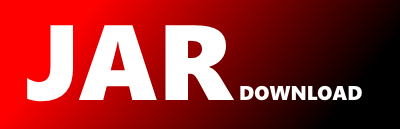
software.amazon.awssdk.services.opsworks.DefaultOpsWorksAsyncClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ScheduledExecutorService;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.opsworks.internal.OpsWorksServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.AssignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.AssignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.AssociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.AttachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.CloneStackRequest;
import software.amazon.awssdk.services.opsworks.model.CloneStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateAppRequest;
import software.amazon.awssdk.services.opsworks.model.CreateAppResponse;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentRequest;
import software.amazon.awssdk.services.opsworks.model.CreateDeploymentResponse;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.CreateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.CreateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.CreateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.CreateStackRequest;
import software.amazon.awssdk.services.opsworks.model.CreateStackResponse;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.CreateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteAppRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteAppResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteLayerResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteStackRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteStackResponse;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DeleteUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.DeregisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAgentVersionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeAppsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeCommandsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeDeploymentsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeEcsClustersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticIpsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeElasticLoadBalancersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLayersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeOperatingSystemsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribePermissionsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRaidArraysResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeRdsDbInstancesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeServiceErrorsResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackProvisioningParametersResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStackSummaryResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeStacksResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeUserProfilesResponse;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesRequest;
import software.amazon.awssdk.services.opsworks.model.DescribeVolumesResponse;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerRequest;
import software.amazon.awssdk.services.opsworks.model.DetachElasticLoadBalancerResponse;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.DisassociateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionRequest;
import software.amazon.awssdk.services.opsworks.model.GetHostnameSuggestionResponse;
import software.amazon.awssdk.services.opsworks.model.GrantAccessRequest;
import software.amazon.awssdk.services.opsworks.model.GrantAccessResponse;
import software.amazon.awssdk.services.opsworks.model.ListTagsRequest;
import software.amazon.awssdk.services.opsworks.model.ListTagsResponse;
import software.amazon.awssdk.services.opsworks.model.OpsWorksException;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RebootInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterEcsClusterResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.RegisterVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ResourceNotFoundException;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetLoadBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.SetPermissionRequest;
import software.amazon.awssdk.services.opsworks.model.SetPermissionResponse;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingRequest;
import software.amazon.awssdk.services.opsworks.model.SetTimeBasedAutoScalingResponse;
import software.amazon.awssdk.services.opsworks.model.StartInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StartInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StartStackRequest;
import software.amazon.awssdk.services.opsworks.model.StartStackResponse;
import software.amazon.awssdk.services.opsworks.model.StopInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.StopInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.StopStackRequest;
import software.amazon.awssdk.services.opsworks.model.StopStackResponse;
import software.amazon.awssdk.services.opsworks.model.TagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.TagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UnassignVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.UntagResourceRequest;
import software.amazon.awssdk.services.opsworks.model.UntagResourceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateAppRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateAppResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateElasticIpResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateLayerResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateMyUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateRdsDbInstanceResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateStackRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateStackResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateUserProfileResponse;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeRequest;
import software.amazon.awssdk.services.opsworks.model.UpdateVolumeResponse;
import software.amazon.awssdk.services.opsworks.model.ValidationException;
import software.amazon.awssdk.services.opsworks.transform.AssignInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AssignVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AssociateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.AttachElasticLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CloneStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateDeploymentRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.CreateUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeleteUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterEcsClusterRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DeregisterVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeAgentVersionsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeAppsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeCommandsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeDeploymentsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeEcsClustersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeElasticIpsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeElasticLoadBalancersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeInstancesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeLayersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeLoadBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeMyUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeOperatingSystemsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribePermissionsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeRaidArraysRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeRdsDbInstancesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeServiceErrorsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStackProvisioningParametersRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStackSummaryRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeStacksRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeTimeBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeUserProfilesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DescribeVolumesRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DetachElasticLoadBalancerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.DisassociateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.GetHostnameSuggestionRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.GrantAccessRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.ListTagsRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RebootInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterEcsClusterRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.RegisterVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetLoadBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetPermissionRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.SetTimeBasedAutoScalingRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StartInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StartStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StopInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.StopStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UnassignInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UnassignVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateAppRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateElasticIpRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateLayerRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateMyUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateRdsDbInstanceRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateStackRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateUserProfileRequestMarshaller;
import software.amazon.awssdk.services.opsworks.transform.UpdateVolumeRequestMarshaller;
import software.amazon.awssdk.services.opsworks.waiters.OpsWorksAsyncWaiter;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link OpsWorksAsyncClient}.
