRdsDbInstanceRequest)
.build());
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by another stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterVolumeRequest
* @return Result of the DeregisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterVolume
* @see AWS API
* Documentation
*/
default DeregisterVolumeResponse deregisterVolume(DeregisterVolumeRequest deregisterVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by another stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DeregisterVolumeRequest.Builder} avoiding the need
* to create one manually via {@link DeregisterVolumeRequest#builder()}
*
*
* @param deregisterVolumeRequest
* A {@link Consumer} that will call methods on {@link DeregisterVolumeRequest.Builder} to create a request.
* @return Result of the DeregisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DeregisterVolume
* @see AWS API
* Documentation
*/
default DeregisterVolumeResponse deregisterVolume(Consumer deregisterVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return deregisterVolume(DeregisterVolumeRequest.builder().applyMutation(deregisterVolumeRequest).build());
}
/**
*
* Describes the available AWS OpsWorks Stacks agent versions. You must specify a stack ID or a configuration
* manager. DescribeAgentVersions
returns a list of available agent versions for the specified stack or
* configuration manager.
*
*
* @param describeAgentVersionsRequest
* @return Result of the DescribeAgentVersions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeAgentVersions
* @see AWS API Documentation
*/
default DescribeAgentVersionsResponse describeAgentVersions(DescribeAgentVersionsRequest describeAgentVersionsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the available AWS OpsWorks Stacks agent versions. You must specify a stack ID or a configuration
* manager. DescribeAgentVersions
returns a list of available agent versions for the specified stack or
* configuration manager.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAgentVersionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeAgentVersionsRequest#builder()}
*
*
* @param describeAgentVersionsRequest
* A {@link Consumer} that will call methods on {@link DescribeAgentVersionsRequest.Builder} to create a
* request.
* @return Result of the DescribeAgentVersions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeAgentVersions
* @see AWS API Documentation
*/
default DescribeAgentVersionsResponse describeAgentVersions(
Consumer describeAgentVersionsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeAgentVersions(DescribeAgentVersionsRequest.builder().applyMutation(describeAgentVersionsRequest).build());
}
/**
*
* Requests a description of a specified set of apps.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeAppsRequest
* @return Result of the DescribeApps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeApps
* @see AWS API
* Documentation
*/
default DescribeAppsResponse describeApps(DescribeAppsRequest describeAppsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of a specified set of apps.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeAppsRequest.Builder} avoiding the need to
* create one manually via {@link DescribeAppsRequest#builder()}
*
*
* @param describeAppsRequest
* A {@link Consumer} that will call methods on {@link DescribeAppsRequest.Builder} to create a request.
* @return Result of the DescribeApps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeApps
* @see AWS API
* Documentation
*/
default DescribeAppsResponse describeApps(Consumer describeAppsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeApps(DescribeAppsRequest.builder().applyMutation(describeAppsRequest).build());
}
/**
*
* Describes the results of specified commands.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeCommandsRequest
* @return Result of the DescribeCommands operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeCommands
* @see AWS API
* Documentation
*/
default DescribeCommandsResponse describeCommands(DescribeCommandsRequest describeCommandsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the results of specified commands.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeCommandsRequest.Builder} avoiding the need
* to create one manually via {@link DescribeCommandsRequest#builder()}
*
*
* @param describeCommandsRequest
* A {@link Consumer} that will call methods on {@link DescribeCommandsRequest.Builder} to create a request.
* @return Result of the DescribeCommands operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeCommands
* @see AWS API
* Documentation
*/
default DescribeCommandsResponse describeCommands(Consumer describeCommandsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeCommands(DescribeCommandsRequest.builder().applyMutation(describeCommandsRequest).build());
}
/**
*
* Requests a description of a specified set of deployments.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeDeploymentsRequest
* @return Result of the DescribeDeployments operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeDeployments
* @see AWS
* API Documentation
*/
default DescribeDeploymentsResponse describeDeployments(DescribeDeploymentsRequest describeDeploymentsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of a specified set of deployments.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeDeploymentsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeDeploymentsRequest#builder()}
*
*
* @param describeDeploymentsRequest
* A {@link Consumer} that will call methods on {@link DescribeDeploymentsRequest.Builder} to create a
* request.
* @return Result of the DescribeDeployments operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeDeployments
* @see AWS
* API Documentation
*/
default DescribeDeploymentsResponse describeDeployments(
Consumer describeDeploymentsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeDeployments(DescribeDeploymentsRequest.builder().applyMutation(describeDeploymentsRequest).build());
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeEcsClustersRequest
* @return Result of the DescribeEcsClusters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
default DescribeEcsClustersResponse describeEcsClusters(DescribeEcsClustersRequest describeEcsClustersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeEcsClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeEcsClustersRequest#builder()}
*
*
* @param describeEcsClustersRequest
* A {@link Consumer} that will call methods on {@link DescribeEcsClustersRequest.Builder} to create a
* request.
* @return Result of the DescribeEcsClusters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
default DescribeEcsClustersResponse describeEcsClusters(
Consumer describeEcsClustersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeEcsClusters(DescribeEcsClustersRequest.builder().applyMutation(describeEcsClustersRequest).build());
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a variant of
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client
* .describeEcsClustersPaginator(request);
* for (software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation.
*
*
* @param describeEcsClustersRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
default DescribeEcsClustersIterable describeEcsClustersPaginator(DescribeEcsClustersRequest describeEcsClustersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, AWS OpsWorks
* Stacks currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a variant of
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client
* .describeEcsClustersPaginator(request);
* for (software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.opsworks.paginators.DescribeEcsClustersIterable responses = client.describeEcsClustersPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #describeEcsClusters(software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link DescribeEcsClustersRequest.Builder} avoiding the
* need to create one manually via {@link DescribeEcsClustersRequest#builder()}
*
*
* @param describeEcsClustersRequest
* A {@link Consumer} that will call methods on {@link DescribeEcsClustersRequest.Builder} to create a
* request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
default DescribeEcsClustersIterable describeEcsClustersPaginator(
Consumer describeEcsClustersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeEcsClustersPaginator(DescribeEcsClustersRequest.builder().applyMutation(describeEcsClustersRequest)
.build());
}
/**
*
* Describes Elastic IP
* addresses.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticIpsRequest
* @return Result of the DescribeElasticIps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticIps
* @see AWS
* API Documentation
*/
default DescribeElasticIpsResponse describeElasticIps(DescribeElasticIpsRequest describeElasticIpsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes Elastic IP
* addresses.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeElasticIpsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeElasticIpsRequest#builder()}
*
*
* @param describeElasticIpsRequest
* A {@link Consumer} that will call methods on {@link DescribeElasticIpsRequest.Builder} to create a
* request.
