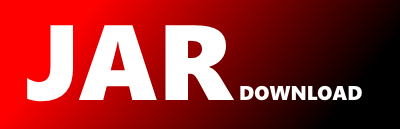
software.amazon.awssdk.services.organizations.DefaultOrganizationsAsyncClient Maven / Gradle / Ivy
Show all versions of organizations Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.organizations;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.ApiName;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.util.VersionInfo;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.organizations.model.AcceptHandshakeRequest;
import software.amazon.awssdk.services.organizations.model.AcceptHandshakeResponse;
import software.amazon.awssdk.services.organizations.model.AccessDeniedException;
import software.amazon.awssdk.services.organizations.model.AccessDeniedForDependencyException;
import software.amazon.awssdk.services.organizations.model.AccountAlreadyRegisteredException;
import software.amazon.awssdk.services.organizations.model.AccountNotFoundException;
import software.amazon.awssdk.services.organizations.model.AccountNotRegisteredException;
import software.amazon.awssdk.services.organizations.model.AccountOwnerNotVerifiedException;
import software.amazon.awssdk.services.organizations.model.AlreadyInOrganizationException;
import software.amazon.awssdk.services.organizations.model.AttachPolicyRequest;
import software.amazon.awssdk.services.organizations.model.AttachPolicyResponse;
import software.amazon.awssdk.services.organizations.model.AwsOrganizationsNotInUseException;
import software.amazon.awssdk.services.organizations.model.CancelHandshakeRequest;
import software.amazon.awssdk.services.organizations.model.CancelHandshakeResponse;
import software.amazon.awssdk.services.organizations.model.ChildNotFoundException;
import software.amazon.awssdk.services.organizations.model.ConcurrentModificationException;
import software.amazon.awssdk.services.organizations.model.ConstraintViolationException;
import software.amazon.awssdk.services.organizations.model.CreateAccountRequest;
import software.amazon.awssdk.services.organizations.model.CreateAccountResponse;
import software.amazon.awssdk.services.organizations.model.CreateAccountStatusNotFoundException;
import software.amazon.awssdk.services.organizations.model.CreateGovCloudAccountRequest;
import software.amazon.awssdk.services.organizations.model.CreateGovCloudAccountResponse;
import software.amazon.awssdk.services.organizations.model.CreateOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.CreateOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.CreateOrganizationalUnitRequest;
import software.amazon.awssdk.services.organizations.model.CreateOrganizationalUnitResponse;
import software.amazon.awssdk.services.organizations.model.CreatePolicyRequest;
import software.amazon.awssdk.services.organizations.model.CreatePolicyResponse;
import software.amazon.awssdk.services.organizations.model.DeclineHandshakeRequest;
import software.amazon.awssdk.services.organizations.model.DeclineHandshakeResponse;
import software.amazon.awssdk.services.organizations.model.DeleteOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.DeleteOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.DeleteOrganizationalUnitRequest;
import software.amazon.awssdk.services.organizations.model.DeleteOrganizationalUnitResponse;
import software.amazon.awssdk.services.organizations.model.DeletePolicyRequest;
import software.amazon.awssdk.services.organizations.model.DeletePolicyResponse;
import software.amazon.awssdk.services.organizations.model.DeregisterDelegatedAdministratorRequest;
import software.amazon.awssdk.services.organizations.model.DeregisterDelegatedAdministratorResponse;
import software.amazon.awssdk.services.organizations.model.DescribeAccountRequest;
import software.amazon.awssdk.services.organizations.model.DescribeAccountResponse;
import software.amazon.awssdk.services.organizations.model.DescribeCreateAccountStatusRequest;
import software.amazon.awssdk.services.organizations.model.DescribeCreateAccountStatusResponse;
import software.amazon.awssdk.services.organizations.model.DescribeEffectivePolicyRequest;
import software.amazon.awssdk.services.organizations.model.DescribeEffectivePolicyResponse;
import software.amazon.awssdk.services.organizations.model.DescribeHandshakeRequest;
import software.amazon.awssdk.services.organizations.model.DescribeHandshakeResponse;
import software.amazon.awssdk.services.organizations.model.DescribeOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.DescribeOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.DescribeOrganizationalUnitRequest;
import software.amazon.awssdk.services.organizations.model.DescribeOrganizationalUnitResponse;
import software.amazon.awssdk.services.organizations.model.DescribePolicyRequest;
import software.amazon.awssdk.services.organizations.model.DescribePolicyResponse;
import software.amazon.awssdk.services.organizations.model.DestinationParentNotFoundException;
import software.amazon.awssdk.services.organizations.model.DetachPolicyRequest;
import software.amazon.awssdk.services.organizations.model.DetachPolicyResponse;
import software.amazon.awssdk.services.organizations.model.DisableAWSServiceAccessResponse;
import software.amazon.awssdk.services.organizations.model.DisableAwsServiceAccessRequest;
import software.amazon.awssdk.services.organizations.model.DisablePolicyTypeRequest;
import software.amazon.awssdk.services.organizations.model.DisablePolicyTypeResponse;
import software.amazon.awssdk.services.organizations.model.DuplicateAccountException;
import software.amazon.awssdk.services.organizations.model.DuplicateHandshakeException;
import software.amazon.awssdk.services.organizations.model.DuplicateOrganizationalUnitException;
import software.amazon.awssdk.services.organizations.model.DuplicatePolicyAttachmentException;
import software.amazon.awssdk.services.organizations.model.DuplicatePolicyException;
import software.amazon.awssdk.services.organizations.model.EffectivePolicyNotFoundException;
import software.amazon.awssdk.services.organizations.model.EnableAWSServiceAccessResponse;
import software.amazon.awssdk.services.organizations.model.EnableAllFeaturesRequest;
import software.amazon.awssdk.services.organizations.model.EnableAllFeaturesResponse;
import software.amazon.awssdk.services.organizations.model.EnableAwsServiceAccessRequest;
import software.amazon.awssdk.services.organizations.model.EnablePolicyTypeRequest;
import software.amazon.awssdk.services.organizations.model.EnablePolicyTypeResponse;
import software.amazon.awssdk.services.organizations.model.FinalizingOrganizationException;
import software.amazon.awssdk.services.organizations.model.HandshakeAlreadyInStateException;
import software.amazon.awssdk.services.organizations.model.HandshakeConstraintViolationException;
import software.amazon.awssdk.services.organizations.model.HandshakeNotFoundException;
import software.amazon.awssdk.services.organizations.model.InvalidHandshakeTransitionException;
import software.amazon.awssdk.services.organizations.model.InvalidInputException;
import software.amazon.awssdk.services.organizations.model.InviteAccountToOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.InviteAccountToOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.LeaveOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.LeaveOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.ListAccountsForParentRequest;
import software.amazon.awssdk.services.organizations.model.ListAccountsForParentResponse;
import software.amazon.awssdk.services.organizations.model.ListAccountsRequest;
import software.amazon.awssdk.services.organizations.model.ListAccountsResponse;
import software.amazon.awssdk.services.organizations.model.ListAwsServiceAccessForOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.ListAwsServiceAccessForOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.ListChildrenRequest;
import software.amazon.awssdk.services.organizations.model.ListChildrenResponse;
import software.amazon.awssdk.services.organizations.model.ListCreateAccountStatusRequest;
import software.amazon.awssdk.services.organizations.model.ListCreateAccountStatusResponse;
import software.amazon.awssdk.services.organizations.model.ListDelegatedAdministratorsRequest;
import software.amazon.awssdk.services.organizations.model.ListDelegatedAdministratorsResponse;
import software.amazon.awssdk.services.organizations.model.ListDelegatedServicesForAccountRequest;
import software.amazon.awssdk.services.organizations.model.ListDelegatedServicesForAccountResponse;
import software.amazon.awssdk.services.organizations.model.ListHandshakesForAccountRequest;
import software.amazon.awssdk.services.organizations.model.ListHandshakesForAccountResponse;
import software.amazon.awssdk.services.organizations.model.ListHandshakesForOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.ListHandshakesForOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.ListOrganizationalUnitsForParentRequest;
import software.amazon.awssdk.services.organizations.model.ListOrganizationalUnitsForParentResponse;
import software.amazon.awssdk.services.organizations.model.ListParentsRequest;
import software.amazon.awssdk.services.organizations.model.ListParentsResponse;
import software.amazon.awssdk.services.organizations.model.ListPoliciesForTargetRequest;
import software.amazon.awssdk.services.organizations.model.ListPoliciesForTargetResponse;
import software.amazon.awssdk.services.organizations.model.ListPoliciesRequest;
import software.amazon.awssdk.services.organizations.model.ListPoliciesResponse;
import software.amazon.awssdk.services.organizations.model.ListRootsRequest;
import software.amazon.awssdk.services.organizations.model.ListRootsResponse;
import software.amazon.awssdk.services.organizations.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.organizations.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.organizations.model.ListTargetsForPolicyRequest;
import software.amazon.awssdk.services.organizations.model.ListTargetsForPolicyResponse;
import software.amazon.awssdk.services.organizations.model.MalformedPolicyDocumentException;
import software.amazon.awssdk.services.organizations.model.MasterCannotLeaveOrganizationException;
import software.amazon.awssdk.services.organizations.model.MoveAccountRequest;
import software.amazon.awssdk.services.organizations.model.MoveAccountResponse;
import software.amazon.awssdk.services.organizations.model.OrganizationNotEmptyException;
import software.amazon.awssdk.services.organizations.model.OrganizationalUnitNotEmptyException;
import software.amazon.awssdk.services.organizations.model.OrganizationalUnitNotFoundException;
import software.amazon.awssdk.services.organizations.model.OrganizationsException;
import software.amazon.awssdk.services.organizations.model.OrganizationsRequest;
import software.amazon.awssdk.services.organizations.model.ParentNotFoundException;
import software.amazon.awssdk.services.organizations.model.PolicyChangesInProgressException;
import software.amazon.awssdk.services.organizations.model.PolicyInUseException;
import software.amazon.awssdk.services.organizations.model.PolicyNotAttachedException;
import software.amazon.awssdk.services.organizations.model.PolicyNotFoundException;
import software.amazon.awssdk.services.organizations.model.PolicyTypeAlreadyEnabledException;
import software.amazon.awssdk.services.organizations.model.PolicyTypeNotAvailableForOrganizationException;
import software.amazon.awssdk.services.organizations.model.PolicyTypeNotEnabledException;
import software.amazon.awssdk.services.organizations.model.RegisterDelegatedAdministratorRequest;
import software.amazon.awssdk.services.organizations.model.RegisterDelegatedAdministratorResponse;
import software.amazon.awssdk.services.organizations.model.RemoveAccountFromOrganizationRequest;
import software.amazon.awssdk.services.organizations.model.RemoveAccountFromOrganizationResponse;
import software.amazon.awssdk.services.organizations.model.RootNotFoundException;
import software.amazon.awssdk.services.organizations.model.ServiceException;
import software.amazon.awssdk.services.organizations.model.SourceParentNotFoundException;
import software.amazon.awssdk.services.organizations.model.TagResourceRequest;
import software.amazon.awssdk.services.organizations.model.TagResourceResponse;
import software.amazon.awssdk.services.organizations.model.TargetNotFoundException;
import software.amazon.awssdk.services.organizations.model.TooManyRequestsException;
import software.amazon.awssdk.services.organizations.model.UnsupportedApiEndpointException;
import software.amazon.awssdk.services.organizations.model.UntagResourceRequest;
import software.amazon.awssdk.services.organizations.model.UntagResourceResponse;
import software.amazon.awssdk.services.organizations.model.UpdateOrganizationalUnitRequest;
import software.amazon.awssdk.services.organizations.model.UpdateOrganizationalUnitResponse;
import software.amazon.awssdk.services.organizations.model.UpdatePolicyRequest;
import software.amazon.awssdk.services.organizations.model.UpdatePolicyResponse;
import software.amazon.awssdk.services.organizations.paginators.ListAWSServiceAccessForOrganizationPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListAccountsForParentPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListAccountsPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListChildrenPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListCreateAccountStatusPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListDelegatedAdministratorsPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListDelegatedServicesForAccountPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListHandshakesForAccountPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListHandshakesForOrganizationPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListOrganizationalUnitsForParentPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListParentsPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListPoliciesForTargetPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListPoliciesPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListRootsPublisher;
import software.amazon.awssdk.services.organizations.paginators.ListTagsForResourcePublisher;
import software.amazon.awssdk.services.organizations.paginators.ListTargetsForPolicyPublisher;
import software.amazon.awssdk.services.organizations.transform.AcceptHandshakeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.AttachPolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CancelHandshakeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CreateAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CreateGovCloudAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CreateOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CreateOrganizationalUnitRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.CreatePolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DeclineHandshakeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DeleteOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DeleteOrganizationalUnitRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DeletePolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DeregisterDelegatedAdministratorRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeCreateAccountStatusRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeEffectivePolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeHandshakeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribeOrganizationalUnitRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DescribePolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DetachPolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DisableAwsServiceAccessRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.DisablePolicyTypeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.EnableAllFeaturesRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.EnableAwsServiceAccessRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.EnablePolicyTypeRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.InviteAccountToOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.LeaveOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListAccountsForParentRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListAccountsRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListAwsServiceAccessForOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListChildrenRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListCreateAccountStatusRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListDelegatedAdministratorsRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListDelegatedServicesForAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListHandshakesForAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListHandshakesForOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListOrganizationalUnitsForParentRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListParentsRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListPoliciesForTargetRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListPoliciesRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListRootsRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.ListTargetsForPolicyRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.MoveAccountRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.RegisterDelegatedAdministratorRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.RemoveAccountFromOrganizationRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.UpdateOrganizationalUnitRequestMarshaller;
import software.amazon.awssdk.services.organizations.transform.UpdatePolicyRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link OrganizationsAsyncClient}.
