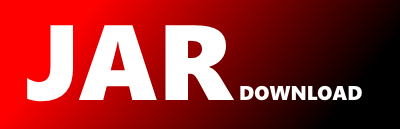
software.amazon.awssdk.services.opsworks.model.UpdateInstanceRequest Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateInstanceRequest extends OpsWorksRequest implements
ToCopyableBuilder {
private static final SdkField INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceId").getter(getter(UpdateInstanceRequest::instanceId)).setter(setter(Builder::instanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceId").build()).build();
private static final SdkField> LAYER_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LayerIds")
.getter(getter(UpdateInstanceRequest::layerIds))
.setter(setter(Builder::layerIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LayerIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(UpdateInstanceRequest::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField AUTO_SCALING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoScalingType").getter(getter(UpdateInstanceRequest::autoScalingTypeAsString))
.setter(setter(Builder::autoScalingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingType").build()).build();
private static final SdkField HOSTNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Hostname").getter(getter(UpdateInstanceRequest::hostname)).setter(setter(Builder::hostname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Hostname").build()).build();
private static final SdkField OS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Os")
.getter(getter(UpdateInstanceRequest::os)).setter(setter(Builder::os))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Os").build()).build();
private static final SdkField AMI_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("AmiId")
.getter(getter(UpdateInstanceRequest::amiId)).setter(setter(Builder::amiId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmiId").build()).build();
private static final SdkField SSH_KEY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SshKeyName").getter(getter(UpdateInstanceRequest::sshKeyName)).setter(setter(Builder::sshKeyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SshKeyName").build()).build();
private static final SdkField ARCHITECTURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Architecture").getter(getter(UpdateInstanceRequest::architectureAsString))
.setter(setter(Builder::architecture))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Architecture").build()).build();
private static final SdkField INSTALL_UPDATES_ON_BOOT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("InstallUpdatesOnBoot").getter(getter(UpdateInstanceRequest::installUpdatesOnBoot))
.setter(setter(Builder::installUpdatesOnBoot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstallUpdatesOnBoot").build())
.build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized").getter(getter(UpdateInstanceRequest::ebsOptimized)).setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized").build()).build();
private static final SdkField AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AgentVersion").getter(getter(UpdateInstanceRequest::agentVersion)).setter(setter(Builder::agentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AgentVersion").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INSTANCE_ID_FIELD,
LAYER_IDS_FIELD, INSTANCE_TYPE_FIELD, AUTO_SCALING_TYPE_FIELD, HOSTNAME_FIELD, OS_FIELD, AMI_ID_FIELD,
SSH_KEY_NAME_FIELD, ARCHITECTURE_FIELD, INSTALL_UPDATES_ON_BOOT_FIELD, EBS_OPTIMIZED_FIELD, AGENT_VERSION_FIELD));
private final String instanceId;
private final List layerIds;
private final String instanceType;
private final String autoScalingType;
private final String hostname;
private final String os;
private final String amiId;
private final String sshKeyName;
private final String architecture;
private final Boolean installUpdatesOnBoot;
private final Boolean ebsOptimized;
private final String agentVersion;
private UpdateInstanceRequest(BuilderImpl builder) {
super(builder);
this.instanceId = builder.instanceId;
this.layerIds = builder.layerIds;
this.instanceType = builder.instanceType;
this.autoScalingType = builder.autoScalingType;
this.hostname = builder.hostname;
this.os = builder.os;
this.amiId = builder.amiId;
this.sshKeyName = builder.sshKeyName;
this.architecture = builder.architecture;
this.installUpdatesOnBoot = builder.installUpdatesOnBoot;
this.ebsOptimized = builder.ebsOptimized;
this.agentVersion = builder.agentVersion;
}
/**
*
* The instance ID.
*
*
* @return The instance ID.
*/
public final String instanceId() {
return instanceId;
}
/**
* For responses, this returns true if the service returned a value for the LayerIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasLayerIds() {
return layerIds != null && !(layerIds instanceof SdkAutoConstructList);
}
/**
*
* The instance's layer IDs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLayerIds} method.
