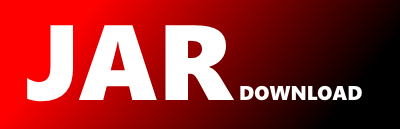
software.amazon.awssdk.services.opsworks.model.CloudWatchLogsLogStream Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the CloudWatch Logs configuration for a layer. For detailed information about members of this data type,
* see the CloudWatch Logs Agent
* Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CloudWatchLogsLogStream implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField LOG_GROUP_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogGroupName").getter(getter(CloudWatchLogsLogStream::logGroupName))
.setter(setter(Builder::logGroupName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogGroupName").build()).build();
private static final SdkField DATETIME_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DatetimeFormat").getter(getter(CloudWatchLogsLogStream::datetimeFormat))
.setter(setter(Builder::datetimeFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DatetimeFormat").build()).build();
private static final SdkField TIME_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TimeZone").getter(getter(CloudWatchLogsLogStream::timeZoneAsString)).setter(setter(Builder::timeZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TimeZone").build()).build();
private static final SdkField FILE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("File")
.getter(getter(CloudWatchLogsLogStream::file)).setter(setter(Builder::file))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("File").build()).build();
private static final SdkField FILE_FINGERPRINT_LINES_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FileFingerprintLines").getter(getter(CloudWatchLogsLogStream::fileFingerprintLines))
.setter(setter(Builder::fileFingerprintLines))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FileFingerprintLines").build())
.build();
private static final SdkField MULTI_LINE_START_PATTERN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MultiLineStartPattern").getter(getter(CloudWatchLogsLogStream::multiLineStartPattern))
.setter(setter(Builder::multiLineStartPattern))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiLineStartPattern").build())
.build();
private static final SdkField INITIAL_POSITION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InitialPosition").getter(getter(CloudWatchLogsLogStream::initialPositionAsString))
.setter(setter(Builder::initialPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InitialPosition").build()).build();
private static final SdkField ENCODING_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Encoding").getter(getter(CloudWatchLogsLogStream::encodingAsString)).setter(setter(Builder::encoding))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Encoding").build()).build();
private static final SdkField BUFFER_DURATION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BufferDuration").getter(getter(CloudWatchLogsLogStream::bufferDuration))
.setter(setter(Builder::bufferDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BufferDuration").build()).build();
private static final SdkField BATCH_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BatchCount").getter(getter(CloudWatchLogsLogStream::batchCount)).setter(setter(Builder::batchCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchCount").build()).build();
private static final SdkField BATCH_SIZE_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("BatchSize").getter(getter(CloudWatchLogsLogStream::batchSize)).setter(setter(Builder::batchSize))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BatchSize").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOG_GROUP_NAME_FIELD,
DATETIME_FORMAT_FIELD, TIME_ZONE_FIELD, FILE_FIELD, FILE_FINGERPRINT_LINES_FIELD, MULTI_LINE_START_PATTERN_FIELD,
INITIAL_POSITION_FIELD, ENCODING_FIELD, BUFFER_DURATION_FIELD, BATCH_COUNT_FIELD, BATCH_SIZE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String logGroupName;
private final String datetimeFormat;
private final String timeZone;
private final String file;
private final String fileFingerprintLines;
private final String multiLineStartPattern;
private final String initialPosition;
private final String encoding;
private final Integer bufferDuration;
private final Integer batchCount;
private final Integer batchSize;
private CloudWatchLogsLogStream(BuilderImpl builder) {
this.logGroupName = builder.logGroupName;
this.datetimeFormat = builder.datetimeFormat;
this.timeZone = builder.timeZone;
this.file = builder.file;
this.fileFingerprintLines = builder.fileFingerprintLines;
this.multiLineStartPattern = builder.multiLineStartPattern;
this.initialPosition = builder.initialPosition;
this.encoding = builder.encoding;
this.bufferDuration = builder.bufferDuration;
this.batchCount = builder.batchCount;
this.batchSize = builder.batchSize;
}
/**
*
* Specifies the destination log group. A log group is created automatically if it doesn't already exist. Log group
* names can be between 1 and 512 characters long. Allowed characters include a-z, A-Z, 0-9, '_' (underscore), '-'
* (hyphen), '/' (forward slash), and '.' (period).
*
*
* @return Specifies the destination log group. A log group is created automatically if it doesn't already exist.
* Log group names can be between 1 and 512 characters long. Allowed characters include a-z, A-Z, 0-9, '_'
* (underscore), '-' (hyphen), '/' (forward slash), and '.' (period).
*/
public final String logGroupName() {
return logGroupName;
}
/**
*
* Specifies how the time stamp is extracted from logs. For more information, see the CloudWatch Logs Agent
* Reference.
*
*
* @return Specifies how the time stamp is extracted from logs. For more information, see the CloudWatch Logs Agent
* Reference.
*/
public final String datetimeFormat() {
return datetimeFormat;
}
/**
*
* Specifies the time zone of log event time stamps.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timeZone} will
* return {@link CloudWatchLogsTimeZone#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #timeZoneAsString}.