*
* @see OpsWorksAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultOpsWorksAsyncClient implements OpsWorksAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultOpsWorksAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.AWS_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final ScheduledExecutorService executorService;
protected DefaultOpsWorksAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
this.executorService = clientConfiguration.option(SdkClientOption.SCHEDULED_EXECUTOR_SERVICE);
}
/**
*
* Assign a registered instance to a layer.
*
*
* -
*
* You can assign registered on-premises instances to any layer type.
*
*
* -
*
* You can assign registered Amazon EC2 instances only to custom layers.
*
*
* -
*
* You cannot use this action with instances that were created with OpsWorks Stacks.
*
*
*
*
* Required Permissions: To use this action, an Identity and Access Management (IAM) user must have a Manage
* permissions level for the stack or an attached policy that explicitly grants permissions. For more information on
* user permissions, see Managing User
* Permissions.
*
*
* @param assignInstanceRequest
* @return A Java Future containing the result of the AssignInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.AssignInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture assignInstance(AssignInstanceRequest assignInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(assignInstanceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, assignInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssignInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssignInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssignInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssignInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(assignInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Assigns one of the stack's registered Amazon EBS volumes to a specified instance. The volume must first be
* registered with the stack by calling RegisterVolume. After you register the volume, you must call
* UpdateVolume to specify a mount point before calling AssignVolume
. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param assignVolumeRequest
* @return A Java Future containing the result of the AssignVolume operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.AssignVolume
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture assignVolume(AssignVolumeRequest assignVolumeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(assignVolumeRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, assignVolumeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssignVolume");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AssignVolumeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssignVolume").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssignVolumeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(assignVolumeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Associates one of the stack's registered Elastic IP addresses with a specified instance. The address must first
* be registered with the stack by calling RegisterElasticIp. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param associateElasticIpRequest
* @return A Java Future containing the result of the AssociateElasticIp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.AssociateElasticIp
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture associateElasticIp(AssociateElasticIpRequest associateElasticIpRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(associateElasticIpRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateElasticIp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AssociateElasticIp").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AssociateElasticIpRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(associateElasticIpRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches an Elastic Load Balancing load balancer to a specified layer. OpsWorks Stacks does not support
* Application Load Balancer. You can only use Classic Load Balancer with OpsWorks Stacks. For more information, see
* Elastic Load Balancing.
*
*
*
* You must create the Elastic Load Balancing instance separately, by using the Elastic Load Balancing console, API,
* or CLI. For more information, see the Elastic Load Balancing
* Developer Guide.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param attachElasticLoadBalancerRequest
* @return A Java Future containing the result of the AttachElasticLoadBalancer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.AttachElasticLoadBalancer
* @see AWS API Documentation
*/
@Override
public CompletableFuture attachElasticLoadBalancer(
AttachElasticLoadBalancerRequest attachElasticLoadBalancerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(attachElasticLoadBalancerRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, attachElasticLoadBalancerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AttachElasticLoadBalancer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AttachElasticLoadBalancerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachElasticLoadBalancer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new AttachElasticLoadBalancerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(attachElasticLoadBalancerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a clone of a specified stack. For more information, see Clone a Stack. By
* default, all parameters are set to the values used by the parent stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param cloneStackRequest
* @return A Java Future containing the result of the CloneStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CloneStack
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cloneStack(CloneStackRequest cloneStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cloneStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cloneStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CloneStack");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CloneStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CloneStack")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CloneStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cloneStackRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an app for a specified stack. For more information, see Creating Apps.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createAppRequest
* @return A Java Future containing the result of the CreateApp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateApp
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createApp(CreateAppRequest createAppRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createAppRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateApp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateApp")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateAppRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(createAppRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Runs deployment or stack commands. For more information, see Deploying Apps and Run Stack Commands.
