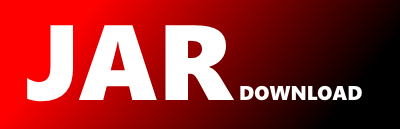
software.amazon.awssdk.services.outposts.DefaultOutpostsAsyncClient Maven / Gradle / Ivy
Show all versions of outposts Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.outposts;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.outposts.internal.OutpostsServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.outposts.model.AccessDeniedException;
import software.amazon.awssdk.services.outposts.model.CancelCapacityTaskRequest;
import software.amazon.awssdk.services.outposts.model.CancelCapacityTaskResponse;
import software.amazon.awssdk.services.outposts.model.CancelOrderRequest;
import software.amazon.awssdk.services.outposts.model.CancelOrderResponse;
import software.amazon.awssdk.services.outposts.model.ConflictException;
import software.amazon.awssdk.services.outposts.model.CreateOrderRequest;
import software.amazon.awssdk.services.outposts.model.CreateOrderResponse;
import software.amazon.awssdk.services.outposts.model.CreateOutpostRequest;
import software.amazon.awssdk.services.outposts.model.CreateOutpostResponse;
import software.amazon.awssdk.services.outposts.model.CreateSiteRequest;
import software.amazon.awssdk.services.outposts.model.CreateSiteResponse;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostRequest;
import software.amazon.awssdk.services.outposts.model.DeleteOutpostResponse;
import software.amazon.awssdk.services.outposts.model.DeleteSiteRequest;
import software.amazon.awssdk.services.outposts.model.DeleteSiteResponse;
import software.amazon.awssdk.services.outposts.model.GetCapacityTaskRequest;
import software.amazon.awssdk.services.outposts.model.GetCapacityTaskResponse;
import software.amazon.awssdk.services.outposts.model.GetCatalogItemRequest;
import software.amazon.awssdk.services.outposts.model.GetCatalogItemResponse;
import software.amazon.awssdk.services.outposts.model.GetConnectionRequest;
import software.amazon.awssdk.services.outposts.model.GetConnectionResponse;
import software.amazon.awssdk.services.outposts.model.GetOrderRequest;
import software.amazon.awssdk.services.outposts.model.GetOrderResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostInstanceTypesResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostResponse;
import software.amazon.awssdk.services.outposts.model.GetOutpostSupportedInstanceTypesRequest;
import software.amazon.awssdk.services.outposts.model.GetOutpostSupportedInstanceTypesResponse;
import software.amazon.awssdk.services.outposts.model.GetSiteAddressRequest;
import software.amazon.awssdk.services.outposts.model.GetSiteAddressResponse;
import software.amazon.awssdk.services.outposts.model.GetSiteRequest;
import software.amazon.awssdk.services.outposts.model.GetSiteResponse;
import software.amazon.awssdk.services.outposts.model.InternalServerException;
import software.amazon.awssdk.services.outposts.model.ListAssetsRequest;
import software.amazon.awssdk.services.outposts.model.ListAssetsResponse;
import software.amazon.awssdk.services.outposts.model.ListCapacityTasksRequest;
import software.amazon.awssdk.services.outposts.model.ListCapacityTasksResponse;
import software.amazon.awssdk.services.outposts.model.ListCatalogItemsRequest;
import software.amazon.awssdk.services.outposts.model.ListCatalogItemsResponse;
import software.amazon.awssdk.services.outposts.model.ListOrdersRequest;
import software.amazon.awssdk.services.outposts.model.ListOrdersResponse;
import software.amazon.awssdk.services.outposts.model.ListOutpostsRequest;
import software.amazon.awssdk.services.outposts.model.ListOutpostsResponse;
import software.amazon.awssdk.services.outposts.model.ListSitesRequest;
import software.amazon.awssdk.services.outposts.model.ListSitesResponse;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.outposts.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.outposts.model.NotFoundException;
import software.amazon.awssdk.services.outposts.model.OutpostsException;
import software.amazon.awssdk.services.outposts.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.outposts.model.StartCapacityTaskRequest;
import software.amazon.awssdk.services.outposts.model.StartCapacityTaskResponse;
import software.amazon.awssdk.services.outposts.model.StartConnectionRequest;
import software.amazon.awssdk.services.outposts.model.StartConnectionResponse;
import software.amazon.awssdk.services.outposts.model.TagResourceRequest;
import software.amazon.awssdk.services.outposts.model.TagResourceResponse;
import software.amazon.awssdk.services.outposts.model.UntagResourceRequest;
import software.amazon.awssdk.services.outposts.model.UntagResourceResponse;
import software.amazon.awssdk.services.outposts.model.UpdateOutpostRequest;
import software.amazon.awssdk.services.outposts.model.UpdateOutpostResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteAddressRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteAddressResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRackPhysicalPropertiesRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRackPhysicalPropertiesResponse;
import software.amazon.awssdk.services.outposts.model.UpdateSiteRequest;
import software.amazon.awssdk.services.outposts.model.UpdateSiteResponse;
import software.amazon.awssdk.services.outposts.model.ValidationException;
import software.amazon.awssdk.services.outposts.transform.CancelCapacityTaskRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.CancelOrderRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.CreateOrderRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.CreateOutpostRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.CreateSiteRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.DeleteOutpostRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.DeleteSiteRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetCapacityTaskRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetCatalogItemRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetConnectionRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetOrderRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetOutpostInstanceTypesRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetOutpostRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetOutpostSupportedInstanceTypesRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetSiteAddressRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.GetSiteRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListAssetsRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListCapacityTasksRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListCatalogItemsRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListOrdersRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListOutpostsRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListSitesRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.StartCapacityTaskRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.StartConnectionRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.UpdateOutpostRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.UpdateSiteAddressRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.UpdateSiteRackPhysicalPropertiesRequestMarshaller;
import software.amazon.awssdk.services.outposts.transform.UpdateSiteRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link OutpostsAsyncClient}.
