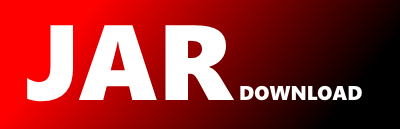
software.amazon.awssdk.services.opsworks.model.Instance Maven / Gradle / Ivy
Show all versions of opsworks Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.opsworks.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes an instance.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Instance implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AgentVersion").getter(getter(Instance::agentVersion)).setter(setter(Builder::agentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AgentVersion").build()).build();
private static final SdkField AMI_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("AmiId")
.getter(getter(Instance::amiId)).setter(setter(Builder::amiId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AmiId").build()).build();
private static final SdkField ARCHITECTURE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Architecture").getter(getter(Instance::architectureAsString)).setter(setter(Builder::architecture))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Architecture").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Instance::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField AUTO_SCALING_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AutoScalingType").getter(getter(Instance::autoScalingTypeAsString))
.setter(setter(Builder::autoScalingType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AutoScalingType").build()).build();
private static final SdkField AVAILABILITY_ZONE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("AvailabilityZone").getter(getter(Instance::availabilityZone)).setter(setter(Builder::availabilityZone))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailabilityZone").build()).build();
private static final SdkField> BLOCK_DEVICE_MAPPINGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("BlockDeviceMappings")
.getter(getter(Instance::blockDeviceMappings))
.setter(setter(Builder::blockDeviceMappings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BlockDeviceMappings").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(BlockDeviceMapping::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_AT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CreatedAt").getter(getter(Instance::createdAt)).setter(setter(Builder::createdAt))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreatedAt").build()).build();
private static final SdkField EBS_OPTIMIZED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("EbsOptimized").getter(getter(Instance::ebsOptimized)).setter(setter(Builder::ebsOptimized))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EbsOptimized").build()).build();
private static final SdkField EC2_INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Ec2InstanceId").getter(getter(Instance::ec2InstanceId)).setter(setter(Builder::ec2InstanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Ec2InstanceId").build()).build();
private static final SdkField ECS_CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EcsClusterArn").getter(getter(Instance::ecsClusterArn)).setter(setter(Builder::ecsClusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EcsClusterArn").build()).build();
private static final SdkField ECS_CONTAINER_INSTANCE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("EcsContainerInstanceArn").getter(getter(Instance::ecsContainerInstanceArn))
.setter(setter(Builder::ecsContainerInstanceArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EcsContainerInstanceArn").build())
.build();
private static final SdkField ELASTIC_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ElasticIp").getter(getter(Instance::elasticIp)).setter(setter(Builder::elasticIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ElasticIp").build()).build();
private static final SdkField HOSTNAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Hostname").getter(getter(Instance::hostname)).setter(setter(Builder::hostname))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Hostname").build()).build();
private static final SdkField INFRASTRUCTURE_CLASS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InfrastructureClass").getter(getter(Instance::infrastructureClass))
.setter(setter(Builder::infrastructureClass))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InfrastructureClass").build())
.build();
private static final SdkField INSTALL_UPDATES_ON_BOOT_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("InstallUpdatesOnBoot").getter(getter(Instance::installUpdatesOnBoot))
.setter(setter(Builder::installUpdatesOnBoot))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstallUpdatesOnBoot").build())
.build();
private static final SdkField INSTANCE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceId").getter(getter(Instance::instanceId)).setter(setter(Builder::instanceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceId").build()).build();
private static final SdkField INSTANCE_PROFILE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceProfileArn").getter(getter(Instance::instanceProfileArn))
.setter(setter(Builder::instanceProfileArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceProfileArn").build())
.build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(Instance::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InstanceType").build()).build();
private static final SdkField LAST_SERVICE_ERROR_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LastServiceErrorId").getter(getter(Instance::lastServiceErrorId))