* @return Result of the DescribeElasticIps operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticIps
* @see AWS
* API Documentation
*/
default DescribeElasticIpsResponse describeElasticIps(Consumer describeElasticIpsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeElasticIps(DescribeElasticIpsRequest.builder().applyMutation(describeElasticIpsRequest).build());
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticLoadBalancersRequest
* @return Result of the DescribeElasticLoadBalancers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticLoadBalancers
* @see AWS API Documentation
*/
default DescribeElasticLoadBalancersResponse describeElasticLoadBalancers(
DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeElasticLoadBalancersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeElasticLoadBalancersRequest#builder()}
*
*
* @param describeElasticLoadBalancersRequest
* A {@link Consumer} that will call methods on {@link DescribeElasticLoadBalancersRequest.Builder} to create
* a request.
* @return Result of the DescribeElasticLoadBalancers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeElasticLoadBalancers
* @see AWS API Documentation
*/
default DescribeElasticLoadBalancersResponse describeElasticLoadBalancers(
Consumer describeElasticLoadBalancersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeElasticLoadBalancers(DescribeElasticLoadBalancersRequest.builder()
.applyMutation(describeElasticLoadBalancersRequest).build());
}
/**
*
* Requests a description of a set of instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeInstancesRequest
* @return Result of the DescribeInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeInstances
* @see AWS
* API Documentation
*/
default DescribeInstancesResponse describeInstances(DescribeInstancesRequest describeInstancesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of a set of instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeInstancesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeInstancesRequest#builder()}
*
*
* @param describeInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeInstancesRequest.Builder} to create a request.
* @return Result of the DescribeInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeInstances
* @see AWS
* API Documentation
*/
default DescribeInstancesResponse describeInstances(Consumer describeInstancesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeInstances(DescribeInstancesRequest.builder().applyMutation(describeInstancesRequest).build());
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLayersRequest
* @return Result of the DescribeLayers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLayers
* @see AWS API
* Documentation
*/
default DescribeLayersResponse describeLayers(DescribeLayersRequest describeLayersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLayersRequest.Builder} avoiding the need to
* create one manually via {@link DescribeLayersRequest#builder()}
*
*
* @param describeLayersRequest
* A {@link Consumer} that will call methods on {@link DescribeLayersRequest.Builder} to create a request.
* @return Result of the DescribeLayers operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLayers
* @see AWS API
* Documentation
*/
default DescribeLayersResponse describeLayers(Consumer describeLayersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeLayers(DescribeLayersRequest.builder().applyMutation(describeLayersRequest).build());
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLoadBasedAutoScalingRequest
* @return Result of the DescribeLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLoadBasedAutoScaling
* @see AWS API Documentation
*/
default DescribeLoadBasedAutoScalingResponse describeLoadBasedAutoScaling(
DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeLoadBasedAutoScalingRequest.Builder}
* avoiding the need to create one manually via {@link DescribeLoadBasedAutoScalingRequest#builder()}
*
*
* @param describeLoadBasedAutoScalingRequest
* A {@link Consumer} that will call methods on {@link DescribeLoadBasedAutoScalingRequest.Builder} to create
* a request.
* @return Result of the DescribeLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeLoadBasedAutoScaling
* @see AWS API Documentation
*/
default DescribeLoadBasedAutoScalingResponse describeLoadBasedAutoScaling(
Consumer describeLoadBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeLoadBasedAutoScaling(DescribeLoadBasedAutoScalingRequest.builder()
.applyMutation(describeLoadBasedAutoScalingRequest).build());
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @return Result of the DescribeMyUserProfile operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeMyUserProfile
* @see #describeMyUserProfile(DescribeMyUserProfileRequest)
* @see AWS API Documentation
*/
default DescribeMyUserProfileResponse describeMyUserProfile() throws AwsServiceException, SdkClientException,
OpsWorksException {
return describeMyUserProfile(DescribeMyUserProfileRequest.builder().build());
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param describeMyUserProfileRequest
* @return Result of the DescribeMyUserProfile operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeMyUserProfile
* @see AWS API Documentation
*/
default DescribeMyUserProfileResponse describeMyUserProfile(DescribeMyUserProfileRequest describeMyUserProfileRequest)
throws AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeMyUserProfileRequest.Builder} avoiding the
* need to create one manually via {@link DescribeMyUserProfileRequest#builder()}
*
*
* @param describeMyUserProfileRequest
* A {@link Consumer} that will call methods on {@link DescribeMyUserProfileRequest.Builder} to create a
* request.
* @return Result of the DescribeMyUserProfile operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeMyUserProfile
* @see AWS API Documentation
*/
default DescribeMyUserProfileResponse describeMyUserProfile(
Consumer describeMyUserProfileRequest) throws AwsServiceException,
SdkClientException, OpsWorksException {
return describeMyUserProfile(DescribeMyUserProfileRequest.builder().applyMutation(describeMyUserProfileRequest).build());
}
/**
*
* Describes the operating systems that are supported by AWS OpsWorks Stacks.
*
*
* @return Result of the DescribeOperatingSystems operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeOperatingSystems
* @see #describeOperatingSystems(DescribeOperatingSystemsRequest)
* @see AWS API Documentation
*/
default DescribeOperatingSystemsResponse describeOperatingSystems() throws AwsServiceException, SdkClientException,
OpsWorksException {
return describeOperatingSystems(DescribeOperatingSystemsRequest.builder().build());
}
/**
*
* Describes the operating systems that are supported by AWS OpsWorks Stacks.
*
*
* @param describeOperatingSystemsRequest
* @return Result of the DescribeOperatingSystems operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeOperatingSystems
* @see AWS API Documentation
*/
default DescribeOperatingSystemsResponse describeOperatingSystems(
DescribeOperatingSystemsRequest describeOperatingSystemsRequest) throws AwsServiceException, SdkClientException,
OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the operating systems that are supported by AWS OpsWorks Stacks.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeOperatingSystemsRequest.Builder} avoiding
* the need to create one manually via {@link DescribeOperatingSystemsRequest#builder()}
*
*
* @param describeOperatingSystemsRequest
* A {@link Consumer} that will call methods on {@link DescribeOperatingSystemsRequest.Builder} to create a
* request.
* @return Result of the DescribeOperatingSystems operation returned by the service.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeOperatingSystems
* @see AWS API Documentation
*/
default DescribeOperatingSystemsResponse describeOperatingSystems(
Consumer describeOperatingSystemsRequest) throws AwsServiceException,
SdkClientException, OpsWorksException {
return describeOperatingSystems(DescribeOperatingSystemsRequest.builder().applyMutation(describeOperatingSystemsRequest)
.build());
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param describePermissionsRequest
* @return Result of the DescribePermissions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribePermissions
* @see AWS
* API Documentation
*/
default DescribePermissionsResponse describePermissions(DescribePermissionsRequest describePermissionsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribePermissionsRequest.Builder} avoiding the
* need to create one manually via {@link DescribePermissionsRequest#builder()}
*
*
* @param describePermissionsRequest
* A {@link Consumer} that will call methods on {@link DescribePermissionsRequest.Builder} to create a
* request.