*
*
* @return The instance's layer IDs.
*/
public final List layerIds() {
return layerIds;
}
/**
*
* The instance type, such as t2.micro
. For a list of supported instance types, open the stack in the
* console, choose Instances, and choose + Instance. The Size list contains the currently
* supported types. For more information, see Instance Families and Types.
* The parameter values that you use to specify the various types are in the API Name column of the
* Available Instance Types table.
*
*
* @return The instance type, such as t2.micro
. For a list of supported instance types, open the stack
* in the console, choose Instances, and choose + Instance. The Size list contains the
* currently supported types. For more information, see Instance Families and
* Types. The parameter values that you use to specify the various types are in the API Name
* column of the Available Instance Types table.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* For load-based or time-based instances, the type. Windows stacks can use only time-based instances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoScalingType}
* will return {@link AutoScalingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoScalingTypeAsString}.
*
*
* @return For load-based or time-based instances, the type. Windows stacks can use only time-based instances.
* @see AutoScalingType
*/
public final AutoScalingType autoScalingType() {
return AutoScalingType.fromValue(autoScalingType);
}
/**
*
* For load-based or time-based instances, the type. Windows stacks can use only time-based instances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoScalingType}
* will return {@link AutoScalingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoScalingTypeAsString}.
*
*
* @return For load-based or time-based instances, the type. Windows stacks can use only time-based instances.
* @see AutoScalingType
*/
public final String autoScalingTypeAsString() {
return autoScalingType;
}
/**
*
* The instance host name. The following are character limits for instance host names.
*
*
* -
*
* Linux-based instances: 63 characters
*
*
* -
*
* Windows-based instances: 15 characters
*
*
*
*
* @return The instance host name. The following are character limits for instance host names.
*
* -
*
* Linux-based instances: 63 characters
*
*
* -
*
* Windows-based instances: 15 characters
*
*
*/
public final String hostname() {
return hostname;
}
/**
*
* The instance's operating system, which must be set to one of the following. You cannot update an instance that is
* using a custom AMI.
*
*
* -
*
* A supported Linux operating system: An Amazon Linux version, such as Amazon Linux 2
,
* Amazon Linux 2018.03
, Amazon Linux 2017.09
, Amazon Linux 2017.03
,
* Amazon Linux 2016.09
, Amazon Linux 2016.03
, Amazon Linux 2015.09
, or
* Amazon Linux 2015.03
.
*
*
* -
*
* A supported Ubuntu operating system, such as Ubuntu 18.04 LTS
, Ubuntu 16.04 LTS
,
* Ubuntu 14.04 LTS
, or Ubuntu 12.04 LTS
.
*
*
* -
*
* CentOS Linux 7
*
*
* -
*
* Red Hat Enterprise Linux 7
*
*
* -
*
* A supported Windows operating system, such as Microsoft Windows Server 2012 R2 Base
,
* Microsoft Windows Server 2012 R2 with SQL Server Express
,
* Microsoft Windows Server 2012 R2 with SQL Server Standard
, or
* Microsoft Windows Server 2012 R2 with SQL Server Web
.
*
*
*
*
* Not all operating systems are supported with all versions of Chef. For more information about supported operating
* systems, see OpsWorks
* Stacks Operating Systems.
*
*
* The default option is the current Amazon Linux version. If you set this parameter to Custom
, you
* must use the AmiId parameter to specify the custom AMI that you want to use. For more information about how to
* use custom AMIs with OpsWorks, see Using Custom
* AMIs.
*
*
*
* You can specify a different Linux operating system for the updated stack, but you cannot change from Linux to
* Windows or Windows to Linux.
*
*
*
* @return The instance's operating system, which must be set to one of the following. You cannot update an instance
* that is using a custom AMI.
*
* -
*
* A supported Linux operating system: An Amazon Linux version, such as Amazon Linux 2
,
* Amazon Linux 2018.03
, Amazon Linux 2017.09
, Amazon Linux 2017.03
,
* Amazon Linux 2016.09
, Amazon Linux 2016.03
, Amazon Linux 2015.09
,
* or Amazon Linux 2015.03
.