.setter(setter(Builder::lastServiceErrorId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastServiceErrorId").build())
.build();
private static final SdkField> LAYER_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("LayerIds")
.getter(getter(Instance::layerIds))
.setter(setter(Builder::layerIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LayerIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField OS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Os")
.getter(getter(Instance::os)).setter(setter(Builder::os))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Os").build()).build();
private static final SdkField PLATFORM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Platform").getter(getter(Instance::platform)).setter(setter(Builder::platform))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Platform").build()).build();
private static final SdkField PRIVATE_DNS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PrivateDns").getter(getter(Instance::privateDns)).setter(setter(Builder::privateDns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateDns").build()).build();
private static final SdkField PRIVATE_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PrivateIp").getter(getter(Instance::privateIp)).setter(setter(Builder::privateIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PrivateIp").build()).build();
private static final SdkField PUBLIC_DNS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PublicDns").getter(getter(Instance::publicDns)).setter(setter(Builder::publicDns))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicDns").build()).build();
private static final SdkField PUBLIC_IP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PublicIp").getter(getter(Instance::publicIp)).setter(setter(Builder::publicIp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PublicIp").build()).build();
private static final SdkField REGISTERED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RegisteredBy").getter(getter(Instance::registeredBy)).setter(setter(Builder::registeredBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RegisteredBy").build()).build();
private static final SdkField REPORTED_AGENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ReportedAgentVersion").getter(getter(Instance::reportedAgentVersion))
.setter(setter(Builder::reportedAgentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReportedAgentVersion").build())
.build();
private static final SdkField REPORTED_OS_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ReportedOs").getter(getter(Instance::reportedOs)).setter(setter(Builder::reportedOs))
.constructor(ReportedOs::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReportedOs").build()).build();
private static final SdkField ROOT_DEVICE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RootDeviceType").getter(getter(Instance::rootDeviceTypeAsString))
.setter(setter(Builder::rootDeviceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RootDeviceType").build()).build();
private static final SdkField ROOT_DEVICE_VOLUME_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RootDeviceVolumeId").getter(getter(Instance::rootDeviceVolumeId))
.setter(setter(Builder::rootDeviceVolumeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RootDeviceVolumeId").build())
.build();
private static final SdkField> SECURITY_GROUP_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroupIds")
.getter(getter(Instance::securityGroupIds))
.setter(setter(Builder::securityGroupIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SecurityGroupIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField SSH_HOST_DSA_KEY_FINGERPRINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SshHostDsaKeyFingerprint").getter(getter(Instance::sshHostDsaKeyFingerprint))
.setter(setter(Builder::sshHostDsaKeyFingerprint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SshHostDsaKeyFingerprint").build())
.build();
private static final SdkField SSH_HOST_RSA_KEY_FINGERPRINT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SshHostRsaKeyFingerprint").getter(getter(Instance::sshHostRsaKeyFingerprint))
.setter(setter(Builder::sshHostRsaKeyFingerprint))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SshHostRsaKeyFingerprint").build())
.build();
private static final SdkField SSH_KEY_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SshKeyName").getter(getter(Instance::sshKeyName)).setter(setter(Builder::sshKeyName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SshKeyName").build()).build();
private static final SdkField STACK_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StackId").getter(getter(Instance::stackId)).setter(setter(Builder::stackId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StackId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Status")
.getter(getter(Instance::status)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Status").build()).build();
private static final SdkField SUBNET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SubnetId").getter(getter(Instance::subnetId)).setter(setter(Builder::subnetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SubnetId").build()).build();
private static final SdkField TENANCY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Tenancy")
.getter(getter(Instance::tenancy)).setter(setter(Builder::tenancy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tenancy").build()).build();
private static final SdkField VIRTUALIZATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("VirtualizationType").getter(getter(Instance::virtualizationTypeAsString))