* @return Result of the DescribePermissions operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribePermissions
* @see AWS
* API Documentation
*/
default DescribePermissionsResponse describePermissions(
Consumer describePermissionsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describePermissions(DescribePermissionsRequest.builder().applyMutation(describePermissionsRequest).build());
}
/**
*
* Describe an instance's RAID arrays.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeRaidArraysRequest
* @return Result of the DescribeRaidArrays operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRaidArrays
* @see AWS
* API Documentation
*/
default DescribeRaidArraysResponse describeRaidArrays(DescribeRaidArraysRequest describeRaidArraysRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describe an instance's RAID arrays.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRaidArraysRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRaidArraysRequest#builder()}
*
*
* @param describeRaidArraysRequest
* A {@link Consumer} that will call methods on {@link DescribeRaidArraysRequest.Builder} to create a
* request.
* @return Result of the DescribeRaidArrays operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRaidArrays
* @see AWS
* API Documentation
*/
default DescribeRaidArraysResponse describeRaidArrays(Consumer describeRaidArraysRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeRaidArrays(DescribeRaidArraysRequest.builder().applyMutation(describeRaidArraysRequest).build());
}
/**
*
* Describes Amazon RDS instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeRdsDbInstancesRequest
* @return Result of the DescribeRdsDbInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRdsDbInstances
* @see AWS API Documentation
*/
default DescribeRdsDbInstancesResponse describeRdsDbInstances(DescribeRdsDbInstancesRequest describeRdsDbInstancesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes Amazon RDS instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeRdsDbInstancesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeRdsDbInstancesRequest#builder()}
*
*
* @param describeRdsDbInstancesRequest
* A {@link Consumer} that will call methods on {@link DescribeRdsDbInstancesRequest.Builder} to create a
* request.
* @return Result of the DescribeRdsDbInstances operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeRdsDbInstances
* @see AWS API Documentation
*/
default DescribeRdsDbInstancesResponse describeRdsDbInstances(
Consumer describeRdsDbInstancesRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeRdsDbInstances(DescribeRdsDbInstancesRequest.builder().applyMutation(describeRdsDbInstancesRequest)
.build());
}
/**
*
* Describes AWS OpsWorks Stacks service errors.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @return Result of the DescribeServiceErrors operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeServiceErrors
* @see #describeServiceErrors(DescribeServiceErrorsRequest)
* @see AWS API Documentation
*/
default DescribeServiceErrorsResponse describeServiceErrors() throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
return describeServiceErrors(DescribeServiceErrorsRequest.builder().build());
}
/**
*
* Describes AWS OpsWorks Stacks service errors.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeServiceErrorsRequest
* @return Result of the DescribeServiceErrors operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeServiceErrors
* @see AWS API Documentation
*/
default DescribeServiceErrorsResponse describeServiceErrors(DescribeServiceErrorsRequest describeServiceErrorsRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes AWS OpsWorks Stacks service errors.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeServiceErrorsRequest.Builder} avoiding the
* need to create one manually via {@link DescribeServiceErrorsRequest#builder()}
*
*
* @param describeServiceErrorsRequest
* A {@link Consumer} that will call methods on {@link DescribeServiceErrorsRequest.Builder} to create a
* request.
* @return Result of the DescribeServiceErrors operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeServiceErrors
* @see AWS API Documentation
*/
default DescribeServiceErrorsResponse describeServiceErrors(
Consumer describeServiceErrorsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeServiceErrors(DescribeServiceErrorsRequest.builder().applyMutation(describeServiceErrorsRequest).build());
}
/**
*
* Requests a description of a stack's provisioning parameters.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStackProvisioningParametersRequest
* @return Result of the DescribeStackProvisioningParameters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackProvisioningParameters
* @see AWS API Documentation
*/
default DescribeStackProvisioningParametersResponse describeStackProvisioningParameters(
DescribeStackProvisioningParametersRequest describeStackProvisioningParametersRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of a stack's provisioning parameters.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackProvisioningParametersRequest.Builder}
* avoiding the need to create one manually via {@link DescribeStackProvisioningParametersRequest#builder()}
*
*
* @param describeStackProvisioningParametersRequest
* A {@link Consumer} that will call methods on {@link DescribeStackProvisioningParametersRequest.Builder} to
* create a request.
* @return Result of the DescribeStackProvisioningParameters operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackProvisioningParameters
* @see AWS API Documentation
*/
default DescribeStackProvisioningParametersResponse describeStackProvisioningParameters(
Consumer describeStackProvisioningParametersRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeStackProvisioningParameters(DescribeStackProvisioningParametersRequest.builder()
.applyMutation(describeStackProvisioningParametersRequest).build());
}
/**
*
* Describes the number of layers and apps in a specified stack, and the number of instances in each state, such as
* running_setup
or online
.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStackSummaryRequest
* @return Result of the DescribeStackSummary operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackSummary
* @see AWS
* API Documentation
*/
default DescribeStackSummaryResponse describeStackSummary(DescribeStackSummaryRequest describeStackSummaryRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes the number of layers and apps in a specified stack, and the number of instances in each state, such as
* running_setup
or online
.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStackSummaryRequest.Builder} avoiding the
* need to create one manually via {@link DescribeStackSummaryRequest#builder()}
*
*
* @param describeStackSummaryRequest
* A {@link Consumer} that will call methods on {@link DescribeStackSummaryRequest.Builder} to create a
* request.
* @return Result of the DescribeStackSummary operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStackSummary
* @see AWS
* API Documentation
*/
default DescribeStackSummaryResponse describeStackSummary(
Consumer describeStackSummaryRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeStackSummary(DescribeStackSummaryRequest.builder().applyMutation(describeStackSummaryRequest).build());
}
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @return Result of the DescribeStacks operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStacks
* @see #describeStacks(DescribeStacksRequest)
* @see AWS API
* Documentation
*/
default DescribeStacksResponse describeStacks() throws ValidationException, ResourceNotFoundException, AwsServiceException,
SdkClientException, OpsWorksException {
return describeStacks(DescribeStacksRequest.builder().build());
}
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeStacksRequest
* @return Result of the DescribeStacks operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStacks
* @see AWS API
* Documentation
*/
default DescribeStacksResponse describeStacks(DescribeStacksRequest describeStacksRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Requests a description of one or more stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeStacksRequest.Builder} avoiding the need to
* create one manually via {@link DescribeStacksRequest#builder()}
*
*
* @param describeStacksRequest
* A {@link Consumer} that will call methods on {@link DescribeStacksRequest.Builder} to create a request.