*
*
* -
*
* A supported Ubuntu operating system, such as Ubuntu 18.04 LTS
, Ubuntu 16.04 LTS
, Ubuntu 14.04 LTS
, or Ubuntu 12.04 LTS
.
*
*
* -
*
* CentOS Linux 7
*
*
* -
*
* Red Hat Enterprise Linux 7
*
*
* -
*
* A supported Windows operating system, such as Microsoft Windows Server 2012 R2 Base
,
* Microsoft Windows Server 2012 R2 with SQL Server Express
,
* Microsoft Windows Server 2012 R2 with SQL Server Standard
, or
* Microsoft Windows Server 2012 R2 with SQL Server Web
.
*
*
*
*
* Not all operating systems are supported with all versions of Chef. For more information about supported
* operating systems, see OpsWorks Stacks
* Operating Systems.
*
*
* The default option is the current Amazon Linux version. If you set this parameter to Custom
,
* you must use the AmiId parameter to specify the custom AMI that you want to use. For more information
* about how to use custom AMIs with OpsWorks, see Using
* Custom AMIs.
*
*
*
* You can specify a different Linux operating system for the updated stack, but you cannot change from
* Linux to Windows or Windows to Linux.
*
*/
public final String os() {
return os;
}
/**
*
* The ID of the AMI that was used to create the instance. The value of this parameter must be the same AMI ID that
* the instance is already using. You cannot apply a new AMI to an instance by running UpdateInstance.
* UpdateInstance does not work on instances that are using custom AMIs.
*
*
* @return The ID of the AMI that was used to create the instance. The value of this parameter must be the same AMI
* ID that the instance is already using. You cannot apply a new AMI to an instance by running
* UpdateInstance. UpdateInstance does not work on instances that are using custom AMIs.
*/
public final String amiId() {
return amiId;
}
/**
*
* The instance's Amazon EC2 key name.
*
*
* @return The instance's Amazon EC2 key name.
*/
public final String sshKeyName() {
return sshKeyName;
}
/**
*
* The instance architecture. Instance types do not necessarily support both architectures. For a list of the
* architectures that are supported by the different instance types, see Instance Families and Types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #architecture} will
* return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #architectureAsString}.
*
*
* @return The instance architecture. Instance types do not necessarily support both architectures. For a list of
* the architectures that are supported by the different instance types, see Instance Families and
* Types.
* @see Architecture
*/
public final Architecture architecture() {
return Architecture.fromValue(architecture);
}
/**
*
* The instance architecture. Instance types do not necessarily support both architectures. For a list of the
* architectures that are supported by the different instance types, see Instance Families and Types.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #architecture} will
* return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #architectureAsString}.
*
*
* @return The instance architecture. Instance types do not necessarily support both architectures. For a list of
* the architectures that are supported by the different instance types, see Instance Families and
* Types.
* @see Architecture
*/
public final String architectureAsString() {
return architecture;
}
/**
*
* Whether to install operating system and package updates when the instance boots. The default value is
* true
. To control when updates are installed, set this value to false
. You must then
* update your instances manually by using CreateDeployment to run the update_dependencies
stack
* command or by manually running yum
(Amazon Linux) or apt-get
(Ubuntu) on the instances.
*
*
*
* We strongly recommend using the default value of true
, to ensure that your instances have the latest
* security updates.
*
*
*
* @return Whether to install operating system and package updates when the instance boots. The default value is
* true
. To control when updates are installed, set this value to false
. You must
* then update your instances manually by using CreateDeployment to run the
* update_dependencies
stack command or by manually running yum
(Amazon Linux) or
* apt-get
(Ubuntu) on the instances.
*
* We strongly recommend using the default value of true
, to ensure that your instances have
* the latest security updates.
*
*/
public final Boolean installUpdatesOnBoot() {
return installUpdatesOnBoot;
}
/**
*
* This property cannot be updated.
*
*
* @return This property cannot be updated.
