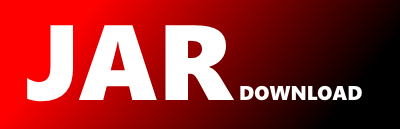
software.amazon.awssdk.services.opsworks.model.InstancesCount Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes how many instances a stack has for each status.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InstancesCount implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ASSIGNING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Assigning").getter(getter(InstancesCount::assigning)).setter(setter(Builder::assigning))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Assigning").build()).build();
private static final SdkField BOOTING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Booting").getter(getter(InstancesCount::booting)).setter(setter(Builder::booting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Booting").build()).build();
private static final SdkField CONNECTION_LOST_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ConnectionLost").getter(getter(InstancesCount::connectionLost)).setter(setter(Builder::connectionLost))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ConnectionLost").build()).build();
private static final SdkField DEREGISTERING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Deregistering").getter(getter(InstancesCount::deregistering)).setter(setter(Builder::deregistering))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Deregistering").build()).build();
private static final SdkField ONLINE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Online").getter(getter(InstancesCount::online)).setter(setter(Builder::online))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Online").build()).build();
private static final SdkField PENDING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Pending").getter(getter(InstancesCount::pending)).setter(setter(Builder::pending))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Pending").build()).build();
private static final SdkField REBOOTING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Rebooting").getter(getter(InstancesCount::rebooting)).setter(setter(Builder::rebooting))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Rebooting").build()).build();
private static final SdkField REGISTERED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Registered").getter(getter(InstancesCount::registered)).setter(setter(Builder::registered))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Registered").build()).build();
private static final SdkField REGISTERING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Registering").getter(getter(InstancesCount::registering)).setter(setter(Builder::registering))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Registering").build()).build();
private static final SdkField REQUESTED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Requested").getter(getter(InstancesCount::requested)).setter(setter(Builder::requested))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Requested").build()).build();
private static final SdkField RUNNING_SETUP_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RunningSetup").getter(getter(InstancesCount::runningSetup)).setter(setter(Builder::runningSetup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RunningSetup").build()).build();
private static final SdkField SETUP_FAILED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("SetupFailed").getter(getter(InstancesCount::setupFailed)).setter(setter(Builder::setupFailed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SetupFailed").build()).build();
private static final SdkField SHUTTING_DOWN_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ShuttingDown").getter(getter(InstancesCount::shuttingDown)).setter(setter(Builder::shuttingDown))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ShuttingDown").build()).build();
private static final SdkField START_FAILED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StartFailed").getter(getter(InstancesCount::startFailed)).setter(setter(Builder::startFailed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartFailed").build()).build();
private static final SdkField STOP_FAILED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("StopFailed").getter(getter(InstancesCount::stopFailed)).setter(setter(Builder::stopFailed))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StopFailed").build()).build();
private static final SdkField STOPPED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Stopped").getter(getter(InstancesCount::stopped)).setter(setter(Builder::stopped))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Stopped").build()).build();
private static final SdkField STOPPING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Stopping").getter(getter(InstancesCount::stopping)).setter(setter(Builder::stopping))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Stopping").build()).build();
private static final SdkField TERMINATED_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Terminated").getter(getter(InstancesCount::terminated)).setter(setter(Builder::terminated))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Terminated").build()).build();
private static final SdkField TERMINATING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Terminating").getter(getter(InstancesCount::terminating)).setter(setter(Builder::terminating))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Terminating").build()).build();
private static final SdkField UNASSIGNING_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("Unassigning").getter(getter(InstancesCount::unassigning)).setter(setter(Builder::unassigning))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Unassigning").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ASSIGNING_FIELD,
BOOTING_FIELD, CONNECTION_LOST_FIELD, DEREGISTERING_FIELD, ONLINE_FIELD, PENDING_FIELD, REBOOTING_FIELD,
REGISTERED_FIELD, REGISTERING_FIELD, REQUESTED_FIELD, RUNNING_SETUP_FIELD, SETUP_FAILED_FIELD, SHUTTING_DOWN_FIELD,
START_FAILED_FIELD, STOP_FAILED_FIELD, STOPPED_FIELD, STOPPING_FIELD, TERMINATED_FIELD, TERMINATING_FIELD,
UNASSIGNING_FIELD));
private static final long serialVersionUID = 1L;
private final Integer assigning;
private final Integer booting;
private final Integer connectionLost;
private final Integer deregistering;
private final Integer online;
private final Integer pending;
private final Integer rebooting;
private final Integer registered;
private final Integer registering;
private final Integer requested;
private final Integer runningSetup;
private final Integer setupFailed;
private final Integer shuttingDown;
private final Integer startFailed;
private final Integer stopFailed;
private final Integer stopped;
private final Integer stopping;
private final Integer terminated;
private final Integer terminating;
private final Integer unassigning;
private InstancesCount(BuilderImpl builder) {
this.assigning = builder.assigning;
this.booting = builder.booting;
this.connectionLost = builder.connectionLost;
this.deregistering = builder.deregistering;
this.online = builder.online;
this.pending = builder.pending;
this.rebooting = builder.rebooting;
this.registered = builder.registered;
this.registering = builder.registering;
this.requested = builder.requested;
this.runningSetup = builder.runningSetup;
this.setupFailed = builder.setupFailed;
this.shuttingDown = builder.shuttingDown;
this.startFailed = builder.startFailed;
this.stopFailed = builder.stopFailed;
this.stopped = builder.stopped;
this.stopping = builder.stopping;
this.terminated = builder.terminated;
this.terminating = builder.terminating;
this.unassigning = builder.unassigning;
}
/**
*
* The number of instances in the Assigning state.