* @return Result of the DescribeStacks operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeStacks
* @see AWS API
* Documentation
*/
default DescribeStacksResponse describeStacks(Consumer describeStacksRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeStacks(DescribeStacksRequest.builder().applyMutation(describeStacksRequest).build());
}
/**
*
* Describes time-based auto scaling configurations for specified instances.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeTimeBasedAutoScalingRequest
* @return Result of the DescribeTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeTimeBasedAutoScaling
* @see AWS API Documentation
*/
default DescribeTimeBasedAutoScalingResponse describeTimeBasedAutoScaling(
DescribeTimeBasedAutoScalingRequest describeTimeBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes time-based auto scaling configurations for specified instances.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeTimeBasedAutoScalingRequest.Builder}
* avoiding the need to create one manually via {@link DescribeTimeBasedAutoScalingRequest#builder()}
*
*
* @param describeTimeBasedAutoScalingRequest
* A {@link Consumer} that will call methods on {@link DescribeTimeBasedAutoScalingRequest.Builder} to create
* a request.
* @return Result of the DescribeTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeTimeBasedAutoScaling
* @see AWS API Documentation
*/
default DescribeTimeBasedAutoScalingResponse describeTimeBasedAutoScaling(
Consumer describeTimeBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeTimeBasedAutoScaling(DescribeTimeBasedAutoScalingRequest.builder()
.applyMutation(describeTimeBasedAutoScalingRequest).build());
}
/**
*
* Describe specified users.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @return Result of the DescribeUserProfiles operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeUserProfiles
* @see #describeUserProfiles(DescribeUserProfilesRequest)
* @see AWS
* API Documentation
*/
default DescribeUserProfilesResponse describeUserProfiles() throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
return describeUserProfiles(DescribeUserProfilesRequest.builder().build());
}
/**
*
* Describe specified users.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param describeUserProfilesRequest
* @return Result of the DescribeUserProfiles operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeUserProfiles
* @see AWS
* API Documentation
*/
default DescribeUserProfilesResponse describeUserProfiles(DescribeUserProfilesRequest describeUserProfilesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describe specified users.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeUserProfilesRequest.Builder} avoiding the
* need to create one manually via {@link DescribeUserProfilesRequest#builder()}
*
*
* @param describeUserProfilesRequest
* A {@link Consumer} that will call methods on {@link DescribeUserProfilesRequest.Builder} to create a
* request.
* @return Result of the DescribeUserProfiles operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeUserProfiles
* @see AWS
* API Documentation
*/
default DescribeUserProfilesResponse describeUserProfiles(
Consumer describeUserProfilesRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeUserProfiles(DescribeUserProfilesRequest.builder().applyMutation(describeUserProfilesRequest).build());
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeVolumesRequest
* @return Result of the DescribeVolumes operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeVolumes
* @see AWS API
* Documentation
*/
default DescribeVolumesResponse describeVolumes(DescribeVolumesRequest describeVolumesRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Describes an instance's Amazon EBS volumes.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DescribeVolumesRequest.Builder} avoiding the need
* to create one manually via {@link DescribeVolumesRequest#builder()}
*
*
* @param describeVolumesRequest
* A {@link Consumer} that will call methods on {@link DescribeVolumesRequest.Builder} to create a request.
* @return Result of the DescribeVolumes operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DescribeVolumes
* @see AWS API
* Documentation
*/
default DescribeVolumesResponse describeVolumes(Consumer describeVolumesRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return describeVolumes(DescribeVolumesRequest.builder().applyMutation(describeVolumesRequest).build());
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param detachElasticLoadBalancerRequest
* @return Result of the DetachElasticLoadBalancer operation returned by the service.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DetachElasticLoadBalancer
* @see AWS API Documentation
*/
default DetachElasticLoadBalancerResponse detachElasticLoadBalancer(
DetachElasticLoadBalancerRequest detachElasticLoadBalancerRequest) throws ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Detaches a specified Elastic Load Balancing instance from its layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DetachElasticLoadBalancerRequest.Builder} avoiding
* the need to create one manually via {@link DetachElasticLoadBalancerRequest#builder()}
*
*
* @param detachElasticLoadBalancerRequest
* A {@link Consumer} that will call methods on {@link DetachElasticLoadBalancerRequest.Builder} to create a
* request.
* @return Result of the DetachElasticLoadBalancer operation returned by the service.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DetachElasticLoadBalancer
* @see AWS API Documentation
*/
default DetachElasticLoadBalancerResponse detachElasticLoadBalancer(
Consumer detachElasticLoadBalancerRequest)
throws ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return detachElasticLoadBalancer(DetachElasticLoadBalancerRequest.builder()
.applyMutation(detachElasticLoadBalancerRequest).build());
}
/**
*
* Disassociates an Elastic IP address from its instance. The address remains registered with the stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param disassociateElasticIpRequest
* @return Result of the DisassociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DisassociateElasticIp
* @see AWS API Documentation
*/
default DisassociateElasticIpResponse disassociateElasticIp(DisassociateElasticIpRequest disassociateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Disassociates an Elastic IP address from its instance. The address remains registered with the stack. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link DisassociateElasticIpRequest.Builder} avoiding the
* need to create one manually via {@link DisassociateElasticIpRequest#builder()}
*
*
* @param disassociateElasticIpRequest
* A {@link Consumer} that will call methods on {@link DisassociateElasticIpRequest.Builder} to create a
* request.
* @return Result of the DisassociateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.DisassociateElasticIp
* @see AWS API Documentation
*/
default DisassociateElasticIpResponse disassociateElasticIp(
Consumer disassociateElasticIpRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return disassociateElasticIp(DisassociateElasticIpRequest.builder().applyMutation(disassociateElasticIpRequest).build());
}
/**
*
* Gets a generated host name for the specified layer, based on the current host name theme.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param getHostnameSuggestionRequest
* @return Result of the GetHostnameSuggestion operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GetHostnameSuggestion
* @see AWS API Documentation
*/
default GetHostnameSuggestionResponse getHostnameSuggestion(GetHostnameSuggestionRequest getHostnameSuggestionRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Gets a generated host name for the specified layer, based on the current host name theme.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link GetHostnameSuggestionRequest.Builder} avoiding the
* need to create one manually via {@link GetHostnameSuggestionRequest#builder()}
*
*
* @param getHostnameSuggestionRequest
* A {@link Consumer} that will call methods on {@link GetHostnameSuggestionRequest.Builder} to create a
* request.