.setter(setter(Builder::virtualizationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("VirtualizationType").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AGENT_VERSION_FIELD,
AMI_ID_FIELD, ARCHITECTURE_FIELD, ARN_FIELD, AUTO_SCALING_TYPE_FIELD, AVAILABILITY_ZONE_FIELD,
BLOCK_DEVICE_MAPPINGS_FIELD, CREATED_AT_FIELD, EBS_OPTIMIZED_FIELD, EC2_INSTANCE_ID_FIELD, ECS_CLUSTER_ARN_FIELD,
ECS_CONTAINER_INSTANCE_ARN_FIELD, ELASTIC_IP_FIELD, HOSTNAME_FIELD, INFRASTRUCTURE_CLASS_FIELD,
INSTALL_UPDATES_ON_BOOT_FIELD, INSTANCE_ID_FIELD, INSTANCE_PROFILE_ARN_FIELD, INSTANCE_TYPE_FIELD,
LAST_SERVICE_ERROR_ID_FIELD, LAYER_IDS_FIELD, OS_FIELD, PLATFORM_FIELD, PRIVATE_DNS_FIELD, PRIVATE_IP_FIELD,
PUBLIC_DNS_FIELD, PUBLIC_IP_FIELD, REGISTERED_BY_FIELD, REPORTED_AGENT_VERSION_FIELD, REPORTED_OS_FIELD,
ROOT_DEVICE_TYPE_FIELD, ROOT_DEVICE_VOLUME_ID_FIELD, SECURITY_GROUP_IDS_FIELD, SSH_HOST_DSA_KEY_FINGERPRINT_FIELD,
SSH_HOST_RSA_KEY_FINGERPRINT_FIELD, SSH_KEY_NAME_FIELD, STACK_ID_FIELD, STATUS_FIELD, SUBNET_ID_FIELD, TENANCY_FIELD,
VIRTUALIZATION_TYPE_FIELD));
private static final long serialVersionUID = 1L;
private final String agentVersion;
private final String amiId;
private final String architecture;
private final String arn;
private final String autoScalingType;
private final String availabilityZone;
private final List blockDeviceMappings;
private final String createdAt;
private final Boolean ebsOptimized;
private final String ec2InstanceId;
private final String ecsClusterArn;
private final String ecsContainerInstanceArn;
private final String elasticIp;
private final String hostname;
private final String infrastructureClass;
private final Boolean installUpdatesOnBoot;
private final String instanceId;
private final String instanceProfileArn;
private final String instanceType;
private final String lastServiceErrorId;
private final List layerIds;
private final String os;
private final String platform;
private final String privateDns;
private final String privateIp;
private final String publicDns;
private final String publicIp;
private final String registeredBy;
private final String reportedAgentVersion;
private final ReportedOs reportedOs;
private final String rootDeviceType;
private final String rootDeviceVolumeId;
private final List securityGroupIds;
private final String sshHostDsaKeyFingerprint;
private final String sshHostRsaKeyFingerprint;
private final String sshKeyName;
private final String stackId;
private final String status;
private final String subnetId;
private final String tenancy;
private final String virtualizationType;
private Instance(BuilderImpl builder) {
this.agentVersion = builder.agentVersion;
this.amiId = builder.amiId;
this.architecture = builder.architecture;
this.arn = builder.arn;
this.autoScalingType = builder.autoScalingType;
this.availabilityZone = builder.availabilityZone;
this.blockDeviceMappings = builder.blockDeviceMappings;
this.createdAt = builder.createdAt;
this.ebsOptimized = builder.ebsOptimized;
this.ec2InstanceId = builder.ec2InstanceId;
this.ecsClusterArn = builder.ecsClusterArn;
this.ecsContainerInstanceArn = builder.ecsContainerInstanceArn;
this.elasticIp = builder.elasticIp;
this.hostname = builder.hostname;
this.infrastructureClass = builder.infrastructureClass;
this.installUpdatesOnBoot = builder.installUpdatesOnBoot;
this.instanceId = builder.instanceId;
this.instanceProfileArn = builder.instanceProfileArn;
this.instanceType = builder.instanceType;
this.lastServiceErrorId = builder.lastServiceErrorId;
this.layerIds = builder.layerIds;
this.os = builder.os;
this.platform = builder.platform;
this.privateDns = builder.privateDns;
this.privateIp = builder.privateIp;
this.publicDns = builder.publicDns;
this.publicIp = builder.publicIp;
this.registeredBy = builder.registeredBy;
this.reportedAgentVersion = builder.reportedAgentVersion;
this.reportedOs = builder.reportedOs;
this.rootDeviceType = builder.rootDeviceType;
this.rootDeviceVolumeId = builder.rootDeviceVolumeId;
this.securityGroupIds = builder.securityGroupIds;
this.sshHostDsaKeyFingerprint = builder.sshHostDsaKeyFingerprint;
this.sshHostRsaKeyFingerprint = builder.sshHostRsaKeyFingerprint;
this.sshKeyName = builder.sshKeyName;
this.stackId = builder.stackId;
this.status = builder.status;
this.subnetId = builder.subnetId;
this.tenancy = builder.tenancy;
this.virtualizationType = builder.virtualizationType;
}
/**
*
* The agent version. This parameter is set to INHERIT
if the instance inherits the default stack
* setting or to a a version number for a fixed agent version.
*
*
* @return The agent version. This parameter is set to INHERIT
if the instance inherits the default
* stack setting or to a a version number for a fixed agent version.
*/
public final String agentVersion() {
return agentVersion;
}
/**
*
* A custom AMI ID to be used to create the instance. For more information, see Instances
*
*
* @return A custom AMI ID to be used to create the instance. For more information, see Instances
*/
public final String amiId() {
return amiId;
}
/**
*
* The instance architecture: "i386" or "x86_64".
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #architecture} will
* return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #architectureAsString}.
*
*
* @return The instance architecture: "i386" or "x86_64".
* @see Architecture
*/
public final Architecture architecture() {
return Architecture.fromValue(architecture);
}
/**
*
* The instance architecture: "i386" or "x86_64".
