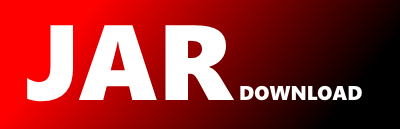
software.amazon.awssdk.services.organizations.model.Organization Maven / Gradle / Ivy
Show all versions of organizations Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.organizations.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains details about an organization. An organization is a collection of accounts that are centrally managed
* together using consolidated billing, organized hierarchically with organizational units (OUs), and controlled with
* policies .
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Organization implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Id")
.getter(getter(Organization::id)).setter(setter(Builder::id))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Id").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Arn")
.getter(getter(Organization::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField FEATURE_SET_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FeatureSet").getter(getter(Organization::featureSetAsString)).setter(setter(Builder::featureSet))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FeatureSet").build()).build();
private static final SdkField MASTER_ACCOUNT_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterAccountArn").getter(getter(Organization::masterAccountArn))
.setter(setter(Builder::masterAccountArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterAccountArn").build()).build();
private static final SdkField MASTER_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterAccountId").getter(getter(Organization::masterAccountId)).setter(setter(Builder::masterAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterAccountId").build()).build();
private static final SdkField MASTER_ACCOUNT_EMAIL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MasterAccountEmail").getter(getter(Organization::masterAccountEmail))
.setter(setter(Builder::masterAccountEmail))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MasterAccountEmail").build())
.build();
private static final SdkField> AVAILABLE_POLICY_TYPES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("AvailablePolicyTypes")
.getter(getter(Organization::availablePolicyTypes))
.setter(setter(Builder::availablePolicyTypes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AvailablePolicyTypes").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PolicyTypeSummary::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ID_FIELD, ARN_FIELD,
FEATURE_SET_FIELD, MASTER_ACCOUNT_ARN_FIELD, MASTER_ACCOUNT_ID_FIELD, MASTER_ACCOUNT_EMAIL_FIELD,
AVAILABLE_POLICY_TYPES_FIELD));
private static final long serialVersionUID = 1L;
private final String id;
private final String arn;
private final String featureSet;
private final String masterAccountArn;
private final String masterAccountId;
private final String masterAccountEmail;
private final List availablePolicyTypes;
private Organization(BuilderImpl builder) {
this.id = builder.id;
this.arn = builder.arn;
this.featureSet = builder.featureSet;
this.masterAccountArn = builder.masterAccountArn;
this.masterAccountId = builder.masterAccountId;
this.masterAccountEmail = builder.masterAccountEmail;
this.availablePolicyTypes = builder.availablePolicyTypes;
}
/**
*
* The unique identifier (ID) of an organization.
*
*
* The regex pattern for an organization ID string requires "o-"
* followed by from 10 to 32 lowercase letters or digits.
*
*
* @return The unique identifier (ID) of an organization.
*
* The regex pattern for an organization ID string requires
* "o-" followed by from 10 to 32 lowercase letters or digits.
*/
public final String id() {
return id;
}
/**
*
* The Amazon Resource Name (ARN) of an organization.
*
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization Reference.
*
*
* @return The Amazon Resource Name (ARN) of an organization.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
*/
public final String arn() {
return arn;
}
/**
*
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all features
* are enabled and policies can be applied to accounts in the organization. If set to "CONSOLIDATED_BILLING", then
* only consolidated billing functionality is available. For more information, see Enabling all features in your organization in the Organizations User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureSet} will
* return {@link OrganizationFeatureSet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureSetAsString}.
*
*
* @return Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more information,
* see Enabling all features in your organization in the Organizations User Guide.
* @see OrganizationFeatureSet
*/
public final OrganizationFeatureSet featureSet() {
return OrganizationFeatureSet.fromValue(featureSet);
}
/**
*
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all features
* are enabled and policies can be applied to accounts in the organization. If set to "CONSOLIDATED_BILLING", then
* only consolidated billing functionality is available. For more information, see Enabling all features in your organization in the Organizations User Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #featureSet} will
* return {@link OrganizationFeatureSet#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #featureSetAsString}.
*
*
* @return Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more information,
* see Enabling all features in your organization in the Organizations User Guide.
* @see OrganizationFeatureSet
*/
public final String featureSetAsString() {
return featureSet;
}
/**
*
* The Amazon Resource Name (ARN) of the account that is designated as the management account for the organization.
*
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization Reference.
*
*
* @return The Amazon Resource Name (ARN) of the account that is designated as the management account for the
* organization.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
*/
public final String masterAccountArn() {
return masterAccountArn;
}
/**
*
* The unique identifier (ID) of the management account of an organization.
*
*
* The regex pattern for an account ID string requires exactly 12
* digits.
*
*
* @return The unique identifier (ID) of the management account of an organization.