* @return Result of the GetHostnameSuggestion operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GetHostnameSuggestion
* @see AWS API Documentation
*/
default GetHostnameSuggestionResponse getHostnameSuggestion(
Consumer getHostnameSuggestionRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return getHostnameSuggestion(GetHostnameSuggestionRequest.builder().applyMutation(getHostnameSuggestionRequest).build());
}
/**
*
*
* This action can be used only with Windows stacks.
*
*
*
* Grants RDP access to a Windows instance for a specified time period.
*
*
* @param grantAccessRequest
* @return Result of the GrantAccess operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GrantAccess
* @see AWS API
* Documentation
*/
default GrantAccessResponse grantAccess(GrantAccessRequest grantAccessRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
*
* This action can be used only with Windows stacks.
*
*
*
* Grants RDP access to a Windows instance for a specified time period.
*
*
*
* This is a convenience which creates an instance of the {@link GrantAccessRequest.Builder} avoiding the need to
* create one manually via {@link GrantAccessRequest#builder()}
*
*
* @param grantAccessRequest
* A {@link Consumer} that will call methods on {@link GrantAccessRequest.Builder} to create a request.
* @return Result of the GrantAccess operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.GrantAccess
* @see AWS API
* Documentation
*/
default GrantAccessResponse grantAccess(Consumer grantAccessRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return grantAccess(GrantAccessRequest.builder().applyMutation(grantAccessRequest).build());
}
/**
*
* Returns a list of tags that are applied to the specified stack or layer.
*
*
* @param listTagsRequest
* @return Result of the ListTags operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsResponse listTags(ListTagsRequest listTagsRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Returns a list of tags that are applied to the specified stack or layer.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsRequest.Builder} avoiding the need to
* create one manually via {@link ListTagsRequest#builder()}
*
*
* @param listTagsRequest
* A {@link Consumer} that will call methods on {@link ListTagsRequest.Builder} to create a request.
* @return Result of the ListTags operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.ListTags
* @see AWS API
* Documentation
*/
default ListTagsResponse listTags(Consumer listTagsRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return listTags(ListTagsRequest.builder().applyMutation(listTagsRequest).build());
}
/**
*
* Reboots a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param rebootInstanceRequest
* @return Result of the RebootInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RebootInstance
* @see AWS API
* Documentation
*/
default RebootInstanceResponse rebootInstance(RebootInstanceRequest rebootInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Reboots a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RebootInstanceRequest.Builder} avoiding the need to
* create one manually via {@link RebootInstanceRequest#builder()}
*
*
* @param rebootInstanceRequest
* A {@link Consumer} that will call methods on {@link RebootInstanceRequest.Builder} to create a request.
* @return Result of the RebootInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RebootInstance
* @see AWS API
* Documentation
*/
default RebootInstanceResponse rebootInstance(Consumer rebootInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return rebootInstance(RebootInstanceRequest.builder().applyMutation(rebootInstanceRequest).build());
}
/**
*
* Registers a specified Amazon ECS cluster with a stack. You can register only one cluster with a stack. A cluster
* can be registered with only one stack. For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerEcsClusterRequest
* @return Result of the RegisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterEcsCluster
* @see AWS
* API Documentation
*/
default RegisterEcsClusterResponse registerEcsCluster(RegisterEcsClusterRequest registerEcsClusterRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Registers a specified Amazon ECS cluster with a stack. You can register only one cluster with a stack. A cluster
* can be registered with only one stack. For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterEcsClusterRequest.Builder} avoiding the
* need to create one manually via {@link RegisterEcsClusterRequest#builder()}
*
*
* @param registerEcsClusterRequest
* A {@link Consumer} that will call methods on {@link RegisterEcsClusterRequest.Builder} to create a
* request.
* @return Result of the RegisterEcsCluster operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterEcsCluster
* @see AWS
* API Documentation
*/
default RegisterEcsClusterResponse registerEcsCluster(Consumer registerEcsClusterRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return registerEcsCluster(RegisterEcsClusterRequest.builder().applyMutation(registerEcsClusterRequest).build());
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can be registered with only one stack at a
* time. If the address is already registered, you must first deregister it by calling DeregisterElasticIp.
* For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerElasticIpRequest
* @return Result of the RegisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterElasticIp
* @see AWS
* API Documentation
*/
default RegisterElasticIpResponse registerElasticIp(RegisterElasticIpRequest registerElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Registers an Elastic IP address with a specified stack. An address can be registered with only one stack at a
* time. If the address is already registered, you must first deregister it by calling DeregisterElasticIp.
* For more information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterElasticIpRequest.Builder} avoiding the need
* to create one manually via {@link RegisterElasticIpRequest#builder()}
*
*
* @param registerElasticIpRequest
* A {@link Consumer} that will call methods on {@link RegisterElasticIpRequest.Builder} to create a request.
* @return Result of the RegisterElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterElasticIp
* @see AWS
* API Documentation
*/
default RegisterElasticIpResponse registerElasticIp(Consumer registerElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return registerElasticIp(RegisterElasticIpRequest.builder().applyMutation(registerElasticIpRequest).build());
}
/**
*
* Registers instances that were created outside of AWS OpsWorks Stacks with a specified stack.
*
*
*
* We do not recommend using this action to register instances. The complete registration operation includes two
* tasks: installing the AWS OpsWorks Stacks agent on the instance, and registering the instance with the stack.
* RegisterInstance
handles only the second step. You should instead use the AWS CLI
* register
command, which performs the entire registration operation. For more information, see Registering an
* Instance with an AWS OpsWorks Stacks Stack.
*
*
*
* Registered instances have the same requirements as instances that are created by using the CreateInstance
* API. For example, registered instances must be running a supported Linux-based operating system, and they must
* have a supported instance type. For more information about requirements for instances that you want to register,
* see
* Preparing the Instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerInstanceRequest
* @return Result of the RegisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterInstance
* @see AWS API
* Documentation
*/
default RegisterInstanceResponse registerInstance(RegisterInstanceRequest registerInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Registers instances that were created outside of AWS OpsWorks Stacks with a specified stack.
*
*
*
* We do not recommend using this action to register instances. The complete registration operation includes two
* tasks: installing the AWS OpsWorks Stacks agent on the instance, and registering the instance with the stack.
* RegisterInstance
handles only the second step. You should instead use the AWS CLI
* register
command, which performs the entire registration operation. For more information, see Registering an
* Instance with an AWS OpsWorks Stacks Stack.
*
*
*
* Registered instances have the same requirements as instances that are created by using the CreateInstance
* API. For example, registered instances must be running a supported Linux-based operating system, and they must
* have a supported instance type. For more information about requirements for instances that you want to register,
* see
* Preparing the Instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterInstanceRequest.Builder} avoiding the need
* to create one manually via {@link RegisterInstanceRequest#builder()}
*
*
* @param registerInstanceRequest
* A {@link Consumer} that will call methods on {@link RegisterInstanceRequest.Builder} to create a request.