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #architecture} will
* return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #architectureAsString}.
*
*
* @return The instance architecture: "i386" or "x86_64".
* @see Architecture
*/
public final String architectureAsString() {
return architecture;
}
/**
*
* The instance's Amazon Resource Number (ARN).
*
*
* @return The instance's Amazon Resource Number (ARN).
*/
public final String arn() {
return arn;
}
/**
*
* For load-based or time-based instances, the type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoScalingType}
* will return {@link AutoScalingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoScalingTypeAsString}.
*
*
* @return For load-based or time-based instances, the type.
* @see AutoScalingType
*/
public final AutoScalingType autoScalingType() {
return AutoScalingType.fromValue(autoScalingType);
}
/**
*
* For load-based or time-based instances, the type.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #autoScalingType}
* will return {@link AutoScalingType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #autoScalingTypeAsString}.
*
*
* @return For load-based or time-based instances, the type.
* @see AutoScalingType
*/
public final String autoScalingTypeAsString() {
return autoScalingType;
}
/**
*
* The instance Availability Zone. For more information, see Regions and Endpoints.
*
*
* @return The instance Availability Zone. For more information, see Regions and Endpoints.
*/
public final String availabilityZone() {
return availabilityZone;
}
/**
* For responses, this returns true if the service returned a value for the BlockDeviceMappings property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasBlockDeviceMappings() {
return blockDeviceMappings != null && !(blockDeviceMappings instanceof SdkAutoConstructList);
}
/**
*
* An array of BlockDeviceMapping
objects that specify the instance's block device mappings.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasBlockDeviceMappings} method.
*
*
* @return An array of BlockDeviceMapping
objects that specify the instance's block device mappings.
*/
public final List blockDeviceMappings() {
return blockDeviceMappings;
}
/**
*
* The time that the instance was created.
*
*
* @return The time that the instance was created.
*/
public final String createdAt() {
return createdAt;
}
/**
*
* Whether this is an Amazon EBS-optimized instance.
*
*
* @return Whether this is an Amazon EBS-optimized instance.
*/
public final Boolean ebsOptimized() {
return ebsOptimized;
}
/**
*
* The ID of the associated Amazon EC2 instance.
*
*
* @return The ID of the associated Amazon EC2 instance.
*/
public final String ec2InstanceId() {
return ec2InstanceId;
}
/**
*
* For container instances, the Amazon ECS cluster's ARN.
*
*
* @return For container instances, the Amazon ECS cluster's ARN.
*/
public final String ecsClusterArn() {
return ecsClusterArn;
}
/**
*
* For container instances, the instance's ARN.
*
*
* @return For container instances, the instance's ARN.
*/
public final String ecsContainerInstanceArn() {
return ecsContainerInstanceArn;
}
/**
*
* The instance Elastic
* IP address.
*
*
* @return The instance Elastic IP
* address.
*/
public final String elasticIp() {
return elasticIp;
}
/**
*
* The instance host name. The following are character limits for instance host names.
*
*
* -
*
* Linux-based instances: 63 characters
*
*
* -
*
* Windows-based instances: 15 characters
*
*
*
*
* @return The instance host name. The following are character limits for instance host names.
*
* -
*
* Linux-based instances: 63 characters
*
*
* -
*
* Windows-based instances: 15 characters
*
*
*/
public final String hostname() {
return hostname;
}
/**
*
* For registered instances, the infrastructure class: ec2
or on-premises
.
*
*
* @return For registered instances, the infrastructure class: ec2
or on-premises
.
*/
public final String infrastructureClass() {
return infrastructureClass;
}
/**
*
* Whether to install operating system and package updates when the instance boots. The default value is
* true
. If this value is set to false
, you must update instances manually by using
* CreateDeployment to run the update_dependencies
stack command or by manually running
* yum
(Amazon Linux) or apt-get
(Ubuntu) on the instances.
*
*
*
* We strongly recommend using the default value of true
to ensure that your instances have the latest
* security updates.
*
*
*
* @return Whether to install operating system and package updates when the instance boots. The default value is
* true
. If this value is set to false
, you must update instances manually by
* using CreateDeployment to run the update_dependencies
stack command or by manually
* running yum
(Amazon Linux) or apt-get
(Ubuntu) on the instances.
*
* We strongly recommend using the default value of true
to ensure that your instances have the
* latest security updates.
*
*/
public final Boolean installUpdatesOnBoot() {
return installUpdatesOnBoot;
}
/**
*
* The instance ID.
*
*
* @return The instance ID.
*/
public final String instanceId() {
return instanceId;
}
/**
*
* The ARN of the instance's IAM profile. For more information about IAM ARNs, see Using Identifiers.
