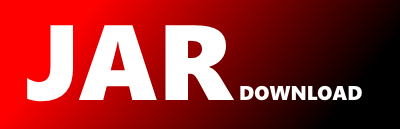
software.amazon.awssdk.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts.model;
import java.beans.Transient;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateSiteRackPhysicalPropertiesRequest extends OutpostsRequest implements
ToCopyableBuilder {
private static final SdkField SITE_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("SiteId")
.getter(getter(UpdateSiteRackPhysicalPropertiesRequest::siteId)).setter(setter(Builder::siteId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("SiteId").build()).build();
private static final SdkField POWER_DRAW_KVA_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerDrawKva").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::powerDrawKvaAsString))
.setter(setter(Builder::powerDrawKva))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerDrawKva").build()).build();
private static final SdkField POWER_PHASE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerPhase").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::powerPhaseAsString))
.setter(setter(Builder::powerPhase))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerPhase").build()).build();
private static final SdkField POWER_CONNECTOR_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerConnector").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::powerConnectorAsString))
.setter(setter(Builder::powerConnector))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerConnector").build()).build();
private static final SdkField POWER_FEED_DROP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("PowerFeedDrop").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::powerFeedDropAsString))
.setter(setter(Builder::powerFeedDrop))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("PowerFeedDrop").build()).build();
private static final SdkField UPLINK_GBPS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UplinkGbps").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::uplinkGbpsAsString))
.setter(setter(Builder::uplinkGbps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UplinkGbps").build()).build();
private static final SdkField UPLINK_COUNT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UplinkCount").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::uplinkCountAsString))
.setter(setter(Builder::uplinkCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UplinkCount").build()).build();
private static final SdkField FIBER_OPTIC_CABLE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FiberOpticCableType")
.getter(getter(UpdateSiteRackPhysicalPropertiesRequest::fiberOpticCableTypeAsString))
.setter(setter(Builder::fiberOpticCableType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("FiberOpticCableType").build())
.build();
private static final SdkField OPTICAL_STANDARD_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OpticalStandard").getter(getter(UpdateSiteRackPhysicalPropertiesRequest::opticalStandardAsString))
.setter(setter(Builder::opticalStandard))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OpticalStandard").build()).build();
private static final SdkField MAXIMUM_SUPPORTED_WEIGHT_LBS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MaximumSupportedWeightLbs")
.getter(getter(UpdateSiteRackPhysicalPropertiesRequest::maximumSupportedWeightLbsAsString))
.setter(setter(Builder::maximumSupportedWeightLbs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MaximumSupportedWeightLbs").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SITE_ID_FIELD,
POWER_DRAW_KVA_FIELD, POWER_PHASE_FIELD, POWER_CONNECTOR_FIELD, POWER_FEED_DROP_FIELD, UPLINK_GBPS_FIELD,
UPLINK_COUNT_FIELD, FIBER_OPTIC_CABLE_TYPE_FIELD, OPTICAL_STANDARD_FIELD, MAXIMUM_SUPPORTED_WEIGHT_LBS_FIELD));
private final String siteId;
private final String powerDrawKva;
private final String powerPhase;
private final String powerConnector;
private final String powerFeedDrop;
private final String uplinkGbps;
private final String uplinkCount;
private final String fiberOpticCableType;
private final String opticalStandard;
private final String maximumSupportedWeightLbs;
private UpdateSiteRackPhysicalPropertiesRequest(BuilderImpl builder) {
super(builder);
this.siteId = builder.siteId;
this.powerDrawKva = builder.powerDrawKva;
this.powerPhase = builder.powerPhase;
this.powerConnector = builder.powerConnector;
this.powerFeedDrop = builder.powerFeedDrop;
this.uplinkGbps = builder.uplinkGbps;
this.uplinkCount = builder.uplinkCount;
this.fiberOpticCableType = builder.fiberOpticCableType;
this.opticalStandard = builder.opticalStandard;
this.maximumSupportedWeightLbs = builder.maximumSupportedWeightLbs;
}
/**
*
* The ID or the Amazon Resource Name (ARN) of the site.
*
*
* @return The ID or the Amazon Resource Name (ARN) of the site.
*/
public final String siteId() {
return siteId;
}
/**
*
* Specify in kVA the power draw available at the hardware placement position for the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerDrawKva} will
* return {@link PowerDrawKva#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerDrawKvaAsString}.