* @return Result of the RegisterInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterInstance
* @see AWS API
* Documentation
*/
default RegisterInstanceResponse registerInstance(Consumer registerInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return registerInstance(RegisterInstanceRequest.builder().applyMutation(registerInstanceRequest).build());
}
/**
*
* Registers an Amazon RDS instance with a stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerRdsDbInstanceRequest
* @return Result of the RegisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterRdsDbInstance
* @see AWS API Documentation
*/
default RegisterRdsDbInstanceResponse registerRdsDbInstance(RegisterRdsDbInstanceRequest registerRdsDbInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Registers an Amazon RDS instance with a stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterRdsDbInstanceRequest.Builder} avoiding the
* need to create one manually via {@link RegisterRdsDbInstanceRequest#builder()}
*
*
* @param registerRdsDbInstanceRequest
* A {@link Consumer} that will call methods on {@link RegisterRdsDbInstanceRequest.Builder} to create a
* request.
* @return Result of the RegisterRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterRdsDbInstance
* @see AWS API Documentation
*/
default RegisterRdsDbInstanceResponse registerRdsDbInstance(
Consumer registerRdsDbInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return registerRdsDbInstance(RegisterRdsDbInstanceRequest.builder().applyMutation(registerRdsDbInstanceRequest).build());
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be registered with only one stack at a time.
* If the volume is already registered, you must first deregister it by calling DeregisterVolume. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param registerVolumeRequest
* @return Result of the RegisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterVolume
* @see AWS API
* Documentation
*/
default RegisterVolumeResponse registerVolume(RegisterVolumeRequest registerVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Registers an Amazon EBS volume with a specified stack. A volume can be registered with only one stack at a time.
* If the volume is already registered, you must first deregister it by calling DeregisterVolume. For more
* information, see Resource
* Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link RegisterVolumeRequest.Builder} avoiding the need to
* create one manually via {@link RegisterVolumeRequest#builder()}
*
*
* @param registerVolumeRequest
* A {@link Consumer} that will call methods on {@link RegisterVolumeRequest.Builder} to create a request.
* @return Result of the RegisterVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.RegisterVolume
* @see AWS API
* Documentation
*/
default RegisterVolumeResponse registerVolume(Consumer registerVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return registerVolume(RegisterVolumeRequest.builder().applyMutation(registerVolumeRequest).build());
}
/**
*
* Specify the load-based auto scaling configuration for a specified layer. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
*
* To use load-based auto scaling, you must create a set of load-based auto scaling instances. Load-based auto
* scaling operates only on the instances from that set, so you must ensure that you have created enough instances
* to handle the maximum anticipated load.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setLoadBasedAutoScalingRequest
* @return Result of the SetLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetLoadBasedAutoScaling
* @see AWS API Documentation
*/
default SetLoadBasedAutoScalingResponse setLoadBasedAutoScaling(SetLoadBasedAutoScalingRequest setLoadBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Specify the load-based auto scaling configuration for a specified layer. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
*
* To use load-based auto scaling, you must create a set of load-based auto scaling instances. Load-based auto
* scaling operates only on the instances from that set, so you must ensure that you have created enough instances
* to handle the maximum anticipated load.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link SetLoadBasedAutoScalingRequest.Builder} avoiding
* the need to create one manually via {@link SetLoadBasedAutoScalingRequest#builder()}
*
*
* @param setLoadBasedAutoScalingRequest
* A {@link Consumer} that will call methods on {@link SetLoadBasedAutoScalingRequest.Builder} to create a
* request.
* @return Result of the SetLoadBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetLoadBasedAutoScaling
* @see AWS API Documentation
*/
default SetLoadBasedAutoScalingResponse setLoadBasedAutoScaling(
Consumer setLoadBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return setLoadBasedAutoScaling(SetLoadBasedAutoScalingRequest.builder().applyMutation(setLoadBasedAutoScalingRequest)
.build());
}
/**
*
* Specifies a user's permissions. For more information, see Security and Permissions.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setPermissionRequest
* @return Result of the SetPermission operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetPermission
* @see AWS API
* Documentation
*/
default SetPermissionResponse setPermission(SetPermissionRequest setPermissionRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Specifies a user's permissions. For more information, see Security and Permissions.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link SetPermissionRequest.Builder} avoiding the need to
* create one manually via {@link SetPermissionRequest#builder()}
*
*
* @param setPermissionRequest
* A {@link Consumer} that will call methods on {@link SetPermissionRequest.Builder} to create a request.
* @return Result of the SetPermission operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetPermission
* @see AWS API
* Documentation
*/
default SetPermissionResponse setPermission(Consumer setPermissionRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return setPermission(SetPermissionRequest.builder().applyMutation(setPermissionRequest).build());
}
/**
*
* Specify the time-based auto scaling configuration for a specified instance. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param setTimeBasedAutoScalingRequest
* @return Result of the SetTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetTimeBasedAutoScaling
* @see AWS API Documentation
*/
default SetTimeBasedAutoScalingResponse setTimeBasedAutoScaling(SetTimeBasedAutoScalingRequest setTimeBasedAutoScalingRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Specify the time-based auto scaling configuration for a specified instance. For more information, see Managing Load with
* Time-based and Load-based Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link SetTimeBasedAutoScalingRequest.Builder} avoiding
* the need to create one manually via {@link SetTimeBasedAutoScalingRequest#builder()}
*
*
* @param setTimeBasedAutoScalingRequest
* A {@link Consumer} that will call methods on {@link SetTimeBasedAutoScalingRequest.Builder} to create a
* request.
* @return Result of the SetTimeBasedAutoScaling operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.SetTimeBasedAutoScaling
* @see AWS API Documentation
*/
default SetTimeBasedAutoScalingResponse setTimeBasedAutoScaling(
Consumer setTimeBasedAutoScalingRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return setTimeBasedAutoScaling(SetTimeBasedAutoScalingRequest.builder().applyMutation(setTimeBasedAutoScalingRequest)
.build());
}
/**
*
* Starts a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param startInstanceRequest
* @return Result of the StartInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartInstance
* @see AWS API
* Documentation
*/
default StartInstanceResponse startInstance(StartInstanceRequest startInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Starts a specified instance. For more information, see Starting, Stopping,
* and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link StartInstanceRequest.Builder} avoiding the need to
* create one manually via {@link StartInstanceRequest#builder()}
*
*
* @param startInstanceRequest
* A {@link Consumer} that will call methods on {@link StartInstanceRequest.Builder} to create a request.