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* The default OpsWorks Stacks agent version. You have the following options:
*
*
* -
*
* INHERIT
- Use the stack's default agent version setting.
*
*
* -
*
* version_number - Use the specified agent version. This value overrides the stack's default setting. To
* update the agent version, you must edit the instance configuration and specify a new version. OpsWorks Stacks
* installs that version on the instance.
*
*
*
*
* The default setting is INHERIT
. To specify an agent version, you must use the complete version
* number, not the abbreviated number shown on the console. For a list of available agent version numbers, call
* DescribeAgentVersions.
*
*
* AgentVersion cannot be set to Chef 12.2.
*
*
* @return The default OpsWorks Stacks agent version. You have the following options:
*
* -
*
* INHERIT
- Use the stack's default agent version setting.
*
*
* -
*
* version_number - Use the specified agent version. This value overrides the stack's default
* setting. To update the agent version, you must edit the instance configuration and specify a new version.
* OpsWorks Stacks installs that version on the instance.
*
*
*
*
* The default setting is INHERIT
. To specify an agent version, you must use the complete
* version number, not the abbreviated number shown on the console. For a list of available agent version
* numbers, call DescribeAgentVersions.
*
*
* AgentVersion cannot be set to Chef 12.2.
*/
public final String agentVersion() {
return agentVersion;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(instanceId());
hashCode = 31 * hashCode + Objects.hashCode(hasLayerIds() ? layerIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hostname());
hashCode = 31 * hashCode + Objects.hashCode(os());
hashCode = 31 * hashCode + Objects.hashCode(amiId());
hashCode = 31 * hashCode + Objects.hashCode(sshKeyName());
hashCode = 31 * hashCode + Objects.hashCode(architectureAsString());
hashCode = 31 * hashCode + Objects.hashCode(installUpdatesOnBoot());
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(agentVersion());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateInstanceRequest)) {
return false;
}
UpdateInstanceRequest other = (UpdateInstanceRequest) obj;
return Objects.equals(instanceId(), other.instanceId()) && hasLayerIds() == other.hasLayerIds()
&& Objects.equals(layerIds(), other.layerIds()) && Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(autoScalingTypeAsString(), other.autoScalingTypeAsString())
&& Objects.equals(hostname(), other.hostname()) && Objects.equals(os(), other.os())
&& Objects.equals(amiId(), other.amiId()) && Objects.equals(sshKeyName(), other.sshKeyName())
&& Objects.equals(architectureAsString(), other.architectureAsString())
&& Objects.equals(installUpdatesOnBoot(), other.installUpdatesOnBoot())
&& Objects.equals(ebsOptimized(), other.ebsOptimized()) && Objects.equals(agentVersion(), other.agentVersion());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateInstanceRequest").add("InstanceId", instanceId())
.add("LayerIds", hasLayerIds() ? layerIds() : null).add("InstanceType", instanceType())
.add("AutoScalingType", autoScalingTypeAsString()).add("Hostname", hostname()).add("Os", os())
.add("AmiId", amiId()).add("SshKeyName", sshKeyName()).add("Architecture", architectureAsString())
.add("InstallUpdatesOnBoot", installUpdatesOnBoot()).add("EbsOptimized", ebsOptimized())
.add("AgentVersion", agentVersion()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InstanceId":
return Optional.ofNullable(clazz.cast(instanceId()));
case "LayerIds":
return Optional.ofNullable(clazz.cast(layerIds()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "AutoScalingType":
return Optional.ofNullable(clazz.cast(autoScalingTypeAsString()));
case "Hostname":
return Optional.ofNullable(clazz.cast(hostname()));
case "Os":
return Optional.ofNullable(clazz.cast(os()));
case "AmiId":
return Optional.ofNullable(clazz.cast(amiId()));
case "SshKeyName":
return Optional.ofNullable(clazz.cast(sshKeyName()));
case "Architecture":
return Optional.ofNullable(clazz.cast(architectureAsString()));
case "InstallUpdatesOnBoot":
return Optional.ofNullable(clazz.cast(installUpdatesOnBoot()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "AgentVersion":
return Optional.ofNullable(clazz.cast(agentVersion()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* Linux-based instances: 63 characters
*
*
* -
*
* Windows-based instances: 15 characters
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder hostname(String hostname);
/**
*
* The instance's operating system, which must be set to one of the following. You cannot update an instance
* that is using a custom AMI.