*
*
* @return Specify in kVA the power draw available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public final PowerDrawKva powerDrawKva() {
return PowerDrawKva.fromValue(powerDrawKva);
}
/**
*
* Specify in kVA the power draw available at the hardware placement position for the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerDrawKva} will
* return {@link PowerDrawKva#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerDrawKvaAsString}.
*
*
* @return Specify in kVA the power draw available at the hardware placement position for the rack.
* @see PowerDrawKva
*/
public final String powerDrawKvaAsString() {
return powerDrawKva;
}
/**
*
* Specify the power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerPhase} will
* return {@link PowerPhase#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerPhaseAsString}.
*
*
* @return Specify the power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @see PowerPhase
*/
public final PowerPhase powerPhase() {
return PowerPhase.fromValue(powerPhase);
}
/**
*
* Specify the power option that you can provide for hardware.
*
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerPhase} will
* return {@link PowerPhase#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerPhaseAsString}.
*
*
* @return Specify the power option that you can provide for hardware.
*
* -
*
* Single-phase AC feed: 200 V to 277 V, 50 Hz or 60 Hz
*
*
* -
*
* Three-phase AC feed: 346 V to 480 V, 50 Hz or 60 Hz
*
*
* @see PowerPhase
*/
public final String powerPhaseAsString() {
return powerPhase;
}
/**
*
* Specify the power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerConnector}
* will return {@link PowerConnector#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #powerConnectorAsString}.
*
*
* @return Specify the power connector that Amazon Web Services should plan to provide for connections to the
* hardware. Note the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @see PowerConnector
*/
public final PowerConnector powerConnector() {
return PowerConnector.fromValue(powerConnector);
}
/**
*
* Specify the power connector that Amazon Web Services should plan to provide for connections to the hardware. Note
* the correlation between PowerPhase
and PowerConnector
.
*
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerConnector}
* will return {@link PowerConnector#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #powerConnectorAsString}.
*
*
* @return Specify the power connector that Amazon Web Services should plan to provide for connections to the
* hardware. Note the correlation between PowerPhase
and PowerConnector
.
*
* -
*
* Single-phase AC feed
*
*
* -
*
* L6-30P – (common in US); 30A; single phase
*
*
* -
*
* IEC309 (blue) – P+N+E, 6hr; 32 A; single phase
*
*
*
*
* -
*
* Three-phase AC feed
*
*
* -
*
* AH530P7W (red) – 3P+N+E, 7hr; 30A; three phase
*
*
* -
*
* AH532P6W (red) – 3P+N+E, 6hr; 32A; three phase
*
*
*
*
* @see PowerConnector
*/
public final String powerConnectorAsString() {
return powerConnector;
}
/**
*
* Specify whether the power feed comes above or below the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerFeedDrop}
* will return {@link PowerFeedDrop#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerFeedDropAsString}.
*
*
* @return Specify whether the power feed comes above or below the rack.
* @see PowerFeedDrop
*/
public final PowerFeedDrop powerFeedDrop() {
return PowerFeedDrop.fromValue(powerFeedDrop);
}
/**
*
* Specify whether the power feed comes above or below the rack.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #powerFeedDrop}
* will return {@link PowerFeedDrop#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #powerFeedDropAsString}.
*
*
* @return Specify whether the power feed comes above or below the rack.
* @see PowerFeedDrop
*/
public final String powerFeedDropAsString() {
return powerFeedDrop;
}
/**
*
* Specify the uplink speed the rack should support for the connection to the Region.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkGbps} will
* return {@link UplinkGbps#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkGbpsAsString}.
*
*
* @return Specify the uplink speed the rack should support for the connection to the Region.
* @see UplinkGbps
*/
public final UplinkGbps uplinkGbps() {
return UplinkGbps.fromValue(uplinkGbps);
}
/**
*
* Specify the uplink speed the rack should support for the connection to the Region.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkGbps} will
* return {@link UplinkGbps#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkGbpsAsString}.
*
*
* @return Specify the uplink speed the rack should support for the connection to the Region.
* @see UplinkGbps
*/
public final String uplinkGbpsAsString() {
return uplinkGbps;
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkCount} will
* return {@link UplinkCount#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkCountAsString}.
