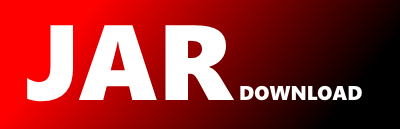
software.amazon.awssdk.services.opsworks.model.VolumeConfiguration Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an Amazon EBS volume configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class VolumeConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField MOUNT_POINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MountPoint").getter(getter(VolumeConfiguration::mountPoint)).setter(setter(Builder::mountPoint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MountPoint").build()).build();
private static final SdkField RAID_LEVEL_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RaidLevel").getter(getter(VolumeConfiguration::raidLevel)).setter(setter(Builder::raidLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RaidLevel").build()).build();
private static final SdkField NUMBER_OF_DISKS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("NumberOfDisks").getter(getter(VolumeConfiguration::numberOfDisks))
.setter(setter(Builder::numberOfDisks))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NumberOfDisks").build()).build();
private static final SdkField SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Size")
.getter(getter(VolumeConfiguration::size)).setter(setter(Builder::size))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Size").build()).build();
private static final SdkField VOLUME_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VolumeType").getter(getter(VolumeConfiguration::volumeType)).setter(setter(Builder::volumeType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VolumeType").build()).build();
private static final SdkField IOPS_FIELD = SdkField. builder(MarshallingType.INTEGER).memberName("Iops")
.getter(getter(VolumeConfiguration::iops)).setter(setter(Builder::iops))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Iops").build()).build();
private static final SdkField ENCRYPTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("Encrypted").getter(getter(VolumeConfiguration::encrypted)).setter(setter(Builder::encrypted))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encrypted").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(MOUNT_POINT_FIELD,
RAID_LEVEL_FIELD, NUMBER_OF_DISKS_FIELD, SIZE_FIELD, VOLUME_TYPE_FIELD, IOPS_FIELD, ENCRYPTED_FIELD));
private static final long serialVersionUID = 1L;
private final String mountPoint;
private final Integer raidLevel;
private final Integer numberOfDisks;
private final Integer size;
private final String volumeType;
private final Integer iops;
private final Boolean encrypted;
private VolumeConfiguration(BuilderImpl builder) {
this.mountPoint = builder.mountPoint;
this.raidLevel = builder.raidLevel;
this.numberOfDisks = builder.numberOfDisks;
this.size = builder.size;
this.volumeType = builder.volumeType;
this.iops = builder.iops;
this.encrypted = builder.encrypted;
}
/**
*
* The volume mount point. For example "/dev/sdh".
*
*
* @return The volume mount point. For example "/dev/sdh".
*/
public final String mountPoint() {
return mountPoint;
}
/**
*
* The volume RAID level.
*
*
* @return The volume RAID level.
*/
public final Integer raidLevel() {
return raidLevel;
}
/**
*
* The number of disks in the volume.
*
*
* @return The number of disks in the volume.
*/
public final Integer numberOfDisks() {
return numberOfDisks;
}
/**
*
* The volume size.
*
*
* @return The volume size.
*/
public final Integer size() {
return size;
}
/**
*
* The volume type. For more information, see Amazon EBS Volume Types.
*
*
* -
*
* standard
- Magnetic. Magnetic volumes must have a minimum size of 1 GiB and a maximum size of 1024
* GiB.
*
*
* -
*
* io1
- Provisioned IOPS (SSD). PIOPS volumes must have a minimum size of 4 GiB and a maximum size of
* 16384 GiB.
*
*
* -
*
* gp2
- General Purpose (SSD). General purpose volumes must have a minimum size of 1 GiB and a maximum
* size of 16384 GiB.
*
*
* -
*
* st1
- Throughput Optimized hard disk drive (HDD). Throughput optimized HDD volumes must have a
* minimum size of 500 GiB and a maximum size of 16384 GiB.
*
*
* -
*
* sc1
- Cold HDD. Cold HDD volumes must have a minimum size of 500 GiB and a maximum size of 16384
* GiB.
*
*
*
*
* @return The volume type. For more information, see Amazon EBS Volume
* Types.
*
* -
*
* standard
- Magnetic. Magnetic volumes must have a minimum size of 1 GiB and a maximum size
* of 1024 GiB.
*
*
* -
*
* io1
- Provisioned IOPS (SSD). PIOPS volumes must have a minimum size of 4 GiB and a maximum
* size of 16384 GiB.
*
*
* -
*
* gp2
- General Purpose (SSD). General purpose volumes must have a minimum size of 1 GiB and a
* maximum size of 16384 GiB.
*
*
* -
*
* st1
- Throughput Optimized hard disk drive (HDD). Throughput optimized HDD volumes must have
* a minimum size of 500 GiB and a maximum size of 16384 GiB.
*
*
* -
*
* sc1
- Cold HDD. Cold HDD volumes must have a minimum size of 500 GiB and a maximum size of
* 16384 GiB.
*
*
*/
public final String volumeType() {
return volumeType;
}
/**
*
* For PIOPS volumes, the IOPS per disk.
*
*
* @return For PIOPS volumes, the IOPS per disk.
*/
public final Integer iops() {
return iops;
}
/**
*
* Specifies whether an Amazon EBS volume is encrypted. For more information, see Amazon EBS Encryption.
*
*
* @return Specifies whether an Amazon EBS volume is encrypted. For more information, see Amazon EBS Encryption.
*/
public final Boolean encrypted() {
return encrypted;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(mountPoint());
hashCode = 31 * hashCode + Objects.hashCode(raidLevel());
hashCode = 31 * hashCode + Objects.hashCode(numberOfDisks());
hashCode = 31 * hashCode + Objects.hashCode(size());
hashCode = 31 * hashCode + Objects.hashCode(volumeType());
hashCode = 31 * hashCode + Objects.hashCode(iops());
hashCode = 31 * hashCode + Objects.hashCode(encrypted());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof VolumeConfiguration)) {
return false;
}
VolumeConfiguration other = (VolumeConfiguration) obj;
return Objects.equals(mountPoint(), other.mountPoint()) && Objects.equals(raidLevel(), other.raidLevel())
&& Objects.equals(numberOfDisks(), other.numberOfDisks()) && Objects.equals(size(), other.size())
&& Objects.equals(volumeType(), other.volumeType()) && Objects.equals(iops(), other.iops())
&& Objects.equals(encrypted(), other.encrypted());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("VolumeConfiguration").add("MountPoint", mountPoint()).add("RaidLevel", raidLevel())
.add("NumberOfDisks", numberOfDisks()).add("Size", size()).add("VolumeType", volumeType()).add("Iops", iops())
.add("Encrypted", encrypted()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "MountPoint":
return Optional.ofNullable(clazz.cast(mountPoint()));
case "RaidLevel":
return Optional.ofNullable(clazz.cast(raidLevel()));
case "NumberOfDisks":
return Optional.ofNullable(clazz.cast(numberOfDisks()));
case "Size":
return Optional.ofNullable(clazz.cast(size()));
case "VolumeType":
return Optional.ofNullable(clazz.cast(volumeType()));
case "Iops":
return Optional.ofNullable(clazz.cast(iops()));
case "Encrypted":
return Optional.ofNullable(clazz.cast(encrypted()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function