*
*
* -
*
* A supported Linux operating system: An Amazon Linux version, such as Amazon Linux 2
,
* Amazon Linux 2018.03
, Amazon Linux 2017.09
, Amazon Linux 2017.03
,
* Amazon Linux 2016.09
, Amazon Linux 2016.03
, Amazon Linux 2015.09
, or
* Amazon Linux 2015.03
.
*
*
* -
*
* A supported Ubuntu operating system, such as Ubuntu 18.04 LTS
, Ubuntu 16.04 LTS
,
* Ubuntu 14.04 LTS
, or Ubuntu 12.04 LTS
.
*
*
* -
*
* CentOS Linux 7
*
*
* -
*
* Red Hat Enterprise Linux 7
*
*
* -
*
* A supported Windows operating system, such as Microsoft Windows Server 2012 R2 Base
,
* Microsoft Windows Server 2012 R2 with SQL Server Express
,
* Microsoft Windows Server 2012 R2 with SQL Server Standard
, or
* Microsoft Windows Server 2012 R2 with SQL Server Web
.
*
*
*
*
* Not all operating systems are supported with all versions of Chef. For more information about supported
* operating systems, see OpsWorks Stacks
* Operating Systems.
*
*
* The default option is the current Amazon Linux version. If you set this parameter to Custom
, you
* must use the AmiId parameter to specify the custom AMI that you want to use. For more information about how
* to use custom AMIs with OpsWorks, see Using Custom
* AMIs.
*
*
*
* You can specify a different Linux operating system for the updated stack, but you cannot change from Linux to
* Windows or Windows to Linux.
*
*
*
* @param os
* The instance's operating system, which must be set to one of the following. You cannot update an
* instance that is using a custom AMI.
*
* -
*
* A supported Linux operating system: An Amazon Linux version, such as Amazon Linux 2
,
* Amazon Linux 2018.03
, Amazon Linux 2017.09
,
* Amazon Linux 2017.03
, Amazon Linux 2016.09
,
* Amazon Linux 2016.03
, Amazon Linux 2015.09
, or
* Amazon Linux 2015.03
.
*
*
* -
*
* A supported Ubuntu operating system, such as Ubuntu 18.04 LTS
,
* Ubuntu 16.04 LTS
, Ubuntu 14.04 LTS
, or Ubuntu 12.04 LTS
.
*
*
* -
*
* CentOS Linux 7
*
*
* -
*
* Red Hat Enterprise Linux 7
*
*
* -
*
* A supported Windows operating system, such as Microsoft Windows Server 2012 R2 Base
,
* Microsoft Windows Server 2012 R2 with SQL Server Express
,
* Microsoft Windows Server 2012 R2 with SQL Server Standard
, or
* Microsoft Windows Server 2012 R2 with SQL Server Web
.
*
*
*
*
* Not all operating systems are supported with all versions of Chef. For more information about
* supported operating systems, see OpsWorks Stacks
* Operating Systems.
*
*
* The default option is the current Amazon Linux version. If you set this parameter to
* Custom
, you must use the AmiId parameter to specify the custom AMI that you want to use.
* For more information about how to use custom AMIs with OpsWorks, see Using
* Custom AMIs.
*
*
*
* You can specify a different Linux operating system for the updated stack, but you cannot change from
* Linux to Windows or Windows to Linux.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder os(String os);
/**
*
* The ID of the AMI that was used to create the instance. The value of this parameter must be the same AMI ID
* that the instance is already using. You cannot apply a new AMI to an instance by running UpdateInstance.
* UpdateInstance does not work on instances that are using custom AMIs.