*
*
* @return Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @see UplinkCount
*/
public final UplinkCount uplinkCount() {
return UplinkCount.fromValue(uplinkCount);
}
/**
*
* Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the Outpost
* network devices provide a variable number of uplinks. Specify the number of uplinks for each Outpost network
* device that you intend to use to connect the rack to your network. Note the correlation between
* UplinkGbps
and UplinkCount
.
*
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #uplinkCount} will
* return {@link UplinkCount#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #uplinkCountAsString}.
*
*
* @return Racks come with two Outpost network devices. Depending on the supported uplink speed at the site, the
* Outpost network devices provide a variable number of uplinks. Specify the number of uplinks for each
* Outpost network device that you intend to use to connect the rack to your network. Note the correlation
* between UplinkGbps
and UplinkCount
.
*
* -
*
* 1Gbps - Uplinks available: 1, 2, 4, 6, 8
*
*
* -
*
* 10Gbps - Uplinks available: 1, 2, 4, 8, 12, 16
*
*
* -
*
* 40 and 100 Gbps- Uplinks available: 1, 2, 4
*
*
* @see UplinkCount
*/
public final String uplinkCountAsString() {
return uplinkCount;
}
/**
*
* Specify the type of fiber that you will use to attach the Outpost to your network.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #fiberOpticCableType} will return {@link FiberOpticCableType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #fiberOpticCableTypeAsString}.
*
*
* @return Specify the type of fiber that you will use to attach the Outpost to your network.
* @see FiberOpticCableType
*/
public final FiberOpticCableType fiberOpticCableType() {
return FiberOpticCableType.fromValue(fiberOpticCableType);
}
/**
*
* Specify the type of fiber that you will use to attach the Outpost to your network.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #fiberOpticCableType} will return {@link FiberOpticCableType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #fiberOpticCableTypeAsString}.
*
*
* @return Specify the type of fiber that you will use to attach the Outpost to your network.
* @see FiberOpticCableType
*/
public final String fiberOpticCableTypeAsString() {
return fiberOpticCableType;
}
/**
*
* Specify the type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking
* requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #opticalStandard}
* will return {@link OpticalStandard#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #opticalStandardAsString}.
*
*
* @return Specify the type of optical standard that you will use to attach the Outpost to your network. This field
* is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @see OpticalStandard
*/
public final OpticalStandard opticalStandard() {
return OpticalStandard.fromValue(opticalStandard);
}
/**
*
* Specify the type of optical standard that you will use to attach the Outpost to your network. This field is
* dependent on uplink speed, fiber type, and distance to the upstream device. For more information about networking
* requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #opticalStandard}
* will return {@link OpticalStandard#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #opticalStandardAsString}.
*
*
* @return Specify the type of optical standard that you will use to attach the Outpost to your network. This field
* is dependent on uplink speed, fiber type, and distance to the upstream device. For more information about
* networking requirements for racks, see Network in the Amazon Web Services Outposts User Guide.
*
* -
*
* OPTIC_10GBASE_SR
: 10GBASE-SR
*
*
* -
*
* OPTIC_10GBASE_IR
: 10GBASE-IR
*
*
* -
*
* OPTIC_10GBASE_LR
: 10GBASE-LR
*
*
* -
*
* OPTIC_40GBASE_SR
: 40GBASE-SR
*
*
* -
*
* OPTIC_40GBASE_ESR
: 40GBASE-ESR
*
*
* -
*
* OPTIC_40GBASE_IR4_LR4L
: 40GBASE-IR (LR4L)
*
*
* -
*
* OPTIC_40GBASE_LR4
: 40GBASE-LR4
*
*
* -
*
* OPTIC_100GBASE_SR4
: 100GBASE-SR4
*
*
* -
*
* OPTIC_100GBASE_CWDM4
: 100GBASE-CWDM4
*
*
* -
*
* OPTIC_100GBASE_LR4
: 100GBASE-LR4
*
*
* -
*
* OPTIC_100G_PSM4_MSA
: 100G PSM4 MSA
*
*
* -
*
* OPTIC_1000BASE_LX
: 1000Base-LX
*
*
* -
*
* OPTIC_1000BASE_SX
: 1000Base-SX
*
*
* @see OpticalStandard
*/
public final String opticalStandardAsString() {
return opticalStandard;
}
/**
*
* Specify the maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #maximumSupportedWeightLbs} will return {@link MaximumSupportedWeightLbs#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #maximumSupportedWeightLbsAsString}.
*
*
* @return Specify the maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @see MaximumSupportedWeightLbs
*/
public final MaximumSupportedWeightLbs maximumSupportedWeightLbs() {
return MaximumSupportedWeightLbs.fromValue(maximumSupportedWeightLbs);
}
/**
*
* Specify the maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #maximumSupportedWeightLbs} will return {@link MaximumSupportedWeightLbs#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #maximumSupportedWeightLbsAsString}.
*
*
* @return Specify the maximum rack weight that this site can support. NO_LIMIT
is over 2000lbs.
* @see MaximumSupportedWeightLbs
*/
public final String maximumSupportedWeightLbsAsString() {
return maximumSupportedWeightLbs;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(siteId());
hashCode = 31 * hashCode + Objects.hashCode(powerDrawKvaAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerPhaseAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerConnectorAsString());
hashCode = 31 * hashCode + Objects.hashCode(powerFeedDropAsString());
hashCode = 31 * hashCode + Objects.hashCode(uplinkGbpsAsString());
hashCode = 31 * hashCode + Objects.hashCode(uplinkCountAsString());
hashCode = 31 * hashCode + Objects.hashCode(fiberOpticCableTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(opticalStandardAsString());
hashCode = 31 * hashCode + Objects.hashCode(maximumSupportedWeightLbsAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateSiteRackPhysicalPropertiesRequest)) {
return false;
}
UpdateSiteRackPhysicalPropertiesRequest other = (UpdateSiteRackPhysicalPropertiesRequest) obj;
return Objects.equals(siteId(), other.siteId()) && Objects.equals(powerDrawKvaAsString(), other.powerDrawKvaAsString())
&& Objects.equals(powerPhaseAsString(), other.powerPhaseAsString())
&& Objects.equals(powerConnectorAsString(), other.powerConnectorAsString())
&& Objects.equals(powerFeedDropAsString(), other.powerFeedDropAsString())
&& Objects.equals(uplinkGbpsAsString(), other.uplinkGbpsAsString())
&& Objects.equals(uplinkCountAsString(), other.uplinkCountAsString())
&& Objects.equals(fiberOpticCableTypeAsString(), other.fiberOpticCableTypeAsString())
&& Objects.equals(opticalStandardAsString(), other.opticalStandardAsString())
&& Objects.equals(maximumSupportedWeightLbsAsString(), other.maximumSupportedWeightLbsAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateSiteRackPhysicalPropertiesRequest").add("SiteId", siteId())
.add("PowerDrawKva", powerDrawKvaAsString()).add("PowerPhase", powerPhaseAsString())
.add("PowerConnector", powerConnectorAsString()).add("PowerFeedDrop", powerFeedDropAsString())
.add("UplinkGbps", uplinkGbpsAsString()).add("UplinkCount", uplinkCountAsString())
.add("FiberOpticCableType", fiberOpticCableTypeAsString()).add("OpticalStandard", opticalStandardAsString())
.add("MaximumSupportedWeightLbs", maximumSupportedWeightLbsAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "SiteId":
return Optional.ofNullable(clazz.cast(siteId()));
case "PowerDrawKva":
return Optional.ofNullable(clazz.cast(powerDrawKvaAsString()));
case "PowerPhase":
return Optional.ofNullable(clazz.cast(powerPhaseAsString()));
case "PowerConnector":
return Optional.ofNullable(clazz.cast(powerConnectorAsString()));
case "PowerFeedDrop":
return Optional.ofNullable(clazz.cast(powerFeedDropAsString()));
case "UplinkGbps":
return Optional.ofNullable(clazz.cast(uplinkGbpsAsString()));
case "UplinkCount":
return Optional.ofNullable(clazz.cast(uplinkCountAsString()));
case "FiberOpticCableType":
return Optional.ofNullable(clazz.cast(fiberOpticCableTypeAsString()));
case "OpticalStandard":
return Optional.ofNullable(clazz.cast(opticalStandardAsString()));
case "MaximumSupportedWeightLbs":
return Optional.ofNullable(clazz.cast(maximumSupportedWeightLbsAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function