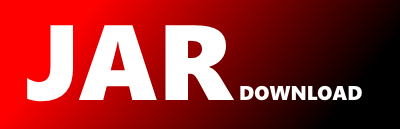
software.amazon.awssdk.services.outposts.OutpostsClient Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.regions.ServiceMetadata;
import software.amazon.awssdk.services.outposts.model.AccessDeniedException;
import software.amazon.awssdk.services.outposts.model.ConflictException;
import software.amazon.awssdk.services.outposts.model.CreateOutpostRequest;
import software.amazon.awssdk.services.outposts.model.CreateOutpostResponse;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostRequest;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostResponse;
import software.amazon.awssdk.services.outposts.model.DeleteSiteRequest;
import software.amazon.awssdk.services.outposts.model.DeleteSiteResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostResponse;
import software.amazon.awssdk.services.outposts.model.InternalServerException;
import software.amazon.awssdk.services.outposts.model.ListOutpostsRequest;
import software.amazon.awssdk.services.outposts.model.ListOutpostsResponse;
import software.amazon.awssdk.services.outposts.model.ListSitesRequest;
import software.amazon.awssdk.services.outposts.model.ListSitesResponse;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.outposts.model.NotFoundException;
import software.amazon.awssdk.services.outposts.model.OutpostsException;
import software.amazon.awssdk.services.outposts.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.outposts.model.TagResourceRequest;
import software.amazon.awssdk.services.outposts.model.TagResourceResponse;
import software.amazon.awssdk.services.outposts.model.UntagResourceRequest;
import software.amazon.awssdk.services.outposts.model.UntagResourceResponse;
import software.amazon.awssdk.services.outposts.model.ValidationException;
import software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable;
import software.amazon.awssdk.services.outposts.paginators.ListSitesIterable;
/**
* Service client for accessing Outposts. This can be created using the static {@link #builder()} method.
*
*
* AWS Outposts is a fully managed service that extends AWS infrastructure, APIs, and tools to customer premises. By
* providing local access to AWS managed infrastructure, AWS Outposts enables customers to build and run applications on
* premises using the same programming interfaces as in AWS Regions, while using local compute and storage resources for
* lower latency and local data processing needs.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface OutpostsClient extends SdkClient {
String SERVICE_NAME = "outposts";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "outposts";
/**
* Create a {@link OutpostsClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static OutpostsClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link OutpostsClient}.
*/
static OutpostsClientBuilder builder() {
return new DefaultOutpostsClientBuilder();
}
/**
*
* Creates an Outpost.
*
*
* You can specify AvailabilityZone
or AvailabilityZoneId
.
*
*
* @param createOutpostRequest
* @return Result of the CreateOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws ServiceQuotaExceededException
* You have exceeded a service quota.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.CreateOutpost
* @see AWS API
* Documentation
*/
default CreateOutpostResponse createOutpost(CreateOutpostRequest createOutpostRequest) throws ValidationException,
ConflictException, NotFoundException, AccessDeniedException, InternalServerException, ServiceQuotaExceededException,
AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Creates an Outpost.
*
*
* You can specify AvailabilityZone
or AvailabilityZoneId
.
*
*
*
* This is a convenience which creates an instance of the {@link CreateOutpostRequest.Builder} avoiding the need to
* create one manually via {@link CreateOutpostRequest#builder()}
*
*
* @param createOutpostRequest
* A {@link Consumer} that will call methods on {@link CreateOutpostInput.Builder} to create a request.
* @return Result of the CreateOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws ServiceQuotaExceededException
* You have exceeded a service quota.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.CreateOutpost
* @see AWS API
* Documentation
*/
default CreateOutpostResponse createOutpost(Consumer createOutpostRequest)
throws ValidationException, ConflictException, NotFoundException, AccessDeniedException, InternalServerException,
ServiceQuotaExceededException, AwsServiceException, SdkClientException, OutpostsException {
return createOutpost(CreateOutpostRequest.builder().applyMutation(createOutpostRequest).build());
}
/**
*
* Deletes the Outpost.
*
*
* @param deleteOutpostRequest
* @return Result of the DeleteOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.DeleteOutpost
* @see AWS API
* Documentation
*/
default DeleteOutpostResponse deleteOutpost(DeleteOutpostRequest deleteOutpostRequest) throws ValidationException,
ConflictException, NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException,
SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteOutpostRequest.Builder} avoiding the need to
* create one manually via {@link DeleteOutpostRequest#builder()}
*
*
* @param deleteOutpostRequest
* A {@link Consumer} that will call methods on {@link DeleteOutpostInput.Builder} to create a request.
* @return Result of the DeleteOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.DeleteOutpost
* @see AWS API
* Documentation
*/
default DeleteOutpostResponse deleteOutpost(Consumer deleteOutpostRequest)
throws ValidationException, ConflictException, NotFoundException, AccessDeniedException, InternalServerException,
AwsServiceException, SdkClientException, OutpostsException {
return deleteOutpost(DeleteOutpostRequest.builder().applyMutation(deleteOutpostRequest).build());
}
/**
*
* Deletes the site.
*
*
* @param deleteSiteRequest
* @return Result of the DeleteSite operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.DeleteSite
* @see AWS API
* Documentation
*/
default DeleteSiteResponse deleteSite(DeleteSiteRequest deleteSiteRequest) throws ValidationException, ConflictException,
NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException,
OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the site.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSiteRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSiteRequest#builder()}
*
*
* @param deleteSiteRequest
* A {@link Consumer} that will call methods on {@link DeleteSiteInput.Builder} to create a request.
* @return Result of the DeleteSite operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws ConflictException
* Updating or deleting this resource can cause an inconsistent state.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.DeleteSite
* @see AWS API
* Documentation
*/
default DeleteSiteResponse deleteSite(Consumer deleteSiteRequest) throws ValidationException,
ConflictException, NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException,
SdkClientException, OutpostsException {
return deleteSite(DeleteSiteRequest.builder().applyMutation(deleteSiteRequest).build());
}
/**
*
* Gets information about the specified Outpost.
*
*
* @param getOutpostRequest
* @return Result of the GetOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.GetOutpost
* @see AWS API
* Documentation
*/
default GetOutpostResponse getOutpost(GetOutpostRequest getOutpostRequest) throws ValidationException, NotFoundException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the specified Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link GetOutpostRequest.Builder} avoiding the need to
* create one manually via {@link GetOutpostRequest#builder()}
*
*
* @param getOutpostRequest
* A {@link Consumer} that will call methods on {@link GetOutpostInput.Builder} to create a request.
* @return Result of the GetOutpost operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.GetOutpost
* @see AWS API
* Documentation
*/
default GetOutpostResponse getOutpost(Consumer getOutpostRequest) throws ValidationException,
NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException,
OutpostsException {
return getOutpost(GetOutpostRequest.builder().applyMutation(getOutpostRequest).build());
}
/**
*
* Lists the instance types for the specified Outpost.
*
*
* @param getOutpostInstanceTypesRequest
* @return Result of the GetOutpostInstanceTypes operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
default GetOutpostInstanceTypesResponse getOutpostInstanceTypes(GetOutpostInstanceTypesRequest getOutpostInstanceTypesRequest)
throws ValidationException, NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException,
SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the instance types for the specified Outpost.
*
*
*
* This is a convenience which creates an instance of the {@link GetOutpostInstanceTypesRequest.Builder} avoiding
* the need to create one manually via {@link GetOutpostInstanceTypesRequest#builder()}
*
*
* @param getOutpostInstanceTypesRequest
* A {@link Consumer} that will call methods on {@link GetOutpostInstanceTypesInput.Builder} to create a
* request.
* @return Result of the GetOutpostInstanceTypes operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
default GetOutpostInstanceTypesResponse getOutpostInstanceTypes(
Consumer getOutpostInstanceTypesRequest) throws ValidationException,
NotFoundException, AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException,
OutpostsException {
return getOutpostInstanceTypes(GetOutpostInstanceTypesRequest.builder().applyMutation(getOutpostInstanceTypesRequest)
.build());
}
/**
*
* Create a list of the Outposts for your AWS account. Add filters to your request to return a more specific list of
* results. Use filters to match an Outpost lifecycle status, Availibility Zone (us-east-1a
), and AZ ID
* (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
* @param listOutpostsRequest
* @return Result of the ListOutposts operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsResponse listOutposts(ListOutpostsRequest listOutpostsRequest) throws ValidationException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outposts for your AWS account. Add filters to your request to return a more specific list of
* results. Use filters to match an Outpost lifecycle status, Availibility Zone (us-east-1a
), and AZ ID
* (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a convenience which creates an instance of the {@link ListOutpostsRequest.Builder} avoiding the need to
* create one manually via {@link ListOutpostsRequest#builder()}
*
*
* @param listOutpostsRequest
* A {@link Consumer} that will call methods on {@link ListOutpostsInput.Builder} to create a request.
* @return Result of the ListOutposts operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsResponse listOutposts(Consumer listOutpostsRequest)
throws ValidationException, AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException,
OutpostsException {
return listOutposts(ListOutpostsRequest.builder().applyMutation(listOutpostsRequest).build());
}
/**
*
* Create a list of the Outposts for your AWS account. Add filters to your request to return a more specific list of
* results. Use filters to match an Outpost lifecycle status, Availibility Zone (us-east-1a
), and AZ ID
* (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* for (software.amazon.awssdk.services.outposts.model.ListOutpostsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)} operation.
*
*
* @param listOutpostsRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsIterable listOutpostsPaginator(ListOutpostsRequest listOutpostsRequest) throws ValidationException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Create a list of the Outposts for your AWS account. Add filters to your request to return a more specific list of
* results. Use filters to match an Outpost lifecycle status, Availibility Zone (us-east-1a
), and AZ ID
* (use1-az1
).
*
*
* If you specify multiple filters, the filters are joined with an AND
, and the request returns only
* results that match all of the specified filters.