*
* @see OutpostsAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultOutpostsAsyncClient implements OutpostsAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultOutpostsAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultOutpostsAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Cancels the capacity task.
*
*
* @param cancelCapacityTaskRequest
* @return A Java Future containing the result of the CancelCapacityTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CancelCapacityTask
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture cancelCapacityTask(CancelCapacityTaskRequest cancelCapacityTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelCapacityTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelCapacityTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelCapacityTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CancelCapacityTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelCapacityTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelCapacityTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelCapacityTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Cancels the specified order for an Outpost.
*
*
* @param cancelOrderRequest
* @return A Java Future containing the result of the CancelOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CancelOrder
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture cancelOrder(CancelOrderRequest cancelOrderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(cancelOrderRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, cancelOrderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CancelOrder");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CancelOrderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CancelOrder").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CancelOrderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(cancelOrderRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an order for an Outpost.
*
*
* @param createOrderRequest
* @return A Java Future containing the result of the CreateOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOrder
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createOrder(CreateOrderRequest createOrderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createOrderRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createOrderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateOrder");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateOrderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOrder").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateOrderRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createOrderRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates an Outpost.
*
*
* You can specify either an Availability one or an AZ ID.
*
*
* @param createOutpostRequest
* @return A Java Future containing the result of the CreateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateOutpost
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createOutpost(CreateOutpostRequest createOutpostRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createOutpostRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createOutpostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateOutpost");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateOutpostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateOutpost").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateOutpostRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createOutpostRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a site for an Outpost.
*
*
* @param createSiteRequest
* @return A Java Future containing the result of the CreateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - ServiceQuotaExceededException You have exceeded a service quota.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.CreateSite
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createSite(CreateSiteRequest createSiteRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createSiteRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSite");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateSiteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateSite")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateSiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createSiteRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified Outpost.
*
*
* @param deleteOutpostRequest
* @return A Java Future containing the result of the DeleteOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteOutpost
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteOutpost(DeleteOutpostRequest deleteOutpostRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteOutpostRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteOutpostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteOutpost");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteOutpostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteOutpost").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteOutpostRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteOutpostRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified site.
*
*
* @param deleteSiteRequest
* @return A Java Future containing the result of the DeleteSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.DeleteSite
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteSite(DeleteSiteRequest deleteSiteRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteSiteRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSite");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteSiteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteSite")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteSiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteSiteRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets details of the specified capacity task.
*
*
* @param getCapacityTaskRequest
* @return A Java Future containing the result of the GetCapacityTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetCapacityTask
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getCapacityTask(GetCapacityTaskRequest getCapacityTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCapacityTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCapacityTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCapacityTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCapacityTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCapacityTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCapacityTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCapacityTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about the specified catalog item.
*
*
* @param getCatalogItemRequest
* @return A Java Future containing the result of the GetCatalogItem operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetCatalogItem
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getCatalogItem(GetCatalogItemRequest getCatalogItemRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getCatalogItemRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCatalogItemRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCatalogItem");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetCatalogItemResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetCatalogItem").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetCatalogItemRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getCatalogItemRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Gets information about the specified connection.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param getConnectionRequest
* @return A Java Future containing the result of the GetConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have permission to perform this operation.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetConnection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getConnection(GetConnectionRequest getConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getConnectionRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about the specified order.