*
*
* @return The ARN of the instance's IAM profile. For more information about IAM ARNs, see Using Identifiers.
*/
public final String instanceProfileArn() {
return instanceProfileArn;
}
/**
*
* The instance type, such as t2.micro
.
*
*
* @return The instance type, such as t2.micro
.
*/
public final String instanceType() {
return instanceType;
}
/**
*
* The ID of the last service error. For more information, call DescribeServiceErrors.
*
*
* @return The ID of the last service error. For more information, call DescribeServiceErrors.
*/
public final String lastServiceErrorId() {
return lastServiceErrorId;
}
/**
* For responses, this returns true if the service returned a value for the LayerIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasLayerIds() {
return layerIds != null && !(layerIds instanceof SdkAutoConstructList);
}
/**
*
* An array containing the instance layer IDs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasLayerIds} method.
*
*
* @return An array containing the instance layer IDs.
*/
public final List layerIds() {
return layerIds;
}
/**
*
* The instance's operating system.
*
*
* @return The instance's operating system.
*/
public final String os() {
return os;
}
/**
*
* The instance's platform.
*
*
* @return The instance's platform.
*/
public final String platform() {
return platform;
}
/**
*
* The instance's private DNS name.
*
*
* @return The instance's private DNS name.
*/
public final String privateDns() {
return privateDns;
}
/**
*
* The instance's private IP address.
*
*
* @return The instance's private IP address.
*/
public final String privateIp() {
return privateIp;
}
/**
*
* The instance public DNS name.
*
*
* @return The instance public DNS name.
*/
public final String publicDns() {
return publicDns;
}
/**
*
* The instance public IP address.
*
*
* @return The instance public IP address.
*/
public final String publicIp() {
return publicIp;
}
/**
*
* For registered instances, who performed the registration.
*
*
* @return For registered instances, who performed the registration.
*/
public final String registeredBy() {
return registeredBy;
}
/**
*
* The instance's reported OpsWorks Stacks agent version.
*
*
* @return The instance's reported OpsWorks Stacks agent version.
*/
public final String reportedAgentVersion() {
return reportedAgentVersion;
}
/**
*
* For registered instances, the reported operating system.
*
*
* @return For registered instances, the reported operating system.
*/
public final ReportedOs reportedOs() {
return reportedOs;
}
/**
*
* The instance's root device type. For more information, see Storage for the Root Device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rootDeviceType}
* will return {@link RootDeviceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rootDeviceTypeAsString}.
*
*
* @return The instance's root device type. For more information, see Storage for the Root Device.
* @see RootDeviceType
*/
public final RootDeviceType rootDeviceType() {
return RootDeviceType.fromValue(rootDeviceType);
}
/**
*
* The instance's root device type. For more information, see Storage for the Root Device.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #rootDeviceType}
* will return {@link RootDeviceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #rootDeviceTypeAsString}.
*
*
* @return The instance's root device type. For more information, see Storage for the Root Device.
* @see RootDeviceType
*/
public final String rootDeviceTypeAsString() {
return rootDeviceType;
}
/**
*
* The root device volume ID.
*
*
* @return The root device volume ID.
*/
public final String rootDeviceVolumeId() {
return rootDeviceVolumeId;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroupIds property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroupIds() {
return securityGroupIds != null && !(securityGroupIds instanceof SdkAutoConstructList);
}
/**
*
* An array containing the instance security group IDs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroupIds} method.
*
*
* @return An array containing the instance security group IDs.
*/
public final List securityGroupIds() {
return securityGroupIds;
}
/**
*
* The SSH key's Deep Security Agent (DSA) fingerprint.
*
*
* @return The SSH key's Deep Security Agent (DSA) fingerprint.
*/
public final String sshHostDsaKeyFingerprint() {
return sshHostDsaKeyFingerprint;
}
/**
*
* The SSH key's RSA fingerprint.
*
*
* @return The SSH key's RSA fingerprint.
*/
public final String sshHostRsaKeyFingerprint() {
return sshHostRsaKeyFingerprint;
}
/**
*
* The instance's Amazon EC2 key-pair name.
*
*
* @return The instance's Amazon EC2 key-pair name.
*/
public final String sshKeyName() {
return sshKeyName;
}
/**
*
* The stack ID.
*
*
* @return The stack ID.
