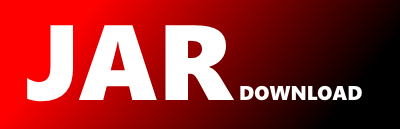
software.amazon.awssdk.services.outposts.OutpostsAsyncClient Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.outposts.model.CancelOrderRequest;
import software.amazon.awssdk.services.outposts.model.CancelOrderResponse;
import software.amazon.awssdk.services.outposts.model.CreateOrderRequest;
import software.amazon.awssdk.services.outposts.model.CreateOrderResponse;
import software.amazon.awssdk.services.outposts.model.CreateOutpostRequest;
import software.amazon.awssdk.services.outposts.model.CreateOutpostResponse;
import software.amazon.awssdk.services.outposts.model.CreateSiteRequest;
import software.amazon.awssdk.services.outposts.model.CreateSiteResponse;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostRequest;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostResponse;
import software.amazon.awssdk.services.outposts.model.DeleteSiteRequest;
import software.amazon.awssdk.services.outposts.model.DeleteSiteResponse;
import software.amazon.awssdk.services.outposts.model.GetCatalogItemRequest;
import software.amazon.awssdk.services.outposts.model.GetCatalogItemResponse;
import software.amazon.awssdk.services.outposts.model.GetOrderRequest;
import software.amazon.awssdk.services.outposts.model.GetOrderResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostResponse;
import software.amazon.awssdk.services.outposts.model.GetSiteAddressRequest;
import software.amazon.awssdk.services.outposts.model.GetSiteAddressResponse;
import software.amazon.awssdk.services.outposts.model.GetSiteRequest;
import software.amazon.awssdk.services.outposts.model.GetSiteResponse;
import software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest;
import software.amazon.awssdk.services.outposts.model.ListCatalogItemsResponse;
import software.amazon.awssdk.services.outposts.model.ListOrdersRequest;
import software.amazon.awssdk.services.outposts.model.ListOrdersResponse;
import software.amazon.awssdk.services.outposts.model.ListOutpostsRequest;
import software.amazon.awssdk.services.outposts.model.ListOutpostsResponse;
import software.amazon.awssdk.services.outposts.model.ListSitesRequest;
import software.amazon.awssdk.services.outposts.model.ListSitesResponse;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.outposts.model.TagResourceRequest;
import software.amazon.awssdk.services.outposts.model.TagResourceResponse;
import software.amazon.awssdk.services.outposts.model.UntagResourceRequest;
import software.amazon.awssdk.services.outposts.model.UntagResourceResponse;
import software.amazon.awssdk.services.outposts.model.UpdateOutpostRequest;
import software.amazon.awssdk.services.outposts.model.UpdateOutpostResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteAddressRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteAddressResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRackPhysicalPropertiesResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteResponse;
import software.amazon.awssdk.services.outposts.paginators.ListCatalogItemsPublisher;
import software.amazon.awssdk.services.outposts.paginators.ListOrdersPublisher;
import software.amazon.awssdk.services.outposts.paginators.ListOutpostsPublisher;
import software.amazon.awssdk.services.outposts.paginators.ListSitesPublisher;
/**
* Service client for accessing Outposts asynchronously. This can be created using the static {@link #builder()} method.
*
*
* Amazon Web Services Outposts is a fully managed service that extends Amazon Web Services infrastructure, APIs, and
* tools to customer premises. By providing local access to Amazon Web Services managed infrastructure, Amazon Web
* Services Outposts enables customers to build and run applications on premises using the same programming interfaces
* as in Amazon Web Services Regions, while using local compute and storage resources for lower latency and local data
* processing needs.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface OutpostsAsyncClient extends SdkClient {
String SERVICE_NAME = "outposts";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "outposts";
/**
* Create a {@link OutpostsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static OutpostsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link OutpostsAsyncClient}.
*/
static OutpostsAsyncClientBuilder builder() {
return new DefaultOutpostsAsyncClientBuilder();
}
/**
*
* Cancels an order for an Outpost.
*
*
* @param cancelOrderRequest
* @return A Java Future containing the result of the CancelOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CancelOrder
* @see AWS API
* Documentation
*/
default CompletableFuture cancelOrder(CancelOrderRequest cancelOrderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Cancels an order for an Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link CancelOrderRequest.Builder} avoiding the need to
* create one manually via {@link CancelOrderRequest#builder()}
*
*
* @param cancelOrderRequest
* A {@link Consumer} that will call methods on {@link CancelOrderInput.Builder} to create a request.
* @return A Java Future containing the result of the CancelOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CancelOrder
* @see AWS API
* Documentation
*/
default CompletableFuture cancelOrder(Consumer cancelOrderRequest) {
return cancelOrder(CancelOrderRequest.builder().applyMutation(cancelOrderRequest).build());
}
/**
*
* Creates an order for an Outpost.
*
*
* @param createOrderRequest
* @return A Java Future containing the result of the CreateOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOrder
* @see AWS API
* Documentation
*/
default CompletableFuture createOrder(CreateOrderRequest createOrderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an order for an Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOrderRequest.Builder} avoiding the need to
* create one manually via {@link CreateOrderRequest#builder()}
*
*
* @param createOrderRequest
* A {@link Consumer} that will call methods on {@link CreateOrderInput.Builder} to create a request.
* @return A Java Future containing the result of the CreateOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOrder
* @see AWS API
* Documentation
*/
default CompletableFuture createOrder(Consumer createOrderRequest) {
return createOrder(CreateOrderRequest.builder().applyMutation(createOrderRequest).build());
}
/**
*
* Creates an Outpost.
*
*
* You can specify AvailabilityZone
or AvailabilityZoneId
.
*
*
* @param createOutpostRequest
* @return A Java Future containing the result of the CreateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture createOutpost(CreateOutpostRequest createOutpostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Outpost.
*
*
* You can specify AvailabilityZone
or AvailabilityZoneId
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOutpostRequest.Builder} avoiding the need to
* create one manually via {@link CreateOutpostRequest#builder()}
*
*
* @param createOutpostRequest
* A {@link Consumer} that will call methods on {@link CreateOutpostInput.Builder} to create a request.
* @return A Java Future containing the result of the CreateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture createOutpost(Consumer createOutpostRequest) {
return createOutpost(CreateOutpostRequest.builder().applyMutation(createOutpostRequest).build());
}
/**
*
* Creates a site for an Outpost.
*
*
* @param createSiteRequest
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(CreateSiteRequest createSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a site for an Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSiteRequest.Builder} avoiding the need to
* create one manually via {@link CreateSiteRequest#builder()}
*
*
* @param createSiteRequest
* A {@link Consumer} that will call methods on {@link CreateSiteInput.Builder} to create a request.
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
default CompletableFuture createSite(Consumer createSiteRequest) {
return createSite(CreateSiteRequest.builder().applyMutation(createSiteRequest).build());
}
/**
*
* Deletes the Outpost.
*
*
* @param deleteOutpostRequest
* @return A Java Future containing the result of the DeleteOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOutpost(DeleteOutpostRequest deleteOutpostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteOutpostRequest.Builder} avoiding the need to
* create one manually via {@link DeleteOutpostRequest#builder()}
*
*
* @param deleteOutpostRequest
* A {@link Consumer} that will call methods on {@link DeleteOutpostInput.Builder} to create a request.
* @return A Java Future containing the result of the DeleteOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture deleteOutpost(Consumer deleteOutpostRequest) {
return deleteOutpost(DeleteOutpostRequest.builder().applyMutation(deleteOutpostRequest).build());
}
/**
*
* Deletes the site.
*
*
* @param deleteSiteRequest
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(DeleteSiteRequest deleteSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the site.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSiteRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSiteRequest#builder()}
*
*
* @param deleteSiteRequest
* A {@link Consumer} that will call methods on {@link DeleteSiteInput.Builder} to create a request.
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSite(Consumer deleteSiteRequest) {
return deleteSite(DeleteSiteRequest.builder().applyMutation(deleteSiteRequest).build());
}
/**
*
* Gets information about a catalog item.
*
*
* @param getCatalogItemRequest
* @return A Java Future containing the result of the GetCatalogItem operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetCatalogItem
* @see AWS API
* Documentation
*/
default CompletableFuture getCatalogItem(GetCatalogItemRequest getCatalogItemRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a catalog item.
*
*
*
* This is a convenience which creates an instance of the {@link GetCatalogItemRequest.Builder} avoiding the need to
* create one manually via {@link GetCatalogItemRequest#builder()}
*
*
* @param getCatalogItemRequest
* A {@link Consumer} that will call methods on {@link GetCatalogItemInput.Builder} to create a request.
* @return A Java Future containing the result of the GetCatalogItem operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetCatalogItem
* @see AWS API
* Documentation
*/
default CompletableFuture getCatalogItem(Consumer getCatalogItemRequest) {
return getCatalogItem(GetCatalogItemRequest.builder().applyMutation(getCatalogItemRequest).build());
}
/**
*
* Gets an order.
*
*
* @param getOrderRequest
* @return A Java Future containing the result of the GetOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOrder
* @see AWS API
* Documentation
*/
default CompletableFuture getOrder(GetOrderRequest getOrderRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets an order.
*
*
*
* This is a convenience which creates an instance of the {@link GetOrderRequest.Builder} avoiding the need to
* create one manually via {@link GetOrderRequest#builder()}
*
*
* @param getOrderRequest
* A {@link Consumer} that will call methods on {@link GetOrderInput.Builder} to create a request.
* @return A Java Future containing the result of the GetOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOrder
* @see AWS API
* Documentation
*/
default CompletableFuture getOrder(Consumer getOrderRequest) {
return getOrder(GetOrderRequest.builder().applyMutation(getOrderRequest).build());
}
/**
*
* Gets information about the specified Outpost.
*
*
* @param getOutpostRequest
* @return A Java Future containing the result of the GetOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture getOutpost(GetOutpostRequest getOutpostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link GetOutpostRequest.Builder} avoiding the need to
* create one manually via {@link GetOutpostRequest#builder()}
*
*
* @param getOutpostRequest
* A {@link Consumer} that will call methods on {@link GetOutpostInput.Builder} to create a request.
* @return A Java Future containing the result of the GetOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture getOutpost(Consumer getOutpostRequest) {
return getOutpost(GetOutpostRequest.builder().applyMutation(getOutpostRequest).build());
}
/**
*
* Lists the instance types for the specified Outpost.
*
*
* @param getOutpostInstanceTypesRequest
* @return A Java Future containing the result of the GetOutpostInstanceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
default CompletableFuture getOutpostInstanceTypes(
GetOutpostInstanceTypesRequest getOutpostInstanceTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance types for the specified Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link GetOutpostInstanceTypesRequest.Builder} avoiding
* the need to create one manually via {@link GetOutpostInstanceTypesRequest#builder()}
*
*
* @param getOutpostInstanceTypesRequest
* A {@link Consumer} that will call methods on {@link GetOutpostInstanceTypesInput.Builder} to create a
* request.
* @return A Java Future containing the result of the GetOutpostInstanceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
default CompletableFuture getOutpostInstanceTypes(
Consumer getOutpostInstanceTypesRequest) {
return getOutpostInstanceTypes(GetOutpostInstanceTypesRequest.builder().applyMutation(getOutpostInstanceTypesRequest)
.build());
}
/**
*
* Gets information about the specified Outpost site.
*
*
* @param getSiteRequest
* @return A Java Future containing the result of the GetSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSite
* @see AWS API
* Documentation
*/
default CompletableFuture getSite(GetSiteRequest getSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified Outpost site.
*
*
*
* This is a convenience which creates an instance of the {@link GetSiteRequest.Builder} avoiding the need to create
* one manually via {@link GetSiteRequest#builder()}
*
*
* @param getSiteRequest
* A {@link Consumer} that will call methods on {@link GetSiteInput.Builder} to create a request.
* @return A Java Future containing the result of the GetSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSite
* @see AWS API
* Documentation
*/
default CompletableFuture getSite(Consumer getSiteRequest) {
return getSite(GetSiteRequest.builder().applyMutation(getSiteRequest).build());
}
/**
*
* Gets the site address.
*
*
* @param getSiteAddressRequest
* @return A Java Future containing the result of the GetSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSiteAddress
* @see AWS API
* Documentation
*/
default CompletableFuture getSiteAddress(GetSiteAddressRequest getSiteAddressRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the site address.
*
*
*
* This is a convenience which creates an instance of the {@link GetSiteAddressRequest.Builder} avoiding the need to
* create one manually via {@link GetSiteAddressRequest#builder()}
*
*
* @param getSiteAddressRequest
* A {@link Consumer} that will call methods on {@link GetSiteAddressInput.Builder} to create a request.
* @return A Java Future containing the result of the GetSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSiteAddress
* @see AWS API
* Documentation
*/
default CompletableFuture getSiteAddress(Consumer getSiteAddressRequest) {
return getSiteAddress(GetSiteAddressRequest.builder().applyMutation(getSiteAddressRequest).build());
}
/**
*
* Use to create a list of every item in the catalog. Add filters to your request to return a more specific list of
* results. Use filters to match an item class, storage option, or EC2 family.
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
* @param listCatalogItemsRequest
* @return A Java Future containing the result of the ListCatalogItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCatalogItems
* @see AWS API
* Documentation
*/
default CompletableFuture listCatalogItems(ListCatalogItemsRequest listCatalogItemsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use to create a list of every item in the catalog. Add filters to your request to return a more specific list of
* results. Use filters to match an item class, storage option, or EC2 family.
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a convenience which creates an instance of the {@link ListCatalogItemsRequest.Builder} avoiding the need
* to create one manually via {@link ListCatalogItemsRequest#builder()}
*
*
* @param listCatalogItemsRequest
* A {@link Consumer} that will call methods on {@link ListCatalogItemsInput.Builder} to create a request.
* @return A Java Future containing the result of the ListCatalogItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCatalogItems
* @see AWS API
* Documentation
*/
default CompletableFuture listCatalogItems(
Consumer listCatalogItemsRequest) {
return listCatalogItems(ListCatalogItemsRequest.builder().applyMutation(listCatalogItemsRequest).build());
}
/**
*
* Use to create a list of every item in the catalog. Add filters to your request to return a more specific list of
* results. Use filters to match an item class, storage option, or EC2 family.
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of
* {@link #listCatalogItems(software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListCatalogItemsPublisher publisher = client.listCatalogItemsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListCatalogItemsPublisher publisher = client.listCatalogItemsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListCatalogItemsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCatalogItems(software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest)} operation.
*
*
* @param listCatalogItemsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCatalogItems
* @see AWS API
* Documentation
*/
default ListCatalogItemsPublisher listCatalogItemsPaginator(ListCatalogItemsRequest listCatalogItemsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Use to create a list of every item in the catalog. Add filters to your request to return a more specific list of
* results. Use filters to match an item class, storage option, or EC2 family.
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of
* {@link #listCatalogItems(software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListCatalogItemsPublisher publisher = client.listCatalogItemsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListCatalogItemsPublisher publisher = client.listCatalogItemsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListCatalogItemsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listCatalogItems(software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListCatalogItemsRequest.Builder} avoiding the need
* to create one manually via {@link ListCatalogItemsRequest#builder()}
*
*
* @param listCatalogItemsRequest
* A {@link Consumer} that will call methods on {@link ListCatalogItemsInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCatalogItems
* @see AWS API
* Documentation
*/
default ListCatalogItemsPublisher listCatalogItemsPaginator(Consumer listCatalogItemsRequest) {
return listCatalogItemsPaginator(ListCatalogItemsRequest.builder().applyMutation(listCatalogItemsRequest).build());
}
/**
*
* Create a list of the Outpost orders for your Amazon Web Services account. You can filter your request by Outpost
* to return a more specific list of results.
*
*
* @param listOrdersRequest
* @return A Java Future containing the result of the ListOrders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOrders
* @see AWS API
* Documentation
*/
default CompletableFuture listOrders(ListOrdersRequest listOrdersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outpost orders for your Amazon Web Services account. You can filter your request by Outpost
* to return a more specific list of results.
*
*
*
* This is a convenience which creates an instance of the {@link ListOrdersRequest.Builder} avoiding the need to
* create one manually via {@link ListOrdersRequest#builder()}
*
*
* @param listOrdersRequest
* A {@link Consumer} that will call methods on {@link ListOrdersInput.Builder} to create a request.
* @return A Java Future containing the result of the ListOrders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOrders
* @see AWS API
* Documentation
*/
default CompletableFuture listOrders(Consumer listOrdersRequest) {
return listOrders(ListOrdersRequest.builder().applyMutation(listOrdersRequest).build());
}
/**
*
* Create a list of the Outpost orders for your Amazon Web Services account. You can filter your request by Outpost
* to return a more specific list of results.
*
*
*
* This is a variant of {@link #listOrders(software.amazon.awssdk.services.outposts.model.ListOrdersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOrdersPublisher publisher = client.listOrdersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOrdersPublisher publisher = client.listOrdersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListOrdersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOrders(software.amazon.awssdk.services.outposts.model.ListOrdersRequest)} operation.
*
*
* @param listOrdersRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOrders
* @see AWS API
* Documentation
*/
default ListOrdersPublisher listOrdersPaginator(ListOrdersRequest listOrdersRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outpost orders for your Amazon Web Services account. You can filter your request by Outpost
* to return a more specific list of results.
*
*
*
* This is a variant of {@link #listOrders(software.amazon.awssdk.services.outposts.model.ListOrdersRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOrdersPublisher publisher = client.listOrdersPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOrdersPublisher publisher = client.listOrdersPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListOrdersResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOrders(software.amazon.awssdk.services.outposts.model.ListOrdersRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListOrdersRequest.Builder} avoiding the need to
* create one manually via {@link ListOrdersRequest#builder()}
*
*
* @param listOrdersRequest
* A {@link Consumer} that will call methods on {@link ListOrdersInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOrders
* @see AWS API
* Documentation
*/
default ListOrdersPublisher listOrdersPaginator(Consumer listOrdersRequest) {
return listOrdersPaginator(ListOrdersRequest.builder().applyMutation(listOrdersRequest).build());
}
/**
*
* Create a list of the Outposts for your Amazon Web Services account. Add filters to your request to return a more
* specific list of results. Use filters to match an Outpost lifecycle status, Availability Zone (
* us-east-1a
), and AZ ID (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
* @param listOutpostsRequest
* @return A Java Future containing the result of the ListOutposts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOutposts
* @see AWS API
* Documentation
*/
default CompletableFuture listOutposts(ListOutpostsRequest listOutpostsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outposts for your Amazon Web Services account. Add filters to your request to return a more
* specific list of results. Use filters to match an Outpost lifecycle status, Availability Zone (
* us-east-1a
), and AZ ID (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a convenience which creates an instance of the {@link ListOutpostsRequest.Builder} avoiding the need to
* create one manually via {@link ListOutpostsRequest#builder()}
*
*
* @param listOutpostsRequest
* A {@link Consumer} that will call methods on {@link ListOutpostsInput.Builder} to create a request.
* @return A Java Future containing the result of the ListOutposts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOutposts
* @see AWS API
* Documentation
*/
default CompletableFuture listOutposts(Consumer listOutpostsRequest) {
return listOutposts(ListOutpostsRequest.builder().applyMutation(listOutpostsRequest).build());
}
/**
*
* Create a list of the Outposts for your Amazon Web Services account. Add filters to your request to return a more
* specific list of results. Use filters to match an Outpost lifecycle status, Availability Zone (
* us-east-1a
), and AZ ID (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsPublisher publisher = client.listOutpostsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsPublisher publisher = client.listOutpostsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListOutpostsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)} operation.
*
*
* @param listOutpostsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsPublisher listOutpostsPaginator(ListOutpostsRequest listOutpostsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outposts for your Amazon Web Services account. Add filters to your request to return a more
* specific list of results. Use filters to match an Outpost lifecycle status, Availability Zone (
* us-east-1a
), and AZ ID (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsPublisher publisher = client.listOutpostsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsPublisher publisher = client.listOutpostsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListOutpostsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListOutpostsRequest.Builder} avoiding the need to
* create one manually via {@link ListOutpostsRequest#builder()}
*
*
* @param listOutpostsRequest
* A {@link Consumer} that will call methods on {@link ListOutpostsInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsPublisher listOutpostsPaginator(Consumer listOutpostsRequest) {
return listOutpostsPaginator(ListOutpostsRequest.builder().applyMutation(listOutpostsRequest).build());
}
/**
*
* Lists the sites for your Amazon Web Services account.
*
*
* @param listSitesRequest
* @return A Java Future containing the result of the ListSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListSites
* @see AWS API
* Documentation
*/
default CompletableFuture listSites(ListSitesRequest listSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the sites for your Amazon Web Services account.
*
*
*
* This is a convenience which creates an instance of the {@link ListSitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSitesRequest#builder()}
*
*
* @param listSitesRequest
* A {@link Consumer} that will call methods on {@link ListSitesInput.Builder} to create a request.
* @return A Java Future containing the result of the ListSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListSites
* @see AWS API
* Documentation
*/
default CompletableFuture listSites(Consumer listSitesRequest) {
return listSites(ListSitesRequest.builder().applyMutation(listSitesRequest).build());
}
/**
*
* Lists the sites for your Amazon Web Services account.
*
*
*
* This is a variant of {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesPublisher publisher = client.listSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesPublisher publisher = client.listSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)} operation.
*
*
* @param listSitesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesPublisher listSitesPaginator(ListSitesRequest listSitesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the sites for your Amazon Web Services account.
*
*
*
* This is a variant of {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesPublisher publisher = client.listSitesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesPublisher publisher = client.listSitesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.outposts.model.ListSitesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSitesRequest#builder()}
*
*
* @param listSitesRequest
* A {@link Consumer} that will call methods on {@link ListSitesInput.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesPublisher listSitesPaginator(Consumer listSitesRequest) {
return listSitesPaginator(ListSitesRequest.builder().applyMutation(listSitesRequest).build());
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates an Outpost.
*
*
* @param updateOutpostRequest
* @return A Java Future containing the result of the UpdateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture updateOutpost(UpdateOutpostRequest updateOutpostRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateOutpostRequest.Builder} avoiding the need to
* create one manually via {@link UpdateOutpostRequest#builder()}
*
*
* @param updateOutpostRequest
* A {@link Consumer} that will call methods on {@link UpdateOutpostInput.Builder} to create a request.
* @return A Java Future containing the result of the UpdateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateOutpost
* @see AWS API
* Documentation
*/
default CompletableFuture updateOutpost(Consumer updateOutpostRequest) {
return updateOutpost(UpdateOutpostRequest.builder().applyMutation(updateOutpostRequest).build());
}
/**
*
* Updates the site.
*
*
* @param updateSiteRequest
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(UpdateSiteRequest updateSiteRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the site.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSiteRequest.Builder} avoiding the need to
* create one manually via {@link UpdateSiteRequest#builder()}
*
*
* @param updateSiteRequest
* A {@link Consumer} that will call methods on {@link UpdateSiteInput.Builder} to create a request.
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
default CompletableFuture updateSite(Consumer updateSiteRequest) {
return updateSite(UpdateSiteRequest.builder().applyMutation(updateSiteRequest).build());
}
/**
*
* Updates the site address.
*
*
* To update a site address with an order IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* You can update the operating address before you place an order at the site, or after all Outposts that belong to
* the site have been deactivated.
*
*
* @param updateSiteAddressRequest
* @return A Java Future containing the result of the UpdateSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteAddress
* @see AWS
* API Documentation
*/
default CompletableFuture updateSiteAddress(UpdateSiteAddressRequest updateSiteAddressRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates the site address.
*
*
* To update a site address with an order IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* You can update the operating address before you place an order at the site, or after all Outposts that belong to
* the site have been deactivated.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSiteAddressRequest.Builder} avoiding the need
* to create one manually via {@link UpdateSiteAddressRequest#builder()}
*
*
* @param updateSiteAddressRequest
* A {@link Consumer} that will call methods on {@link UpdateSiteAddressInput.Builder} to create a request.
* @return A Java Future containing the result of the UpdateSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteAddress
* @see AWS
* API Documentation
*/
default CompletableFuture updateSiteAddress(
Consumer updateSiteAddressRequest) {
return updateSiteAddress(UpdateSiteAddressRequest.builder().applyMutation(updateSiteAddressRequest).build());
}
/**
*
* Update the physical and logistical details for a rack at a site. For more information about hardware requirements
* for racks, see Network
* readiness checklist in the Amazon Web Services Outposts User Guide.
*
*
* To update a rack at a site with an order of IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* @param updateSiteRackPhysicalPropertiesRequest
* @return A Java Future containing the result of the UpdateSiteRackPhysicalProperties operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteRackPhysicalProperties
* @see AWS API Documentation
*/
default CompletableFuture updateSiteRackPhysicalProperties(
UpdateSiteRackPhysicalPropertiesRequest updateSiteRackPhysicalPropertiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Update the physical and logistical details for a rack at a site. For more information about hardware requirements
* for racks, see Network
* readiness checklist in the Amazon Web Services Outposts User Guide.
*
*
* To update a rack at a site with an order of IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSiteRackPhysicalPropertiesRequest.Builder}
* avoiding the need to create one manually via {@link UpdateSiteRackPhysicalPropertiesRequest#builder()}
*
*
* @param updateSiteRackPhysicalPropertiesRequest
* A {@link Consumer} that will call methods on {@link UpdateSiteRackPhysicalPropertiesInput.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateSiteRackPhysicalProperties operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteRackPhysicalProperties
* @see AWS API Documentation
*/
default CompletableFuture updateSiteRackPhysicalProperties(
Consumer updateSiteRackPhysicalPropertiesRequest) {
return updateSiteRackPhysicalProperties(UpdateSiteRackPhysicalPropertiesRequest.builder()
.applyMutation(updateSiteRackPhysicalPropertiesRequest).build());
}
}