*
*
* @param getOrderRequest
* @return A Java Future containing the result of the GetOrder operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOrder
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getOrder(GetOrderRequest getOrderRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getOrderRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOrderRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOrder");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetOrderResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetOrder")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetOrderRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(getOrderRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about the specified Outpost.
*
*
* @param getOutpostRequest
* @return A Java Future containing the result of the GetOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpost
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getOutpost(GetOutpostRequest getOutpostRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getOutpostRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOutpostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOutpost");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetOutpostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetOutpost")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetOutpostRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getOutpostRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the instance types for the specified Outpost.
*
*
* @param getOutpostInstanceTypesRequest
* @return A Java Future containing the result of the GetOutpostInstanceTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpostInstanceTypes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getOutpostInstanceTypes(
GetOutpostInstanceTypesRequest getOutpostInstanceTypesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getOutpostInstanceTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getOutpostInstanceTypesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOutpostInstanceTypes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetOutpostInstanceTypesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetOutpostInstanceTypes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetOutpostInstanceTypesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getOutpostInstanceTypesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the instance types that an Outpost can support in InstanceTypeCapacity
. This will generally
* include instance types that are not currently configured and therefore cannot be launched with the current
* Outpost capacity configuration.
*
*
* @param getOutpostSupportedInstanceTypesRequest
* @return A Java Future containing the result of the GetOutpostSupportedInstanceTypes operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetOutpostSupportedInstanceTypes
* @see AWS API Documentation
*/
@Override
public CompletableFuture getOutpostSupportedInstanceTypes(
GetOutpostSupportedInstanceTypesRequest getOutpostSupportedInstanceTypesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getOutpostSupportedInstanceTypesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
getOutpostSupportedInstanceTypesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetOutpostSupportedInstanceTypes");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, GetOutpostSupportedInstanceTypesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetOutpostSupportedInstanceTypes").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetOutpostSupportedInstanceTypesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getOutpostSupportedInstanceTypesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about the specified Outpost site.
*
*
* @param getSiteRequest
* @return A Java Future containing the result of the GetSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSite
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSite(GetSiteRequest getSiteRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSiteRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSite");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSiteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetSite")
.withProtocolMetadata(protocolMetadata).withMarshaller(new GetSiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSiteRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the site address of the specified site.
*
*
* @param getSiteAddressRequest
* @return A Java Future containing the result of the GetSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.GetSiteAddress
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getSiteAddress(GetSiteAddressRequest getSiteAddressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getSiteAddressRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSiteAddressRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSiteAddress");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetSiteAddressResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetSiteAddress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetSiteAddressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getSiteAddressRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the hardware assets for the specified Outpost.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listAssetsRequest
* @return A Java Future containing the result of the ListAssets operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListAssets
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listAssets(ListAssetsRequest listAssetsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listAssetsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listAssetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListAssets");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListAssetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListAssets")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListAssetsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listAssetsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the capacity tasks for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCapacityTasksRequest
* @return A Java Future containing the result of the ListCapacityTasks operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCapacityTasks
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listCapacityTasks(ListCapacityTasksRequest listCapacityTasksRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCapacityTasksRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCapacityTasksRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCapacityTasks");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCapacityTasksResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCapacityTasks").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListCapacityTasksRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listCapacityTasksRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the items in the catalog.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listCatalogItemsRequest
* @return A Java Future containing the result of the ListCatalogItems operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListCatalogItems
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listCatalogItems(ListCatalogItemsRequest listCatalogItemsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listCatalogItemsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCatalogItemsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCatalogItems");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListCatalogItemsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListCatalogItems").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListCatalogItemsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listCatalogItemsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the Outpost orders for your Amazon Web Services account.
*
*
* @param listOrdersRequest
* @return A Java Future containing the result of the ListOrders operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOrders
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listOrders(ListOrdersRequest listOrdersRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listOrdersRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listOrdersRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOrders");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListOrdersResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListOrders")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListOrdersRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listOrdersRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the Outposts for your Amazon Web Services account.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listOutpostsRequest
* @return A Java Future containing the result of the ListOutposts operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListOutposts
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listOutposts(ListOutpostsRequest listOutpostsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listOutpostsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listOutpostsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListOutposts");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListOutpostsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListOutposts").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListOutpostsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listOutpostsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the Outpost sites for your Amazon Web Services account. Use filters to return specific results.
*
*
* Use filters to return specific results. If you specify multiple filters, the results include only the resources
* that match all of the specified filters. For a filter where you can specify multiple values, the results include
* items that match any of the values that you specify for the filter.