*
*
* Required Permissions: To use this action, an IAM user must have a Deploy or Manage permissions level for
* the stack, or an attached policy that explicitly grants permissions. For more information on user permissions,
* see Managing User
* Permissions.
*
*
* @param createDeploymentRequest
* @return A Java Future containing the result of the CreateDeployment operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateDeployment
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createDeployment(CreateDeploymentRequest createDeploymentRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createDeploymentRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDeploymentRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDeployment");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDeploymentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateDeployment").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateDeploymentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createDeploymentRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an instance in a specified stack. For more information, see Adding an Instance to a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createInstanceRequest
* @return A Java Future containing the result of the CreateInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createInstance(CreateInstanceRequest createInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createInstanceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a layer. For more information, see How to Create a
* Layer.
*
*
*
* You should use CreateLayer for noncustom layer types such as PHP App Server only if the stack does not
* have an existing layer of that type. A stack can have at most one instance of each noncustom layer; if you
* attempt to create a second instance, CreateLayer fails. A stack can have an arbitrary number of custom
* layers, so you can call CreateLayer as many times as you like for that layer type.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param createLayerRequest
* @return A Java Future containing the result of the CreateLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateLayer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createLayer(CreateLayerRequest createLayerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLayerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLayerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLayer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateLayerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLayer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateLayerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createLayerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new stack. For more information, see Create a New Stack.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createStackRequest
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateStack
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createStack(CreateStackRequest createStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateStack");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateStack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createStackRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a new user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param createUserProfileRequest
* @return A Java Future containing the result of the CreateUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.CreateUserProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createUserProfile(CreateUserProfileRequest createUserProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createUserProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateUserProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateUserProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateUserProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createUserProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified app.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteAppRequest
* @return A Java Future containing the result of the DeleteApp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeleteApp
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteApp(DeleteAppRequest deleteAppRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteAppRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteAppRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteApp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteAppResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteApp")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteAppRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(deleteAppRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified instance, which terminates the associated Amazon EC2 instance. You must stop an instance
* before you can delete it.
*
*
* For more information, see Deleting Instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteInstanceRequest
* @return A Java Future containing the result of the DeleteInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeleteInstance
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteInstance(DeleteInstanceRequest deleteInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteInstanceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified layer. You must first stop and then delete all associated instances or unassign registered
* instances. For more information, see How to Delete a
* Layer.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteLayerRequest
* @return A Java Future containing the result of the DeleteLayer operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeleteLayer
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteLayer(DeleteLayerRequest deleteLayerRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLayerRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLayerRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLayer");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteLayerResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLayer").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteLayerRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteLayerRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a specified stack. You must first delete all instances, layers, and apps or deregister registered
* instances. For more information, see Shut Down a Stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deleteStackRequest
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeleteStack
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteStack(DeleteStackRequest deleteStackRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteStackRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteStackRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteStack");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteStackResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteStack").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteStackRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteStackRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes a user profile.
*
*
* Required Permissions: To use this action, an IAM user must have an attached policy that explicitly grants
* permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param deleteUserProfileRequest
* @return A Java Future containing the result of the DeleteUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeleteUserProfile
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deleteUserProfile(DeleteUserProfileRequest deleteUserProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteUserProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteUserProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteUserProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteUserProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteUserProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters a specified Amazon ECS cluster from a stack. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see https://docs.aws.amazon.com/opsworks/latest/userguide/opsworks-security-users.html.