*/
public final String stackId() {
return stackId;
}
/**
*
* The instance status:
*
*
* -
*
* booting
*
*
* -
*
* connection_lost
*
*
* -
*
* online
*
*
* -
*
* pending
*
*
* -
*
* rebooting
*
*
* -
*
* requested
*
*
* -
*
* running_setup
*
*
* -
*
* setup_failed
*
*
* -
*
* shutting_down
*
*
* -
*
* start_failed
*
*
* -
*
* stop_failed
*
*
* -
*
* stopped
*
*
* -
*
* stopping
*
*
* -
*
* terminated
*
*
* -
*
* terminating
*
*
*
*
* @return The instance status:
*
* -
*
* booting
*
*
* -
*
* connection_lost
*
*
* -
*
* online
*
*
* -
*
* pending
*
*
* -
*
* rebooting
*
*
* -
*
* requested
*
*
* -
*
* running_setup
*
*
* -
*
* setup_failed
*
*
* -
*
* shutting_down
*
*
* -
*
* start_failed
*
*
* -
*
* stop_failed
*
*
* -
*
* stopped
*
*
* -
*
* stopping
*
*
* -
*
* terminated
*
*
* -
*
* terminating
*
*
*/
public final String status() {
return status;
}
/**
*
* The instance's subnet ID; applicable only if the stack is running in a VPC.
*
*
* @return The instance's subnet ID; applicable only if the stack is running in a VPC.
*/
public final String subnetId() {
return subnetId;
}
/**
*
* The instance's tenancy option, such as dedicated
or host
.
*
*
* @return The instance's tenancy option, such as dedicated
or host
.
*/
public final String tenancy() {
return tenancy;
}
/**
*
* The instance's virtualization type: paravirtual
or hvm
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #virtualizationType} will return {@link VirtualizationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #virtualizationTypeAsString}.
*
*
* @return The instance's virtualization type: paravirtual
or hvm
.
* @see VirtualizationType
*/
public final VirtualizationType virtualizationType() {
return VirtualizationType.fromValue(virtualizationType);
}
/**
*
* The instance's virtualization type: paravirtual
or hvm
.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #virtualizationType} will return {@link VirtualizationType#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #virtualizationTypeAsString}.
*
*
* @return The instance's virtualization type: paravirtual
or hvm
.
* @see VirtualizationType
*/
public final String virtualizationTypeAsString() {
return virtualizationType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(agentVersion());
hashCode = 31 * hashCode + Objects.hashCode(amiId());
hashCode = 31 * hashCode + Objects.hashCode(architectureAsString());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(autoScalingTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(availabilityZone());
hashCode = 31 * hashCode + Objects.hashCode(hasBlockDeviceMappings() ? blockDeviceMappings() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdAt());
hashCode = 31 * hashCode + Objects.hashCode(ebsOptimized());
hashCode = 31 * hashCode + Objects.hashCode(ec2InstanceId());
hashCode = 31 * hashCode + Objects.hashCode(ecsClusterArn());
hashCode = 31 * hashCode + Objects.hashCode(ecsContainerInstanceArn());
hashCode = 31 * hashCode + Objects.hashCode(elasticIp());
hashCode = 31 * hashCode + Objects.hashCode(hostname());
hashCode = 31 * hashCode + Objects.hashCode(infrastructureClass());
hashCode = 31 * hashCode + Objects.hashCode(installUpdatesOnBoot());
hashCode = 31 * hashCode + Objects.hashCode(instanceId());
hashCode = 31 * hashCode + Objects.hashCode(instanceProfileArn());
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(lastServiceErrorId());
hashCode = 31 * hashCode + Objects.hashCode(hasLayerIds() ? layerIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(os());
hashCode = 31 * hashCode + Objects.hashCode(platform());
hashCode = 31 * hashCode + Objects.hashCode(privateDns());
hashCode = 31 * hashCode + Objects.hashCode(privateIp());
hashCode = 31 * hashCode + Objects.hashCode(publicDns());
hashCode = 31 * hashCode + Objects.hashCode(publicIp());
hashCode = 31 * hashCode + Objects.hashCode(registeredBy());
hashCode = 31 * hashCode + Objects.hashCode(reportedAgentVersion());
hashCode = 31 * hashCode + Objects.hashCode(reportedOs());
hashCode = 31 * hashCode + Objects.hashCode(rootDeviceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(rootDeviceVolumeId());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroupIds() ? securityGroupIds() : null);