*
*
* @return The number of instances in the Assigning state.
*/
public final Integer assigning() {
return assigning;
}
/**
*
* The number of instances with booting
status.
*
*
* @return The number of instances with booting
status.
*/
public final Integer booting() {
return booting;
}
/**
*
* The number of instances with connection_lost
status.
*
*
* @return The number of instances with connection_lost
status.
*/
public final Integer connectionLost() {
return connectionLost;
}
/**
*
* The number of instances in the Deregistering state.
*
*
* @return The number of instances in the Deregistering state.
*/
public final Integer deregistering() {
return deregistering;
}
/**
*
* The number of instances with online
status.
*
*
* @return The number of instances with online
status.
*/
public final Integer online() {
return online;
}
/**
*
* The number of instances with pending
status.
*
*
* @return The number of instances with pending
status.
*/
public final Integer pending() {
return pending;
}
/**
*
* The number of instances with rebooting
status.
*
*
* @return The number of instances with rebooting
status.
*/
public final Integer rebooting() {
return rebooting;
}
/**
*
* The number of instances in the Registered state.
*
*
* @return The number of instances in the Registered state.
*/
public final Integer registered() {
return registered;
}
/**
*
* The number of instances in the Registering state.
*
*
* @return The number of instances in the Registering state.
*/
public final Integer registering() {
return registering;
}
/**
*
* The number of instances with requested
status.
*
*
* @return The number of instances with requested
status.
*/
public final Integer requested() {
return requested;
}
/**
*
* The number of instances with running_setup
status.
*
*
* @return The number of instances with running_setup
status.
*/
public final Integer runningSetup() {
return runningSetup;
}
/**
*
* The number of instances with setup_failed
status.
*
*
* @return The number of instances with setup_failed
status.
*/
public final Integer setupFailed() {
return setupFailed;
}
/**
*
* The number of instances with shutting_down
status.
*
*
* @return The number of instances with shutting_down
status.
*/
public final Integer shuttingDown() {
return shuttingDown;
}
/**
*
* The number of instances with start_failed
status.
*
*
* @return The number of instances with start_failed
status.
*/
public final Integer startFailed() {
return startFailed;
}
/**
*
* The number of instances with stop_failed
status.
*
*
* @return The number of instances with stop_failed
status.
*/
public final Integer stopFailed() {
return stopFailed;
}
/**
*
* The number of instances with stopped
status.
*
*
* @return The number of instances with stopped
status.
*/
public final Integer stopped() {
return stopped;
}
/**
*
* The number of instances with stopping
status.
*
*
* @return The number of instances with stopping
status.
*/
public final Integer stopping() {
return stopping;
}
/**
*
* The number of instances with terminated
status.
*
*
* @return The number of instances with terminated
status.
*/
public final Integer terminated() {
return terminated;
}
/**
*
* The number of instances with terminating
status.
*
*
* @return The number of instances with terminating
status.
*/
public final Integer terminating() {
return terminating;
}
/**
*
* The number of instances in the Unassigning state.
*
*
* @return The number of instances in the Unassigning state.