*
*
* @param amiId
* The ID of the AMI that was used to create the instance. The value of this parameter must be the same
* AMI ID that the instance is already using. You cannot apply a new AMI to an instance by running
* UpdateInstance. UpdateInstance does not work on instances that are using custom AMIs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder amiId(String amiId);
/**
*
* The instance's Amazon EC2 key name.
*
*
* @param sshKeyName
* The instance's Amazon EC2 key name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder sshKeyName(String sshKeyName);
/**
*
* The instance architecture. Instance types do not necessarily support both architectures. For a list of the
* architectures that are supported by the different instance types, see Instance Families and
* Types.
*
*
* @param architecture
* The instance architecture. Instance types do not necessarily support both architectures. For a list of
* the architectures that are supported by the different instance types, see Instance Families and
* Types.
* @see Architecture
* @return Returns a reference to this object so that method calls can be chained together.
* @see Architecture
*/
Builder architecture(String architecture);
/**
*
* The instance architecture. Instance types do not necessarily support both architectures. For a list of the
* architectures that are supported by the different instance types, see Instance Families and
* Types.
*
*
* @param architecture
* The instance architecture. Instance types do not necessarily support both architectures. For a list of
* the architectures that are supported by the different instance types, see Instance Families and
* Types.
* @see Architecture
* @return Returns a reference to this object so that method calls can be chained together.
* @see Architecture
*/
Builder architecture(Architecture architecture);
/**
*
* Whether to install operating system and package updates when the instance boots. The default value is
* true
. To control when updates are installed, set this value to false
. You must then
* update your instances manually by using CreateDeployment to run the update_dependencies
* stack command or by manually running yum
(Amazon Linux) or apt-get
(Ubuntu) on the
* instances.
*
*
*
* We strongly recommend using the default value of true
, to ensure that your instances have the
* latest security updates.
*
*
*
* @param installUpdatesOnBoot
* Whether to install operating system and package updates when the instance boots. The default value is
* true
. To control when updates are installed, set this value to false
. You
* must then update your instances manually by using CreateDeployment to run the
* update_dependencies
stack command or by manually running yum
(Amazon Linux)
* or apt-get
(Ubuntu) on the instances.
*
* We strongly recommend using the default value of true
, to ensure that your instances have
* the latest security updates.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder installUpdatesOnBoot(Boolean installUpdatesOnBoot);
/**
*
* This property cannot be updated.
*
*
* @param ebsOptimized
* This property cannot be updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder ebsOptimized(Boolean ebsOptimized);
/**
*
* The default OpsWorks Stacks agent version. You have the following options:
*
*
* -
*
* INHERIT
- Use the stack's default agent version setting.
*
*
* -
*
* version_number - Use the specified agent version. This value overrides the stack's default setting. To
* update the agent version, you must edit the instance configuration and specify a new version. OpsWorks Stacks
* installs that version on the instance.
*
*
*
*
* The default setting is INHERIT
. To specify an agent version, you must use the complete version
* number, not the abbreviated number shown on the console. For a list of available agent version numbers, call
* DescribeAgentVersions.
*
*
* AgentVersion cannot be set to Chef 12.2.
*
*
* @param agentVersion
* The default OpsWorks Stacks agent version. You have the following options:
*
* -
*
* INHERIT
- Use the stack's default agent version setting.
*
*
* -
*
* version_number - Use the specified agent version. This value overrides the stack's default
* setting. To update the agent version, you must edit the instance configuration and specify a new
* version. OpsWorks Stacks installs that version on the instance.
*
*
*
*
* The default setting is INHERIT
. To specify an agent version, you must use the complete
* version number, not the abbreviated number shown on the console. For a list of available agent version
* numbers, call DescribeAgentVersions.