*
*
* @param deregisterEcsClusterRequest
* @return A Java Future containing the result of the DeregisterEcsCluster operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeregisterEcsCluster
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deregisterEcsCluster(
DeregisterEcsClusterRequest deregisterEcsClusterRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterEcsClusterRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterEcsClusterRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterEcsCluster");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterEcsClusterResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterEcsCluster").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterEcsClusterRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterEcsClusterRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters a specified Elastic IP address. The address can be registered by another stack after it is
* deregistered. For more information, see Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterElasticIpRequest
* @return A Java Future containing the result of the DeregisterElasticIp operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeregisterElasticIp
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deregisterElasticIp(
DeregisterElasticIpRequest deregisterElasticIpRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterElasticIpRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterElasticIpRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterElasticIp");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterElasticIpResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterElasticIp").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterElasticIpRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterElasticIpRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregister an instance from OpsWorks Stacks. The instance can be a registered instance (Amazon EC2 or
* on-premises) or an instance created with OpsWorks. This action removes the instance from the stack and returns it
* to your control.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterInstanceRequest
* @return A Java Future containing the result of the DeregisterInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeregisterInstance
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture deregisterInstance(DeregisterInstanceRequest deregisterInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters an Amazon RDS instance.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterRdsDbInstanceRequest
* @return A Java Future containing the result of the DeregisterRdsDbInstance operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeregisterRdsDbInstance
* @see AWS API Documentation
*/
@Override
public CompletableFuture deregisterRdsDbInstance(
DeregisterRdsDbInstanceRequest deregisterRdsDbInstanceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterRdsDbInstanceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterRdsDbInstanceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterRdsDbInstance");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterRdsDbInstanceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterRdsDbInstance").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterRdsDbInstanceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterRdsDbInstanceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deregisters an Amazon EBS volume. The volume can then be registered by another stack. For more information, see
* Resource Management.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param deregisterVolumeRequest
* @return A Java Future containing the result of the DeregisterVolume operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DeregisterVolume
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deregisterVolume(DeregisterVolumeRequest deregisterVolumeRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deregisterVolumeRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deregisterVolumeRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeregisterVolume");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeregisterVolumeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterVolume").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeregisterVolumeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deregisterVolumeRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the available OpsWorks Stacks agent versions. You must specify a stack ID or a configuration manager.
* DescribeAgentVersions
returns a list of available agent versions for the specified stack or
* configuration manager.
*
*
* @param describeAgentVersionsRequest
* @return A Java Future containing the result of the DescribeAgentVersions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeAgentVersions
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeAgentVersions(
DescribeAgentVersionsRequest describeAgentVersionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAgentVersionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAgentVersionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeAgentVersions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAgentVersionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAgentVersions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAgentVersionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAgentVersionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Requests a description of a specified set of apps.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeAppsRequest
* @return A Java Future containing the result of the DescribeApps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeApps
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeApps(DescribeAppsRequest describeAppsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeAppsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeAppsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeApps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DescribeAppsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeApps").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeAppsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeAppsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the results of specified commands.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeCommandsRequest
* @return A Java Future containing the result of the DescribeCommands operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeCommands
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeCommands(DescribeCommandsRequest describeCommandsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeCommandsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeCommandsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeCommands");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCommandsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCommands").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeCommandsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeCommandsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Requests a description of a specified set of deployments.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeDeploymentsRequest
* @return A Java Future containing the result of the DescribeDeployments operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeDeployments
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeDeployments(
DescribeDeploymentsRequest describeDeploymentsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeDeploymentsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeDeploymentsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeDeployments");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeDeploymentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeDeployments").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeDeploymentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeDeploymentsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes Amazon ECS clusters that are registered with a stack. If you specify only a stack ID, you can use the
* MaxResults
and NextToken
parameters to paginate the response. However, OpsWorks Stacks
* currently supports only one cluster per layer, so the result set has a maximum of one element.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack or an attached policy that explicitly grants permission. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeEcsClustersRequest
* @return A Java Future containing the result of the DescribeEcsClusters operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeEcsClusters
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeEcsClusters(
DescribeEcsClustersRequest describeEcsClustersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeEcsClustersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeEcsClustersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeEcsClusters");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEcsClustersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEcsClusters").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeEcsClustersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeEcsClustersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes Elastic IP
* addresses.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticIpsRequest
* @return A Java Future containing the result of the DescribeElasticIps operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeElasticIps
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeElasticIps(DescribeElasticIpsRequest describeElasticIpsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeElasticIpsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeElasticIpsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeElasticIps");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeElasticIpsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeElasticIps").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeElasticIpsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeElasticIpsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a stack's Elastic Load Balancing instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeElasticLoadBalancersRequest
* @return A Java Future containing the result of the DescribeElasticLoadBalancers operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeElasticLoadBalancers
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeElasticLoadBalancers(
DescribeElasticLoadBalancersRequest describeElasticLoadBalancersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeElasticLoadBalancersRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeElasticLoadBalancersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeElasticLoadBalancers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeElasticLoadBalancersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeElasticLoadBalancers").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeElasticLoadBalancersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeElasticLoadBalancersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Requests a description of a set of instances.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeInstancesRequest
* @return A Java Future containing the result of the DescribeInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeInstances
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeInstances(DescribeInstancesRequest describeInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeInstances").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeInstancesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeInstancesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Requests a description of one or more layers in a specified stack.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLayersRequest
* @return A Java Future containing the result of the DescribeLayers operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeLayers
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture describeLayers(DescribeLayersRequest describeLayersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLayersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLayersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLayers");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeLayersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLayers").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeLayersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeLayersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes load-based auto scaling configurations for specified layers.