*/
public final Integer unassigning() {
return unassigning;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(assigning());
hashCode = 31 * hashCode + Objects.hashCode(booting());
hashCode = 31 * hashCode + Objects.hashCode(connectionLost());
hashCode = 31 * hashCode + Objects.hashCode(deregistering());
hashCode = 31 * hashCode + Objects.hashCode(online());
hashCode = 31 * hashCode + Objects.hashCode(pending());
hashCode = 31 * hashCode + Objects.hashCode(rebooting());
hashCode = 31 * hashCode + Objects.hashCode(registered());
hashCode = 31 * hashCode + Objects.hashCode(registering());
hashCode = 31 * hashCode + Objects.hashCode(requested());
hashCode = 31 * hashCode + Objects.hashCode(runningSetup());
hashCode = 31 * hashCode + Objects.hashCode(setupFailed());
hashCode = 31 * hashCode + Objects.hashCode(shuttingDown());
hashCode = 31 * hashCode + Objects.hashCode(startFailed());
hashCode = 31 * hashCode + Objects.hashCode(stopFailed());
hashCode = 31 * hashCode + Objects.hashCode(stopped());
hashCode = 31 * hashCode + Objects.hashCode(stopping());
hashCode = 31 * hashCode + Objects.hashCode(terminated());
hashCode = 31 * hashCode + Objects.hashCode(terminating());
hashCode = 31 * hashCode + Objects.hashCode(unassigning());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InstancesCount)) {
return false;
}
InstancesCount other = (InstancesCount) obj;
return Objects.equals(assigning(), other.assigning()) && Objects.equals(booting(), other.booting())
&& Objects.equals(connectionLost(), other.connectionLost())
&& Objects.equals(deregistering(), other.deregistering()) && Objects.equals(online(), other.online())
&& Objects.equals(pending(), other.pending()) && Objects.equals(rebooting(), other.rebooting())
&& Objects.equals(registered(), other.registered()) && Objects.equals(registering(), other.registering())
&& Objects.equals(requested(), other.requested()) && Objects.equals(runningSetup(), other.runningSetup())
&& Objects.equals(setupFailed(), other.setupFailed()) && Objects.equals(shuttingDown(), other.shuttingDown())
&& Objects.equals(startFailed(), other.startFailed()) && Objects.equals(stopFailed(), other.stopFailed())
&& Objects.equals(stopped(), other.stopped()) && Objects.equals(stopping(), other.stopping())
&& Objects.equals(terminated(), other.terminated()) && Objects.equals(terminating(), other.terminating())
&& Objects.equals(unassigning(), other.unassigning());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InstancesCount").add("Assigning", assigning()).add("Booting", booting())
.add("ConnectionLost", connectionLost()).add("Deregistering", deregistering()).add("Online", online())
.add("Pending", pending()).add("Rebooting", rebooting()).add("Registered", registered())
.add("Registering", registering()).add("Requested", requested()).add("RunningSetup", runningSetup())
.add("SetupFailed", setupFailed()).add("ShuttingDown", shuttingDown()).add("StartFailed", startFailed())
.add("StopFailed", stopFailed()).add("Stopped", stopped()).add("Stopping", stopping())
.add("Terminated", terminated()).add("Terminating", terminating()).add("Unassigning", unassigning()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Assigning":
return Optional.ofNullable(clazz.cast(assigning()));
case "Booting":
return Optional.ofNullable(clazz.cast(booting()));
case "ConnectionLost":
return Optional.ofNullable(clazz.cast(connectionLost()));
case "Deregistering":
return Optional.ofNullable(clazz.cast(deregistering()));
case "Online":
return Optional.ofNullable(clazz.cast(online()));
case "Pending":
return Optional.ofNullable(clazz.cast(pending()));
case "Rebooting":
return Optional.ofNullable(clazz.cast(rebooting()));
case "Registered":
return Optional.ofNullable(clazz.cast(registered()));
case "Registering":
return Optional.ofNullable(clazz.cast(registering()));
case "Requested":
return Optional.ofNullable(clazz.cast(requested()));
case "RunningSetup":
return Optional.ofNullable(clazz.cast(runningSetup()));
case "SetupFailed":
return Optional.ofNullable(clazz.cast(setupFailed()));
case "ShuttingDown":
return Optional.ofNullable(clazz.cast(shuttingDown()));
case "StartFailed":
return Optional.ofNullable(clazz.cast(startFailed()));
case "StopFailed":
return Optional.ofNullable(clazz.cast(stopFailed()));
case "Stopped":
return Optional.ofNullable(clazz.cast(stopped()));
case "Stopping":
return Optional.ofNullable(clazz.cast(stopping()));
case "Terminated":
return Optional.ofNullable(clazz.cast(terminated()));
case "Terminating":
return Optional.ofNullable(clazz.cast(terminating()));
case "Unassigning":
return Optional.ofNullable(clazz.cast(unassigning()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function