*
* @see OrganizationsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultOrganizationsAsyncClient implements OrganizationsAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultOrganizationsAsyncClient.class);
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultOrganizationsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Sends a response to the originator of a handshake agreeing to the action proposed by the handshake request.
*
*
* This operation can be called only by the following principals when they also have the relevant IAM permissions:
*
*
* -
*
* Invitation to join or Approve all features request handshakes: only a principal from the member
* account.
*
*
* The user who calls the API for an invitation to join must have the organizations:AcceptHandshake
* permission. If you enabled all features in the organization, the user must also have the
* iam:CreateServiceLinkedRole
permission so that AWS Organizations can create the required
* service-linked role named AWSServiceRoleForOrganizations
. For more information, see AWS Organizations and Service-Linked Roles in the AWS Organizations User Guide.
*
*
* -
*
* Enable all features final confirmation handshake: only a principal from the master account.
*
*
* For more information about invitations, see Inviting an
* AWS Account to Join Your Organization in the AWS Organizations User Guide. For more information about
* requests to enable all features in the organization, see Enabling All Features in Your Organization in the AWS Organizations User Guide.
*
*
*
*
* After you accept a handshake, it continues to appear in the results of relevant APIs for only 30 days. After
* that, it's deleted.
*
*
* @param acceptHandshakeRequest
* @return A Java Future containing the result of the AcceptHandshake operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - HandshakeConstraintViolationException The requested operation would violate the constraint identified
* in the reason code.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. Note that deleted and closed accounts still count toward your limit.
*
*
*
* If you get this exception immediately after creating the organization, wait one hour and try again. If
* after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* ALREADY_IN_AN_ORGANIZATION: The handshake request is invalid because the invited account is already a
* member of an organization.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* INVITE_DISABLED_DURING_ENABLE_ALL_FEATURES: You can't issue new invitations to join an organization while
* it's in the process of enabling all features. You can resume inviting accounts after you finalize the
* process when all accounts have agreed to the change.
*
*
* -
*
* ORGANIZATION_ALREADY_HAS_ALL_FEATURES: The handshake request is invalid because the organization has
* already enabled all features.
*
*
* -
*
* ORGANIZATION_FROM_DIFFERENT_SELLER_OF_RECORD: The request failed because the account is from a different
* marketplace than the accounts in the organization. For example, accounts with India addresses must be
* associated with the AISPL marketplace. All accounts in an organization must be from the same marketplace.
*
*
* -
*
* ORGANIZATION_MEMBERSHIP_CHANGE_RATE_LIMIT_EXCEEDED: You attempted to change the membership of an account
* too quickly after its previous change.
*
*
* -
*
* PAYMENT_INSTRUMENT_REQUIRED: You can't complete the operation with an account that doesn't have a payment
* instrument, such as a credit card, associated with it.
*
*
* - HandshakeNotFoundException We can't find a handshake with the
HandshakeId
that you
* specified.
* - InvalidHandshakeTransitionException You can't perform the operation on the handshake in its current
* state. For example, you can't cancel a handshake that was already accepted or accept a handshake that was
* already declined.
* - HandshakeAlreadyInStateException The specified handshake is already in the requested state. For
* example, you can't accept a handshake that was already accepted.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - AccessDeniedForDependencyException The operation that you attempted requires you to have the
*
iam:CreateServiceLinkedRole
for organizations.amazonaws.com
permission so that
* AWS Organizations can create the required service-linked role. You don't have that permission.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.AcceptHandshake
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture acceptHandshake(AcceptHandshakeRequest acceptHandshakeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AcceptHandshakeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AcceptHandshake")
.withMarshaller(new AcceptHandshakeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(acceptHandshakeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Attaches a policy to a root, an organizational unit (OU), or an individual account. How the policy affects
* accounts depends on the type of policy:
*
*
* -
*
* Service control policy (SCP) - An SCP specifies what permissions can be delegated to users in affected
* member accounts. The scope of influence for a policy depends on what you attach the policy to:
*
*
* -
*
* If you attach an SCP to a root, it affects all accounts in the organization.
*
*
* -
*
* If you attach an SCP to an OU, it affects all accounts in that OU and in any child OUs.
*
*
* -
*
* If you attach the policy directly to an account, it affects only that account.
*
*
*
*
* SCPs are JSON policies that specify the maximum permissions for an organization or organizational unit (OU). You
* can attach one SCP to a higher level root or OU, and a different SCP to a child OU or to an account. The child
* policy can further restrict only the permissions that pass through the parent filter and are available to the
* child. An SCP that is attached to a child can't grant a permission that the parent hasn't already granted. For
* example, imagine that the parent SCP allows permissions A, B, C, D, and E. The child SCP allows C, D, E, F, and
* G. The result is that the accounts affected by the child SCP are allowed to use only C, D, and E. They can't use
* A or B because the child OU filtered them out. They also can't use F and G because the parent OU filtered them
* out. They can't be granted back by the child SCP; child SCPs can only filter the permissions they receive from
* the parent SCP.
*
*
* AWS Organizations attaches a default SCP named "FullAWSAccess
to every root, OU, and account. This
* default SCP allows all services and actions, enabling any new child OU or account to inherit the permissions of
* the parent root or OU. If you detach the default policy, you must replace it with a policy that specifies the
* permissions that you want to allow in that OU or account.
*
*
* For more information about how AWS Organizations policies permissions work, see Using Service
* Control Policies in the AWS Organizations User Guide.
*
*
*
*
* This operation can be called only from the organization's master account.
*
*
* @param attachPolicyRequest
* @return A Java Future containing the result of the AttachPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - DuplicatePolicyAttachmentException The selected policy is already attached to the specified target.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - PolicyTypeNotEnabledException The specified policy type isn't currently enabled in this root. You
* can't attach policies of the specified type to entities in a root until you enable that type in the root.
* For more information, see Enabling All Features in Your Organization in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - PolicyChangesInProgressException Changes to the effective policy are in progress, and its contents
* can't be returned. Try the operation again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.AttachPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture attachPolicy(AttachPolicyRequest attachPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
AttachPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("AttachPolicy").withMarshaller(new AttachPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(attachPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels a handshake. Canceling a handshake sets the handshake state to CANCELED
.
*
*
* This operation can be called only from the account that originated the handshake. The recipient of the handshake
* can't cancel it, but can use DeclineHandshake instead. After a handshake is canceled, the recipient can no
* longer respond to that handshake.
*
*
* After you cancel a handshake, it continues to appear in the results of relevant APIs for only 30 days. After
* that, it's deleted.
*
*
* @param cancelHandshakeRequest
* @return A Java Future containing the result of the CancelHandshake operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - HandshakeNotFoundException We can't find a handshake with the
HandshakeId
that you
* specified.
* - InvalidHandshakeTransitionException You can't perform the operation on the handshake in its current
* state. For example, you can't cancel a handshake that was already accepted or accept a handshake that was
* already declined.
* - HandshakeAlreadyInStateException The specified handshake is already in the requested state. For
* example, you can't accept a handshake that was already accepted.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CancelHandshake
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelHandshake(CancelHandshakeRequest cancelHandshakeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelHandshakeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelHandshake")
.withMarshaller(new CancelHandshakeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(cancelHandshakeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an AWS account that is automatically a member of the organization whose credentials made the request.
* This is an asynchronous request that AWS performs in the background. Because CreateAccount
operates
* asynchronously, it can return a successful completion message even though account initialization might still be
* in progress. You might need to wait a few minutes before you can successfully access the account. To check the
* status of the request, do one of the following:
*
*
* -
*
* Use the OperationId
response element from this operation to provide as a parameter to the
* DescribeCreateAccountStatus operation.
*
*
* -
*
* Check the AWS CloudTrail log for the CreateAccountResult
event. For information on using AWS
* CloudTrail with AWS Organizations, see Monitoring the Activity in
* Your Organization in the AWS Organizations User Guide.
*
*
*
*
*
* The user who calls the API to create an account must have the organizations:CreateAccount
* permission. If you enabled all features in the organization, AWS Organizations creates the required
* service-linked role named AWSServiceRoleForOrganizations
. For more information, see AWS Organizations and Service-Linked Roles in the AWS Organizations User Guide.
*
*
* AWS Organizations preconfigures the new member account with a role (named
* OrganizationAccountAccessRole
by default) that grants users in the master account administrator
* permissions in the new member account. Principals in the master account can assume the role. AWS Organizations
* clones the company name and address information for the new account from the organization's master account.
*
*
* This operation can be called only from the organization's master account.
*
*
* For more information about creating accounts, see Creating an
* AWS Account in Your Organization in the AWS Organizations User Guide.
*
*
*
* -
*
* When you create an account in an organization using the AWS Organizations console, API, or CLI commands, the
* information required for the account to operate as a standalone account, such as a payment method and signing the
* end user license agreement (EULA) is not automatically collected. If you must remove an account from your
* organization later, you can do so only after you provide the missing information. Follow the steps at To leave an organization as a member account in the AWS Organizations User Guide.
*
*
* -
*
* If you get an exception that indicates that you exceeded your account limits for the organization, contact AWS Support.
*
*
* -
*
* If you get an exception that indicates that the operation failed because your organization is still initializing,
* wait one hour and then try again. If the error persists, contact AWS Support.
*
*
* -
*
* Using CreateAccount
to create multiple temporary accounts isn't recommended. You can only close an
* account from the Billing and Cost Management Console, and you must be signed in as the root user. For information
* on the requirements and process for closing an account, see Closing an AWS
* Account in the AWS Organizations User Guide.
*
*
*
*
*
* When you create a member account with this operation, you can choose whether to create the account with the
* IAM User and Role Access to Billing Information switch enabled. If you enable it, IAM users and roles that
* have appropriate permissions can view billing information for the account. If you disable it, only the account
* root user can access billing information. For information about how to disable this switch for an account, see Granting Access to Your
* Billing Information and Tools.
*
*
*
* @param createAccountRequest
* @return A Java Future containing the result of the CreateAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - FinalizingOrganizationException AWS Organizations couldn't perform the operation because your
* organization hasn't finished initializing. This can take up to an hour. Try again later. If after one
* hour you continue to receive this error, contact AWS Support.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CreateAccount
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture createAccount(CreateAccountRequest createAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateAccount")
.withMarshaller(new CreateAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* This action is available if all of the following are true:
*
*
* -
*
* You're authorized to create accounts in the AWS GovCloud (US) Region. For more information on the AWS GovCloud
* (US) Region, see the AWS
* GovCloud User Guide.
*
*
* -
*
* You already have an account in the AWS GovCloud (US) Region that is associated with your master account in the
* commercial Region.
*
*
* -
*
* You call this action from the master account of your organization in the commercial Region.
*
*
* -
*
* You have the organizations:CreateGovCloudAccount
permission. AWS Organizations creates the required
* service-linked role named AWSServiceRoleForOrganizations
. For more information, see AWS Organizations and Service-Linked Roles in the AWS Organizations User Guide.
*
*
*
*
* AWS automatically enables AWS CloudTrail for AWS GovCloud (US) accounts, but you should also do the following:
*
*
* -
*
* Verify that AWS CloudTrail is enabled to store logs.
*
*
* -
*
* Create an S3 bucket for AWS CloudTrail log storage.
*
*
* For more information, see Verifying AWS CloudTrail
* Is Enabled in the AWS GovCloud User Guide.
*
*
*
*
* You call this action from the master account of your organization in the commercial Region to create a standalone
* AWS account in the AWS GovCloud (US) Region. After the account is created, the master account of an organization
* in the AWS GovCloud (US) Region can invite it to that organization. For more information on inviting standalone
* accounts in the AWS GovCloud (US) to join an organization, see AWS Organizations
* in the AWS GovCloud User Guide.
*
*
* Calling CreateGovCloudAccount
is an asynchronous request that AWS performs in the background.
* Because CreateGovCloudAccount
operates asynchronously, it can return a successful completion message
* even though account initialization might still be in progress. You might need to wait a few minutes before you
* can successfully access the account. To check the status of the request, do one of the following:
*
*
* -
*
* Use the OperationId
response element from this operation to provide as a parameter to the
* DescribeCreateAccountStatus operation.
*
*
* -
*
* Check the AWS CloudTrail log for the CreateAccountResult
event. For information on using AWS
* CloudTrail with Organizations, see Monitoring the Activity in
* Your Organization in the AWS Organizations User Guide.
*
*
*
*
*
* When you call the CreateGovCloudAccount
action, you create two accounts: a standalone account in the
* AWS GovCloud (US) Region and an associated account in the commercial Region for billing and support purposes. The
* account in the commercial Region is automatically a member of the organization whose credentials made the
* request. Both accounts are associated with the same email address.
*
*
* A role is created in the new account in the commercial Region that allows the master account in the organization
* in the commercial Region to assume it. An AWS GovCloud (US) account is then created and associated with the
* commercial account that you just created. A role is created in the new AWS GovCloud (US) account that can be
* assumed by the AWS GovCloud (US) account that is associated with the master account of the commercial
* organization. For more information and to view a diagram that explains how account access works, see AWS Organizations
* in the AWS GovCloud User Guide.
*
*
* For more information about creating accounts, see Creating an
* AWS Account in Your Organization in the AWS Organizations User Guide.
*
*
*
* -
*
* When you create an account in an organization using the AWS Organizations console, API, or CLI commands, the
* information required for the account to operate as a standalone account, such as a payment method and signing the
* end user license agreement (EULA) is not automatically collected. If you must remove an account from your
* organization later, you can do so only after you provide the missing information. Follow the steps at To leave an organization as a member account in the AWS Organizations User Guide.
*
*
* -
*
* If you get an exception that indicates that you exceeded your account limits for the organization, contact AWS Support.
*
*
* -
*
* If you get an exception that indicates that the operation failed because your organization is still initializing,
* wait one hour and then try again. If the error persists, contact AWS Support.
*
*
* -
*
* Using CreateGovCloudAccount
to create multiple temporary accounts isn't recommended. You can only
* close an account from the AWS Billing and Cost Management console, and you must be signed in as the root user.
* For information on the requirements and process for closing an account, see Closing an AWS
* Account in the AWS Organizations User Guide.
*
*
*
*
*
* When you create a member account with this operation, you can choose whether to create the account with the
* IAM User and Role Access to Billing Information switch enabled. If you enable it, IAM users and roles that
* have appropriate permissions can view billing information for the account. If you disable it, only the account
* root user can access billing information. For information about how to disable this switch for an account, see Granting Access to Your
* Billing Information and Tools.
*
*
*
* @param createGovCloudAccountRequest
* @return A Java Future containing the result of the CreateGovCloudAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - FinalizingOrganizationException AWS Organizations couldn't perform the operation because your
* organization hasn't finished initializing. This can take up to an hour. Try again later. If after one
* hour you continue to receive this error, contact AWS Support.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CreateGovCloudAccount
* @see AWS API Documentation
*/
@Override
public CompletableFuture createGovCloudAccount(
CreateGovCloudAccountRequest createGovCloudAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateGovCloudAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateGovCloudAccount")
.withMarshaller(new CreateGovCloudAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createGovCloudAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an AWS organization. The account whose user is calling the CreateOrganization
operation
* automatically becomes the master
* account of the new organization.
*
*
* This operation must be called using credentials from the account that is to become the new organization's master
* account. The principal must also have the relevant IAM permissions.
*
*
* By default (or if you set the FeatureSet
parameter to ALL
), the new organization is
* created with all features enabled and service control policies automatically enabled in the root. If you instead
* choose to create the organization supporting only the consolidated billing features by setting the
* FeatureSet
parameter to CONSOLIDATED_BILLING"
, no policy types are enabled by default,
* and you can't use organization policies
*
*
* @param createOrganizationRequest
* @return A Java Future containing the result of the CreateOrganization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AlreadyInOrganizationException This account is already a member of an organization. An account can
* belong to only one organization at a time.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - AccessDeniedForDependencyException The operation that you attempted requires you to have the
*
iam:CreateServiceLinkedRole
for organizations.amazonaws.com
permission so that
* AWS Organizations can create the required service-linked role. You don't have that permission.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CreateOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture createOrganization(CreateOrganizationRequest createOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOrganization")
.withMarshaller(new CreateOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an organizational unit (OU) within a root or parent OU. An OU is a container for accounts that enables
* you to organize your accounts to apply policies according to your business requirements. The number of levels
* deep that you can nest OUs is dependent upon the policy types enabled for that root. For service control
* policies, the limit is five.
*
*
* For more information about OUs, see Managing Organizational
* Units in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param createOrganizationalUnitRequest
* @return A Java Future containing the result of the CreateOrganizationalUnit operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - DuplicateOrganizationalUnitException An OU with the same name already exists.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CreateOrganizationalUnit
* @see AWS API Documentation
*/
@Override
public CompletableFuture createOrganizationalUnit(
CreateOrganizationalUnitRequest createOrganizationalUnitRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateOrganizationalUnitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOrganizationalUnit")
.withMarshaller(new CreateOrganizationalUnitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createOrganizationalUnitRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a policy of a specified type that you can attach to a root, an organizational unit (OU), or an individual
* AWS account.
*
*
* For more information about policies and their use, see Managing Organization
* Policies.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param createPolicyRequest
* @return A Java Future containing the result of the CreatePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - DuplicatePolicyException A policy with the same name already exists.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - MalformedPolicyDocumentException The provided policy document doesn't meet the requirements of the
* specified policy type. For example, the syntax might be incorrect. For details about service control
* policy syntax, see Service
* Control Policy Syntax in the AWS Organizations User Guide.
* - PolicyTypeNotAvailableForOrganizationException You can't use the specified policy type with the
* feature set currently enabled for this organization. For example, you can enable SCPs only after you
* enable all features in the organization. For more information, see Enabling and Disabling a Policy Type on a Root in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.CreatePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createPolicy(CreatePolicyRequest createPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreatePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreatePolicy").withMarshaller(new CreatePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(createPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Declines a handshake request. This sets the handshake state to DECLINED
and effectively deactivates
* the request.
*
*
* This operation can be called only from the account that received the handshake. The originator of the handshake
* can use CancelHandshake instead. The originator can't reactivate a declined request, but can reinitiate
* the process with a new handshake request.
*
*
* After you decline a handshake, it continues to appear in the results of relevant APIs for only 30 days. After
* that, it's deleted.
*
*
* @param declineHandshakeRequest
* @return A Java Future containing the result of the DeclineHandshake operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - HandshakeNotFoundException We can't find a handshake with the
HandshakeId
that you
* specified.
* - InvalidHandshakeTransitionException You can't perform the operation on the handshake in its current
* state. For example, you can't cancel a handshake that was already accepted or accept a handshake that was
* already declined.
* - HandshakeAlreadyInStateException The specified handshake is already in the requested state. For
* example, you can't accept a handshake that was already accepted.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DeclineHandshake
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture declineHandshake(DeclineHandshakeRequest declineHandshakeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeclineHandshakeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeclineHandshake")
.withMarshaller(new DeclineHandshakeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(declineHandshakeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the organization. You can delete an organization only by using credentials from the master account. The
* organization must be empty of member accounts.
*
*
* @param deleteOrganizationRequest
* @return A Java Future containing the result of the DeleteOrganization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - OrganizationNotEmptyException The organization isn't empty. To delete an organization, you must first
* remove all accounts except the master account, delete all OUs, and delete all policies.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DeleteOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteOrganization(DeleteOrganizationRequest deleteOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOrganization")
.withMarshaller(new DeleteOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes an organizational unit (OU) from a root or another OU. You must first remove all accounts and child OUs
* from the OU that you want to delete.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param deleteOrganizationalUnitRequest
* @return A Java Future containing the result of the DeleteOrganizationalUnit operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - OrganizationalUnitNotEmptyException The specified OU is not empty. Move all accounts to another root
* or to other OUs, remove all child OUs, and try the operation again.
* - OrganizationalUnitNotFoundException We can't find an OU with the
OrganizationalUnitId
* that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DeleteOrganizationalUnit
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteOrganizationalUnit(
DeleteOrganizationalUnitRequest deleteOrganizationalUnitRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteOrganizationalUnitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOrganizationalUnit")
.withMarshaller(new DeleteOrganizationalUnitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deleteOrganizationalUnitRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified policy from your organization. Before you perform this operation, you must first detach the
* policy from all organizational units (OUs), roots, and accounts.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param deletePolicyRequest
* @return A Java Future containing the result of the DeletePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyInUseException The policy is attached to one or more entities. You must detach it from all
* roots, OUs, and accounts before performing this operation.
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DeletePolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deletePolicy(DeletePolicyRequest deletePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeletePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeletePolicy").withMarshaller(new DeletePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deletePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified member AWS account as a delegated administrator for the specified AWS service.
*
*
* You can run this action only for AWS services that support this feature. For a current list of services that
* support it, see the column Supports Delegated Administrator in the table at AWS Services
* that you can use with AWS Organizations in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param deregisterDelegatedAdministratorRequest
* @return A Java Future containing the result of the DeregisterDelegatedAdministrator operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AccountNotRegisteredException The specified account is not a delegated administrator for this AWS
* service.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DeregisterDelegatedAdministrator
* @see AWS API Documentation
*/
@Override
public CompletableFuture deregisterDelegatedAdministrator(
DeregisterDelegatedAdministratorRequest deregisterDelegatedAdministratorRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, DeregisterDelegatedAdministratorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeregisterDelegatedAdministrator")
.withMarshaller(new DeregisterDelegatedAdministratorRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(deregisterDelegatedAdministratorRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves AWS Organizations-related information about the specified account.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param describeAccountRequest
* @return A Java Future containing the result of the DescribeAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeAccount
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describeAccount(DescribeAccountRequest describeAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeAccount")
.withMarshaller(new DescribeAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves the current status of an asynchronous request to create an account.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param describeCreateAccountStatusRequest
* @return A Java Future containing the result of the DescribeCreateAccountStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - CreateAccountStatusNotFoundException We can't find an create account request with the
*
CreateAccountRequestId
that you specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeCreateAccountStatus
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeCreateAccountStatus(
DescribeCreateAccountStatusRequest describeCreateAccountStatusRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeCreateAccountStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeCreateAccountStatus")
.withMarshaller(new DescribeCreateAccountStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeCreateAccountStatusRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns the contents of the effective tag policy for the account. The effective tag policy is the aggregation of
* any tag policies the account inherits, plus any policy directly that is attached to the account.
*
*
* This action returns information on tag policies only.
*
*
* For more information on policy inheritance, see How Policy
* Inheritance Works in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param describeEffectivePolicyRequest
* @return A Java Future containing the result of the DescribeEffectivePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - EffectivePolicyNotFoundException If you ran this action on the master account, this policy type is
* not enabled. If you ran the action on a member account, the account doesn't have an effective policy of
* this type. Contact the administrator of your organization about attaching a policy of this type to the
* account.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeEffectivePolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeEffectivePolicy(
DescribeEffectivePolicyRequest describeEffectivePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeEffectivePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeEffectivePolicy")
.withMarshaller(new DescribeEffectivePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeEffectivePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about a previously requested handshake. The handshake ID comes from the response to the
* original InviteAccountToOrganization operation that generated the handshake.
*
*
* You can access handshakes that are ACCEPTED
, DECLINED
, or CANCELED
for
* only 30 days after they change to that state. They're then deleted and no longer accessible.
*
*
* This operation can be called from any account in the organization.
*
*
* @param describeHandshakeRequest
* @return A Java Future containing the result of the DescribeHandshake operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - HandshakeNotFoundException We can't find a handshake with the
HandshakeId
that you
* specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeHandshake
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeHandshake(DescribeHandshakeRequest describeHandshakeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeHandshakeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeHandshake")
.withMarshaller(new DescribeHandshakeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeHandshakeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about the organization that the user's account belongs to.
*
*
* This operation can be called from any account in the organization.
*
*
*
* Even if a policy type is shown as available in the organization, you can disable it separately at the root level
* with DisablePolicyType. Use ListRoots to see the status of policy types for a specified root.
*
*
*
* @param describeOrganizationRequest
* @return A Java Future containing the result of the DescribeOrganization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeOrganization(
DescribeOrganizationRequest describeOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOrganization")
.withMarshaller(new DescribeOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about an organizational unit (OU).
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param describeOrganizationalUnitRequest
* @return A Java Future containing the result of the DescribeOrganizationalUnit operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - OrganizationalUnitNotFoundException We can't find an OU with the
OrganizationalUnitId
* that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribeOrganizationalUnit
* @see AWS API Documentation
*/
@Override
public CompletableFuture describeOrganizationalUnit(
DescribeOrganizationalUnitRequest describeOrganizationalUnitRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribeOrganizationalUnitResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribeOrganizationalUnit")
.withMarshaller(new DescribeOrganizationalUnitRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describeOrganizationalUnitRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Retrieves information about a policy.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param describePolicyRequest
* @return A Java Future containing the result of the DescribePolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DescribePolicy
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture describePolicy(DescribePolicyRequest describePolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DescribePolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DescribePolicy")
.withMarshaller(new DescribePolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(describePolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Detaches a policy from a target root, organizational unit (OU), or account. If the policy being detached is a
* service control policy (SCP), the changes to permissions for IAM users and roles in affected accounts are
* immediate.
*
*
* Note: Every root, OU, and account must have at least one SCP attached. If you want to replace the default
* FullAWSAccess
policy with one that limits the permissions that can be delegated, you must attach the
* replacement policy before you can remove the default one. This is the authorization strategy of an
* "allow list". If you instead attach a second SCP and leave the
* FullAWSAccess
SCP still attached, and specify "Effect": "Deny"
in the second SCP to
* override the "Effect": "Allow"
in the FullAWSAccess
policy (or any other attached SCP),
* you're using the authorization strategy of a
* "deny list".
*
*
* This operation can be called only from the organization's master account.
*
*
* @param detachPolicyRequest
* @return A Java Future containing the result of the DetachPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyNotAttachedException The policy isn't attached to the specified target in the specified root.
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - PolicyChangesInProgressException Changes to the effective policy are in progress, and its contents
* can't be returned. Try the operation again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DetachPolicy
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture detachPolicy(DetachPolicyRequest detachPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DetachPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DetachPolicy").withMarshaller(new DetachPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(detachPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables the integration of an AWS service (the service that is specified by ServicePrincipal
) with
* AWS Organizations. When you disable integration, the specified service no longer can create a service-linked role in
* new accounts in your organization. This means the service can't perform operations on your behalf on any
* new accounts in your organization. The service can still perform operations in older accounts until the service
* completes its clean-up from AWS Organizations.
*
*
*
*
* We recommend that you disable integration between AWS Organizations and the specified AWS service by using the
* console or commands that are provided by the specified service. Doing so ensures that the other service is aware
* that it can clean up any resources that are required only for the integration. How the service cleans up its
* resources in the organization's accounts depends on that service. For more information, see the documentation for
* the other AWS service.
*
*
*
* After you perform the DisableAWSServiceAccess
operation, the specified service can no longer perform
* operations in your organization's accounts unless the operations are explicitly permitted by the IAM policies
* that are attached to your roles.
*
*
* For more information about integrating other services with AWS Organizations, including the list of services that
* work with Organizations, see Integrating AWS
* Organizations with Other AWS Services in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param disableAwsServiceAccessRequest
* @return A Java Future containing the result of the DisableAWSServiceAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DisableAWSServiceAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture disableAWSServiceAccess(
DisableAwsServiceAccessRequest disableAwsServiceAccessRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisableAWSServiceAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisableAWSServiceAccess")
.withMarshaller(new DisableAwsServiceAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(disableAwsServiceAccessRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disables an organizational control policy type in a root. A policy of a certain type can be attached to entities
* in a root only if that type is enabled in the root. After you perform this operation, you no longer can attach
* policies of the specified type to that root or to any organizational unit (OU) or account in that root. You can
* undo this by using the EnablePolicyType operation.
*
*
* This is an asynchronous request that AWS performs in the background. If you disable a policy for a root, it still
* appears enabled for the organization if all
* features are enabled for the organization. AWS recommends that you first use ListRoots to see the
* status of policy types for a specified root, and then use this operation.
*
*
* This operation can be called only from the organization's master account.
*
*
* To view the status of available policy types in the organization, use DescribeOrganization.
*
*
* @param disablePolicyTypeRequest
* @return A Java Future containing the result of the DisablePolicyType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyTypeNotEnabledException The specified policy type isn't currently enabled in this root. You
* can't attach policies of the specified type to entities in a root until you enable that type in the root.
* For more information, see Enabling All Features in Your Organization in the AWS Organizations User Guide.
* - RootNotFoundException We can't find a root with the
RootId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - PolicyChangesInProgressException Changes to the effective policy are in progress, and its contents
* can't be returned. Try the operation again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.DisablePolicyType
* @see AWS API Documentation
*/
@Override
public CompletableFuture disablePolicyType(DisablePolicyTypeRequest disablePolicyTypeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisablePolicyTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisablePolicyType")
.withMarshaller(new DisablePolicyTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(disablePolicyTypeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables the integration of an AWS service (the service that is specified by ServicePrincipal
) with
* AWS Organizations. When you enable integration, you allow the specified service to create a service-linked role in
* all the accounts in your organization. This allows the service to perform operations on your behalf in your
* organization and its accounts.
*
*
*
* We recommend that you enable integration between AWS Organizations and the specified AWS service by using the
* console or commands that are provided by the specified service. Doing so ensures that the service is aware that
* it can create the resources that are required for the integration. How the service creates those resources in the
* organization's accounts depends on that service. For more information, see the documentation for the other AWS
* service.
*
*
*
* For more information about enabling services to integrate with AWS Organizations, see Integrating AWS
* Organizations with Other AWS Services in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account and only if the organization has enabled all
* features.
*
*
* @param enableAwsServiceAccessRequest
* @return A Java Future containing the result of the EnableAWSServiceAccess operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.EnableAWSServiceAccess
* @see AWS API Documentation
*/
@Override
public CompletableFuture enableAWSServiceAccess(
EnableAwsServiceAccessRequest enableAwsServiceAccessRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, EnableAWSServiceAccessResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableAWSServiceAccess")
.withMarshaller(new EnableAwsServiceAccessRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(enableAwsServiceAccessRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables all features in an organization. This enables the use of organization policies that can restrict the
* services and actions that can be called in each account. Until you enable all features, you have access only to
* consolidated billing, and you can't use any of the advanced account administration features that AWS
* Organizations supports. For more information, see Enabling All Features in Your Organization in the AWS Organizations User Guide.
*
*
*
* This operation is required only for organizations that were created explicitly with only the consolidated billing
* features enabled. Calling this operation sends a handshake to every invited account in the organization. The
* feature set change can be finalized and the additional features enabled only after all administrators in the
* invited accounts approve the change by accepting the handshake.
*
*
*
* After you enable all features, you can separately enable or disable individual policy types in a root using
* EnablePolicyType and DisablePolicyType. To see the status of policy types in a root, use
* ListRoots.
*
*
* After all invited member accounts accept the handshake, you finalize the feature set change by accepting the
* handshake that contains "Action": "ENABLE_ALL_FEATURES"
. This completes the change.
*
*
* After you enable all features in your organization, the master account in the organization can apply policies on
* all member accounts. These policies can restrict what users and even administrators in those accounts can do. The
* master account can apply policies that prevent accounts from leaving the organization. Ensure that your account
* administrators are aware of this.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param enableAllFeaturesRequest
* @return A Java Future containing the result of the EnableAllFeatures operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - HandshakeConstraintViolationException The requested operation would violate the constraint identified
* in the reason code.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. Note that deleted and closed accounts still count toward your limit.
*
*
*
* If you get this exception immediately after creating the organization, wait one hour and try again. If
* after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* ALREADY_IN_AN_ORGANIZATION: The handshake request is invalid because the invited account is already a
* member of an organization.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* INVITE_DISABLED_DURING_ENABLE_ALL_FEATURES: You can't issue new invitations to join an organization while
* it's in the process of enabling all features. You can resume inviting accounts after you finalize the
* process when all accounts have agreed to the change.
*
*
* -
*
* ORGANIZATION_ALREADY_HAS_ALL_FEATURES: The handshake request is invalid because the organization has
* already enabled all features.
*
*
* -
*
* ORGANIZATION_FROM_DIFFERENT_SELLER_OF_RECORD: The request failed because the account is from a different
* marketplace than the accounts in the organization. For example, accounts with India addresses must be
* associated with the AISPL marketplace. All accounts in an organization must be from the same marketplace.
*
*
* -
*
* ORGANIZATION_MEMBERSHIP_CHANGE_RATE_LIMIT_EXCEEDED: You attempted to change the membership of an account
* too quickly after its previous change.
*
*
* -
*
* PAYMENT_INSTRUMENT_REQUIRED: You can't complete the operation with an account that doesn't have a payment
* instrument, such as a credit card, associated with it.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.EnableAllFeatures
* @see AWS API Documentation
*/
@Override
public CompletableFuture enableAllFeatures(EnableAllFeaturesRequest enableAllFeaturesRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, EnableAllFeaturesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnableAllFeatures")
.withMarshaller(new EnableAllFeaturesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(enableAllFeaturesRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables a policy type in a root. After you enable a policy type in a root, you can attach policies of that type
* to the root, any organizational unit (OU), or account in that root. You can undo this by using the
* DisablePolicyType operation.
*
*
* This is an asynchronous request that AWS performs in the background. AWS recommends that you first use
* ListRoots to see the status of policy types for a specified root, and then use this operation.
*
*
* This operation can be called only from the organization's master account.
*
*
* You can enable a policy type in a root only if that policy type is available in the organization. To view the
* status of available policy types in the organization, use DescribeOrganization.
*
*
* @param enablePolicyTypeRequest
* @return A Java Future containing the result of the EnablePolicyType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyTypeAlreadyEnabledException The specified policy type is already enabled in the specified root.
*
* - RootNotFoundException We can't find a root with the
RootId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - PolicyTypeNotAvailableForOrganizationException You can't use the specified policy type with the
* feature set currently enabled for this organization. For example, you can enable SCPs only after you
* enable all features in the organization. For more information, see Enabling and Disabling a Policy Type on a Root in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - PolicyChangesInProgressException Changes to the effective policy are in progress, and its contents
* can't be returned. Try the operation again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.EnablePolicyType
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture enablePolicyType(EnablePolicyTypeRequest enablePolicyTypeRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, EnablePolicyTypeResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("EnablePolicyType")
.withMarshaller(new EnablePolicyTypeRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(enablePolicyTypeRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sends an invitation to another account to join your organization as a member account. AWS Organizations sends
* email on your behalf to the email address that is associated with the other account's owner. The invitation is
* implemented as a Handshake whose details are in the response.
*
*
*
* -
*
* You can invite AWS accounts only from the same seller as the master account. For example, if your organization's
* master account was created by Amazon Internet Services Pvt. Ltd (AISPL), an AWS seller in India, you can invite
* only other AISPL accounts to your organization. You can't combine accounts from AISPL and AWS or from any other
* AWS seller. For more information, see Consolidated Billing in India.
*
*
* -
*
* If you receive an exception that indicates that you exceeded your account limits for the organization or that the
* operation failed because your organization is still initializing, wait one hour and then try again. If the error
* persists after an hour, contact AWS Support.
*
*
*
*
*
* This operation can be called only from the organization's master account.
*
*
* @param inviteAccountToOrganizationRequest
* @return A Java Future containing the result of the InviteAccountToOrganization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - AccountOwnerNotVerifiedException You can't invite an existing account to your organization until you
* verify that you own the email address associated with the master account. For more information, see Email Address Verification in the AWS Organizations User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - HandshakeConstraintViolationException The requested operation would violate the constraint identified
* in the reason code.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. Note that deleted and closed accounts still count toward your limit.
*
*
*
* If you get this exception immediately after creating the organization, wait one hour and try again. If
* after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* ALREADY_IN_AN_ORGANIZATION: The handshake request is invalid because the invited account is already a
* member of an organization.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* INVITE_DISABLED_DURING_ENABLE_ALL_FEATURES: You can't issue new invitations to join an organization while
* it's in the process of enabling all features. You can resume inviting accounts after you finalize the
* process when all accounts have agreed to the change.
*
*
* -
*
* ORGANIZATION_ALREADY_HAS_ALL_FEATURES: The handshake request is invalid because the organization has
* already enabled all features.
*
*
* -
*
* ORGANIZATION_FROM_DIFFERENT_SELLER_OF_RECORD: The request failed because the account is from a different
* marketplace than the accounts in the organization. For example, accounts with India addresses must be
* associated with the AISPL marketplace. All accounts in an organization must be from the same marketplace.
*
*
* -
*
* ORGANIZATION_MEMBERSHIP_CHANGE_RATE_LIMIT_EXCEEDED: You attempted to change the membership of an account
* too quickly after its previous change.
*
*
* -
*
* PAYMENT_INSTRUMENT_REQUIRED: You can't complete the operation with an account that doesn't have a payment
* instrument, such as a credit card, associated with it.
*
*
* - DuplicateHandshakeException A handshake with the same action and target already exists. For example,
* if you invited an account to join your organization, the invited account might already have a pending
* invitation from this organization. If you intend to resend an invitation to an account, ensure that
* existing handshakes that might be considered duplicates are canceled or declined.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - FinalizingOrganizationException AWS Organizations couldn't perform the operation because your
* organization hasn't finished initializing. This can take up to an hour. Try again later. If after one
* hour you continue to receive this error, contact AWS Support.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.InviteAccountToOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture inviteAccountToOrganization(
InviteAccountToOrganizationRequest inviteAccountToOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, InviteAccountToOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("InviteAccountToOrganization")
.withMarshaller(new InviteAccountToOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(inviteAccountToOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes a member account from its parent organization. This version of the operation is performed by the account
* that wants to leave. To remove a member account as a user in the master account, use
* RemoveAccountFromOrganization instead.
*
*
* This operation can be called only from a member account in the organization.
*
*
*
* -
*
* The master account in an organization with all features enabled can set service control policies (SCPs) that can
* restrict what administrators of member accounts can do. This includes preventing them from successfully calling
* LeaveOrganization
and leaving the organization.
*
*
* -
*
* You can leave an organization as a member account only if the account is configured with the information required
* to operate as a standalone account. When you create an account in an organization using the AWS Organizations
* console, API, or CLI commands, the information required of standalone accounts is not automatically
* collected. For each account that you want to make standalone, you must do the following steps:
*
*
* -
*
* Accept the end user license agreement (EULA)
*
*
* -
*
* Choose a support plan
*
*
* -
*
* Provide and verify the required contact information
*
*
* -
*
* Provide a current payment method
*
*
*
*
* AWS uses the payment method to charge for any billable (not free tier) AWS activity that occurs while the account
* isn't attached to an organization. Follow the steps at To leave an organization when all required account information has not yet been provided in the AWS
* Organizations User Guide.
*
*
* -
*
* You can leave an organization only after you enable IAM user access to billing in your account. For more
* information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost Management User
* Guide.
*
*
*
*
*
* @param leaveOrganizationRequest
* @return A Java Future containing the result of the LeaveOrganization operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - MasterCannotLeaveOrganizationException You can't remove a master account from an organization. If you
* want the master account to become a member account in another organization, you must first delete the
* current organization of the master account.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.LeaveOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture leaveOrganization(LeaveOrganizationRequest leaveOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, LeaveOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("LeaveOrganization")
.withMarshaller(new LeaveOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(leaveOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the AWS services that you enabled to integrate with your organization. After a service on this
* list creates the resources that it requires for the integration, it can perform operations on your organization
* and its accounts.
*
*
* For more information about integrating other services with AWS Organizations, including the list of services that
* currently work with Organizations, see Integrating AWS
* Organizations with Other AWS Services in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listAwsServiceAccessForOrganizationRequest
* @return A Java Future containing the result of the ListAWSServiceAccessForOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAWSServiceAccessForOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture listAWSServiceAccessForOrganization(
ListAwsServiceAccessForOrganizationRequest listAwsServiceAccessForOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListAwsServiceAccessForOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAWSServiceAccessForOrganization")
.withMarshaller(new ListAwsServiceAccessForOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listAwsServiceAccessForOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Returns a list of the AWS services that you enabled to integrate with your organization. After a service on this
* list creates the resources that it requires for the integration, it can perform operations on your organization
* and its accounts.
*
*
* For more information about integrating other services with AWS Organizations, including the list of services that
* currently work with Organizations, see Integrating AWS
* Organizations with Other AWS Services in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listAWSServiceAccessForOrganization(software.amazon.awssdk.services.organizations.model.ListAwsServiceAccessForOrganizationRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAWSServiceAccessForOrganizationPublisher publisher = client.listAWSServiceAccessForOrganizationPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAWSServiceAccessForOrganizationPublisher publisher = client.listAWSServiceAccessForOrganizationPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListAwsServiceAccessForOrganizationResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAWSServiceAccessForOrganization(software.amazon.awssdk.services.organizations.model.ListAwsServiceAccessForOrganizationRequest)}
* operation.
*
*
* @param listAwsServiceAccessForOrganizationRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAWSServiceAccessForOrganization
* @see AWS API Documentation
*/
public ListAWSServiceAccessForOrganizationPublisher listAWSServiceAccessForOrganizationPaginator(
ListAwsServiceAccessForOrganizationRequest listAwsServiceAccessForOrganizationRequest) {
return new ListAWSServiceAccessForOrganizationPublisher(this,
applyPaginatorUserAgent(listAwsServiceAccessForOrganizationRequest));
}
/**
*
* Lists all the accounts in the organization. To request only the accounts in a specified root or organizational
* unit (OU), use the ListAccountsForParent operation instead.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listAccountsRequest
* @return A Java Future containing the result of the ListAccounts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAccounts(ListAccountsRequest listAccountsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAccountsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAccounts").withMarshaller(new ListAccountsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listAccountsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the accounts in an organization that are contained by the specified target root or organizational unit
* (OU). If you specify the root, you get a list of all the accounts that aren't in any OU. If you specify an OU,
* you get a list of all the accounts in only that OU and not in any child OUs. To get a list of all accounts in the
* organization, use the ListAccounts operation.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listAccountsForParentRequest
* @return A Java Future containing the result of the ListAccountsForParent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAccountsForParent
* @see AWS API Documentation
*/
@Override
public CompletableFuture listAccountsForParent(
ListAccountsForParentRequest listAccountsForParentRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListAccountsForParentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListAccountsForParent")
.withMarshaller(new ListAccountsForParentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listAccountsForParentRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the accounts in an organization that are contained by the specified target root or organizational unit
* (OU). If you specify the root, you get a list of all the accounts that aren't in any OU. If you specify an OU,
* you get a list of all the accounts in only that OU and not in any child OUs. To get a list of all accounts in the
* organization, use the ListAccounts operation.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listAccountsForParent(software.amazon.awssdk.services.organizations.model.ListAccountsForParentRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAccountsForParentPublisher publisher = client.listAccountsForParentPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAccountsForParentPublisher publisher = client.listAccountsForParentPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListAccountsForParentResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccountsForParent(software.amazon.awssdk.services.organizations.model.ListAccountsForParentRequest)}
* operation.
*
*
* @param listAccountsForParentRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAccountsForParent
* @see AWS API Documentation
*/
public ListAccountsForParentPublisher listAccountsForParentPaginator(ListAccountsForParentRequest listAccountsForParentRequest) {
return new ListAccountsForParentPublisher(this, applyPaginatorUserAgent(listAccountsForParentRequest));
}
/**
*
* Lists all the accounts in the organization. To request only the accounts in a specified root or organizational
* unit (OU), use the ListAccountsForParent operation instead.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listAccounts(software.amazon.awssdk.services.organizations.model.ListAccountsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListAccountsPublisher publisher = client.listAccountsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListAccountsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listAccounts(software.amazon.awssdk.services.organizations.model.ListAccountsRequest)} operation.
*
*
* @param listAccountsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListAccounts
* @see AWS API
* Documentation
*/
public ListAccountsPublisher listAccountsPaginator(ListAccountsRequest listAccountsRequest) {
return new ListAccountsPublisher(this, applyPaginatorUserAgent(listAccountsRequest));
}
/**
*
* Lists all of the organizational units (OUs) or accounts that are contained in the specified parent OU or root.
* This operation, along with ListParents enables you to traverse the tree structure that makes up this root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listChildrenRequest
* @return A Java Future containing the result of the ListChildren operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListChildren
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listChildren(ListChildrenRequest listChildrenRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListChildrenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListChildren").withMarshaller(new ListChildrenRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listChildrenRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all of the organizational units (OUs) or accounts that are contained in the specified parent OU or root.
* This operation, along with ListParents enables you to traverse the tree structure that makes up this root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listChildren(software.amazon.awssdk.services.organizations.model.ListChildrenRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListChildrenPublisher publisher = client.listChildrenPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListChildrenPublisher publisher = client.listChildrenPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListChildrenResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChildren(software.amazon.awssdk.services.organizations.model.ListChildrenRequest)} operation.
*
*
* @param listChildrenRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListChildren
* @see AWS API
* Documentation
*/
public ListChildrenPublisher listChildrenPaginator(ListChildrenRequest listChildrenRequest) {
return new ListChildrenPublisher(this, applyPaginatorUserAgent(listChildrenRequest));
}
/**
*
* Lists the account creation requests that match the specified status that is currently being tracked for the
* organization.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listCreateAccountStatusRequest
* @return A Java Future containing the result of the ListCreateAccountStatus operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListCreateAccountStatus
* @see AWS API Documentation
*/
@Override
public CompletableFuture listCreateAccountStatus(
ListCreateAccountStatusRequest listCreateAccountStatusRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCreateAccountStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCreateAccountStatus")
.withMarshaller(new ListCreateAccountStatusRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listCreateAccountStatusRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the account creation requests that match the specified status that is currently being tracked for the
* organization.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listCreateAccountStatus(software.amazon.awssdk.services.organizations.model.ListCreateAccountStatusRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListCreateAccountStatusPublisher publisher = client.listCreateAccountStatusPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListCreateAccountStatusPublisher publisher = client.listCreateAccountStatusPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListCreateAccountStatusResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCreateAccountStatus(software.amazon.awssdk.services.organizations.model.ListCreateAccountStatusRequest)}
* operation.
*
*
* @param listCreateAccountStatusRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListCreateAccountStatus
* @see AWS API Documentation
*/
public ListCreateAccountStatusPublisher listCreateAccountStatusPaginator(
ListCreateAccountStatusRequest listCreateAccountStatusRequest) {
return new ListCreateAccountStatusPublisher(this, applyPaginatorUserAgent(listCreateAccountStatusRequest));
}
/**
*
* Lists the AWS accounts that are designated as delegated administrators in this organization.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listDelegatedAdministratorsRequest
* @return A Java Future containing the result of the ListDelegatedAdministrators operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListDelegatedAdministrators
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDelegatedAdministrators(
ListDelegatedAdministratorsRequest listDelegatedAdministratorsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDelegatedAdministratorsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDelegatedAdministrators")
.withMarshaller(new ListDelegatedAdministratorsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listDelegatedAdministratorsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the AWS accounts that are designated as delegated administrators in this organization.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listDelegatedAdministrators(software.amazon.awssdk.services.organizations.model.ListDelegatedAdministratorsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListDelegatedAdministratorsPublisher publisher = client.listDelegatedAdministratorsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListDelegatedAdministratorsPublisher publisher = client.listDelegatedAdministratorsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListDelegatedAdministratorsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDelegatedAdministrators(software.amazon.awssdk.services.organizations.model.ListDelegatedAdministratorsRequest)}
* operation.
*
*
* @param listDelegatedAdministratorsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListDelegatedAdministrators
* @see AWS API Documentation
*/
public ListDelegatedAdministratorsPublisher listDelegatedAdministratorsPaginator(
ListDelegatedAdministratorsRequest listDelegatedAdministratorsRequest) {
return new ListDelegatedAdministratorsPublisher(this, applyPaginatorUserAgent(listDelegatedAdministratorsRequest));
}
/**
*
* List the AWS services for which the specified account is a delegated administrator.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listDelegatedServicesForAccountRequest
* @return A Java Future containing the result of the ListDelegatedServicesForAccount operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AccountNotRegisteredException The specified account is not a delegated administrator for this AWS
* service.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListDelegatedServicesForAccount
* @see AWS API Documentation
*/
@Override
public CompletableFuture listDelegatedServicesForAccount(
ListDelegatedServicesForAccountRequest listDelegatedServicesForAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDelegatedServicesForAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDelegatedServicesForAccount")
.withMarshaller(new ListDelegatedServicesForAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listDelegatedServicesForAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* List the AWS services for which the specified account is a delegated administrator.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listDelegatedServicesForAccount(software.amazon.awssdk.services.organizations.model.ListDelegatedServicesForAccountRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListDelegatedServicesForAccountPublisher publisher = client.listDelegatedServicesForAccountPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListDelegatedServicesForAccountPublisher publisher = client.listDelegatedServicesForAccountPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListDelegatedServicesForAccountResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listDelegatedServicesForAccount(software.amazon.awssdk.services.organizations.model.ListDelegatedServicesForAccountRequest)}
* operation.
*
*
* @param listDelegatedServicesForAccountRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AccountNotRegisteredException The specified account is not a delegated administrator for this AWS
* service.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListDelegatedServicesForAccount
* @see AWS API Documentation
*/
public ListDelegatedServicesForAccountPublisher listDelegatedServicesForAccountPaginator(
ListDelegatedServicesForAccountRequest listDelegatedServicesForAccountRequest) {
return new ListDelegatedServicesForAccountPublisher(this, applyPaginatorUserAgent(listDelegatedServicesForAccountRequest));
}
/**
*
* Lists the current handshakes that are associated with the account of the requesting user.
*
*
* Handshakes that are ACCEPTED
, DECLINED
, or CANCELED
appear in the results
* of this API for only 30 days after changing to that state. After that, they're deleted and no longer accessible.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listHandshakesForAccountRequest
* @return A Java Future containing the result of the ListHandshakesForAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListHandshakesForAccount
* @see AWS API Documentation
*/
@Override
public CompletableFuture listHandshakesForAccount(
ListHandshakesForAccountRequest listHandshakesForAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListHandshakesForAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListHandshakesForAccount")
.withMarshaller(new ListHandshakesForAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listHandshakesForAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the current handshakes that are associated with the account of the requesting user.
*
*
* Handshakes that are ACCEPTED
, DECLINED
, or CANCELED
appear in the results
* of this API for only 30 days after changing to that state. After that, they're deleted and no longer accessible.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listHandshakesForAccount(software.amazon.awssdk.services.organizations.model.ListHandshakesForAccountRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListHandshakesForAccountPublisher publisher = client.listHandshakesForAccountPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListHandshakesForAccountPublisher publisher = client.listHandshakesForAccountPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListHandshakesForAccountResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHandshakesForAccount(software.amazon.awssdk.services.organizations.model.ListHandshakesForAccountRequest)}
* operation.
*
*
* @param listHandshakesForAccountRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListHandshakesForAccount
* @see AWS API Documentation
*/
public ListHandshakesForAccountPublisher listHandshakesForAccountPaginator(
ListHandshakesForAccountRequest listHandshakesForAccountRequest) {
return new ListHandshakesForAccountPublisher(this, applyPaginatorUserAgent(listHandshakesForAccountRequest));
}
/**
*
* Lists the handshakes that are associated with the organization that the requesting user is part of. The
* ListHandshakesForOrganization
operation returns a list of handshake structures. Each structure
* contains details and status about a handshake.
*
*
* Handshakes that are ACCEPTED
, DECLINED
, or CANCELED
appear in the results
* of this API for only 30 days after changing to that state. After that, they're deleted and no longer accessible.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listHandshakesForOrganizationRequest
* @return A Java Future containing the result of the ListHandshakesForOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListHandshakesForOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture listHandshakesForOrganization(
ListHandshakesForOrganizationRequest listHandshakesForOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListHandshakesForOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListHandshakesForOrganization")
.withMarshaller(new ListHandshakesForOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listHandshakesForOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the handshakes that are associated with the organization that the requesting user is part of. The
* ListHandshakesForOrganization
operation returns a list of handshake structures. Each structure
* contains details and status about a handshake.
*
*
* Handshakes that are ACCEPTED
, DECLINED
, or CANCELED
appear in the results
* of this API for only 30 days after changing to that state. After that, they're deleted and no longer accessible.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listHandshakesForOrganization(software.amazon.awssdk.services.organizations.model.ListHandshakesForOrganizationRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListHandshakesForOrganizationPublisher publisher = client.listHandshakesForOrganizationPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListHandshakesForOrganizationPublisher publisher = client.listHandshakesForOrganizationPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListHandshakesForOrganizationResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listHandshakesForOrganization(software.amazon.awssdk.services.organizations.model.ListHandshakesForOrganizationRequest)}
* operation.