*
*
*
* You must specify at least one of the parameters.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeLoadBasedAutoScalingRequest
* @return A Java Future containing the result of the DescribeLoadBasedAutoScaling operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeLoadBasedAutoScaling
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeLoadBasedAutoScaling(
DescribeLoadBasedAutoScalingRequest describeLoadBasedAutoScalingRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeLoadBasedAutoScalingRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeLoadBasedAutoScalingRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeLoadBasedAutoScaling");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeLoadBasedAutoScalingResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeLoadBasedAutoScaling").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeLoadBasedAutoScalingRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeLoadBasedAutoScalingRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes a user's SSH information.
*
*
* Required Permissions: To use this action, an IAM user must have self-management enabled or an attached
* policy that explicitly grants permissions. For more information about user permissions, see Managing User
* Permissions.
*
*
* @param describeMyUserProfileRequest
* @return A Java Future containing the result of the DescribeMyUserProfile operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeMyUserProfile
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeMyUserProfile(
DescribeMyUserProfileRequest describeMyUserProfileRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeMyUserProfileRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeMyUserProfileRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeMyUserProfile");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeMyUserProfileResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeMyUserProfile").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeMyUserProfileRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeMyUserProfileRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the operating systems that are supported by OpsWorks Stacks.
*
*
* @param describeOperatingSystemsRequest
* @return A Java Future containing the result of the DescribeOperatingSystems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeOperatingSystems
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeOperatingSystems(
DescribeOperatingSystemsRequest describeOperatingSystemsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeOperatingSystemsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeOperatingSystemsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeOperatingSystems");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOperatingSystemsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOperatingSystems").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeOperatingSystemsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeOperatingSystemsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes the permissions for a specified stack.
*
*
* Required Permissions: To use this action, an IAM user must have a Manage permissions level for the stack,
* or an attached policy that explicitly grants permissions. For more information on user permissions, see Managing User
* Permissions.
*
*
* @param describePermissionsRequest
* @return A Java Future containing the result of the DescribePermissions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribePermissions
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describePermissions(
DescribePermissionsRequest describePermissionsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describePermissionsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describePermissionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribePermissions");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePermissionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePermissions").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribePermissionsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describePermissionsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describe an instance's RAID arrays.
*
*
*
* This call accepts only one resource-identifying parameter.
*
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* @param describeRaidArraysRequest
* @return A Java Future containing the result of the DescribeRaidArrays operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeRaidArrays
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeRaidArrays(DescribeRaidArraysRequest describeRaidArraysRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeRaidArraysRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRaidArraysRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRaidArrays");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRaidArraysResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeRaidArrays").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DescribeRaidArraysRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(describeRaidArraysRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Describes Amazon RDS instances.
*
*
* Required Permissions: To use this action, an IAM user must have a Show, Deploy, or Manage permissions
* level for the stack, or an attached policy that explicitly grants permissions. For more information about user
* permissions, see Managing User
* Permissions.
*
*
* This call accepts only one resource-identifying parameter.
*
*
* @param describeRdsDbInstancesRequest
* @return A Java Future containing the result of the DescribeRdsDbInstances operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Indicates that a request was not valid.
* - ResourceNotFoundException Indicates that a resource was not found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OpsWorksException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OpsWorksAsyncClient.DescribeRdsDbInstances
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeRdsDbInstances(
DescribeRdsDbInstancesRequest describeRdsDbInstancesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(describeRdsDbInstancesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, describeRdsDbInstancesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "OpsWorks");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DescribeRdsDbInstances");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeRdsDbInstancesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams