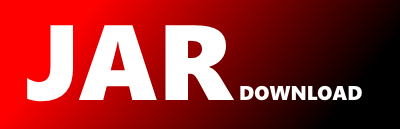
software.amazon.awssdk.services.organizations.model.CreateGovCloudAccountRequest Maven / Gradle / Ivy
Show all versions of organizations Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.organizations.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateGovCloudAccountRequest extends OrganizationsRequest implements
ToCopyableBuilder {
private static final SdkField EMAIL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateGovCloudAccountRequest::email)).setter(setter(Builder::email))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Email").build()).build();
private static final SdkField ACCOUNT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateGovCloudAccountRequest::accountName)).setter(setter(Builder::accountName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AccountName").build()).build();
private static final SdkField ROLE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateGovCloudAccountRequest::roleName)).setter(setter(Builder::roleName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RoleName").build()).build();
private static final SdkField IAM_USER_ACCESS_TO_BILLING_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateGovCloudAccountRequest::iamUserAccessToBillingAsString))
.setter(setter(Builder::iamUserAccessToBilling))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IamUserAccessToBilling").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(EMAIL_FIELD,
ACCOUNT_NAME_FIELD, ROLE_NAME_FIELD, IAM_USER_ACCESS_TO_BILLING_FIELD));
private final String email;
private final String accountName;
private final String roleName;
private final String iamUserAccessToBilling;
private CreateGovCloudAccountRequest(BuilderImpl builder) {
super(builder);
this.email = builder.email;
this.accountName = builder.accountName;
this.roleName = builder.roleName;
this.iamUserAccessToBilling = builder.iamUserAccessToBilling;
}
/**
*
* The email address of the owner to assign to the new member account in the commercial Region. This email address
* must not already be associated with another AWS account. You must use a valid email address to complete account
* creation. You can't access the root user of the account or remove an account that was created with an invalid
* email address. Like all request parameters for CreateGovCloudAccount
, the request for the email
* address for the AWS GovCloud (US) account originates from the commercial Region, not from the AWS GovCloud (US)
* Region.
*
*
* @return The email address of the owner to assign to the new member account in the commercial Region. This email
* address must not already be associated with another AWS account. You must use a valid email address to
* complete account creation. You can't access the root user of the account or remove an account that was
* created with an invalid email address. Like all request parameters for CreateGovCloudAccount
* , the request for the email address for the AWS GovCloud (US) account originates from the commercial
* Region, not from the AWS GovCloud (US) Region.
*/
public String email() {
return email;
}
/**
*
* The friendly name of the member account.
*
*
* @return The friendly name of the member account.
*/
public String accountName() {
return accountName;
}
/**
*
* (Optional)
*
*
* The name of an IAM role that AWS Organizations automatically preconfigures in the new member accounts in both the
* AWS GovCloud (US) Region and in the commercial Region. This role trusts the master account, allowing users in the
* master account to assume the role, as permitted by the master account administrator. The role has administrator
* permissions in the new member account.
*
*
* If you don't specify this parameter, the role name defaults to OrganizationAccountAccessRole
.
*
*
* For more information about how to use this role to access the member account, see Accessing and Administering the Member Accounts in Your Organization in the AWS Organizations User
* Guide and steps 2 and 3 in Tutorial: Delegate
* Access Across AWS Accounts Using IAM Roles in the IAM User Guide.
*
*
* The regex pattern that is used to validate this parameter is a
* string of characters that can consist of uppercase letters, lowercase letters, digits with no spaces, and any of
* the following characters: =,.@-
*
*
* @return (Optional)
*
* The name of an IAM role that AWS Organizations automatically preconfigures in the new member accounts in
* both the AWS GovCloud (US) Region and in the commercial Region. This role trusts the master account,
* allowing users in the master account to assume the role, as permitted by the master account
* administrator. The role has administrator permissions in the new member account.
*
*
* If you don't specify this parameter, the role name defaults to OrganizationAccountAccessRole
* .
*
*
* For more information about how to use this role to access the member account, see Accessing and Administering the Member Accounts in Your Organization in the AWS Organizations
* User Guide and steps 2 and 3 in Tutorial:
* Delegate Access Across AWS Accounts Using IAM Roles in the IAM User Guide.
*
*
* The regex pattern that is used to validate this parameter
* is a string of characters that can consist of uppercase letters, lowercase letters, digits with no
* spaces, and any of the following characters: =,.@-
*/
public String roleName() {
return roleName;
}
/**
*
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access account
* billing information if they have the required permissions. If set to DENY
, only the root user
* of the new account can access account billing information. For more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost Management User
* Guide.
*
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles with the
* required permissions can access billing information for the new account.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #iamUserAccessToBilling} will return {@link IAMUserAccessToBilling#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #iamUserAccessToBillingAsString}.
*
*
* @return If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access
* account billing information if they have the required permissions. If set to DENY
,
* only the root user of the new account can access account billing information. For more information, see
* Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost
* Management User Guide.
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles
* with the required permissions can access billing information for the new account.
* @see IAMUserAccessToBilling
*/
public IAMUserAccessToBilling iamUserAccessToBilling() {
return IAMUserAccessToBilling.fromValue(iamUserAccessToBilling);
}
/**
*
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access account
* billing information if they have the required permissions. If set to DENY
, only the root user
* of the new account can access account billing information. For more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost Management User
* Guide.
*
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles with the
* required permissions can access billing information for the new account.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #iamUserAccessToBilling} will return {@link IAMUserAccessToBilling#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #iamUserAccessToBillingAsString}.
*
*
* @return If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access
* account billing information if they have the required permissions. If set to DENY
,
* only the root user of the new account can access account billing information. For more information, see
* Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost
* Management User Guide.