*
*
* @param listHandshakesForOrganizationRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListHandshakesForOrganization
* @see AWS API Documentation
*/
public ListHandshakesForOrganizationPublisher listHandshakesForOrganizationPaginator(
ListHandshakesForOrganizationRequest listHandshakesForOrganizationRequest) {
return new ListHandshakesForOrganizationPublisher(this, applyPaginatorUserAgent(listHandshakesForOrganizationRequest));
}
/**
*
* Lists the organizational units (OUs) in a parent organizational unit or root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listOrganizationalUnitsForParentRequest
* @return A Java Future containing the result of the ListOrganizationalUnitsForParent operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListOrganizationalUnitsForParent
* @see AWS API Documentation
*/
@Override
public CompletableFuture listOrganizationalUnitsForParent(
ListOrganizationalUnitsForParentRequest listOrganizationalUnitsForParentRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListOrganizationalUnitsForParentResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListOrganizationalUnitsForParent")
.withMarshaller(new ListOrganizationalUnitsForParentRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listOrganizationalUnitsForParentRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the organizational units (OUs) in a parent organizational unit or root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listOrganizationalUnitsForParent(software.amazon.awssdk.services.organizations.model.ListOrganizationalUnitsForParentRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListOrganizationalUnitsForParentPublisher publisher = client.listOrganizationalUnitsForParentPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListOrganizationalUnitsForParentPublisher publisher = client.listOrganizationalUnitsForParentPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListOrganizationalUnitsForParentResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOrganizationalUnitsForParent(software.amazon.awssdk.services.organizations.model.ListOrganizationalUnitsForParentRequest)}
* operation.
*
*
* @param listOrganizationalUnitsForParentRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ParentNotFoundException We can't find a root or OU with the
ParentId
that you specified.
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListOrganizationalUnitsForParent
* @see AWS API Documentation
*/
public ListOrganizationalUnitsForParentPublisher listOrganizationalUnitsForParentPaginator(
ListOrganizationalUnitsForParentRequest listOrganizationalUnitsForParentRequest) {
return new ListOrganizationalUnitsForParentPublisher(this,
applyPaginatorUserAgent(listOrganizationalUnitsForParentRequest));
}
/**
*
* Lists the root or organizational units (OUs) that serve as the immediate parent of the specified child OU or
* account. This operation, along with ListChildren enables you to traverse the tree structure that makes up
* this root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* In the current release, a child can have only a single parent.
*
*
*
* @param listParentsRequest
* @return A Java Future containing the result of the ListParents operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ChildNotFoundException We can't find an organizational unit (OU) or AWS account with the
*
ChildId
that you specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListParents
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listParents(ListParentsRequest listParentsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListParentsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListParents").withMarshaller(new ListParentsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listParentsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the root or organizational units (OUs) that serve as the immediate parent of the specified child OU or
* account. This operation, along with ListChildren enables you to traverse the tree structure that makes up
* this root.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* In the current release, a child can have only a single parent.
*
*
*
* This is a variant of {@link #listParents(software.amazon.awssdk.services.organizations.model.ListParentsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListParentsPublisher publisher = client.listParentsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListParentsPublisher publisher = client.listParentsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListParentsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listParents(software.amazon.awssdk.services.organizations.model.ListParentsRequest)} operation.
*
*
* @param listParentsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ChildNotFoundException We can't find an organizational unit (OU) or AWS account with the
*
ChildId
that you specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListParents
* @see AWS API
* Documentation
*/
public ListParentsPublisher listParentsPaginator(ListParentsRequest listParentsRequest) {
return new ListParentsPublisher(this, applyPaginatorUserAgent(listParentsRequest));
}
/**
*
* Retrieves the list of all policies in an organization of a specified type.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listPoliciesRequest
* @return A Java Future containing the result of the ListPolicies operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listPolicies(ListPoliciesRequest listPoliciesRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListPoliciesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPolicies").withMarshaller(new ListPoliciesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listPoliciesRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the policies that are directly attached to the specified target root, organizational unit (OU), or account.
* You must specify the policy type that you want included in the returned list.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listPoliciesForTargetRequest
* @return A Java Future containing the result of the ListPoliciesForTarget operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListPoliciesForTarget
* @see AWS API Documentation
*/
@Override
public CompletableFuture listPoliciesForTarget(
ListPoliciesForTargetRequest listPoliciesForTargetRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListPoliciesForTargetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListPoliciesForTarget")
.withMarshaller(new ListPoliciesForTargetRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listPoliciesForTargetRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the policies that are directly attached to the specified target root, organizational unit (OU), or account.
* You must specify the policy type that you want included in the returned list.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listPoliciesForTarget(software.amazon.awssdk.services.organizations.model.ListPoliciesForTargetRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListPoliciesForTargetPublisher publisher = client.listPoliciesForTargetPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListPoliciesForTargetPublisher publisher = client.listPoliciesForTargetPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListPoliciesForTargetResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPoliciesForTarget(software.amazon.awssdk.services.organizations.model.ListPoliciesForTargetRequest)}
* operation.
*
*
* @param listPoliciesForTargetRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListPoliciesForTarget
* @see AWS API Documentation
*/
public ListPoliciesForTargetPublisher listPoliciesForTargetPaginator(ListPoliciesForTargetRequest listPoliciesForTargetRequest) {
return new ListPoliciesForTargetPublisher(this, applyPaginatorUserAgent(listPoliciesForTargetRequest));
}
/**
*
* Retrieves the list of all policies in an organization of a specified type.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listPolicies(software.amazon.awssdk.services.organizations.model.ListPoliciesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListPoliciesPublisher publisher = client.listPoliciesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListPoliciesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPolicies(software.amazon.awssdk.services.organizations.model.ListPoliciesRequest)} operation.
*
*
* @param listPoliciesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListPolicies
* @see AWS API
* Documentation
*/
public ListPoliciesPublisher listPoliciesPaginator(ListPoliciesRequest listPoliciesRequest) {
return new ListPoliciesPublisher(this, applyPaginatorUserAgent(listPoliciesRequest));
}
/**
*
* Lists the roots that are defined in the current organization.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* Policy types can be enabled and disabled in roots. This is distinct from whether they're available in the
* organization. When you enable all features, you make policy types available for use in that organization.
* Individual policy types can then be enabled and disabled in a root. To see the availability of a policy type in
* an organization, use DescribeOrganization.
*
*
*
* @param listRootsRequest
* @return A Java Future containing the result of the ListRoots operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListRoots
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listRoots(ListRootsRequest listRootsRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListRootsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListRoots")
.withMarshaller(new ListRootsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listRootsRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the roots that are defined in the current organization.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* Policy types can be enabled and disabled in roots. This is distinct from whether they're available in the
* organization. When you enable all features, you make policy types available for use in that organization.
* Individual policy types can then be enabled and disabled in a root. To see the availability of a policy type in
* an organization, use DescribeOrganization.
*
*
*
* This is a variant of {@link #listRoots(software.amazon.awssdk.services.organizations.model.ListRootsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListRootsPublisher publisher = client.listRootsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListRootsPublisher publisher = client.listRootsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListRootsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRoots(software.amazon.awssdk.services.organizations.model.ListRootsRequest)} operation.
*
*
* @param listRootsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListRoots
* @see AWS API
* Documentation
*/
public ListRootsPublisher listRootsPaginator(ListRootsRequest listRootsRequest) {
return new ListRootsPublisher(this, applyPaginatorUserAgent(listRootsRequest));
}
/**
*
* Lists tags for the specified resource.
*
*
* Currently, you can list tags on an account in AWS Organizations.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource")
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listTagsForResourceRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists tags for the specified resource.
*
*
* Currently, you can list tags on an account in AWS Organizations.
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listTagsForResource(software.amazon.awssdk.services.organizations.model.ListTagsForResourceRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListTagsForResourcePublisher publisher = client.listTagsForResourcePaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListTagsForResourceResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTagsForResource(software.amazon.awssdk.services.organizations.model.ListTagsForResourceRequest)}
* operation.
*
*
* @param listTagsForResourceRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
public ListTagsForResourcePublisher listTagsForResourcePaginator(ListTagsForResourceRequest listTagsForResourceRequest) {
return new ListTagsForResourcePublisher(this, applyPaginatorUserAgent(listTagsForResourceRequest));
}
/**
*
* Lists all the roots, organizational units (OUs), and accounts that the specified policy is attached to.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
* @param listTargetsForPolicyRequest
* @return A Java Future containing the result of the ListTargetsForPolicy operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListTargetsForPolicy
* @see AWS API Documentation
*/
@Override
public CompletableFuture listTargetsForPolicy(
ListTargetsForPolicyRequest listTargetsForPolicyRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTargetsForPolicyResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTargetsForPolicy")
.withMarshaller(new ListTargetsForPolicyRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listTargetsForPolicyRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists all the roots, organizational units (OUs), and accounts that the specified policy is attached to.
*
*
*
* Always check the NextToken
response parameter for a null
value when calling a
* List*
operation. These operations can occasionally return an empty set of results even when there
* are more results available. The NextToken
response parameter value is null
only
* when there are no more results to display.
*
*
*
* This operation can be called only from the organization's master account or by a member account that is a
* delegated administrator for an AWS service.
*
*
*
* This is a variant of
* {@link #listTargetsForPolicy(software.amazon.awssdk.services.organizations.model.ListTargetsForPolicyRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListTargetsForPolicyPublisher publisher = client.listTargetsForPolicyPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.organizations.paginators.ListTargetsForPolicyPublisher publisher = client.listTargetsForPolicyPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.organizations.model.ListTargetsForPolicyResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Note: If you prefer to have control on service calls, use the
* {@link #listTargetsForPolicy(software.amazon.awssdk.services.organizations.model.ListTargetsForPolicyRequest)}
* operation.
*
*
* @param listTargetsForPolicyRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - PolicyNotFoundException We can't find a policy with the
PolicyId
that you specified.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.ListTargetsForPolicy
* @see AWS API Documentation
*/
public ListTargetsForPolicyPublisher listTargetsForPolicyPaginator(ListTargetsForPolicyRequest listTargetsForPolicyRequest) {
return new ListTargetsForPolicyPublisher(this, applyPaginatorUserAgent(listTargetsForPolicyRequest));
}
/**
*
* Moves an account from its current source parent root or organizational unit (OU) to the specified destination
* parent root or OU.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param moveAccountRequest
* @return A Java Future containing the result of the MoveAccount operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - SourceParentNotFoundException We can't find a source root or OU with the
ParentId
that
* you specified.
* - DestinationParentNotFoundException We can't find the destination container (a root or OU) with the
*
ParentId
that you specified.
* - DuplicateAccountException That account is already present in the specified destination.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.MoveAccount
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture moveAccount(MoveAccountRequest moveAccountRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
MoveAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("MoveAccount").withMarshaller(new MoveAccountRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(moveAccountRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Enables the specified member account to administer the Organizations features of the specified AWS service. It
* grants read-only access to AWS Organizations service data. The account still requires IAM permissions to access
* and administer the AWS service.