*
*
* @return Specifies the time zone of log event time stamps.
* @see CloudWatchLogsTimeZone
*/
public final CloudWatchLogsTimeZone timeZone() {
return CloudWatchLogsTimeZone.fromValue(timeZone);
}
/**
*
* Specifies the time zone of log event time stamps.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #timeZone} will
* return {@link CloudWatchLogsTimeZone#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #timeZoneAsString}.
*
*
* @return Specifies the time zone of log event time stamps.
* @see CloudWatchLogsTimeZone
*/
public final String timeZoneAsString() {
return timeZone;
}
/**
*
* Specifies log files that you want to push to CloudWatch Logs.
*
*
* File
can point to a specific file or multiple files (by using wild card characters such as
* /var/log/system.log*
). Only the latest file is pushed to CloudWatch Logs, based on file modification
* time. We recommend that you use wild card characters to specify a series of files of the same type, such as
* access_log.2014-06-01-01
, access_log.2014-06-01-02
, and so on by using a pattern like
* access_log.*
. Don't use a wildcard to match multiple file types, such as access_log_80
* and access_log_443
. To specify multiple, different file types, add another log stream entry to the
* configuration file, so that each log file type is stored in a different log group.
*
*
* Zipped files are not supported.
*
*
* @return Specifies log files that you want to push to CloudWatch Logs.
*
* File
can point to a specific file or multiple files (by using wild card characters such as
* /var/log/system.log*
). Only the latest file is pushed to CloudWatch Logs, based on file
* modification time. We recommend that you use wild card characters to specify a series of files of the
* same type, such as access_log.2014-06-01-01
, access_log.2014-06-01-02
, and so
* on by using a pattern like access_log.*
. Don't use a wildcard to match multiple file types,
* such as access_log_80
and access_log_443
. To specify multiple, different file
* types, add another log stream entry to the configuration file, so that each log file type is stored in a
* different log group.
*
*
* Zipped files are not supported.
*/
public final String file() {
return file;
}
/**
*
* Specifies the range of lines for identifying a file. The valid values are one number, or two dash-delimited
* numbers, such as '1', '2-5'. The default value is '1', meaning the first line is used to calculate the
* fingerprint. Fingerprint lines are not sent to CloudWatch Logs unless all specified lines are available.
*
*
* @return Specifies the range of lines for identifying a file. The valid values are one number, or two
* dash-delimited numbers, such as '1', '2-5'. The default value is '1', meaning the first line is used to
* calculate the fingerprint. Fingerprint lines are not sent to CloudWatch Logs unless all specified lines
* are available.
*/
public final String fileFingerprintLines() {
return fileFingerprintLines;
}
/**
*
* Specifies the pattern for identifying the start of a log message.
*
*
* @return Specifies the pattern for identifying the start of a log message.
*/
public final String multiLineStartPattern() {
return multiLineStartPattern;
}
/**
*
* Specifies where to start to read data (start_of_file or end_of_file). The default is start_of_file. This setting
* is only used if there is no state persisted for that log stream.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #initialPosition}
* will return {@link CloudWatchLogsInitialPosition#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #initialPositionAsString}.
*
*
* @return Specifies where to start to read data (start_of_file or end_of_file). The default is start_of_file. This
* setting is only used if there is no state persisted for that log stream.
* @see CloudWatchLogsInitialPosition
*/
public final CloudWatchLogsInitialPosition initialPosition() {
return CloudWatchLogsInitialPosition.fromValue(initialPosition);
}
/**
*
* Specifies where to start to read data (start_of_file or end_of_file). The default is start_of_file. This setting
* is only used if there is no state persisted for that log stream.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #initialPosition}
* will return {@link CloudWatchLogsInitialPosition#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service
* is available from {@link #initialPositionAsString}.
*
*
* @return Specifies where to start to read data (start_of_file or end_of_file). The default is start_of_file. This
* setting is only used if there is no state persisted for that log stream.
* @see CloudWatchLogsInitialPosition
*/
public final String initialPositionAsString() {
return initialPosition;
}
/**
*
* Specifies the encoding of the log file so that the file can be read correctly. The default is utf_8
.
* Encodings supported by Python codecs.decode()
can be used here.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encoding} will
* return {@link CloudWatchLogsEncoding#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #encodingAsString}.
*
*
* @return Specifies the encoding of the log file so that the file can be read correctly. The default is
* utf_8
. Encodings supported by Python codecs.decode()
can be used here.
* @see CloudWatchLogsEncoding
*/
public final CloudWatchLogsEncoding encoding() {
return CloudWatchLogsEncoding.fromValue(encoding);
}
/**
*
* Specifies the encoding of the log file so that the file can be read correctly. The default is utf_8
.
* Encodings supported by Python codecs.decode()
can be used here.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #encoding} will
* return {@link CloudWatchLogsEncoding#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #encodingAsString}.
*
*
* @return Specifies the encoding of the log file so that the file can be read correctly. The default is
* utf_8
. Encodings supported by Python codecs.decode()
can be used here.