* @return Result of the StartInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartInstance
* @see AWS API
* Documentation
*/
default StartInstanceResponse startInstance(Consumer startInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return startInstance(StartInstanceRequest.builder().applyMutation(startInstanceRequest).build());
}
/**
*
* Starts a stack's instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param startStackRequest
* @return Result of the StartStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartStack
* @see AWS API
* Documentation
*/
default StartStackResponse startStack(StartStackRequest startStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Starts a stack's instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link StartStackRequest.Builder} avoiding the need to
* create one manually via {@link StartStackRequest#builder()}
*
*
* @param startStackRequest
* A {@link Consumer} that will call methods on {@link StartStackRequest.Builder} to create a request.
* @return Result of the StartStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StartStack
* @see AWS API
* Documentation
*/
default StartStackResponse startStack(Consumer startStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return startStack(StartStackRequest.builder().applyMutation(startStackRequest).build());
}
/**
*
* Stops a specified instance. When you stop a standard instance, the data disappears and must be reinstalled when
* you restart the instance. You can stop an Amazon EBS-backed instance without losing data. For more information,
* see Starting,
* Stopping, and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param stopInstanceRequest
* @return Result of the StopInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopInstance
* @see AWS API
* Documentation
*/
default StopInstanceResponse stopInstance(StopInstanceRequest stopInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Stops a specified instance. When you stop a standard instance, the data disappears and must be reinstalled when
* you restart the instance. You can stop an Amazon EBS-backed instance without losing data. For more information,
* see Starting,
* Stopping, and Rebooting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link StopInstanceRequest.Builder} avoiding the need to
* create one manually via {@link StopInstanceRequest#builder()}
*
*
* @param stopInstanceRequest
* A {@link Consumer} that will call methods on {@link StopInstanceRequest.Builder} to create a request.
* @return Result of the StopInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopInstance
* @see AWS API
* Documentation
*/
default StopInstanceResponse stopInstance(Consumer stopInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return stopInstance(StopInstanceRequest.builder().applyMutation(stopInstanceRequest).build());
}
/**
*
* Stops a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param stopStackRequest
* @return Result of the StopStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopStack
* @see AWS API
* Documentation
*/
default StopStackResponse stopStack(StopStackRequest stopStackRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Stops a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link StopStackRequest.Builder} avoiding the need to
* create one manually via {@link StopStackRequest#builder()}
*
*
* @param stopStackRequest
* A {@link Consumer} that will call methods on {@link StopStackRequest.Builder} to create a request.
* @return Result of the StopStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.StopStack
* @see AWS API
* Documentation
*/
default StopStackResponse stopStack(Consumer stopStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return stopStack(StopStackRequest.builder().applyMutation(stopStackRequest).build());
}
/**
*
* Apply cost-allocation tags to a specified stack or layer in AWS OpsWorks Stacks. For more information about how
* tagging works, see Tags in the
* AWS OpsWorks User Guide.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Apply cost-allocation tags to a specified stack or layer in AWS OpsWorks Stacks. For more information about how
* tagging works, see Tags in the
* AWS OpsWorks User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Unassigns a registered instance from all layers that are using the instance. The instance remains in the stack as
* an unassigned instance, and can be assigned to another layer as needed. You cannot use this action with instances
* that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param unassignInstanceRequest
* @return Result of the UnassignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UnassignInstance
* @see AWS API
* Documentation
*/
default UnassignInstanceResponse unassignInstance(UnassignInstanceRequest unassignInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Unassigns a registered instance from all layers that are using the instance. The instance remains in the stack as
* an unassigned instance, and can be assigned to another layer as needed. You cannot use this action with instances
* that were created with AWS OpsWorks Stacks.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UnassignInstanceRequest.Builder} avoiding the need
* to create one manually via {@link UnassignInstanceRequest#builder()}
*
*
* @param unassignInstanceRequest
* A {@link Consumer} that will call methods on {@link UnassignInstanceRequest.Builder} to create a request.
* @return Result of the UnassignInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UnassignInstance
* @see AWS API
* Documentation
*/
default UnassignInstanceResponse unassignInstance(Consumer unassignInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return unassignInstance(UnassignInstanceRequest.builder().applyMutation(unassignInstanceRequest).build());
}
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered with the stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param unassignVolumeRequest
* @return Result of the UnassignVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UnassignVolume
* @see AWS API
* Documentation
*/
default UnassignVolumeResponse unassignVolume(UnassignVolumeRequest unassignVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Unassigns an assigned Amazon EBS volume. The volume remains registered with the stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UnassignVolumeRequest.Builder} avoiding the need to
* create one manually via {@link UnassignVolumeRequest#builder()}
*
*
* @param unassignVolumeRequest
* A {@link Consumer} that will call methods on {@link UnassignVolumeRequest.Builder} to create a request.
* @return Result of the UnassignVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UnassignVolume
* @see AWS API
* Documentation
*/
default UnassignVolumeResponse unassignVolume(Consumer unassignVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return unassignVolume(UnassignVolumeRequest.builder().applyMutation(unassignVolumeRequest).build());
}
/**
*
* Removes tags from a specified stack or layer.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a specified stack or layer.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
* @param updateAppRequest
* @return Result of the UpdateApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateApp
* @see AWS API
* Documentation
*/
default UpdateAppResponse updateApp(UpdateAppRequest updateAppRequest) throws ValidationException, ResourceNotFoundException,
AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateAppRequest.Builder} avoiding the need to
* create one manually via {@link UpdateAppRequest#builder()}
*
*
* @param updateAppRequest
* A {@link Consumer} that will call methods on {@link UpdateAppRequest.Builder} to create a request.
* @return Result of the UpdateApp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateApp
* @see AWS API
* Documentation
*/
default UpdateAppResponse updateApp(Consumer updateAppRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateApp(UpdateAppRequest.builder().applyMutation(updateAppRequest).build());
}
/**
*
* Updates a registered Elastic IP address's name. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateElasticIpRequest
* @return Result of the UpdateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateElasticIp
* @see AWS API
* Documentation
*/
default UpdateElasticIpResponse updateElasticIp(UpdateElasticIpRequest updateElasticIpRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a registered Elastic IP address's name. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateElasticIpRequest.Builder} avoiding the need
* to create one manually via {@link UpdateElasticIpRequest#builder()}
*
*
* @param updateElasticIpRequest
* A {@link Consumer} that will call methods on {@link UpdateElasticIpRequest.Builder} to create a request.