*
*
* @param listSitesRequest
* @return A Java Future containing the result of the ListSites operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListSites
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listSites(ListSitesRequest listSitesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listSitesRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSitesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSites");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListSitesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListSites")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListSitesRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(listSitesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Lists the tags for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Starts the specified capacity task. You can have one active capacity task for an order.
*
*
* @param startCapacityTaskRequest
* @return A Java Future containing the result of the StartCapacityTask operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.StartCapacityTask
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture startCapacityTask(StartCapacityTaskRequest startCapacityTaskRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startCapacityTaskRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startCapacityTaskRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartCapacityTask");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartCapacityTaskResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartCapacityTask").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartCapacityTaskRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startCapacityTaskRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
*
* Amazon Web Services uses this action to install Outpost servers.
*
*
*
* Starts the connection required for Outpost server installation.
*
*
* Use CloudTrail to monitor this action or Amazon Web Services managed policy for Amazon Web Services Outposts to
* secure it. For more information, see Amazon Web Services
* managed policies for Amazon Web Services Outposts and Logging Amazon Web
* Services Outposts API calls with Amazon Web Services CloudTrail in the Amazon Web Services Outposts User
* Guide.
*
*
* @param startConnectionRequest
* @return A Java Future containing the result of the StartConnection operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException You do not have permission to perform this operation.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.StartConnection
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture startConnection(StartConnectionRequest startConnectionRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(startConnectionRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, startConnectionRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "StartConnection");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, StartConnectionResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("StartConnection").withProtocolMetadata(protocolMetadata)
.withMarshaller(new StartConnectionRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(startConnectionRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds tags to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes tags from the specified resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException An internal error has occurred.
* - ValidationException A parameter is not valid.
* - NotFoundException The specified request is not valid.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates an Outpost.
*
*
* @param updateOutpostRequest
* @return A Java Future containing the result of the UpdateOutpost operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateOutpost
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateOutpost(UpdateOutpostRequest updateOutpostRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateOutpostRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateOutpostRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateOutpost");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateOutpostResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateOutpost").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateOutpostRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateOutpostRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the specified site.
*
*
* @param updateSiteRequest
* @return A Java Future containing the result of the UpdateSite operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSite
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateSite(UpdateSiteRequest updateSiteRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateSiteRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSiteRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSite");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateSiteResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("UpdateSite")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateSiteRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateSiteRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the address of the specified site.
*
*
* You can't update a site address if there is an order in progress. You must wait for the order to complete or
* cancel the order.
*
*
* You can update the operating address before you place an order at the site, or after all Outposts that belong to
* the site have been deactivated.
*
*
* @param updateSiteAddressRequest
* @return A Java Future containing the result of the UpdateSiteAddress operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - NotFoundException The specified request is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteAddress
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture updateSiteAddress(UpdateSiteAddressRequest updateSiteAddressRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateSiteAddressRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSiteAddressRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSiteAddress");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateSiteAddressResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateSiteAddress").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateSiteAddressRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateSiteAddressRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Update the physical and logistical details for a rack at a site. For more information about hardware requirements
* for racks, see Network
* readiness checklist in the Amazon Web Services Outposts User Guide.
*
*
* To update a rack at a site with an order of IN_PROGRESS
, you must wait for the order to complete or
* cancel the order.
*
*
* @param updateSiteRackPhysicalPropertiesRequest
* @return A Java Future containing the result of the UpdateSiteRackPhysicalProperties operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException A parameter is not valid.
* - ConflictException Updating or deleting this resource can cause an inconsistent state.
* - NotFoundException The specified request is not valid.
* - AccessDeniedException You do not have permission to perform this operation.
* - InternalServerException An internal error has occurred.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - OutpostsException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample OutpostsAsyncClient.UpdateSiteRackPhysicalProperties
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateSiteRackPhysicalProperties(
UpdateSiteRackPhysicalPropertiesRequest updateSiteRackPhysicalPropertiesRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateSiteRackPhysicalPropertiesRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
updateSiteRackPhysicalPropertiesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "Outposts");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSiteRackPhysicalProperties");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, UpdateSiteRackPhysicalPropertiesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateSiteRackPhysicalProperties").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateSiteRackPhysicalPropertiesRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateSiteRackPhysicalPropertiesRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final OutpostsServiceClientConfiguration serviceClientConfiguration() {
return new OutpostsServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(OutpostsException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("NotFoundException")
.exceptionBuilderSupplier(NotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
OutpostsServiceClientConfigurationBuilder serviceConfigBuilder = new OutpostsServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
@Override
public void close() {
clientHandler.close();
}
}