*
*
* AgentVersion cannot be set to Chef 12.2.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder agentVersion(String agentVersion);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends OpsWorksRequest.BuilderImpl implements Builder {
private String instanceId;
private List layerIds = DefaultSdkAutoConstructList.getInstance();
private String instanceType;
private String autoScalingType;
private String hostname;
private String os;
private String amiId;
private String sshKeyName;
private String architecture;
private Boolean installUpdatesOnBoot;
private Boolean ebsOptimized;
private String agentVersion;
private BuilderImpl() {
}
private BuilderImpl(UpdateInstanceRequest model) {
super(model);
instanceId(model.instanceId);
layerIds(model.layerIds);
instanceType(model.instanceType);
autoScalingType(model.autoScalingType);
hostname(model.hostname);
os(model.os);
amiId(model.amiId);
sshKeyName(model.sshKeyName);
architecture(model.architecture);
installUpdatesOnBoot(model.installUpdatesOnBoot);
ebsOptimized(model.ebsOptimized);
agentVersion(model.agentVersion);
}
public final String getInstanceId() {
return instanceId;
}
public final void setInstanceId(String instanceId) {
this.instanceId = instanceId;
}
@Override
public final Builder instanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
public final Collection getLayerIds() {
if (layerIds instanceof SdkAutoConstructList) {
return null;
}
return layerIds;
}
public final void setLayerIds(Collection layerIds) {
this.layerIds = StringsCopier.copy(layerIds);
}
@Override
public final Builder layerIds(Collection layerIds) {
this.layerIds = StringsCopier.copy(layerIds);
return this;
}
@Override
@SafeVarargs
public final Builder layerIds(String... layerIds) {
layerIds(Arrays.asList(layerIds));
return this;
}
public final String getInstanceType() {
return instanceType;
}
public final void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
@Override
public final Builder instanceType(String instanceType) {
this.instanceType = instanceType;
return this;
}
public final String getAutoScalingType() {
return autoScalingType;
}
public final void setAutoScalingType(String autoScalingType) {
this.autoScalingType = autoScalingType;
}
@Override
public final Builder autoScalingType(String autoScalingType) {
this.autoScalingType = autoScalingType;
return this;
}
@Override
public final Builder autoScalingType(AutoScalingType autoScalingType) {
this.autoScalingType(autoScalingType == null ? null : autoScalingType.toString());
return this;
}
public final String getHostname() {
return hostname;
}
public final void setHostname(String hostname) {
this.hostname = hostname;
}
@Override
public final Builder hostname(String hostname) {
this.hostname = hostname;
return this;
}
public final String getOs() {
return os;
}
public final void setOs(String os) {
this.os = os;
}
@Override
public final Builder os(String os) {
this.os = os;
return this;
}
public final String getAmiId() {
return amiId;
}
public final void setAmiId(String amiId) {
this.amiId = amiId;
}
@Override
public final Builder amiId(String amiId) {
this.amiId = amiId;
return this;
}
public final String getSshKeyName() {
return sshKeyName;
}
public final void setSshKeyName(String sshKeyName) {
this.sshKeyName = sshKeyName;
}
@Override
public final Builder sshKeyName(String sshKeyName) {
this.sshKeyName = sshKeyName;
return this;
}
public final String getArchitecture() {
return architecture;
}
public final void setArchitecture(String architecture) {
this.architecture = architecture;
}
@Override
public final Builder architecture(String architecture) {
this.architecture = architecture;
return this;
}
@Override
public final Builder architecture(Architecture architecture) {
this.architecture(architecture == null ? null : architecture.toString());
return this;
}
public final Boolean getInstallUpdatesOnBoot() {
return installUpdatesOnBoot;
}
public final void setInstallUpdatesOnBoot(Boolean installUpdatesOnBoot) {
this.installUpdatesOnBoot = installUpdatesOnBoot;
}
@Override
public final Builder installUpdatesOnBoot(Boolean installUpdatesOnBoot) {
this.installUpdatesOnBoot = installUpdatesOnBoot;
return this;
}
public final Boolean getEbsOptimized() {
return ebsOptimized;
}
public final void setEbsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
}
@Override
public final Builder ebsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
return this;
}
public final String getAgentVersion() {
return agentVersion;
}
public final void setAgentVersion(String agentVersion) {
this.agentVersion = agentVersion;
}
@Override
public final Builder agentVersion(String agentVersion) {
this.agentVersion = agentVersion;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateInstanceRequest build() {
return new UpdateInstanceRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}