* @see CloudWatchLogsEncoding
*/
public final String encodingAsString() {
return encoding;
}
/**
*
* Specifies the time duration for the batching of log events. The minimum value is 5000ms and default value is
* 5000ms.
*
*
* @return Specifies the time duration for the batching of log events. The minimum value is 5000ms and default value
* is 5000ms.
*/
public final Integer bufferDuration() {
return bufferDuration;
}
/**
*
* Specifies the max number of log events in a batch, up to 10000. The default value is 1000.
*
*
* @return Specifies the max number of log events in a batch, up to 10000. The default value is 1000.
*/
public final Integer batchCount() {
return batchCount;
}
/**
*
* Specifies the maximum size of log events in a batch, in bytes, up to 1048576 bytes. The default value is 32768
* bytes. This size is calculated as the sum of all event messages in UTF-8, plus 26 bytes for each log event.
*
*
* @return Specifies the maximum size of log events in a batch, in bytes, up to 1048576 bytes. The default value is
* 32768 bytes. This size is calculated as the sum of all event messages in UTF-8, plus 26 bytes for each
* log event.
*/
public final Integer batchSize() {
return batchSize;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(logGroupName());
hashCode = 31 * hashCode + Objects.hashCode(datetimeFormat());
hashCode = 31 * hashCode + Objects.hashCode(timeZoneAsString());
hashCode = 31 * hashCode + Objects.hashCode(file());
hashCode = 31 * hashCode + Objects.hashCode(fileFingerprintLines());
hashCode = 31 * hashCode + Objects.hashCode(multiLineStartPattern());
hashCode = 31 * hashCode + Objects.hashCode(initialPositionAsString());
hashCode = 31 * hashCode + Objects.hashCode(encodingAsString());
hashCode = 31 * hashCode + Objects.hashCode(bufferDuration());
hashCode = 31 * hashCode + Objects.hashCode(batchCount());
hashCode = 31 * hashCode + Objects.hashCode(batchSize());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CloudWatchLogsLogStream)) {
return false;
}
CloudWatchLogsLogStream other = (CloudWatchLogsLogStream) obj;
return Objects.equals(logGroupName(), other.logGroupName()) && Objects.equals(datetimeFormat(), other.datetimeFormat())
&& Objects.equals(timeZoneAsString(), other.timeZoneAsString()) && Objects.equals(file(), other.file())
&& Objects.equals(fileFingerprintLines(), other.fileFingerprintLines())
&& Objects.equals(multiLineStartPattern(), other.multiLineStartPattern())
&& Objects.equals(initialPositionAsString(), other.initialPositionAsString())
&& Objects.equals(encodingAsString(), other.encodingAsString())
&& Objects.equals(bufferDuration(), other.bufferDuration()) && Objects.equals(batchCount(), other.batchCount())
&& Objects.equals(batchSize(), other.batchSize());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CloudWatchLogsLogStream").add("LogGroupName", logGroupName())
.add("DatetimeFormat", datetimeFormat()).add("TimeZone", timeZoneAsString()).add("File", file())
.add("FileFingerprintLines", fileFingerprintLines()).add("MultiLineStartPattern", multiLineStartPattern())
.add("InitialPosition", initialPositionAsString()).add("Encoding", encodingAsString())
.add("BufferDuration", bufferDuration()).add("BatchCount", batchCount()).add("BatchSize", batchSize()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LogGroupName":
return Optional.ofNullable(clazz.cast(logGroupName()));
case "DatetimeFormat":
return Optional.ofNullable(clazz.cast(datetimeFormat()));
case "TimeZone":
return Optional.ofNullable(clazz.cast(timeZoneAsString()));
case "File":
return Optional.ofNullable(clazz.cast(file()));
case "FileFingerprintLines":
return Optional.ofNullable(clazz.cast(fileFingerprintLines()));
case "MultiLineStartPattern":
return Optional.ofNullable(clazz.cast(multiLineStartPattern()));
case "InitialPosition":
return Optional.ofNullable(clazz.cast(initialPositionAsString()));
case "Encoding":
return Optional.ofNullable(clazz.cast(encodingAsString()));
case "BufferDuration":
return Optional.ofNullable(clazz.cast(bufferDuration()));
case "BatchCount":
return Optional.ofNullable(clazz.cast(batchCount()));
case "BatchSize":
return Optional.ofNullable(clazz.cast(batchSize()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("LogGroupName", LOG_GROUP_NAME_FIELD);
map.put("DatetimeFormat", DATETIME_FORMAT_FIELD);
map.put("TimeZone", TIME_ZONE_FIELD);
map.put("File", FILE_FIELD);
map.put("FileFingerprintLines", FILE_FINGERPRINT_LINES_FIELD);
map.put("MultiLineStartPattern", MULTI_LINE_START_PATTERN_FIELD);
map.put("InitialPosition", INITIAL_POSITION_FIELD);
map.put("Encoding", ENCODING_FIELD);
map.put("BufferDuration", BUFFER_DURATION_FIELD);
map.put("BatchCount", BATCH_COUNT_FIELD);
map.put("BatchSize", BATCH_SIZE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function