*
*
*
* This is a variant of {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* for (software.amazon.awssdk.services.outposts.model.ListOutpostsResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListOutpostsIterable responses = client.listOutpostsPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listOutposts(software.amazon.awssdk.services.outposts.model.ListOutpostsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListOutpostsRequest.Builder} avoiding the need to
* create one manually via {@link ListOutpostsRequest#builder()}
*
*
* @param listOutpostsRequest
* A {@link Consumer} that will call methods on {@link ListOutpostsInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListOutposts
* @see AWS API
* Documentation
*/
default ListOutpostsIterable listOutpostsPaginator(Consumer listOutpostsRequest)
throws ValidationException, AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException,
OutpostsException {
return listOutpostsPaginator(ListOutpostsRequest.builder().applyMutation(listOutpostsRequest).build());
}
/**
*
* Lists the sites for the specified AWS account.
*
*
* @param listSitesRequest
* @return Result of the ListSites operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesResponse listSites(ListSitesRequest listSitesRequest) throws ValidationException, AccessDeniedException,
InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the sites for the specified AWS account.
*
*
*
* This is a convenience which creates an instance of the {@link ListSitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSitesRequest#builder()}
*
*
* @param listSitesRequest
* A {@link Consumer} that will call methods on {@link ListSitesInput.Builder} to create a request.
* @return Result of the ListSites operation returned by the service.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesResponse listSites(Consumer listSitesRequest) throws ValidationException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
return listSites(ListSitesRequest.builder().applyMutation(listSitesRequest).build());
}
/**
*
* Lists the sites for the specified AWS account.
*
*
*
* This is a variant of {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* for (software.amazon.awssdk.services.outposts.model.ListSitesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)} operation.
*
*
* @param listSitesRequest
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesIterable listSitesPaginator(ListSitesRequest listSitesRequest) throws ValidationException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the sites for the specified AWS account.
*
*
*
* This is a variant of {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)}
* operation. The return type is a custom iterable that can be used to iterate through all the pages. SDK will
* internally handle making service calls for you.
*
*
* When this operation is called, a custom iterable is returned but no service calls are made yet. So there is no
* guarantee that the request is valid. As you iterate through the iterable, SDK will start lazily loading response
* pages by making service calls until there are no pages left or your iteration stops. If there are errors in your
* request, you will see the failures only after you start iterating through the iterable.
*
*
*
* The following are few ways to iterate through the response pages:
*
* 1) Using a Stream
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* responses.stream().forEach(....);
* }
*
*
* 2) Using For loop
*
*
* {
* @code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* for (software.amazon.awssdk.services.outposts.model.ListSitesResponse response : responses) {
* // do something;
* }
* }
*
*
* 3) Use iterator directly
*
*
* {@code
* software.amazon.awssdk.services.outposts.paginators.ListSitesIterable responses = client.listSitesPaginator(request);
* responses.iterator().forEachRemaining(....);
* }
*
*
* Please notice that the configuration of MaxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSites(software.amazon.awssdk.services.outposts.model.ListSitesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListSitesRequest.Builder} avoiding the need to
* create one manually via {@link ListSitesRequest#builder()}
*
*
* @param listSitesRequest
* A {@link Consumer} that will call methods on {@link ListSitesInput.Builder} to create a request.
* @return A custom iterable that can be used to iterate through all the response pages.
* @throws ValidationException
* A parameter is not valid.
* @throws AccessDeniedException
* You do not have permission to perform this operation.
* @throws InternalServerException
* An internal error has occurred.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListSites
* @see AWS API
* Documentation
*/
default ListSitesIterable listSitesPaginator(Consumer listSitesRequest) throws ValidationException,
AccessDeniedException, InternalServerException, AwsServiceException, SdkClientException, OutpostsException {
return listSitesPaginator(ListSitesRequest.builder().applyMutation(listSitesRequest).build());
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws InternalServerException, ValidationException, NotFoundException, AwsServiceException, SdkClientException,
OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags for the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return Result of the ListTagsForResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default ListTagsForResourceResponse listTagsForResource(
Consumer listTagsForResourceRequest) throws InternalServerException,
ValidationException, NotFoundException, AwsServiceException, SdkClientException, OutpostsException {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Adds tags to the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws InternalServerException,
ValidationException, NotFoundException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return Result of the TagResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.TagResource
* @see AWS API
* Documentation
*/
default TagResourceResponse tagResource(Consumer tagResourceRequest)
throws InternalServerException, ValidationException, NotFoundException, AwsServiceException, SdkClientException,
OutpostsException {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws InternalServerException,
ValidationException, NotFoundException, AwsServiceException, SdkClientException, OutpostsException {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from the specified resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return Result of the UntagResource operation returned by the service.
* @throws InternalServerException
* An internal error has occurred.
* @throws ValidationException
* A parameter is not valid.
* @throws NotFoundException
* The specified request is not valid.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws OutpostsException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample OutpostsClient.UntagResource
* @see AWS API
* Documentation
*/
default UntagResourceResponse untagResource(Consumer untagResourceRequest)
throws InternalServerException, ValidationException, NotFoundException, AwsServiceException, SdkClientException,
OutpostsException {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
static ServiceMetadata serviceMetadata() {
return ServiceMetadata.of(SERVICE_METADATA_ID);
}
}