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles
* with the required permissions can access billing information for the new account.
* @see IAMUserAccessToBilling
*/
public String iamUserAccessToBillingAsString() {
return iamUserAccessToBilling;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(email());
hashCode = 31 * hashCode + Objects.hashCode(accountName());
hashCode = 31 * hashCode + Objects.hashCode(roleName());
hashCode = 31 * hashCode + Objects.hashCode(iamUserAccessToBillingAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateGovCloudAccountRequest)) {
return false;
}
CreateGovCloudAccountRequest other = (CreateGovCloudAccountRequest) obj;
return Objects.equals(email(), other.email()) && Objects.equals(accountName(), other.accountName())
&& Objects.equals(roleName(), other.roleName())
&& Objects.equals(iamUserAccessToBillingAsString(), other.iamUserAccessToBillingAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateGovCloudAccountRequest")
.add("Email", email() == null ? null : "*** Sensitive Data Redacted ***")
.add("AccountName", accountName() == null ? null : "*** Sensitive Data Redacted ***").add("RoleName", roleName())
.add("IamUserAccessToBilling", iamUserAccessToBillingAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Email":
return Optional.ofNullable(clazz.cast(email()));
case "AccountName":
return Optional.ofNullable(clazz.cast(accountName()));
case "RoleName":
return Optional.ofNullable(clazz.cast(roleName()));
case "IamUserAccessToBilling":
return Optional.ofNullable(clazz.cast(iamUserAccessToBillingAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* The name of an IAM role that AWS Organizations automatically preconfigures in the new member accounts
* in both the AWS GovCloud (US) Region and in the commercial Region. This role trusts the master
* account, allowing users in the master account to assume the role, as permitted by the master account
* administrator. The role has administrator permissions in the new member account.
*
*
* If you don't specify this parameter, the role name defaults to
* OrganizationAccountAccessRole
.
*
*
* For more information about how to use this role to access the member account, see Accessing and Administering the Member Accounts in Your Organization in the AWS Organizations
* User Guide and steps 2 and 3 in Tutorial: Delegate Access Across AWS Accounts Using IAM Roles in the IAM User Guide.
*
*
* The regex pattern that is used to validate this
* parameter is a string of characters that can consist of uppercase letters, lowercase letters, digits
* with no spaces, and any of the following characters: =,.@-
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder roleName(String roleName);
/**
*
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access
* account billing information if they have the required permissions. If set to DENY
, only
* the root user of the new account can access account billing information. For more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost Management
* User Guide.
*
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles with
* the required permissions can access billing information for the new account.
*
*
* @param iamUserAccessToBilling
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to
* access account billing information if they have the required permissions. If set to
* DENY
, only the root user of the new account can access account billing information. For
* more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost
* Management User Guide.
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles
* with the required permissions can access billing information for the new account.
* @see IAMUserAccessToBilling
* @return Returns a reference to this object so that method calls can be chained together.
* @see IAMUserAccessToBilling
*/
Builder iamUserAccessToBilling(String iamUserAccessToBilling);
/**
*
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to access
* account billing information if they have the required permissions. If set to DENY
, only
* the root user of the new account can access account billing information. For more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost Management
* User Guide.
*
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles with
* the required permissions can access billing information for the new account.
*
*
* @param iamUserAccessToBilling
* If set to ALLOW
, the new linked account in the commercial Region enables IAM users to
* access account billing information if they have the required permissions. If set to
* DENY
, only the root user of the new account can access account billing information. For
* more information, see Activating Access to the Billing and Cost Management Console in the AWS Billing and Cost
* Management User Guide.
*
* If you don't specify this parameter, the value defaults to ALLOW
, and IAM users and roles
* with the required permissions can access billing information for the new account.
* @see IAMUserAccessToBilling
* @return Returns a reference to this object so that method calls can be chained together.
* @see IAMUserAccessToBilling
*/
Builder iamUserAccessToBilling(IAMUserAccessToBilling iamUserAccessToBilling);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends OrganizationsRequest.BuilderImpl implements Builder {
private String email;
private String accountName;
private String roleName;
private String iamUserAccessToBilling;
private BuilderImpl() {
}
private BuilderImpl(CreateGovCloudAccountRequest model) {
super(model);
email(model.email);
accountName(model.accountName);
roleName(model.roleName);
iamUserAccessToBilling(model.iamUserAccessToBilling);
}
public final String getEmail() {
return email;
}
@Override
public final Builder email(String email) {
this.email = email;
return this;
}
public final void setEmail(String email) {
this.email = email;
}
public final String getAccountName() {
return accountName;
}
@Override
public final Builder accountName(String accountName) {
this.accountName = accountName;
return this;
}
public final void setAccountName(String accountName) {
this.accountName = accountName;
}
public final String getRoleName() {
return roleName;
}
@Override
public final Builder roleName(String roleName) {
this.roleName = roleName;
return this;
}
public final void setRoleName(String roleName) {
this.roleName = roleName;
}
public final String getIamUserAccessToBillingAsString() {
return iamUserAccessToBilling;
}
@Override
public final Builder iamUserAccessToBilling(String iamUserAccessToBilling) {
this.iamUserAccessToBilling = iamUserAccessToBilling;
return this;
}
@Override
public final Builder iamUserAccessToBilling(IAMUserAccessToBilling iamUserAccessToBilling) {
this.iamUserAccessToBilling(iamUserAccessToBilling.toString());
return this;
}
public final void setIamUserAccessToBilling(String iamUserAccessToBilling) {
this.iamUserAccessToBilling = iamUserAccessToBilling;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateGovCloudAccountRequest build() {
return new CreateGovCloudAccountRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}