hashCode = 31 * hashCode + Objects.hashCode(sshHostDsaKeyFingerprint());
hashCode = 31 * hashCode + Objects.hashCode(sshHostRsaKeyFingerprint());
hashCode = 31 * hashCode + Objects.hashCode(sshKeyName());
hashCode = 31 * hashCode + Objects.hashCode(stackId());
hashCode = 31 * hashCode + Objects.hashCode(status());
hashCode = 31 * hashCode + Objects.hashCode(subnetId());
hashCode = 31 * hashCode + Objects.hashCode(tenancy());
hashCode = 31 * hashCode + Objects.hashCode(virtualizationTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Instance)) {
return false;
}
Instance other = (Instance) obj;
return Objects.equals(agentVersion(), other.agentVersion()) && Objects.equals(amiId(), other.amiId())
&& Objects.equals(architectureAsString(), other.architectureAsString()) && Objects.equals(arn(), other.arn())
&& Objects.equals(autoScalingTypeAsString(), other.autoScalingTypeAsString())
&& Objects.equals(availabilityZone(), other.availabilityZone())
&& hasBlockDeviceMappings() == other.hasBlockDeviceMappings()
&& Objects.equals(blockDeviceMappings(), other.blockDeviceMappings())
&& Objects.equals(createdAt(), other.createdAt()) && Objects.equals(ebsOptimized(), other.ebsOptimized())
&& Objects.equals(ec2InstanceId(), other.ec2InstanceId())
&& Objects.equals(ecsClusterArn(), other.ecsClusterArn())
&& Objects.equals(ecsContainerInstanceArn(), other.ecsContainerInstanceArn())
&& Objects.equals(elasticIp(), other.elasticIp()) && Objects.equals(hostname(), other.hostname())
&& Objects.equals(infrastructureClass(), other.infrastructureClass())
&& Objects.equals(installUpdatesOnBoot(), other.installUpdatesOnBoot())
&& Objects.equals(instanceId(), other.instanceId())
&& Objects.equals(instanceProfileArn(), other.instanceProfileArn())
&& Objects.equals(instanceType(), other.instanceType())
&& Objects.equals(lastServiceErrorId(), other.lastServiceErrorId()) && hasLayerIds() == other.hasLayerIds()
&& Objects.equals(layerIds(), other.layerIds()) && Objects.equals(os(), other.os())
&& Objects.equals(platform(), other.platform()) && Objects.equals(privateDns(), other.privateDns())
&& Objects.equals(privateIp(), other.privateIp()) && Objects.equals(publicDns(), other.publicDns())
&& Objects.equals(publicIp(), other.publicIp()) && Objects.equals(registeredBy(), other.registeredBy())
&& Objects.equals(reportedAgentVersion(), other.reportedAgentVersion())
&& Objects.equals(reportedOs(), other.reportedOs())
&& Objects.equals(rootDeviceTypeAsString(), other.rootDeviceTypeAsString())
&& Objects.equals(rootDeviceVolumeId(), other.rootDeviceVolumeId())
&& hasSecurityGroupIds() == other.hasSecurityGroupIds()
&& Objects.equals(securityGroupIds(), other.securityGroupIds())
&& Objects.equals(sshHostDsaKeyFingerprint(), other.sshHostDsaKeyFingerprint())
&& Objects.equals(sshHostRsaKeyFingerprint(), other.sshHostRsaKeyFingerprint())
&& Objects.equals(sshKeyName(), other.sshKeyName()) && Objects.equals(stackId(), other.stackId())
&& Objects.equals(status(), other.status()) && Objects.equals(subnetId(), other.subnetId())
&& Objects.equals(tenancy(), other.tenancy())
&& Objects.equals(virtualizationTypeAsString(), other.virtualizationTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Instance").add("AgentVersion", agentVersion()).add("AmiId", amiId())
.add("Architecture", architectureAsString()).add("Arn", arn()).add("AutoScalingType", autoScalingTypeAsString())
.add("AvailabilityZone", availabilityZone())
.add("BlockDeviceMappings", hasBlockDeviceMappings() ? blockDeviceMappings() : null)
.add("CreatedAt", createdAt()).add("EbsOptimized", ebsOptimized()).add("Ec2InstanceId", ec2InstanceId())
.add("EcsClusterArn", ecsClusterArn()).add("EcsContainerInstanceArn", ecsContainerInstanceArn())
.add("ElasticIp", elasticIp()).add("Hostname", hostname()).add("InfrastructureClass", infrastructureClass())
.add("InstallUpdatesOnBoot", installUpdatesOnBoot()).add("InstanceId", instanceId())
.add("InstanceProfileArn", instanceProfileArn()).add("InstanceType", instanceType())