*
* The regex pattern for an account ID string requires exactly
* 12 digits.
*/
public final String masterAccountId() {
return masterAccountId;
}
/**
*
* The email address that is associated with the Amazon Web Services account that is designated as the management
* account for the organization.
*
*
* @return The email address that is associated with the Amazon Web Services account that is designated as the
* management account for the organization.
*/
public final String masterAccountEmail() {
return masterAccountEmail;
}
/**
* For responses, this returns true if the service returned a value for the AvailablePolicyTypes property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasAvailablePolicyTypes() {
return availablePolicyTypes != null && !(availablePolicyTypes instanceof SdkAutoConstructList);
}
/**
*
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in your
* organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the ListRoots
* operation instead.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAvailablePolicyTypes} method.
*
*
* @return
* Do not use. This field is deprecated and doesn't provide complete information about the policies in your
* organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
*/
public final List availablePolicyTypes() {
return availablePolicyTypes;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(id());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(featureSetAsString());
hashCode = 31 * hashCode + Objects.hashCode(masterAccountArn());
hashCode = 31 * hashCode + Objects.hashCode(masterAccountId());
hashCode = 31 * hashCode + Objects.hashCode(masterAccountEmail());
hashCode = 31 * hashCode + Objects.hashCode(hasAvailablePolicyTypes() ? availablePolicyTypes() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Organization)) {
return false;
}
Organization other = (Organization) obj;
return Objects.equals(id(), other.id()) && Objects.equals(arn(), other.arn())
&& Objects.equals(featureSetAsString(), other.featureSetAsString())
&& Objects.equals(masterAccountArn(), other.masterAccountArn())
&& Objects.equals(masterAccountId(), other.masterAccountId())
&& Objects.equals(masterAccountEmail(), other.masterAccountEmail())
&& hasAvailablePolicyTypes() == other.hasAvailablePolicyTypes()
&& Objects.equals(availablePolicyTypes(), other.availablePolicyTypes());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Organization").add("Id", id()).add("Arn", arn()).add("FeatureSet", featureSetAsString())
.add("MasterAccountArn", masterAccountArn()).add("MasterAccountId", masterAccountId())
.add("MasterAccountEmail", masterAccountEmail() == null ? null : "*** Sensitive Data Redacted ***")
.add("AvailablePolicyTypes", hasAvailablePolicyTypes() ? availablePolicyTypes() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Id":
return Optional.ofNullable(clazz.cast(id()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "FeatureSet":
return Optional.ofNullable(clazz.cast(featureSetAsString()));
case "MasterAccountArn":
return Optional.ofNullable(clazz.cast(masterAccountArn()));
case "MasterAccountId":
return Optional.ofNullable(clazz.cast(masterAccountId()));
case "MasterAccountEmail":
return Optional.ofNullable(clazz.cast(masterAccountEmail()));
case "AvailablePolicyTypes":
return Optional.ofNullable(clazz.cast(availablePolicyTypes()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* The regex pattern for an organization ID string requires
* "o-" followed by from 10 to 32 lowercase letters or digits.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder id(String id);
/**
*
* The Amazon Resource Name (ARN) of an organization.
*
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
*
*
* @param arn
* The Amazon Resource Name (ARN) of an organization.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder arn(String arn);
/**
*
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more information, see
* Enabling all features in your organization in the Organizations User Guide.
*
*
* @param featureSet
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more
* information, see Enabling all features in your organization in the Organizations User Guide.
* @see OrganizationFeatureSet
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrganizationFeatureSet
*/
Builder featureSet(String featureSet);
/**
*
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more information, see
* Enabling all features in your organization in the Organizations User Guide.
*
*
* @param featureSet
* Specifies the functionality that currently is available to the organization. If set to "ALL", then all
* features are enabled and policies can be applied to accounts in the organization. If set to
* "CONSOLIDATED_BILLING", then only consolidated billing functionality is available. For more
* information, see Enabling all features in your organization in the Organizations User Guide.
* @see OrganizationFeatureSet
* @return Returns a reference to this object so that method calls can be chained together.
* @see OrganizationFeatureSet
*/
Builder featureSet(OrganizationFeatureSet featureSet);
/**
*
* The Amazon Resource Name (ARN) of the account that is designated as the management account for the
* organization.
*
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
*
*
* @param masterAccountArn
* The Amazon Resource Name (ARN) of the account that is designated as the management account for the
* organization.
*
* For more information about ARNs in Organizations, see ARN Formats Supported by Organizations in the Amazon Web Services Service Authorization
* Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterAccountArn(String masterAccountArn);
/**
*
* The unique identifier (ID) of the management account of an organization.
*
*
* The regex pattern for an account ID string requires exactly 12
* digits.