*
*
* You can run this action only for AWS services that support this feature. For a current list of services that
* support it, see the column Supports Delegated Administrator in the table at AWS Services
* that you can use with AWS Organizations in the AWS Organizations User Guide.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param registerDelegatedAdministratorRequest
* @return A Java Future containing the result of the RegisterDelegatedAdministrator operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountAlreadyRegisteredException The specified account is already a delegated administrator for this
* AWS service.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - UnsupportedApiEndpointException This action isn't available in the current Region.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.RegisterDelegatedAdministrator
* @see AWS API Documentation
*/
@Override
public CompletableFuture registerDelegatedAdministrator(
RegisterDelegatedAdministratorRequest registerDelegatedAdministratorRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RegisterDelegatedAdministratorResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RegisterDelegatedAdministrator")
.withMarshaller(new RegisterDelegatedAdministratorRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(registerDelegatedAdministratorRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes the specified account from the organization.
*
*
* The removed account becomes a standalone account that isn't a member of any organization. It's no longer subject
* to any policies and is responsible for its own bill payments. The organization's master account is no longer
* charged for any expenses accrued by the member account after it's removed from the organization.
*
*
* This operation can be called only from the organization's master account. Member accounts can remove themselves
* with LeaveOrganization instead.
*
*
*
* You can remove an account from your organization only if the account is configured with the information required
* to operate as a standalone account. When you create an account in an organization using the AWS Organizations
* console, API, or CLI commands, the information required of standalone accounts is not automatically
* collected. For an account that you want to make standalone, you must accept the end user license agreement
* (EULA), choose a support plan, provide and verify the required contact information, and provide a current payment
* method. AWS uses the payment method to charge for any billable (not free tier) AWS activity that occurs while the
* account isn't attached to an organization. To remove an account that doesn't yet have this information, you must
* sign in as the member account and follow the steps at To leave an organization when all required account information has not yet been provided in the AWS
* Organizations User Guide.
*
*
*
* @param removeAccountFromOrganizationRequest
* @return A Java Future containing the result of the RemoveAccountFromOrganization operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AccountNotFoundException We can't find an AWS account with the
AccountId
that you
* specified, or the account whose credentials you used to make this request isn't a member of an
* organization.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - MasterCannotLeaveOrganizationException You can't remove a master account from an organization. If you
* want the master account to become a member account in another organization, you must first delete the
* current organization of the master account.
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.RemoveAccountFromOrganization
* @see AWS API Documentation
*/
@Override
public CompletableFuture removeAccountFromOrganization(
RemoveAccountFromOrganizationRequest removeAccountFromOrganizationRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, RemoveAccountFromOrganizationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("RemoveAccountFromOrganization")
.withMarshaller(new RemoveAccountFromOrganizationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(removeAccountFromOrganizationRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds one or more tags to the specified resource.
*
*
* Currently, you can tag and untag accounts in AWS Organizations.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(tagResourceRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes a tag from the specified resource.
*
*
* Currently, you can tag and untag accounts in AWS Organizations.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - TargetNotFoundException We can't find a root, OU, or account with the
TargetId
that you
* specified.
* - ConstraintViolationException Performing this operation violates a minimum or maximum value limit. For
* example, attempting to remove the last service control policy (SCP) from an OU or root, inviting or
* creating too many accounts to the organization, or attaching too many policies to an account, OU, or
* root. This exception includes a reason that contains additional information about the violated limit.
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_EULA: You attempted to remove an account from the organization that doesn't
* yet have enough information to exist as a standalone account. This account requires you to first agree to
* the AWS Customer Agreement. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CANNOT_LEAVE_WITHOUT_PHONE_VERIFICATION: You attempted to remove an account from the organization
* that doesn't yet have enough information to exist as a standalone account. This account requires you to
* first complete phone verification. Follow the steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* ACCOUNT_CREATION_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of accounts that you can create
* in one day.
*
*
* -
*
* ACCOUNT_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the limit on the number of accounts in an
* organization. If you need more accounts, contact AWS Support to request an increase in your
* limit.
*
*
* Or the number of invitations that you tried to send would cause you to exceed the limit of accounts in
* your organization. Send fewer invitations or contact AWS Support to request an increase in the number of
* accounts.
*
*
*
* Deleted and closed accounts still count toward your limit.
*
*
*
* If you get receive this exception when running a command immediately after creating the organization,
* wait one hour and try again. If after an hour it continues to fail with this error, contact AWS Support.
*
*
* -
*
* CANNOT_REGISTER_MASTER_AS_DELEGATED_ADMINISTRATOR: You can designate only a member account as a delegated
* administrator.
*
*
* -
*
* CANNOT_REMOVE_DELEGATED_ADMINISTRATOR_FROM_ORG: To complete this operation, you must first deregister
* this account as a delegated administrator.
*
*
* -
*
* DELEGATED_ADMINISTRATOR_EXISTS_FOR_THIS_SERVICE: To complete this operation, you must first deregister
* all delegated administrators for this service.
*
*
* -
*
* HANDSHAKE_RATE_LIMIT_EXCEEDED: You attempted to exceed the number of handshakes that you can send in one
* day.
*
*
* -
*
* MASTER_ACCOUNT_ADDRESS_DOES_NOT_MATCH_MARKETPLACE: To create an account in this organization, you first
* must migrate the organization's master account to the marketplace that corresponds to the master
* account's address. For example, accounts with India addresses must be associated with the AISPL
* marketplace. All accounts in an organization must be associated with the same marketplace.
*
*
* -
*
* MASTER_ACCOUNT_MISSING_CONTACT_INFO: To complete this operation, you must first provide contact a valid
* address and phone number for the master account. Then try the operation again.
*
*
* -
*
* MASTER_ACCOUNT_NOT_GOVCLOUD_ENABLED: To complete this operation, the master account must have an
* associated account in the AWS GovCloud (US-West) Region. For more information, see AWS
* Organizations in the AWS GovCloud User Guide.
*
*
* -
*
* MASTER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To create an organization with this master account, you first
* must associate a valid payment instrument, such as a credit card, with the account. Follow the steps at
* To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MAX_DELEGATED_ADMINISTRATORS_FOR_SERVICE_LIMIT_EXCEEDED: You attempted to register more delegated
* administrators than allowed for the service principal.
*
*
* -
*
* MAX_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to exceed the number of policies of a certain
* type that can be attached to an entity at one time.
*
*
* -
*
* MAX_TAG_LIMIT_EXCEEDED: You have exceeded the number of tags allowed on this resource.
*
*
* -
*
* MEMBER_ACCOUNT_PAYMENT_INSTRUMENT_REQUIRED: To complete this operation with this member account, you
* first must associate a valid payment instrument, such as a credit card, with the account. Follow the
* steps at To leave an organization when all required account information has not yet been provided in the
* AWS Organizations User Guide.
*
*
* -
*
* MIN_POLICY_TYPE_ATTACHMENT_LIMIT_EXCEEDED: You attempted to detach a policy from an entity that would
* cause the entity to have fewer than the minimum number of policies of a certain type required.
*
*
* -
*
* OU_DEPTH_LIMIT_EXCEEDED: You attempted to create an OU tree that is too many levels deep.
*
*
* -
*
* ORGANIZATION_NOT_IN_ALL_FEATURES_MODE: You attempted to perform an operation that requires the
* organization to be configured to support all features. An organization that supports only consolidated
* billing features can't perform this operation.
*
*
* -
*
* OU_NUMBER_LIMIT_EXCEEDED: You attempted to exceed the number of OUs that you can have in an organization.
*
*
* -
*
* POLICY_NUMBER_LIMIT_EXCEEDED. You attempted to exceed the number of policies that you can have in an
* organization.
*
*
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the NextToken
parameter from the response to a
* previous call of the operation.
*
*
* -
*
* INVALID_PARTY_TYPE_TARGET: You specified the wrong type of entity (account, organization, or email) as a
* party.
*
*
* -
*
* INVALID_PATTERN: You provided a value that doesn't match the required pattern.
*
*
* -
*
* INVALID_PATTERN_TARGET_ID: You specified a policy target ID that doesn't match the required pattern.
*
*
* -
*
* INVALID_ROLE_NAME: You provided a role name that isn't valid. A role name can't begin with the reserved
* prefix AWSServiceRoleFor
.
*
*
* -
*
* INVALID_SYNTAX_ORGANIZATION_ARN: You specified an invalid Amazon Resource Name (ARN) for the
* organization.
*
*
* -
*
* INVALID_SYNTAX_POLICY_ID: You specified an invalid policy ID.
*
*
* -
*
* INVALID_SYSTEM_TAGS_PARAMETER: You specified a tag key that is a system tag. You can’t add, edit, or
* delete system tag keys because they're reserved for AWS use. System tags don’t count against your tags
* per resource limit.
*
*
* -
*
* MAX_FILTER_LIMIT_EXCEEDED: You can specify only one filter parameter for the operation.
*
*
* -
*
* MAX_LENGTH_EXCEEDED: You provided a string parameter that is longer than allowed.
*
*
* -
*
* MAX_VALUE_EXCEEDED: You provided a numeric parameter that has a larger value than allowed.
*
*
* -
*
* MIN_LENGTH_EXCEEDED: You provided a string parameter that is shorter than allowed.
*
*
* -
*
* MIN_VALUE_EXCEEDED: You provided a numeric parameter that has a smaller value than allowed.
*
*
* -
*
* MOVING_ACCOUNT_BETWEEN_DIFFERENT_ROOTS: You can move an account only between entities in the same root.
*
*
* - ServiceException AWS Organizations can't complete your request because of an internal service error.
* Try again later.
* - TooManyRequestsException You have sent too many requests in too short a period of time. The limit
* helps protect against denial-of-service attacks. Try again later.
*
* For information on limits that affect AWS Organizations, see Limits of
* AWS Organizations in the AWS Organizations User Guide.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OrganizationsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OrganizationsAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
try {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource")
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(untagResourceRequest));
return executeFuture;
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Renames the specified organizational unit (OU). The ID and ARN don't change. The child OUs and accounts remain in
* place, and any attached policies of the OU remain attached.
*
*
* This operation can be called only from the organization's master account.
*
*
* @param updateOrganizationalUnitRequest
* @return A Java Future containing the result of the UpdateOrganizationalUnit operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException You don't have permissions to perform the requested operation. The user or role
* that is making the request must have at least one IAM permissions policy attached that grants the
* required permissions. For more information, see Access Management in the IAM
* User Guide.
* - AwsOrganizationsNotInUseException Your account isn't a member of an organization. To make this
* request, you must use the credentials of an account that belongs to an organization.
* - ConcurrentModificationException The target of the operation is currently being modified by a
* different request. Try again later.
* - DuplicateOrganizationalUnitException An OU with the same name already exists.
* - InvalidInputException The requested operation failed because you provided invalid values for one or
* more of the request parameters. This exception includes a reason that contains additional information
* about the violated limit:
*
* Some of the reasons in the following list might not be applicable to this specific API or operation:
*
*
*
* -
*
* IMMUTABLE_POLICY: You specified a policy that is managed by AWS and can't be modified.
*
*
* -
*
* INPUT_REQUIRED: You must include a value for all required parameters.
*
*
* -
*
* INVALID_ENUM: You specified an invalid value.
*
*
* -
*
* INVALID_FULL_NAME_TARGET: You specified a full name that contains invalid characters.
*
*
* -
*
* INVALID_LIST_MEMBER: You provided a list to a parameter that contains at least one invalid value.
*
*
* -
*
* INVALID_PAGINATION_TOKEN: Get the value for the