.add("LastServiceErrorId", lastServiceErrorId()).add("LayerIds", hasLayerIds() ? layerIds() : null)
.add("Os", os()).add("Platform", platform()).add("PrivateDns", privateDns()).add("PrivateIp", privateIp())
.add("PublicDns", publicDns()).add("PublicIp", publicIp()).add("RegisteredBy", registeredBy())
.add("ReportedAgentVersion", reportedAgentVersion()).add("ReportedOs", reportedOs())
.add("RootDeviceType", rootDeviceTypeAsString()).add("RootDeviceVolumeId", rootDeviceVolumeId())
.add("SecurityGroupIds", hasSecurityGroupIds() ? securityGroupIds() : null)
.add("SshHostDsaKeyFingerprint", sshHostDsaKeyFingerprint())
.add("SshHostRsaKeyFingerprint", sshHostRsaKeyFingerprint()).add("SshKeyName", sshKeyName())
.add("StackId", stackId()).add("Status", status()).add("SubnetId", subnetId()).add("Tenancy", tenancy())
.add("VirtualizationType", virtualizationTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AgentVersion":
return Optional.ofNullable(clazz.cast(agentVersion()));
case "AmiId":
return Optional.ofNullable(clazz.cast(amiId()));
case "Architecture":
return Optional.ofNullable(clazz.cast(architectureAsString()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "AutoScalingType":
return Optional.ofNullable(clazz.cast(autoScalingTypeAsString()));
case "AvailabilityZone":
return Optional.ofNullable(clazz.cast(availabilityZone()));
case "BlockDeviceMappings":
return Optional.ofNullable(clazz.cast(blockDeviceMappings()));
case "CreatedAt":
return Optional.ofNullable(clazz.cast(createdAt()));
case "EbsOptimized":
return Optional.ofNullable(clazz.cast(ebsOptimized()));
case "Ec2InstanceId":
return Optional.ofNullable(clazz.cast(ec2InstanceId()));
case "EcsClusterArn":
return Optional.ofNullable(clazz.cast(ecsClusterArn()));
case "EcsContainerInstanceArn":
return Optional.ofNullable(clazz.cast(ecsContainerInstanceArn()));
case "ElasticIp":
return Optional.ofNullable(clazz.cast(elasticIp()));
case "Hostname":
return Optional.ofNullable(clazz.cast(hostname()));
case "InfrastructureClass":
return Optional.ofNullable(clazz.cast(infrastructureClass()));
case "InstallUpdatesOnBoot":
return Optional.ofNullable(clazz.cast(installUpdatesOnBoot()));
case "InstanceId":
return Optional.ofNullable(clazz.cast(instanceId()));
case "InstanceProfileArn":
return Optional.ofNullable(clazz.cast(instanceProfileArn()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "LastServiceErrorId":
return Optional.ofNullable(clazz.cast(lastServiceErrorId()));
case "LayerIds":
return Optional.ofNullable(clazz.cast(layerIds()));
case "Os":
return Optional.ofNullable(clazz.cast(os()));
case "Platform":
return Optional.ofNullable(clazz.cast(platform()));
case "PrivateDns":
return Optional.ofNullable(clazz.cast(privateDns()));
case "PrivateIp":
return Optional.ofNullable(clazz.cast(privateIp()));
case "PublicDns":
return Optional.ofNullable(clazz.cast(publicDns()));
case "PublicIp":
return Optional.ofNullable(clazz.cast(publicIp()));
case "RegisteredBy":
return Optional.ofNullable(clazz.cast(registeredBy()));
case "ReportedAgentVersion":
return Optional.ofNullable(clazz.cast(reportedAgentVersion()));
case "ReportedOs":
return Optional.ofNullable(clazz.cast(reportedOs()));
case "RootDeviceType":
return Optional.ofNullable(clazz.cast(rootDeviceTypeAsString()));
case "RootDeviceVolumeId":
return Optional.ofNullable(clazz.cast(rootDeviceVolumeId()));
case "SecurityGroupIds":
return Optional.ofNullable(clazz.cast(securityGroupIds()));
case "SshHostDsaKeyFingerprint":
return Optional.ofNullable(clazz.cast(sshHostDsaKeyFingerprint()));
case "SshHostRsaKeyFingerprint":
return Optional.ofNullable(clazz.cast(sshHostRsaKeyFingerprint()));
case "SshKeyName":
return Optional.ofNullable(clazz.cast(sshKeyName()));
case "StackId":
return Optional.ofNullable(clazz.cast(stackId()));
case "Status":
return Optional.ofNullable(clazz.cast(status()));
case "SubnetId":
return Optional.ofNullable(clazz.cast(subnetId()));
case "Tenancy":
return Optional.ofNullable(clazz.cast(tenancy()));
case "VirtualizationType":
return Optional.ofNullable(clazz.cast(virtualizationTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function