* @return Result of the UpdateElasticIp operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateElasticIp
* @see AWS API
* Documentation
*/
default UpdateElasticIpResponse updateElasticIp(Consumer updateElasticIpRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateElasticIp(UpdateElasticIpRequest.builder().applyMutation(updateElasticIpRequest).build());
}
/**
*
* Updates a specified instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateInstanceRequest
* @return Result of the UpdateInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateInstance
* @see AWS API
* Documentation
*/
default UpdateInstanceResponse updateInstance(UpdateInstanceRequest updateInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateInstanceRequest.Builder} avoiding the need to
* create one manually via {@link UpdateInstanceRequest#builder()}
*
*
* @param updateInstanceRequest
* A {@link Consumer} that will call methods on {@link UpdateInstanceRequest.Builder} to create a request.
* @return Result of the UpdateInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateInstance
* @see AWS API
* Documentation
*/
default UpdateInstanceResponse updateInstance(Consumer updateInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateInstance(UpdateInstanceRequest.builder().applyMutation(updateInstanceRequest).build());
}
/**
*
* Updates a specified layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateLayerRequest
* @return Result of the UpdateLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateLayer
* @see AWS API
* Documentation
*/
default UpdateLayerResponse updateLayer(UpdateLayerRequest updateLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLayerRequest.Builder} avoiding the need to
* create one manually via {@link UpdateLayerRequest#builder()}
*
*
* @param updateLayerRequest
* A {@link Consumer} that will call methods on {@link UpdateLayerRequest.Builder} to create a request.
* @return Result of the UpdateLayer operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateLayer
* @see AWS API
* Documentation
*/
default UpdateLayerResponse updateLayer(Consumer updateLayerRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateLayer(UpdateLayerRequest.builder().applyMutation(updateLayerRequest).build());
}
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param updateMyUserProfileRequest
* @return Result of the UpdateMyUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateMyUserProfile
* @see AWS
* API Documentation
*/
default UpdateMyUserProfileResponse updateMyUserProfile(UpdateMyUserProfileRequest updateMyUserProfileRequest)
throws ValidationException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a user's SSH public key.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateMyUserProfileRequest.Builder} avoiding the
* need to create one manually via {@link UpdateMyUserProfileRequest#builder()}
*
*
* @param updateMyUserProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateMyUserProfileRequest.Builder} to create a
* request.
* @return Result of the UpdateMyUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateMyUserProfile
* @see AWS
* API Documentation
*/
default UpdateMyUserProfileResponse updateMyUserProfile(
Consumer updateMyUserProfileRequest) throws ValidationException,
AwsServiceException, SdkClientException, OpsWorksException {
return updateMyUserProfile(UpdateMyUserProfileRequest.builder().applyMutation(updateMyUserProfileRequest).build());
}
/**
*
* Updates an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateRdsDbInstanceRequest
* @return Result of the UpdateRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateRdsDbInstance
* @see AWS
* API Documentation
*/
default UpdateRdsDbInstanceResponse updateRdsDbInstance(UpdateRdsDbInstanceRequest updateRdsDbInstanceRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRdsDbInstanceRequest.Builder} avoiding the
* need to create one manually via {@link UpdateRdsDbInstanceRequest#builder()}
*
*
* @param updateRdsDbInstanceRequest
* A {@link Consumer} that will call methods on {@link UpdateRdsDbInstanceRequest.Builder} to create a
* request.
* @return Result of the UpdateRdsDbInstance operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateRdsDbInstance
* @see AWS
* API Documentation
*/
default UpdateRdsDbInstanceResponse updateRdsDbInstance(
Consumer updateRdsDbInstanceRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateRdsDbInstance(UpdateRdsDbInstanceRequest.builder().applyMutation(updateRdsDbInstanceRequest).build());
}
/**
*
* Updates a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateStackRequest
* @return Result of the UpdateStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateStack
* @see AWS API
* Documentation
*/
default UpdateStackResponse updateStack(UpdateStackRequest updateStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateStackRequest.Builder} avoiding the need to
* create one manually via {@link UpdateStackRequest#builder()}
*
*
* @param updateStackRequest
* A {@link Consumer} that will call methods on {@link UpdateStackRequest.Builder} to create a request.
* @return Result of the UpdateStack operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateStack
* @see AWS API
* Documentation
*/
default UpdateStackResponse updateStack(Consumer updateStackRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateStack(UpdateStackRequest.builder().applyMutation(updateStackRequest).build());
}
/**
*
* Updates a specified user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param updateUserProfileRequest
* @return Result of the UpdateUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateUserProfile
* @see AWS
* API Documentation
*/
default UpdateUserProfileResponse updateUserProfile(UpdateUserProfileRequest updateUserProfileRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateUserProfileRequest.Builder} avoiding the need
* to create one manually via {@link UpdateUserProfileRequest#builder()}
*
*
* @param updateUserProfileRequest
* A {@link Consumer} that will call methods on {@link UpdateUserProfileRequest.Builder} to create a request.
* @return Result of the UpdateUserProfile operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateUserProfile
* @see AWS
* API Documentation
*/
default UpdateUserProfileResponse updateUserProfile(Consumer updateUserProfileRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateUserProfile(UpdateUserProfileRequest.builder().applyMutation(updateUserProfileRequest).build());
}
/**
*
* Updates an Amazon EBS volume's name or mount point. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param updateVolumeRequest
* @return Result of the UpdateVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateVolume
* @see AWS API
* Documentation
*/
default UpdateVolumeResponse updateVolume(UpdateVolumeRequest updateVolumeRequest) throws ValidationException,
ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Amazon EBS volume's name or mount point. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateVolumeRequest.Builder} avoiding the need to
* create one manually via {@link UpdateVolumeRequest#builder()}
*
*
* @param updateVolumeRequest
* A {@link Consumer} that will call methods on {@link UpdateVolumeRequest.Builder} to create a request.
* @return Result of the UpdateVolume operation returned by the service.
* @throws ValidationException
* Indicates that a request was not valid.
* @throws ResourceNotFoundException
* Indicates that a resource was not found.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OpsWorksException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OpsWorksClient.UpdateVolume
* @see AWS API
* Documentation
*/
default UpdateVolumeResponse updateVolume(Consumer updateVolumeRequest)
throws ValidationException, ResourceNotFoundException, AwsServiceException, SdkClientException, OpsWorksException {
return updateVolume(UpdateVolumeRequest.builder().applyMutation(updateVolumeRequest).build());
}
/**
* Create an instance of {@link OpsWorksWaiter} using this client.
*
* Waiters created via this method are managed by the SDK and resources will be released when the service client is
* closed.
*
* @return an instance of {@link OpsWorksWaiter}
*/
default OpsWorksWaiter waiter() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link OpsWorksClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static OpsWorksClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link OpsWorksClient}.
*/
static OpsWorksClientBuilder builder() {
return new DefaultOpsWorksClientBuilder();
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
@Override
default OpsWorksServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
}