*
*
* @param masterAccountId
* The unique identifier (ID) of the management account of an organization.
*
* The regex pattern for an account ID string requires
* exactly 12 digits.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterAccountId(String masterAccountId);
/**
*
* The email address that is associated with the Amazon Web Services account that is designated as the
* management account for the organization.
*
*
* @param masterAccountEmail
* The email address that is associated with the Amazon Web Services account that is designated as the
* management account for the organization.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder masterAccountEmail(String masterAccountEmail);
/**
*
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in your
* organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
*
*
* @param availablePolicyTypes
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in
* your organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder availablePolicyTypes(Collection availablePolicyTypes);
/**
*
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in your
* organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
*
*
* @param availablePolicyTypes
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in
* your organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder availablePolicyTypes(PolicyTypeSummary... availablePolicyTypes);
/**
*
*
* Do not use. This field is deprecated and doesn't provide complete information about the policies in your
* organization.
*
*
*
* To determine the policies that are enabled and available for use in your organization, use the
* ListRoots operation instead.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.organizations.model.PolicyTypeSummary.Builder} avoiding the need to
* create one manually via
* {@link software.amazon.awssdk.services.organizations.model.PolicyTypeSummary#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.organizations.model.PolicyTypeSummary.Builder#build()} is called
* immediately and its result is passed to {@link #availablePolicyTypes(List)}.
*
* @param availablePolicyTypes
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.organizations.model.PolicyTypeSummary.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #availablePolicyTypes(java.util.Collection)
*/
Builder availablePolicyTypes(Consumer... availablePolicyTypes);
}
static final class BuilderImpl implements Builder {
private String id;
private String arn;
private String featureSet;
private String masterAccountArn;
private String masterAccountId;
private String masterAccountEmail;
private List availablePolicyTypes = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(Organization model) {
id(model.id);
arn(model.arn);
featureSet(model.featureSet);
masterAccountArn(model.masterAccountArn);
masterAccountId(model.masterAccountId);
masterAccountEmail(model.masterAccountEmail);
availablePolicyTypes(model.availablePolicyTypes);
}
public final String getId() {
return id;
}
public final void setId(String id) {
this.id = id;
}
@Override
public final Builder id(String id) {
this.id = id;
return this;
}
public final String getArn() {
return arn;
}
public final void setArn(String arn) {
this.arn = arn;
}
@Override
public final Builder arn(String arn) {
this.arn = arn;
return this;
}
public final String getFeatureSet() {
return featureSet;
}
public final void setFeatureSet(String featureSet) {
this.featureSet = featureSet;
}
@Override
public final Builder featureSet(String featureSet) {
this.featureSet = featureSet;
return this;
}
@Override
public final Builder featureSet(OrganizationFeatureSet featureSet) {
this.featureSet(featureSet == null ? null : featureSet.toString());
return this;
}
public final String getMasterAccountArn() {
return masterAccountArn;
}
public final void setMasterAccountArn(String masterAccountArn) {
this.masterAccountArn = masterAccountArn;
}
@Override
public final Builder masterAccountArn(String masterAccountArn) {
this.masterAccountArn = masterAccountArn;
return this;
}
public final String getMasterAccountId() {
return masterAccountId;
}
public final void setMasterAccountId(String masterAccountId) {
this.masterAccountId = masterAccountId;
}
@Override
public final Builder masterAccountId(String masterAccountId) {
this.masterAccountId = masterAccountId;
return this;
}
public final String getMasterAccountEmail() {
return masterAccountEmail;
}
public final void setMasterAccountEmail(String masterAccountEmail) {
this.masterAccountEmail = masterAccountEmail;
}
@Override
public final Builder masterAccountEmail(String masterAccountEmail) {
this.masterAccountEmail = masterAccountEmail;
return this;
}
public final List getAvailablePolicyTypes() {
List result = PolicyTypesCopier.copyToBuilder(this.availablePolicyTypes);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setAvailablePolicyTypes(Collection availablePolicyTypes) {
this.availablePolicyTypes = PolicyTypesCopier.copyFromBuilder(availablePolicyTypes);
}
@Override
public final Builder availablePolicyTypes(Collection availablePolicyTypes) {
this.availablePolicyTypes = PolicyTypesCopier.copy(availablePolicyTypes);
return this;
}
@Override
@SafeVarargs
public final Builder availablePolicyTypes(PolicyTypeSummary... availablePolicyTypes) {
availablePolicyTypes(Arrays.asList(availablePolicyTypes));
return this;
}
@Override
@SafeVarargs
public final Builder availablePolicyTypes(Consumer... availablePolicyTypes) {
availablePolicyTypes(Stream.of(availablePolicyTypes).map(c -> PolicyTypeSummary.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
@Override
public Organization build() {
return new Organization(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}